
New version of quadcopter software written to OO principles
Dependencies: mbed MODSERIAL filter mbed-rtos ConfigFile PID PPM FreeIMU_external_magnetometer TinyGPS
Imu.h
00001 #include "mbed.h" 00002 #include "Global.h" 00003 #include "FreeIMU.h" 00004 #include "filter.h" 00005 #include "ConfigFileWrapper.h" 00006 #include "Kalman.h" 00007 00008 #ifndef Imu_H 00009 #define Imu_H 00010 00011 class Imu 00012 { 00013 public: 00014 Imu(ConfigFileWrapper& configFileWrapper); 00015 ~Imu(); 00016 00017 struct Rate 00018 { 00019 double yaw; 00020 double pitch; 00021 double roll; 00022 }; 00023 00024 struct Angle 00025 { 00026 double yaw; 00027 double pitch; 00028 double roll; 00029 }; 00030 00031 struct Velocity 00032 { 00033 double x; 00034 double y; 00035 double z; 00036 }; 00037 00038 struct Acceleration 00039 { 00040 double x; 00041 double y; 00042 double z; 00043 }; 00044 00045 void enable(bool enable); 00046 00047 Rate getRate(); 00048 Angle getAngle(bool bias = true); 00049 Velocity getVelocity(float time); 00050 Velocity getVelocity(); 00051 Acceleration getAcceleration(); 00052 double getAltitude(); 00053 00054 void zeroGyro(); 00055 void zeroBarometer(); 00056 void zeroAccel(); 00057 void setCurrentVelocity(Velocity velocity); 00058 00059 private: 00060 FreeIMU _freeImu; 00061 filter* _barometerZeroFilter; 00062 filter* _barometerFilter; 00063 Rate _rate; 00064 Angle _angle; 00065 Velocity _velocity; 00066 float _barometerZero; 00067 ConfigFileWrapper& _configFileWrapper; 00068 float _accelZeroPitch; 00069 float _accelZeroRoll; 00070 Kalman* _kalmanXVelFilter; 00071 Kalman* _kalmanYVelFilter; 00072 Kalman* _kalmanZVelFilter; 00073 }; 00074 00075 #endif
Generated on Fri Jul 15 2022 00:21:58 by
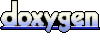