This module provides a simple API to the Maxim MAX7456 on-screen display chip
Embed:
(wiki syntax)
Show/hide line numbers
example1.cpp
00001 #ifdef COMPILE_EXAMPLE_CODE_MODOSD7456 00002 00003 #include "mbed.h" 00004 #include "OSD7456.h" 00005 00006 DigitalOut led1(LED1); 00007 00008 OSD7456 *osd; 00009 00010 int main() { 00011 00012 osd = new OSD7456(MAX7456_MOSI, MAX7456_MISO, MAX7456_SCLK, MAX7456_CS, MAX7456_RST, MAX7456_VSYNC); 00013 00014 // Set the character "local background" to 42% 00015 osd->max7456->backGround(MAX7456::Percent_42); 00016 00017 // Set the blink rate to 133ms with a duty cycle of 3:1 00018 osd->max7456->blinkRate(MAX7456::ms_133, MAX7456::BT3_BT); 00019 00020 osd->print(1, " Hello World"); 00021 osd->print(7, 4, "Positioned text"); 00022 osd->print(7, 6, "Positioned text", MAX7456::LocalBG); 00023 osd->print(3, 8, "Positioned text blinks", MAX7456::Blink | MAX7456::LocalBG); 00024 osd->print(4, 10, " Blinks and inverse ", MAX7456::Blink | MAX7456::LocalBG | MAX7456::Inverse); 00025 00026 while(1) { 00027 led1 = 1; 00028 wait(0.5); 00029 led1 = 0; 00030 wait(0.5); 00031 } 00032 } 00033 00034 #endif
Generated on Wed Jul 13 2022 11:53:43 by
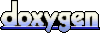