A basic graphics package for the LPC4088 Display Module.
Dependents: lpc4088_displaymodule_demo_sphere sampleGUI sampleEmptyGUI lpc4088_displaymodule_fs_aid ... more
Fork of DMBasicGUI by
lpc_swim_image.h
00001 /* 00002 * @brief SWIM image management 00003 * 00004 * @note 00005 * Copyright(C) NXP Semiconductors, 2012 00006 * All rights reserved. 00007 * 00008 * @par 00009 * Software that is described herein is for illustrative purposes only 00010 * which provides customers with programming information regarding the 00011 * LPC products. This software is supplied "AS IS" without any warranties of 00012 * any kind, and NXP Semiconductors and its licensor disclaim any and 00013 * all warranties, express or implied, including all implied warranties of 00014 * merchantability, fitness for a particular purpose and non-infringement of 00015 * intellectual property rights. NXP Semiconductors assumes no responsibility 00016 * or liability for the use of the software, conveys no license or rights under any 00017 * patent, copyright, mask work right, or any other intellectual property rights in 00018 * or to any products. NXP Semiconductors reserves the right to make changes 00019 * in the software without notification. NXP Semiconductors also makes no 00020 * representation or warranty that such application will be suitable for the 00021 * specified use without further testing or modification. 00022 * 00023 * @par 00024 * Permission to use, copy, modify, and distribute this software and its 00025 * documentation is hereby granted, under NXP Semiconductors' and its 00026 * licensor's relevant copyrights in the software, without fee, provided that it 00027 * is used in conjunction with NXP Semiconductors microcontrollers. This 00028 * copyright, permission, and disclaimer notice must appear in all copies of 00029 * this code. 00030 */ 00031 00032 #ifndef __LPC_SWIM_IMAGE_H_ 00033 #define __LPC_SWIM_IMAGE_H_ 00034 00035 #include "lpc_types.h" 00036 #include "lpc_swim.h" 00037 #include "lpc_colors.h" 00038 00039 #if defined(__cplusplus) 00040 extern "C" 00041 { 00042 #endif 00043 00044 /** @defgroup GUI_SWIM_IMAGE SWIM image manager 00045 * @ingroup GUI_SWIM 00046 * This package provides basic SWIM image management capabilities such as 00047 * image scaling, rotation, and clipping. All image data passed to SWIM 00048 * must be raw image data (stored left to right, top to bottom) in the 00049 * same color format as COLOR_T. 00050 * @{ 00051 */ 00052 00053 /** 00054 * Image rotation tags 00055 */ 00056 typedef enum {NOROTATION, RIGHT, INVERT, LEFT} SWIM_ROTATION_T; 00057 00058 /** 00059 * @brief Puts a raw image into a window 00060 * @param win : Pointer to window data structure 00061 * @param image : Pointer to raw image data 00062 * @param xsize : Horizontal size of image data 00063 * @param ysize : Vertical size of image data 00064 * @return Nothing 00065 * @note Places an image in the upper left corner of the window. 00066 * Image is cropped to the window size. 00067 */ 00068 void swim_put_image(SWIM_WINDOW_T *win, 00069 const COLOR_T *image, 00070 int32_t xsize, 00071 int32_t ysize); 00072 00073 /** 00074 * @brief Puts a raw image at the virtual X, Y coordinate in the window 00075 * @param win : Pointer to window data structure 00076 * @param image : Pointer to raw image data 00077 * @param xsize : Horizontal size of image data 00078 * @param ysize : Vertical size of image data 00079 * @param x1 : Virtual X position of pixel 00080 * @param y1 : Virtual Y position of pixel 00081 * @return Nothing 00082 * @note Places an image at the specified position in the window. 00083 * Image is cropped to the window size. 00084 */ 00085 void swim_put_image_xy(SWIM_WINDOW_T *win, 00086 const COLOR_T *image, 00087 int32_t xsize, 00088 int32_t ysize, 00089 int32_t x1, 00090 int32_t y1); 00091 00092 /** 00093 * @brief Puts a raw transparent image at the virtual X, Y coordinate in the window 00094 * @param win : Pointer to window data structure 00095 * @param image : Pointer to raw image data 00096 * @param xsize : Horizontal size of image data 00097 * @param ysize : Vertical size of image data 00098 * @param x1 : Virtual X position of pixel 00099 * @param y1 : Virtual Y position of pixel 00100 * @param tColor : Transparent color 00101 * @return Nothing 00102 * @note Places an image at the specified position in the window. 00103 * Image is cropped to the window size. Pixels matching the tColor are not drawn. 00104 */ 00105 void swim_put_transparent_image_xy(SWIM_WINDOW_T *win, 00106 const COLOR_T *image, 00107 int32_t xsize, 00108 int32_t ysize, 00109 int32_t x1, 00110 int32_t y1, 00111 COLOR_T tColor); 00112 00113 /** 00114 * @brief Puts a raw image into a window inverted 00115 * @param win : Pointer to window data structure 00116 * @param image : Pointer to raw image data 00117 * @param xsize : Horizontal size of image data 00118 * @param ysize : Vertical size of image data 00119 * @return Nothing 00120 * @note Places an image in the upper left corner of the window, 00121 * but inverts it. Image is cropped to the window size. 00122 */ 00123 void swim_put_invert_image(SWIM_WINDOW_T *win, 00124 const COLOR_T *image, 00125 int32_t xsize, 00126 int32_t ysize); 00127 00128 /** 00129 * @brief Puts a raw image into a window rotated left 00130 * @param win : Pointer to window data structure 00131 * @param image : Pointer to raw image data 00132 * @param xsize : Horizontal size of image data 00133 * @param ysize : Vertical size of image data 00134 * @return Nothing 00135 * @note Places an image in the upper left corner of the window, 00136 * but rotates it 90 degrees left. Image is cropped to the 00137 * window size. 00138 */ 00139 void swim_put_left_image(SWIM_WINDOW_T *win, 00140 const COLOR_T *image, 00141 int32_t xsize, 00142 int32_t ysize); 00143 00144 /** 00145 * @brief Puts a raw image into a window rotated right 00146 * @param win : Pointer to window data structure 00147 * @param image : Pointer to raw image data 00148 * @param xsize : Horizontal size of image data 00149 * @param ysize : Vertical size of image data 00150 * @return Nothing 00151 * @note Places an image in the upper left corner of the window, 00152 * but rotates it 90 degrees right. Image is cropped to the 00153 * window size. 00154 */ 00155 void swim_put_right_image(SWIM_WINDOW_T *win, 00156 const COLOR_T *image, 00157 int32_t xsize, 00158 int32_t ysize); 00159 00160 /** 00161 * @brief Puts and scales a raw image into a window 00162 * @param win : Pointer to window data structure 00163 * @param image : Pointer to raw image data 00164 * @param xsize : Horizontal size of image data 00165 * @param ysize : Vertical size of image data 00166 * @return Nothing 00167 * @note Scales the image to the current window. Image will be 00168 * increased or decreased in size to fit completely in 00169 * the window. 00170 */ 00171 void swim_put_scale_image(SWIM_WINDOW_T *win, 00172 const COLOR_T *image, 00173 int32_t xsize, 00174 int32_t ysize); 00175 00176 /** 00177 * @brief Puts and scales a raw image into a window inverted 00178 * @param win : Pointer to window data structure 00179 * @param image : Pointer to raw image data 00180 * @param xsize : Horizontal size of image data 00181 * @param ysize : Vertical size of image data 00182 * @return Nothing 00183 * @note Scales and inverts the image to the current window. Image 00184 * will be increased or decreased in size to fit in the window. 00185 */ 00186 void swim_put_scale_invert_image(SWIM_WINDOW_T *win, 00187 const COLOR_T *image, 00188 int32_t xsize, 00189 int32_t ysize); 00190 00191 /** 00192 * @brief Puts and scales a raw image into a window rotated left 00193 * @param win : Pointer to window data structure 00194 * @param image : Pointer to raw image data 00195 * @param xsize : Horizontal size of image data 00196 * @param ysize : Vertical size of image data 00197 * @return Nothing 00198 * @note Scales and rotates 90 degrees left the image to the current 00199 * window. Image will be increased or decreased in size to fit 00200 * in the window. 00201 */ 00202 void swim_put_scale_left_image(SWIM_WINDOW_T *win, 00203 const COLOR_T *image, 00204 int32_t xsize, 00205 int32_t ysize); 00206 00207 /** 00208 * @brief Puts and scales a raw image into a window rotated right 00209 * @param win : Pointer to window data structure 00210 * @param image : Pointer to raw image data 00211 * @param xsize : Horizontal size of image data 00212 * @param ysize : Vertical size of image data 00213 * @return Nothing 00214 * @note Scales and rotates 90 degrees right the image to the current 00215 * window. Image will be increased or decreased in size to fit 00216 * in the window. 00217 */ 00218 void swim_put_scale_right_image(SWIM_WINDOW_T *win, 00219 const COLOR_T *image, 00220 int32_t xsize, 00221 int32_t ysize); 00222 00223 /** 00224 * @brief SWIM image draw composite function 00225 * @param win : Pointer to window data structure 00226 * @param image : Pointer to raw image data 00227 * @param xsize : Horizontal size of image data 00228 * @param ysize : Vertical size of image data 00229 * @param scale : Set to 1 to scale, or 0 for cropping 00230 * @param rtype : Image rotation type 00231 * @return Nothing 00232 * @note This function provides a simple call that supports all SWIM 00233 * image functions. 00234 */ 00235 void swim_put_win_image(SWIM_WINDOW_T *win, 00236 const COLOR_T *image, 00237 int32_t xsize, 00238 int32_t ysize, 00239 int32_t scale, 00240 SWIM_ROTATION_T rtype); 00241 00242 #if defined(__cplusplus) 00243 } 00244 #endif 00245 00246 /** 00247 * @} 00248 */ 00249 00250 #endif /* __LPC_SWIM_IMAGE_H_ */ 00251
Generated on Tue Jul 12 2022 21:27:04 by
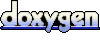