
My modifications/additions to the code
Dependencies: ADXL345 ADXL345_I2C IMUfilter ITG3200 Servo fishgait mbed-rtos mbed pixy_cam
Fork of robotic_fish_ver_4_8 by
PwmReader.cpp
00001 #include "PwmReader.h" 00002 00003 //Constructors 00004 PwmReader::PwmReader(PinName pwmInPort, float min, float max) 00005 { 00006 if (min > max) 00007 { 00008 error("PwmReader min value greater than max value!"); 00009 } 00010 00011 di = new InterruptIn(pwmInPort); 00012 pwmMin = min; 00013 pwmMax = max; 00014 lastRise = 0; 00015 period = 0; 00016 duty = 0.0; 00017 di->mode(PullDown); 00018 di->rise(this,&PwmReader::pwmRise); // attach the address of the flip function to the rising edge 00019 di->fall(this,&PwmReader::pwmFall); 00020 00021 t.start(); 00022 } 00023 00024 PwmReader::~PwmReader() 00025 { 00026 delete di; 00027 t.stop(); 00028 } 00029 00030 // public methods 00031 float PwmReader::getDuty() 00032 { 00033 float smallDelta = (duty > pwmMin) ? (duty - pwmMin) : 0.0; 00034 float dutyAdjusted = smallDelta / (pwmMax-pwmMin); 00035 return dutyAdjusted; 00036 } 00037 00038 // private methods 00039 void PwmReader::pwmRise() 00040 { 00041 int rise = t.read_us(); 00042 if( (lastRise > 0) && (rise > lastRise) ) { 00043 period = rise - lastRise; 00044 } 00045 lastRise = rise; 00046 00047 } 00048 00049 void PwmReader::pwmFall() 00050 { 00051 int fall = t.read_us(); 00052 if(period > 0 ) { 00053 int delta = fall - lastRise; 00054 duty = float(delta)/float(period); 00055 } 00056 }
Generated on Sun Jul 17 2022 22:28:23 by
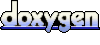