MAX77658 Ultra-Low Power PMIC Mbed Driver
Embed:
(wiki syntax)
Show/hide line numbers
MAX77658.cpp
00001 /******************************************************************************* 00002 * Copyright(C) Analog Devices Inc., All Rights Reserved. 00003 * 00004 * Permission is hereby granted, free of charge, to any person obtaining a 00005 * copy of this software and associated documentation files(the "Software"), 00006 * to deal in the Software without restriction, including without limitation 00007 * the rights to use, copy, modify, merge, publish, distribute, sublicense, 00008 * and/or sell copies of the Software, and to permit persons to whom the 00009 * Software is furnished to do so, subject to the following conditions: 00010 * 00011 * The above copyright notice and this permission notice shall be included 00012 * in all copies or substantial portions of the Software. 00013 * 00014 * THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS 00015 * OR IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF 00016 * MERCHANTABILITY, FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. 00017 * IN NO EVENT SHALL MAXIM INTEGRATED BE LIABLE FOR ANY CLAIM, DAMAGES 00018 * OR OTHER LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, 00019 * ARISING FROM, OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR 00020 * OTHER DEALINGS IN THE SOFTWARE. 00021 * 00022 * Except as contained in this notice, the name of Analog Devices Inc. 00023 * shall not be used except as stated in the Analog Devices Inc. 00024 * Branding Policy. 00025 * 00026 * The mere transfer of this software does not imply any licenses 00027 * of trade secrets, proprietary technology, copyrights, patents, 00028 * trademarks, maskwork rights, or any other form of intellectual 00029 * property whatsoever. Analog Devices Inc.retains all ownership rights. 00030 ******************************************************************************* 00031 */ 00032 00033 #include <Thread.h> 00034 #include "MAX77658.h" 00035 #include <math.h> 00036 00037 #define POST_INTR_WORK_SIGNAL_ID 0x1 00038 #define TO_UINT8 0xFF 00039 #define TO_UINT16 0xFFFF 00040 00041 MAX77658::MAX77658(I2C *i2c, PinName IRQPin) 00042 { 00043 if (i2c == NULL) 00044 return; 00045 00046 i2c_handler = i2c; 00047 00048 interrupt_handler_list = new handler[INT_CHG_END] {}; 00049 00050 if (IRQPin != NC) { 00051 irq_disable_all(); 00052 post_intr_work_thread = new Thread(); 00053 post_intr_work_thread->start(Callback<void()>(this, &MAX77658::post_interrupt_work)); 00054 00055 this->irq_pin = new InterruptIn(IRQPin); 00056 this->irq_pin->fall(Callback<void()>(this, &MAX77658::interrupt_handler)); 00057 this->irq_pin->enable_irq(); 00058 } else { 00059 this->irq_pin = NULL; 00060 } 00061 } 00062 00063 MAX77658::~MAX77658() 00064 { 00065 if (post_intr_work_thread) 00066 delete post_intr_work_thread; 00067 00068 if (irq_pin) 00069 delete irq_pin; 00070 00071 if (interrupt_handler_list) 00072 delete [] interrupt_handler_list; 00073 } 00074 00075 int MAX77658::read_register(uint8_t reg, uint8_t *value) 00076 { 00077 int rtn_val; 00078 00079 if (value == NULL) 00080 return MAX77658_VALUE_NULL; 00081 00082 rtn_val = i2c_handler->write(MAX77658_I2C_ADDRESS_PMIC_1, (const char *)®, 1, true); 00083 if (rtn_val != 0) 00084 return MAX77658_WRITE_DATA_FAILED; 00085 00086 rtn_val = i2c_handler->read(MAX77658_I2C_ADDRESS_PMIC_1, (char *) value, 1, false); 00087 if (rtn_val < 0) 00088 return MAX77658_READ_DATA_FAILED; 00089 00090 return MAX77658_NO_ERROR; 00091 } 00092 00093 int MAX77658::write_register(uint8_t reg, const uint8_t *value) 00094 { 00095 int rtn_val; 00096 unsigned char local_data[2]; 00097 00098 if (value == NULL) 00099 return MAX77658_VALUE_NULL; 00100 00101 local_data[0] = reg; 00102 00103 memcpy(&local_data[1], value, 1); 00104 00105 rtn_val = i2c_handler->write(MAX77658_I2C_ADDRESS_PMIC_1, (const char *)local_data, sizeof(local_data)); 00106 if (rtn_val != MAX77658_NO_ERROR) 00107 return MAX77658_WRITE_DATA_FAILED; 00108 00109 return MAX77658_NO_ERROR; 00110 } 00111 00112 int MAX77658::read_fg_register(uint8_t reg, uint8_t *value) 00113 { 00114 int rtn_val; 00115 00116 if (value == NULL) 00117 return MAX77658_VALUE_NULL; 00118 00119 rtn_val = i2c_handler->write(MAX77658_I2C_ADDRESS_FG, (const char *)®, 1, true); 00120 if (rtn_val != 0) 00121 return MAX77658_WRITE_DATA_FAILED; 00122 00123 rtn_val = i2c_handler->read(MAX77658_I2C_ADDRESS_FG, (char *) value, 2, false); 00124 if (rtn_val < 0) 00125 return MAX77658_READ_DATA_FAILED; 00126 00127 return MAX77658_NO_ERROR; 00128 } 00129 00130 int MAX77658::write_fg_register(uint8_t reg, const uint8_t *value) 00131 { 00132 int rtn_val; 00133 unsigned char local_data[3]; 00134 00135 if (value == NULL) 00136 return MAX77658_VALUE_NULL; 00137 00138 local_data[0] = reg; 00139 00140 memcpy(&local_data[1], value, 2); 00141 00142 rtn_val = i2c_handler->write(MAX77658_I2C_ADDRESS_FG, (const char *)local_data, sizeof(local_data)); 00143 if (rtn_val != MAX77658_NO_ERROR) 00144 return MAX77658_WRITE_DATA_FAILED; 00145 00146 return MAX77658_NO_ERROR; 00147 } 00148 00149 #define SET_BIT_FIELD(address, reg_name, bit_field_name, value) \ 00150 int ret_val; \ 00151 ret_val = read_register(address, (uint8_t *)&(reg_name)); \ 00152 if (ret_val) { \ 00153 return ret_val; \ 00154 } \ 00155 bit_field_name = value; \ 00156 ret_val = write_register(address, (uint8_t *)&(reg_name)); \ 00157 if (ret_val) { \ 00158 return ret_val; \ 00159 } 00160 00161 00162 00163 #define SET_FG_BIT_FIELD(address, reg_name, bit_field_name, value) \ 00164 int ret_val_fg; \ 00165 ret_val_fg = read_fg_register(address, (uint8_t *)&(reg_name)); \ 00166 if (ret_val_fg) { \ 00167 return ret_val_fg; \ 00168 } \ 00169 bit_field_name = value; \ 00170 ret_val_fg = write_fg_register(address, (uint8_t *)&(reg_name)); \ 00171 if (ret_val_fg) { \ 00172 return ret_val_fg; \ 00173 } 00174 00175 int MAX77658::get_ercflag(reg_bit_ercflag_t bit_field, uint8_t *flag) 00176 { 00177 int ret; 00178 reg_ercflag_t reg_ercflag = {0}; 00179 00180 ret = read_register(ERCFLAG, (uint8_t *)&(reg_ercflag)); 00181 if (ret != MAX77658_NO_ERROR) return ret; 00182 00183 switch (bit_field) 00184 { 00185 case ERCFLAG_TOVLD: 00186 *flag = (uint8_t)reg_ercflag.bits.tovld; 00187 break; 00188 case ERCFLAG_SYSOVLO: 00189 *flag = (uint8_t)reg_ercflag.bits.sysovlo; 00190 break; 00191 case ERCFLAG_SYSUVLO: 00192 *flag = (uint8_t)reg_ercflag.bits.sysuvlo; 00193 break; 00194 case ERCFLAG_MRST_F: 00195 *flag = (uint8_t)reg_ercflag.bits.mrst_f; 00196 break; 00197 case ERCFLAG_SFT_OFF_F: 00198 *flag = (uint8_t)reg_ercflag.bits.sft_off_f; 00199 break; 00200 case ERCFLAG_SFT_CRST_F: 00201 *flag = (uint8_t)reg_ercflag.bits.sft_crst_f; 00202 break; 00203 case ERCFLAG_WDT_OFF: 00204 *flag = (uint8_t)reg_ercflag.bits.wdt_off; 00205 break; 00206 case ERCFLAG_WDT_RST: 00207 *flag = (uint8_t)reg_ercflag.bits.wdt_rst; 00208 break; 00209 default: 00210 ret = MAX77658_INVALID_DATA; 00211 break; 00212 } 00213 00214 return ret; 00215 } 00216 00217 int MAX77658::get_stat_glbl(reg_bit_stat_glbl_t bit_field, uint8_t *status) 00218 { 00219 int ret; 00220 reg_stat_glbl_t reg_stat_glbl = {0}; 00221 00222 ret = read_register(STAT_GLBL, (uint8_t *)&(reg_stat_glbl)); 00223 if (ret != MAX77658_NO_ERROR) return ret; 00224 00225 switch (bit_field) 00226 { 00227 case STAT_GLBL_STAT_IRQ: 00228 *status = (uint8_t)reg_stat_glbl.bits.stat_irq; 00229 break; 00230 case STAT_GLBL_STAT_EN: 00231 *status = (uint8_t)reg_stat_glbl.bits.stat_en; 00232 break; 00233 case STAT_GLBL_TJAL1_S: 00234 *status = (uint8_t)reg_stat_glbl.bits.tjal1_s; 00235 break; 00236 case STAT_GLBL_TJAL2_S: 00237 *status = (uint8_t)reg_stat_glbl.bits.tjal2_s; 00238 break; 00239 case STAT_GLBL_DOD1_S: 00240 *status = (uint8_t)reg_stat_glbl.bits.dod1_s; 00241 break; 00242 case STAT_GLBL_DOD0_S: 00243 *status = (uint8_t)reg_stat_glbl.bits.dod0_s; 00244 break; 00245 case STAT_GLBL_BOK: 00246 *status = (uint8_t)reg_stat_glbl.bits.bok; 00247 break; 00248 case STAT_GLBL_DIDM: 00249 *status = (uint8_t)reg_stat_glbl.bits.didm; 00250 break; 00251 default: 00252 ret = MAX77658_INVALID_DATA; 00253 break; 00254 } 00255 00256 return ret; 00257 } 00258 00259 int MAX77658::set_interrupt_mask(reg_bit_int_mask_t bit_field, uint8_t maskBit) 00260 { 00261 int ret; 00262 uint8_t reg_addr; 00263 reg_int_m_chg_t reg_int_m_chg = {0}; 00264 reg_intm_glbl0_t reg_intm_glbl0 = {0}; 00265 reg_intm_glbl1_t reg_intm_glbl1 = {0}; 00266 00267 //INT_M_CHG (0x07), INTM_GLBL0 (0x08) and INTM_GLBL1 (0x09) 00268 reg_addr = (uint8_t)floor((static_cast<uint8_t>(bit_field)) / 8) + 0x07; 00269 00270 if (reg_addr == INT_M_CHG) 00271 ret = read_register(INT_M_CHG, (uint8_t *)&(reg_int_m_chg)); 00272 else if (reg_addr == INTM_GLBL0) 00273 ret = read_register(INTM_GLBL0, (uint8_t *)&(reg_intm_glbl0)); 00274 else if (reg_addr == INTM_GLBL1) 00275 ret = read_register(INTM_GLBL1, (uint8_t *)&(reg_intm_glbl1)); 00276 else 00277 return MAX77658_INVALID_DATA; 00278 00279 if (ret != MAX77658_NO_ERROR) return ret; 00280 00281 switch (bit_field) 00282 { 00283 case INT_M_CHG_THM_M: 00284 reg_int_m_chg.bits.thm_m = maskBit; 00285 break; 00286 case INT_M_CHG_CHG_M: 00287 reg_int_m_chg.bits.chg_m = maskBit; 00288 break; 00289 case INT_M_CHG_CHGIN_M: 00290 reg_int_m_chg.bits.chgin_m = maskBit; 00291 break; 00292 case INT_M_CHG_TJ_REG_M: 00293 reg_int_m_chg.bits.tj_reg_m = maskBit; 00294 break; 00295 case INT_M_CHG_CHGIN_CTRL_M: 00296 reg_int_m_chg.bits.chgin_ctrl_m = maskBit; 00297 break; 00298 case INT_M_CHG_SYS_CTRL_M: 00299 reg_int_m_chg.bits.sys_ctrl_m = maskBit; 00300 break; 00301 case INT_M_CHG_SYS_CNFG_M: 00302 reg_int_m_chg.bits.sys_cnfg_m = maskBit; 00303 break; 00304 case INT_M_CHG_DIS_AICL: 00305 reg_int_m_chg.bits.dis_aicl = maskBit; 00306 break; 00307 case INTM_GLBL0_GPI0_FM: 00308 reg_intm_glbl0.bits.gpi0_fm = maskBit; 00309 break; 00310 case INTM_GLBL0_GPI0_RM: 00311 reg_intm_glbl0.bits.gpi0_rm = maskBit; 00312 break; 00313 case INTM_GLBL0_nEN_FM: 00314 reg_intm_glbl0.bits.nen_fm = maskBit; 00315 break; 00316 case INTM_GLBL0_nEN_RM: 00317 reg_intm_glbl0.bits.nen_rm = maskBit; 00318 break; 00319 case INTM_GLBL0_TJAL1_RM: 00320 reg_intm_glbl0.bits.tjal1_rm = maskBit; 00321 break; 00322 case INTM_GLBL0_TJAL2_RM: 00323 reg_intm_glbl0.bits.tjal2_rm = maskBit; 00324 break; 00325 case INTM_GLBL0_DOD1_RM: 00326 reg_intm_glbl0.bits.dod1_rm = maskBit; 00327 break; 00328 case INTM_GLBL0_DOD0_RM: 00329 reg_intm_glbl0.bits.dod0_rm = maskBit; 00330 break; 00331 case INTM_GLBL1_GPI1_FM: 00332 reg_intm_glbl1.bits.gpi1_fm = maskBit; 00333 break; 00334 case INTM_GLBL1_GPI1_RM: 00335 reg_intm_glbl1.bits.gpi1_rm = maskBit; 00336 break; 00337 case INTM_GLBL1_SBB0_FM: 00338 reg_intm_glbl1.bits.sbb0_fm = maskBit; 00339 break; 00340 case INTM_GLBL1_SBB1_FM: 00341 reg_intm_glbl1.bits.sbb1_fm = maskBit; 00342 break; 00343 case INTM_GLBL1_SBB2_FM: 00344 reg_intm_glbl1.bits.sbb2_fm = maskBit; 00345 break; 00346 case INTM_GLBL1_LDO0_M: 00347 reg_intm_glbl1.bits.ldo0_m = maskBit; 00348 break; 00349 case INTM_GLBL1_LDO1_M: 00350 reg_intm_glbl1.bits.ldo1_m = maskBit; 00351 break; 00352 case INTM_GLBL1_RSVD: 00353 reg_intm_glbl1.bits.rsvd = maskBit; 00354 break; 00355 default: 00356 return MAX77658_INVALID_DATA; 00357 break; 00358 } 00359 00360 if (reg_addr == INT_M_CHG) 00361 return write_register(INT_M_CHG, (uint8_t *)&(reg_int_m_chg)); 00362 else if (reg_addr == INTM_GLBL0) 00363 return write_register(INTM_GLBL0, (uint8_t *)&(reg_intm_glbl0)); 00364 else if (reg_addr == INTM_GLBL1) 00365 return write_register(INTM_GLBL1, (uint8_t *)&(reg_intm_glbl1)); 00366 } 00367 00368 int MAX77658::get_interrupt_mask(reg_bit_int_mask_t bit_field, uint8_t *maskBit) 00369 { 00370 int ret; 00371 uint8_t reg_addr; 00372 reg_int_m_chg_t reg_int_m_chg = {0}; 00373 reg_intm_glbl0_t reg_intm_glbl0 = {0}; 00374 reg_intm_glbl1_t reg_intm_glbl1 = {0}; 00375 00376 //INT_M_CHG (0x07), INTM_GLBL0 (0x08) and INTM_GLBL1 (0x09) 00377 reg_addr = (uint8_t)floor((static_cast<uint8_t>(bit_field)) / 8) + 0x07; 00378 00379 if (reg_addr == INT_M_CHG) 00380 ret = read_register(INT_M_CHG, (uint8_t *)&(reg_int_m_chg)); 00381 else if (reg_addr == INTM_GLBL0) 00382 ret = read_register(INTM_GLBL0, (uint8_t *)&(reg_intm_glbl0)); 00383 else if (reg_addr == INTM_GLBL1) 00384 ret = read_register(INTM_GLBL1, (uint8_t *)&(reg_intm_glbl1)); 00385 else 00386 return MAX77658_INVALID_DATA; 00387 00388 if (ret != MAX77658_NO_ERROR) return ret; 00389 00390 switch (bit_field) 00391 { 00392 case INT_M_CHG_THM_M: 00393 *maskBit = (uint8_t)reg_int_m_chg.bits.thm_m; 00394 break; 00395 case INT_M_CHG_CHG_M: 00396 *maskBit = (uint8_t)reg_int_m_chg.bits.chg_m; 00397 break; 00398 case INT_M_CHG_CHGIN_M: 00399 *maskBit = (uint8_t)reg_int_m_chg.bits.chgin_m; 00400 break; 00401 case INT_M_CHG_TJ_REG_M: 00402 *maskBit = (uint8_t)reg_int_m_chg.bits.tj_reg_m; 00403 break; 00404 case INT_M_CHG_CHGIN_CTRL_M: 00405 *maskBit = (uint8_t)reg_int_m_chg.bits.chgin_ctrl_m; 00406 break; 00407 case INT_M_CHG_SYS_CTRL_M: 00408 *maskBit = (uint8_t)reg_int_m_chg.bits.sys_ctrl_m; 00409 break; 00410 case INT_M_CHG_SYS_CNFG_M: 00411 *maskBit = (uint8_t)reg_int_m_chg.bits.sys_cnfg_m; 00412 break; 00413 case INT_M_CHG_DIS_AICL: 00414 *maskBit = (uint8_t)reg_int_m_chg.bits.dis_aicl; 00415 break; 00416 case INTM_GLBL0_GPI0_FM: 00417 *maskBit = (uint8_t)reg_intm_glbl0.bits.gpi0_fm; 00418 break; 00419 case INTM_GLBL0_GPI0_RM: 00420 *maskBit = (uint8_t)reg_intm_glbl0.bits.gpi0_rm; 00421 break; 00422 case INTM_GLBL0_nEN_FM: 00423 *maskBit = (uint8_t)reg_intm_glbl0.bits.nen_fm; 00424 break; 00425 case INTM_GLBL0_nEN_RM: 00426 *maskBit = (uint8_t)reg_intm_glbl0.bits.nen_rm; 00427 break; 00428 case INTM_GLBL0_TJAL1_RM: 00429 *maskBit = (uint8_t)reg_intm_glbl0.bits.tjal1_rm; 00430 break; 00431 case INTM_GLBL0_TJAL2_RM: 00432 *maskBit = (uint8_t)reg_intm_glbl0.bits.tjal2_rm; 00433 break; 00434 case INTM_GLBL0_DOD1_RM: 00435 *maskBit = (uint8_t)reg_intm_glbl0.bits.dod1_rm; 00436 break; 00437 case INTM_GLBL0_DOD0_RM: 00438 *maskBit = (uint8_t)reg_intm_glbl0.bits.dod0_rm; 00439 break; 00440 case INTM_GLBL1_GPI1_FM: 00441 *maskBit = (uint8_t)reg_intm_glbl1.bits.gpi1_fm; 00442 break; 00443 case INTM_GLBL1_GPI1_RM: 00444 *maskBit = (uint8_t)reg_intm_glbl1.bits.gpi1_rm; 00445 break; 00446 case INTM_GLBL1_SBB0_FM: 00447 *maskBit = (uint8_t)reg_intm_glbl1.bits.sbb0_fm; 00448 break; 00449 case INTM_GLBL1_SBB1_FM: 00450 *maskBit = (uint8_t)reg_intm_glbl1.bits.sbb1_fm; 00451 break; 00452 case INTM_GLBL1_SBB2_FM: 00453 *maskBit = (uint8_t)reg_intm_glbl1.bits.sbb2_fm; 00454 break; 00455 case INTM_GLBL1_LDO0_M: 00456 *maskBit = (uint8_t)reg_intm_glbl1.bits.ldo0_m; 00457 break; 00458 case INTM_GLBL1_LDO1_M: 00459 *maskBit = (uint8_t)reg_intm_glbl1.bits.ldo1_m; 00460 break; 00461 case INTM_GLBL1_RSVD: 00462 *maskBit = (uint8_t)reg_intm_glbl1.bits.rsvd; 00463 break; 00464 default: 00465 return MAX77658_INVALID_DATA; 00466 break; 00467 } 00468 00469 return MAX77658_NO_ERROR; 00470 } 00471 00472 int MAX77658::set_cnfg_glbl(reg_bit_cnfg_glbl_t bit_field, uint8_t config) 00473 { 00474 int ret; 00475 reg_cnfg_glbl_t reg_cnfg_glbl = {0}; 00476 00477 ret = read_register(CNFG_GLBL, (uint8_t *)&(reg_cnfg_glbl)); 00478 if (ret != MAX77658_NO_ERROR) return ret; 00479 00480 switch (bit_field) 00481 { 00482 case CNFG_GLBL_SFT_CTRL: 00483 reg_cnfg_glbl.bits.sft_ctrl = config; 00484 break; 00485 case CNFG_GLBL_DBEN_nEN: 00486 reg_cnfg_glbl.bits.dben_nen = config; 00487 break; 00488 case CNFG_GLBL_nEN_MODE: 00489 reg_cnfg_glbl.bits.nen_mode = config; 00490 break; 00491 case CNFG_GLBL_SBIA_LPM: 00492 reg_cnfg_glbl.bits.sbia_lpm = config; 00493 break; 00494 case CNFG_GLBL_T_MRST: 00495 reg_cnfg_glbl.bits.t_mrst = config; 00496 break; 00497 case CNFG_GLBL_PU_DIS: 00498 reg_cnfg_glbl.bits.pu_dis = config; 00499 break; 00500 default: 00501 return MAX77658_INVALID_DATA; 00502 break; 00503 } 00504 00505 return write_register(CNFG_GLBL, (uint8_t *)&(reg_cnfg_glbl)); 00506 } 00507 00508 int MAX77658::get_cnfg_glbl(reg_bit_cnfg_glbl_t bit_field, uint8_t *config) 00509 { 00510 int ret; 00511 reg_cnfg_glbl_t reg_cnfg_glbl = {0}; 00512 00513 ret = read_register(CNFG_GLBL, (uint8_t *)&(reg_cnfg_glbl)); 00514 if (ret != MAX77658_NO_ERROR) return ret; 00515 00516 switch (bit_field) 00517 { 00518 case CNFG_GLBL_SFT_CTRL: 00519 *config = (uint8_t)reg_cnfg_glbl.bits.sft_ctrl; 00520 break; 00521 case CNFG_GLBL_DBEN_nEN: 00522 *config = (uint8_t)reg_cnfg_glbl.bits.dben_nen; 00523 break; 00524 case CNFG_GLBL_nEN_MODE: 00525 *config = (uint8_t)reg_cnfg_glbl.bits.nen_mode; 00526 break; 00527 case CNFG_GLBL_SBIA_LPM: 00528 *config = (uint8_t)reg_cnfg_glbl.bits.sbia_lpm; 00529 break; 00530 case CNFG_GLBL_T_MRST: 00531 *config = (uint8_t)reg_cnfg_glbl.bits.t_mrst; 00532 break; 00533 case CNFG_GLBL_PU_DIS: 00534 *config = (uint8_t)reg_cnfg_glbl.bits.pu_dis; 00535 break; 00536 default: 00537 ret = MAX77658_INVALID_DATA; 00538 break; 00539 } 00540 00541 return ret; 00542 } 00543 00544 int MAX77658::set_cnfg_gpio(reg_bit_cnfg_gpio_t bit_field, uint8_t channel, uint8_t config) 00545 { 00546 int ret; 00547 reg_cnfg_gpio0_t reg_cnfg_gpio0 = {0}; 00548 reg_cnfg_gpio1_t reg_cnfg_gpio1 = {0}; 00549 reg_cnfg_gpio2_t reg_cnfg_gpio2 = {0}; 00550 00551 if (channel == 0) 00552 { 00553 ret = read_register(CNFG_GPIO0, (uint8_t *)&(reg_cnfg_gpio0)); 00554 if (ret != MAX77658_NO_ERROR) return ret; 00555 00556 switch (bit_field) 00557 { 00558 case CNFG_GPIO_DIR: 00559 reg_cnfg_gpio0.bits.gpo_dir = config; 00560 break; 00561 case CNFG_GPIO_DI: 00562 reg_cnfg_gpio0.bits.gpo_di = config; 00563 break; 00564 case CNFG_GPIO_DRV: 00565 reg_cnfg_gpio0.bits.gpo_drv = config; 00566 break; 00567 case CNFG_GPIO_DO: 00568 reg_cnfg_gpio0.bits.gpo_do = config; 00569 break; 00570 case CNFG_GPIO_DBEN_GPI: 00571 reg_cnfg_gpio0.bits.dben_gpi = config; 00572 break; 00573 case CNFG_GPIO_ALT_GPIO: 00574 reg_cnfg_gpio0.bits.alt_gpio = config; 00575 break; 00576 case CNFG_GPIO_RSVD: 00577 default: 00578 return MAX77658_INVALID_DATA; 00579 break; 00580 } 00581 00582 return write_register(CNFG_GPIO0, (uint8_t *)&(reg_cnfg_gpio0)); 00583 } 00584 else if (channel == 1) 00585 { 00586 ret = read_register(CNFG_GPIO1, (uint8_t *)&(reg_cnfg_gpio1)); 00587 if (ret != MAX77658_NO_ERROR) return ret; 00588 00589 switch (bit_field) 00590 { 00591 case CNFG_GPIO_DIR: 00592 reg_cnfg_gpio1.bits.gpo_dir = config; 00593 break; 00594 case CNFG_GPIO_DI: 00595 reg_cnfg_gpio1.bits.gpo_di = config; 00596 break; 00597 case CNFG_GPIO_DRV: 00598 reg_cnfg_gpio1.bits.gpo_drv = config; 00599 break; 00600 case CNFG_GPIO_DO: 00601 reg_cnfg_gpio1.bits.gpo_do = config; 00602 break; 00603 case CNFG_GPIO_DBEN_GPI: 00604 reg_cnfg_gpio1.bits.dben_gpi = config; 00605 break; 00606 case CNFG_GPIO_ALT_GPIO: 00607 reg_cnfg_gpio1.bits.alt_gpio = config; 00608 break; 00609 case CNFG_GPIO_RSVD: 00610 default: 00611 return MAX77658_INVALID_DATA; 00612 break; 00613 } 00614 00615 return write_register(CNFG_GPIO1, (uint8_t *)&(reg_cnfg_gpio1)); 00616 } 00617 else if (channel == 2) 00618 { 00619 ret = read_register(CNFG_GPIO2, (uint8_t *)&(reg_cnfg_gpio2)); 00620 if (ret != MAX77658_NO_ERROR) return ret; 00621 00622 switch (bit_field) 00623 { 00624 case CNFG_GPIO_DIR: 00625 reg_cnfg_gpio2.bits.gpo_dir = config; 00626 break; 00627 case CNFG_GPIO_DI: 00628 reg_cnfg_gpio2.bits.gpo_di = config; 00629 break; 00630 case CNFG_GPIO_DRV: 00631 reg_cnfg_gpio2.bits.gpo_drv = config; 00632 break; 00633 case CNFG_GPIO_DO: 00634 reg_cnfg_gpio2.bits.gpo_do = config; 00635 break; 00636 case CNFG_GPIO_DBEN_GPI: 00637 reg_cnfg_gpio2.bits.dben_gpi = config; 00638 break; 00639 case CNFG_GPIO_ALT_GPIO: 00640 reg_cnfg_gpio2.bits.alt_gpio = config; 00641 break; 00642 case CNFG_GPIO_RSVD: 00643 default: 00644 return MAX77658_INVALID_DATA; 00645 break; 00646 } 00647 00648 return write_register(CNFG_GPIO2, (uint8_t *)&(reg_cnfg_gpio2)); 00649 } 00650 else { 00651 return MAX77658_INVALID_DATA; 00652 } 00653 } 00654 00655 int MAX77658::get_cnfg_gpio(reg_bit_cnfg_gpio_t bit_field, uint8_t channel, uint8_t *config) 00656 { 00657 int ret; 00658 reg_cnfg_gpio0_t reg_cnfg_gpio0 = {0}; 00659 reg_cnfg_gpio1_t reg_cnfg_gpio1 = {0}; 00660 reg_cnfg_gpio2_t reg_cnfg_gpio2 = {0}; 00661 00662 if (channel == 0) 00663 { 00664 ret = read_register(CNFG_GPIO0, (uint8_t *)&(reg_cnfg_gpio0)); 00665 if (ret != MAX77658_NO_ERROR) return ret; 00666 00667 switch (bit_field) 00668 { 00669 case CNFG_GPIO_DIR: 00670 *config = (uint8_t)reg_cnfg_gpio0.bits.gpo_dir; 00671 break; 00672 case CNFG_GPIO_DI: 00673 *config = (uint8_t)reg_cnfg_gpio0.bits.gpo_di; 00674 break; 00675 case CNFG_GPIO_DRV: 00676 *config = (uint8_t)reg_cnfg_gpio0.bits.gpo_drv; 00677 break; 00678 case CNFG_GPIO_DO: 00679 *config = (uint8_t)reg_cnfg_gpio0.bits.gpo_do; 00680 break; 00681 case CNFG_GPIO_DBEN_GPI: 00682 *config = (uint8_t)reg_cnfg_gpio0.bits.dben_gpi; 00683 break; 00684 case CNFG_GPIO_ALT_GPIO: 00685 *config = (uint8_t)reg_cnfg_gpio0.bits.alt_gpio; 00686 break; 00687 case CNFG_GPIO_RSVD: 00688 default: 00689 return MAX77658_INVALID_DATA; 00690 break; 00691 } 00692 } 00693 else if (channel == 1) 00694 { 00695 ret = read_register(CNFG_GPIO1, (uint8_t *)&(reg_cnfg_gpio1)); 00696 if (ret != MAX77658_NO_ERROR) return ret; 00697 00698 switch (bit_field) 00699 { 00700 case CNFG_GPIO_DIR: 00701 *config = (uint8_t)reg_cnfg_gpio1.bits.gpo_dir; 00702 break; 00703 case CNFG_GPIO_DI: 00704 *config = (uint8_t)reg_cnfg_gpio1.bits.gpo_di; 00705 break; 00706 case CNFG_GPIO_DRV: 00707 *config = (uint8_t)reg_cnfg_gpio1.bits.gpo_drv; 00708 break; 00709 case CNFG_GPIO_DO: 00710 *config = (uint8_t)reg_cnfg_gpio1.bits.gpo_do; 00711 break; 00712 case CNFG_GPIO_DBEN_GPI: 00713 *config = (uint8_t)reg_cnfg_gpio1.bits.dben_gpi; 00714 break; 00715 case CNFG_GPIO_ALT_GPIO: 00716 *config = (uint8_t)reg_cnfg_gpio1.bits.alt_gpio; 00717 break; 00718 case CNFG_GPIO_RSVD: 00719 default: 00720 return MAX77658_INVALID_DATA; 00721 break; 00722 } 00723 } 00724 else if (channel == 2) 00725 { 00726 ret = read_register(CNFG_GPIO2, (uint8_t *)&(reg_cnfg_gpio2)); 00727 if (ret != MAX77658_NO_ERROR) return ret; 00728 00729 switch (bit_field) 00730 { 00731 case CNFG_GPIO_DIR: 00732 *config = (uint8_t)reg_cnfg_gpio2.bits.gpo_dir; 00733 break; 00734 case CNFG_GPIO_DI: 00735 *config = (uint8_t)reg_cnfg_gpio2.bits.gpo_di; 00736 break; 00737 case CNFG_GPIO_DRV: 00738 *config = (uint8_t)reg_cnfg_gpio2.bits.gpo_drv; 00739 break; 00740 case CNFG_GPIO_DO: 00741 *config = (uint8_t)reg_cnfg_gpio2.bits.gpo_do; 00742 break; 00743 case CNFG_GPIO_DBEN_GPI: 00744 *config = (uint8_t)reg_cnfg_gpio2.bits.dben_gpi; 00745 break; 00746 case CNFG_GPIO_ALT_GPIO: 00747 *config = (uint8_t)reg_cnfg_gpio2.bits.alt_gpio; 00748 break; 00749 case CNFG_GPIO_RSVD: 00750 default: 00751 return MAX77658_INVALID_DATA; 00752 break; 00753 } 00754 } 00755 else { 00756 return MAX77658_INVALID_DATA; 00757 } 00758 00759 return ret; 00760 } 00761 00762 int MAX77658::get_cid(void) { 00763 char rbuf[1]; 00764 int ret; 00765 00766 ret = read_register(CID, (uint8_t *)&(rbuf)); 00767 if (ret != MAX77658_NO_ERROR) return ret; 00768 00769 return *rbuf; 00770 } 00771 00772 int MAX77658::set_cnfg_wdt(reg_bit_cnfg_wdt_t bit_field, uint8_t config) 00773 { 00774 int ret; 00775 reg_cnfg_wdt_t reg_cnfg_wdt = {0}; 00776 00777 ret = read_register(CNFG_WDT, (uint8_t *)&(reg_cnfg_wdt)); 00778 if (ret != MAX77658_NO_ERROR) return ret; 00779 00780 switch (bit_field) 00781 { 00782 case CNFG_WDT_WDT_LOCK: 00783 reg_cnfg_wdt.bits.wdt_lock = config; 00784 break; 00785 case CNFG_WDT_WDT_EN: 00786 reg_cnfg_wdt.bits.wdt_en = config; 00787 break; 00788 case CNFG_WDT_WDT_CLR: 00789 reg_cnfg_wdt.bits.wdt_clr = config; 00790 break; 00791 case CNFG_WDT_WDT_MODE: 00792 reg_cnfg_wdt.bits.wdt_mode = config; 00793 break; 00794 case CNFG_WDT_WDT_PER: 00795 reg_cnfg_wdt.bits.wdt_per = config; 00796 break; 00797 case CNFG_WDT_RSVD: 00798 default: 00799 return MAX77658_INVALID_DATA; 00800 break; 00801 } 00802 00803 return write_register(CNFG_WDT, (uint8_t *)&(reg_cnfg_wdt)); 00804 } 00805 00806 int MAX77658::get_cnfg_wdt(reg_bit_cnfg_wdt_t bit_field, uint8_t *config) 00807 { 00808 int ret; 00809 reg_cnfg_wdt_t reg_cnfg_wdt = {0}; 00810 00811 ret = read_register(CNFG_WDT, (uint8_t *)&(reg_cnfg_wdt)); 00812 if (ret != MAX77658_NO_ERROR) return ret; 00813 00814 switch (bit_field) 00815 { 00816 case CNFG_WDT_WDT_LOCK: 00817 *config = (uint8_t)reg_cnfg_wdt.bits.wdt_lock; 00818 break; 00819 case CNFG_WDT_WDT_EN: 00820 *config = (uint8_t)reg_cnfg_wdt.bits.wdt_en; 00821 break; 00822 case CNFG_WDT_WDT_CLR: 00823 *config = (uint8_t)reg_cnfg_wdt.bits.wdt_clr; 00824 break; 00825 case CNFG_WDT_WDT_MODE: 00826 *config = (uint8_t)reg_cnfg_wdt.bits.wdt_mode; 00827 break; 00828 case CNFG_WDT_WDT_PER: 00829 *config = (uint8_t)reg_cnfg_wdt.bits.wdt_per; 00830 break; 00831 case CNFG_WDT_RSVD: 00832 default: 00833 return MAX77658_INVALID_DATA; 00834 break; 00835 } 00836 00837 return MAX77658_NO_ERROR; 00838 } 00839 00840 int MAX77658::get_stat_chg_a(reg_bit_stat_chg_a_t bit_field, uint8_t *status) 00841 { 00842 int ret; 00843 reg_stat_chg_a_t reg_stat_chg_a = {0}; 00844 00845 ret = read_register(STAT_CHG_A, (uint8_t *)&(reg_stat_chg_a)); 00846 if (ret != MAX77658_NO_ERROR) return ret; 00847 00848 switch (bit_field) 00849 { 00850 case STAT_CHG_A_THM_DTLS: 00851 *status = (uint8_t)reg_stat_chg_a.bits.thm_dtls; 00852 break; 00853 case STAT_CHG_A_TJ_REG_STAT: 00854 *status = (uint8_t)reg_stat_chg_a.bits.tj_reg_stat; 00855 break; 00856 case STAT_CHG_A_VSYS_MIN_STAT: 00857 *status = (uint8_t)reg_stat_chg_a.bits.vsys_min_stat; 00858 break; 00859 case STAT_CHG_A_ICHGIN_LIM_STAT: 00860 *status = (uint8_t)reg_stat_chg_a.bits.ichgin_lim_stat; 00861 break; 00862 case STAT_CHG_A_VCHGIN_MIN_STAT: 00863 *status = (uint8_t)reg_stat_chg_a.bits.vchgin_min_stat; 00864 break; 00865 case STAT_CHG_A_RSVD: 00866 default: 00867 return MAX77658_INVALID_DATA; 00868 break; 00869 } 00870 00871 return MAX77658_NO_ERROR; 00872 } 00873 00874 int MAX77658::get_thm_dtls(decode_thm_dtls_t *thm_dtls) 00875 { 00876 int ret; 00877 reg_stat_chg_a_t reg_stat_chg_a = {0}; 00878 00879 ret = read_register(STAT_CHG_A, (uint8_t *)&(reg_stat_chg_a)); 00880 if (ret != MAX77658_NO_ERROR) return ret; 00881 00882 *thm_dtls = (decode_thm_dtls_t)reg_stat_chg_a.bits.thm_dtls; 00883 00884 return MAX77658_NO_ERROR; 00885 } 00886 00887 int MAX77658::get_stat_chg_b(reg_bit_stat_chg_b_t bit_field, uint8_t *status) 00888 { 00889 int ret; 00890 reg_stat_chg_b_t reg_stat_chg_b = {0}; 00891 00892 ret = read_register(STAT_CHG_B, (uint8_t *)&(reg_stat_chg_b)); 00893 if (ret != MAX77658_NO_ERROR) return ret; 00894 00895 switch (bit_field) 00896 { 00897 case STAT_CHG_B_TIME_SUS: 00898 *status = (uint8_t)reg_stat_chg_b.bits.time_sus; 00899 break; 00900 case STAT_CHG_B_CHG: 00901 *status = (uint8_t)reg_stat_chg_b.bits.chg; 00902 break; 00903 case STAT_CHG_B_CHGIN_DTLS: 00904 *status = (uint8_t)reg_stat_chg_b.bits.chgin_dtls; 00905 break; 00906 case STAT_CHG_B_CHG_DTLS: 00907 *status = (uint8_t)reg_stat_chg_b.bits.chg_dtls; 00908 break; 00909 default: 00910 return MAX77658_INVALID_DATA; 00911 break; 00912 } 00913 00914 return MAX77658_NO_ERROR; 00915 } 00916 00917 int MAX77658::get_chg_dtls(decode_chg_dtls_t *chg_dtls) 00918 { 00919 int ret; 00920 reg_stat_chg_b_t reg_stat_chg_b = {0}; 00921 00922 ret = read_register(STAT_CHG_B, (uint8_t *)&(reg_stat_chg_b)); 00923 if (ret != MAX77658_NO_ERROR) return ret; 00924 00925 *chg_dtls = (decode_chg_dtls_t)reg_stat_chg_b.bits.chg_dtls; 00926 00927 return MAX77658_NO_ERROR; 00928 } 00929 00930 int MAX77658::set_thm_hot(int tempDegC) 00931 { 00932 uint8_t value; 00933 reg_cnfg_chg_a_t reg_cnfg_chg_a = {0}; 00934 00935 if (tempDegC < 45) tempDegC = 45; 00936 else if (tempDegC > 60) tempDegC = 60; 00937 00938 value = (tempDegC - 45) / 5; 00939 00940 SET_BIT_FIELD(CNFG_CHG_A, reg_cnfg_chg_a, reg_cnfg_chg_a.bits.thm_hot, value); 00941 return MAX77658_NO_ERROR; 00942 } 00943 00944 int MAX77658::get_thm_hot(int *tempDegC) 00945 { 00946 int ret; 00947 uint8_t bit_value; 00948 reg_cnfg_chg_a_t reg_cnfg_chg_a = {0}; 00949 00950 ret = read_register(CNFG_CHG_A, (uint8_t *)&(reg_cnfg_chg_a)); 00951 if (ret != MAX77658_NO_ERROR) return ret; 00952 00953 bit_value = (uint8_t)reg_cnfg_chg_a.bits.thm_hot; 00954 if (bit_value <= 3) 00955 *tempDegC = (bit_value * 5) + 45; 00956 else 00957 return MAX77658_INVALID_DATA; 00958 00959 return MAX77658_NO_ERROR; 00960 } 00961 00962 int MAX77658::set_thm_warm(int tempDegC) 00963 { 00964 uint8_t value; 00965 reg_cnfg_chg_a_t reg_cnfg_chg_a = {0}; 00966 00967 if (tempDegC < 35) tempDegC = 35; 00968 else if (tempDegC > 50) tempDegC = 50; 00969 00970 value = (tempDegC - 35) / 5; 00971 00972 SET_BIT_FIELD(CNFG_CHG_A, reg_cnfg_chg_a, reg_cnfg_chg_a.bits.thm_warm, value); 00973 return MAX77658_NO_ERROR; 00974 } 00975 00976 int MAX77658::get_thm_warm(int *tempDegC) 00977 { 00978 int ret; 00979 uint8_t bit_value; 00980 reg_cnfg_chg_a_t reg_cnfg_chg_a = {0}; 00981 00982 ret = read_register(CNFG_CHG_A, (uint8_t *)&(reg_cnfg_chg_a)); 00983 if (ret != MAX77658_NO_ERROR) return ret; 00984 00985 bit_value = (uint8_t)reg_cnfg_chg_a.bits.thm_warm; 00986 if (bit_value <= 3) 00987 *tempDegC = (bit_value * 5) + 35; 00988 else 00989 return MAX77658_INVALID_DATA; 00990 00991 return MAX77658_NO_ERROR; 00992 } 00993 00994 int MAX77658::set_thm_cool(int tempDegC) 00995 { 00996 uint8_t value; 00997 reg_cnfg_chg_a_t reg_cnfg_chg_a = {0}; 00998 00999 if (tempDegC < 0) tempDegC = 0; 01000 else if (tempDegC > 15) tempDegC = 15; 01001 01002 value = tempDegC / 5; 01003 01004 SET_BIT_FIELD(CNFG_CHG_A, reg_cnfg_chg_a, reg_cnfg_chg_a.bits.thm_cool, value); 01005 return MAX77658_NO_ERROR; 01006 } 01007 01008 int MAX77658::get_thm_cool(int *tempDegC) 01009 { 01010 int ret; 01011 uint8_t bit_value; 01012 reg_cnfg_chg_a_t reg_cnfg_chg_a = {0}; 01013 01014 ret = read_register(CNFG_CHG_A, (uint8_t *)&(reg_cnfg_chg_a)); 01015 if (ret != MAX77658_NO_ERROR) return ret; 01016 01017 bit_value = (uint8_t)reg_cnfg_chg_a.bits.thm_cool; 01018 if (bit_value <= 3) 01019 *tempDegC = bit_value * 5; 01020 else 01021 return MAX77658_INVALID_DATA; 01022 01023 return MAX77658_NO_ERROR; 01024 } 01025 01026 int MAX77658::set_thm_cold(int tempDegC) 01027 { 01028 uint8_t value; 01029 reg_cnfg_chg_a_t reg_cnfg_chg_a = {0}; 01030 01031 if (tempDegC < -10) tempDegC = -10; 01032 else if (tempDegC > 5) tempDegC = 5; 01033 01034 value = (tempDegC + 10) / 5; 01035 01036 SET_BIT_FIELD(CNFG_CHG_A, reg_cnfg_chg_a, reg_cnfg_chg_a.bits.thm_cold, value); 01037 return MAX77658_NO_ERROR; 01038 } 01039 01040 int MAX77658::get_thm_cold(int *tempDegC) 01041 { 01042 int ret; 01043 uint8_t bit_value; 01044 reg_cnfg_chg_a_t reg_cnfg_chg_a = {0}; 01045 01046 ret = read_register(CNFG_CHG_A, (uint8_t *)&(reg_cnfg_chg_a)); 01047 if (ret != MAX77658_NO_ERROR) return ret; 01048 01049 bit_value = (uint8_t)reg_cnfg_chg_a.bits.thm_cold; 01050 if (bit_value <= 3) 01051 *tempDegC = (bit_value * 5) - 10; 01052 else 01053 return MAX77658_INVALID_DATA; 01054 01055 return MAX77658_NO_ERROR; 01056 } 01057 01058 int MAX77658::set_cnfg_chg_b(reg_bit_cnfg_chg_b_t bit_field, uint8_t config) 01059 { 01060 int ret; 01061 reg_cnfg_chg_b_t reg_cnfg_chg_b = {0}; 01062 01063 ret = read_register(CNFG_CHG_B, (uint8_t *)&(reg_cnfg_chg_b)); 01064 if (ret != MAX77658_NO_ERROR) return ret; 01065 01066 switch (bit_field) 01067 { 01068 case CNFG_CHG_B_CHG_EN: 01069 reg_cnfg_chg_b.bits.chg_en = config; 01070 break; 01071 case CNFG_CHG_B_I_PQ: 01072 reg_cnfg_chg_b.bits.i_pq = config; 01073 break; 01074 case CNFG_CHG_B_ICHGIN_LIM: 01075 reg_cnfg_chg_b.bits.ichgin_lim = config; 01076 break; 01077 case CNFG_CHG_B_VCHGIN_MIN: 01078 reg_cnfg_chg_b.bits.vchgin_min = config; 01079 break; 01080 default: 01081 return MAX77658_INVALID_DATA; 01082 break; 01083 } 01084 01085 return write_register(CNFG_CHG_B, (uint8_t *)&(reg_cnfg_chg_b)); 01086 } 01087 01088 int MAX77658::get_cnfg_chg_b(reg_bit_cnfg_chg_b_t bit_field, uint8_t *config) 01089 { 01090 int ret; 01091 reg_cnfg_chg_b_t reg_cnfg_chg_b = {0}; 01092 01093 ret = read_register(CNFG_CHG_B, (uint8_t *)&(reg_cnfg_chg_b)); 01094 if (ret != MAX77658_NO_ERROR) return ret; 01095 01096 switch (bit_field) 01097 { 01098 case CNFG_CHG_B_CHG_EN: 01099 *config = (uint8_t)reg_cnfg_chg_b.bits.chg_en; 01100 break; 01101 case CNFG_CHG_B_I_PQ: 01102 *config = (uint8_t)reg_cnfg_chg_b.bits.i_pq; 01103 break; 01104 case CNFG_CHG_B_ICHGIN_LIM: 01105 *config = (uint8_t)reg_cnfg_chg_b.bits.ichgin_lim; 01106 break; 01107 case CNFG_CHG_B_VCHGIN_MIN: 01108 *config = (uint8_t)reg_cnfg_chg_b.bits.vchgin_min; 01109 break; 01110 default: 01111 return MAX77658_INVALID_DATA; 01112 break; 01113 } 01114 01115 return MAX77658_NO_ERROR; 01116 } 01117 01118 int MAX77658::set_vchgin_min(float voltV) 01119 { 01120 uint8_t value; 01121 reg_cnfg_chg_b_t reg_cnfg_chg_b = {0}; 01122 float voltmV = voltV * 1000; 01123 01124 if (voltmV < 4000) voltmV = 4000; 01125 else if (voltmV > 4700) voltmV = 4700; 01126 01127 value = (voltmV - 4000) / 100; 01128 01129 SET_BIT_FIELD(CNFG_CHG_B, reg_cnfg_chg_b, reg_cnfg_chg_b.bits.vchgin_min, value); 01130 return MAX77658_NO_ERROR; 01131 } 01132 01133 int MAX77658::get_vchgin_min(float *voltV) 01134 { 01135 int ret; 01136 uint8_t value; 01137 reg_cnfg_chg_b_t reg_cnfg_chg_b = {0}; 01138 01139 ret = read_register(CNFG_CHG_B, (uint8_t *)&(reg_cnfg_chg_b)); 01140 if (ret != MAX77658_NO_ERROR) return ret; 01141 01142 value = (uint8_t)reg_cnfg_chg_b.bits.vchgin_min; 01143 *voltV = (float)(value * 0.1f) + 4.0f; 01144 return MAX77658_NO_ERROR; 01145 } 01146 01147 int MAX77658::set_ichgin_lim(int currentmA) 01148 { 01149 uint8_t value; 01150 reg_cnfg_chg_b_t reg_cnfg_chg_b = {0}; 01151 01152 if (currentmA < 95) currentmA = 95; 01153 else if (currentmA > 475) currentmA = 475; 01154 01155 value = (currentmA - 95) / 95; 01156 01157 SET_BIT_FIELD(CNFG_CHG_B, reg_cnfg_chg_b, reg_cnfg_chg_b.bits.ichgin_lim, value); 01158 return MAX77658_NO_ERROR; 01159 } 01160 01161 int MAX77658::get_ichgin_lim(int *currentmA) 01162 { 01163 int ret; 01164 uint8_t bit_value; 01165 reg_cnfg_chg_b_t reg_cnfg_chg_b = {0}; 01166 01167 ret = read_register(CNFG_CHG_B, (uint8_t *)&(reg_cnfg_chg_b)); 01168 if (ret != MAX77658_NO_ERROR) return ret; 01169 01170 bit_value = (uint8_t)reg_cnfg_chg_b.bits.ichgin_lim; 01171 *currentmA = (bit_value * 95) + 95; 01172 return MAX77658_NO_ERROR; 01173 } 01174 01175 int MAX77658::set_chg_pq(float voltV) 01176 { 01177 uint8_t value; 01178 reg_cnfg_chg_c_t reg_cnfg_chg_c = {0}; 01179 float voltmV = voltV * 1000; 01180 01181 if (voltmV < 2300) voltmV = 2300; 01182 else if (voltmV > 3000) voltmV = 3000; 01183 01184 value = (voltmV - 2300) / 100; 01185 01186 SET_BIT_FIELD(CNFG_CHG_C, reg_cnfg_chg_c, reg_cnfg_chg_c.bits.chg_pq, value); 01187 return MAX77658_NO_ERROR; 01188 } 01189 01190 int MAX77658::get_chg_pq(float *voltV) 01191 { 01192 int ret; 01193 uint8_t bit_value; 01194 reg_cnfg_chg_c_t reg_cnfg_chg_c = {0}; 01195 01196 ret = read_register(CNFG_CHG_C, (uint8_t *)&(reg_cnfg_chg_c)); 01197 if (ret != MAX77658_NO_ERROR) return ret; 01198 01199 bit_value = (uint8_t)reg_cnfg_chg_c.bits.chg_pq; 01200 *voltV = (bit_value * 0.1f) + 2.3f; 01201 return MAX77658_NO_ERROR; 01202 } 01203 01204 int MAX77658::set_i_term(float percent) 01205 { 01206 uint8_t value; 01207 reg_cnfg_chg_c_t reg_cnfg_chg_c = {0}; 01208 01209 if (percent < 7.5f) value = 0; 01210 else if ((percent >= 7.5f) && (percent < 10)) value = 1; 01211 else if ((percent >= 10) && (percent < 15)) value = 2; 01212 else if (percent >= 15) value = 3; 01213 01214 SET_BIT_FIELD(CNFG_CHG_C, reg_cnfg_chg_c, reg_cnfg_chg_c.bits.i_term, value); 01215 return MAX77658_NO_ERROR; 01216 } 01217 01218 int MAX77658::get_i_term(float *percent) 01219 { 01220 int ret; 01221 uint8_t bit_value; 01222 reg_cnfg_chg_c_t reg_cnfg_chg_c = {0}; 01223 01224 ret = read_register(CNFG_CHG_C, (uint8_t *)&(reg_cnfg_chg_c)); 01225 if (ret != MAX77658_NO_ERROR) return ret; 01226 01227 bit_value = (uint8_t)reg_cnfg_chg_c.bits.i_term; 01228 01229 if (bit_value == 0) *percent = 5.0f; 01230 else if (bit_value == 1) *percent = 7.5f; 01231 else if (bit_value == 2) *percent = 10.0f; 01232 else if (bit_value == 3) *percent = 15.0f; 01233 else return MAX77658_INVALID_DATA; 01234 01235 return MAX77658_NO_ERROR; 01236 } 01237 01238 int MAX77658::set_t_topoff(uint8_t minute) 01239 { 01240 uint8_t value; 01241 reg_cnfg_chg_c_t reg_cnfg_chg_c = {0}; 01242 01243 if (minute > 35) minute = 35; 01244 01245 value = (uint8_t)(minute / 5); 01246 01247 SET_BIT_FIELD(CNFG_CHG_C, reg_cnfg_chg_c, reg_cnfg_chg_c.bits.t_topoff, value); 01248 return MAX77658_NO_ERROR; 01249 } 01250 01251 int MAX77658::get_t_topoff(uint8_t *minute) 01252 { 01253 int ret; 01254 uint8_t bit_value; 01255 reg_cnfg_chg_c_t reg_cnfg_chg_c = {0}; 01256 01257 ret = read_register(CNFG_CHG_C, (uint8_t *)&(reg_cnfg_chg_c)); 01258 if (ret != MAX77658_NO_ERROR) return ret; 01259 01260 bit_value = (uint8_t)reg_cnfg_chg_c.bits.t_topoff; 01261 *minute = (bit_value * 5); 01262 return MAX77658_NO_ERROR; 01263 } 01264 01265 int MAX77658::set_tj_reg(uint8_t tempDegC) 01266 { 01267 uint8_t value; 01268 reg_cnfg_chg_d_t reg_cnfg_chg_d = {0}; 01269 01270 if (tempDegC < 60) tempDegC = 60; 01271 else if (tempDegC > 100) tempDegC = 100; 01272 01273 value = (tempDegC - 60) / 10; 01274 01275 SET_BIT_FIELD(CNFG_CHG_D, reg_cnfg_chg_d, reg_cnfg_chg_d.bits.tj_reg, value); 01276 return MAX77658_NO_ERROR; 01277 } 01278 01279 int MAX77658::get_tj_reg(uint8_t *tempDegC) 01280 { 01281 int ret; 01282 uint8_t bit_value; 01283 reg_cnfg_chg_d_t reg_cnfg_chg_d = {0}; 01284 01285 ret = read_register(CNFG_CHG_D, (uint8_t *)&(reg_cnfg_chg_d)); 01286 if (ret != MAX77658_NO_ERROR) return ret; 01287 01288 bit_value = (uint8_t)reg_cnfg_chg_d.bits.tj_reg; 01289 *tempDegC = (bit_value * 10) + 60; 01290 return MAX77658_NO_ERROR; 01291 } 01292 01293 int MAX77658::set_vsys_reg(float voltV) 01294 { 01295 uint8_t value; 01296 reg_cnfg_chg_d_t reg_cnfg_chg_d = {0}; 01297 float voltmV = voltV * 1000; 01298 01299 if (voltmV < 3300) voltmV = 3300; 01300 else if (voltmV > 4800) voltmV = 4800; 01301 01302 value = (voltmV - 3300) / 50; 01303 01304 SET_BIT_FIELD(CNFG_CHG_D, reg_cnfg_chg_d, reg_cnfg_chg_d.bits.vsys_reg, value); 01305 return MAX77658_NO_ERROR; 01306 } 01307 01308 int MAX77658::get_vsys_reg(float *voltV) 01309 { 01310 int ret; 01311 uint8_t bit_value; 01312 reg_cnfg_chg_d_t reg_cnfg_chg_d = {0}; 01313 01314 ret = read_register(CNFG_CHG_D, (uint8_t *)&(reg_cnfg_chg_d)); 01315 if (ret != MAX77658_NO_ERROR) return ret; 01316 01317 bit_value = (uint8_t)reg_cnfg_chg_d.bits.vsys_reg; 01318 *voltV = (bit_value * 0.05f) + 3.3f; 01319 return MAX77658_NO_ERROR; 01320 } 01321 01322 int MAX77658::set_chg_cc(float currentmA) 01323 { 01324 uint8_t value; 01325 reg_cnfg_chg_e_t reg_cnfg_chg_e = {0}; 01326 float currentuA = currentmA * 1000; 01327 01328 if (currentuA < 7500) currentuA = 7500; 01329 if (currentuA > 300000) currentuA = 300000; 01330 01331 value = (currentuA - 7500) / 7500; 01332 01333 SET_BIT_FIELD(CNFG_CHG_E, reg_cnfg_chg_e, reg_cnfg_chg_e.bits.chg_cc, value); 01334 return MAX77658_NO_ERROR; 01335 } 01336 01337 int MAX77658::get_chg_cc(float *currentmA) 01338 { 01339 int ret; 01340 uint8_t bit_value; 01341 reg_cnfg_chg_e_t reg_cnfg_chg_e = {0}; 01342 01343 ret = read_register(CNFG_CHG_E, (uint8_t *)&(reg_cnfg_chg_e)); 01344 if (ret != MAX77658_NO_ERROR) return ret; 01345 01346 bit_value = (uint8_t)reg_cnfg_chg_e.bits.chg_cc; 01347 if (bit_value >= 39) bit_value = 39; //0x27 to 0x3F = 300.0mA 01348 01349 *currentmA = (bit_value * 7.5f) + 7.5f; 01350 01351 return MAX77658_NO_ERROR; 01352 } 01353 01354 int MAX77658::set_t_fast_chg(decode_t_fast_chg_t t_fast_chg) 01355 { 01356 reg_cnfg_chg_e_t reg_cnfg_chg_e = {0}; 01357 01358 SET_BIT_FIELD(CNFG_CHG_E, reg_cnfg_chg_e, reg_cnfg_chg_e.bits.t_fast_chg, t_fast_chg); 01359 return MAX77658_NO_ERROR; 01360 } 01361 01362 int MAX77658::get_t_fast_chg(decode_t_fast_chg_t *t_fast_chg) 01363 { 01364 int ret; 01365 reg_cnfg_chg_e_t reg_cnfg_chg_e = {0}; 01366 01367 ret = read_register(CNFG_CHG_E, (uint8_t *)&(reg_cnfg_chg_e)); 01368 if (ret != MAX77658_NO_ERROR) return ret; 01369 01370 *t_fast_chg = (decode_t_fast_chg_t)reg_cnfg_chg_e.bits.t_fast_chg; 01371 01372 return MAX77658_NO_ERROR; 01373 } 01374 01375 int MAX77658::set_chg_cc_jeita(float currentmA) 01376 { 01377 uint8_t value; 01378 reg_cnfg_chg_f_t reg_cnfg_chg_f = {0}; 01379 float currentuA = currentmA * 1000; 01380 01381 if (currentuA < 7500) currentuA = 7500; 01382 else if (currentuA > 300000) currentuA = 300000; 01383 01384 value = round(currentuA - 7500) / 7500; 01385 01386 SET_BIT_FIELD(CNFG_CHG_F, reg_cnfg_chg_f, reg_cnfg_chg_f.bits.chg_cc_jeita, value); 01387 return MAX77658_NO_ERROR; 01388 } 01389 01390 int MAX77658::get_chg_cc_jeita(float *currentmA) 01391 { 01392 int ret; 01393 uint8_t bit_value; 01394 reg_cnfg_chg_f_t reg_cnfg_chg_f = {0}; 01395 01396 ret = read_register(CNFG_CHG_F, (uint8_t *)&(reg_cnfg_chg_f)); 01397 if (ret != MAX77658_NO_ERROR) return ret; 01398 01399 bit_value = (uint8_t)reg_cnfg_chg_f.bits.chg_cc_jeita; 01400 if (bit_value >= 39) bit_value = 39; //0x27 to 0x3F = 300.0mA 01401 01402 *currentmA = (bit_value * 7.5f) + 7.5f; 01403 return MAX77658_NO_ERROR; 01404 } 01405 01406 int MAX77658::set_cnfg_chg_g(reg_bit_cnfg_chg_g_t bit_field, uint8_t config) 01407 { 01408 int ret; 01409 reg_cnfg_chg_g_t reg_cnfg_chg_g = {0}; 01410 01411 ret = read_register(CNFG_CHG_G, (uint8_t *)&(reg_cnfg_chg_g)); 01412 if (ret != MAX77658_NO_ERROR) return ret; 01413 01414 switch (bit_field) 01415 { 01416 case CNFG_CHG_G_FUS_M: 01417 reg_cnfg_chg_g.bits.fus_m = config; 01418 break; 01419 case CNFG_CHG_G_USBS: 01420 reg_cnfg_chg_g.bits.usbs = config; 01421 break; 01422 case CNFG_CHG_G_CHG_CV: 01423 reg_cnfg_chg_g.bits.chg_cv = config; 01424 break; 01425 default: 01426 return MAX77658_INVALID_DATA; 01427 break; 01428 } 01429 01430 return write_register(CNFG_CHG_G, (uint8_t *)&(reg_cnfg_chg_g)); 01431 } 01432 01433 int MAX77658::get_cnfg_chg_g(reg_bit_cnfg_chg_g_t bit_field, uint8_t *config) 01434 { 01435 int ret; 01436 reg_cnfg_chg_g_t reg_cnfg_chg_g = {0}; 01437 01438 ret = read_register(CNFG_CHG_G, (uint8_t *)&(reg_cnfg_chg_g)); 01439 if (ret != MAX77658_NO_ERROR) return ret; 01440 01441 switch (bit_field) 01442 { 01443 case CNFG_CHG_G_FUS_M: 01444 *config = (uint8_t)reg_cnfg_chg_g.bits.fus_m; 01445 break; 01446 case CNFG_CHG_G_USBS: 01447 *config = (uint8_t)reg_cnfg_chg_g.bits.usbs; 01448 break; 01449 case CNFG_CHG_G_CHG_CV: 01450 *config = (uint8_t)reg_cnfg_chg_g.bits.chg_cv; 01451 break; 01452 default: 01453 return MAX77658_INVALID_DATA; 01454 break; 01455 } 01456 01457 return MAX77658_NO_ERROR; 01458 } 01459 01460 int MAX77658::set_chg_cv(float voltV) 01461 { 01462 uint8_t value; 01463 reg_cnfg_chg_g_t reg_cnfg_chg_g = {0}; 01464 float voltmV = voltV * 1000; 01465 01466 if (voltmV < 3600) voltmV = 3600; 01467 else if (voltmV > 4600) voltmV = 4600; 01468 01469 value = (voltmV - 3600) / 25; 01470 01471 SET_BIT_FIELD(CNFG_CHG_G, reg_cnfg_chg_g, reg_cnfg_chg_g.bits.chg_cv, value); 01472 return MAX77658_NO_ERROR; 01473 } 01474 01475 int MAX77658::get_chg_cv(float *voltV) 01476 { 01477 int ret; 01478 uint8_t bit_value; 01479 reg_cnfg_chg_g_t reg_cnfg_chg_g = {0}; 01480 01481 ret = read_register(CNFG_CHG_G, (uint8_t *)&(reg_cnfg_chg_g)); 01482 if (ret != MAX77658_NO_ERROR) return ret; 01483 01484 bit_value = (uint8_t)reg_cnfg_chg_g.bits.chg_cv; 01485 *voltV = (bit_value * 0.025f) + 3.6f; 01486 return MAX77658_NO_ERROR; 01487 } 01488 01489 int MAX77658::set_cnfg_chg_h(reg_bit_cnfg_chg_h_t bit_field, uint8_t config) 01490 { 01491 int ret; 01492 reg_cnfg_chg_h_t reg_cnfg_chg_h = {0}; 01493 01494 ret = read_register(CNFG_CHG_H, (uint8_t *)&(reg_cnfg_chg_h)); 01495 if (ret != MAX77658_NO_ERROR) return ret; 01496 01497 switch (bit_field) 01498 { 01499 case CNFG_CHG_H_CHR_TH_DIS: 01500 reg_cnfg_chg_h.bits.chr_th_dis = config; 01501 break; 01502 case CNFG_CHG_H_SYS_BAT_PRT: 01503 reg_cnfg_chg_h.bits.sys_bat_prt = config; 01504 break; 01505 case CNFG_CHG_H_CHG_CV_JEITA: 01506 reg_cnfg_chg_h.bits.chg_cv_jeita = config; 01507 break; 01508 default: 01509 return MAX77658_INVALID_DATA; 01510 break; 01511 } 01512 01513 return write_register(CNFG_CHG_H, (uint8_t *)&(reg_cnfg_chg_h)); 01514 } 01515 01516 int MAX77658::get_cnfg_chg_h(reg_bit_cnfg_chg_h_t bit_field, uint8_t *config) 01517 { 01518 int ret; 01519 reg_cnfg_chg_h_t reg_cnfg_chg_h = {0}; 01520 01521 ret = read_register(CNFG_CHG_H, (uint8_t *)&(reg_cnfg_chg_h)); 01522 if (ret != MAX77658_NO_ERROR) return ret; 01523 01524 switch (bit_field) 01525 { 01526 case CNFG_CHG_H_CHR_TH_DIS: 01527 *config = (uint8_t)reg_cnfg_chg_h.bits.chr_th_dis; 01528 break; 01529 case CNFG_CHG_H_SYS_BAT_PRT: 01530 *config = (uint8_t)reg_cnfg_chg_h.bits.sys_bat_prt; 01531 break; 01532 case CNFG_CHG_H_CHG_CV_JEITA: 01533 *config = (uint8_t)reg_cnfg_chg_h.bits.chg_cv_jeita; 01534 break; 01535 default: 01536 return MAX77658_INVALID_DATA; 01537 break; 01538 } 01539 01540 return MAX77658_NO_ERROR; 01541 } 01542 01543 int MAX77658::set_chg_cv_jeita(float voltV) 01544 { 01545 uint8_t value; 01546 reg_cnfg_chg_h_t reg_cnfg_chg_h = {0}; 01547 float voltmV = voltV * 1000; 01548 01549 if (voltmV < 3600) voltmV = 3600; 01550 else if (voltmV > 4600) voltmV = 4600; 01551 01552 value = round(voltmV - 3600) / 25; 01553 01554 SET_BIT_FIELD(CNFG_CHG_H, reg_cnfg_chg_h, reg_cnfg_chg_h.bits.chg_cv_jeita, value); 01555 return MAX77658_NO_ERROR; 01556 } 01557 01558 int MAX77658::get_chg_cv_jeita(float *voltV) 01559 { 01560 int ret; 01561 uint8_t bit_value; 01562 reg_cnfg_chg_h_t reg_cnfg_chg_h = {0}; 01563 01564 ret = read_register(CNFG_CHG_H, (uint8_t *)&(reg_cnfg_chg_h)); 01565 if (ret != MAX77658_NO_ERROR) return ret; 01566 01567 bit_value = (uint8_t)reg_cnfg_chg_h.bits.chg_cv_jeita; 01568 *voltV = (bit_value * 0.025f) + 3.6f; 01569 return MAX77658_NO_ERROR; 01570 } 01571 01572 int MAX77658::set_imon_dischg_scale(float currentmA) 01573 { 01574 uint8_t value; 01575 reg_cnfg_chg_i_t reg_cnfg_chg_i = {0}; 01576 01577 if (currentmA < 40.5f) value = 0; 01578 else if ((currentmA >= 40.5f) && (currentmA < 72.3f)) value = 1; 01579 else if ((currentmA >= 72.3f) && (currentmA < 103.4f)) value = 2; 01580 else if ((currentmA >= 103.4f) && (currentmA < 134.1f)) value = 3; 01581 else if ((currentmA >= 134.1f) && (currentmA < 164.1f)) value = 4; 01582 else if ((currentmA >= 164.1f) && (currentmA < 193.7f)) value = 5; 01583 else if ((currentmA >= 193.7f) && (currentmA < 222.7f)) value = 6; 01584 else if ((currentmA >= 222.7f) && (currentmA < 251.2f)) value = 7; 01585 else if ((currentmA >= 251.2f) && (currentmA < 279.3f)) value = 8; 01586 else if ((currentmA >= 279.3f) && (currentmA < 300.0f)) value = 9; 01587 else if (currentmA >= 300.0f) value = 10; 01588 01589 SET_BIT_FIELD(CNFG_CHG_I, reg_cnfg_chg_i, reg_cnfg_chg_i.bits.imon_dischg_scale, value); 01590 return MAX77658_NO_ERROR; 01591 } 01592 01593 int MAX77658::get_imon_dischg_scale(float *currentmA) 01594 { 01595 int ret; 01596 uint8_t bit_value; 01597 reg_cnfg_chg_i_t reg_cnfg_chg_i = {0}; 01598 01599 ret = read_register(CNFG_CHG_I, (uint8_t *)&(reg_cnfg_chg_i)); 01600 if (ret != MAX77658_NO_ERROR) { 01601 return ret; 01602 } 01603 01604 bit_value = (uint8_t)reg_cnfg_chg_i.bits.imon_dischg_scale; 01605 01606 if (bit_value == 0) *currentmA = 8.2f; 01607 else if (bit_value == 1) *currentmA = 40.5f; 01608 else if (bit_value == 2) *currentmA = 72.3f; 01609 else if (bit_value == 3) *currentmA = 103.4f; 01610 else if (bit_value == 4) *currentmA = 134.1f; 01611 else if (bit_value == 5) *currentmA = 164.1f; 01612 else if (bit_value == 6) *currentmA = 193.7f; 01613 else if (bit_value == 7) *currentmA = 222.7f; 01614 else if (bit_value == 8) *currentmA = 251.2f; 01615 else if (bit_value == 9) *currentmA = 279.3f; 01616 else *currentmA = 300.0f; //0xA to 0xF = 300.0mA 01617 01618 return MAX77658_NO_ERROR; 01619 } 01620 01621 int MAX77658::set_mux_sel(decode_mux_sel_t selection) 01622 { 01623 reg_cnfg_chg_i_t reg_cnfg_chg_i = {0}; 01624 01625 SET_BIT_FIELD(CNFG_CHG_I, reg_cnfg_chg_i, reg_cnfg_chg_i.bits.mux_sel, selection); 01626 return MAX77658_NO_ERROR; 01627 } 01628 01629 int MAX77658::get_mux_sel(decode_mux_sel_t *selection) 01630 { 01631 int ret; 01632 reg_cnfg_chg_i_t reg_cnfg_chg_i = {0}; 01633 01634 ret = read_register(CNFG_CHG_I, (uint8_t *)&(reg_cnfg_chg_i)); 01635 if (ret != MAX77658_NO_ERROR) return ret; 01636 01637 *selection = (decode_mux_sel_t)reg_cnfg_chg_i.bits.mux_sel; 01638 return MAX77658_NO_ERROR; 01639 } 01640 01641 int MAX77658::set_cnfg_sbb_top(reg_bit_cnfg_sbb_top_t bit_field, uint8_t config) 01642 { 01643 int ret; 01644 reg_cnfg_sbb_top_t reg_cnfg_sbb_top = {0}; 01645 01646 ret = read_register(CNFG_SBB_TOP, (uint8_t *)&(reg_cnfg_sbb_top)); 01647 if (ret != MAX77658_NO_ERROR) return ret; 01648 01649 switch (bit_field) 01650 { 01651 case CNFG_SBB_TOP_DRV_SBB: 01652 reg_cnfg_sbb_top.bits.drv_sbb = config; 01653 break; 01654 case CNFG_SBB_TOP_DIS_LPM: 01655 reg_cnfg_sbb_top.bits.dis_lpm = config; 01656 break; 01657 default: 01658 return MAX77658_INVALID_DATA; 01659 break; 01660 } 01661 01662 return write_register(CNFG_SBB_TOP, (uint8_t *)&(reg_cnfg_sbb_top)); 01663 } 01664 01665 int MAX77658::get_cnfg_sbb_top(reg_bit_cnfg_sbb_top_t bit_field, uint8_t *config) 01666 { 01667 int ret; 01668 reg_cnfg_sbb_top_t reg_cnfg_sbb_top = {0}; 01669 01670 ret = read_register(CNFG_SBB_TOP, (uint8_t *)&(reg_cnfg_sbb_top)); 01671 if (ret != MAX77658_NO_ERROR) return ret; 01672 01673 switch (bit_field) 01674 { 01675 case CNFG_SBB_TOP_DRV_SBB: 01676 *config = (uint8_t)reg_cnfg_sbb_top.bits.drv_sbb; 01677 break; 01678 case CNFG_SBB_TOP_DIS_LPM: 01679 *config = (uint8_t)reg_cnfg_sbb_top.bits.dis_lpm; 01680 break; 01681 default: 01682 return MAX77658_INVALID_DATA; 01683 break; 01684 } 01685 01686 return MAX77658_NO_ERROR; 01687 } 01688 01689 int MAX77658::set_tv_sbb_a(uint8_t channel, float voltV) 01690 { 01691 uint8_t value; 01692 reg_cnfg_sbb0_a_t reg_cnfg_sbb0_a = {0}; 01693 reg_cnfg_sbb1_a_t reg_cnfg_sbb1_a = {0}; 01694 reg_cnfg_sbb2_a_t reg_cnfg_sbb2_a = {0}; 01695 float voltmV = voltV * 1000; 01696 01697 if (voltmV < 500) voltmV = 500; 01698 else if (voltmV > 5500) voltmV = 5500; 01699 01700 value = (voltmV - 500) / 25; 01701 01702 if (channel == 0) { 01703 SET_BIT_FIELD(CNFG_SBB0_A, reg_cnfg_sbb0_a, reg_cnfg_sbb0_a.bits.tv_sbb0, value); 01704 } 01705 else if (channel == 1) { 01706 SET_BIT_FIELD(CNFG_SBB1_A, reg_cnfg_sbb1_a, reg_cnfg_sbb1_a.bits.tv_sbb1, value); 01707 } 01708 else if (channel == 2) { 01709 SET_BIT_FIELD(CNFG_SBB2_A, reg_cnfg_sbb2_a, reg_cnfg_sbb2_a.bits.tv_sbb2, value); 01710 } 01711 else { 01712 return MAX77658_INVALID_DATA; 01713 } 01714 01715 return MAX77658_NO_ERROR; 01716 } 01717 01718 int MAX77658::get_tv_sbb_a(uint8_t channel, float *voltV) 01719 { 01720 int ret; 01721 uint8_t bit_value; 01722 reg_cnfg_sbb0_a_t reg_cnfg_sbb0_a = {0}; 01723 reg_cnfg_sbb1_a_t reg_cnfg_sbb1_a = {0}; 01724 reg_cnfg_sbb2_a_t reg_cnfg_sbb2_a = {0}; 01725 01726 if (channel == 0) { 01727 ret = read_register(CNFG_SBB0_A, (uint8_t *)&(reg_cnfg_sbb0_a)); 01728 if (ret != MAX77658_NO_ERROR) return ret; 01729 01730 bit_value = (uint8_t)reg_cnfg_sbb0_a.bits.tv_sbb0; 01731 } 01732 else if (channel == 1) { 01733 ret = read_register(CNFG_SBB1_A, (uint8_t *)&(reg_cnfg_sbb1_a)); 01734 if (ret != MAX77658_NO_ERROR) return ret; 01735 01736 bit_value = (uint8_t)reg_cnfg_sbb1_a.bits.tv_sbb1; 01737 } 01738 else if (channel == 2) { 01739 ret = read_register(CNFG_SBB2_A, (uint8_t *)&(reg_cnfg_sbb2_a)); 01740 if (ret != MAX77658_NO_ERROR) return ret; 01741 01742 bit_value = (uint8_t)reg_cnfg_sbb2_a.bits.tv_sbb2; 01743 } 01744 else return MAX77658_INVALID_DATA; 01745 01746 if (bit_value > 200) bit_value = 200; 01747 *voltV = (bit_value * 0.025f) + 0.5f; 01748 01749 return MAX77658_NO_ERROR; 01750 } 01751 01752 int MAX77658::set_op_mode(uint8_t channel, decode_op_mode_t mode) 01753 { 01754 reg_cnfg_sbb0_b_t reg_cnfg_sbb0_b = {0}; 01755 reg_cnfg_sbb1_b_t reg_cnfg_sbb1_b = {0}; 01756 reg_cnfg_sbb2_b_t reg_cnfg_sbb2_b = {0}; 01757 01758 if (channel == 0) { 01759 SET_BIT_FIELD(CNFG_SBB0_B, reg_cnfg_sbb0_b, reg_cnfg_sbb0_b.bits.op_mode0, mode); 01760 } 01761 else if (channel == 1) { 01762 SET_BIT_FIELD(CNFG_SBB1_B, reg_cnfg_sbb1_b, reg_cnfg_sbb1_b.bits.op_mode1, mode); 01763 } 01764 else if (channel == 2) { 01765 SET_BIT_FIELD(CNFG_SBB2_B, reg_cnfg_sbb2_b, reg_cnfg_sbb2_b.bits.op_mode2, mode); 01766 } 01767 else { 01768 return MAX77658_INVALID_DATA; 01769 } 01770 01771 return MAX77658_NO_ERROR; 01772 } 01773 01774 int MAX77658::get_op_mode(uint8_t channel, decode_op_mode_t *mode) 01775 { 01776 int ret; 01777 reg_cnfg_sbb0_b_t reg_cnfg_sbb0_b = {0}; 01778 reg_cnfg_sbb1_b_t reg_cnfg_sbb1_b = {0}; 01779 reg_cnfg_sbb2_b_t reg_cnfg_sbb2_b = {0}; 01780 01781 if (channel == 0) { 01782 ret = read_register(CNFG_SBB0_B, (uint8_t *)&(reg_cnfg_sbb0_b)); 01783 if (ret != MAX77658_NO_ERROR) return ret; 01784 01785 *mode = (decode_op_mode_t)reg_cnfg_sbb0_b.bits.op_mode0; 01786 } 01787 else if (channel == 1) { 01788 ret = read_register(CNFG_SBB1_B, (uint8_t *)&(reg_cnfg_sbb1_b)); 01789 if (ret != MAX77658_NO_ERROR) return ret; 01790 01791 *mode = (decode_op_mode_t)reg_cnfg_sbb1_b.bits.op_mode1; 01792 } 01793 else if (channel == 2) { 01794 ret = read_register(CNFG_SBB2_B, (uint8_t *)&(reg_cnfg_sbb2_b)); 01795 if (ret != MAX77658_NO_ERROR) return ret; 01796 01797 *mode = (decode_op_mode_t)reg_cnfg_sbb2_b.bits.op_mode2; 01798 } 01799 else { 01800 return MAX77658_INVALID_DATA; 01801 } 01802 01803 return MAX77658_NO_ERROR; 01804 } 01805 01806 int MAX77658::set_ip_sbb(uint8_t channel, decode_ip_sbb_t ip_sbb) 01807 { 01808 reg_cnfg_sbb0_b_t reg_cnfg_sbb0_b = {0}; 01809 reg_cnfg_sbb1_b_t reg_cnfg_sbb1_b = {0}; 01810 reg_cnfg_sbb2_b_t reg_cnfg_sbb2_b = {0}; 01811 01812 if (channel == 0) { 01813 SET_BIT_FIELD(CNFG_SBB0_B, reg_cnfg_sbb0_b, reg_cnfg_sbb0_b.bits.ip_sbb0, ip_sbb); 01814 } 01815 else if (channel == 1) { 01816 SET_BIT_FIELD(CNFG_SBB1_B, reg_cnfg_sbb1_b, reg_cnfg_sbb1_b.bits.ip_sbb1, ip_sbb); 01817 } 01818 else if (channel == 2) { 01819 SET_BIT_FIELD(CNFG_SBB2_B, reg_cnfg_sbb2_b, reg_cnfg_sbb2_b.bits.ip_sbb2, ip_sbb); 01820 } 01821 else { 01822 return MAX77658_INVALID_DATA; 01823 } 01824 01825 return MAX77658_NO_ERROR; 01826 } 01827 01828 int MAX77658::get_ip_sbb(uint8_t channel, decode_ip_sbb_t *ip_sbb) 01829 { 01830 int ret; 01831 reg_cnfg_sbb0_b_t reg_cnfg_sbb0_b = {0}; 01832 reg_cnfg_sbb1_b_t reg_cnfg_sbb1_b = {0}; 01833 reg_cnfg_sbb2_b_t reg_cnfg_sbb2_b = {0}; 01834 01835 if (channel == 0) { 01836 ret = read_register(CNFG_SBB0_B, (uint8_t *)&(reg_cnfg_sbb0_b)); 01837 if (ret != MAX77658_NO_ERROR) return ret; 01838 01839 *ip_sbb = (decode_ip_sbb_t)reg_cnfg_sbb0_b.bits.ip_sbb0; 01840 } 01841 else if (channel == 1) { 01842 ret = read_register(CNFG_SBB1_B, (uint8_t *)&(reg_cnfg_sbb1_b)); 01843 if (ret != MAX77658_NO_ERROR) return ret; 01844 01845 *ip_sbb = (decode_ip_sbb_t)reg_cnfg_sbb1_b.bits.ip_sbb1; 01846 } 01847 else if (channel == 2) { 01848 ret = read_register(CNFG_SBB2_B, (uint8_t *)&(reg_cnfg_sbb2_b)); 01849 if (ret != MAX77658_NO_ERROR) return ret; 01850 01851 *ip_sbb = (decode_ip_sbb_t)reg_cnfg_sbb2_b.bits.ip_sbb2; 01852 } 01853 else { 01854 return MAX77658_INVALID_DATA; 01855 } 01856 01857 return MAX77658_NO_ERROR; 01858 } 01859 01860 int MAX77658::set_ade_sbb(uint8_t channel, decode_ade_sbb_t ade_sbb) 01861 { 01862 reg_cnfg_sbb0_b_t reg_cnfg_sbb0_b = {0}; 01863 reg_cnfg_sbb1_b_t reg_cnfg_sbb1_b = {0}; 01864 reg_cnfg_sbb2_b_t reg_cnfg_sbb2_b = {0}; 01865 01866 if (channel == 0) { 01867 SET_BIT_FIELD(CNFG_SBB0_B, reg_cnfg_sbb0_b, reg_cnfg_sbb0_b.bits.ade_sbb0, ade_sbb); 01868 } 01869 else if (channel == 1) { 01870 SET_BIT_FIELD(CNFG_SBB1_B, reg_cnfg_sbb1_b, reg_cnfg_sbb1_b.bits.ade_sbb1, ade_sbb); 01871 } 01872 else if (channel == 2) { 01873 SET_BIT_FIELD(CNFG_SBB2_B, reg_cnfg_sbb2_b, reg_cnfg_sbb2_b.bits.ade_sbb2, ade_sbb); 01874 } 01875 else { 01876 return MAX77658_INVALID_DATA; 01877 } 01878 01879 return MAX77658_NO_ERROR; 01880 } 01881 01882 int MAX77658::get_ade_sbb(uint8_t channel, decode_ade_sbb_t *ade_sbb) 01883 { 01884 int ret; 01885 reg_cnfg_sbb0_b_t reg_cnfg_sbb0_b = {0}; 01886 reg_cnfg_sbb1_b_t reg_cnfg_sbb1_b = {0}; 01887 reg_cnfg_sbb2_b_t reg_cnfg_sbb2_b = {0}; 01888 01889 if (channel == 0) { 01890 ret = read_register(CNFG_SBB0_B, (uint8_t *)&(reg_cnfg_sbb0_b)); 01891 if (ret != MAX77658_NO_ERROR) return ret; 01892 01893 *ade_sbb = (decode_ade_sbb_t)reg_cnfg_sbb0_b.bits.ade_sbb0; 01894 } 01895 else if (channel == 1) { 01896 ret = read_register(CNFG_SBB1_B, (uint8_t *)&(reg_cnfg_sbb1_b)); 01897 if (ret != MAX77658_NO_ERROR) return ret; 01898 01899 *ade_sbb = (decode_ade_sbb_t)reg_cnfg_sbb1_b.bits.ade_sbb1; 01900 } 01901 else if (channel == 2) { 01902 ret = read_register(CNFG_SBB2_B, (uint8_t *)&(reg_cnfg_sbb2_b)); 01903 if (ret != MAX77658_NO_ERROR) return ret; 01904 01905 *ade_sbb = (decode_ade_sbb_t)reg_cnfg_sbb2_b.bits.ade_sbb2; 01906 } 01907 else { 01908 return MAX77658_INVALID_DATA; 01909 } 01910 01911 return MAX77658_NO_ERROR; 01912 } 01913 01914 int MAX77658::set_en_sbb(uint8_t channel, decode_en_sbb_t en_sbb) 01915 { 01916 reg_cnfg_sbb0_b_t reg_cnfg_sbb0_b = {0}; 01917 reg_cnfg_sbb1_b_t reg_cnfg_sbb1_b = {0}; 01918 reg_cnfg_sbb2_b_t reg_cnfg_sbb2_b = {0}; 01919 01920 if (channel == 0) { 01921 SET_BIT_FIELD(CNFG_SBB0_B, reg_cnfg_sbb0_b, reg_cnfg_sbb0_b.bits.en_sbb0, en_sbb); 01922 } 01923 else if (channel == 1) { 01924 SET_BIT_FIELD(CNFG_SBB1_B, reg_cnfg_sbb1_b, reg_cnfg_sbb1_b.bits.en_sbb1, en_sbb); 01925 } 01926 else if (channel == 2) { 01927 SET_BIT_FIELD(CNFG_SBB2_B, reg_cnfg_sbb2_b, reg_cnfg_sbb2_b.bits.en_sbb2, en_sbb); 01928 } 01929 else { 01930 return MAX77658_INVALID_DATA; 01931 } 01932 01933 return MAX77658_NO_ERROR; 01934 } 01935 01936 int MAX77658::get_en_sbb(uint8_t channel, decode_en_sbb_t *en_sbb) 01937 { 01938 int ret; 01939 reg_cnfg_sbb0_b_t reg_cnfg_sbb0_b = {0}; 01940 reg_cnfg_sbb1_b_t reg_cnfg_sbb1_b = {0}; 01941 reg_cnfg_sbb2_b_t reg_cnfg_sbb2_b = {0}; 01942 01943 if (channel == 0) { 01944 ret = read_register(CNFG_SBB0_B, (uint8_t *)&(reg_cnfg_sbb0_b)); 01945 if (ret != MAX77658_NO_ERROR) return ret; 01946 01947 *en_sbb = (decode_en_sbb_t)reg_cnfg_sbb0_b.bits.en_sbb0; 01948 } 01949 else if (channel == 1) { 01950 ret = read_register(CNFG_SBB1_B, (uint8_t *)&(reg_cnfg_sbb1_b)); 01951 if (ret != MAX77658_NO_ERROR) return ret; 01952 01953 *en_sbb = (decode_en_sbb_t)reg_cnfg_sbb1_b.bits.en_sbb1; 01954 } 01955 else if (channel == 2) { 01956 ret = read_register(CNFG_SBB2_B, (uint8_t *)&(reg_cnfg_sbb2_b)); 01957 if (ret != MAX77658_NO_ERROR) return ret; 01958 01959 *en_sbb = (decode_en_sbb_t)reg_cnfg_sbb2_b.bits.en_sbb2; 01960 } 01961 else return MAX77658_INVALID_DATA; 01962 01963 return MAX77658_NO_ERROR; 01964 } 01965 01966 int MAX77658::set_tv_sbb_dvs(float voltV) 01967 { 01968 uint8_t value; 01969 reg_cnfg_dvs_sbb0_a_t reg_cnfg_dvs_sbb0_a = {0}; 01970 float voltmV = voltV * 1000; 01971 01972 if (voltmV < 500) voltmV = 500; 01973 else if (voltmV > 5500) voltmV = 5500; 01974 01975 value = (voltmV - 500) / 25; 01976 01977 SET_BIT_FIELD(CNFG_DVS_SBB0_A, reg_cnfg_dvs_sbb0_a, reg_cnfg_dvs_sbb0_a.bits.tv_sbb0_dvs, value); 01978 return MAX77658_NO_ERROR; 01979 } 01980 01981 int MAX77658::get_tv_sbb_dvs(float *voltV) 01982 { 01983 int ret; 01984 uint8_t bit_value; 01985 reg_cnfg_dvs_sbb0_a_t reg_cnfg_dvs_sbb0_a = {0}; 01986 01987 ret = read_register(CNFG_DVS_SBB0_A, (uint8_t *)&(reg_cnfg_dvs_sbb0_a)); 01988 if (ret != MAX77658_NO_ERROR) return ret; 01989 01990 bit_value = (uint8_t)reg_cnfg_dvs_sbb0_a.bits.tv_sbb0_dvs; 01991 01992 if (bit_value > 200) bit_value = 200; 01993 01994 *voltV = (bit_value * 0.025f) + 0.5f; 01995 return MAX77658_NO_ERROR; 01996 } 01997 01998 int MAX77658::set_tv_ldo_volt_a(uint8_t channel, float voltV) 01999 { 02000 int ret; 02001 uint8_t value; 02002 reg_cnfg_ldo0_a_t reg_cnfg_ldo0_a = {0}; 02003 reg_cnfg_ldo1_a_t reg_cnfg_ldo1_a = {0}; 02004 float voltmV = voltV * 1000; 02005 02006 if (channel == 0) { 02007 ret = read_register(CNFG_LDO0_A, (uint8_t *)&(reg_cnfg_ldo0_a)); 02008 if (ret != MAX77658_NO_ERROR) return ret; 02009 02010 if ((uint8_t)reg_cnfg_ldo0_a.bits.tv_ldo0_7 == 0) { //No Offset 02011 if (voltmV < 500) voltmV = 500; 02012 else if (voltmV > 3675) voltmV = 3675; 02013 02014 value = (voltmV - 500) / 25; 02015 } 02016 else { //1.325V Offset 02017 if (voltmV < 1825) voltmV = 1825; 02018 else if (voltmV > 5000) voltmV = 5000; 02019 02020 value = (voltmV - 1825) / 25; 02021 } 02022 02023 SET_BIT_FIELD(CNFG_LDO0_A, reg_cnfg_ldo0_a, reg_cnfg_ldo0_a.bits.tv_ldo0_6_0, value); 02024 } 02025 else { 02026 ret = read_register(CNFG_LDO1_A, (uint8_t *)&(reg_cnfg_ldo1_a)); 02027 if (ret != MAX77658_NO_ERROR) return ret; 02028 02029 if ((uint8_t)reg_cnfg_ldo1_a.bits.tv_ldo1_7 == 0) { //No Offset 02030 if (voltmV < 500) voltmV = 500; 02031 else if (voltmV > 3675) voltmV = 3675; 02032 02033 value = (voltmV - 500) / 25; 02034 } 02035 else { //1.325V Offset 02036 if (voltmV < 1825) voltmV = 1825; 02037 else if (voltmV > 5000) voltmV = 5000; 02038 02039 value = (voltmV - 1825) / 25; 02040 } 02041 02042 SET_BIT_FIELD(CNFG_LDO1_A, reg_cnfg_ldo1_a, reg_cnfg_ldo1_a.bits.tv_ldo1_6_0, value); 02043 } 02044 return MAX77658_NO_ERROR; 02045 } 02046 02047 int MAX77658::get_tv_ldo_volt_a(uint8_t channel, float *voltV) 02048 { 02049 int ret; 02050 uint8_t bit_value; 02051 reg_cnfg_ldo0_a_t reg_cnfg_ldo0_a = {0}; 02052 reg_cnfg_ldo1_a_t reg_cnfg_ldo1_a = {0}; 02053 02054 if (channel == 0){ 02055 ret = read_register(CNFG_LDO0_A, (uint8_t *)&(reg_cnfg_ldo0_a)); 02056 if (ret != MAX77658_NO_ERROR) return ret; 02057 02058 bit_value = (uint8_t)reg_cnfg_ldo0_a.bits.tv_ldo0_6_0; 02059 if ((uint8_t)reg_cnfg_ldo0_a.bits.tv_ldo0_7 == 0) //No Offset 02060 *voltV = (bit_value * 0.025f) + 0.5f; 02061 else //1.325V Offset 02062 *voltV = (bit_value * 0.025f) + 1.825f; 02063 } 02064 else { 02065 ret = read_register(CNFG_LDO1_A, (uint8_t *)&(reg_cnfg_ldo1_a)); 02066 if (ret != MAX77658_NO_ERROR) return ret; 02067 02068 bit_value = (uint8_t)reg_cnfg_ldo1_a.bits.tv_ldo1_6_0; 02069 if ((uint8_t)reg_cnfg_ldo1_a.bits.tv_ldo1_7 == 0) //No Offset 02070 *voltV = (bit_value * 0.025f) + 0.5f; 02071 else //1.325V Offset 02072 *voltV = (bit_value * 0.025f) + 1.825f; 02073 } 02074 02075 return MAX77658_NO_ERROR; 02076 } 02077 02078 int MAX77658::set_tv_ldo_offset_a(uint8_t channel, decode_tv_ldo_offset_a_t offset) 02079 { 02080 reg_cnfg_ldo0_a_t reg_cnfg_ldo0_a = {0}; 02081 reg_cnfg_ldo1_a_t reg_cnfg_ldo1_a = {0}; 02082 02083 if (channel == 0) { 02084 SET_BIT_FIELD(CNFG_LDO0_A, reg_cnfg_ldo0_a, reg_cnfg_ldo0_a.bits.tv_ldo0_7, offset); 02085 } 02086 else if (channel == 1) { 02087 SET_BIT_FIELD(CNFG_LDO1_A, reg_cnfg_ldo1_a, reg_cnfg_ldo1_a.bits.tv_ldo1_7, offset); 02088 } 02089 else { 02090 return MAX77658_INVALID_DATA; 02091 } 02092 02093 return MAX77658_NO_ERROR; 02094 } 02095 02096 int MAX77658::get_tv_ldo_offset_a(uint8_t channel, decode_tv_ldo_offset_a_t *offset) 02097 { 02098 int ret; 02099 reg_cnfg_ldo0_a_t reg_cnfg_ldo0_a = {0}; 02100 reg_cnfg_ldo1_a_t reg_cnfg_ldo1_a = {0}; 02101 02102 if (channel == 0) { 02103 ret = read_register(CNFG_LDO0_A, (uint8_t *)&(reg_cnfg_ldo0_a)); 02104 if (ret != MAX77658_NO_ERROR) return ret; 02105 02106 *offset = (decode_tv_ldo_offset_a_t)reg_cnfg_ldo0_a.bits.tv_ldo0_7; 02107 } 02108 else if (channel == 1) { 02109 ret = read_register(CNFG_LDO1_A, (uint8_t *)&(reg_cnfg_ldo1_a)); 02110 if (ret != MAX77658_NO_ERROR) return ret; 02111 02112 *offset = (decode_tv_ldo_offset_a_t)reg_cnfg_ldo1_a.bits.tv_ldo1_7; 02113 } 02114 else { 02115 return MAX77658_INVALID_DATA; 02116 } 02117 02118 return MAX77658_NO_ERROR; 02119 } 02120 02121 int MAX77658::set_en_ldo(uint8_t channel, decode_en_ldo_t en_ldo) 02122 { 02123 reg_cnfg_ldo0_b_t reg_cnfg_ldo0_b = {0}; 02124 reg_cnfg_ldo1_b_t reg_cnfg_ldo1_b = {0}; 02125 02126 if (channel == 0) { 02127 SET_BIT_FIELD(CNFG_LDO0_B, reg_cnfg_ldo0_b, reg_cnfg_ldo0_b.bits.en_ldo0, en_ldo); 02128 } 02129 else if (channel == 1) { 02130 SET_BIT_FIELD(CNFG_LDO1_B, reg_cnfg_ldo1_b, reg_cnfg_ldo1_b.bits.en_ldo1, en_ldo); 02131 } 02132 else { 02133 return MAX77658_INVALID_DATA; 02134 } 02135 02136 return MAX77658_NO_ERROR; 02137 } 02138 02139 int MAX77658::get_en_ldo(uint8_t channel, decode_en_ldo_t *en_ldo) 02140 { 02141 int ret; 02142 reg_cnfg_ldo0_b_t reg_cnfg_ldo0_b = {0}; 02143 reg_cnfg_ldo1_b_t reg_cnfg_ldo1_b = {0}; 02144 02145 if (channel == 0) { 02146 ret = read_register(CNFG_LDO0_B, (uint8_t *)&(reg_cnfg_ldo0_b)); 02147 if (ret != MAX77658_NO_ERROR) return ret; 02148 02149 *en_ldo = (decode_en_ldo_t)reg_cnfg_ldo0_b.bits.en_ldo0; 02150 } 02151 else if (channel == 1) { 02152 ret = read_register(CNFG_LDO1_B, (uint8_t *)&(reg_cnfg_ldo1_b)); 02153 if (ret != MAX77658_NO_ERROR) return ret; 02154 02155 *en_ldo = (decode_en_ldo_t)reg_cnfg_ldo1_b.bits.en_ldo1; 02156 } 02157 else return MAX77658_INVALID_DATA; 02158 02159 return MAX77658_NO_ERROR; 02160 } 02161 02162 int MAX77658::set_ade_ldo(uint8_t channel, decode_ade_ldo_t ade_ldo) 02163 { 02164 reg_cnfg_ldo0_b_t reg_cnfg_ldo0_b = {0}; 02165 reg_cnfg_ldo1_b_t reg_cnfg_ldo1_b = {0}; 02166 02167 if (channel == 0) { 02168 SET_BIT_FIELD(CNFG_LDO0_B, reg_cnfg_ldo0_b, reg_cnfg_ldo0_b.bits.ade_ldo0, ade_ldo); 02169 } 02170 else if (channel == 1) { 02171 SET_BIT_FIELD(CNFG_LDO1_B, reg_cnfg_ldo1_b, reg_cnfg_ldo1_b.bits.ade_ldo1, ade_ldo); 02172 } 02173 else { 02174 return MAX77658_INVALID_DATA; 02175 } 02176 02177 return MAX77658_NO_ERROR; 02178 } 02179 02180 int MAX77658::get_ade_ldo(uint8_t channel, decode_ade_ldo_t *ade_ldo) 02181 { 02182 int ret; 02183 reg_cnfg_ldo0_b_t reg_cnfg_ldo0_b = {0}; 02184 reg_cnfg_ldo1_b_t reg_cnfg_ldo1_b = {0}; 02185 02186 if (channel == 0) { 02187 ret = read_register(CNFG_LDO0_B, (uint8_t *)&(reg_cnfg_ldo0_b)); 02188 if (ret != MAX77658_NO_ERROR) return ret; 02189 02190 *ade_ldo = (decode_ade_ldo_t)reg_cnfg_ldo0_b.bits.ade_ldo0; 02191 } 02192 else if (channel == 1) { 02193 ret = read_register(CNFG_LDO1_B, (uint8_t *)&(reg_cnfg_ldo1_b)); 02194 if (ret != MAX77658_NO_ERROR) return ret; 02195 02196 *ade_ldo = (decode_ade_ldo_t)reg_cnfg_ldo1_b.bits.ade_ldo1; 02197 } 02198 else { 02199 return MAX77658_INVALID_DATA; 02200 } 02201 02202 return MAX77658_NO_ERROR; 02203 } 02204 02205 int MAX77658::set_ldo_md(uint8_t channel, decode_ldo_md_t mode) 02206 { 02207 reg_cnfg_ldo0_b_t reg_cnfg_ldo0_b = {0}; 02208 reg_cnfg_ldo1_b_t reg_cnfg_ldo1_b = {0}; 02209 02210 if (channel == 0) { 02211 SET_BIT_FIELD(CNFG_LDO0_B, reg_cnfg_ldo0_b, reg_cnfg_ldo0_b.bits.ldo0_md, mode); 02212 } 02213 else if (channel == 1) { 02214 SET_BIT_FIELD(CNFG_LDO1_B, reg_cnfg_ldo1_b, reg_cnfg_ldo1_b.bits.ldo1_md, mode); 02215 } 02216 else { 02217 return MAX77658_INVALID_DATA; 02218 } 02219 02220 return MAX77658_NO_ERROR; 02221 } 02222 02223 int MAX77658::get_ldo_md(uint8_t channel, decode_ldo_md_t *mode) 02224 { 02225 int ret; 02226 reg_cnfg_ldo0_b_t reg_cnfg_ldo0_b = {0}; 02227 reg_cnfg_ldo1_b_t reg_cnfg_ldo1_b = {0}; 02228 02229 if (channel == 0) { 02230 ret = read_register(CNFG_LDO0_B, (uint8_t *)&(reg_cnfg_ldo0_b)); 02231 if (ret != MAX77658_NO_ERROR) return ret; 02232 02233 *mode = (decode_ldo_md_t)reg_cnfg_ldo0_b.bits.ldo0_md; 02234 } 02235 else if (channel == 1) { 02236 ret = read_register(CNFG_LDO1_B, (uint8_t *)&(reg_cnfg_ldo1_b)); 02237 if (ret != MAX77658_NO_ERROR) return ret; 02238 02239 *mode = (decode_ldo_md_t)reg_cnfg_ldo1_b.bits.ldo1_md; 02240 } 02241 else { 02242 return MAX77658_INVALID_DATA; 02243 } 02244 02245 return MAX77658_NO_ERROR; 02246 } 02247 02248 int MAX77658::set_fg_status(reg_bit_status_t bit_field, uint8_t status) 02249 { 02250 int ret; 02251 reg_status_t reg_status = {0}; 02252 02253 ret = read_fg_register(Status, (uint8_t *)&(reg_status)); 02254 if (ret != MAX77658_NO_ERROR) return ret; 02255 02256 switch (bit_field) 02257 { 02258 case Status_Imn: 02259 reg_status.bits.imn = status; 02260 break; 02261 case Status_POR: 02262 reg_status.bits.por = status; 02263 break; 02264 case Status_SPR_2: 02265 reg_status.bits.spr_2 = status; 02266 break; 02267 case Status_Bst: 02268 reg_status.bits.bst = status; 02269 break; 02270 case Status_Isysmx: 02271 reg_status.bits.isysmx = status; 02272 break; 02273 case Status_SPR_5: 02274 reg_status.bits.spr_5 = status; 02275 break; 02276 case Status_ThmHot: 02277 reg_status.bits.thmhot = status; 02278 break; 02279 case Status_dSOCi: 02280 reg_status.bits.dsoci = status; 02281 break; 02282 case Status_Vmn: 02283 reg_status.bits.vmn = status; 02284 break; 02285 case Status_Tmn: 02286 reg_status.bits.tmn = status; 02287 break; 02288 case Status_Smn: 02289 reg_status.bits.smn = status; 02290 break; 02291 case Status_Bi: 02292 reg_status.bits.bi = status; 02293 break; 02294 case Status_Vmx: 02295 reg_status.bits.vmx = status; 02296 break; 02297 case Status_Tmx: 02298 reg_status.bits.tmx = status; 02299 break; 02300 case Status_Smx: 02301 reg_status.bits.smx = status; 02302 break; 02303 case Status_Br: 02304 reg_status.bits.br = status; 02305 break; 02306 default: 02307 return MAX77658_INVALID_DATA; 02308 break; 02309 } 02310 02311 return write_fg_register(Status, (uint8_t *)&(reg_status)); 02312 } 02313 02314 int MAX77658::get_fg_status(reg_bit_status_t bit_field, uint8_t *status) 02315 { 02316 int ret; 02317 reg_status_t reg_status = {0}; 02318 02319 ret = read_fg_register(Status, (uint8_t *)&(reg_status)); 02320 if (ret != MAX77658_NO_ERROR) return ret; 02321 02322 switch (bit_field) 02323 { 02324 case Status_Imn: 02325 *status = (uint8_t)reg_status.bits.imn; 02326 break; 02327 case Status_POR: 02328 *status = (uint8_t)reg_status.bits.por; 02329 break; 02330 case Status_SPR_2: 02331 *status = (uint8_t)reg_status.bits.spr_2; 02332 break; 02333 case Status_Bst: 02334 *status = (uint8_t)reg_status.bits.bst; 02335 break; 02336 case Status_Isysmx: 02337 *status = (uint8_t)reg_status.bits.isysmx; 02338 break; 02339 case Status_SPR_5: 02340 *status = (uint8_t)reg_status.bits.spr_5; 02341 break; 02342 case Status_ThmHot: 02343 *status = (uint8_t)reg_status.bits.thmhot; 02344 break; 02345 case Status_dSOCi: 02346 *status = (uint8_t)reg_status.bits.dsoci; 02347 break; 02348 case Status_Vmn: 02349 *status = (uint8_t)reg_status.bits.vmn; 02350 break; 02351 case Status_Tmn: 02352 *status = (uint8_t)reg_status.bits.tmn; 02353 break; 02354 case Status_Smn: 02355 *status = (uint8_t)reg_status.bits.smn; 02356 break; 02357 case Status_Bi: 02358 *status = (uint8_t)reg_status.bits.bi; 02359 break; 02360 case Status_Vmx: 02361 *status = (uint8_t)reg_status.bits.vmx; 02362 break; 02363 case Status_Tmx: 02364 *status = (uint8_t)reg_status.bits.tmx; 02365 break; 02366 case Status_Smx: 02367 *status = (uint8_t)reg_status.bits.smx; 02368 break; 02369 case Status_Br: 02370 *status = (uint8_t)reg_status.bits.br; 02371 break; 02372 default: 02373 return MAX77658_INVALID_DATA; 02374 break; 02375 } 02376 02377 return MAX77658_NO_ERROR; 02378 } 02379 02380 int MAX77658::set_fg_valrt_th(reg_bit_valrt_th_t bit_field, float voltV) 02381 { 02382 int ret; 02383 uint8_t voltRaw; 02384 reg_valrt_th_t reg_valrt_th = {0}; 02385 float voltmV = voltV * 1000; 02386 02387 ret = read_fg_register(VAlrtTh, (uint8_t *)&(reg_valrt_th)); 02388 if (ret != MAX77658_NO_ERROR) return ret; 02389 02390 //20mV resolution 02391 voltRaw = (int)(voltmV / 20) & TO_UINT8; 02392 02393 switch (bit_field) 02394 { 02395 case VAlrtTh_MinVoltageAlrt: 02396 reg_valrt_th.bits.min_voltage_alrt = voltRaw; 02397 break; 02398 case VAlrtTh_MaxVoltageAlrt: 02399 reg_valrt_th.bits.max_voltage_alrt = voltRaw; 02400 break; 02401 default: 02402 return MAX77658_INVALID_DATA; 02403 break; 02404 } 02405 02406 return write_fg_register(VAlrtTh, (uint8_t *)&(reg_valrt_th)); 02407 } 02408 02409 int MAX77658::get_fg_valrt_th(reg_bit_valrt_th_t bit_field, float *voltV) 02410 { 02411 int ret; 02412 int8_t voltSigned; 02413 reg_valrt_th_t reg_valrt_th = {0}; 02414 02415 ret = read_fg_register(VAlrtTh, (uint8_t *)&(reg_valrt_th)); 02416 if (ret != MAX77658_NO_ERROR) return ret; 02417 02418 switch (bit_field) 02419 { 02420 case VAlrtTh_MinVoltageAlrt: 02421 voltSigned = (int8_t)reg_valrt_th.bits.min_voltage_alrt; 02422 break; 02423 case VAlrtTh_MaxVoltageAlrt: 02424 voltSigned = (int8_t)reg_valrt_th.bits.max_voltage_alrt; 02425 break; 02426 default: 02427 return MAX77658_INVALID_DATA; 02428 break; 02429 } 02430 02431 //20mV resolution 02432 *voltV = (float)((voltSigned * 20.0f) / 1000.0f); 02433 02434 return MAX77658_NO_ERROR; 02435 } 02436 02437 int MAX77658::set_fg_talrt_th(reg_bit_talrt_th_t bit_field, int tempDegC) 02438 { 02439 int ret; 02440 uint8_t tempRaw; 02441 reg_talrt_th_t reg_talrt_th = {0}; 02442 02443 ret = read_fg_register(TAlrtTh, (uint8_t *)&(reg_talrt_th)); 02444 if (ret != MAX77658_NO_ERROR) return ret; 02445 02446 //1°C resolution 02447 tempRaw = tempDegC & TO_UINT8; 02448 02449 switch (bit_field) 02450 { 02451 case TAlrtTh_MinTempAlrt: 02452 if(tempRaw < 128) tempRaw = 128; 02453 reg_talrt_th.bits.min_temp_alrt = tempRaw; 02454 break; 02455 case TAlrtTh_MaxTempAlrt: 02456 if(tempRaw > 127) tempRaw = 127; 02457 reg_talrt_th.bits.max_temp_alrt = tempRaw; 02458 break; 02459 default: 02460 return MAX77658_INVALID_DATA; 02461 break; 02462 } 02463 02464 return write_fg_register(TAlrtTh, (uint8_t *)&(reg_talrt_th)); 02465 } 02466 02467 int MAX77658::get_fg_talrt_th(reg_bit_talrt_th_t bit_field, int *tempDegC) 02468 { 02469 int ret, tempSigned; 02470 reg_talrt_th_t reg_talrt_th = {0}; 02471 02472 ret = read_fg_register(TAlrtTh, (uint8_t *)&(reg_talrt_th)); 02473 if (ret != MAX77658_NO_ERROR) return ret; 02474 02475 switch (bit_field) 02476 { 02477 case TAlrtTh_MinTempAlrt: 02478 tempSigned = (int)reg_talrt_th.bits.min_temp_alrt; 02479 if(tempSigned < -128) tempSigned = -128; 02480 break; 02481 case TAlrtTh_MaxTempAlrt: 02482 tempSigned = (int)reg_talrt_th.bits.max_temp_alrt; 02483 if(tempSigned > 127) tempSigned = 127; 02484 break; 02485 default: 02486 return MAX77658_INVALID_DATA; 02487 break; 02488 } 02489 02490 //1°C resolution 02491 *tempDegC = (int)tempSigned & 0xFF; 02492 02493 return MAX77658_NO_ERROR; 02494 } 02495 02496 int MAX77658::set_fg_salrt_th(reg_bit_salrt_th_t bit_field, uint8_t soc) 02497 { 02498 int ret; 02499 uint8_t capRaw; 02500 reg_salrt_th_t reg_salrt_th = {0}; 02501 02502 ret = read_fg_register(SAlrtTh, (uint8_t *)&(reg_salrt_th)); 02503 if (ret != MAX77658_NO_ERROR) return ret; 02504 02505 //%1 resolution 02506 capRaw = (uint8_t)(soc); 02507 02508 switch (bit_field) 02509 { 02510 case SAlrtTh_MinSocAlrt: 02511 reg_salrt_th.bits.min_soc_alrt = capRaw; 02512 break; 02513 case SAlrtTh_MaxSocAlrt: 02514 reg_salrt_th.bits.max_soc_alrt = capRaw; 02515 break; 02516 default: 02517 return MAX77658_INVALID_DATA; 02518 break; 02519 } 02520 02521 return write_fg_register(SAlrtTh, (uint8_t *)&(reg_salrt_th)); 02522 } 02523 02524 int MAX77658::get_fg_salrt_th(reg_bit_salrt_th_t bit_field, uint8_t *soc) 02525 { 02526 int ret; 02527 uint8_t capRaw; 02528 reg_salrt_th_t reg_salrt_th = {0}; 02529 02530 ret = read_fg_register(SAlrtTh, (uint8_t *)&(reg_salrt_th)); 02531 if (ret != MAX77658_NO_ERROR) return ret; 02532 02533 switch (bit_field) 02534 { 02535 case SAlrtTh_MinSocAlrt: 02536 capRaw = (uint8_t)reg_salrt_th.bits.min_soc_alrt; 02537 break; 02538 case SAlrtTh_MaxSocAlrt: 02539 capRaw = (uint8_t)reg_salrt_th.bits.max_soc_alrt; 02540 break; 02541 default: 02542 return MAX77658_INVALID_DATA; 02543 break; 02544 } 02545 02546 //%1 resolution 02547 *soc = (uint8_t)(capRaw); 02548 02549 return MAX77658_NO_ERROR; 02550 } 02551 02552 int MAX77658::set_fg_full_soc_thr(float soc_thr) 02553 { 02554 int capRaw; 02555 reg_full_soc_thr_t reg_full_soc_thr = {0}; 02556 02557 //LSB unit is 1/256%. 02558 capRaw = (int)round(soc_thr * 256); 02559 02560 SET_FG_BIT_FIELD(FullSocThr, reg_full_soc_thr, reg_full_soc_thr.bits.full_soc_thr, capRaw); 02561 return MAX77658_NO_ERROR; 02562 } 02563 02564 int MAX77658::get_fg_full_soc_thr(float *soc_thr) 02565 { 02566 int ret, capRaw; 02567 reg_full_soc_thr_t reg_full_soc_thr = {0}; 02568 02569 ret = read_fg_register(FullSocThr, (uint8_t *)&(reg_full_soc_thr)); 02570 if (ret != MAX77658_NO_ERROR) return ret; 02571 02572 //LSB unit is 1/256%. 02573 capRaw = (int)reg_full_soc_thr.bits.full_soc_thr; 02574 *soc_thr = (float)(capRaw / 256.0f); 02575 02576 return MAX77658_NO_ERROR; 02577 } 02578 02579 int MAX77658::set_fg_design_cap(float capacitymAh) 02580 { 02581 int capRaw; 02582 reg_design_cap_t reg_design_cap = {0}; 02583 02584 //Min is 0.0mAh and Max is 6553.5mAh. 02585 if (capacitymAh < 0) capacitymAh = 0; 02586 else if (capacitymAh > 6553.5f) capacitymAh = 6553.5; 02587 02588 //LSB unit is 0.1mAh. 02589 capRaw = (int)(capacitymAh * 10); 02590 02591 SET_FG_BIT_FIELD(DesignCap, reg_design_cap, reg_design_cap.bits.design_cap, capRaw); 02592 return MAX77658_NO_ERROR; 02593 } 02594 02595 int MAX77658::get_fg_design_cap(float *capacitymAh) 02596 { 02597 int ret, capRaw; 02598 reg_design_cap_t reg_design_cap = {0}; 02599 02600 ret = read_fg_register(DesignCap, (uint8_t *)&(reg_design_cap)); 02601 if (ret != MAX77658_NO_ERROR) return ret; 02602 02603 //LSB unit is 0.1mAh. Min is 0.0mAh and Max is 6553.5mAh. 02604 capRaw = (int)reg_design_cap.bits.design_cap; 02605 *capacitymAh = (float)(capRaw * 0.1f); 02606 02607 return MAX77658_NO_ERROR; 02608 } 02609 02610 int MAX77658::set_fg_config(reg_bit_config_t bit_field, uint8_t config) 02611 { 02612 int ret; 02613 reg_config_t reg_config = {0}; 02614 02615 ret = read_fg_register(Config, (uint8_t *)&(reg_config)); 02616 if (ret != MAX77658_NO_ERROR) return ret; 02617 02618 switch (bit_field) 02619 { 02620 case Config_Ber: 02621 reg_config.bits.ber = config; 02622 break; 02623 case Config_Bei: 02624 reg_config.bits.bei = config; 02625 break; 02626 case Config_Aen: 02627 reg_config.bits.aen = config; 02628 break; 02629 case Config_FTHRM: 02630 reg_config.bits.fthrm = config; 02631 break; 02632 case Config_ETHRM: 02633 reg_config.bits.ethrm = config; 02634 break; 02635 case Config_SPR_5: 02636 reg_config.bits.spr_5 = config; 02637 break; 02638 case Config_I2CSH: 02639 reg_config.bits.i2csh = config; 02640 break; 02641 case Config_SHDN: 02642 reg_config.bits.shdn = config; 02643 break; 02644 case Config_Tex: 02645 reg_config.bits.tex = config; 02646 break; 02647 case Config_Ten: 02648 reg_config.bits.ten = config; 02649 break; 02650 case Config_AINSH: 02651 reg_config.bits.ainsh = config; 02652 break; 02653 case Config_SPR_11: 02654 reg_config.bits.spr_11 = config; 02655 break; 02656 case Config_Vs: 02657 reg_config.bits.vs = config; 02658 break; 02659 case Config_Ts: 02660 reg_config.bits.ts = config; 02661 break; 02662 case Config_Ss: 02663 reg_config.bits.ss = config; 02664 break; 02665 case Config_SPR_15: 02666 reg_config.bits.spr_15 = config; 02667 break; 02668 default: 02669 return MAX77658_INVALID_DATA; 02670 break; 02671 } 02672 02673 return write_fg_register(Config, (uint8_t *)&(reg_config)); 02674 } 02675 02676 int MAX77658::get_fg_config(reg_bit_config_t bit_field, uint8_t *config) 02677 { 02678 int ret; 02679 reg_config_t reg_config = {0}; 02680 02681 ret = read_fg_register(Config, (uint8_t *)&(reg_config)); 02682 if (ret != MAX77658_NO_ERROR) return ret; 02683 02684 switch (bit_field) 02685 { 02686 case Config_Ber: 02687 *config = (uint8_t)reg_config.bits.ber; 02688 break; 02689 case Config_Bei: 02690 *config = (uint8_t)reg_config.bits.bei; 02691 break; 02692 case Config_Aen: 02693 *config = (uint8_t)reg_config.bits.aen; 02694 break; 02695 case Config_FTHRM: 02696 *config = (uint8_t)reg_config.bits.fthrm; 02697 break; 02698 case Config_ETHRM: 02699 *config = (uint8_t)reg_config.bits.ethrm; 02700 break; 02701 case Config_SPR_5: 02702 *config = (uint8_t)reg_config.bits.spr_5; 02703 break; 02704 case Config_I2CSH: 02705 *config = (uint8_t)reg_config.bits.i2csh; 02706 break; 02707 case Config_SHDN: 02708 *config = (uint8_t)reg_config.bits.shdn; 02709 break; 02710 case Config_Tex: 02711 *config = (uint8_t)reg_config.bits.tex; 02712 break; 02713 case Config_Ten: 02714 *config = (uint8_t)reg_config.bits.ten; 02715 break; 02716 case Config_AINSH: 02717 *config = (uint8_t)reg_config.bits.ainsh; 02718 break; 02719 case Config_SPR_11: 02720 *config = (uint8_t)reg_config.bits.spr_11; 02721 break; 02722 case Config_Vs: 02723 *config = (uint8_t)reg_config.bits.vs; 02724 break; 02725 case Config_Ts: 02726 *config = (uint8_t)reg_config.bits.ts; 02727 break; 02728 case Config_Ss: 02729 *config = (uint8_t)reg_config.bits.ss; 02730 break; 02731 case Config_SPR_15: 02732 *config = (uint8_t)reg_config.bits.spr_15; 02733 break; 02734 default: 02735 return MAX77658_INVALID_DATA; 02736 break; 02737 } 02738 02739 return MAX77658_NO_ERROR; 02740 } 02741 02742 int MAX77658::set_fg_ichg_term(float currentA) 02743 { 02744 uint16_t currentRaw; 02745 reg_ichg_term_t reg_ichg_term = {0}; 02746 02747 //Register scale range of ± 5.12 A 02748 if (currentA < -5.12f) currentA = -5.12f; 02749 else if (currentA > 5.12f) currentA = 5.12f; 02750 02751 //LSB value of 156.25μA 02752 currentRaw = (int)round(currentA * 1000000 / 156.25f) & TO_UINT16; 02753 02754 SET_FG_BIT_FIELD(IChgTerm, reg_ichg_term, reg_ichg_term.bits.ichg_term, currentRaw); 02755 return MAX77658_NO_ERROR; 02756 } 02757 02758 int MAX77658::get_fg_ichg_term(float *currentA) 02759 { 02760 int ret; 02761 int16_t currentSigned; 02762 reg_ichg_term_t reg_ichg_term = {0}; 02763 02764 ret = read_fg_register(IChgTerm, (uint8_t *)&(reg_ichg_term)); 02765 if (ret != MAX77658_NO_ERROR) return ret; 02766 02767 //LSB value of 156.25μA 02768 currentSigned = (int16_t)reg_ichg_term.bits.ichg_term; 02769 *currentA = (float)(((int)currentSigned) * 156.25f / 1000000); 02770 02771 //Register scale range of ± 5.12 A 02772 if (*currentA < -5.12f) *currentA = -5.12f; 02773 else if (*currentA > 5.12f) *currentA = 5.12f; 02774 02775 return MAX77658_NO_ERROR; 02776 } 02777 02778 int MAX77658::get_fg_dev_name(uint16_t *value) 02779 { 02780 int ret; 02781 reg_dev_name_t reg_dev_name = {0}; 02782 02783 ret = read_fg_register(DevName, (uint8_t *)&(reg_dev_name)); 02784 if (ret != MAX77658_NO_ERROR) return ret; 02785 02786 *value = (uint16_t)(reg_dev_name.bits.dev_name); 02787 02788 return MAX77658_NO_ERROR; 02789 } 02790 02791 int MAX77658::set_fg_nempty(uint8_t nempty) 02792 { 02793 reg_filter_cfg_t reg_filter_cfg = {0}; 02794 02795 SET_FG_BIT_FIELD(FilterCfg, reg_filter_cfg, reg_filter_cfg.bits.nempty, nempty); 02796 return MAX77658_NO_ERROR; 02797 } 02798 02799 int MAX77658::get_fg_nempty(uint8_t *nempty) 02800 { 02801 int ret; 02802 reg_filter_cfg_t reg_filter_cfg = {0}; 02803 02804 ret = read_fg_register(FilterCfg, (uint8_t *)&(reg_filter_cfg)); 02805 if (ret != MAX77658_NO_ERROR) return ret; 02806 02807 *nempty = (uint8_t)reg_filter_cfg.bits.nempty; 02808 02809 return MAX77658_NO_ERROR; 02810 } 02811 02812 int MAX77658::set_fg_nmix(float second) 02813 { 02814 int nmixRaw; 02815 reg_filter_cfg_t reg_filter_cfg = {0}; 02816 02817 //Mixing Period = 175.8ms × 2^(5+NMIX) 02818 nmixRaw = (int)round((log2(second * 1000.0f / 175.8f)) - 5.0f); 02819 02820 SET_FG_BIT_FIELD(FilterCfg, reg_filter_cfg, reg_filter_cfg.bits.nmix, nmixRaw); 02821 return MAX77658_NO_ERROR; 02822 } 02823 02824 int MAX77658::get_fg_nmix(float *second) 02825 { 02826 int ret, nmixRaw; 02827 reg_filter_cfg_t reg_filter_cfg = {0}; 02828 02829 ret = read_fg_register(FilterCfg, (uint8_t *)&(reg_filter_cfg)); 02830 if (ret != MAX77658_NO_ERROR) return ret; 02831 02832 //Mixing Period = 175.8ms × 2^(5+NMIX) 02833 nmixRaw = (int)reg_filter_cfg.bits.nmix; 02834 *second = (float)(175.8f * pow(2, (5 + nmixRaw)) / 1000.0f); 02835 02836 return MAX77658_NO_ERROR; 02837 } 02838 02839 int MAX77658::set_fg_navgcell(float second) 02840 { 02841 int navgcellRaw; 02842 reg_filter_cfg_t reg_filter_cfg = {0}; 02843 02844 //AverageVCELL time constant = 175.8ms × 2^(6+NAVGVCELL) 02845 navgcellRaw = (int)round((log2(second * 1000.0f / 175.8f)) - 6.0f); 02846 02847 SET_FG_BIT_FIELD(FilterCfg, reg_filter_cfg, reg_filter_cfg.bits.navgcell, navgcellRaw); 02848 return MAX77658_NO_ERROR; 02849 } 02850 02851 int MAX77658::get_fg_navgcell(float *second) 02852 { 02853 int ret, navgcellRaw; 02854 reg_filter_cfg_t reg_filter_cfg = {0}; 02855 02856 ret = read_fg_register(FilterCfg, (uint8_t *)&(reg_filter_cfg)); 02857 if (ret != MAX77658_NO_ERROR) return ret; 02858 02859 //AverageVCELL time constant = 175.8ms × 2^(6+NAVGVCELL) 02860 navgcellRaw = (int)reg_filter_cfg.bits.navgcell; 02861 *second = (float)(175.8f * pow(2, (6 + navgcellRaw)) / 1000.0f); 02862 02863 return MAX77658_NO_ERROR; 02864 } 02865 02866 int MAX77658::set_fg_ncurr(float second) 02867 { 02868 int ncurrRaw; 02869 reg_filter_cfg_t reg_filter_cfg = {0}; 02870 02871 //AverageVCELL time constant = 175.8ms × 2^(2+NCURR) 02872 ncurrRaw = (int)round((log2(second * 1000.0f / 175.8f)) - 2.0f); 02873 02874 SET_FG_BIT_FIELD(FilterCfg, reg_filter_cfg, reg_filter_cfg.bits.navgcell, ncurrRaw); 02875 return MAX77658_NO_ERROR; 02876 } 02877 02878 int MAX77658::get_fg_ncurr(float *second) 02879 { 02880 int ret, ncurrRaw; 02881 reg_filter_cfg_t reg_filter_cfg = {0}; 02882 02883 ret = read_fg_register(FilterCfg, (uint8_t *)&(reg_filter_cfg)); 02884 if (ret != MAX77658_NO_ERROR) return ret; 02885 02886 //AverageVCELL time constant = 175.8ms × 2^(2+NCURR) 02887 ncurrRaw = (int)reg_filter_cfg.bits.ncurr; 02888 *second = (float)(175.8f * pow(2, (2 + ncurrRaw)) / 1000.0f); 02889 02890 return MAX77658_NO_ERROR; 02891 } 02892 02893 int MAX77658::set_fg_iavg_empty(float currentA) 02894 { 02895 uint16_t currentRaw; 02896 reg_iavg_empty_t reg_iavg_empty = {0}; 02897 02898 //Register scale range of ± 5.12 A 02899 if (currentA < -5.12f) currentA = -5.12f; 02900 else if (currentA > 5.12f) currentA = 5.12f; 02901 02902 //LSB value of 156.25μA 02903 currentRaw = (int)round(currentA * 1000000 / 156.25f) & TO_UINT16; 02904 02905 SET_FG_BIT_FIELD(IAvgEmpty, reg_iavg_empty, reg_iavg_empty.bits.rsvd, currentRaw); 02906 return MAX77658_NO_ERROR; 02907 } 02908 02909 int MAX77658::get_fg_iavg_empty(float *currentA) 02910 { 02911 int ret; 02912 int16_t currentSigned; 02913 reg_iavg_empty_t reg_iavg_empty = {0}; 02914 02915 ret = read_fg_register(IAvgEmpty, (uint8_t *)&(reg_iavg_empty)); 02916 if (ret != MAX77658_NO_ERROR) return ret; 02917 02918 //LSB value of 156.25μA 02919 currentSigned = (int16_t)reg_iavg_empty.bits.rsvd; 02920 *currentA = (float)(((int)currentSigned) * 156.25f / 1000000); 02921 02922 //Register scale range of ± 5.12 A 02923 if (*currentA < -5.12f) *currentA = -5.12f; 02924 else if (*currentA > 5.12f) *currentA = 5.12f; 02925 02926 return MAX77658_NO_ERROR; 02927 } 02928 02929 int MAX77658::set_fg_v_empty(float voltV) 02930 { 02931 int voltRaw; 02932 reg_v_empty_t reg_v_empty = {0}; 02933 float voltmV = voltV * 1000; 02934 02935 //A 10mV resolution gives a 0 to 5.11V range. 02936 if (voltmV < 0) voltmV = 0; 02937 else if (voltmV > 5110) voltmV = 5110; 02938 02939 voltRaw = (int)(voltmV / 10); 02940 02941 SET_FG_BIT_FIELD(VEmpty, reg_v_empty, reg_v_empty.bits.v_empty, voltRaw); 02942 return MAX77658_NO_ERROR; 02943 } 02944 02945 int MAX77658::get_fg_v_empty(float *voltV) 02946 { 02947 int ret, voltRaw; 02948 reg_v_empty_t reg_v_empty = {0}; 02949 02950 ret = read_fg_register(VEmpty, (uint8_t *)&(reg_v_empty)); 02951 if (ret != MAX77658_NO_ERROR) return ret; 02952 02953 //A 10mV resolution gives a 0 to 5.11V range. 02954 voltRaw = (int)reg_v_empty.bits.v_empty; 02955 *voltV = ((float)voltRaw * 10.0f) / 1000.0f; 02956 02957 if (*voltV < 0) *voltV = 0; 02958 else if (*voltV > 5.11f) *voltV = 5.11f; 02959 02960 return MAX77658_NO_ERROR; 02961 } 02962 02963 int MAX77658::set_fg_v_recover(float voltV) 02964 { 02965 int voltRaw; 02966 reg_v_empty_t reg_v_empty = {0}; 02967 float voltmV = voltV * 1000; 02968 02969 //A 40mV resolution gives a 0 to 5.08V range. 02970 if (voltmV < 0) voltmV = 0; 02971 else if (voltmV > 5080) voltmV = 5080; 02972 02973 voltRaw = (int)(voltmV / 40); 02974 02975 SET_FG_BIT_FIELD(VEmpty, reg_v_empty, reg_v_empty.bits.v_recover, voltRaw); 02976 return MAX77658_NO_ERROR; 02977 } 02978 02979 int MAX77658::get_fg_v_recover(float *voltV) 02980 { 02981 int ret, voltRaw; 02982 reg_v_empty_t reg_v_empty = {0}; 02983 02984 ret = read_fg_register(VEmpty, (uint8_t *)&(reg_v_empty)); 02985 if (ret != MAX77658_NO_ERROR) return ret; 02986 02987 //A 40mV resolution gives a 0 to 5.08V range. 02988 voltRaw = (int)reg_v_empty.bits.v_recover; 02989 *voltV = ((float)voltRaw * 40.0f) / 1000.0f; 02990 02991 02992 if (*voltV < 0) *voltV = 0; 02993 else if (*voltV > 5.08f) *voltV = 5.08f; 02994 02995 return MAX77658_NO_ERROR; 02996 } 02997 02998 int MAX77658::set_fg_config2(reg_bit_config2_t bit_field, uint8_t config) 02999 { 03000 int ret; 03001 reg_config2_t reg_config2 = {0}; 03002 03003 ret = read_fg_register(Config2, (uint8_t *)&(reg_config2)); 03004 if (ret != MAX77658_NO_ERROR) return ret; 03005 03006 switch (bit_field) 03007 { 03008 case Config2_ISysNCurr: 03009 reg_config2.bits.isys_ncurr = config; 03010 break; 03011 case Config2_OCVQen: 03012 reg_config2.bits.qcvqen = config; 03013 break; 03014 case Config2_LdMdl: 03015 reg_config2.bits.ldmdl = config; 03016 break; 03017 case Config2_TAlrtEn: 03018 reg_config2.bits.tairt_en = config; 03019 break; 03020 case Config2_dSOCen: 03021 reg_config2.bits.dsocen = config; 03022 break; 03023 case Config2_ThmHotAlrtEn: 03024 reg_config2.bits.thm_hotairt_en = config; 03025 break; 03026 case Config2_ThmHotEn: 03027 reg_config2.bits.thmhot_en = config; 03028 break; 03029 case Config2_FCThmHot: 03030 reg_config2.bits.fc_thmhot = config; 03031 break; 03032 case Config2_SPR: 03033 reg_config2.bits.spr = config; 03034 break; 03035 default: 03036 return MAX77658_INVALID_DATA; 03037 break; 03038 } 03039 03040 return write_fg_register(Config2, (uint8_t *)&(reg_config2)); 03041 } 03042 03043 int MAX77658::get_fg_config2(reg_bit_config2_t bit_field, uint8_t *config) 03044 { 03045 int ret; 03046 reg_config2_t reg_config2 = {0}; 03047 03048 ret = read_fg_register(Config2, (uint8_t *)&(reg_config2)); 03049 if (ret != MAX77658_NO_ERROR) return ret; 03050 03051 switch (bit_field) 03052 { 03053 case Config2_ISysNCurr: 03054 *config = (uint8_t)reg_config2.bits.isys_ncurr; 03055 break; 03056 case Config2_OCVQen: 03057 *config = (uint8_t)reg_config2.bits.qcvqen; 03058 break; 03059 case Config2_LdMdl: 03060 *config = (uint8_t)reg_config2.bits.ldmdl; 03061 break; 03062 case Config2_TAlrtEn: 03063 *config = (uint8_t)reg_config2.bits.tairt_en; 03064 break; 03065 case Config2_dSOCen: 03066 *config = (uint8_t)reg_config2.bits.dsocen; 03067 break; 03068 case Config2_ThmHotAlrtEn: 03069 *config = (uint8_t)reg_config2.bits.thm_hotairt_en; 03070 break; 03071 case Config2_ThmHotEn: 03072 *config = (uint8_t)reg_config2.bits.thmhot_en; 03073 break; 03074 case Config2_FCThmHot: 03075 *config = (uint8_t)reg_config2.bits.fc_thmhot; 03076 break; 03077 case Config2_SPR: 03078 *config = (uint8_t)reg_config2.bits.spr; 03079 break; 03080 default: 03081 return MAX77658_INVALID_DATA; 03082 break; 03083 } 03084 03085 return MAX77658_NO_ERROR; 03086 } 03087 03088 int MAX77658::set_fg_isys_ncurr(float second) 03089 { 03090 int secondRaw; 03091 reg_config2_t reg_config2 = {0}; 03092 03093 //AvgISys time constant = 45s x 2^(ISysNCurr-7) 03094 secondRaw = (int)round(log2(second * 1000 / 45000) + 7); 03095 03096 SET_FG_BIT_FIELD(Config2, reg_config2, reg_config2.bits.isys_ncurr, secondRaw); 03097 return MAX77658_NO_ERROR; 03098 } 03099 03100 int MAX77658::get_fg_isys_ncurr(float *second) 03101 { 03102 int ret, secondRaw; 03103 reg_config2_t reg_config2 = {0}; 03104 03105 ret = read_fg_register(Config2, (uint8_t *)&(reg_config2)); 03106 if (ret != MAX77658_NO_ERROR) return ret; 03107 03108 //AvgISys time constant = 45s x 2^(ISysNCurr-7) 03109 secondRaw = (int)reg_config2.bits.isys_ncurr; 03110 *second = (float)(45.0f * pow(2, secondRaw - 7)); 03111 03112 return MAX77658_NO_ERROR; 03113 } 03114 03115 int MAX77658::set_fg_temp(float tempDegC) 03116 { 03117 uint16_t tempRaw; 03118 reg_temp_t reg_temp = {0}; 03119 03120 //Min value is -128.0°C and Max value is 127.996°C. 03121 if (tempDegC < -128) tempDegC = -128; 03122 else if (tempDegC > 127.996f) tempDegC = 127.996f; 03123 03124 //LSB is 1/256°C = 0.0039˚C. 03125 tempRaw = (int)round(tempDegC * 256) & TO_UINT16; 03126 03127 SET_FG_BIT_FIELD(Temp, reg_temp, reg_temp.bits.temp, tempRaw); 03128 return MAX77658_NO_ERROR; 03129 } 03130 03131 int MAX77658::get_fg_temp(float *tempDegC) 03132 { 03133 int ret; 03134 int16_t tempSigned; 03135 reg_temp_t reg_temp = {0}; 03136 03137 ret = read_fg_register(Temp, (uint8_t *)&(reg_temp)); 03138 if (ret != MAX77658_NO_ERROR) return ret; 03139 03140 //LSB is 1/256°C = 0.0039˚C. 03141 tempSigned = (int16_t)reg_temp.bits.temp; 03142 *tempDegC = (float)(tempSigned / 256.0f); 03143 03144 //Min value is -128.0°C and Max value is 127.996°C. 03145 if (*tempDegC < -128) *tempDegC = -128; 03146 else if (*tempDegC > 127.996f) *tempDegC = 127.996f; 03147 03148 return MAX77658_NO_ERROR; 03149 } 03150 03151 int MAX77658::set_fg_vcell(float voltV) 03152 { 03153 uint16_t voltRaw; 03154 reg_vcell_t reg_vcell = {0}; 03155 float voltmV = voltV * 1000; 03156 03157 //Register scale range between 0V and 5.11992V. //LSB value of 1.25mV/16 03158 if (voltmV < 0) voltmV = 0; 03159 else if (voltmV > 5119.92f) voltmV = 5119.92f; 03160 03161 voltRaw = (int)round(voltmV / 1.25 * 16) & TO_UINT16; 03162 03163 SET_FG_BIT_FIELD(Vcell, reg_vcell, reg_vcell.bits.vcell, voltRaw); 03164 return MAX77658_NO_ERROR; 03165 } 03166 03167 int MAX77658::get_fg_vcell(float *voltV) 03168 { 03169 int ret, voltRaw; 03170 reg_vcell_t reg_vcell = {0}; 03171 03172 ret = read_fg_register(Vcell, (uint8_t *)&(reg_vcell)); 03173 if (ret != MAX77658_NO_ERROR) return ret; 03174 03175 //LSB value of 1.25mV/16 03176 voltRaw = (int)reg_vcell.bits.vcell; 03177 *voltV = (float)(voltRaw * 1.25f / 16 / 1000); 03178 03179 if (*voltV < 0) *voltV = 0; 03180 else if (*voltV > 5.11992f) *voltV = 5.11992f; 03181 03182 return MAX77658_NO_ERROR; 03183 } 03184 03185 int MAX77658::set_fg_current(float currentA) 03186 { 03187 uint16_t currentRaw; 03188 reg_current_t reg_current = {0}; 03189 float currentmA = currentA * 1000; 03190 03191 if (currentmA < -1024) currentmA = -1024; 03192 else if (currentmA > 1024) currentmA = 1024; 03193 03194 //The current register has a LSB value of 31.25uA, a register scale of 1.024A 03195 currentRaw = (int)round(currentmA * 1000 / 31.25f) & TO_UINT16; 03196 03197 SET_FG_BIT_FIELD(Current, reg_current, reg_current.bits.current, currentRaw); 03198 return MAX77658_NO_ERROR; 03199 } 03200 03201 int MAX77658::get_fg_current(float *currentA) 03202 { 03203 int ret; 03204 int16_t currentSigned; 03205 reg_current_t reg_current = {0}; 03206 03207 ret = read_fg_register(Current, (uint8_t *)&(reg_current)); 03208 if (ret != MAX77658_NO_ERROR) return ret; 03209 03210 //The current register has a LSB value of 31.25uA, a register scale of 1.024A 03211 currentSigned = (int16_t)(reg_current.bits.current); 03212 *currentA = (float)(currentSigned * 31.25f / 1000000); 03213 03214 if (*currentA < -1.024f) *currentA = -1.024f; 03215 else if (*currentA > 1.024f) *currentA = 1.024f; 03216 03217 return MAX77658_NO_ERROR; 03218 } 03219 03220 int MAX77658::set_fg_avg_current(float currentA) 03221 { 03222 uint16_t currentRaw; 03223 reg_avg_current_t reg_avg_current = {0}; 03224 float currentmA = currentA * 1000; 03225 03226 if (currentmA < -1024) currentmA = -1024; 03227 else if (currentmA > 1024) currentmA = 1024; 03228 03229 //The current register has a LSB value of 31.25uA, a register scale of 1.024A 03230 currentRaw = (int)round(currentmA * 1000 / 31.25f) & TO_UINT16; 03231 03232 SET_FG_BIT_FIELD(AvgCurrent, reg_avg_current, reg_avg_current.bits.avg_current, currentRaw); 03233 return MAX77658_NO_ERROR; 03234 } 03235 03236 int MAX77658::get_fg_avg_current(float *currentA) 03237 { 03238 int ret; 03239 int16_t currentSigned; 03240 reg_avg_current_t reg_avg_current = {0}; 03241 03242 ret = read_fg_register(AvgCurrent, (uint8_t *)&(reg_avg_current)); 03243 if (ret != MAX77658_NO_ERROR) return ret; 03244 03245 //The current register has a LSB value of 31.25uA, a register scale of 1.024A 03246 currentSigned = (int16_t)(reg_avg_current.bits.avg_current); 03247 *currentA = (float)(currentSigned * 31.25f / 1000000); 03248 03249 if (*currentA < -1.024f) *currentA = -1.024f; 03250 else if (*currentA > 1.024f) *currentA = 1.024f; 03251 03252 return MAX77658_NO_ERROR; 03253 } 03254 03255 int MAX77658::set_fg_avgta(float tempDegC) 03256 { 03257 uint16_t tempRaw; 03258 reg_avg_ta_t reg_avg_ta = {0}; 03259 03260 //Min value is -128.0°C and Max value is 127.996°C. 03261 if (tempDegC < -128) tempDegC = -128; 03262 else if (tempDegC > 127.996f) tempDegC = 127.996f; 03263 03264 //LSB is 1/256°C = 0.0039˚C. 03265 tempRaw = (int)round(tempDegC * 256) & TO_UINT16; 03266 03267 SET_FG_BIT_FIELD(AvgTA, reg_avg_ta, reg_avg_ta.bits.avg_ta, tempRaw); 03268 return MAX77658_NO_ERROR; 03269 } 03270 03271 int MAX77658::get_fg_avgta(float *tempDegC) 03272 { 03273 int ret; 03274 int16_t tempSigned; 03275 reg_avg_ta_t reg_avg_ta = {0}; 03276 03277 ret = read_fg_register(AvgTA, (uint8_t *)&(reg_avg_ta)); 03278 if (ret != MAX77658_NO_ERROR) return ret; 03279 03280 //LSB is 1/256°C = 0.0039˚C. 03281 tempSigned = (int16_t)reg_avg_ta.bits.avg_ta; 03282 *tempDegC = (float)(tempSigned / 256.0f); 03283 03284 //Min value is -128.0°C and Max value is 127.996°C. 03285 if (*tempDegC < -128) *tempDegC = -128; 03286 else if (*tempDegC > 127.996f) *tempDegC = 127.996f; 03287 03288 return MAX77658_NO_ERROR; 03289 } 03290 03291 int MAX77658::set_fg_avgvcell(float voltV) 03292 { 03293 uint16_t voltRaw; 03294 reg_avg_vcell_t reg_avg_vcell = {0}; 03295 float voltmV = voltV * 1000; 03296 03297 //Register scale range between 0V and 5.11992V. //LSB value of 1.25mV/16 03298 if (voltmV < 0) voltmV = 0; 03299 else if (voltmV > 5119.92f) voltmV = 5119.92f; 03300 03301 voltRaw = (int)round(voltmV / 1.25 * 16) & TO_UINT16; 03302 03303 SET_FG_BIT_FIELD(AvgVCell, reg_avg_vcell, reg_avg_vcell.bits.avg_vcell, voltRaw); 03304 return MAX77658_NO_ERROR; 03305 } 03306 03307 int MAX77658::get_fg_avgvcell(float *voltV) 03308 { 03309 int ret, voltRaw; 03310 reg_avg_vcell_t reg_avg_vcell = {0}; 03311 03312 ret = read_fg_register(AvgVCell, (uint8_t *)&(reg_avg_vcell)); 03313 if (ret != MAX77658_NO_ERROR) return ret; 03314 03315 //LSB value of 1.25mV/16 03316 voltRaw = (int)reg_avg_vcell.bits.avg_vcell; 03317 *voltV = (float)(voltRaw * 1.25f / 16 / 1000); 03318 03319 //Min value is 0.0V and Max value is 5.11992V. 03320 if (*voltV < 0) *voltV = 0; 03321 else if (*voltV > 5.11992f) *voltV = 5.11992f; 03322 03323 return MAX77658_NO_ERROR; 03324 } 03325 03326 int MAX77658::set_fg_max_min_temp(reg_bit_max_min_temp_t bit_field, int tempDegC) 03327 { 03328 int ret; 03329 uint8_t tempRaw; 03330 reg_max_min_temp_t reg_max_min_temp = {0}; 03331 03332 ret = read_fg_register(MaxMinTemp, (uint8_t *)&(reg_max_min_temp)); 03333 if (ret != MAX77658_NO_ERROR) return ret; 03334 03335 tempRaw = tempDegC & TO_UINT8; 03336 03337 //1°C resolution 03338 switch (bit_field) 03339 { 03340 case MaxMinTemp_MinTemperature: 03341 reg_max_min_temp.bits.min_temp = tempRaw; 03342 break; 03343 case MaxMinTemp_MaxTemperature: 03344 reg_max_min_temp.bits.max_temp = tempRaw; 03345 break; 03346 default: 03347 return MAX77658_INVALID_DATA; 03348 break; 03349 } 03350 03351 return write_fg_register(MaxMinTemp, (uint8_t *)&(reg_max_min_temp)); 03352 } 03353 03354 int MAX77658::get_fg_max_min_temp(reg_bit_max_min_temp_t bit_field, int *tempDegC) 03355 { 03356 int ret; 03357 int8_t tempSigned; 03358 reg_max_min_temp_t reg_max_min_temp = {0}; 03359 03360 ret = read_fg_register(MaxMinTemp, (uint8_t *)&(reg_max_min_temp)); 03361 if (ret != MAX77658_NO_ERROR) return ret; 03362 03363 switch (bit_field) 03364 { 03365 case MaxMinTemp_MinTemperature: 03366 tempSigned = (int8_t)reg_max_min_temp.bits.min_temp; 03367 break; 03368 case MaxMinTemp_MaxTemperature: 03369 tempSigned = (int8_t)reg_max_min_temp.bits.max_temp; 03370 break; 03371 default: 03372 return MAX77658_INVALID_DATA; 03373 break; 03374 } 03375 03376 //1°C resolution 03377 *tempDegC = (int)tempSigned; 03378 03379 return MAX77658_NO_ERROR; 03380 } 03381 03382 int MAX77658::set_fg_max_min_volt(reg_bit_max_min_volt_t bit_field, float voltV) 03383 { 03384 int ret; 03385 uint8_t voltRaw; 03386 reg_max_min_volt_t reg_max_min_volt = {0}; 03387 float voltmV = voltV * 1000; 03388 03389 ret = read_fg_register(MaxMinVolt, (uint8_t *)&(reg_max_min_volt)); 03390 if (ret != MAX77658_NO_ERROR) return ret; 03391 03392 //20mV resolution 03393 voltRaw = (int)(voltmV / 20) & TO_UINT8; 03394 03395 switch (bit_field) 03396 { 03397 case MaxMinVolt_MinVoltage: 03398 reg_max_min_volt.bits.min_volt = voltRaw; 03399 break; 03400 case MaxMinVolt_MaxVoltage: 03401 reg_max_min_volt.bits.max_volt = voltRaw; 03402 break; 03403 default: 03404 return MAX77658_INVALID_DATA; 03405 break; 03406 } 03407 03408 return write_fg_register(MaxMinVolt, (uint8_t *)&(reg_max_min_volt)); 03409 } 03410 03411 int MAX77658::get_fg_max_min_volt(reg_bit_max_min_volt_t bit_field, float *voltV) 03412 { 03413 int ret; 03414 int8_t voltSigned; 03415 reg_max_min_volt_t reg_max_min_volt = {0}; 03416 03417 ret = read_fg_register(MaxMinVolt, (uint8_t *)&(reg_max_min_volt)); 03418 if (ret != MAX77658_NO_ERROR) return ret; 03419 03420 switch (bit_field) 03421 { 03422 case MaxMinVolt_MinVoltage: 03423 voltSigned = (int8_t)reg_max_min_volt.bits.min_volt; 03424 break; 03425 case MaxMinVolt_MaxVoltage: 03426 voltSigned = (int8_t)reg_max_min_volt.bits.max_volt; 03427 break; 03428 default: 03429 return MAX77658_INVALID_DATA; 03430 break; 03431 } 03432 03433 //20mV resolution 03434 *voltV = (float)((voltSigned * 20.0f) / 1000.0f); 03435 03436 return MAX77658_NO_ERROR; 03437 } 03438 03439 int MAX77658::set_fg_max_min_curr(reg_bit_max_min_curr_t bit_field, float currentA) 03440 { 03441 int ret; 03442 uint8_t currentRaw; 03443 reg_max_min_curr_t reg_max_min_curr = {0}; 03444 float currentmA = currentA * 1000; 03445 03446 ret = read_fg_register(MaxMinCurr, (uint8_t *)&(reg_max_min_curr)); 03447 if (ret != MAX77658_NO_ERROR) return ret; 03448 03449 //8mA resolution. 03450 currentRaw = (int)round(currentmA / 8) & TO_UINT8; 03451 03452 switch (bit_field) 03453 { 03454 case MaxMinCurr_MaxDisCurrent: 03455 reg_max_min_curr.bits.min_charge_curr = currentRaw; 03456 break; 03457 case MaxMinCurr_MaxChargeCurrent: 03458 reg_max_min_curr.bits.max_charge_curr = currentRaw; 03459 break; 03460 default: 03461 return MAX77658_INVALID_DATA; 03462 break; 03463 } 03464 03465 return write_fg_register(MaxMinCurr, (uint8_t *)&(reg_max_min_curr)); 03466 } 03467 03468 int MAX77658::get_fg_max_min_curr(reg_bit_max_min_curr_t bit_field, float *currentA) 03469 { 03470 int ret; 03471 int8_t currentSigned; 03472 reg_max_min_curr_t reg_max_min_curr = {0}; 03473 03474 ret = read_fg_register(MaxMinCurr, (uint8_t *)&(reg_max_min_curr)); 03475 if (ret != MAX77658_NO_ERROR) return ret; 03476 03477 switch (bit_field) 03478 { 03479 case MaxMinCurr_MaxDisCurrent: 03480 currentSigned = (int8_t)reg_max_min_curr.bits.min_charge_curr; 03481 break; 03482 case MaxMinCurr_MaxChargeCurrent: 03483 currentSigned = (int8_t)reg_max_min_curr.bits.max_charge_curr; 03484 break; 03485 default: 03486 return MAX77658_INVALID_DATA; 03487 break; 03488 } 03489 03490 //8mA resolution 03491 *currentA = (float)(currentSigned * 8.0f / 1000.0f); 03492 03493 return MAX77658_NO_ERROR; 03494 } 03495 03496 int MAX77658::set_fg_ain0(float percent) 03497 { 03498 int percentRaw; 03499 reg_ain0_t reg_ain0 = {0}; 03500 03501 //LSB of 0.0122% 03502 percentRaw = (int)round(percent / 0.0122f); 03503 03504 SET_FG_BIT_FIELD(AIN0, reg_ain0, reg_ain0.bits.ain0, percentRaw); 03505 return MAX77658_NO_ERROR; 03506 } 03507 03508 int MAX77658::get_fg_ain0(float *percent) 03509 { 03510 int ret, percentRaw; 03511 reg_ain0_t reg_ain0 = {0}; 03512 03513 ret = read_fg_register(AIN0, (uint8_t *)&(reg_ain0)); 03514 if (ret != MAX77658_NO_ERROR) return ret; 03515 03516 //LSB of 0.0122% 03517 percentRaw = (int)reg_ain0.bits.ain0; 03518 *percent = (float)(percentRaw * 0.0122f); 03519 03520 return MAX77658_NO_ERROR; 03521 } 03522 03523 int MAX77658::set_fg_timer(float second) 03524 { 03525 int secondRaw; 03526 reg_timer_t reg_timer = {0}; 03527 03528 //full-scale range of 0 to 3.2 hours= 11520 sec 03529 if (second < 0) second = 0; 03530 else if (second > 11520.0f) second = 11520.0f; 03531 03532 //LSB is 175.8ms 03533 secondRaw = (int)round(second * 1000 / 175.8f); 03534 03535 SET_FG_BIT_FIELD(Timer, reg_timer, reg_timer.bits.timer, secondRaw); 03536 return MAX77658_NO_ERROR; 03537 } 03538 03539 int MAX77658::get_fg_timer(float *second) 03540 { 03541 int ret, secondRaw; 03542 reg_timer_t reg_timer = {0}; 03543 03544 ret = read_fg_register(Timer, (uint8_t *)&(reg_timer)); 03545 if (ret != MAX77658_NO_ERROR) return ret; 03546 03547 //LSB is 175.8ms 03548 secondRaw = (int)reg_timer.bits.timer; 03549 *second = (float)((float)secondRaw * 175.8f / 1000); 03550 03551 //full-scale range of 0 to 3.2 hours 03552 if (*second < 0) *second = 0; 03553 else if (*second > 11520.0f) *second = 11520.0f; 03554 03555 return MAX77658_NO_ERROR; 03556 } 03557 03558 int MAX77658::set_fg_shdnctr(float second) 03559 { 03560 int secondRaw; 03561 reg_shdn_timer_t reg_shdn_timer = {0}; 03562 03563 //The counter LSB is 1.4s 03564 secondRaw = (int)round(second / 1.4f); 03565 03566 SET_FG_BIT_FIELD(ShdnTimer, reg_shdn_timer, reg_shdn_timer.bits.shdnctr, secondRaw); 03567 return MAX77658_NO_ERROR; 03568 } 03569 03570 int MAX77658::get_fg_shdnctr(float *second) 03571 { 03572 int ret, secondRaw; 03573 reg_shdn_timer_t reg_shdn_timer = {0}; 03574 03575 ret = read_fg_register(ShdnTimer, (uint8_t *)&(reg_shdn_timer)); 03576 if (ret != MAX77658_NO_ERROR) return ret; 03577 03578 //Period = 175.8ms × 2^(8+THR) 03579 secondRaw = (int)reg_shdn_timer.bits.shdnctr; 03580 *second = (float)(secondRaw * 1.4f); 03581 03582 return MAX77658_NO_ERROR; 03583 } 03584 03585 int MAX77658::set_fg_shdn_thr(float second) 03586 { 03587 int secondRaw; 03588 reg_shdn_timer_t reg_shdn_timer = {0}; 03589 03590 //minimum of 45s to a maximum of 1.6h=5760sec 03591 if (second < 45) second = 45; 03592 else if (second > 5760.0f) second = 5760.0f; 03593 03594 //Period = 175.8ms × 2^(8+THR) 03595 secondRaw = (int)round(log2(second * 1000 / 175.8f) - 8); 03596 03597 SET_FG_BIT_FIELD(ShdnTimer, reg_shdn_timer, reg_shdn_timer.bits.shdn_thr, secondRaw); 03598 return MAX77658_NO_ERROR; 03599 } 03600 03601 int MAX77658::get_fg_shdn_thr(float *second) 03602 { 03603 int ret, secondRaw; 03604 reg_shdn_timer_t reg_shdn_timer = {0}; 03605 03606 ret = read_fg_register(ShdnTimer, (uint8_t *)&(reg_shdn_timer)); 03607 if (ret != MAX77658_NO_ERROR) return ret; 03608 03609 //Period = 175.8ms × 2^(8+THR) 03610 secondRaw = (int)reg_shdn_timer.bits.shdn_thr; 03611 *second = (float)(175.8f / 1000 * pow(2, secondRaw + 8)); 03612 03613 //minimum of 45s to a maximum of 1.6h 03614 if (*second < 45) *second = 45; 03615 else if (*second > 5760.0f) *second = 5760.0f; 03616 03617 return MAX77658_NO_ERROR; 03618 } 03619 03620 int MAX77658::set_fg_timerh(float hour) 03621 { 03622 int hourRaw; 03623 reg_timerh_t reg_timerh = {0}; 03624 03625 // Full-scale range up to 23.94 years = 209853.5577138 hr 03626 if (hour > 209853.5577138f) hour = (209853.5577138f); 03627 03628 //A 3.2-hour LSB 03629 hourRaw = (int)round(hour / 3.2f); 03630 03631 SET_FG_BIT_FIELD(TimerH, reg_timerh, reg_timerh.bits.timerh, hourRaw); 03632 return MAX77658_NO_ERROR; 03633 } 03634 03635 int MAX77658::get_fg_timerh(float *hour) 03636 { 03637 int ret, hourRaw; 03638 reg_timerh_t reg_timerh = {0}; 03639 03640 ret = read_fg_register(TimerH, (uint8_t *)&(reg_timerh)); 03641 if (ret != MAX77658_NO_ERROR) return ret; 03642 03643 //A 3.2-hour LSB 03644 hourRaw = (int)reg_timerh.bits.timerh; 03645 *hour = (float)(hourRaw * 3.2f); 03646 03647 // Full-scale range up to 23.94 years = 209853.5577138 hr 03648 if (*hour > 209853.5577138f) *hour = 209853.5577138f; 03649 03650 return MAX77658_NO_ERROR; 03651 } 03652 03653 int MAX77658::set_fg_rep_cap(float repCapmAh) 03654 { 03655 int repCapRaw; 03656 reg_rep_cap_t reg_rep_cap = {0}; 03657 03658 //Min value is 0.0mAh and Max value is 6553.5mAh 03659 if (repCapmAh < 0) repCapmAh = 0; 03660 else if (repCapmAh > 6553.5f) repCapmAh = 6553.5f; 03661 03662 //LSB is 0.1mAh. 03663 repCapRaw = (int)round(repCapmAh / 0.1f); 03664 03665 SET_FG_BIT_FIELD(RepCap, reg_rep_cap, reg_rep_cap.bits.rep_cap, repCapRaw); 03666 return MAX77658_NO_ERROR; 03667 } 03668 03669 int MAX77658::get_fg_rep_cap(float *repCapmAh) 03670 { 03671 int ret, repCapRaw; 03672 reg_rep_cap_t reg_rep_cap = {0}; 03673 03674 ret = read_fg_register(RepCap, (uint8_t *)&(reg_rep_cap)); 03675 if (ret != MAX77658_NO_ERROR) return ret; 03676 03677 //LSB is 0.1mAh. 03678 repCapRaw = (int)reg_rep_cap.bits.rep_cap; 03679 *repCapmAh = (float)(repCapRaw * 0.1f); 03680 03681 //Min value is 0.0mAh and Max value is 6553.5mAh 03682 if (*repCapmAh < 0) *repCapmAh = 0; 03683 else if (*repCapmAh > 6553.5f) *repCapmAh = 6553.5f; 03684 03685 return MAX77658_NO_ERROR; 03686 } 03687 03688 int MAX77658::set_fg_rep_soc(float percent) 03689 { 03690 int percentRaw; 03691 reg_rep_soc_t reg_rep_soc = {0}; 03692 03693 //Min value is 0.0% and Max value is 255.9961% 03694 if (percent < 0) percent = 0; 03695 else if (percent > 255.9961f) percent = 255.9961f; 03696 03697 //LSB is 1/256% 03698 percentRaw = (int)round(percent * 256); 03699 03700 SET_FG_BIT_FIELD(RepSOC, reg_rep_soc, reg_rep_soc.bits.rep_soc, percentRaw); 03701 return MAX77658_NO_ERROR; 03702 } 03703 03704 int MAX77658::get_fg_rep_soc(float *percent) 03705 { 03706 int ret, percentRaw; 03707 reg_rep_soc_t reg_rep_soc = {0}; 03708 03709 ret = read_fg_register(RepSOC, (uint8_t *)&(reg_rep_soc)); 03710 if (ret != MAX77658_NO_ERROR) return ret; 03711 03712 //LSB is 1/256% 03713 percentRaw = (int)reg_rep_soc.bits.rep_soc; 03714 *percent = (float)((float)percentRaw / 256.0f); 03715 03716 //Min value is 0.0% and Max value is 255.9961% 03717 if (*percent < 0) *percent = 0; 03718 else if (*percent > 255.9961f) *percent = 255.9961f; 03719 03720 return MAX77658_NO_ERROR; 03721 } 03722 03723 int MAX77658::set_fg_av_soc(float percent) 03724 { 03725 int percentRaw; 03726 reg_av_soc_t reg_av_soc = {0}; 03727 03728 //Min value is 0.0% and Max value is 255.9961% 03729 if (percent < 0) percent = 0; 03730 else if (percent > 255.9961f) percent = 255.9961f; 03731 03732 //LSB is 1/256% 03733 percentRaw = (int)round(percent * 256); 03734 03735 SET_FG_BIT_FIELD(AvSOC, reg_av_soc, reg_av_soc.bits.av_soc, percentRaw); 03736 return MAX77658_NO_ERROR; 03737 } 03738 03739 int MAX77658::get_fg_av_soc(float *percent) 03740 { 03741 int ret, percentRaw; 03742 reg_av_soc_t reg_av_soc = {0}; 03743 03744 ret = read_fg_register(AvSOC, (uint8_t *)&(reg_av_soc)); 03745 if (ret != MAX77658_NO_ERROR) return ret; 03746 03747 //LSB is 1/256% 03748 percentRaw = (int)reg_av_soc.bits.av_soc; 03749 *percent = (float)(percentRaw / 256.0f); 03750 03751 //Min value is 0.0% and Max value is 255.9961% 03752 if (*percent < 0) *percent = 0; 03753 else if (*percent > 255.9961f) *percent = 255.9961f; 03754 03755 return MAX77658_NO_ERROR; 03756 } 03757 03758 int MAX77658::set_fg_full_cap_reg(float repCapmAh) 03759 { 03760 int repCapRaw; 03761 reg_full_cap_rep_t reg_full_cap_rep = {0}; 03762 03763 //Min value is 0.0mAh and Max value is 6553.5mAh 03764 if (repCapmAh < 0) repCapmAh = 0; 03765 else if (repCapmAh > 6553.5f) repCapmAh = 6553.5f; 03766 03767 //LSB is 0.1mAh. 03768 repCapRaw = (int)round(repCapmAh / 0.1f); 03769 03770 SET_FG_BIT_FIELD(FullCapRep, reg_full_cap_rep, reg_full_cap_rep.bits.full_cap_rep, repCapRaw); 03771 return MAX77658_NO_ERROR; 03772 } 03773 03774 int MAX77658::get_fg_full_cap_reg(float *repCapmAh) 03775 { 03776 int ret, repCapRaw; 03777 reg_full_cap_rep_t reg_full_cap_rep = {0}; 03778 03779 ret = read_fg_register(FullCapRep, (uint8_t *)&(reg_full_cap_rep)); 03780 if (ret != MAX77658_NO_ERROR) return ret; 03781 03782 //LSB is 0.1mAh. 03783 repCapRaw = (int)reg_full_cap_rep.bits.full_cap_rep; 03784 *repCapmAh = (float)(repCapRaw * 0.1f); 03785 03786 //Min value is 0.0mAh and Max value is 6553.5mAh 03787 if (*repCapmAh < 0) *repCapmAh = 0; 03788 else if (*repCapmAh > 6553.5f) *repCapmAh = 6553.5f; 03789 03790 return MAX77658_NO_ERROR; 03791 } 03792 03793 int MAX77658::set_fg_tte(float minute) 03794 { 03795 int minuteRaw; 03796 reg_tte_t reg_tte = {0}; 03797 03798 //Min value is 0.0s and Max value is 102.3984h = 6143.904min. 03799 //LSB is 5.625s. 03800 minuteRaw = (int)round((float)minute * 60 / 5.625f); 03801 03802 SET_FG_BIT_FIELD(TTE, reg_tte, reg_tte.bits.tte, minuteRaw); 03803 return MAX77658_NO_ERROR; 03804 } 03805 03806 int MAX77658::get_fg_tte(float *minute) 03807 { 03808 int ret, minuteRaw; 03809 reg_tte_t reg_tte = {0}; 03810 03811 ret = read_fg_register(TTE, (uint8_t *)&(reg_tte)); 03812 if (ret != MAX77658_NO_ERROR) return ret; 03813 03814 //LSB is 5.625s. 03815 minuteRaw = (int)reg_tte.bits.tte; 03816 *minute = (float)((float)minuteRaw * 5.625f / 60); 03817 03818 //Min value is 0.0s and Max value is 102.3984h = = 6143.904 min.min. 03819 if (*minute < 0) *minute = 0; 03820 else if (*minute > 6143.904f) *minute = 6143.904f; 03821 03822 return MAX77658_NO_ERROR; 03823 } 03824 03825 int MAX77658::set_fg_rcell(float resOhm) 03826 { 03827 int resistanceRaw; 03828 reg_rcell_t reg_rcell = {0}; 03829 03830 //Min value is 0.0Ohm and Max value is 15.99976Ohm. 03831 if (resOhm < 0) resOhm = 0; 03832 else if (resOhm > 15.99976f) resOhm = 15.99976f; 03833 03834 //LSB is 1/4096Ohm 03835 resistanceRaw = (int)round(resOhm * 4096); 03836 03837 SET_FG_BIT_FIELD(RCell, reg_rcell, reg_rcell.bits.rcell, resistanceRaw); 03838 return MAX77658_NO_ERROR; 03839 } 03840 03841 int MAX77658::get_fg_rcell(float *resOhm) 03842 { 03843 int ret, resistanceRaw; 03844 reg_rcell_t reg_rcell = {0}; 03845 03846 ret = read_fg_register(RCell, (uint8_t *)&(reg_rcell)); 03847 if (ret != MAX77658_NO_ERROR) return ret; 03848 03849 //LSB is 1/4096Ohm 03850 resistanceRaw = (int)reg_rcell.bits.rcell; 03851 *resOhm = (float)(resistanceRaw / 4096.0f); 03852 03853 //Min value is 0.0Ohm and Max value is 15.99976Ohm. 03854 if (*resOhm < 0) *resOhm = 0; 03855 else if (*resOhm > 15.99976f) *resOhm = 15.99976f; 03856 03857 return MAX77658_NO_ERROR; 03858 } 03859 03860 int MAX77658::set_fg_cycles(uint16_t percent) 03861 { 03862 reg_cycles_t reg_cycles = {0}; 03863 03864 //The LSB indicates 1%. 03865 SET_FG_BIT_FIELD(Cycles, reg_cycles, reg_cycles.bits.cycles, percent); 03866 return MAX77658_NO_ERROR; 03867 } 03868 03869 int MAX77658::get_fg_cycles(uint16_t *percent) 03870 { 03871 int ret; 03872 reg_cycles_t reg_cycles = {0}; 03873 03874 ret = read_fg_register(Cycles, (uint8_t *)&(reg_cycles)); 03875 if (ret != MAX77658_NO_ERROR) return ret; 03876 03877 //The LSB indicates 1%. 03878 *percent = (uint16_t)(reg_cycles.bits.cycles); 03879 return MAX77658_NO_ERROR; 03880 } 03881 03882 int MAX77658::set_fg_av_cap(float avCapmAh) 03883 { 03884 int avCapRaw; 03885 reg_av_cap_t reg_av_cap = {0}; 03886 03887 //LSB is 0.1mAh. Min value is 0.0mAh and Max value is 6553.5mAh. 03888 if (avCapmAh < 0) avCapmAh = 0; 03889 else if (avCapmAh > 6553.5f) avCapmAh = 6553.5f; 03890 03891 avCapRaw = (int)round(avCapmAh / 0.5f); 03892 03893 SET_FG_BIT_FIELD(AvCap, reg_av_cap, reg_av_cap.bits.av_cap, avCapRaw); 03894 return MAX77658_NO_ERROR; 03895 } 03896 03897 int MAX77658::get_fg_av_cap(float *avCapmAh) 03898 { 03899 int ret, avCapRaw; 03900 reg_av_cap_t reg_av_cap = {0}; 03901 03902 ret = read_fg_register(AvCap, (uint8_t *)&(reg_av_cap)); 03903 if (ret != MAX77658_NO_ERROR) return ret; 03904 03905 //LSB is 0.1mAh. Min value is 0.0mAh and Max value is 6553.5mAh. 03906 avCapRaw = (int)reg_av_cap.bits.av_cap; 03907 *avCapmAh = (float)((float)avCapRaw * 0.1f); 03908 03909 if (*avCapmAh < 0) *avCapmAh = 0; 03910 else if (*avCapmAh > 6553.5f) *avCapmAh = 6553.5f; 03911 03912 return MAX77658_NO_ERROR; 03913 } 03914 03915 int MAX77658::set_fg_ttf(float minute) 03916 { 03917 int minuteRaw; 03918 reg_ttf_t reg_ttf = {0}; 03919 03920 //Min value is 0.0s and Max value is 102.3984h = = 6143.904 min.min. 03921 if (minute < 0) minute = 0; 03922 else if (minute > 6143.904f) minute = 6143.904f; 03923 03924 //LSB is 5.625s. 03925 minuteRaw = (int)round((float)minute * 60 / 5.625f); 03926 03927 SET_FG_BIT_FIELD(TTF, reg_ttf, reg_ttf.bits.ttf, minuteRaw); 03928 return MAX77658_NO_ERROR; 03929 } 03930 03931 int MAX77658::get_fg_ttf(float *minute) 03932 { 03933 int ret, minuteRaw; 03934 reg_ttf_t reg_ttf = {0}; 03935 03936 ret = read_fg_register(TTF, (uint8_t *)&(reg_ttf)); 03937 if (ret != MAX77658_NO_ERROR) return ret; 03938 03939 minuteRaw = (int)reg_ttf.bits.ttf; 03940 *minute = (float)((float)minuteRaw * 5.625f / 60); 03941 03942 //Min value is 0.0s and Max value is 102.3984h = = 6143.904 min.min. 03943 if (*minute < 0) *minute = 0; 03944 else if (*minute > 6143.904f) *minute = 6143.904f; 03945 03946 return MAX77658_NO_ERROR; 03947 } 03948 03949 int MAX77658::irq_disable_all() 03950 { 03951 int ret; 03952 uint8_t reg = 0; 03953 uint8_t status = 0; 03954 03955 //Disable Masks in INTM_GLBL1 03956 ret = write_register(INTM_GLBL1, ®); 03957 if (ret != MAX77658_NO_ERROR) return ret; 03958 03959 //Disable Masks in INTM_GLBL0 03960 ret = write_register(INTM_GLBL0, ®); 03961 if (ret != MAX77658_NO_ERROR) return ret; 03962 03963 //Disable Masks in INT_M_CHG 03964 ret = write_register(INT_M_CHG, ®); 03965 if (ret != MAX77658_NO_ERROR) return ret; 03966 03967 // Clear Interrupt Flags in INT_GLBL1 03968 ret = read_register(INT_GLBL1, &status); 03969 if (ret != MAX77658_NO_ERROR) return ret; 03970 03971 // Clear Interrupt Flags in INT_GLBL0 03972 ret = read_register(INT_GLBL0, &status); 03973 if (ret != MAX77658_NO_ERROR) return ret; 03974 03975 // Clear Interrupt Flags in INT_CHG 03976 ret = read_register(INT_CHG, &status); 03977 if (ret != MAX77658_NO_ERROR) return ret; 03978 03979 return MAX77658_NO_ERROR; 03980 } 03981 03982 void MAX77658::set_interrupt_handler(reg_bit_int_glbl_t id, interrupt_handler_function func, void *cb) 03983 { 03984 interrupt_handler_list[id].func = func; 03985 interrupt_handler_list[id].cb = cb; 03986 } 03987 03988 void MAX77658::post_interrupt_work() 03989 { 03990 int ret; 03991 uint8_t reg = 0, inten = 0, not_inten = 0, mask = 0; 03992 03993 while (true) { 03994 03995 ThisThread::flags_wait_any(POST_INTR_WORK_SIGNAL_ID); 03996 03997 // Check Interrupt Flags in INT_GLBL0 03998 ret = read_register(INT_GLBL0, ®); 03999 if (ret != MAX77658_NO_ERROR) return; 04000 04001 ret = read_register(INTM_GLBL0, &inten); 04002 if (ret != MAX77658_NO_ERROR) return; 04003 04004 not_inten = ~inten; // 0 means unmasked. 04005 04006 for (int i = 0; i < INT_GLBL1_GPI1_F; i++) { 04007 mask = (1 << i); 04008 if ((reg & mask) && (not_inten & mask)) { 04009 if (interrupt_handler_list[i].func != NULL) { 04010 interrupt_handler_list[i] 04011 .func(interrupt_handler_list[i].cb); 04012 } 04013 } 04014 } 04015 04016 // Check Interrupt Flags in INT_GLBL1 04017 ret = read_register(INT_GLBL1, ®); 04018 if (ret != MAX77658_NO_ERROR) return; 04019 04020 ret = read_register(INTM_GLBL1, &inten); 04021 if (ret != MAX77658_NO_ERROR) return; 04022 04023 not_inten = ~inten; // 0 means unmasked. 04024 04025 for (int i = INT_GLBL1_GPI1_F; i < INT_CHG_THM_I; i++) { 04026 mask = (1 << (i - INT_GLBL1_GPI1_F)); 04027 if ((reg & mask) && (not_inten & mask)) { 04028 if (interrupt_handler_list[i].func != NULL) { 04029 interrupt_handler_list[i] 04030 .func(interrupt_handler_list[i].cb); 04031 } 04032 } 04033 } 04034 04035 // Check Interrupt Flags in INT_CHG 04036 ret = read_register(INT_CHG, ®); 04037 if (ret != MAX77658_NO_ERROR) return; 04038 04039 ret = read_register(INT_M_CHG, &inten); 04040 if (ret != MAX77658_NO_ERROR) return; 04041 04042 not_inten = ~inten; // 0 means unmasked. 04043 04044 for (int i = INT_CHG_THM_I; i < INT_CHG_END; i++) { 04045 mask = (1 << (i - INT_CHG_THM_I)); 04046 if ((reg & mask) && (not_inten & mask)) { 04047 if (interrupt_handler_list[i].func != NULL) { 04048 interrupt_handler_list[i] 04049 .func(interrupt_handler_list[i].cb); 04050 } 04051 } 04052 } 04053 } 04054 } 04055 04056 void MAX77658::interrupt_handler() 04057 { 04058 post_intr_work_thread->flags_set(POST_INTR_WORK_SIGNAL_ID); 04059 } 04060
Generated on Fri Aug 26 2022 12:03:39 by
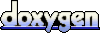