
Rtos API example
Embed:
(wiki syntax)
Show/hide line numbers
NetworkInterface.cpp
00001 /* Socket 00002 * Copyright (c) 2015 ARM Limited 00003 * 00004 * Licensed under the Apache License, Version 2.0 (the "License"); 00005 * you may not use this file except in compliance with the License. 00006 * You may obtain a copy of the License at 00007 * 00008 * http://www.apache.org/licenses/LICENSE-2.0 00009 * 00010 * Unless required by applicable law or agreed to in writing, software 00011 * distributed under the License is distributed on an "AS IS" BASIS, 00012 * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 00013 * See the License for the specific language governing permissions and 00014 * limitations under the License. 00015 */ 00016 00017 #include "netsocket/NetworkInterface.h" 00018 #include "netsocket/NetworkStack.h" 00019 #include <string.h> 00020 00021 00022 // Default network-interface state 00023 const char *NetworkInterface::get_mac_address() 00024 { 00025 return 0; 00026 } 00027 00028 const char *NetworkInterface::get_ip_address() 00029 { 00030 return 0; 00031 } 00032 00033 const char *NetworkInterface::get_netmask() 00034 { 00035 return 0; 00036 } 00037 00038 const char *NetworkInterface::get_gateway() 00039 { 00040 return 0; 00041 } 00042 00043 nsapi_error_t NetworkInterface::set_network(const char *ip_address, const char *netmask, const char *gateway) 00044 { 00045 return NSAPI_ERROR_UNSUPPORTED ; 00046 } 00047 00048 nsapi_error_t NetworkInterface::set_dhcp(bool dhcp) 00049 { 00050 if (!dhcp) { 00051 return NSAPI_ERROR_UNSUPPORTED ; 00052 } else { 00053 return NSAPI_ERROR_OK ; 00054 } 00055 } 00056 00057 // DNS operations go through the underlying stack by default 00058 nsapi_error_t NetworkInterface::gethostbyname(const char *name, SocketAddress *address, nsapi_version_t version) 00059 { 00060 return get_stack()->gethostbyname(name, address, version); 00061 } 00062 00063 nsapi_error_t NetworkInterface::add_dns_server(const SocketAddress &address) 00064 { 00065 return get_stack()->add_dns_server(address); 00066 } 00067
Generated on Sun Jul 17 2022 08:25:28 by
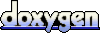