
Level 2 Project Range Device
Dependencies: N5110 SDFileSystem SRF02 TMP102 mbed
Fork of Ranger by
main.h File Reference
Header file containing functions prototypes, defines and global variables. More...
Go to the source code of this file.
Namespaces | |
namespace | LEDs |
Output for Alert LEDs. | |
namespace | BOARDLEDs |
On board LEDs. | |
namespace | Buzzer |
PWM output for Buzzer. | |
namespace | Buttons |
Button triggered Interrupts. | |
namespace | Timers |
Tickers and Timeouts. | |
namespace | Ranger |
Creat the Ranger object. | |
namespace | LCD |
Creats the LCD object. | |
namespace | TimerFlags |
Flags for use with timed interupts. | |
namespace | ButtonFlages |
Flags for use with button interupts. | |
Typedefs | |
typedef struct Alertlevel | STyp |
Functions | |
Serial | serial (USBTX, USBRX) |
for PC debug | |
SDFileSystem | sd (PTE3, PTE1, PTE2, PTE4,"sd") |
Connections to SD card holder on K64F (SPI interface) | |
void | flip () |
Function called to stop buzzer at end of on period and then make buzzer avilable again after off period. | |
void | buzzflag () |
Flag used it indicate if buzzer is avilable or on a off period. | |
void | lcdoutput () |
Controls the LCD while not in a Menu. | |
void | backlight () |
Called to increment to brightness by 0.2 each time when at 1 resets back to 0.0. | |
void | setup () |
Sets up and initalizies switches, LEDs, Tickers and serial connection. | |
void | setalert () |
A fuction used to determin the alert level given a range with the use of IF statments. | |
void | setleds () |
Function for controlling the LED outputs alert changes to element array and so output controlls. | |
void | setbuzzer () |
void | menu () |
Function used to call and navigate Main menu and change settings. | |
void | submenu () |
Function for submenu Controlling the range peramiters. | |
void | save () |
Save function saving settings. | |
void | sw2_isr () |
Inturupt flag for button 2. | |
void | sw1_isr () |
Inturupt flag for button 1. | |
void | timer_isr_led () |
Flag used with ticker for flashing of LEDs. | |
void | timer_isr_srf02 () |
Flag used for Ticker controlling SRF02 sensor reading. | |
void | timer_isr_standby () |
Flag raised for incrementing standby level. | |
Variables | |
volatile int | g_timer_flag_standby = 0 |
Flag rised by interupts. | |
int | r2 = 10 |
Upper limit of alert 2. | |
int | r3 = 20 |
Upper limit of alert 3. | |
int | r4 = 30 |
Upper limit of alert 4. | |
int | r5 = 40 |
Upper limit of alert 5. | |
int | r6 = 50 |
Upper limit of alert 6. | |
int | r7 = 70 |
Upper limit of alert 7. | |
int | totaldistance |
Veriable to hold sumation of distance readings for mean vale calculation. | |
int | page |
veriable to hold the page being viewed with in the menu | |
int | offset = 0 |
veriable to hold the offset and adjust the 0 Range point | |
int | alert |
veriable to hold the current alert level | |
int | distance |
veriable to hold the distance read from the srf02 sensor | |
int | units = 1 |
veriable to hold the current unit type set to 1 = METRIC as default | |
int | standby = 0 |
standby state 1. path clear 2. Temp 3.Temp no backlight | |
int | check_flag = 0 |
flag raised is there is a collision | |
float | Traw |
Temp reading return from TMP120 sensor. | |
float | bright = 1.0 |
veriable to hold the current LED backlight of the 5110 LCD | |
float | T |
Temperature value retured from TMP102 sensor. | |
float | distbar |
Veriable to hold the distance across the screen the distance bar should go. | |
float | avgdistance = 0 |
Averaged Distance vale from ten previous readings. | |
float | c = 1 |
convertion factor from Cm to inch 1 = metric no convertion | |
char | buffer5 [14] |
STyp | Alertlevel [8] |
Detailed Description
Header file containing functions prototypes, defines and global variables.
Ranger Project Revision 1.3.
- Date:
- 05 May 2016
The following code has been writen for the University of Leeds ELEC264501 embedded system project and is intended to create a programe that can read a distance from an SRF02 sensor and then based aponn the reading disply the distance on the screen and increment the LEDs and buzzer according the provision of a temperature sensor is also provied as a secondary function when no object is detected with in range.
The Libarys N5110, TMP102,SRF02 and have been imported from the mbed libary wizard from user Craig evans are are not of my own work.
The SRF02 libary has been edited by my self so as to include a function to read the distance from the imperial distance registers
Definition in file main.h.
Typedef Documentation
typedef struct Alertlevel STyp |
Function Documentation
void backlight | ( | ) |
void buzzflag | ( | ) |
void flip | ( | ) |
Function called to stop buzzer at end of on period and then make buzzer avilable again after off period.
- Parameters:
-
buzz_flag 1 or 0 buzzer = 0.0 buzzer off { buzz_flag = 1; buzzer = 0.0; buzzon.attach(&buzzflag,Alertlevel [alert].toneoff); }
void lcdoutput | ( | ) |
Controls the LCD while not in a Menu.
- Parameters:
-
i used to scale the max distaance to the width of the screen distance Distance read from sensor to be dispayed distbar used to adjust how far along the screen the bar is to go acorrding to distance
if (alert == 0) { if (g_timer_flag_standby) { g_timer_flag_standby = 0; T = tmp102.get_temperature(); standby++; if (standby >3) { standby = 3; } } switch (standby) { case 1: if (check_flag == 1) { sprintf(buffer4,"COLLISIONCHECK"); } lcd.clear(); sprintf(buffer,"**PATH CLEAR**"); lcd.printString(buffer,0,0); lcd.printString(buffer4,0,5); lcd.refresh(); break; case 2: if (check_flag == 1) { sprintf(buffer4,"COLLISIONCHECK"); lcd.clear(); sprintf(buffer3,"TEMP = %.2f",T); sprintf(buffer2,"TEMPERATER"); lcd.printString(buffer3,4,2); lcd.printString(buffer2,12,1); lcd.printString(buffer4,0,5); break; case 3: if (check_flag == 1) { sprintf(buffer4,"COLLISIONCHECK"); lcd.clear(); sprintf(buffer3,"TEMP = %.2f",T); sprintf(buffer2,"TEMPERATER"); lcd.refresh(); lcd.printString(buffer3,4,2); lcd.printString(buffer2,12,1); lcd.printString(buffer4,0,5); lcd.setBrightness(0); break; } } //If alert isn't 0 then the distance is to be dispayed alonng with the the distance bar else { lcd.setBrightness(bright); standby =0; lcd.clear(); if (units == 1) { sprintf(buffer,"%0.2f Cm",avgdistance); sprintf(buffer1,"****RANGE!****"); sprintf(buffer2,"DISTANCE"); sprintf(buffer4,"Menu"); } else { sprintf(buffer,"%0.2f Inches",avgdistance); sprintf(buffer1,"****RANGE!****"); sprintf(buffer2,"***DISTANCE***"); sprintf(buffer4,"Menu"); } lcd.printString(buffer,25,2); lcd.printString(buffer1,0,0); lcd.printString(buffer2,16,1); lcd.printString(buffer4,0,5); float h = (r7/84); float distbar = (avgdistance*h); //drawRect(int x0,int y0,int width,int height,int fill); lcd.drawRect(0,29,distbar,7,1); // lcd.refresh();
Maps length of bar to largest set range r7
void menu | ( | ) |
Function used to call and navigate Main menu and change settings.
{ while(1) { if (g_sw1_flag) { g_sw1_flag = 0; page++; // Moves page lcd.clear(); } switch (page) { case 0: if (g_sw2_flag) { g_sw2_flag = 0; // if it has, clear the flag backlight(); lcd.clear(); } int lightbar = bright*84; sprintf(buffer2,"%.0f%%",bright*100); lcd.drawRect(0,26,lightbar,7,1); // move bar up!!!!!!!!!!!!!!!! lcd.printString("BACKLIGHT",0,1); lcd.printString(buffer2,0,2); lcd.printString("NEXT ADJ",0,5); lcd.refresh(); break; case 1: if (g_sw2_flag) { g_sw2_flag = 0; // if it has, clear the flag if (offset == 20) { offset = 0; lcd.clear(); } else { offset += 1; } } sprintf(buffer2,"%i",offset); lcd.printString("OFFSET",0,1); sprintf(buffer4,"NEXT ADJ"); lcd.printString(buffer4,0,5); break; case 2: if (g_sw2_flag) { g_sw2_flag = 0; // if it has, clear the flag if (units == 1) { units = 0; c = 0.3937; } else { units = 1; c = 1; lcd.clear(); } } if (units == 0) { sprintf(buffer2,"IMPERIAL"); } else { sprintf(buffer2,"METRIC"); } lcd.printString("NEXT ADJ",0,5); lcd.printString("UNITS",0,1); break; case 3: if (g_sw2_flag) { g_sw2_flag = 0; // if it has, clear the flag check_flag =0; lcd.clear(); } if (check_flag == 0) { sprintf(buffer2,"COLLISION"); lcd.printString("NO",0,1); sprintf(buffer4,"NEXT "); } else { sprintf(buffer2,"COLLISION"); lcd.printString("CLEAR",0,1); sprintf(buffer4,"NEXT CLEAR"); } lcd.printString(buffer4,0,5); break; case 4: if (g_sw2_flag) { g_sw2_flag = 0; // if it has, clear the flag bright = 1.0; offset = 0; units = 1; r1 = 03;// Upper limit of alert 1 r2 = 10;// Upper limit of alert 2 r3 = 20;// Upper limit of alert 3 r4 = 30;// Upper limit of alert 4 r5 = 50;// Upper limit of alert 5 r6 = 60;// Upper limit of alert 6 r7 = 80;// Upper limit of alert 7 lcd.clear(); lcd.printString("SETTINGS",0,1); lcd.printString("RESET",0,2); wait(1); return; } sprintf(buffer2,"SETTINGS"); lcd.printString("RESET",0,1); lcd.printString("NEXT RESET",0,5); break; case 5: if (g_sw2_flag) { g_sw2_flag = 0; // if it has, clear the flag submenu(); } sprintf(buffer2,"PARAMETERS"); lcd.printString("RANGE",0,1); lcd.printString("EXIT ADJ",0,5); break; default: lcd.clear(); save (); lcd.printString(" SAVING ",0,2); lcd.printString(" SETTINGS ",0,3); wait (1); return; }// switch bracket lcd.printString(buffer2,0,2); lcd.printString("*****MENU*****",0,0); lcd.refresh(); }//while braket }//functon bracket
void save | ( | ) |
Save function saving settings.
- Parameters:
-
bright - Backlight setting units - Metric or Imperial settings offset - offest distance setting check_flag - Saves a collision alert r1 - upper limit for alert 1 r2 - upper limit for alert 2 r3 - upper limit for alert 3 r4 - upper limit for alert 4 r5 - upper limit for alert 5 r6 - upper limit for alert 6 r7 - upper limit for alert 7 { fp = fopen("/sd/settings.txt", "w"); if (fp == NULL) { // if it can't open the file then print error message serial.printf("Error! Unable to open file!\n"); } else { // opened file so can write serial.printf("Writing to file...."); fprintf(fp, "%f,%i,%i,%i,%i,%i,%i,%i,%i,%i,%i",bright,units,offset,check_flag,r1,r2,r3,r4,r5,r6,r7); // ensure data type matches serial.printf("Done.\n"); fclose(fp); // ensure you close the file after writing } }
SDFileSystem sd | ( | PTE3 | , |
PTE1 | , | ||
PTE2 | , | ||
PTE4 | , | ||
"sd" | |||
) |
Connections to SD card holder on K64F (SPI interface)
Serial serial | ( | USBTX | , |
USBRX | |||
) |
for PC debug
void setalert | ( | ) |
A fuction used to determin the alert level given a range with the use of IF statments.
- Parameters:
-
distance The distance read from sensor alert The level that distance falls with in 0 -7
- Returns:
- alert
if (distance >= r6 && distance < r7) { // r6 150 and r7 200 alert = 1; /// alert 1 distance between preset 150Cm to 200Cm } else if (distance >= r5 && distance < r6) { alert = 2; /// alert 2 when between preset 90Cm to 150Cm } else if (distance >= r4 && distance < r5) { alert = 3; /// alert 3 when distance between 60Cm to 90Cm } else if (distance >= r3 && distance < r4) { alert = 4; /// alert 4 when distance between 40Cm and 60Cm } else if ( distance > r2 && distance < r3) { alert = 5; ///alert 5 when distance between 20Cm and 40m } else if (distance > r1 && distance <= r2) { //r1 3 and r2 20 alert = 6; ///alert 6 when distance between 1 and 20 } else if (distance <=r1) { alert = 7; ///alert 7 when distance below 1Cm } else { alert = 0; /// alert 0 all else } }
alert 1 avgdistance between preset 150Cm to 200Cm
alert 2 when between preset 90Cm to 150Cm
alert 3 when avgdistance between 60Cm to 90Cm
alert 4 when avgdistance between 40Cm and 60Cm
alert 5 when avgdistance between 20Cm and 40m
alert 6 when avgdistance between 1 and 20
alert 7 when avgdistance below 1Cm
alert 0 all else
void setbuzzer | ( | ) |
{ control the PWM to drive the buzzer @param buzzer.period frequncy 1KHz @param buzzer duty cycle equal on/off max volume @param Alertlevel [alert].toneon controls how long the tone will last depending on alert buzzer.period (1.0/1000.0); buzzer = 0.5; buzzoff.attach(&flip, Alertlevel [alert].toneon); }
control the PWM to drive the buzzer
- Parameters:
-
buzzer.period frequncy 1KHz buzzer duty cycle equal on/off max volume Alertlevel[alert].toneon controls how long the tone will last depending on alert
void setleds | ( | ) |
Function for controlling the LED outputs alert changes to element array and so output controlls.
{ int flash = 0; ///Variable to toggle LEDs high low if (g_timer_flag_led) { g_timer_flag_led = 0; flash = !flash; // if it has, clear the flag } if(Alertlevel [alert].fa_led == HIGH) { a_led = flash; } else { a_led = Alertlevel [alert].sa_led; } if (Alertlevel [alert].frr_led == HIGH) { rr_led = flash; } else { rr_led = Alertlevel [alert].srr_led; } if(Alertlevel [alert].fgg_led == HIGH) { gg_led = flash; } else { gg_led = Alertlevel [alert].sgg_led; } }
Variable to toggle LEDs high low
void setup | ( | ) |
Sets up and initalizies switches, LEDs, Tickers and serial connection.
{ serial.baud(115200); // full-speed! ticker.attach(&timer_isr_led,0.35); /// Attach the ticker for the flashig LEDs ticker_srf02.attach(&timer_isr_srf02,0.2);/// Attach the ticker for collecting a range reading ticker_standby.attach(&timer_isr_standby,5.0); sw1.rise(&sw1_isr); /// sw1_isr called when button presed on the rising edge sw2.rise(&sw2_isr); /// sw2_isr called when button presed on the rising edge r_led = 1; //Onboard leds b_led = 1; //Onboard leds g_led = 1; //Onboard leds rr_led = 0; //PCB LEDS a_led = 0; //PCB LEDS gg_led = 0; //PCB LEDS sw2.mode(PullDown); //Turns on use of the pulldown resistors for use with the PCB buttons sw1.mode(PullDown); //Turns on use of the pulldown resistors for use with the PCB buttons }
Attach the ticker for the flashig LEDs
Attach the ticker for collecting a range reading
sw1_isr called when button presed on the rising edge
sw2_isr called when button presed on the rising edge
void submenu | ( | ) |
Function for submenu Controlling the range peramiters.
{ while(1) { /// interupt used to shift page if (g_sw1_flag) { g_sw1_flag = 0; subpage++; } switch (subpage) { ///interupt used to adjust range case 0: if (g_sw2_flag) { g_sw2_flag = 0; // if it has, clear the flag if (r2 == r3) { r2 = 3; } else { r2 = r2+1; } } sprintf(buffer4,"1Cm to %iCm",r2); lcd.printString("*****MENU*****",0,0); lcd.printString("RANGE",0,1); lcd.printString("PARAMETERS",0,2); lcd.printString(buffer4,0,3); lcd.printString("NEXT ADJ",0,5); break; case 1: if (g_sw2_flag) { g_sw2_flag = 0; // if it has, clear the flag if (r3 == r4) { r3 = r2; } else { r3 += 1; } } sprintf(buffer4,"%iCm to %iCm",r2,r3); lcd.printString("*****MENU*****",0,0); lcd.printString("RANGE",0,1); lcd.printString("PARAMETERS",0,2); lcd.printString(buffer4,0,3); lcd.printString("NEXT ADJ",0,5); break; case 2: if (g_sw2_flag) { g_sw2_flag = 0; // if it has, clear the flag if (r4 == r5) { r4 = r3; } else { r4 += 1; } } sprintf(buffer4,"%iCm to %iCm",r3,r4); lcd.printString("*****MENU*****",0,0); lcd.printString("RANGE",0,1); lcd.printString("PARAMETERS",0,2); lcd.printString(buffer4,0,3); lcd.printString("NEXT ADJ",0,5); break; case 3: if (g_sw2_flag) { g_sw2_flag = 0; // if it has, clear the flag if (r5 == r6) { r5 = r4; } else { r5 += 1; } } sprintf(buffer4,"%iCm to %iCm",r4,r5); lcd.printString("*****MENU*****",0,0); lcd.printString("RANGE",0,1); lcd.printString("PARAMETERS",0,2); lcd.printString(buffer4,0,3); lcd.printString("NEXT ADJ",0,5); break; case 4: if (g_sw2_flag) { g_sw2_flag = 0; // if it has, clear the flag if (r6 == r7) { r6 = r5; } else { r6 += 1; } } sprintf(buffer4,"%iCm to %iCm",r5,r6); lcd.printString("*****MENU*****",0,0); lcd.printString("RANGE",0,1); lcd.printString("PARAMETERS",0,2); lcd.printString(buffer4,0,3); lcd.printString("NEXT ADJ",0,5); break; case 5: if (g_sw2_flag) { g_sw2_flag = 0; // if it has, clear the flag if (r7 == 300) { r7 = r6; } else { r7 += 1; } } sprintf(buffer4,"%iCm to %iCm",r6,r7); lcd.printString("*****MENU*****",0,0); lcd.printString("RANGE",0,1); lcd.printString("PARAMETERS",0,2); lcd.printString(buffer4,0,3); lcd.printString("EXIT ADJ",0,5); break; default: lcd.clear(); return; }//switch breaket }//while bracket }//function bracket
interupt used to shift page
interupt used to adjust range
void sw1_isr | ( | ) |
void sw2_isr | ( | ) |
void timer_isr_led | ( | ) |
void timer_isr_srf02 | ( | ) |
void timer_isr_standby | ( | ) |
Flag raised for incrementing standby level.
{ g_timer_flag_standby = 1; // set flag in ISR by ticker_tone @param g_timer_flag_tone 0 or 1 }#
set flag in ISR by ticker_tone
- Parameters:
-
g_timer_flag_tone 0 or 1
Variable Documentation
STyp Alertlevel[8] |
{ {LOW,LOW,LOW,LOW,LOW,LOW,0,1}, {LOW,LOW,LOW,LOW,LOW,HIGH,0.1,1.0}, {LOW,LOW,HIGH,LOW,LOW,LOW,0.1,0.5}, {LOW,LOW,HIGH,LOW,HIGH,LOW,0.1,0.25}, {LOW,HIGH,HIGH,LOW,LOW,LOW,0.1,0.1}, {LOW,HIGH,HIGH,HIGH,LOW,LOW,0.2,0.1}, {HIGH,HIGH,HIGH,LOW,LOW,LOW,0.1,0.05}, {LOW,LOW,LOW,HIGH,HIGH,HIGH,1,0} }
Array contaning structures for diffent outputs
float avgdistance = 0 |
float bright = 1.0 |
char buffer5[14] |
float c = 1 |
int check_flag = 0 |
int distance |
float distbar |
volatile int g_timer_flag_standby = 0 |
int offset = 0 |
int page |
int standby = 0 |
int totaldistance |
Generated on Thu Jul 14 2022 23:13:41 by
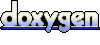