Library for Maxim DS3232M super-accurate, I2C based Real Time Clock chip with 234 bytes of user RAM. Library includes user RAM read/write operations along with CRC routines for accessing the user RAM area.
Dependents: ds3232m_HelloWorld
ds3232m.h
00001 #ifndef DS3232M_H 00002 #define DS3232M_H 00003 00004 /* 00005 Maxim Integrated DS3232M, fully integrated Real Time Clock chip. The 00006 chip contains an I2C interface, compatable time-base registers to 00007 the DS1307 and M11T41, a super-accurate internal oscillator, 2 alarms 00008 and 236 bytes of user defined battery-backed RAM. 00009 */ 00010 00011 //possible error returns from getUserRAM() 00012 #define DS3232_NOERROR 0x00 //ok, no error 00013 #define DS3232_OVERFLOWERROR 0x01 //length + offset is over the highest user RAM location 00014 #define DS3232_OFFSETERROR 0x02 //offset < 0x14, those registers are not in the user RAM area 00015 #define DS3232_LENZEROERROR 0x04 //length = 0 00016 #define DS3232_CRCERROR 0x08 //crc error 00017 00018 /** Class ds3232m implements the real time clock 00019 * 00020 * 00021 */ 00022 00023 class ds3232m { 00024 00025 public: 00026 /** Structure which is used to exchange the time and date 00027 */ 00028 typedef struct { 00029 int sec ; /*!< seconds [0..59] */ 00030 int min ; /*!< minutes {0..59] */ 00031 int hour ; /*!< hours [0..23] */ 00032 int wday ; /*!< weekday [1..7, where 1 = sunday, 2 = monday, ... */ 00033 int date ; /*!< day of month [0..31] */ 00034 int mon ; /*!< month of year [1..12] */ 00035 int year ; /*!< year [2000..2255] */ 00036 uint16_t s_crc ; /*!< stored crc value in RTC RAM */ 00037 uint16_t c_crc ; /*!< calculated crc value */ 00038 char RTCbuffer[256]; /*!< ds3232 ram buffer */ 00039 } Time_rtc; 00040 00041 protected: 00042 I2C* _i2c_; 00043 00044 // static const char *m_weekDays[]; 00045 00046 public: 00047 /** 00048 * Constructor with default i2c clock speed 00049 * - Fixed at I2C address 0x80 00050 * - I2C speed set to 400KHz 00051 * 00052 * @param sda - mbed I2C interface pin 00053 * @param scl - mbed I2C interface pin 00054 */ 00055 ds3232m(PinName sda, PinName scl); 00056 00057 /** 00058 * Constructor with i2c clock setting option 00059 * - Fixed at I2C address 0x80 00060 * - I2C speed set by user 00061 * 00062 * @param sda - mbed I2C interface pin 00063 * @param scl - mbed I2C interface pin 00064 * @param set_I2C_frequency 00065 */ 00066 ds3232m(PinName sda, PinName scl, int i2cFrequency); 00067 00068 /** 00069 * Destructor 00070 * 00071 * @param --none-- 00072 */ 00073 ~ds3232m(); 00074 00075 /** 00076 * See if temperature conversion is busy or not 00077 * 00078 * @param pointer to Time_rtc DS3232 data structure 00079 * 00080 * @return busy_flag - Temperature Conversion is busy 00081 * - true = busy 00082 * - false = ready 00083 */ 00084 bool checkTempBusy(Time_rtc& dsSTR); 00085 00086 /** 00087 * Start a temperature conversion cycle 00088 * - Note: only 1 conversion per second allowed 00089 * 00090 * @param pointer to Time_rtc DS3232 data structure 00091 * 00092 * @return busy_flag Temperature Conversion is Busy 00093 * - true = started new conversion 00094 * - false = already busy from previous converstion start 00095 */ 00096 bool startTempCycle(Time_rtc& dsSTR); 00097 00098 /** 00099 * Get the chip temperature from the DS3232M 00100 * 00101 * @param pointer to Time_rtc DS3232 data structure 00102 * 00103 * @return Temperature 0.25degC accuracy 00104 * @return 255.0 if temperature conversion not complete (still busy) 00105 */ 00106 float getTemperature(Time_rtc& dsSTR); 00107 00108 /** 00109 * Get seconds register from DS3232M 00110 * 00111 * @param pointer to Time_rtc DS3232 data structure 00112 * 00113 * @return BCD-to-decimal corrected seconds register 00114 */ 00115 uint8_t getSeconds(Time_rtc& dsSTR); 00116 00117 /** 00118 * Get day-of-the-week register from DS3232M 00119 * 00120 * @param pointer to Time_rtc DS3232 data structure 00121 * 00122 * @return BCD-to-decimal corrected day of week register (1-7) 00123 */ 00124 uint8_t getDayOfWeek(Time_rtc& dsSTR); 00125 00126 /** 00127 * Set the day-of-the-week register in the DS3232M 00128 * 00129 * @param pointer to Time_rtc DS3232 data structure 00130 * @param uint8_t dow3232 day_of_the_week (1=Mon....7=Sun) 00131 * 00132 * @return --none-- 00133 */ 00134 void putDayOfWeek(Time_rtc& dsSTR, uint8_t dow3232); 00135 00136 /** 00137 * Clear out all 236 bytes of user RAM, from address 0x14 to 0xff 00138 * - 00h is put in all user RAM area 00139 * 00140 * @param pointer to Time_rtc DS3232 data structure 00141 * 00142 * @return --none-- 00143 */ 00144 void clearRAM(Time_rtc& dsSTR); 00145 00146 /** 00147 * Retrieve data from DS3232M's user RAM area 00148 * - addresses range 0x14 - 0xfa 00149 * - CRC is checked of entire RAM contents 00150 * 00151 * @param pointer to I2C data buffer 00152 * @param pointer to Time_rtc DS3232 data structure 00153 * @param int offset into DS3232M's RAM (range 0x14 = 0xfa) 00154 * @param int length number of bytes to get 00155 * 00156 * @return 00 = no error 00157 * @return 01 = length + offset is over the highest user RAM location 00158 * @return 02 = offset < 0x14 00159 * @return 04 = length = 0 00160 * @return 08 = crc error occured from LoadRTCRam() 00161 */ 00162 uint8_t getUserRAM(char *buffer, Time_rtc& dsSTR, int offset, int length); 00163 00164 /** 00165 * Store data DS3232M's user RAM area 00166 * - addresses range 0x14 - 0xfa 00167 * - CRC is added to the last 2 locations using addCRC16() 00168 * 00169 * @param pointer to I2C data buffer 00170 * @param pointer to Time_rtc DS3232 data structure 00171 * @param int offset into DS3232M's RAM (range 0x14 = 0xfa) 00172 * @param int length number of bytes to store 00173 * 00174 * @return 00 = no error 00175 * @return 01 = length + offset is over the highest user RAM location 00176 * @return 02 = offset < 0x14 00177 * @return 04 = length = 0 00178 */ 00179 uint8_t putUserRAM(char *buffer, Time_rtc& dsSTR, int offset, int length); 00180 00181 /** 00182 * Calculate CRC16 00183 * 00184 * @param pointer to I2C data buffer 00185 * @param pointer to Time_rtc DS3232 data structure 00186 * @param int offset into buffer 00187 * @param int length number of bytes to calculate 00188 * 00189 * @return uint16_t CRC16 value 00190 */ 00191 uint16_t calculateCRC16(char input[], Time_rtc& dsSTR, int offset, int length); 00192 00193 /** 00194 * Turn on/off the 32KHz output pin 00195 * - with option to keep 32KHz output ON during battery backup 00196 * - This pin is push-pull, no pullup is required 00197 * 00198 * @param pointer to Time_rtc DS3232 data structure 00199 * @param bool ena 00200 * - true = enable during normal operation 00201 * - false = disable 32KHz output pin during normal operation 00202 * @param bool batt 00203 * - true = enable 32KHz output pin during battery backup mode 00204 * - false = disable 32KHz output pin during battery backup mode 00205 * - Note: "ena" must be true or else batt is ignored 00206 * 00207 * @return --none-- 00208 */ 00209 void set32KhzOutput(Time_rtc& dsSTR, bool ena, bool batt); 00210 00211 /** 00212 * Turn on/off the 1Hz output pin 00213 * - with option to keep 1Hz output ON during battery backup 00214 * - This pin is open-drain 00215 * - Requires a pullup resistor to Vcc or the backup battery 00216 * 00217 * @param pointer to Time_rtc DS3232 data structure 00218 * @param ena 00219 * - true = enable 1Hz output pin during normal operation 00220 * - false = disable 1Hz output pin during normal operation 00221 * @param batt 00222 * - true = enable 1Hz output pin during battery backup mode 00223 * - false = disable 1Hz output pin during battery backup mode 00224 * - Note: "ena" must be true or else batt is ignored 00225 * 00226 * @return --none-- 00227 */ 00228 void set1hzOutput(Time_rtc& dsSTR, bool ena, bool batt); 00229 00230 /** 00231 * Turn on/off the main oscillator during battery backup mode 00232 * - The oscillator always runs when Vcc is valid 00233 * 00234 * @param pointer to Time_rtc DS3232 data structure 00235 * @param batt 00236 * - true = enable the oscillator during battery backup mode 00237 * - false = disable the oscillator during battery backup mode 00238 * 00239 * @return --none-- 00240 */ 00241 void enableBattClock(Time_rtc& dsSTR, bool batt); 00242 00243 /** 00244 * Read all of the current time registers from the DS3232M 00245 * 00246 * @param pointer to Time_rtc DS3232 data structure 00247 * 00248 * @returns --none-- 00249 */ 00250 void getTime(Time_rtc& dsSTR); 00251 00252 /** 00253 * Write all of the current time registers into the DS3232M 00254 * 00255 * @param pointer to Time_rtc DS3232 data structure 00256 * 00257 * @returns --none-- 00258 */ 00259 void setTime(Time_rtc& dsSTR); 00260 00261 /** 00262 * Load the entire contents of the DS3232M into dedicated buffer RTCbuffer[] 00263 * - includes time registers, alarm registers and user RAM 00264 * 00265 * @param pointer to Time_rtc DS3232 data structure 00266 * 00267 * @return CRC_Status - pass/fail of CRC readback of user RAM data 00268 * - true = CRC is ok 00269 * - false = CRC failed 00270 */ 00271 bool LoadRTCRam(Time_rtc& dsSTR); 00272 00273 private: 00274 void getControlStatusRegs(Time_rtc& dsSTR); 00275 char RtcCtlReg; 00276 char RtcStatReg; 00277 void addCRC16(Time_rtc& dsSTR); 00278 00279 // BCD-Decimal Conversions, hacked from Henry Leinen's RTC-DS1307 library 00280 static int BCDToDec(int dat) { 00281 return ((dat & 0xF0) >> 4) * 10 + (dat & 0x0F); 00282 } 00283 00284 static int DecToBCD(int dat) { 00285 return (dat % 10) + ((dat / 10) << 4); 00286 } 00287 }; 00288 00289 #endif // DS3232M_H
Generated on Mon Jul 18 2022 17:28:07 by
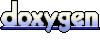