
Level 2 Project Range Device
Dependencies: N5110 SDFileSystem SRF02 TMP102 mbed
Fork of Ranger by
main.h
00001 /** 00002 @file main.h 00003 @brief Header file containing functions prototypes, defines and global variables. 00004 @brief Ranger Project 00005 @brief Revision 1.3. 00006 @author Philip Thompson 00007 @date 05 May 2016 00008 00009 @brief The following code has been writen for the University of Leeds ELEC264501 embedded system project and is intended to 00010 create a programe that can read a distance from an SRF02 sensor and then based aponn the reading disply the distance on the screen 00011 and increment the LEDs and buzzer according the provision of a temperature sensor is also provied as a secondary function when no 00012 object is detected with in range. 00013 00014 00015 @brief The Libarys N5110, TMP102,SRF02 and have been imported from the mbed libary wizard from user Craig evans are are not of my 00016 own work. 00017 00018 00019 @breif The SRF02 libary has been edited by my self so as to include a function to read the distance from the imperial distance registers 00020 */ 00021 00022 #ifndef MAIN_H 00023 #define MAIN_H 00024 #include "mbed.h" 00025 #include "mbed.h" 00026 #include "SRF02.h" 00027 #include "N5110.h" 00028 #include "TMP102.h" 00029 #include "SDFileSystem.h" 00030 00031 #define LOW 0 /// No output 00032 #define HIGH 1 /// High output 00033 00034 00035 /// for PC debug 00036 Serial serial(USBTX, USBRX); 00037 00038 00039 /** 00040 @namespace LEDs 00041 @brief Output for Alert LEDs 00042 */ 00043 DigitalOut rr_led (PTA1); 00044 DigitalOut a_led (PTC2); 00045 DigitalOut gg_led(PTB23); 00046 00047 /** 00048 @namespace BOARDLEDs 00049 @brief On board LEDs 00050 */ 00051 DigitalOut r_led(LED_RED); 00052 DigitalOut g_led(LED_GREEN); 00053 DigitalOut b_led(LED_BLUE); 00054 00055 /** 00056 @namespace Buzzer 00057 @brief PWM output for Buzzer 00058 */ 00059 PwmOut buzzer(PTA2); 00060 00061 /** 00062 @namespace Buttons 00063 @brief Button triggered Interrupts 00064 */ 00065 InterruptIn sw1(PTB19); 00066 InterruptIn sw2(PTB18); 00067 00068 /** 00069 @namespace Timers 00070 @brief Tickers and Timeouts 00071 */ 00072 Ticker ticker; // Ticker to control LED flash 00073 Ticker ticker_srf02; //Ticker to get distance reading 00074 Ticker ticker_standby; //Ticker to control standby 00075 Timeout buzzoff; //Buzzer off duratuion 00076 Timeout buzzon; // buzzer on duration 00077 00078 // Create TMP102 object 00079 TMP102 tmp102(I2C_SDA,I2C_SCL); 00080 00081 /** 00082 @namespace Ranger 00083 @brief Creat the Ranger object 00084 */ 00085 SRF02 srf02(I2C_SDA,I2C_SCL); 00086 00087 /** 00088 @namespace LCD 00089 @brief Creats the LCD object 00090 */ 00091 N5110 lcd(PTE26,PTA0,PTC4,PTD0,PTD2,PTD1,PTC3); 00092 00093 /// Connections to SD card holder on K64F (SPI interface) 00094 SDFileSystem sd(PTE3, PTE1, PTE2, PTE4, "sd"); // MOSI, MISO, SCK, CS 00095 FILE *fp; 00096 00097 //FLAGS\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\ 00098 00099 /** 00100 @namespace TimerFlags 00101 @brief Flags for use with timed interupts 00102 */ 00103 volatile int g_timer_flag_led = 0, g_timer_flag_srf02 = 0; /** Flag rised by interupts*/ 00104 volatile int g_timer_flag_standby = 0; 00105 volatile int buzz_flag = 0; 00106 /** 00107 @namespace ButtonFlages 00108 @brief Flags for use with button interupts 00109 */ 00110 volatile int g_sw1_flag = 0, g_sw2_flag = 0; 00111 00112 00113 00114 //VERIABLES\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\\ 00115 /// Upper limit of alert 1 00116 int r1 = 03; 00117 /// Upper limit of alert 2 00118 int r2 = 10; 00119 /// Upper limit of alert 3 00120 int r3 = 20; 00121 /// Upper limit of alert 4 00122 int r4 = 30; 00123 /// Upper limit of alert 5 00124 int r5 = 40; 00125 /// Upper limit of alert 6 00126 int r6 = 50; 00127 /// Upper limit of alert 7 00128 int r7 = 70; 00129 /// Veriable to hold sumation of distance readings for mean vale calculation 00130 int totaldistance; 00131 ///< veriable to hold the page being viewed with in the submenu 00132 int subpage; 00133 /// veriable to hold the page being viewed with in the menu 00134 int page; 00135 /// veriable to hold the offset and adjust the 0 Range point 00136 int offset = 0; 00137 /// veriable to hold the current alert level 00138 int alert; 00139 /// veriable to hold the distance read from the srf02 sensor 00140 int distance; 00141 /// veriable to hold the current unit type set to 1 = METRIC as default 00142 int units = 1; 00143 ///standby state 1. path clear 2. Temp 3.Temp no backlight 00144 int standby = 0; 00145 ///flag raised is there is a collision 00146 int check_flag = 0; 00147 /// Temp reading return from TMP120 sensor 00148 float Traw; 00149 /// veriable to hold the current LED backlight of the 5110 LCD 00150 float bright = 1.0; 00151 /// Temperature value retured from TMP102 sensor 00152 float T; 00153 /// Veriable to hold the distance across the screen the distance bar should go 00154 float distbar; 00155 /// Averaged Distance vale from ten previous readings 00156 float avgdistance =0; 00157 /// convertion factor from Cm to inch 1 = metric no convertion 00158 float c =1; 00159 // each character is 6 pixels wide, screen is 84 pixels (84/6 = 14) not needed to be globle but reused frquently throuhout 00160 char buffer[14], buffer1[14], buffer2[14], buffer3[14], buffer4[14],buffer5 [14]; 00161 00162 00163 /*!< Stucture to hold all outputs. Steady LEDs, Flashing LEDs, Tone on, Tonne off*/ 00164 struct Alertlevel { 00165 char srr_led; /// stead RED LED state 00166 char sa_led; /// stead AMBER LED state 00167 char sgg_led; /// stead GREEN LED state 00168 char frr_led;///FLASHING RED LED state 00169 char fa_led; ///FLASHING AMBER LED state 00170 char fgg_led; ///FLASHING GREEN LED state 00171 float toneon; ///Tone on time 00172 float toneoff; ///Tone off time 00173 }; 00174 typedef const struct Alertlevel STyp ; 00175 00176 /*!< Array contaning structures for diffent outputs */ 00177 STyp Alertlevel [8] = { 00178 {LOW,LOW,LOW,LOW,LOW,LOW,0,1}, // no output 00179 {LOW,LOW,LOW,LOW,LOW,HIGH,0.1,1.0}, //flash green 00180 {LOW,LOW,HIGH,LOW,LOW,LOW,0.1,0.5}, //steady green 00181 {LOW,LOW,HIGH,LOW,HIGH,LOW,0.1,0.25}, //flash amber 00182 {LOW,HIGH,HIGH,LOW,LOW,LOW,0.1,0.1}, //steady amber 00183 {LOW,HIGH,HIGH,HIGH,LOW,LOW,0.2,0.1}, //flash red 00184 {HIGH,HIGH,HIGH,LOW,LOW,LOW,0.1,0.05},// steady red 00185 {LOW,LOW,LOW,HIGH,HIGH,HIGH,1,0} // all flash 00186 };/*!< Array contaning structures for diffent outputs */ 00187 00188 00189 00190 00191 //FUNCTIONS///////////////////////////////////////////// 00192 00193 /** 00194 Function called to stop buzzer at end of on period and then make buzzer avilable again after off period 00195 @param buzz_flag 1 or 0 00196 @param buzzer = 0.0 buzzer off 00197 @code 00198 { 00199 buzz_flag = 1; 00200 buzzer = 0.0; 00201 buzzon.attach(&buzzflag,Alertlevel[alert].toneoff); 00202 } 00203 @endcode 00204 */ 00205 void flip(); 00206 00207 /** Flag used it indicate if buzzer is avilable or on a off period 00208 @param buzz_flag zero 00209 @code 00210 { 00211 buzz_flag = 0; 00212 return; 00213 } 00214 @endcode 00215 */ 00216 void buzzflag(); 00217 00218 /** Controls the LCD while not in a Menu 00219 00220 @param i used to scale the max distaance to the width of the screen 00221 @param distance Distance read from sensor to be dispayed 00222 @param distbar used to adjust how far along the screen the bar is to go acorrding to distance 00223 00224 @code 00225 if (alert == 0) { 00226 if (g_timer_flag_standby) { 00227 g_timer_flag_standby = 0; 00228 T = tmp102.get_temperature(); 00229 standby++; 00230 if (standby >3) { 00231 standby = 3; 00232 } 00233 } 00234 00235 switch (standby) { 00236 case 1: 00237 if (check_flag == 1) { 00238 sprintf(buffer4,"COLLISIONCHECK"); 00239 } 00240 lcd.clear(); 00241 sprintf(buffer,"**PATH CLEAR**"); 00242 lcd.printString(buffer,0,0); 00243 lcd.printString(buffer4,0,5); 00244 lcd.refresh(); 00245 break; 00246 case 2: 00247 if (check_flag == 1) { 00248 sprintf(buffer4,"COLLISIONCHECK"); 00249 lcd.clear(); 00250 sprintf(buffer3,"TEMP = %.2f",T); 00251 sprintf(buffer2,"TEMPERATER"); 00252 lcd.printString(buffer3,4,2); 00253 lcd.printString(buffer2,12,1); 00254 lcd.printString(buffer4,0,5); 00255 00256 break; 00257 case 3: 00258 if (check_flag == 1) { 00259 sprintf(buffer4,"COLLISIONCHECK"); 00260 lcd.clear(); 00261 sprintf(buffer3,"TEMP = %.2f",T); 00262 sprintf(buffer2,"TEMPERATER"); 00263 lcd.refresh(); 00264 lcd.printString(buffer3,4,2); 00265 lcd.printString(buffer2,12,1); 00266 lcd.printString(buffer4,0,5); 00267 lcd.setBrightness(0); 00268 break; 00269 } 00270 } 00271 00272 //If alert isn't 0 then the distance is to be dispayed alonng with the the distance bar 00273 else { 00274 lcd.setBrightness(bright); 00275 standby =0; 00276 lcd.clear(); 00277 00278 if (units == 1) { 00279 sprintf(buffer,"%0.2f Cm",avgdistance); 00280 sprintf(buffer1,"****RANGE!****"); 00281 sprintf(buffer2,"DISTANCE"); 00282 00283 sprintf(buffer4,"Menu"); 00284 00285 } else { 00286 sprintf(buffer,"%0.2f Inches",avgdistance); 00287 sprintf(buffer1,"****RANGE!****"); 00288 sprintf(buffer2,"***DISTANCE***"); 00289 sprintf(buffer4,"Menu"); 00290 } 00291 lcd.printString(buffer,25,2); 00292 lcd.printString(buffer1,0,0); 00293 lcd.printString(buffer2,16,1); 00294 lcd.printString(buffer4,0,5); 00295 00296 00297 float h = (r7/84); 00298 float distbar = (avgdistance*h); 00299 //drawRect(int x0,int y0,int width,int height,int fill); 00300 lcd.drawRect(0,29,distbar,7,1); // 00301 lcd.refresh(); 00302 @endcode 00303 */ 00304 void lcdoutput(); 00305 00306 /** Called to increment to brightness by 0.2 each time when at 1 resets back to 0.0 00307 @param bright 0.0-1 00308 @returns lcd.setbrightness 00309 @code 00310 if (bright == 1.0) { 00311 bright = 0; 00312 } else { 00313 bright += 0.2; 00314 } 00315 lcd.setBrightness(bright); 00316 @endcode 00317 */ 00318 void backlight(); 00319 00320 /** 00321 Sets up and initalizies switches, LEDs, Tickers and serial connection 00322 @code 00323 { 00324 serial.baud(115200); // full-speed! 00325 ticker.attach(&timer_isr_led,0.35); /// Attach the ticker for the flashig LEDs 00326 ticker_srf02.attach(&timer_isr_srf02,0.2);/// Attach the ticker for collecting a range reading 00327 ticker_standby.attach(&timer_isr_standby,5.0); 00328 sw1.rise(&sw1_isr); /// sw1_isr called when button presed on the rising edge 00329 sw2.rise(&sw2_isr); /// sw2_isr called when button presed on the rising edge 00330 r_led = 1; //Onboard leds 00331 b_led = 1; //Onboard leds 00332 g_led = 1; //Onboard leds 00333 rr_led = 0; //PCB LEDS 00334 a_led = 0; //PCB LEDS 00335 gg_led = 0; //PCB LEDS 00336 sw2.mode(PullDown); //Turns on use of the pulldown resistors for use with the PCB buttons 00337 sw1.mode(PullDown); //Turns on use of the pulldown resistors for use with the PCB buttons 00338 } 00339 @endcode 00340 */ 00341 void setup(); 00342 00343 /** A fuction used to determin the alert level given a range with the use of IF statments 00344 @param distance The distance read from sensor 00345 @param alert The level that distance falls with in 0 -7 00346 @returns alert 00347 @code 00348 if (distance >= r6 && distance < r7) { // r6 150 and r7 200 00349 alert = 1; /// alert 1 distance between preset 150Cm to 200Cm 00350 } else if (distance >= r5 && distance < r6) { 00351 alert = 2; /// alert 2 when between preset 90Cm to 150Cm 00352 } else if (distance >= r4 && distance < r5) { 00353 alert = 3; /// alert 3 when distance between 60Cm to 90Cm 00354 } else if (distance >= r3 && distance < r4) { 00355 alert = 4; /// alert 4 when distance between 40Cm and 60Cm 00356 } else if ( distance > r2 && distance < r3) { 00357 alert = 5; ///alert 5 when distance between 20Cm and 40m 00358 } else if (distance > r1 && distance <= r2) { //r1 3 and r2 20 00359 alert = 6; ///alert 6 when distance between 1 and 20 00360 } else if (distance <=r1) { 00361 alert = 7; ///alert 7 when distance below 1Cm 00362 } else { 00363 alert = 0; /// alert 0 all else 00364 } 00365 } 00366 @endcode 00367 */ 00368 void setalert(); 00369 00370 /** Function for controlling the LED outputs 00371 @para alert changes to element array and so output controlls 00372 @code 00373 { 00374 int flash = 0; ///Variable to toggle LEDs high low 00375 if (g_timer_flag_led) { 00376 g_timer_flag_led = 0; 00377 flash = !flash; // if it has, clear the flag 00378 } 00379 if(Alertlevel[alert].fa_led == HIGH) { 00380 a_led = flash; 00381 } else { 00382 a_led = Alertlevel[alert].sa_led; 00383 } 00384 if (Alertlevel[alert].frr_led == HIGH) { 00385 rr_led = flash; 00386 } else { 00387 rr_led = Alertlevel[alert].srr_led; 00388 } 00389 if(Alertlevel[alert].fgg_led == HIGH) { 00390 gg_led = flash; 00391 } else { 00392 gg_led = Alertlevel[alert].sgg_led; 00393 } 00394 } 00395 @endcode 00396 */ 00397 void setleds(); 00398 00399 /** 00400 @code 00401 { 00402 00403 control the PWM to drive the buzzer 00404 @param buzzer.period frequncy 1KHz 00405 @param buzzer duty cycle equal on/off max volume 00406 @param Alertlevel[alert].toneon controls how long the tone will last depending on alert 00407 00408 buzzer.period (1.0/1000.0); 00409 buzzer = 0.5; 00410 buzzoff.attach(&flip, Alertlevel[alert].toneon); 00411 } 00412 @endcode 00413 */ 00414 void setbuzzer (); 00415 00416 00417 /** 00418 Function used to call and navigate Main menu and change settings 00419 @code 00420 { 00421 while(1) { 00422 if (g_sw1_flag) { 00423 g_sw1_flag = 0; 00424 page++; // Moves page 00425 lcd.clear(); 00426 } 00427 switch (page) { 00428 case 0: 00429 if (g_sw2_flag) { 00430 g_sw2_flag = 0; // if it has, clear the flag 00431 backlight(); 00432 lcd.clear(); 00433 } 00434 int lightbar = bright*84; 00435 sprintf(buffer2,"%.0f%%",bright*100); 00436 lcd.drawRect(0,26,lightbar,7,1); // move bar up!!!!!!!!!!!!!!!! 00437 lcd.printString("BACKLIGHT",0,1); 00438 lcd.printString(buffer2,0,2); 00439 lcd.printString("NEXT ADJ",0,5); 00440 lcd.refresh(); 00441 break; 00442 case 1: 00443 if (g_sw2_flag) { 00444 g_sw2_flag = 0; // if it has, clear the flag 00445 if (offset == 20) { 00446 offset = 0; 00447 lcd.clear(); 00448 } else { 00449 offset += 1; 00450 } 00451 } 00452 sprintf(buffer2,"%i",offset); 00453 lcd.printString("OFFSET",0,1); 00454 sprintf(buffer4,"NEXT ADJ"); 00455 lcd.printString(buffer4,0,5); 00456 break; 00457 case 2: 00458 if (g_sw2_flag) { 00459 g_sw2_flag = 0; // if it has, clear the flag 00460 if (units == 1) { 00461 units = 0; 00462 c = 0.3937; 00463 } else { 00464 units = 1; 00465 c = 1; 00466 lcd.clear(); 00467 } 00468 } 00469 if (units == 0) { 00470 sprintf(buffer2,"IMPERIAL"); 00471 } else { 00472 sprintf(buffer2,"METRIC"); 00473 } 00474 lcd.printString("NEXT ADJ",0,5); 00475 lcd.printString("UNITS",0,1); 00476 break; 00477 case 3: 00478 if (g_sw2_flag) { 00479 g_sw2_flag = 0; // if it has, clear the flag 00480 check_flag =0; 00481 lcd.clear(); 00482 } 00483 if (check_flag == 0) { 00484 sprintf(buffer2,"COLLISION"); 00485 lcd.printString("NO",0,1); 00486 sprintf(buffer4,"NEXT "); 00487 } else { 00488 sprintf(buffer2,"COLLISION"); 00489 lcd.printString("CLEAR",0,1); 00490 sprintf(buffer4,"NEXT CLEAR"); 00491 } 00492 00493 lcd.printString(buffer4,0,5); 00494 break; 00495 case 4: 00496 if (g_sw2_flag) { 00497 g_sw2_flag = 0; // if it has, clear the flag 00498 bright = 1.0; 00499 offset = 0; 00500 units = 1; 00501 r1 = 03;// Upper limit of alert 1 00502 r2 = 10;// Upper limit of alert 2 00503 r3 = 20;// Upper limit of alert 3 00504 r4 = 30;// Upper limit of alert 4 00505 r5 = 50;// Upper limit of alert 5 00506 r6 = 60;// Upper limit of alert 6 00507 r7 = 80;// Upper limit of alert 7 00508 lcd.clear(); 00509 lcd.printString("SETTINGS",0,1); 00510 lcd.printString("RESET",0,2); 00511 wait(1); 00512 return; 00513 } 00514 sprintf(buffer2,"SETTINGS"); 00515 lcd.printString("RESET",0,1); 00516 lcd.printString("NEXT RESET",0,5); 00517 break; 00518 case 5: 00519 if (g_sw2_flag) { 00520 g_sw2_flag = 0; // if it has, clear the flag 00521 submenu(); 00522 } 00523 sprintf(buffer2,"PARAMETERS"); 00524 lcd.printString("RANGE",0,1); 00525 lcd.printString("EXIT ADJ",0,5); 00526 break; 00527 default: 00528 lcd.clear(); 00529 save (); 00530 lcd.printString(" SAVING ",0,2); 00531 lcd.printString(" SETTINGS ",0,3); 00532 wait (1); 00533 return; 00534 }// switch bracket 00535 lcd.printString(buffer2,0,2); 00536 lcd.printString("*****MENU*****",0,0); 00537 lcd.refresh(); 00538 }//while braket 00539 }//functon bracket 00540 @endcode 00541 */ 00542 void menu(); 00543 00544 00545 /** 00546 Function for submenu Controlling the range peramiters 00547 @code 00548 { 00549 while(1) { 00550 /// interupt used to shift page 00551 if (g_sw1_flag) { 00552 g_sw1_flag = 0; 00553 subpage++; 00554 } 00555 switch (subpage) { ///interupt used to adjust range 00556 case 0: 00557 if (g_sw2_flag) { 00558 g_sw2_flag = 0; // if it has, clear the flag 00559 if (r2 == r3) { 00560 r2 = 3; 00561 } else { 00562 r2 = r2+1; 00563 } 00564 } 00565 sprintf(buffer4,"1Cm to %iCm",r2); 00566 lcd.printString("*****MENU*****",0,0); 00567 lcd.printString("RANGE",0,1); 00568 lcd.printString("PARAMETERS",0,2); 00569 lcd.printString(buffer4,0,3); 00570 lcd.printString("NEXT ADJ",0,5); 00571 break; 00572 case 1: 00573 if (g_sw2_flag) { 00574 g_sw2_flag = 0; // if it has, clear the flag 00575 if (r3 == r4) { 00576 r3 = r2; 00577 } else { 00578 r3 += 1; 00579 } 00580 } 00581 sprintf(buffer4,"%iCm to %iCm",r2,r3); 00582 lcd.printString("*****MENU*****",0,0); 00583 lcd.printString("RANGE",0,1); 00584 lcd.printString("PARAMETERS",0,2); 00585 lcd.printString(buffer4,0,3); 00586 lcd.printString("NEXT ADJ",0,5); 00587 break; 00588 case 2: 00589 if (g_sw2_flag) { 00590 g_sw2_flag = 0; // if it has, clear the flag 00591 if (r4 == r5) { 00592 r4 = r3; 00593 } else { 00594 r4 += 1; 00595 } 00596 } 00597 sprintf(buffer4,"%iCm to %iCm",r3,r4); 00598 lcd.printString("*****MENU*****",0,0); 00599 lcd.printString("RANGE",0,1); 00600 lcd.printString("PARAMETERS",0,2); 00601 lcd.printString(buffer4,0,3); 00602 lcd.printString("NEXT ADJ",0,5); 00603 break; 00604 case 3: 00605 if (g_sw2_flag) { 00606 g_sw2_flag = 0; // if it has, clear the flag 00607 if (r5 == r6) { 00608 r5 = r4; 00609 } else { 00610 r5 += 1; 00611 } 00612 } 00613 sprintf(buffer4,"%iCm to %iCm",r4,r5); 00614 lcd.printString("*****MENU*****",0,0); 00615 lcd.printString("RANGE",0,1); 00616 lcd.printString("PARAMETERS",0,2); 00617 lcd.printString(buffer4,0,3); 00618 lcd.printString("NEXT ADJ",0,5); 00619 break; 00620 case 4: 00621 if (g_sw2_flag) { 00622 g_sw2_flag = 0; // if it has, clear the flag 00623 if (r6 == r7) { 00624 r6 = r5; 00625 } else { 00626 r6 += 1; 00627 } 00628 } 00629 sprintf(buffer4,"%iCm to %iCm",r5,r6); 00630 lcd.printString("*****MENU*****",0,0); 00631 lcd.printString("RANGE",0,1); 00632 lcd.printString("PARAMETERS",0,2); 00633 lcd.printString(buffer4,0,3); 00634 lcd.printString("NEXT ADJ",0,5); 00635 break; 00636 case 5: 00637 if (g_sw2_flag) { 00638 g_sw2_flag = 0; // if it has, clear the flag 00639 if (r7 == 300) { 00640 r7 = r6; 00641 } else { 00642 r7 += 1; 00643 } 00644 } 00645 sprintf(buffer4,"%iCm to %iCm",r6,r7); 00646 lcd.printString("*****MENU*****",0,0); 00647 lcd.printString("RANGE",0,1); 00648 lcd.printString("PARAMETERS",0,2); 00649 lcd.printString(buffer4,0,3); 00650 lcd.printString("EXIT ADJ",0,5); 00651 break; 00652 default: 00653 lcd.clear(); 00654 return; 00655 }//switch breaket 00656 }//while bracket 00657 }//function bracket 00658 @endcode 00659 */ 00660 void submenu(); 00661 00662 /** 00663 Save function saving settings 00664 @param bright - Backlight setting 00665 @param units - Metric or Imperial settings 00666 @param offset - offest distance setting 00667 @param check_flag - Saves a collision alert 00668 @param r1 - upper limit for alert 1 00669 @param r2 - upper limit for alert 2 00670 @param r3 - upper limit for alert 3 00671 @param r4 - upper limit for alert 4 00672 @param r5 - upper limit for alert 5 00673 @param r6 - upper limit for alert 6 00674 @param r7 - upper limit for alert 7 00675 @code 00676 { 00677 fp = fopen("/sd/settings.txt", "w"); 00678 if (fp == NULL) { // if it can't open the file then print error message 00679 serial.printf("Error! Unable to open file!\n"); 00680 } else { // opened file so can write 00681 serial.printf("Writing to file...."); 00682 fprintf(fp, "%f,%i,%i,%i,%i,%i,%i,%i,%i,%i,%i",bright,units,offset,check_flag,r1,r2,r3,r4,r5,r6,r7); // ensure data type matches 00683 serial.printf("Done.\n"); 00684 fclose(fp); // ensure you close the file after writing 00685 } 00686 } 00687 @endcode 00688 */ 00689 void save(); 00690 00691 /** 00692 Inturupt flag for button 2 00693 @code 00694 { 00695 g_sw2_flag = 1; //set flag in ISR by button 2 @param g_sw2_flag 0 or 1 00696 } 00697 @endcode 00698 */ 00699 void sw2_isr(); 00700 00701 /** 00702 Inturupt flag for button 1 00703 @code 00704 { 00705 g_sw1_flag = 1; //set flag in ISR by button 2 @param g_sw1_flag 0 or 1 00706 } 00707 @endcode 00708 */ 00709 void sw1_isr(); 00710 00711 /** 00712 Flag used with ticker for flashing of LEDs 00713 @code 00714 { 00715 g_timer_flag_led = 1; // set flag in ISR by timer_isr_led @param g_timer_flag_led 0 or 1 00716 } 00717 @endcode 00718 */ 00719 void timer_isr_led(); 00720 00721 /** 00722 Flag used for Ticker controlling SRF02 sensor reading 00723 @code 00724 { 00725 g_timer_flag_srf02 = 1; // set flag in ISR by ticker_srf02 @param g_timer_flag_srf02 0 or 1 00726 } 00727 @endcode 00728 */ 00729 void timer_isr_srf02(); 00730 00731 /** 00732 Flag raised for incrementing standby level 00733 @code 00734 { 00735 g_timer_flag_standby = 1; // set flag in ISR by ticker_tone @param g_timer_flag_tone 0 or 1 00736 }# 00737 @endcode 00738 */ 00739 void timer_isr_standby(); 00740 00741 #endif
Generated on Thu Jul 14 2022 23:13:41 by
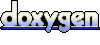