MAX77658 Ultra-Low Power PMIC Mbed Driver
Embed:
(wiki syntax)
Show/hide line numbers
MAX77658_regs.h
00001 /******************************************************************************* 00002 * Copyright(C) Analog Devices Inc., All Rights Reserved. 00003 * 00004 * Permission is hereby granted, free of charge, to any person obtaining a 00005 * copy of this software and associated documentation files(the "Software"), 00006 * to deal in the Software without restriction, including without limitation 00007 * the rights to use, copy, modify, merge, publish, distribute, sublicense, 00008 * and/or sell copies of the Software, and to permit persons to whom the 00009 * Software is furnished to do so, subject to the following conditions: 00010 * 00011 * The above copyright notice and this permission notice shall be included 00012 * in all copies or substantial portions of the Software. 00013 * 00014 * THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS 00015 * OR IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF 00016 * MERCHANTABILITY, FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. 00017 * IN NO EVENT SHALL MAXIM INTEGRATED BE LIABLE FOR ANY CLAIM, DAMAGES 00018 * OR OTHER LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, 00019 * ARISING FROM, OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR 00020 * OTHER DEALINGS IN THE SOFTWARE. 00021 * 00022 * Except as contained in this notice, the name of Analog Devices Inc. 00023 * shall not be used except as stated in the Analog Devices Inc. 00024 * Branding Policy. 00025 * 00026 * The mere transfer of this software does not imply any licenses 00027 * of trade secrets, proprietary technology, copyrights, patents, 00028 * trademarks, maskwork rights, or any other form of intellectual 00029 * property whatsoever. Analog Devices Inc.retains all ownership rights. 00030 ******************************************************************************* 00031 */ 00032 00033 #ifndef MAX77658_REGS_H_ 00034 #define MAX77658_REGS_H_ 00035 00036 /** 00037 * @brief INT_GLBL0 Register 00038 * 00039 * Address : 0x00 00040 */ 00041 typedef union { 00042 unsigned char raw; 00043 struct { 00044 unsigned char gpi0_f : 1; /**< GPI Falling Interrupt. Bit 0. 00045 Note that "GPI" refers to the GPIO programmed to be an input. 00046 0 = No GPI falling edges have occurred since the last time this bit was read. 00047 1 = A GPI falling edge has occurred since the last time this bit was read. */ 00048 unsigned char gpi0_r : 1; /**< GPI Rising Interrupt. Bit 1. 00049 Note that "GPI" refers to the GPIO programmed to be an input. 00050 0 = No GPI rising edges have occurred since the last time this bit was read. 00051 1 = A GPI rising edge has occurred since the last time this bit was read. */ 00052 unsigned char nen_f : 1; /**< nEN Falling Interrupt.Bit 2. 00053 0 = No nEN falling edges have occurred since the last time this bit was read. 00054 1 = A nEN falling edge as occurred since the last time this bit was read. */ 00055 unsigned char nen_r : 1; /**< nEN Rising Interrupt. Bit 3. 00056 0 = No nEN rising edges have occurred since the last time this bit was read. 00057 1 = A nEN rising edge as occurred since the last time this bit was read. */ 00058 unsigned char tjal1_r : 1; /**< Thermal Alarm 1 Rising Interrupt. Bit 4. 00059 0 = The junction temperature has not risen above TJAL1 since the last time this bit was read. 00060 1 = The junction temperature has risen above TJAL1 since the last time this bit was read. */ 00061 unsigned char tjal2_r : 1; /**< Thermal Alarm 2 Rising Interrupt. Bit 5. 00062 0 = The junction temperature has not risen above TJAL2 since the last time this bit was read. 00063 1 = The junction temperature has risen above TJAL2 since the last time this bit was read. */ 00064 unsigned char d0d1_r : 1; /**< LDO Dropout Detector Rising Interrupt. Bit 6. 00065 0 = The LDO has not detected dropout since the last time this bit was read. 00066 1 = The LDO has detected dropout since the last time this bit was read. */ 00067 unsigned char dod0_r : 1; /**< LDO Dropout Detector Rising Interrupt. Bit 7. 00068 0 = The LDO has not detected dropout since the last time this bit was read. 00069 1 = The LDO has detected dropout since the last time this bit was read. */ 00070 } bits; 00071 } reg_int_glbl0_t; 00072 00073 /** 00074 * @brief INT_GLBL1 Register 00075 * 00076 * Address : 0x04 00077 */ 00078 typedef union { 00079 unsigned char raw; 00080 struct { 00081 unsigned char gpi1_f : 1; /**< GPI Falling Interrupt. Bit 0. 00082 Note that "GPI" refers to the GPIO programmed to be an input. 00083 0 = No GPI falling edges have occurred since the last time this bit was read. 00084 1 = A GPI falling edge has occurred since the last time this bit was read. */ 00085 unsigned char gpi1_r : 1; /**< GPI Rising Interrupt. Bit 1. 00086 Note that "GPI" refers to the GPIO programmed to be an input. 00087 0 = No GPI rising edges have occurred since the last time this bit was read. 00088 1 = A GPI rising edge has occurred since the last time this bit was read. */ 00089 unsigned char sbb0_f : 1; /**< SBB0 Fault Indicator. Bit 2. 00090 0 = No fault has occurred on SBB0 since the last time this bit was read. 00091 1 = SBB0 has fallen out of regulation since the last time this bit was read. */ 00092 unsigned char sbb1_f : 1; /**< SBB1 Fault Indicator. Bit 3. 00093 0 = No fault has occurred on SBB1 since the last time this bit was read. 00094 1 = SBB1 has fallen out of regulation since the last time this bit was read. */ 00095 unsigned char sbb2_f : 1; /**< SBB2 Fault Indicator. Bit 4. 00096 0 = No fault has occurred on SBB2 since the last time this bit was read. 00097 1 = SBB2 has fallen out of regulation since the last time this bit was read. */ 00098 unsigned char ldo0_f : 1; /**< LDO0 Fault Interrupt. Bit 5. 00099 0 = No fault has occurred on LDO0 since the last time this bit was read. 00100 1 = LDO0 has fallen out of regulation since the last time this bit was read. */ 00101 unsigned char ldo1_f : 1; /**< LDO1 Fault Interrupt. Bit 6. 00102 0 = No fault has occurred on LDO1 since the last time this bit was read. 00103 1 = LDO1 has fallen out of regulation since the last time this bit was read. */ 00104 unsigned char rsvd : 1; /**< Reserved. 00105 Unutilized bit. Write to 0. Reads are don't care. Bit 7. */ 00106 } bits; 00107 } reg_int_glbl1_t; 00108 00109 /** 00110 * @brief ERCFLAG Register 00111 * 00112 * Address : 0x05 00113 */ 00114 typedef union { 00115 unsigned char raw; 00116 struct { 00117 unsigned char tovld : 1; /**< Thermal Overload. Bit 0. 00118 0 = Thermal overload has not occurred since the last read of this register. 00119 1 = Thermal overload has occurred since the list read of this register. 00120 This indicates that the junction temperature has exceeded 165ºC. */ 00121 unsigned char sysovlo : 1; /**< SYS Domain Overvoltage Lockout. Bit 1. 00122 0 = The SYS domain overvoltage lockout has not occurred since this last read of this register. 00123 1 = The SYS domain overvoltage lockout has occurred since the last read of this register. */ 00124 unsigned char sysuvlo : 1; /**< SYS Domain Undervoltage Lockout. Bit 2. 00125 0 = The SYS domain undervoltage lockout has not occurred since this last read of this register. 00126 1 = The SYS domain undervoltage lockout has occurred since the last read of this register. */ 00127 unsigned char mrst_f : 1; /**< Manual Reset Timer. Bit 3. 00128 0 = A Manual Reset has not occurred since this last read of this register. 00129 1 = A Manual Reset has occurred since this last read of this register. */ 00130 unsigned char sft_off_f : 1; /**< Software Off Flag. Bit 4. 00131 0 = The SFT_OFF function has not occurred since the last read of this register. 00132 1 = The SFT_OFF function has occurred since the last read of this register. */ 00133 unsigned char sft_crst_f: 1; /**< Software Cold Reset Flag. Bit 5. 00134 0 = The software cold reset has not occurred since the last read of this register. 00135 1 = The software cold reset has occurred since the last read of this register. */ 00136 unsigned char wdt_off : 1; /**< Watchdog Timer OFF Flag. Bit 6. 00137 This bit sets when the watchdog timer expires and causes a power-off. 00138 0 = Watchdog timer has not caused a power-off since the last time this bit was read. 00139 1 = Watchdog timer has expired and caused a power-off since the last time this bit was read. */ 00140 unsigned char wdt_rst : 1; /**< Watchdog Timer Reset Flag. Bit 7. 00141 This bit sets when the watchdog timer expires and causes a power-reset. 00142 0 = Watchdog timer has not caused a power-reset since the last time this bit was read. 00143 1 = Watchdog timer has expired and caused a power-reset since the last time this bit was read.*/ 00144 } bits; 00145 } reg_ercflag_t; 00146 00147 /** 00148 * @brief STAT_GLBL Register 00149 * 00150 * Address : 0x06 00151 */ 00152 typedef union { 00153 unsigned char raw; 00154 struct { 00155 unsigned char stat_irq : 1; /**< Software Version of the nIRQ MOSFET gate drive. Bit 0. 00156 0 = unmasked gate drive is logic low 00157 1 = unmasked gate drive is logic high */ 00158 unsigned char stat_en : 1; /**< Debounced Status for the nEN input. Bit 1. 00159 0 = nEN is not active (logic high) 00160 1 = nEN is active (logic low) */ 00161 unsigned char tjal1_s : 1; /**< Thermal Alarm 1 Status. Bit 2. 00162 0 = The junction temperature is less than TJAL1 00163 1 = The junction temperature is greater than TJAL1 */ 00164 unsigned char tjal2_s : 1; /**< Thermal Alarm 2 Status. Bit 3. 00165 0 = The junction temperature is less than TJAL2 00166 1 = The junction temperature is greater than TJAL2 */ 00167 unsigned char dod1_s : 1; /**< LDO1 Dropout Detector Rising Status. Bit 4. 00168 0 = LDO1 is not in dropout 00169 1 = LDO1 is in dropout */ 00170 unsigned char dod0_s : 1; /**< LDO0 Dropout Detector Rising Status. Bit 5. 00171 0 = LDO0 is not in dropout 00172 1 = LDO0 is in dropout */ 00173 unsigned char bok : 1; /**< BOK Interrupt Status. Bit 6. 00174 0 = Main Bias is not ready. 00175 1 = Main Bias enabled and ready. */ 00176 unsigned char didm : 1; /**< Device Identification Bits for Metal Options. Bit 7. 00177 0 = MAX77658 00178 1 = Reserved */ 00179 } bits; 00180 } reg_stat_glbl_t; 00181 00182 /** 00183 * @brief INTM_GLBL0 Register 00184 * 00185 * Address : 0x08 00186 */ 00187 typedef union { 00188 unsigned char raw; 00189 struct { 00190 unsigned char gpi0_fm : 1; /**< GPI Falling Interrupt Mask. Bit 0. 00191 0 = Unmasked. If GPI_F goes from 0 to 1, then nIRQ goes low. 00192 nIRQ goes high when all interrupt bits are cleared. 00193 1 = Masked. nIRQ does not go low due to GPI_F. */ 00194 unsigned char gpi0_rm : 1; /**< GPI Rising Interrupt Mask. Bit 1. 00195 0 = Unmasked. If GPI_R goes from 0 to 1, then nIRQ goes low. 00196 nIRQ goes high when all interrupt bits are cleared. 00197 1 = Masked. nIRQ does not go low due to GPI_R. */ 00198 unsigned char nen_fm : 1; /**< nEN Falling Interrupt Mask. Bit 2. 00199 0 = Unmasked. If nEN_F goes from 0 to 1, then nIRQ goes low. 00200 nIRQ goes high when all interrupt bits are cleared. 00201 1 = Masked. nIRQ does not go low due to nEN_F. */ 00202 unsigned char nen_rm : 1; /**< nEN Rising Interrupt Mask. Bit 3. 00203 0 = Unmasked. If nEN_R goes from 0 to 1, then nIRQ goes low. 00204 nIRQ goes high when all interrupt bits are cleared. 00205 1 = Masked. nIRQ does not go low due to nEN_R. */ 00206 unsigned char tjal1_rm : 1; /**< Thermal Alarm 1 Rising Interrupt Mask. Bit 4. 00207 0 = Unmasked. If TJAL1_R goes from 0 to 1, then nIRQ goes low. 00208 nIRQ goes high when all interrupt bits are cleared. 00209 1 = Masked. nIRQ does not go low due to TJAL1_R. */ 00210 unsigned char tjal2_rm : 1; /**< Thermal Alarm 2 Rising Interrupt Mask. Bit 5. 00211 0 = Unmasked. If TJAL2_R goes from 0 to 1, then nIRQ goes low. 00212 nIRQ goes high when all interrupt bits are cleared. 00213 1 = Masked. nIRQ does not go low due to TJAL2_R. */ 00214 unsigned char dod1_rm : 1; /**< LDO Dropout Detector Rising Interrupt Mask. Bit 6. 00215 0 = Unmasked. If DOD1_R goes from 0 to 1, then nIRQ goes low. 00216 nIRQ goes high when all interrupt bits are cleared. 00217 1 = Masked. nIRQ does not go low due to DOD1_R. */ 00218 unsigned char dod0_rm : 1; /**< LDO Dropout Detector Rising Interrupt Mask. Bit 7. 00219 0 = Unmasked. If DOD0_R goes from 0 to 1, then nIRQ goes low. 00220 nIRQ goes high when all interrupt bits are cleared. 00221 1 = Masked. nIRQ does not go low due to DOD0_R. */ 00222 } bits; 00223 } reg_intm_glbl0_t; 00224 00225 /** 00226 * @brief INTM_GLBL1 Register 00227 * 00228 * Address : 0x09 00229 */ 00230 typedef union { 00231 unsigned char raw; 00232 struct { 00233 unsigned char gpi1_fm : 1; /**< GPI Falling Interrupt Mask. Bit 0. 00234 0 = Unmasked. If GPI_F goes from 0 to 1, then nIRQ goes low. 00235 nIRQ goes high when all interrupt bits are cleared. 00236 1 = Masked. nIRQ does not go low due to GPI_F. */ 00237 unsigned char gpi1_rm : 1; /**< GPI Rising Interrupt Mask. Bit 1. 00238 0 = Unmasked. If GPI_R goes from 0 to 1, then nIRQ goes low. 00239 nIRQ goes high when all interrupt bits are cleared. 00240 1 = Masked. nIRQ does not go low due to GPI_R. */ 00241 unsigned char sbb0_fm : 1; /**< SBB0 Fault Interrupt Mask. Bit 2. 00242 0 = Unmasked. If SBB0_F goes from 0 to 1, then nIRQ goes low. 00243 nIRQ goes high when all interrupt bits are cleared.. 00244 1 = Masked. nIRQ does not go low due to SBB0_F. */ 00245 unsigned char sbb1_fm : 1; /**< SBB1 Fault Interrupt Mask. Bit 3. 00246 0 = Unmasked. If SBB1_F goes from 0 to 1, then nIRQ goes low. 00247 nIRQ goes high when all interrupt bits are cleared.. 00248 1 = Masked. nIRQ does not go low due to SBB1_F. */ 00249 unsigned char sbb2_fm : 1; /**< SBB2 Fault Interrupt Mask. Bit 4. 00250 0 = Unmasked. If SBB2_F goes from 0 to 1, then nIRQ goes low. 00251 nIRQ goes high when all interrupt bits are cleared.. 00252 1 = Masked. nIRQ does not go low due to SBB2_F. */ 00253 unsigned char ldo0_m : 1; /**< LDO0 Fault Interrupt. Bit 5. 00254 0 = Unmasked. If LDO0_F goes from 0 to 1, then nIRQ goes low. 00255 nIRQ goes high when all interrupt bits are cleared. 00256 1 = Masked. nIRQ does not go low due to LDO0_F. */ 00257 unsigned char ldo1_m : 1; /**< LDO1 Fault Interrupt Mask. Bit 6. 00258 0 = Unmasked. If LDO1_F goes from 0 to 1, then nIRQ goes low. 00259 nIRQ goes high when all interrupt bits are cleared. 00260 1 = Masked. nIRQ does not go low due to LDO1_F. */ 00261 unsigned char rsvd : 1; /**< Reserved. 00262 Unutilized bit. Write to 0. Reads are don't care. Bit 7. */ 00263 } bits; 00264 } reg_intm_glbl1_t; 00265 00266 /** 00267 * @brief CNFG_GLBL Register 00268 * 00269 * Address : 0x10 00270 */ 00271 typedef union { 00272 unsigned char raw; 00273 struct { 00274 unsigned char sft_ctrl : 2; /**< Software Reset Functions. Bit 1:0. 00275 0b00 = No Action 00276 0b01 = Software Cold Reset (SFT_CRST). The device powers down, resets, and the powers up again. 00277 0b10 = Software Off (SFT_OFF). The device powers down, resets, and then remains off and waiting for a wake-up event. 00278 0b11 = Factory-Ship Mode Enter (FSM). */ 00279 unsigned char dben_nen : 1; /**< Debounce Timer Enable for the nEN Pin. Bit 2. 00280 0 = 500μs Debounce 00281 1 = 30ms Debounce */ 00282 unsigned char nen_mode : 2; /**< nEN Input (ON-KEY) Default Configuration Mode. Bit 4:3. 00283 0b00 = Push-button mode 00284 0b01 = Slide-switch mode 00285 0b10 = Logic mode 00286 0b11 = Reserved */ 00287 unsigned char sbia_lpm : 1; /**< Main Bias Low-Power Mode Software Request. Bit 5. 00288 0 = Main Bias requested to be in Normal-Power Mode by software. 00289 1 = Main Bias request to be in Low-Power Mode by software. */ 00290 unsigned char t_mrst : 1; /**< Sets the Manual Reset Time (tMRST). Bit 6. 00291 0 = 8s 00292 1 = 4s */ 00293 unsigned char pu_dis : 1; /**< nEN Internal Pullup Resistor. Bit 7. 00294 0 = Strong internal nEN pullup (200kΩ) 00295 1 = Weak internal nEN pullup (10MΩ) */ 00296 } bits; 00297 } reg_cnfg_glbl_t; 00298 00299 /** 00300 * @brief CNFG_GPIO0 Register 00301 * 00302 * Address : 0x11 00303 */ 00304 typedef union { 00305 unsigned char raw; 00306 struct { 00307 unsigned char gpo_dir : 1; /**< GPIO Direction. Bit 0. 00308 0 = General purpose output (GPO) 00309 1 = General purpose input (GPI) */ 00310 unsigned char gpo_di : 1; /**< GPIO Digital Input Value. Bit 1. 00311 0 = Input logic low 00312 1 = Input logic high */ 00313 unsigned char gpo_drv : 1; /**< General Purpose Output Driver Type. Bit 2. 00314 This bit is a don't care when DIR = 1 (configured as input) When set for GPO (DIR = 0): 00315 0 = Open-Drain 00316 1 = Push-Pull */ 00317 unsigned char gpo_do : 1; /**< General Purpose Output Data Output. Bit 3. 00318 This bit is a don't care when DIR = 1 (configured as input). When set for GPO (DIR = 0): 00319 0 = GPIO is output is logic low 00320 1 = GPIO is output logic high when set as push-pull output (DRV = 1). */ 00321 unsigned char dben_gpi : 1; /**< General Purpose Input Debounce Timer Enable. Bit 4. 00322 0 = no debounce 00323 1 = 30ms debounce */ 00324 unsigned char alt_gpio : 1; /**< Alternate Mode Enable for GPIO0. Bit 5. 00325 0 = Standard GPIO. 00326 1 = Active-high input, Force USB Suspend (FUS). FUS is only active if the FUS_M bit is set to 0. */ 00327 unsigned char : 1; /**< Bit 6. */ 00328 unsigned char rsvd : 1; /**< Reserved. Bit 7. 00329 Unutilized bit. Write to 0. Reads are don't care. */ 00330 } bits; 00331 } reg_cnfg_gpio0_t; 00332 00333 /** 00334 * @brief CNFG_GPIO1 Register 00335 * 00336 * Address : 0x12 00337 */ 00338 typedef union { 00339 unsigned char raw; 00340 struct { 00341 unsigned char gpo_dir : 1; /**< GPIO Direction. Bit 0. 00342 0 = General purpose output (GPO) 00343 1 = General purpose input (GPI) */ 00344 unsigned char gpo_di : 1; /**< GPIO Digital Input Value. Bit 1. 00345 0 = Input logic low 00346 1 = Input logic high */ 00347 unsigned char gpo_drv : 1; /**< General Purpose Output Driver Type. Bit 2. 00348 This bit is a don't care when DIR = 1 (configured as input) When set for GPO (DIR = 0): 00349 0 = Open-Drain 00350 1 = Push-Pull */ 00351 unsigned char gpo_do : 1; /**< General Purpose Output Data Output. Bit 3. 00352 This bit is a don't care when DIR = 1 (configured as input). When set for GPO (DIR = 0): 00353 0 = GPIO is output is logic low 00354 1 = GPIO is output logic high when set as push-pull output (DRV = 1). */ 00355 unsigned char dben_gpi : 1; /**< General Purpose Input Debounce Timer Enable. Bit 4. 00356 0 = no debounce 00357 1 = 30ms debounce */ 00358 unsigned char alt_gpio : 1; /**< Alternate Mode Enable for GPIO1. Bit 5. 00359 0 = Standard GPIO. 00360 1 = Active-high output of SBB2's Flexible Power Sequencer (FPS) slot. */ 00361 unsigned char rsvd : 2; /**< Reserved. Bit 7:6. 00362 Unutilized bit. Write to 0. Reads are don't care. */ 00363 } bits; 00364 } reg_cnfg_gpio1_t; 00365 00366 /** 00367 * @brief CNFG_GPIO2 Register 00368 * 00369 * Address : 0x13 00370 */ 00371 typedef union { 00372 unsigned char raw; 00373 struct { 00374 unsigned char gpo_dir : 1; /**< GPIO Direction. Bit 0. 00375 0 = General purpose output (GPO) 00376 1 = General purpose input (GPI) */ 00377 unsigned char gpo_di : 1; /**< GPIO Digital Input Value. Bit 1. 00378 0 = Input logic low 00379 1 = Input logic high */ 00380 unsigned char gpo_drv : 1; /**< General Purpose Output Driver Type. Bit 2. 00381 This bit is a don't care when DIR = 1 (configured as input) When set for GPO (DIR = 0): 00382 0 = Open-Drain 00383 1 = Push-Pull */ 00384 unsigned char gpo_do : 1; /**< General Purpose Output Data Output. Bit 3. 00385 This bit is a don't care when DIR = 1 (configured as input). When set for GPO (DIR = 0): 00386 0 = GPIO is output is logic low 00387 1 = GPIO is output logic high when set as push-pull output (DRV = 1). */ 00388 unsigned char dben_gpi : 1; /**< General Purpose Input Debounce Timer Enable. Bit 4. 00389 0 = no debounce 00390 1 = 30ms debounce */ 00391 unsigned char alt_gpio : 1; /**< Alternate Mode Enable for GPIO2. Bit 5. 00392 0 = Standard GPIO. 00393 1 = Active-high input, Enable DISQBAT. */ 00394 unsigned char rsvd : 2; /**< Reserved. Bit 7:6. 00395 Unutilized bit. Write to 0. Reads are don't care. */ 00396 } bits; 00397 } reg_cnfg_gpio2_t; 00398 00399 /** 00400 * @brief CID Register 00401 * 00402 * Address : 0x14 00403 */ 00404 typedef union { 00405 unsigned char raw; 00406 struct { 00407 unsigned char cid : 4; /**< Chip Identification Code. Bit 4:0. 00408 The Chip Identification Code refers to a set of reset values in the register map, or the "OTP configuration.". */ 00409 unsigned char : 4; /**< Bit 7:4. */ 00410 } bits; 00411 } reg_cid_t; 00412 00413 /** 00414 * @brief CNFG_WDT Register 00415 * 00416 * Address : 0x17 00417 */ 00418 typedef union { 00419 unsigned char raw; 00420 struct { 00421 unsigned char wdt_lock : 1; /**< Factory-Set Safety Bit for the Watchdog Timer. Bit 0. 00422 0 = Watchdog timer can be enabled and disabled with WDT_EN. 00423 1 = Watchdog timer can not be disabled with WDT_EN. 00424 However, WDT_EN can still be used to enable the watchdog timer. */ 00425 unsigned char wdt_en : 1; /**< Watchdog Timer Enable. Bit 1. 00426 0 = Watchdog timer is not enabled. 00427 1 = Watchdog timer is enabled. The timer will expire if not reset by setting WDT_CLR. */ 00428 unsigned char wdt_clr : 1; /**< Watchdog Timer Clear Control. Bit 2. 00429 0 = Watchdog timer period is not reset. 00430 1 = Watchdog timer is reset back to tWD. */ 00431 unsigned char wdt_mode : 1; /**< Watchdog Timer Expired Action. Bit 3. 00432 0 = Watchdog timer expire causes power-off. 00433 1 = Watchdog timer expire causes power-reset. */ 00434 unsigned char wdt_per : 2; /**< Watchdog Timer Period. Bit 5:4. 00435 0b00 = 16 seconds 0b01 = 32 seconds 00436 0b10 = 64 seconds 0b11 = 128 seconds. */ 00437 unsigned char rsvd : 2; /**< Reserved. Bit 7:6. 00438 Unutilized bit. Write to 0. Reads are don't care. */ 00439 } bits; 00440 } reg_cnfg_wdt_t; 00441 00442 /** 00443 * @brief INT_CHG Register 00444 * 00445 * Address : 0x01 00446 */ 00447 typedef union { 00448 unsigned char raw; 00449 struct { 00450 unsigned char thm_i : 1; /**< Thermistor related interrupt. Bit 0. 00451 0 = The bits in THM_DTLS[2:0] have not changed since the last time this bit was read 00452 1 = The bits in THM_DTLS[2:0] have changed since the last time this bit was read */ 00453 unsigned char chg_i : 1; /**< Charger related interrupt. Bit 1. 00454 0 = The bits in CHG_DTLS[3:0] have not changed since the last time this bit was read 00455 1 = The bits in CHG_DTLS[3:0] have changed since the last time this bit was read */ 00456 unsigned char chgin_i : 1; /**< CHGIN related interrupt. Bit 2. 00457 0 = The bits in CHGIN_DTLS[1:0] have not changed since the last time this bit was read 00458 1 = The bits in CHGIN_DTLS[1:0] have changed since the last time this bit was read */ 00459 unsigned char tj_reg_i : 1; /**< Die junction temperature regulation interrupt. Bit 3. 00460 0 = The die temperature has not exceeded TJ-REG since the last time this bit was read 00461 1 = The die temperature has exceeded TJ-REG since the last time this bit was read */ 00462 unsigned char chgin_ctrl_i : 1; /**< CHGIN control-loop related interrupt. Bit 4. 00463 0 = Neither the VCHGIN_MIN_STAT nor the ICHGIN_LIM_STAT bits have changed since the last time this bit was read 00464 1 = The VCHGIN_MIN_STAT or ICHGIN_LIM_STAT bits have changed since the last time this bit was read */ 00465 unsigned char sys_ctrl_i : 1; /**< Minimum System Voltage Regulation-loop related interrupt. Bit 5. 00466 0 = The minimum system voltage regulation loop has not engaged since the last time this bit was read 00467 1 = The minimum system voltage regulation loop has engaged since the last time this bit was read */ 00468 unsigned char sys_cnfg_i : 1; /**< System voltage configuration error interrupt. Bit 6. 00469 0 = The bit combination in CHG_CV has not been forced to change since the last time this bit was read 00470 1 = The bit combination in CHG_CV has been forced to change to ensure VSYS-REG = VFAST-CHG + 200mV 00471 since the last time this bit was read */ 00472 unsigned char rsvd : 1; /**< Reserved. Bit 7. 00473 Unutilized bit. Write to 0. Reads are don't care. */ 00474 } bits; 00475 } reg_int_chg_t; 00476 00477 /** 00478 * @brief STAT_CHG_A 00479 * 00480 * Address : 0x02 00481 */ 00482 typedef union { 00483 unsigned char raw; 00484 struct 00485 { 00486 unsigned char thm_dtls : 3; /**< Battery Temperature Details. Bit 2:0. 00487 0b000 = Thermistor is disabled (THM_EN = 0) 00488 0b001 = Battery is cold as programmed by THM_COLD[1:0] If thermistor and charger are enabled while the battery is cold, a battery temperature fault will occur. 00489 0b010 = Battery is cool as programmed by THM_COOL[1:0] 00490 0b011 = Battery is warm as programmed by THM_WARM[1:0] 00491 0b100 = Battery is hot as programmed by THM_HOT[1:0]. If thermistor and charger are enabled while the battery is hot, a battery temperature fault will occur. 00492 0b101 = Battery is in the normal temperature region 00493 0b110 - 0b111 = reserved */ 00494 unsigned char tj_reg_stat : 1; /**< Maximum Junction Temperature Regulation Loop Status. Bit 3. 00495 0 = The maximum junction temperature regulation loop is not engaged 00496 1 = The maximum junction temperature regulation loop has engaged to regulate the junction temperature to less than TJ-REG */ 00497 unsigned char vsys_min_stat : 1; /**< Minimum System Voltage Regulation Loop Status. Bit 4. 00498 0 = The minimum system voltage regulation loop is not enganged 00499 1 = The minimum system voltage regulation loop is engaged to regulate VSYS ≥ VSYS-MIN */ 00500 unsigned char ichgin_lim_stat : 1; /**< Input Current Limit Loop Status. Bit 5. 00501 0 = The CHGIN current limit loop is not engaged 00502 1 = The CHGIN current limit loop has engaged to regulate ICHGIN ≤ ICHGIN-LIM */ 00503 unsigned char vchgin_min_stat : 1; /**< Minimum Input Voltage Regulation Loop Status. Bit 6. 00504 0 = The minimum CHGIN voltage regulation loop is not engaged 00505 1 = The minimum CHGIN voltage regulation loop has engaged to regulate VCHGIN ≥ VCHGIN-MIN */ 00506 unsigned char rsvd : 1; /**< Reserved. Bit 7. 00507 Unutilized bit. Write to 0. Reads are don't care. */ 00508 } bits; 00509 } reg_stat_chg_a_t; 00510 00511 /** 00512 * @brief STAT_CHG_B 00513 * 00514 * Address : 0x03 00515 */ 00516 typedef union { 00517 unsigned char raw; 00518 struct 00519 { 00520 unsigned char time_sus : 1; /**< Time Suspend Indicator. Bit 0. 00521 0 = The charger's timers are either not active, or not suspended 00522 1 = The charger's active timer is suspended due to one of three reasons: 00523 charge current dropped below 20% of IFAST-CHG while the charger state machine is in FAST CHARGE CC mode, 00524 the charger is in SUPPLEMENT mode, or the charger state machine is in BATTERY TEMPERATURE FAULT mode. */ 00525 unsigned char chg : 1; /**< Quick Charger Status. Bit 1. 00526 0 = Charging is not happening 00527 1 = Charging is happening */ 00528 unsigned char chgin_dtls : 2; /**< CHGIN Status Detail. Bit 3:2. 00529 0b00 = The CHGIN input voltage is below the UVLO threshold (VCHGIN < VUVLO) 00530 0b01 = The CHGIN input voltage is above the OVP threshold (VCHGIN > VOVP) 00531 0b10 = The CHGIN input is being debounced (no power accepted from CHGIN during debounce) 00532 0b11 = The CHGIN input is okay and debounced */ 00533 unsigned char chg_dtls : 4; /**< Charger Details. Bit 7:4. 00534 0b0000 = Off 00535 0b0001 = Prequalification mode 00536 0b0010 = Fast-charge constant-current (CC) mode 00537 0b0011 = JEITA modified fast-charge constant-current mode 00538 0b0100 = Fast-charge constant-voltage (CV) mode 00539 0b0101 = JEITA modified fast-charge constant-voltage mode 00540 0b0110 = Top-off mode 00541 0b0111 = JEITA modified top-off mode 00542 0b1000 = Done 00543 0b1001 = JEITA modified done (done was entered through the JEITA-modified fast-charge states) 00544 0b1010 = Prequalification timer fault 00545 0b1011 = Fast-charge timer fault 00546 0b1100 = Battery temperature fault 00547 0b1101 - 0b1111 = reserved */ 00548 } bits; 00549 } reg_stat_chg_b_t; 00550 00551 /** 00552 * @brief INT_M_CHG Register 00553 * 00554 * Address : 0x07 00555 */ 00556 typedef union { 00557 unsigned char raw; 00558 struct { 00559 unsigned char thm_m : 1; /**< Setting this bit prevents the THM_I bit from causing hardware IRQs. Bit 0. 00560 0 = THM_I is not masked 00561 1 = THM_I is masked */ 00562 unsigned char chg_m : 1; /**< Setting this bit prevents the CHG_I bit from causing hardware IRQs. Bit 1. 00563 0 = CHG_I is not masked 00564 1 = CHG_I is masked */ 00565 unsigned char chgin_m : 1; /**< Setting this bit prevents the CHGIN_I bit from causing hardware IRQs. Bit 2. 00566 0 = CHGIN_I is not masked 00567 1 = CHGIN_I is masked */ 00568 unsigned char tj_reg_m : 1; /**< Setting this bit prevents the TJREG_I bit from causing hardware IRQs. Bit 3. 00569 0 = TJREG_I is not masked 00570 1 = TJREG_I is masked */ 00571 unsigned char chgin_ctrl_m : 1; /**< Setting this bit prevents the CHGIN_CTRL_I bit from causing hardware IRQs. Bit 4. 00572 0 = CHGIN_CTRL_I is not masked 00573 1 = CHGIN_CTRL_I is masked */ 00574 unsigned char sys_ctrl_m : 1; /**< Setting this bit prevents the SYS_CTRL_I bit from causing hardware IRQs. Bit 5. 00575 0 = SYS_CTRL_I is not masked 00576 1 = SYS_CTRL_I is masked */ 00577 unsigned char sys_cnfg_m : 1; /**< Setting this bit prevents the SYS_CNFG_I bit from causing hardware IRQs. Bit 6. 00578 0 = SYS_CNFG_I is not masked 00579 1 = SYS_CNFG_I is masked 00580 since the last time this bit was read */ 00581 unsigned char dis_aicl : 1; /**< Active input current loop. Bit 7. 00582 0 = Active Input Current Loop Active. 00583 1 = Active Input Current Loop Disabled. */ 00584 } bits; 00585 } reg_int_m_chg_t; 00586 00587 /** 00588 * @brief CNFG_CHG_A 00589 * 00590 * Address : 0x20 00591 */ 00592 typedef union { 00593 unsigned char raw; 00594 struct 00595 { 00596 unsigned char thm_cold : 2; /**< Sets the TCOLD JEITA Temperature Threshold. Bit 1:0. 00597 0b00 = TCOLD = -10ºC 00598 0b01 = TCOLD = -5ºC 00599 0b10 = TCOLD = 0ºC 00600 0b11 = TCOLD = 5ºC */ 00601 unsigned char thm_cool : 2; /**< Sets the TCOOL JEITA Temperature Threshold. Bit 3:2. 00602 0b00 = TCOOL = 0ºC 00603 0b01 = TCOOL = 5ºC 00604 0b10 = TCOOL = 10ºC 00605 0b11 = TCOOL = 15ºC */ 00606 unsigned char thm_warm : 2; /**< Sets the TWARM JEITA Temperature Threshold. Bit 5:4. 00607 0b00 = TWARM = 35ºC 00608 0b01 = TWARM = 40ºC 00609 0b10 = TWARM = 45ºC 00610 0b11 = TWARM = 50ºC */ 00611 unsigned char thm_hot : 2; /**< Sets the THOT JEITA Temperature Threshold. Bit 7:6. 00612 0b00 = THOT = 45ºC 00613 0b01 = THOT = 50ºC 00614 0b10 = THOT = 55ºC 00615 0b11 = THOT = 60ºC */ 00616 } bits; 00617 } reg_cnfg_chg_a_t; 00618 00619 /** 00620 * @brief CNFG_CHG_B 00621 * 00622 * Address : 0x21 00623 */ 00624 typedef union { 00625 unsigned char raw; 00626 struct 00627 { 00628 unsigned char chg_en : 1; /**< Charger Enable. Bit 0. Default value defined by OTP bit OTP_CHG_EN: 00629 0 = the battery charger is disabled 00630 1 = the battery charger is enabled */ 00631 unsigned char i_pq : 1; /**< Sets the prequalification charge current (IPQ) as a percentage of IFAST-CHG. Bit 1. 00632 0 = 10% 1 = 20% */ 00633 unsigned char ichgin_lim : 3; /**< CHGIN Input Current Limit (ICHGIN-LIM). Bit 4:2. When CNFG_SBB_TOP.ICHGIN_LIM_DEF = 0: 00634 0b000 = 95mA 0b001 = 190mA 00635 0b010 = 285mA 0b011 = 380mA 00636 0b100 = 475mA 0b101 0b111 = Reserved. */ 00637 unsigned char vchgin_min : 3; /**< Minimum CHGIN regulation voltage (VCHGIN-MIN). Bit 7:5. 00638 0b000 = 4.0V 0b001 = 4.1V 00639 0b010 = 4.2V 0b011 = 4.3V 00640 0b100 = 4.4V 0b101 = 4.5V 00641 0b110 = 4.6V 0b111 = 4.7V */ 00642 } bits; 00643 } reg_cnfg_chg_b_t; 00644 00645 /** 00646 * @brief CNFG_CHG_C 00647 * 00648 * Address : 0x22 00649 */ 00650 typedef union { 00651 unsigned char raw; 00652 struct 00653 { 00654 unsigned char t_topoff : 3; /**< Top-off timer value (tTO). Bit 2:0. 00655 0b000 = 0 minutes 0b001 = 5 minutes 00656 0b010 = 10 minutes 0b011 = 15 minutes 00657 0b100 = 20 minutes 0b101 = 25 minutes 00658 0b110 = 30 minutes 0b111 = 35 minutes */ 00659 unsigned char i_term : 2; /**< Charger Termination Current (ITERM). Bit 4:3. 00660 00 = 5% 01 = 7.5% 00661 10 = 10% 11 = 15% */ 00662 unsigned char chg_pq : 3; /**< Battery prequalification voltage threshold (VPQ). Bit 7:5. 00663 0b000 = 2.3V 0b001 = 2.4V 00664 0b010 = 2.5V 0b011 = 2.6V 00665 0b100 = 2.7V 0b101 = 2.8V 00666 0b110 = 2.9V 0b111 = 3.0V */ 00667 } bits; 00668 } reg_cnfg_chg_c_t; 00669 00670 /** 00671 * @brief CNFG_CHG_D 00672 * 00673 * Address : 0x23 00674 */ 00675 typedef union { 00676 unsigned char raw; 00677 struct 00678 { 00679 unsigned char vsys_reg : 5; /**< System voltage regulation (VSYS-REG). Bit 4:0. 00680 0x0 = 3.300V 0x1 = 3.350V 00681 0x2 = 3.400V ... 00682 0x1D = 4.750V 0x1E - 0x1F = 4.800V */ 00683 unsigned char tj_reg : 3; /**< Sets the die junction temperature regulation point, TJ-REG. Bit 7:5. 00684 0b000 = 60ºC 0b001 = 70ºC 00685 0b010 = 80ºC 0b011 = 90ºC 00686 0b100 - 0b111 = 100ºC */ 00687 } bits; 00688 } reg_cnfg_chg_d_t; 00689 00690 /** 00691 * @brief CNFG_CHG_E 00692 * 00693 * Address : 0x24 00694 */ 00695 typedef union { 00696 unsigned char raw; 00697 struct 00698 { 00699 unsigned char t_fast_chg : 2; /**< Sets the fast-charge safety timer, tFC. Bit 1:0. 00700 0b00 = Timer disabled 0b01 = 3 hours 00701 0b10 = 5 hours 0b11 = 7 hours */ 00702 unsigned char chg_cc : 6; /**< Sets the fast-charge constant current value, IFAST-CHG. Bit 7:2. 00703 0x0 = 7.5mA 0x1 = 15.0mA 00704 0x2 = 22.5mA ... 00705 0x26 = 292.5mA 0x27 - 0x3F = 300.0mA */ 00706 } bits; 00707 } reg_cnfg_chg_e_t; 00708 00709 /** 00710 * @brief CNFG_CHG_F 00711 * 00712 * Address : 0x25 00713 */ 00714 typedef union { 00715 unsigned char raw; 00716 struct 00717 { 00718 unsigned char : 2; /**< Bit 1:0*/ 00719 unsigned char chg_cc_jeita : 6; /**< Sets IFAST-CHG-JEITA for when the battery is either cool or warm as defined 00720 by the VCOOL and VWARM temperature thresholds. Bit 7:2. 00721 0x0 = 7.5mA 0x1 = 15.0mA 00722 0x2 = 22.5mA ... 00723 0x26 = 292.5mA 0x27 - 0x3F = 300.0mA */ 00724 } bits; 00725 } reg_cnfg_chg_f_t; 00726 00727 /** 00728 * @brief CNFG_CHG_G 00729 * 00730 * Address : 0x26 00731 */ 00732 typedef union { 00733 unsigned char raw; 00734 struct 00735 { 00736 unsigned char fus_m : 1; /**< Forced USB Suspend Mask. Bit 0. 00737 FUS is only active if the FUS_M bit is set to 0.*/ 00738 unsigned char usbs : 1; /**< Setting this bit places CHGIN in USB suspend mode. Bit 1. 00739 0 = CHGIN is not suspended and may draw current from an adapter source 00740 1 = CHGIN is suspended and may draw no current from an adapter source */ 00741 unsigned char chg_cv : 6; /**< Sets fast-charge battery regulation voltage, VFAST-CHG. Bit 7:2. 00742 0x0 = 3.600V 0x1 = 3.625V 00743 0x2 = 3.650V ... 00744 0x27 = 4.575V 0x28 - 0x3F = 4.600V */ 00745 } bits; 00746 } reg_cnfg_chg_g_t; 00747 00748 /** 00749 * @brief CNFG_CHG_H 00750 * 00751 * Address : 0x27 00752 */ 00753 typedef union { 00754 unsigned char raw; 00755 struct 00756 { 00757 unsigned char chr_th_dis : 1; /**< Charger restart threshold disable. Bit 0. */ 00758 unsigned char sys_bat_prt : 1; /**< VSYS_REG - CHG_CV clamp. Bit 1. 00759 By default, the VSYS_REG has to be at least 200mV higher than the programmed CHG_CV. 00760 If this bit is set (hardware protection is turned off), the software has to provide 00761 the protection (the SYS voltage has to be 200mV higher than the BATT voltage). 00762 If the VSYS_REG is lower than CHG_CV+200mV, the charger will reduce. */ 00763 unsigned char chg_cv_jeita : 6; /**< Sets the modified VFAST-CHG-JEITA for when the battery is either cool or 00764 warm as defined by the VCOOL and VWARM temperature thresholds. Bit 7:2. 00765 0x0 = 3.600V 0x1 = 3.625V 00766 0x2 = 3.650V ... 00767 0x27 = 4.575V 0x28 - 0x3F = 4.600V */ 00768 } bits; 00769 } reg_cnfg_chg_h_t; 00770 00771 /** 00772 * @brief CNFG_CHG_I 00773 * 00774 * Address : 0x28 00775 */ 00776 typedef union { 00777 unsigned char raw; 00778 struct 00779 { 00780 unsigned char mux_sel : 4; /**< Selects the analog channel to connect to AMUX. Bit 3:0. 00781 0b0000 = Multiplexer is disabled and AMUX is high-impedance. 00782 0b0001 = CHGIN voltage monitor. 00783 0b0010 = CHGIN current monitor. 00784 0b0011 = BATT voltage monitor. 00785 0b0100 = BATT charge current monitor. Valid only while battery charging is happening (CHG = 1). 00786 0b0101 = BATT discharge current monitor normal measurement. 00787 0b0110 = BATT discharge current monitor nulling measurement. 00788 0b0111 = Reserved. 00789 0b1000 = Reserved. 00790 0b1001 = AGND voltage monitor (through 100Ω pull-down resistor) 00791 0b1010 - 0b1111 = SYS voltage monitor */ 00792 unsigned char imon_dischg_scale : 4; /**< Selects the battery discharge current full-scale current value. Bit 7:4. 00793 0x0 = 8.2mA 0x1 = 40.5mA 0x2 = 72.3mA 00794 0x3 = 103.4mA 0x4 = 134.1mA 00795 0x5 = 164.1mA 0x6 = 193.7mA 00796 0x7 = 222.7mA 0x8 = 251.2mA 00797 0x9 = 279.3mA 0xA - 0xF = 300.0mA */ 00798 } bits; 00799 } reg_cnfg_chg_i_t; 00800 00801 /** 00802 * @brief CNFG_SBB_TOP 00803 * 00804 * Address : 0x38 00805 */ 00806 typedef union { 00807 unsigned char raw; 00808 struct 00809 { 00810 unsigned char drv_sbb : 2; /**< SIMO Buck-Boost (all channels) Drive Strength Trim. Bit 1:0. 00811 0b00 = Fastest transition time 00812 0b01 = A little slower than 0b00 00813 0b10 = A little slower than 0b01 00814 0b11 = A little slower than 0b10 */ 00815 unsigned char : 5; /**< Bit 6:2.*/ 00816 unsigned char dis_lpm : 1; /**< Disables the automatic Low Power Mode for Each SIMO Channel. Bit 7. 00817 0b0 = Automatic Low Power Mode for each SIMO channel 00818 0b1 = Disable LPM feature for each SIMO channel */ 00819 } bits; 00820 } reg_cnfg_sbb_top_t; 00821 00822 /** 00823 * @brief CNFG_SBB0_A 00824 * 00825 * Address : 0x39 00826 */ 00827 typedef union { 00828 unsigned char raw; 00829 struct 00830 { 00831 unsigned char tv_sbb0 : 8; /**< SIMO Buck-Boost Channel 0 Target Output Voltage. Bit 7:0. 00832 0x00 = 0.500V 0x01 = 0.525V 0x02 = 0.550V 00833 0x03 = 0.575V 0x04 = 0.600V 0x05 = 0.625V 00834 0x06 = 0.650V 0x07 = 0.675V 0x08 = 0.700V 00835 ... 00836 0xC5 = 5.425V 0xC6 = 5.450V 0xC7 = 5.475V 00837 0xC8 to 0xFF = 5.500V */ 00838 } bits; 00839 } reg_cnfg_sbb0_a_t; 00840 00841 /** 00842 * @brief CNFG_SBB0_B 00843 * 00844 * Address : 0x3A 00845 */ 00846 typedef union { 00847 unsigned char raw; 00848 struct 00849 { 00850 unsigned char en_sbb0 : 3; /**< Enable Control for SIMO Buck-Boost Channel 0, 00851 selecting either an FPS slot the channel powers-up and powers-down in 00852 or whether the channel is forced on or off. Bit 2:0. 00853 0b000 = FPS slot 0 0b001 = FPS slot 1 00854 0b010 = FPS slot 2 0b011 = FPS slot 3 00855 0b100 = Off irrespective of FPS 00856 0b101 = same as 0b100 0b110 = On irrespective of FPS 00857 0b111 = same as 0b110 */ 00858 unsigned char ade_sbb0 : 1; /**< SIMO Buck-Boost Channel 0 Active-Discharge Enable. Bit 3. 00859 0 = The active discharge function is disabled. 00860 When SBB0 is disabled, its discharge rate is a function of the output capacitance and the external load. 00861 1 = The active discharge function is enabled. 00862 When SBB0 is disabled, an internal resistor (RAD_SBB0) is activated from SBB0 to PGND to help the output voltage discharge. */ 00863 unsigned char ip_sbb0 : 2; /**< SIMO Buck-Boost Channel 0 Peak Current Limit. Bit 5:4 00864 0b00 = 1.000A 0b01 = 0.750A 00865 0b10 = 0.500A 0b11 = 0.333A*/ 00866 unsigned char op_mode0 : 2; /**< Operation mode of SBB0. Bit 6. 00867 0b00 = Automatic 00868 0b01 = Buck mode 00869 0b10 = Boost mode 00870 0b11 = Buck-boost mode*/ 00871 } bits; 00872 } reg_cnfg_sbb0_b_t; 00873 00874 /** 00875 * @brief CNFG_SBB1_A 00876 * 00877 * Address : 0x3B 00878 */ 00879 typedef union { 00880 unsigned char raw; 00881 struct 00882 { 00883 unsigned char tv_sbb1 : 8; /**< SIMO Buck-Boost Channel 1 Target Output Voltage. Bit 7:0. 00884 0x00 = 0.500V 0x01 = 0.525V 0x02 = 0.550V 00885 0x03 = 0.575V 0x04 = 0.600V 0x05 = 0.625V 00886 0x06 = 0.650V 0x07 = 0.675V 0x08 = 0.700V 00887 ... 00888 0xC5 = 5.425V 0xC6 = 5.450V 0xC7 = 5.475V 00889 0xC8 to 0xFF = 5.500V */ 00890 } bits; 00891 } reg_cnfg_sbb1_a_t; 00892 00893 /** 00894 * @brief CNFG_SBB1_B 00895 * 00896 * Address : 0x3C 00897 */ 00898 typedef union { 00899 unsigned char raw; 00900 struct 00901 { 00902 unsigned char en_sbb1 : 3; /**< Enable Control for SIMO Buck-Boost Channel 1, 00903 selecting either an FPS slot the channel powers-up and powers-down in 00904 or whether the channel is forced on or off. Bit 2:0. 00905 0b000 = FPS slot 0 0b001 = FPS slot 1 00906 0b010 = FPS slot 2 0b011 = FPS slot 3 00907 0b100 = Off irrespective of FPS 00908 0b101 = same as 0b100 0b110 = On irrespective of FPS 00909 0b111 = same as 0b110 */ 00910 unsigned char ade_sbb1 : 1; /**< SIMO Buck-Boost Channel 1 Active-Discharge Enable. Bit 3. 00911 0 = The active discharge function is disabled. 00912 When SBB0 is disabled, its discharge rate is a function of the output capacitance and the external load. 00913 1 = The active discharge function is enabled. 00914 When SBB0 is disabled, an internal resistor (RAD_SBB0) is activated from SBB0 to PGND to help the output voltage discharge. */ 00915 unsigned char ip_sbb1 : 2; /**< SIMO Buck-Boost Channel 1 Peak Current Limit. Bit 5:4. 00916 0b00 = 1.000A 0b01 = 0.750A 00917 0b10 = 0.500A 0b11 = 0.333A*/ 00918 unsigned char op_mode1 : 2; /**< Operation mode of SBB1. Bit 7:6. 00919 0b00 = Automatic 00920 0b01 = Buck mode 00921 0b10 = Boost mode 00922 0b11 = Buck-boost mode*/ 00923 } bits; 00924 } reg_cnfg_sbb1_b_t; 00925 00926 /** 00927 * @brief CNFG_SBB2_A 00928 * 00929 * Address : 0x3D 00930 */ 00931 typedef union { 00932 unsigned char raw; 00933 struct 00934 { 00935 unsigned char tv_sbb2 : 8; /**< SIMO Buck-Boost Channel 2 Target Output Voltage. Bit 7:0. 00936 0x00 = 0.500V 0x01 = 0.525V 0x02 = 0.550V 00937 0x03 = 0.575V 0x04 = 0.600V 0x05 = 0.625V 00938 0x06 = 0.650V 0x07 = 0.675V 0x08 = 0.700V 00939 ... 00940 0xC5 = 5.425V 0xC6 = 5.450V 0xC7 = 5.475V 00941 0xC8 to 0xFF = 5.500V */ 00942 } bits; 00943 } reg_cnfg_sbb2_a_t; 00944 00945 /** 00946 * @brief CNFG_SBB2_B 00947 * 00948 * Address : 0x3E 00949 */ 00950 typedef union { 00951 unsigned char raw; 00952 struct 00953 { 00954 unsigned char en_sbb2 : 3; /**< Enable Control for SIMO Buck-Boost Channel 2, 00955 selecting either an FPS slot the channel powers-up and powers-down in 00956 or whether the channel is forced on or off. Bit 2:0. 00957 0b000 = FPS slot 0 0b001 = FPS slot 1 00958 0b010 = FPS slot 2 0b011 = FPS slot 3 00959 0b100 = Off irrespective of FPS 00960 0b101 = same as 0b100 0b110 = On irrespective of FPS 00961 0b111 = same as 0b110 */ 00962 unsigned char ade_sbb2 : 1; /**< SIMO Buck-Boost Channel 2 Active-Discharge Enable Bit 3. 00963 0 = The active discharge function is disabled. 00964 When SBB0 is disabled, its discharge rate is a function of the output capacitance and the external load. 00965 1 = The active discharge function is enabled. 00966 When SBB0 is disabled, an internal resistor (RAD_SBB0) is activated from SBB0 to PGND to help the output voltage discharge. */ 00967 unsigned char ip_sbb2 : 2; /**< SIMO Buck-Boost Channel 2 Peak Current Limit. Bit 5:4. 00968 0b00 = 1.000A 0b01 = 0.750A 00969 0b10 = 0.500A 0b11 = 0.333A*/ 00970 unsigned char op_mode2 : 2; /**< Operation mode of SBB2. Bit 7:6. 00971 0b00 = Automatic 00972 0b01 = Buck mode 00973 0b10 = Boost mode 00974 0b11 = Buck-boost mode*/ 00975 } bits; 00976 } reg_cnfg_sbb2_b_t; 00977 00978 /** 00979 * @brief CNFG_DVS_SBB0_A 00980 * 00981 * Address : 0x3F 00982 */ 00983 typedef union { 00984 unsigned char raw; 00985 struct 00986 { 00987 unsigned char tv_sbb0_dvs : 8; /**< SIMO Buck-Boost Channel 0 Target Output Voltage. Bit 7:0. 00988 0x00 = 0.500V 0x01 = 0.525V 0x02 = 0.550V 00989 0x03 = 0.575V 0x04 = 0.600V 0x05 = 0.625V 00990 0x06 = 0.650V 0x07 = 0.675V 0x08 = 0.700V 00991 ... 00992 0xC5 = 5.425V 0xC6 = 5.450V 0xC7 = 5.475V 00993 0xC8 to 0xFF = 5.500V */ 00994 } bits; 00995 } reg_cnfg_dvs_sbb0_a_t; 00996 00997 /** 00998 * @brief CNFG_LDO0_A 00999 * 01000 * Address : 0x48 01001 */ 01002 typedef union { 01003 unsigned char raw; 01004 struct 01005 { 01006 unsigned char tv_ldo0_6_0 : 7; /**< LDO0 Target Output Voltage. Bit 6:0. 01007 0x00 = 0.500V 0x01 = 0.525V 0x02 = 0.550V 01008 0x03 = 0.575V 0x04 = 0.600V 0x05 = 0.625V 01009 0x06 = 0.650V 0x07 = 0.675V 0x08 = 0.700V 01010 ... 01011 0x7E = 3.650V 01012 0x7F = 3.675V 01013 When TV_LDO[7] = 0, TV_LDO[6:0] sets the 01014 LDO's output voltage range from 0.5V to 3.675V. 01015 When TV_LDO[7] = 1, TV_LDO[6:0] sets the 01016 LDO's output voltage from 1.825V to 5V. */ 01017 unsigned char tv_ldo0_7 : 1; /**< LDO0 Output Voltage. Bit7. 01018 This bit applies a 1.325V offset to the output voltage of the LDO0. 01019 0b0 = No Offset, 0b1 = 1.325V Offset 01020 */ 01021 01022 } bits; 01023 } reg_cnfg_ldo0_a_t; 01024 01025 /** 01026 * @brief CNFG_LDO0_B 01027 * 01028 * Address : 0x49 01029 */ 01030 typedef union { 01031 unsigned char raw; 01032 struct 01033 { 01034 unsigned char en_ldo0 : 3; /**< Enable Control for LDO0, 01035 selecting either an FPS slot the channel powers-up and 01036 powersdown in or whether the channel is forced on or off. Bit 2:0. 01037 0b000 = FPS slot 0 0b001 = FPS slot 1 01038 0b010 = FPS slot 2 0b011 = FPS slot 3 01039 0b100 = Off irrespective of FPS 01040 0b101 = same as 0b100 0b110 = On irrespective of FPS 01041 0b111 = same as 0b110 */ 01042 unsigned char ade_ldo0 : 1; /**< LDO0 Active-Discharge Enable. Bit 3. 01043 0 = The active discharge function is disabled. 01044 1 = The active discharge function is enabled.*/ 01045 unsigned char ldo0_md : 1; /**< Operation Mode of LDO0. Bit 4. 01046 0b0 = Low dropout linear regulator (LDO) mode 01047 0b1 = Load switch (LSW) mode*/ 01048 unsigned char : 3; /**< */ 01049 } bits; 01050 } reg_cnfg_ldo0_b_t; 01051 01052 /** 01053 * @brief CNFG_LDO1_A 01054 * 01055 * Address : 0x4A 01056 */ 01057 typedef union { 01058 unsigned char raw; 01059 struct 01060 { 01061 unsigned char tv_ldo1_6_0 : 7; /**< LDO1 Target Output Voltage. Bit 6:0. 01062 0x00 = 0.500V 0x01 = 0.525V 0x02 = 0.550V 01063 0x03 = 0.575V 0x04 = 0.600V 0x05 = 0.625V 01064 0x06 = 0.650V 0x07 = 0.675V 0x08 = 0.700V 01065 ... 01066 0x7E = 3.650V 01067 0x7F = 3.675V 01068 When TV_LDO[7] = 0, TV_LDO[6:0] sets the 01069 LDO's output voltage range from 0.5V to 3.675V. 01070 When TV_LDO[7] = 1, TV_LDO[6:0] sets the 01071 LDO's output voltage from 1.825V to 5V. */ 01072 unsigned char tv_ldo1_7 : 1; /**< LDO1 Output Voltage. Bit7. 01073 This bit applies a 1.325V offset to the output voltage of the LDO0. 01074 0b0 = No Offset, 0b1 = 1.325V Offset 01075 */ 01076 01077 } bits; 01078 } reg_cnfg_ldo1_a_t; 01079 01080 /** 01081 * @brief CNFG_LDO1_B 01082 * 01083 * Address : 0x4B 01084 */ 01085 typedef union { 01086 unsigned char raw; 01087 struct 01088 { 01089 unsigned char en_ldo1 : 3; /**< Enable Control for LDO1, 01090 selecting either an FPS slot the channel powers-up and 01091 powersdown in or whether the channel is forced on or off. Bit 2:0. 01092 0b000 = FPS slot 0 0b001 = FPS slot 1 01093 0b010 = FPS slot 2 0b011 = FPS slot 3 01094 0b100 = Off irrespective of FPS 01095 0b101 = same as 0b100 0b110 = On irrespective of FPS 01096 0b111 = same as 0b110 */ 01097 unsigned char ade_ldo1 : 1; /**< LDO1 Active-Discharge Enable. Bit 3. 01098 0 = The active discharge function is disabled. 01099 1 = The active discharge function is enabled.*/ 01100 unsigned char ldo1_md : 1; /**< Operation Mode of LDO1. Bit 4. 01101 0b0 = Low dropout linear regulator (LDO) mode 01102 0b1 = Load switch (LSW) mode*/ 01103 unsigned char : 3; /**< */ 01104 } bits; 01105 } reg_cnfg_ldo1_b_t; 01106 01107 /*FUEL GAUGE REGISTERS*/ 01108 01109 /** 01110 * @brief Status 01111 * 01112 * Address : 0x00 01113 */ 01114 typedef union { 01115 uint16_t raw; 01116 struct 01117 { 01118 unsigned char imn : 1; /**< Minimum Isys Threshold Exceeded. Bit 0. */ 01119 unsigned char por : 1; /**< Power-On Reset. Bit 1. 01120 This bit is set to 1 when the device detects that a software or hardware POR event has occurred. */ 01121 unsigned char spr_2 : 1; /**< Bit 2. */ 01122 unsigned char bst : 1; /**< Battery Status. Bit 3. 01123 Unutilized bit. Write to 0. Reads are don't care. */ 01124 unsigned char isysmx : 1; /**< SYS current is over OCP limit. Bit 4. 01125 Maximum SYS current threshold exceeded. */ 01126 unsigned char spr_5 : 1; /**< Bit 5. */ 01127 unsigned char thmhot : 1; /**< FG Control Charger Input Current Limit. Bit 6. 01128 Set to 1 to indicate a Thermistor Hot to allow FG control charger input current limit. 01129 ThmHot is set to 0 at power-up. */ 01130 unsigned char dsoci : 1; /**< 1% SOC Change Alert. Bit 7. 01131 This bit is set to 1 to indicate a 1% SOC change alert. dSOCi is set to 0 at power-up. */ 01132 unsigned char vmn : 1; /**< Minimum VALRT Threshold Exceeded. Bit 8. 01133 This bit is set to 1 whenever a VCELL register reading is below the minimum VALRT value. 01134 This bit may or may not need to be cleared by system software to detect the next event. 01135 See VS in the CONFIG register. Vmn is set to 0 at power-up. */ 01136 unsigned char tmn : 1; /**< Minimum TALRT Threshold Exceeded. Bit 9. 01137 This bit is set to 1 whenever a Temperature register reading is below the minimum TALRT value. 01138 This bit may or may not need to be cleared by system software to detect the next event. 01139 See TS in the CONFIG register. Tmn is set to 0 at power-up. */ 01140 unsigned char smn : 1; /**< Minimum SOCALRT Threshold Exceeded. Bit 10. 01141 This bit is set to 1 whenever SOC falls below the minimum SOCALRT value. 01142 This bit may or may not need to be cleared by system software to detect the next event. 01143 See SS in the CONFIG register and SACFG in the MiscCFG register. Smn is set to 0 at power-up.*/ 01144 unsigned char bi : 1; /**< Battery Insertion. Bit 11. 01145 This bit is set to 1 when the device detects that a battery has been inserted into the system by monitoring the AIN pin. 01146 This bit must be cleared by system software to detect the next insertion event. 01147 Bi is set to 0 at power-up. */ 01148 unsigned char vmx : 1; /**< Maximum VALRT Threshold Exceeded. Bit 12. 01149 This bit is set to 1 whenever a VCELL register reading is above the maximum VALRT value. 01150 This bit may or may not need to be cleared by system software to detect the next event. 01151 See VS in the CONFIG register. Vmx is set to 0 at power-up. */ 01152 unsigned char tmx : 1; /**< Maximum TALRT Threshold Exceeded Bit 13. 01153 This bit is set to 1 whenever a Temperature register reading is above the maximum TALRT value. 01154 This bit may or may not need to be cleared by system software to detect the next event. 01155 See TS in the CONFIG register. Tmx is set to 0 at power-up. */ 01156 unsigned char smx : 1; /**< Maximum SOCALRT Threshold Exceeded. Bit 14. 01157 This bit is set to 1 whenever SOC rises above the maximum SOCALRT value. 01158 This bit may or may not need to be cleared by system software to detect the next event. 01159 See SS in the CONFIG register and SACFG in the MiscCFG register. Smx is set to 0 at power-up. */ 01160 unsigned char br : 1; /**< Battery Removal. Bit 15. 01161 This bit is set to 1 when the device detects that a battery has been removed from the system. 01162 This bit must be cleared by system software to detect the next removal event. 01163 Br is set to 0 at power-up. */ 01164 } bits; 01165 } reg_status_t; 01166 01167 /** 01168 * @brief VAlrtTh 01169 * 01170 * Address : 0x01 01171 */ 01172 typedef union { 01173 uint16_t raw; 01174 struct 01175 { 01176 unsigned char min_voltage_alrt : 8; /**< Minimum voltage reading. Bit 7:0, 01177 An alert is generated if the VCell register reading falls below this value. 01178 Register type: special Set Min=0x00 to disable. Units of LSB are 20mV. */ 01179 unsigned char max_voltage_alrt : 8; /**< Maximum voltage reading. Bit 15:8. 01180 An alert is generated if the VCell register reading exceeds this value. 01181 Register type: special Set Max=0xFF to disable. Units of LSB are 20mV. */ 01182 } bits; 01183 } reg_valrt_th_t; 01184 01185 /** 01186 * @brief TAlrtTh 01187 * 01188 * Address : 0x02 01189 */ 01190 typedef union { 01191 uint16_t raw; 01192 struct 01193 { 01194 unsigned char min_temp_alrt : 8; /**< Sets an alert threshold for minimum temperature. Bit 7:0. 01195 Register type: special Set Min=0x80 to disable. Units of LSB are 1˚C. */ 01196 unsigned char max_temp_alrt : 8; /**< Sets an alert threshold for maximum temperature. Bit 15:8. 01197 Register type: special Set Max=0x7F to disable. Units of LSB are 1˚C. */ 01198 } bits; 01199 } reg_talrt_th_t; 01200 01201 /** 01202 * @brief SAlrtTh 01203 * 01204 * Address : 0x03 01205 */ 01206 typedef union { 01207 uint16_t raw; 01208 struct 01209 { 01210 unsigned char min_soc_alrt : 8; /**< Sets an alert for minimum SOC. Bit 7:0. 01211 Register type: special This may be used for charge/discharge termination, or 01212 for power-management near empty. Set Min=0x00 to disable. Units of LSB are 1%. */ 01213 unsigned char max_soc_alrt : 8; /**< Sets an alert for maximum SOC. Bit 15:8. 01214 Register type: special This may be used for charge/discharge termination, or 01215 for power-management near empty. Set Max=0xFF to disable. Units of LSB are 1%. */ 01216 } bits; 01217 } reg_salrt_th_t; 01218 01219 /** 01220 * @brief FullSocThr 01221 * 01222 * Address : 0x13 01223 */ 01224 typedef union { 01225 uint16_t raw; 01226 struct 01227 { 01228 uint16_t full_soc_thr : 16; /**< FullSOCThr comes from OTP if the OTP register is enabled. 01229 Otherwise it POR’s to a default of 95%. Bit 15:0. 01230 LSB unit is 1/256%. */ 01231 } bits; 01232 } reg_full_soc_thr_t; 01233 01234 /** 01235 * @brief DesignCap 01236 * 01237 * Address : 0x18 01238 */ 01239 typedef union { 01240 uint16_t raw; 01241 struct 01242 { 01243 uint16_t design_cap : 16; /**< Bit 15:0. 01244 Register type: capacity. */ 01245 } bits; 01246 } reg_design_cap_t; 01247 01248 /** 01249 * @brief Config 01250 * 01251 * Address : 0x1D 01252 */ 01253 typedef union { 01254 uint16_t raw; 01255 struct 01256 { 01257 unsigned char ber : 1; /**< Enable alert on battery removal. Bit 0. 01258 When Ber = 1, a battery-removal condition, as detected by the AIN pin voltage, 01259 triggers an alert. Set to 0 at power-up. */ 01260 unsigned char bei : 1; /**< Enable alert on battery insertion. Bit 1. 01261 When Bei = 1, a battery-insertion condition, as detected by the AIN pin voltage, 01262 triggers an alert. Set to 0 at power-up. */ 01263 unsigned char aen : 1; /**< Enable alert on fuel-gauge outputs. Bit 2. 01264 When Aen = 1, violation of any of the alert threshold register values by temperature, voltage, 01265 or SOC triggers an alert. This bit affects the INTB pin (FG_INT) operation only. 01266 The Smx, Smn, Tmx, Tmn, Vmx, and Vmn bits are not disabled. This bit is set to 0 at power-up.*/ 01267 unsigned char fthrm : 1; /**< Force Thermistor Bias Switch. Bit 3. 01268 Set FTHRM = 1 to always enable the thermistor bias switch. With a standard 10kOhm thermistor, 01269 this adds an additional ~200μA to the current drain of the circuit. 01270 This bit is set to 0 at power-up. */ 01271 unsigned char ethrm : 1; /**< Enable Thermistor. Bit 4. 01272 Set to logic 1 to enable the automatic THRM output bias and AIN measurement every 1.4s. 01273 This bit is set to 1 at power-up. */ 01274 unsigned char spr_5 : 1; /**< I2C Charge Fail Enable. Bit 5. 01275 Set to 1 to enable I2CChgFail interrupt to drive the INTB pin. 01276 This bit is not accessible by the fuel gauge firmware. */ 01277 unsigned char i2csh : 1; /**< I2C Shutdown. Bit 6. 01278 Set to logic 1 to force the device to enter shutdown mode if both SDA and SCL are held low for 01279 more than timeout of the SHDNTIMER register. This also configures the device to wake up on a 01280 rising edge of either SDA or SCL. Set to 1 at power-up. */ 01281 unsigned char shdn : 1; /**< Shutdown. Bit 7. 01282 Write this bit to logic 1 to force a shutdown of the device after timeout of the SHDNTIMER register. 01283 SHDN is reset to 0 at power-up and upon exiting shutdown mode. */ 01284 unsigned char tex : 1; /**< Temperature External. Bit 8. 01285 When set to 1, the fuel gauge requires external temperature measurements to be written from the host. 01286 When set to 0, measurements on the AIN pin are converted to a temperature value and stored 01287 in the Temperature register instead. */ 01288 unsigned char ten : 1; /**< Enable Temperature Channel. Bit 9. 01289 Set to 1 and set ETHRM or FTHRM to 1 to enable measurements on the AIN pin. 01290 Ten is set to 1 at power-up. */ 01291 unsigned char ainsh : 1; /**< AIN Pin Shutdown. Bit 10. 01292 Set to 1 to enable device shutdown when the battery is removed. 01293 The IC enters shutdown if the AIN pin remains high (AIN reading > VTHRM - VDETR) for longer than 01294 the timeout of the SHDNTIMER register. This also configures the device to wake up when AIN is pulled 01295 low on cell insertion. AINSH is set to 0 at power-up. */ 01296 unsigned char spr_11 : 1; /**< Fuel Gauge Charger Control. Bit 11. 01297 Set to 1 to enable MaxCharge (fuel-gauge controlled charging). */ 01298 unsigned char vs : 1; /**< Voltage ALRT Sticky. Bit 12. 01299 When VS = 1, voltage alerts can only be cleared through software. 01300 When VS = 0, voltage alerts are cleared automatically when the threshold is no longer exceeded. 01301 VS is set to 0 at power-up. */ 01302 unsigned char ts : 1; /**< Temperature ALRT Sticky. Bit 13. 01303 When TS = 1, temperature alerts can only be cleared through software. 01304 When TS = 0, temperature alerts are cleared automatically when the threshold is no longer exceeded. 01305 TS is set to 1 at power-up. */ 01306 unsigned char ss : 1; /**< SOC ALRT Sticky. Bit 14. 01307 When SS = 1, SOC alerts can only be cleared through software. 01308 When SS = 0, SOC alerts are cleared automatically when the threshold is no longer exceeded. 01309 SS is set to 0 at power-up. */ 01310 unsigned char spr_15 : 1; /**< Fuel gauge Charger Fail Enable. Bit 15. 01311 Set to 1 to enable FGCHGFAIL interrupt to drive the INTB pin. 01312 This bit is not accessible by the fuel gauge firmware. */ 01313 } bits; 01314 } reg_config_t; 01315 01316 /** 01317 * @brief IChgTerm 01318 * 01319 * Address : 0x1E 01320 */ 01321 typedef union { 01322 uint16_t raw; 01323 struct 01324 { 01325 uint16_t ichg_term : 16; /**< Program IChgTerm to the exact charge termination current used in the application. Bit 15:0. 01326 Register type: current. */ 01327 } bits; 01328 } reg_ichg_term_t; 01329 01330 /** 01331 * @brief DevName 01332 * 01333 * Address : 0x21 01334 */ 01335 typedef union { 01336 uint16_t raw; 01337 struct 01338 { 01339 uint16_t dev_name : 16; /**< holds revision information. Bit 15:0. */ 01340 } bits; 01341 } reg_dev_name_t; 01342 01343 /** 01344 * @brief FilterCfg 01345 * Use of unsigned char resulted in error in register bit field values. 01346 * Thus, uint16_t is here used to define the size of bit fields. 01347 * Address : 0x29 01348 */ 01349 typedef union { 01350 uint16_t raw; 01351 struct 01352 { 01353 uint16_t ncurr : 4; /**< Sets the time constant for the AverageCurrent register. Bit 3:0. 01354 The default POR value of 4h gives a time constant of 11.25 seconds. 01355 The equation setting the period is:AverageCurrent time constant = 175.8ms × 2^(2+NCURR).*/ 01356 uint16_t navgcell : 3; /**< Sets the time constant for the AverageVCELL register. Bit 6:4. 01357 The default POR value of 2h gives a time constant of 45.0s. 01358 The equation setting the period is:AverageVCELL time constant = 175.8ms × 2(6+NAVGVCELL). */ 01359 uint16_t nmix : 4; /**< Sets the time constant for the mixing algorithm. Bit 10:7. 01360 The default POR value of Dh gives a time constant of 12.8 hours = 46080s. 01361 The equation setting the period is:Mixing Period = 175.8ms × 2(5+NMIX). */ 01362 uint16_t rsvd : 3; /**< Bit 13:11. */ 01363 uint16_t nempty : 2; /**< Sets the filtering for empty learning for both the I_Avgempty and QRTable registers. Bit 15:14. 01364 Iavg_empty is learned with(NEMPTY+3) right shifts. 01365 QRTable is learned with (NEMPTY + sizeof(Iavgempty) – sizeof (AvgCurrent)) right shifts. */ 01366 } bits; 01367 } reg_filter_cfg_t; 01368 01369 /** 01370 * @brief IAvgEmpty 01371 * 01372 * Address : 0x36 01373 */ 01374 typedef union { 01375 uint16_t raw; 01376 struct 01377 { 01378 uint16_t rsvd : 16; /**< Register type: current. Bit 15:0. */ 01379 } bits; 01380 } reg_iavg_empty_t; 01381 01382 /** 01383 * @brief VEmpty 01384 * 01385 * Address : 0x3A 01386 */ 01387 typedef union { 01388 uint16_t raw; 01389 struct 01390 { 01391 unsigned char v_recover : 7; /**< Recovery Voltage. Sets the voltage level for clearing empty detection. Bit 6:0. 01392 Once the cell voltage rises above this point, empty voltage detection is reenabled. 01393 A 40mV resolution gives a 0 to 5.08V range. This value is written to 3.68V at power-up. */ 01394 uint16_t v_empty : 9; /**< Empty Voltage. Sets the voltage level for detecting empty. Bit 15:7. 01395 A 10mV resolution gives a 0 to 5.11V range. This value is written to 3.12V at power-up.*/ 01396 } bits; 01397 } reg_v_empty_t; 01398 01399 /** 01400 * @brief Config2 01401 * 01402 * Address : 0xBB 01403 */ 01404 typedef union { 01405 uint16_t raw; 01406 struct 01407 { 01408 unsigned char isys_ncurr : 4; /**< Sets the time constant for the AvgISys register. Bit 3:0. 01409 The default POR value of 0100b gives a time constant of 5.625. 01410 The equation setting the period is: AvgISys time constant = 45s x 2(ISysNCurr-7).*/ 01411 unsigned char qcvqen : 1; /**< Set OCVQen=1 to enable automatic empty compensation based on VFOCV information. Bit 4. */ 01412 unsigned char ldmdl : 1; /**< Host sets this bit to 1 in order to initiate firmware to finish processing a newly loaded model. Bit 5. 01413 Firmware clears this bit to zero to indicate that model loading is finished. */ 01414 unsigned char tairt_en : 1; /**< Set to 1 to enable temperature alert. Bit 6. 01415 If TAlrtEn=0, the alert on is disabled. */ 01416 unsigned char dsocen : 1; /**< Set to 1 to enable SOC 1% change alert. Bit 7. 01417 If dSCOCen=0, the alert on is disabled.*/ 01418 unsigned char thm_hotairt_en : 1; /**< Set to 1 to enable ThmHotAlrtEn alert. Bit 8. 01419 If ThmHotAlrtEn = 0, the alert on is disabled.*/ 01420 unsigned char thmhot_en : 1; /**< Set to 1 to enable ThmHot function by comparing Voltage/Temp condition with THMHOT (40h). Bit 9. 01421 ThmHot function is FG Charger input current limit control. */ 01422 unsigned char fc_thmhot : 1; /**< Set to 1 to enable thermistor hot forcedly, regardless of actually sense thermistor temperature. Bit 10. */ 01423 unsigned char spr : 5; /**< Bit 15:11. */ 01424 } bits; 01425 } reg_config2_t; 01426 01427 /** 01428 * @brief Temp 01429 * 01430 * Address : 0x08 01431 */ 01432 typedef union { 01433 uint16_t raw; 01434 struct 01435 { 01436 uint16_t temp : 16; /**< This is the most recent trimmed temperature measurement. Bit 15:0. 01437 Temperature is measured every 1.4 seconds. Register type: temperature.*/ 01438 } bits; 01439 } reg_temp_t; 01440 01441 /** 01442 * @brief Vcell 01443 * 01444 * Address : 0x09 01445 */ 01446 typedef union { 01447 uint16_t raw; 01448 struct 01449 { 01450 uint16_t vcell : 16; /**< This is the most recent trimmed cell voltage result. Bit 15:0. 01451 It represents an FIR average of raw results. 01452 The VOLT_Raw is sampled every 175.8ms and gain and offset trim are applied to calculate VCELL. 01453 Register type: voltage.*/ 01454 } bits; 01455 } reg_vcell_t; 01456 01457 /** 01458 * @brief Current 01459 * 01460 * Address : 0x0A 01461 */ 01462 typedef union { 01463 uint16_t raw; 01464 struct 01465 { 01466 uint16_t current : 16; /**< Register type: current. Bit 15:0. */ 01467 } bits; 01468 } reg_current_t; 01469 01470 /** 01471 * @brief AvgCurrent 01472 * 01473 * Address : 0x0B 01474 */ 01475 typedef union { 01476 uint16_t raw; 01477 struct 01478 { 01479 uint16_t avg_current : 16; /**< This is the 0.7s to 6.4hr (configurable) IIR average of the current. Bit 15:0. 01480 This register represents the upper 16 bits of the 32-bit shift register that filters current. 01481 The average should be set equal to Current upon startup. 01482 Register type: current. */ 01483 } bits; 01484 } reg_avg_current_t; 01485 01486 /** 01487 * @brief AvgTA 01488 * 01489 * Address : 0x16 01490 */ 01491 typedef union { 01492 uint16_t raw; 01493 struct 01494 { 01495 uint16_t avg_ta : 16; /**< This is the 6min to 12hr (configurable) IIR average of the Temperature. Bit 15:0. 01496 The average is set equal to Temp upon startup. Register type: temperature. */ 01497 } bits; 01498 } reg_avg_ta_t; 01499 01500 /** 01501 * @brief AvgVCell 01502 * 01503 * Address : 0x19 01504 */ 01505 typedef union { 01506 uint16_t raw; 01507 struct 01508 { 01509 uint16_t avg_vcell : 16; /**< This reports the 12s to 24min (configurable) IIR average of VCELL. Bit 15:0. 01510 The average is set equal to VCELL at startup. Register type: voltage. */ 01511 } bits; 01512 } reg_avg_vcell_t; 01513 01514 /** 01515 * @brief MaxMinTemp 01516 * 01517 * Address : 0x1A 01518 */ 01519 typedef union { 01520 uint16_t raw; 01521 struct 01522 { 01523 unsigned char min_temp : 8; /**< Records the minimum Temperature. Bit 7:0. 01524 Register type: special Two's complement 8-bit value with 1°C resolution. */ 01525 unsigned char max_temp : 8; /**< Records the maximum Temperature. Bit 15:8. 01526 Register type: special Two's complement 8-bit value with 1°C resolution. */ 01527 } bits; 01528 } reg_max_min_temp_t; 01529 01530 /** 01531 * @brief MaxMinVolt 01532 * 01533 * Address : 0x1B 01534 */ 01535 typedef union { 01536 uint16_t raw; 01537 struct 01538 { 01539 unsigned char min_volt : 8; /**< Records the VCELL minimum voltage. Bit 7:0. 01540 Register type: The maximum and minimum voltages are each stored as 8-bit values with a 20mV resolution. */ 01541 unsigned char max_volt : 8; /**< Records the VCELL maximum voltage. Bit 15:8. 01542 Register type: The maximum and minimum voltages are each stored as 8-bit values with a 20mV resolution. */ 01543 } bits; 01544 } reg_max_min_volt_t; 01545 01546 /** 01547 * @brief MaxMinCurr 01548 * 01549 * Address : 0x1C 01550 */ 01551 typedef union { 01552 uint16_t raw; 01553 struct 01554 { 01555 unsigned char min_charge_curr : 8; /**< Records the minimum charge current. Bit 7:0. 01556 Register type: Two's complement 8-bit values with 0.4mV/RSENSE resolution. */ 01557 unsigned char max_charge_curr : 8; /**< Records the maximum charge current. Bit 15:8. 01558 Register type: Two's complement 8-bit values with 0.4mV/RSENSE resolution. */ 01559 } bits; 01560 } reg_max_min_curr_t; 01561 01562 /** 01563 * @brief AIN0 01564 * 01565 * Address : 0x27 01566 */ 01567 typedef union { 01568 uint16_t raw; 01569 struct 01570 { 01571 uint16_t ain0 : 16; /**< The TGain, TOff, and Curve register values are then applied to this ratio-metric reading to 01572 convert the result to temperature. Bit 15:0. 01573 Register type: special The MAX77658 stores the result as a ratio-metric value 01574 from 0% to 100% in the AIN register with an LSB of 0.0122%. */ 01575 } bits; 01576 } reg_ain0_t; 01577 01578 /** 01579 * @brief Timer 01580 * 01581 * Address : 0x3E 01582 */ 01583 typedef union { 01584 uint16_t raw; 01585 struct 01586 { 01587 uint16_t timer : 16; /**< Timer increments once every task period. Bit 15:0. 01588 With default TaskPeriod, timer has units 0.1758 seconds. 01589 Register type: special The Timer register LSB is 175.8ms, giving a full-scale range of 0 to 3.2 hours. */ 01590 } bits; 01591 } reg_timer_t; 01592 01593 /** 01594 * @brief ShdnTimer 01595 * 01596 * Address : 0x3F 01597 */ 01598 typedef union { 01599 uint16_t raw; 01600 struct 01601 { 01602 uint16_t shdnctr : 13; /**< Shutdown Counter. Bit 12:0. 01603 This register counts the total amount of elapsed time since the shutdown trigger event. 01604 Register type: special. This counter value stops and resets to 0 when the shutdown timeout completes. 01605 The counter LSB is 1.4s. */ 01606 unsigned char shdn_thr : 3; /**< Sets the shutdown timeout period from a minimum of 45s to a maximum of 1.6h. Bit 15:13. 01607 The default POR value of 7h gives a shutdown delay of 1.6h. 01608 Register type: special The equation setting the period is: Shutdown Timeout Period = 175.8ms × 2(8+THR). */ 01609 } bits; 01610 } reg_shdn_timer_t; 01611 01612 /** 01613 * @brief TimerH 01614 * 01615 * Address : 0xBE 01616 */ 01617 typedef union { 01618 uint16_t raw; 01619 struct 01620 { 01621 uint16_t timerh : 16; /**< TIMERH is a 16-bit high-word extension to the TIMER register. Bit 15:0. 01622 This extension allows time counting up to 24 years. This register can be enabled in the save & restore registers. 01623 Register type: special A 3.2-hour LSb gives a full-scale range for the register of up to 23.94 years. */ 01624 } bits; 01625 } reg_timerh_t; 01626 01627 /** 01628 * @brief RepCap 01629 * 01630 * Address : 0x05 01631 */ 01632 typedef union { 01633 uint16_t raw; 01634 struct 01635 { 01636 uint16_t rep_cap : 16; /**< RepCap or reported capacity. Bit 15:0. 01637 A filtered version of the AvCap register that prevents large jumps in the reported 01638 value caused by changes in the application such as abrupt changes in tempreature or 01639 load current. Register type: capacity. */ 01640 } bits; 01641 } reg_rep_cap_t; 01642 01643 /** 01644 * @brief RepSOC 01645 * 01646 * Address : 0x06 01647 */ 01648 typedef union { 01649 uint16_t raw; 01650 struct 01651 { 01652 uint16_t rep_soc : 16; /**< RepSOC is the complete calculation for State of Charge. Bit 15:0. 01653 This includes all processing, including: ModelGauge Mixing, Empty Compensation. 01654 Register type: percentage. */ 01655 } bits; 01656 } reg_rep_soc_t; 01657 01658 /** 01659 * @brief AvSOC 01660 * 01661 * Address : 0x0E 01662 */ 01663 typedef union { 01664 uint16_t raw; 01665 struct 01666 { 01667 uint16_t av_soc : 16; /**< This register provides unfiltered results. Bit 15:0. 01668 Jumps in the reported values can be caused by abrupt changes in load current or temperature. 01669 See the Empty Compensation section for details. 01670 This includes all processing, including: ModelGauge Mixing, Empty Compensation. 01671 Register type: percentage. */ 01672 } bits; 01673 } reg_av_soc_t; 01674 01675 /** 01676 * @brief FullCapRep 01677 * 01678 * Address : 0x10 01679 */ 01680 typedef union { 01681 uint16_t raw; 01682 struct 01683 { 01684 uint16_t full_cap_rep : 16; /**< Most applications should only monitor FullCapRep, instead of FullCap or FullCapNom. Bit 15:0. 01685 A new full-capacity value is calculated at the end of every charge cycle in the application. 01686 Register type: capacity. */ 01687 } bits; 01688 } reg_full_cap_rep_t; 01689 01690 /** 01691 * @brief TTE 01692 * 01693 * Address : 0x11 01694 */ 01695 typedef union { 01696 uint16_t raw; 01697 struct 01698 { 01699 uint16_t tte : 16; /**< The TTE value is determined by relating AvCap with AvgCurrent. Bit 15:0. 01700 The corresponding AvgCurrent filtering gives a delay in TTE but provides more stable results. 01701 Register type: time. */ 01702 } bits; 01703 } reg_tte_t; 01704 01705 /** 01706 * @brief RCell 01707 * 01708 * Address : 0x14 01709 */ 01710 typedef union { 01711 uint16_t raw; 01712 struct 01713 { 01714 uint16_t rcell : 16; /**< This register provides the calculated internal resistance of the cell. Bit 15:0. 01715 RCell is determined by comparing open-circuit voltage (VFOCV) against measured voltage (VCell) 01716 over a long time period while under load or charge current. 01717 Register type: resistance. */ 01718 } bits; 01719 } reg_rcell_t; 01720 01721 /** 01722 * @brief Cycles 01723 * 01724 * Address : 0x17 01725 */ 01726 typedef union { 01727 uint16_t raw; 01728 struct 01729 { 01730 uint16_t cycles : 16; /**< This register provides the calculated internal resistance of the cell. Bit 15:0. 01731 RCell is determined by comparing open-circuit voltage (VFOCV) against measured voltage (VCell) 01732 over a long time period while under load or charge current. 01733 Register type: resistance. */ 01734 } bits; 01735 } reg_cycles_t; 01736 01737 /** 01738 * @brief AvCap 01739 * 01740 * Address : 0x1F 01741 */ 01742 typedef union { 01743 uint16_t raw; 01744 struct 01745 { 01746 uint16_t av_cap : 16; /**< This is the remaining capacity with coulomb-counter + Voltage-Fuel-Gauge mixing, 01747 after accounting for capacity that is unavailable due to the discharge rate. Bit 15:0. 01748 Register type: capacity. */ 01749 } bits; 01750 } reg_av_cap_t; 01751 01752 /** 01753 * @brief TTF 01754 * 01755 * Address : 0x20 01756 */ 01757 typedef union { 01758 uint16_t raw; 01759 struct 01760 { 01761 uint16_t ttf : 16; /**< The TTF value is determined by learning the constant current and 01762 constant voltage portions of the charge cycle based on experience of prior charge cycles. Bit 15:0. 01763 Register type: time. */ 01764 } bits; 01765 } reg_ttf_t; 01766 01767 #endif /* MAX77658_REGS_H_ */
Generated on Fri Aug 26 2022 12:03:39 by
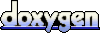