This module provides a simple API to the Maxim MAX7456 on-screen display chip
MAX7456 Class Reference
#include <MAX7456.h>
Public Types | |
enum | Registers |
MAX7456 register definitions. More... | |
enum | RowBrightnessReg |
MAX7456 Row brightness register definitions. More... | |
enum | Attributes { Inverse = (1 << 5), Blink = (1 << 6), LocalBG = (1 << 7) } |
Character attributes. More... | |
enum | BGbrightness { Percent_0 = (0 << 4), Percent_7 = (1 << 4), Percent_14 = (2 << 4), Percent_21 = (3 << 4), Percent_28 = (4 << 4), Percent_35 = (5 << 4), Percent_42 = (6 << 4), Percent_49 = (7 << 4) } |
Background brightness levels (see register VM1) More... | |
enum | BlinkTime { ms_33 = (0 << 2), ms_67 = (1 << 2), ms_100 = (2 << 2), ms_133 = (3 << 2) } |
Character blink time. More... | |
enum | BlinkingDutyCycle { BT_BT = (0 << 0), BT_BT2 = (1 << 0), BT_BT3 = (2 << 0), BT3_BT = (3 << 0) } |
Public Member Functions | |
MAX7456 (PinName mosi, PinName miso, PinName sclk, const char *name, PinName cs, PinName rst, PinName vsync) | |
MAX7456 constructor. | |
MAX7456 (PinName mosi, PinName miso, PinName sclk, PinName cs, PinName rst, PinName vsync) | |
MAX7456 constructor. | |
void | reset (void) |
reset() | |
void | clear_display (void) |
clear_display() | |
void | cursor (int x, int y) |
cursor(x,y) | |
void | convert_string (char *s) |
convert_string(*s) | |
int | string (char *s) |
string(*s) | |
int | stringxy (int x, int y, char *s) |
stringxy(x,y,s) | |
int | stringxy (int x, int y, char *s, uint8_t a) |
stringxy(x,y,s,a) | |
int | stringxy (int x, int y, char *s, char *a) |
stringxy(x,y,s,a) | |
void | stringl (int x, int y, char *s, int len) |
stringxy(x,y,s,len) | |
void | attributes_xyl (int x, int y, char *s, int len) |
attribute_xyl(x,y,s,len) | |
void | backGround (BGbrightness i) |
Set the background brightness. | |
void | blinkRate (BlinkTime bt, BlinkingDutyCycle dc) |
Set the character blink rate. | |
void | read_char_map (unsigned char address, unsigned char *data54) |
read_char_map(address,data54) | |
void | write_char_map (unsigned char address, const unsigned char *data54) |
write_char_map(address,data54) | |
template<typename T > | |
void | vsync_set_callback (T *tptr, void(T::*mptr)(void)) |
attach(tptr,mptr) | |
void | vsync_set_callback (void(*fptr)(void)) |
attach(tptr,mptr) |
Detailed Description
MAX7456 module.
- See also:
- http://mbed.org/cookbook/
- example1.cpp
Example:
#include "mbed.h" #include "MAX7456.h" DigitalOut led1(LED1); MAX7456 *max7456; int main() { max7456 = new MAX7456(p5, p6, p7, p8, p20, p15); max7456->stringxy(1, 1, "Hello World"); while(1) { led1 = 1; wait(0.5); led1 = 0; wait(0.5); } }
Definition at line 61 of file MAX7456.h.
Member Enumeration Documentation
enum Attributes |
enum BGbrightness |
enum BlinkingDutyCycle |
enum BlinkTime |
enum RowBrightnessReg |
Member Function Documentation
void convert_string | ( | char * | s ) |
convert_string(*s)
Takes a pointer to an ascii string and converts it to chars that will display via teh MAX7456 character set. Note, the string is converted.
- Parameters:
-
s A char * pointer to the NULL terminated string to convert.
Definition at line 181 of file MAX7456.cpp.
Generated on Wed Jul 13 2022 11:53:43 by
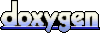