This module provides a simple API to the Maxim MAX7456 on-screen display chip
Embed:
(wiki syntax)
Show/hide line numbers
MAX7456.h
00001 /* 00002 Copyright (c) 2010 Andy Kirkham 00003 00004 Permission is hereby granted, free of charge, to any person obtaining a copy 00005 of this software and associated documentation files (the "Software"), to deal 00006 in the Software without restriction, including without limitation the rights 00007 to use, copy, modify, merge, publish, distribute, sublicense, and/or sell 00008 copies of the Software, and to permit persons to whom the Software is 00009 furnished to do so, subject to the following conditions: 00010 00011 The above copyright notice and this permission notice shall be included in 00012 all copies or substantial portions of the Software. 00013 00014 THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR 00015 IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY, 00016 FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE 00017 AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER 00018 LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM, 00019 OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN 00020 THE SOFTWARE. 00021 */ 00022 00023 #ifndef MODMAX7456_H 00024 #define MODMAX7456_H 00025 00026 #include "mbed.h" 00027 00028 /** @defgroup MAX7456_API */ 00029 /** @defgroup MAX7456_REGISTER_API */ 00030 00031 /** MAX7456 module 00032 * 00033 * @see http://mbed.org/cookbook/ 00034 * @see example1.cpp 00035 * 00036 * Example: 00037 * @code 00038 * #include "mbed.h" 00039 * #include "MAX7456.h" 00040 * 00041 * DigitalOut led1(LED1); 00042 * 00043 * MAX7456 *max7456; 00044 * 00045 * int main() { 00046 * 00047 * max7456 = new MAX7456(p5, p6, p7, p8, p20, p15); 00048 * 00049 * max7456->stringxy(1, 1, "Hello World"); 00050 * 00051 * while(1) { 00052 * led1 = 1; 00053 * wait(0.5); 00054 * led1 = 0; 00055 * wait(0.5); 00056 * } 00057 * } 00058 * @endcode 00059 */ 00060 00061 class MAX7456 00062 { 00063 public: 00064 00065 //! MAX7456 register definitions 00066 enum Registers { 00067 VM0 = 0, VM1, HOS, VOS, DMM, DMAH, DMAL, DMDI, CMM, CMAH, CMAL, CMDI, OSDM, 00068 OSDBL = 0x6C 00069 }; 00070 00071 //! MAX7456 Row brightness register definitions. 00072 enum RowBrightnessReg { 00073 RB0 = 16, RB1, RB2, RB3, RB4, RB5, RB6, RB7, RB8, RB9, RB10, RB11, RB12, RB13, RB14, RB15, 00074 }; 00075 00076 //! Character attributes. 00077 enum Attributes { 00078 //! Inverse background 00079 Inverse = (1 << 5), 00080 //! Character blinks 00081 Blink = (1 << 6), 00082 //! Use "local background" 00083 LocalBG = (1 << 7) 00084 }; 00085 00086 //! Background brightness levels (see register VM1) 00087 enum BGbrightness { 00088 //! 0% 00089 Percent_0 = (0 << 4), 00090 //! 7% 00091 Percent_7 = (1 << 4), 00092 //! 14% 00093 Percent_14 = (2 << 4), 00094 //! 21% 00095 Percent_21 = (3 << 4), 00096 //! 28% 00097 Percent_28 = (4 << 4), 00098 //! 35% 00099 Percent_35 = (5 << 4), 00100 //! 42% 00101 Percent_42 = (6 << 4), 00102 //! 49% 00103 Percent_49 = (7 << 4) 00104 }; 00105 00106 //! Character blink time. 00107 enum BlinkTime { 00108 //! 33milliseconds 00109 ms_33 = (0 << 2), 00110 //! 67milliseconds 00111 ms_67 = (1 << 2), 00112 //! 100milliseconds 00113 ms_100 = (2 << 2), 00114 //! 133milliseconds 00115 ms_133 = (3 << 2) 00116 }; 00117 00118 // Blink Time duty cycle. 00119 enum BlinkingDutyCycle { 00120 //! 1:1 duty cycle 00121 BT_BT = (0 << 0), 00122 //! 1:2 duty cycle 00123 BT_BT2 = (1 << 0), 00124 //! 1:3 duty cycle 00125 BT_BT3 = (2 << 0), 00126 //! 3:1 duty cycle 00127 BT3_BT = (3 << 0) 00128 }; 00129 00130 //! MAX7456 constructor. 00131 /** 00132 * The MAX7456 constructor is used to initialise the MAX7456 object. 00133 * 00134 * Example 1 00135 * @code 00136 * #include "mbed.h" 00137 * #include "MAX7456.h" 00138 * 00139 * MAX7456 max(p5, p6, p7, NULL, p8, p20, p15); 00140 * 00141 * int main() { 00142 * 00143 * max.stringxy(1, 1, "Hello World"); 00144 * 00145 * while(1); 00146 * } 00147 * @endcode 00148 * 00149 * Example 2 00150 * @code 00151 * #include "mbed.h" 00152 * #include "MAX7456.h" 00153 * 00154 * int main() { 00155 * 00156 * MAX7456 max = new MAX7456(p5, p6, p7, NULL, p8, p20, p15); 00157 * 00158 * max->stringxy(1, 1, "Hello World"); 00159 * 00160 * while(1); 00161 * } 00162 * @endcode 00163 * 00164 * @ingroup MAX7456_API 00165 * @param miso PinName p5 or p11 00166 * @param mosi PinName p6 or p12 00167 * @param sclk PinName p7 pr p13 00168 * @param name Optional const char * SSP object name 00169 * @param cs PinName CS signal 00170 * @param rst PinName RESET signal 00171 * @param vsync PinName Vertical sync signal 00172 */ 00173 MAX7456(PinName mosi, PinName miso, PinName sclk, const char *name, PinName cs, PinName rst, PinName vsync); 00174 00175 //! MAX7456 constructor. 00176 /** 00177 * The MAX7456 constructor is used to initialise the MAX7456 object. 00178 * 00179 * Example 1 00180 * @code 00181 * #include "mbed.h" 00182 * #include "MAX7456.h" 00183 * 00184 * MAX7456 max(p5, p6, p7, p8, p20, p15); 00185 * 00186 * int main() { 00187 * 00188 * max.stringxy(1, 1, "Hello World"); 00189 * 00190 * while(1); 00191 * } 00192 * @endcode 00193 * 00194 * Example 2 00195 * @code 00196 * #include "mbed.h" 00197 * #include "MAX7456.h" 00198 * 00199 * int main() { 00200 * 00201 * MAX7456 max = new MAX7456(p5, p6, p7, p8, p20, p15); 00202 * 00203 * max->stringxy(1, 1, "Hello World"); 00204 * 00205 * while(1); 00206 * } 00207 * @endcode 00208 * 00209 * @ingroup MAX7456_API 00210 * @param miso PinName p5 or p11 00211 * @param mosi PinName p6 or p12 00212 * @param sclk PinName p7 pr p13 00213 * @param cs PinName CS signal 00214 * @param rst PinName RESET signal 00215 * @param vsync PinName Vertical sync signal 00216 */ 00217 MAX7456(PinName mosi, PinName miso, PinName sclk, PinName cs, PinName rst, PinName vsync); 00218 00219 ~MAX7456(); 00220 00221 //! reset() 00222 /** 00223 * Resets the MAX7456 device. 00224 * 00225 * @ingroup MAX7456_API 00226 */ 00227 void reset(void); 00228 00229 //! clear_display() 00230 /** 00231 * Cleasr the entire display area. 00232 * 00233 * @ingroup MAX7456_API 00234 */ 00235 void clear_display(void); 00236 00237 //! cursor(x,y) 00238 /** 00239 * Moves the "cursor" to the screen X,Y position. Future writes to the 00240 * screen ram will occur at this position. 00241 * 00242 * @ingroup MAX7456_API 00243 * @param x The X position 00244 * @param y The Y position 00245 */ 00246 void cursor(int x, int y); 00247 00248 //! convert_string(*s) 00249 /** 00250 * Takes a pointer to an ascii string and converts it to chars that will 00251 * display via teh MAX7456 character set. Note, the string is converted. 00252 * 00253 * @param s A char * pointer to the NULL terminated string to convert. 00254 */ 00255 void convert_string(char *s); 00256 00257 //! string(*s) 00258 /** 00259 * Print the string pointed to by s at the current cursor position. 00260 * The string should be an ASCII NULL terminated string. 00261 * 00262 * @ingroup MAX7456_API 00263 * @param s A char * pointer to the NULL terminated string to print. 00264 */ 00265 int string(char *s); 00266 00267 //! stringxy(x,y,s) 00268 /** 00269 * Print the string pointed to by s at the position supplied by x,y. 00270 * The string should be an ASCII NULL terminated string. 00271 * 00272 * @ingroup MAX7456_API 00273 * @param x The X position 00274 * @param y The Y position 00275 * @param s A char * pointer to the NULL terminated string to print. 00276 * @return int The length of the string written. 00277 */ 00278 int stringxy(int x, int y, char *s); 00279 00280 //! stringxy(x,y,s,a) 00281 /** 00282 * Print the string pointed to by s at the position supplied by x,y. 00283 * The string should be an ASCII NULL terminated string. 00284 * 00285 * @ingroup MAX7456_API 00286 * @param x The X position 00287 * @param y The Y position 00288 * @param s A char * pointer to the NULL terminated string to print. 00289 * @param a An attribute byte to appply to the string. 00290 * @return int The length of the string written. 00291 */ 00292 int stringxy(int x, int y, char *s, uint8_t a); 00293 00294 //! stringxy(x,y,s,a) 00295 /** 00296 * Print the string pointed to by s at the position supplied by x,y. 00297 * The string should be an ASCII NULL terminated string. 00298 * 00299 * @ingroup MAX7456_API 00300 * @param x The X position 00301 * @param y The Y position 00302 * @param s A char * pointer to the NULL terminated string to print. 00303 * @param a A char * pointer to the 30byte attribute string. 00304 * @return int The length of the string written. 00305 */ 00306 int stringxy(int x, int y, char *s, char *a); 00307 00308 //! stringxy(x,y,s,len) 00309 /** 00310 * Print the string pointed to by s at the position supplied by x,y. 00311 * The string should be an ASCII terminated string. 00312 * len determines the length of the string to print (not the term NULL). 00313 * 00314 * @ingroup MAX7456_API 00315 * @param x The X position 00316 * @param y The Y position 00317 * @param s A char * pointer to the NULL terminated string to print. 00318 * @param len The length of teh string to print. 00319 */ 00320 void stringl(int x, int y, char *s, int len); 00321 00322 //! attribute_xyl(x,y,s,len) 00323 /** 00324 * Write the character attribute bytes at x,y 00325 * Since attributes can be zero (NULL), the string length 00326 * must be provided. 00327 * 00328 * @ingroup MAX7456_API 00329 * @param x The X position 00330 * @param y The Y position 00331 * @param s A char * pointer to the NULL terminated string of attributes. 00332 * @param len The length of the string of attributes. 00333 */ 00334 void attributes_xyl(int x, int y, char *s, int len); 00335 00336 /** 00337 * Set the background brightness 00338 * 00339 * @see BGbrightness 00340 * @ingroup MAX7456_API 00341 * @param i The brightness level 00342 */ 00343 void backGround(BGbrightness i) { vm1((vm1() & ~(7 << 4)) | i); } 00344 00345 /** 00346 * Set the character blink rate 00347 * 00348 * @see BlinkTime 00349 * @see BlinkingDutyCycle 00350 * @ingroup MAX7456_API 00351 * @param bt The blink time 00352 * @param dc The duty cycle 00353 */ 00354 void blinkRate(BlinkTime bt, BlinkingDutyCycle dc) { vm1((vm1() & ~(0xf)) | (bt | dc)); } 00355 00356 //! read_char_map(address,data54) 00357 /** 00358 * Read the 54byte character map from MAX7456 ROM. 00359 * 00360 * @ingroup MAX7456_API 00361 * @param address The uchar address to read from (which character) 00362 * @param data54 A char * pointer to a buffer where to write to. 00363 */ 00364 void read_char_map(unsigned char address, unsigned char *data54); 00365 00366 //! write_char_map(address,data54) 00367 /** 00368 * Write the 54byte character map to the MAX7456 ROM. 00369 * 00370 * @ingroup MAX7456_API 00371 * @param address The uchar address to write to 00372 * @param data54 A char * pointer to a buffer where to read from. 00373 */ 00374 void write_char_map(unsigned char address, const unsigned char *data54); 00375 00376 //! attach(tptr,mptr) 00377 /** 00378 * Attach a callback method to teh vertical sync interrupt. 00379 * 00380 * @ingroup MAX7456_API 00381 * @param tptr The class object pointer conatining the method. 00382 * @param mptr The method within the object to invoke. 00383 */ 00384 template<typename T> 00385 void vsync_set_callback(T* tptr, void (T::*mptr)(void)) { 00386 _vsync_callback.attach(tptr, mptr); 00387 } 00388 00389 //! attach(tptr,mptr) 00390 /** 00391 * Attach a callback function to the vertical sync interrupt. 00392 * 00393 * @ingroup MAX7456_API 00394 * @param fptr A function pointer to call on vertical sync irq. 00395 */ 00396 void vsync_set_callback(void (*fptr)(void)) { _vsync_callback.attach(fptr); } 00397 00398 // Must be reimplemented by the child class. 00399 virtual void vsync_isr(void) { _vsync_callback.call(); }; 00400 00401 // Register level API. 00402 00403 /** @ingroup MAX7456_REGISTER_API */ 00404 __INLINE void vm0(uint8_t i) { write(VM0, i); } 00405 /** @ingroup MAX7456_REGISTER_API */ 00406 __INLINE uint8_t vm0(void) { return (uint8_t)read(VM0); } 00407 /** @ingroup MAX7456_REGISTER_API */ 00408 __INLINE void vm1(uint8_t i) { write(VM1, i); } 00409 /** @ingroup MAX7456_REGISTER_API */ 00410 __INLINE uint8_t vm1(void) { return (uint8_t)read(VM1); } 00411 /** @ingroup MAX7456_REGISTER_API */ 00412 __INLINE void hos(uint8_t i) { write(HOS, i); } 00413 /** @ingroup MAX7456_REGISTER_API */ 00414 __INLINE uint8_t hos(void) { return (uint8_t)read(HOS); } 00415 /** @ingroup MAX7456_REGISTER_API */ 00416 __INLINE void vos(uint8_t i) { write(VOS, i); } 00417 /** @ingroup MAX7456_REGISTER_API */ 00418 __INLINE uint8_t vos(void) { return (uint8_t)read(VOS); } 00419 /** @ingroup MAX7456_REGISTER_API */ 00420 __INLINE void dmm(uint8_t i) { write(DMM, i); } 00421 /** @ingroup MAX7456_REGISTER_API */ 00422 __INLINE uint8_t dmm(void) { return (uint8_t)read(DMM); } 00423 /** @ingroup MAX7456_REGISTER_API */ 00424 __INLINE void dmah(uint8_t i) { write(DMAH, i); } 00425 /** @ingroup MAX7456_REGISTER_API */ 00426 __INLINE uint8_t dmah(void) { return (uint8_t)read(DMAH); } 00427 /** @ingroup MAX7456_REGISTER_API */ 00428 __INLINE void dmal(uint8_t i) { write(DMAL, i); } 00429 /** @ingroup MAX7456_REGISTER_API */ 00430 __INLINE uint8_t dmal(void) { return (uint8_t)read(DMAL); } 00431 /** @ingroup MAX7456_REGISTER_API */ 00432 __INLINE void dmdi(uint8_t i) { write(DMDI, i); } 00433 /** @ingroup MAX7456_REGISTER_API */ 00434 __INLINE uint8_t dmai(void) { return (uint8_t)read(DMDI); } 00435 /** @ingroup MAX7456_REGISTER_API */ 00436 __INLINE void cmm(uint8_t i) { write(CMM, i); } 00437 /** @ingroup MAX7456_REGISTER_API */ 00438 __INLINE uint8_t cmm(void) { return (uint8_t)read(CMM); } 00439 /** @ingroup MAX7456_REGISTER_API */ 00440 __INLINE void cmah(uint8_t i) { write(CMAH, i); } 00441 /** @ingroup MAX7456_REGISTER_API */ 00442 __INLINE uint8_t cmah(void) { return (uint8_t)read(CMAH); } 00443 /** @ingroup MAX7456_REGISTER_API */ 00444 __INLINE void cmal(uint8_t i) { write(CMAL, i); } 00445 /** @ingroup MAX7456_REGISTER_API */ 00446 __INLINE uint8_t cmal(void) { return (uint8_t)read(CMAL); } 00447 /** @ingroup MAX7456_REGISTER_API */ 00448 __INLINE void cmdi(uint8_t i) { write(CMDI, i); } 00449 /** @ingroup MAX7456_REGISTER_API */ 00450 __INLINE uint8_t cmai(void) { return (uint8_t)read(CMDI); } 00451 /** @ingroup MAX7456_REGISTER_API */ 00452 __INLINE void osdm(uint8_t i) { write(OSDM, i); } 00453 /** @ingroup MAX7456_REGISTER_API */ 00454 __INLINE uint8_t osdm(void) { return (uint8_t)read(OSDM); } 00455 /** @ingroup MAX7456_REGISTER_API */ 00456 00457 /** @ingroup MAX7456_REGISTER_API */ 00458 __INLINE uint8_t stat(void) { return read(0xA0); } 00459 00460 /** @ingroup MAX7456_REGISTER_API */ 00461 __INLINE bool los(void) { return (bool)!(stat() & (1 << 2)); } 00462 00463 /** @ingroup MAX7456_REGISTER_API */ 00464 __INLINE uint8_t osdbl(void) { return (uint8_t)read(OSDBL); } 00465 /** @ingroup MAX7456_REGISTER_API */ 00466 __INLINE void osdbl(bool b) { uint8_t t = osdbl(); if (b) osdbl(t & ~(1 << 4)); else osdbl(t | (1 << 4)); } 00467 00468 /** @ingroup MAX7456_REGISTER_API */ 00469 __INLINE void RowBrightness(RowBrightnessReg r, uint8_t i) { write(r, i); } 00470 /** @ingroup MAX7456_REGISTER_API */ 00471 __INLINE uint8_t RowBrightness(RowBrightnessReg r) { return read (r); } 00472 00473 protected: 00474 void init(PinName mosi, PinName miso, PinName sclk, const char *name, PinName cs, PinName rst, PinName vsync = NC); 00475 void write(unsigned char address, unsigned char byte); 00476 int read(unsigned char address); 00477 00478 SPI *_ssp; 00479 DigitalOut *_cs; 00480 DigitalOut *_rst; 00481 InterruptIn *_vsync; 00482 FunctionPointer _vsync_callback; 00483 }; 00484 00485 #endif
Generated on Wed Jul 13 2022 11:53:43 by
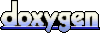