Library for Maxim DS3232M super-accurate, I2C based Real Time Clock chip with 234 bytes of user RAM. Library includes user RAM read/write operations along with CRC routines for accessing the user RAM area.
Dependents: ds3232m_HelloWorld
ds3232m Class Reference
Class ds3232m implements the real time clock. More...
#include <ds3232m.h>
Data Structures | |
struct | Time_rtc |
Structure which is used to exchange the time and date. More... | |
Public Member Functions | |
ds3232m (PinName sda, PinName scl) | |
Constructor with default i2c clock speed
| |
ds3232m (PinName sda, PinName scl, int i2cFrequency) | |
Constructor with i2c clock setting option
| |
~ds3232m () | |
Destructor. | |
bool | checkTempBusy (Time_rtc &dsSTR) |
See if temperature conversion is busy or not. | |
bool | startTempCycle (Time_rtc &dsSTR) |
Start a temperature conversion cycle
| |
float | getTemperature (Time_rtc &dsSTR) |
Get the chip temperature from the DS3232M. | |
uint8_t | getSeconds (Time_rtc &dsSTR) |
Get seconds register from DS3232M. | |
uint8_t | getDayOfWeek (Time_rtc &dsSTR) |
Get day-of-the-week register from DS3232M. | |
void | putDayOfWeek (Time_rtc &dsSTR, uint8_t dow3232) |
Set the day-of-the-week register in the DS3232M. | |
void | clearRAM (Time_rtc &dsSTR) |
Clear out all 236 bytes of user RAM, from address 0x14 to 0xff
| |
uint8_t | getUserRAM (char *buffer, Time_rtc &dsSTR, int offset, int length) |
Retrieve data from DS3232M's user RAM area
| |
uint8_t | putUserRAM (char *buffer, Time_rtc &dsSTR, int offset, int length) |
Store data DS3232M's user RAM area
| |
uint16_t | calculateCRC16 (char input[], Time_rtc &dsSTR, int offset, int length) |
Calculate CRC16. | |
void | set32KhzOutput (Time_rtc &dsSTR, bool ena, bool batt) |
Turn on/off the 32KHz output pin
| |
void | set1hzOutput (Time_rtc &dsSTR, bool ena, bool batt) |
Turn on/off the 1Hz output pin
| |
void | enableBattClock (Time_rtc &dsSTR, bool batt) |
Turn on/off the main oscillator during battery backup mode
| |
void | getTime (Time_rtc &dsSTR) |
Read all of the current time registers from the DS3232M. | |
void | setTime (Time_rtc &dsSTR) |
Write all of the current time registers into the DS3232M. | |
bool | LoadRTCRam (Time_rtc &dsSTR) |
Load the entire contents of the DS3232M into dedicated buffer RTCbuffer[]
|
Detailed Description
Class ds3232m implements the real time clock.
Definition at line 23 of file ds3232m.h.
Constructor & Destructor Documentation
ds3232m | ( | PinName | sda, |
PinName | scl | ||
) |
Constructor with default i2c clock speed
- Fixed at I2C address 0x80
- I2C speed set to 400KHz.
- Parameters:
-
sda - mbed I2C interface pin scl - mbed I2C interface pin
Definition at line 37 of file ds3232m.cpp.
ds3232m | ( | PinName | sda, |
PinName | scl, | ||
int | i2cFrequency | ||
) |
Constructor with i2c clock setting option
- Fixed at I2C address 0x80
- I2C speed set by user.
- Parameters:
-
sda - mbed I2C interface pin scl - mbed I2C interface pin set_I2C_frequency
Definition at line 47 of file ds3232m.cpp.
~ds3232m | ( | ) |
Member Function Documentation
uint16_t calculateCRC16 | ( | char | input[], |
Time_rtc & | dsSTR, | ||
int | offset, | ||
int | length | ||
) |
Calculate CRC16.
- Parameters:
-
pointer to I2C data buffer pointer to Time_rtc DS3232 data structure int offset into buffer int length number of bytes to calculate
- Returns:
- uint16_t CRC16 value
Definition at line 328 of file ds3232m.cpp.
bool checkTempBusy | ( | Time_rtc & | dsSTR ) |
See if temperature conversion is busy or not.
- Parameters:
-
pointer to Time_rtc DS3232 data structure
- Returns:
- busy_flag - Temperature Conversion is busy
- true = busy
- false = ready
Definition at line 122 of file ds3232m.cpp.
void clearRAM | ( | Time_rtc & | dsSTR ) |
Clear out all 236 bytes of user RAM, from address 0x14 to 0xff
- 00h is put in all user RAM area.
- Parameters:
-
pointer to Time_rtc DS3232 data structure
- Returns:
- --none--
Definition at line 205 of file ds3232m.cpp.
void enableBattClock | ( | Time_rtc & | dsSTR, |
bool | batt | ||
) |
Turn on/off the main oscillator during battery backup mode
- The oscillator always runs when Vcc is valid.
- Parameters:
-
pointer to Time_rtc DS3232 data structure batt - true = enable the oscillator during battery backup mode
- false = disable the oscillator during battery backup mode
- Returns:
- --none--
Definition at line 105 of file ds3232m.cpp.
uint8_t getDayOfWeek | ( | Time_rtc & | dsSTR ) |
Get day-of-the-week register from DS3232M.
- Parameters:
-
pointer to Time_rtc DS3232 data structure
- Returns:
- BCD-to-decimal corrected day of week register (1-7)
Definition at line 184 of file ds3232m.cpp.
uint8_t getSeconds | ( | Time_rtc & | dsSTR ) |
Get seconds register from DS3232M.
- Parameters:
-
pointer to Time_rtc DS3232 data structure
- Returns:
- BCD-to-decimal corrected seconds register
Definition at line 172 of file ds3232m.cpp.
float getTemperature | ( | Time_rtc & | dsSTR ) |
Get the chip temperature from the DS3232M.
- Parameters:
-
pointer to Time_rtc DS3232 data structure
- Returns:
- Temperature 0.25degC accuracy
- 255.0 if temperature conversion not complete (still busy)
Definition at line 148 of file ds3232m.cpp.
void getTime | ( | Time_rtc & | dsSTR ) |
Read all of the current time registers from the DS3232M.
- Parameters:
-
pointer to Time_rtc DS3232 data structure
- Returns:
- --none--
Definition at line 77 of file ds3232m.cpp.
uint8_t getUserRAM | ( | char * | buffer, |
Time_rtc & | dsSTR, | ||
int | offset, | ||
int | length | ||
) |
Retrieve data from DS3232M's user RAM area
- addresses range 0x14 - 0xfa
- CRC is checked of entire RAM contents.
- Parameters:
-
pointer to I2C data buffer pointer to Time_rtc DS3232 data structure int offset into DS3232M's RAM (range 0x14 = 0xfa) int length number of bytes to get
- Returns:
- 00 = no error
- 01 = length + offset is over the highest user RAM location
- 02 = offset < 0x14
- 04 = length = 0
- 08 = crc error occured from LoadRTCRam()
Definition at line 214 of file ds3232m.cpp.
bool LoadRTCRam | ( | Time_rtc & | dsSTR ) |
Load the entire contents of the DS3232M into dedicated buffer RTCbuffer[]
- includes time registers, alarm registers and user RAM.
- Parameters:
-
pointer to Time_rtc DS3232 data structure
- Returns:
- CRC_Status - pass/fail of CRC readback of user RAM data
- true = CRC is ok
- false = CRC failed
Definition at line 342 of file ds3232m.cpp.
void putDayOfWeek | ( | Time_rtc & | dsSTR, |
uint8_t | dow3232 | ||
) |
Set the day-of-the-week register in the DS3232M.
- Parameters:
-
pointer to Time_rtc DS3232 data structure uint8_t dow3232 day_of_the_week (1=Mon....7=Sun)
- Returns:
- --none--
Definition at line 195 of file ds3232m.cpp.
uint8_t putUserRAM | ( | char * | buffer, |
Time_rtc & | dsSTR, | ||
int | offset, | ||
int | length | ||
) |
Store data DS3232M's user RAM area
- addresses range 0x14 - 0xfa
- CRC is added to the last 2 locations using addCRC16()
- Parameters:
-
pointer to I2C data buffer pointer to Time_rtc DS3232 data structure int offset into DS3232M's RAM (range 0x14 = 0xfa) int length number of bytes to store
- Returns:
- 00 = no error
- 01 = length + offset is over the highest user RAM location
- 02 = offset < 0x14
- 04 = length = 0
Definition at line 228 of file ds3232m.cpp.
void set1hzOutput | ( | Time_rtc & | dsSTR, |
bool | ena, | ||
bool | batt | ||
) |
Turn on/off the 1Hz output pin
- with option to keep 1Hz output ON during battery backup
- This pin is open-drain
- Requires a pullup resistor to Vcc or the backup battery.
- Parameters:
-
pointer to Time_rtc DS3232 data structure ena - true = enable 1Hz output pin during normal operation
- false = disable 1Hz output pin during normal operation
batt - true = enable 1Hz output pin during battery backup mode
- false = disable 1Hz output pin during battery backup mode
- Note: "ena" must be true or else batt is ignored
- Returns:
- --none--
Definition at line 266 of file ds3232m.cpp.
void set32KhzOutput | ( | Time_rtc & | dsSTR, |
bool | ena, | ||
bool | batt | ||
) |
Turn on/off the 32KHz output pin
- with option to keep 32KHz output ON during battery backup
- This pin is push-pull, no pullup is required.
- Parameters:
-
pointer to Time_rtc DS3232 data structure bool ena - true = enable during normal operation
- false = disable 32KHz output pin during normal operation
bool batt - true = enable 32KHz output pin during battery backup mode
- false = disable 32KHz output pin during battery backup mode
- Note: "ena" must be true or else batt is ignored
- Returns:
- --none--
Definition at line 244 of file ds3232m.cpp.
void setTime | ( | Time_rtc & | dsSTR ) |
Write all of the current time registers into the DS3232M.
- Parameters:
-
pointer to Time_rtc DS3232 data structure
- Returns:
- --none--
Definition at line 61 of file ds3232m.cpp.
bool startTempCycle | ( | Time_rtc & | dsSTR ) |
Start a temperature conversion cycle
- Note: only 1 conversion per second allowed.
- Parameters:
-
pointer to Time_rtc DS3232 data structure
- Returns:
- busy_flag Temperature Conversion is Busy
- true = started new conversion
- false = already busy from previous converstion start
Definition at line 133 of file ds3232m.cpp.
Generated on Mon Jul 18 2022 17:28:07 by
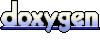