Embedded Artists
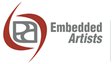
We are the leading providers of products and services around prototyping, evaluation and OEM platforms using NXP's ARM-based microcontrollers.
LPC4088DM Using Touch
All display modules have displays with touch controllers. The touch controllers could be either resistive or capacitive depending on module.
The DMSupport library handles both capacitive and resistive touch controllers with the same interface:
Import library
Public Member Functions |
|
virtual TouchError | read (touch_coordinate_t &coord)=0 |
Read coordinates from the touch panel.
|
|
virtual TouchError | read (touch_coordinate_t *coord, int num)=0 |
Read up to num coordinates from the touch panel.
|
|
virtual TouchError | info (bool *resistive, int *maxPoints, bool *calibrated)=0 |
Returns information about the touch panel.
|
|
virtual TouchError | calibrateStart ()=0 |
Start to calibrate the display.
|
|
virtual TouchError | getNextCalibratePoint (uint16_t *x, uint16_t *y, bool *last=NULL)=0 |
Get the next calibration point.
|
|
virtual TouchError | waitForCalibratePoint (bool *morePoints, uint32_t timeout)=0 |
Wait for a calibration point to have been pressed and recored.
|
Using Touch¶
To use touch it must first be enabled in the dm_board_config.h file:
#define DM_BOARD_USE_TOUCH
The initialization of the touch controller is done in the init() function in DMBoard.
The interface can be used directly by repeatedly calling the read function (i.e. polling), but the preferred way is to listen for changes like this:
// A selected event flag. Can be any that the sender and receiver agrees upon #define MY_TOUCH_FLAG (1<<0) EventFlags event_flags; static void onTouchEvent() { event_flags.set(MY_TOUCH_FLAG); } void doStuff() { TouchPanel* touch = DMBoard::instance().touchPanel(); touch->setListener(callback(onTouchEvent)); // wait for touch events touch_coordinate_t coord; while (true) { event_flags.wait_any(MY_TOUCH_FLAG); if (touch->read(coord) != TouchPanel::TouchError_Ok) { // failed to read the coordinates continue; } // use coord.x, coord.y, coord.z } }
Multitouch¶
Capacitive touch controllers normally supports multiple fingers at the same time. To test how many fingers are supported by your controller call the info() function:
TouchPanel* touch = DMBoard::instance().touchPanel(); bool isResistive; int maxFingers; bool canCalibrate; if (touch->info(&isResistive, &maxFingers, &canCalibrate) == TouchPanel::TouchError_Ok) { DMBoard::instance().logger()->printf("The touch controller supports up to %d fingers\n", maxFingers); }
Reading multiple fingers at the same time requires only small changes to the program.
// A selected signal ID. Can be any 2^x that the sender and receiver agrees upon #define MY_TOUCH_FLAG (1<<0) EventFlags event_flags; static void onTouchEvent() { event_flags.set(MY_TOUCH_FLAG); } void doStuff() { TouchPanel* touch = DMBoard::instance().touchPanel(); touch->setListener(callback(onTouchEvent)); // wait for touch events bool dontCare; int fingers; if (touch->info(&dontcare, &fingers, &dontCare) != TouchPanel::TouchError_Ok) { // something is wrong } if (fingers > 5) { fingers = 5; } touch_coordinate_t coords[5]; while (true) { event_flags.wait_any(MY_TOUCH_FLAG); if (touch->read(coords, fingers) != TouchPanel::TouchError_Ok) { // failed to read the coordinates continue; } // use coord[0].x, coord[0].y, coord[0].z, coord[1].x, ... } }
For controllers that doesn't support multitouch the number of fingers will be 1, so the same setup as for multitouch can be used. The opposite is also true, a multitouch controller can be called with the read(coord) function to only get the coordinate of the first finger.
Calibration¶
Some touch controllers can be calibrated for a better result. Use the info() function as shown above to determine if the touch controller supports calibration. The procedure of calibrating consists of:
- Call TouchPanel::calibrateStart()
- Call TouchPanel::getNextCalibratePoint() to get X and Y coordinates
- Draw a marker on the display at X, Y
- Call TouchPanel::waitForCalibratePoint() to block until the user has clicked at X, Y
- While the #morePoints# parameter to waitForCalibratePoint() is true, repeat from step 2 above
This procedure is implemented in the AppTouchCalibration app which is part of the DMBasicGUI library.