Embedded Artists
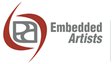
We are the leading providers of products and services around prototyping, evaluation and OEM platforms using NXP's ARM-based microcontrollers.
LPC4088DM Using SWIM
The DMBasicGUI library comes with, among other things, Simple Window Interface Manager (SWIM) developed by NXP.
Note
The SWIM library only support a display resolution with 16 bits per pixel. This is the default resolution for the display modules is 16-bit so it works unless you switch resolution.
Windows¶
All SWIM operations are based on the SWIM_WINDOW. A SWIM_WINDOW has:
- position on the display
- size (could be entire display or part of it)
- colors (background, pen and forground)
To create a SWIM_WINDOW is simple:
#include "lpc_swim.h" ... void main() { // Initialize DMBoard ... // create the frame buffer Display* disp = DMBoard::instance().display(); void* fb = disp->allocateFramebuffer(); // create a fullscreen window SWIM_WINDOW_T win; swim_window_open(&win, disp->width(), disp->height(), // full size (COLOR_T*)fb, 0,0,disp->width()-1, disp->height()-1, // window position and size 1, // border WHITE, BLUE, BLACK); // colors: pen, backgr, forgr ... }
The code above will add a 1px white border on a blue background. Set the border parameter to 0 to skip the border.
A window can be given a title using the following call:
swim_set_title(&win, "My Program", BLACK);
Texts¶
The SWIM library supports basic drawing of strings in windows. There are a lot of functions in lpc_swim.h, but the more commonly used ones are:
swim_put_text_xy(&win, "this text will be put at x=100,y=150", 100, 150); swim_put_text_centered_win(&win, "horizontally centered in the window at y=200", 200);
To have the text transparent (i.e. the background is not drawn) use this function:
swim_set_font_transparency(&win, 1); //1 for transparent backgrounds, 0 for solid color
The SWIM library comes with a couple of fonts and to switch between them use this function:
#include "lpc_swim_font.h" #include "lpc_helvr10.h" #include "lpc_rom8x8.h" #include "lpc_rom8x16.h" ... //swim_set_font(&win, font_rom8x8); swim_set_font(&win, font_rom8x16); //swim_set_font(&win, font_helvr10);
Note
The current font support only handles characters that are at most 16 pixels wide. This limits the font selection and more importantly the font sizes.
When creating a window it will automatically set font_helvr10 as font. This behavior can be changed with the swim_set_default_font() function.
#include "lpc_swim.h" #include "lpc_winfreesystem14x16.h" ... swim_set_default_font(&win, &font_winfreesys14x16); ...
All windows created after this will use the new font instead. It is of course still possible to change the font on individual windows with the swim_set_font() function as shown above.
Images¶
The SWIM library supports different ways of showing images. It does not have image decoding capabilities so use the Using Images guide to learn how to decode image files.
There are a lot of image functions in lpc_swim_image.h, but here is an example showing how to draw an image in the center of the display regardless of it's size:
#include "mbed.h" #include "DMBoard.h" #include "Image.h" #include "lpc_swim.h" #include "lpc_swim_image.h" ... void main() { // initialize DMBoard ... // create the frame buffer void* fb = disp->allocateFramebuffer(); // create a window without border swim_window_open(&win, disp->width(), disp->height(), // full size (COLOR_T*)fb, 0,0,disp->width()-1, disp->height()-1, // window position and size 0, // no border WHITE, BLUE, BLACK); // colors: pen, backgr, forgr // decode the image Image::ImageData_t img; if (Image::decode("/ram/image.png", &img) == 0) { // draw on display using SWIM swim_put_image_xy(&win, img.pixels, img.width, img.height, (disp->width() - img.width) / 2, (disp->height() - img.height) / 2); // now that the image has been copied, the raw data can be freed free(img.pointerToFree); // Start display in default mode (16-bit) Display::DisplayError disperr = disp->powerUp(fb); if (disperr != Display::DisplayError_Ok) { // Failed to initialize the display } } ... }
The example above centers the image, to instead have it streched to fill the entire display replace the swim_put_image_xy call with:
swim_put_scale_image(&win, img.pixels, disp->width(), disp->height());
Drawing¶
The SWIM library has some drawing primitives as well. They are all documented in lpc_swim.h. The primitives include:
- swim_put_pixel()
- swim_put_line()
- swim_put_circle()
- swim_put_box()
- swim_clear_screen()