A basic graphics package for the LPC4088 Display Module.
Dependents: lpc4088_displaymodule_demo_sphere sampleGUI sampleEmptyGUI lpc4088_displaymodule_fs_aid ... more
Fork of DMBasicGUI by
SWIM image manager
This package provides basic SWIM image management capabilities such as image scaling, rotation, and clipping. More...
Enumerations | |
enum | SWIM_ROTATION_T |
Image rotation tags. More... | |
Functions | |
void | swim_put_image (SWIM_WINDOW_T *win, const COLOR_T *image, int32_t xsize, int32_t ysize) |
Puts a raw image into a window. | |
void | swim_put_image_xy (SWIM_WINDOW_T *win, const COLOR_T *image, int32_t xsize, int32_t ysize, int32_t x1, int32_t y1) |
Puts a raw image at the virtual X, Y coordinate in the window. | |
void | swim_put_transparent_image_xy (SWIM_WINDOW_T *win, const COLOR_T *image, int32_t xsize, int32_t ysize, int32_t x1, int32_t y1, COLOR_T tColor) |
Puts a raw transparent image at the virtual X, Y coordinate in the window. | |
void | swim_put_invert_image (SWIM_WINDOW_T *win, const COLOR_T *image, int32_t xsize, int32_t ysize) |
Puts a raw image into a window inverted. | |
void | swim_put_left_image (SWIM_WINDOW_T *win, const COLOR_T *image, int32_t xsize, int32_t ysize) |
Puts a raw image into a window rotated left. | |
void | swim_put_right_image (SWIM_WINDOW_T *win, const COLOR_T *image, int32_t xsize, int32_t ysize) |
Puts a raw image into a window rotated right. | |
void | swim_put_scale_image (SWIM_WINDOW_T *win, const COLOR_T *image, int32_t xsize, int32_t ysize) |
Puts and scales a raw image into a window. | |
void | swim_put_scale_invert_image (SWIM_WINDOW_T *win, const COLOR_T *image, int32_t xsize, int32_t ysize) |
Puts and scales a raw image into a window inverted. | |
void | swim_put_scale_left_image (SWIM_WINDOW_T *win, const COLOR_T *image, int32_t xsize, int32_t ysize) |
Puts and scales a raw image into a window rotated left. | |
void | swim_put_scale_right_image (SWIM_WINDOW_T *win, const COLOR_T *image, int32_t xsize, int32_t ysize) |
Puts and scales a raw image into a window rotated right. | |
void | swim_put_win_image (SWIM_WINDOW_T *win, const COLOR_T *image, int32_t xsize, int32_t ysize, int32_t scale, SWIM_ROTATION_T rtype) |
SWIM image draw composite function. |
Detailed Description
This package provides basic SWIM image management capabilities such as image scaling, rotation, and clipping.
All image data passed to SWIM must be raw image data (stored left to right, top to bottom) in the same color format as COLOR_T.
Enumeration Type Documentation
enum SWIM_ROTATION_T |
Image rotation tags.
Definition at line 56 of file lpc_swim_image.h.
Function Documentation
void swim_put_image | ( | SWIM_WINDOW_T * | win, |
const COLOR_T * | image, | ||
int32_t | xsize, | ||
int32_t | ysize | ||
) |
Puts a raw image into a window.
- Parameters:
-
win : Pointer to window data structure image : Pointer to raw image data xsize : Horizontal size of image data ysize : Vertical size of image data
- Returns:
- Nothing
- Note:
- Places an image in the upper left corner of the window. Image is cropped to the window size.
Definition at line 52 of file lpc_swim_image.c.
void swim_put_image_xy | ( | SWIM_WINDOW_T * | win, |
const COLOR_T * | image, | ||
int32_t | xsize, | ||
int32_t | ysize, | ||
int32_t | x1, | ||
int32_t | y1 | ||
) |
Puts a raw image at the virtual X, Y coordinate in the window.
- Parameters:
-
win : Pointer to window data structure image : Pointer to raw image data xsize : Horizontal size of image data ysize : Vertical size of image data x1 : Virtual X position of pixel y1 : Virtual Y position of pixel
- Returns:
- Nothing
- Note:
- Places an image at the specified position in the window. Image is cropped to the window size.
Definition at line 85 of file lpc_swim_image.c.
void swim_put_invert_image | ( | SWIM_WINDOW_T * | win, |
const COLOR_T * | image, | ||
int32_t | xsize, | ||
int32_t | ysize | ||
) |
Puts a raw image into a window inverted.
- Parameters:
-
win : Pointer to window data structure image : Pointer to raw image data xsize : Horizontal size of image data ysize : Vertical size of image data
- Returns:
- Nothing
- Note:
- Places an image in the upper left corner of the window, but inverts it. Image is cropped to the window size.
Definition at line 158 of file lpc_swim_image.c.
void swim_put_left_image | ( | SWIM_WINDOW_T * | win, |
const COLOR_T * | image, | ||
int32_t | xsize, | ||
int32_t | ysize | ||
) |
Puts a raw image into a window rotated left.
- Parameters:
-
win : Pointer to window data structure image : Pointer to raw image data xsize : Horizontal size of image data ysize : Vertical size of image data
- Returns:
- Nothing
- Note:
- Places an image in the upper left corner of the window, but rotates it 90 degrees left. Image is cropped to the window size.
Definition at line 187 of file lpc_swim_image.c.
void swim_put_right_image | ( | SWIM_WINDOW_T * | win, |
const COLOR_T * | image, | ||
int32_t | xsize, | ||
int32_t | ysize | ||
) |
Puts a raw image into a window rotated right.
- Parameters:
-
win : Pointer to window data structure image : Pointer to raw image data xsize : Horizontal size of image data ysize : Vertical size of image data
- Returns:
- Nothing
- Note:
- Places an image in the upper left corner of the window, but rotates it 90 degrees right. Image is cropped to the window size.
Definition at line 221 of file lpc_swim_image.c.
void swim_put_scale_image | ( | SWIM_WINDOW_T * | win, |
const COLOR_T * | image, | ||
int32_t | xsize, | ||
int32_t | ysize | ||
) |
Puts and scales a raw image into a window.
- Parameters:
-
win : Pointer to window data structure image : Pointer to raw image data xsize : Horizontal size of image data ysize : Vertical size of image data
- Returns:
- Nothing
- Note:
- Scales the image to the current window. Image will be increased or decreased in size to fit completely in the window.
Definition at line 253 of file lpc_swim_image.c.
void swim_put_scale_invert_image | ( | SWIM_WINDOW_T * | win, |
const COLOR_T * | image, | ||
int32_t | xsize, | ||
int32_t | ysize | ||
) |
Puts and scales a raw image into a window inverted.
- Parameters:
-
win : Pointer to window data structure image : Pointer to raw image data xsize : Horizontal size of image data ysize : Vertical size of image data
- Returns:
- Nothing
- Note:
- Scales and inverts the image to the current window. Image will be increased or decreased in size to fit in the window.
Definition at line 284 of file lpc_swim_image.c.
void swim_put_scale_left_image | ( | SWIM_WINDOW_T * | win, |
const COLOR_T * | image, | ||
int32_t | xsize, | ||
int32_t | ysize | ||
) |
Puts and scales a raw image into a window rotated left.
- Parameters:
-
win : Pointer to window data structure image : Pointer to raw image data xsize : Horizontal size of image data ysize : Vertical size of image data
- Returns:
- Nothing
- Note:
- Scales and rotates 90 degrees left the image to the current window. Image will be increased or decreased in size to fit in the window.
Definition at line 316 of file lpc_swim_image.c.
void swim_put_scale_right_image | ( | SWIM_WINDOW_T * | win, |
const COLOR_T * | image, | ||
int32_t | xsize, | ||
int32_t | ysize | ||
) |
Puts and scales a raw image into a window rotated right.
- Parameters:
-
win : Pointer to window data structure image : Pointer to raw image data xsize : Horizontal size of image data ysize : Vertical size of image data
- Returns:
- Nothing
- Note:
- Scales and rotates 90 degrees right the image to the current window. Image will be increased or decreased in size to fit in the window.
Definition at line 347 of file lpc_swim_image.c.
void swim_put_transparent_image_xy | ( | SWIM_WINDOW_T * | win, |
const COLOR_T * | image, | ||
int32_t | xsize, | ||
int32_t | ysize, | ||
int32_t | x1, | ||
int32_t | y1, | ||
COLOR_T | tColor | ||
) |
Puts a raw transparent image at the virtual X, Y coordinate in the window.
- Parameters:
-
win : Pointer to window data structure image : Pointer to raw image data xsize : Horizontal size of image data ysize : Vertical size of image data x1 : Virtual X position of pixel y1 : Virtual Y position of pixel tColor : Transparent color
- Returns:
- Nothing
- Note:
- Places an image at the specified position in the window. Image is cropped to the window size. Pixels matching the tColor are not drawn.
Definition at line 120 of file lpc_swim_image.c.
void swim_put_win_image | ( | SWIM_WINDOW_T * | win, |
const COLOR_T * | image, | ||
int32_t | xsize, | ||
int32_t | ysize, | ||
int32_t | scale, | ||
SWIM_ROTATION_T | rtype | ||
) |
SWIM image draw composite function.
- Parameters:
-
win : Pointer to window data structure image : Pointer to raw image data xsize : Horizontal size of image data ysize : Vertical size of image data scale : Set to 1 to scale, or 0 for cropping rtype : Image rotation type
- Returns:
- Nothing
- Note:
- This function provides a simple call that supports all SWIM image functions.
Definition at line 378 of file lpc_swim_image.c.
Generated on Tue Jul 12 2022 21:27:04 by
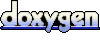