
New version of quadcopter software written to OO principles
Dependencies: mbed MODSERIAL filter mbed-rtos ConfigFile PID PPM FreeIMU_external_magnetometer TinyGPS
Status.h
00001 #include "mbed.h" 00002 #include "Global.h" 00003 #include "StatusLights.h" 00004 00005 #ifndef Status_H 00006 #define Status_H 00007 00008 class Status 00009 { 00010 public: 00011 Status(); 00012 ~Status(); 00013 00014 enum State 00015 { 00016 PREFLIGHT, 00017 STANDBY, 00018 GROUND_READY, 00019 MANUAL, 00020 STABILISED, 00021 AUTO, 00022 ERROR 00023 }; 00024 00025 enum FlightMode 00026 { 00027 RATE, 00028 STAB 00029 }; 00030 00031 enum NavigationMode 00032 { 00033 NONE, 00034 ALTITUDE_HOLD, 00035 POSITION_HOLD 00036 }; 00037 00038 enum BaseStationMode 00039 { 00040 MOTOR_POWER, 00041 PID_OUTPUTS, 00042 IMU_OUTPUTS, 00043 STATUS, 00044 RC, 00045 PID_TUNING, 00046 GPS, 00047 ZERO, 00048 RATE_TUNING, 00049 STAB_TUNING, 00050 ALTITUDE, 00051 VELOCITY, 00052 ALTITUDE_STATUS, 00053 LIDAR 00054 }; 00055 00056 bool update(); 00057 State getState(); 00058 bool setFlightMode(FlightMode flightMode); 00059 FlightMode getFlightMode(); 00060 bool setNavigationMode(NavigationMode navigationMode); 00061 NavigationMode getNavigationMode(); 00062 bool setBaseStationMode(BaseStationMode baseStationMode); 00063 BaseStationMode getBaseStationMode(); 00064 bool setBatteryLevel(double batteryLevel); 00065 double getBatteryLevel(); 00066 bool setArmed(bool armed); 00067 bool getArmed(); 00068 bool setInitialised(bool initialised); 00069 bool getInitialised(); 00070 bool setRcConnected(bool rcConnected); 00071 bool getRcConnected(); 00072 bool setMotorsSpinning(bool flying); 00073 bool getMotorsSpinning(); 00074 bool setDeadZone(bool flying); 00075 bool getDeadZone(); 00076 00077 private: 00078 State _state; 00079 FlightMode _flightMode; 00080 NavigationMode _navigationMode; 00081 BaseStationMode _baseStationMode; 00082 StatusLights _statusLights; 00083 bool setState(State state); 00084 void flash(); 00085 double _batteryLevel; 00086 bool _armed; 00087 bool _initialised; 00088 bool _rcConnected; 00089 bool _flying; 00090 bool _deadZone; 00091 }; 00092 00093 #endif
Generated on Fri Jul 15 2022 00:21:58 by
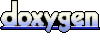