Embedded Artists
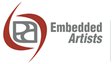
We are the leading providers of products and services around prototyping, evaluation and OEM platforms using NXP's ARM-based microcontrollers.
LPC4088DM Using the App Framework
The DMBasicGUI library comes with, among other things, a framework for creating small "Apps".
Apps should inherit from the App.h interface:
Import library
Public Member Functions |
|
virtual bool | setup () |
Prepare the
App
before it is started.
|
|
virtual void | runToCompletion ()=0 |
Runs the
App
to completion.
|
|
virtual bool | teardown () |
Cleanup.
|
The interface forces the application to implement the following three functions:
setup() | Called before the App is started to allow it to preload all resources. It may not draw on the display yet |
runToCompletion() | Should not return until the App is finished. The App owns the touch events and the display while running and should restore the display before returning. |
teardown() | Called after the runToCompletion() function has returned to free up used resources |
The DMBasicGUI includes one special App, the AppLauncher:
Import library
Public Member Functions |
|
virtual bool | setup () |
Prepare the
App
before it is started.
|
|
virtual void | runToCompletion () |
Runs the
App
to completion.
|
|
virtual bool | teardown () |
Cleanup.
|
The launcher allows up to 10 programs to be shown with icons in a graphical menu. Clicking on an application launches it.
/****************************************************************************** * Includes *****************************************************************************/ #include "mbed.h" #include "mbed_interface.h" #include "rtos.h" #include "DMBoard.h" #include "AppLauncher.h" #include "AppTouchCalibration.h" #include "AppTemplate.h" #include "image_data.h" /****************************************************************************** * Typedefs and defines *****************************************************************************/ typedef enum { MyFirstApp, // Add an application ID here CalibrationApp = AppLauncher::CalibrationApp, } myapps_t; /****************************************************************************** * Local functions *****************************************************************************/ static App* launchApp(uint32_t id) { App* a = NULL; switch ((myapps_t)id) { case CalibrationApp: a = new AppTouchCalibration(); break; case MyFirstApp: a = new AppTemplate(); break; // Create your application here default: break; } return a; } void guiTask(void const* args) { RtosLog* log = DMBoard::instance().logger(); log->printf("guiTask started\n"); AppLauncher launcher; if (launcher.setup()) { launcher.addImageButton(MyFirstApp, "Something", RED, img_empty, img_size_empty); // Add more buttons here launcher.setAppCreatorFunc(launchApp); log->printf("Starting menu system\n"); launcher.runToCompletion(); launcher.teardown(); } else { log->printf("Failed to prepare menu system\n"); } // Should never end up here mbed_die(); } /****************************************************************************** * Main function *****************************************************************************/ int main() { DMBoard::BoardError err; DMBoard* board = &DMBoard::instance(); RtosLog* log = board->logger(); err = board->init(); if (err != DMBoard::Ok) { log->printf("Failed to initialize the board, got error %d\r\n", err); wait_ms(2000); // allow RtosLog to flush messages mbed_die(); } log->printf("\n\n---\nApplication Launcher Example\nBuilt: " __DATE__ " at " __TIME__ "\n\n"); Thread tGui(guiTask, NULL, osPriorityNormal, 8192); while(1) { // Wait forever (in 1h increments) to prevent the tGui // thread from being garbage collected. Thread::wait(3600*1000); } }
Examples¶
Some examples using the App framework:
Import programlpc4088_displaymodule_everything
Example using the support package for LPC4088 DisplayModule
Import programlpc4088_displaymodule_ew2015
Demo for Embedded World 2015.
Import programlpc4088_displaymodule_empty_launcher
Example using the application launcher.
Import programlpc4088_displaymodule_shipped_demo
The out-of-the-box demo application flashed on all display modules before they are shipped.