Eigne Matrix Class Library
Dependents: Eigen_test Odometry_test AttitudeEstimation_usingTicker MPU9250_Quaternion_Binary_Serial ... more
Matrix.h
00001 // This file is part of Eigen, a lightweight C++ template library 00002 // for linear algebra. 00003 // 00004 // Copyright (C) 2006-2010 Benoit Jacob <jacob.benoit.1@gmail.com> 00005 // Copyright (C) 2008-2009 Gael Guennebaud <gael.guennebaud@inria.fr> 00006 // 00007 // This Source Code Form is subject to the terms of the Mozilla 00008 // Public License v. 2.0. If a copy of the MPL was not distributed 00009 // with this file, You can obtain one at http://mozilla.org/MPL/2.0/. 00010 00011 #ifndef EIGEN_MATRIX_H 00012 #define EIGEN_MATRIX_H 00013 00014 namespace Eigen { 00015 00016 /** \class Matrix 00017 * \ingroup Core_Module 00018 * 00019 * \brief The matrix class, also used for vectors and row-vectors 00020 * 00021 * The %Matrix class is the work-horse for all \em dense (\ref dense "note") matrices and vectors within Eigen. 00022 * Vectors are matrices with one column, and row-vectors are matrices with one row. 00023 * 00024 * The %Matrix class encompasses \em both fixed-size and dynamic-size objects (\ref fixedsize "note"). 00025 * 00026 * The first three template parameters are required: 00027 * \tparam _Scalar \anchor matrix_tparam_scalar Numeric type, e.g. float, double, int or std::complex<float>. 00028 * User defined sclar types are supported as well (see \ref user_defined_scalars "here"). 00029 * \tparam _Rows Number of rows, or \b Dynamic 00030 * \tparam _Cols Number of columns, or \b Dynamic 00031 * 00032 * The remaining template parameters are optional -- in most cases you don't have to worry about them. 00033 * \tparam _Options \anchor matrix_tparam_options A combination of either \b #RowMajor or \b #ColMajor, and of either 00034 * \b #AutoAlign or \b #DontAlign. 00035 * The former controls \ref TopicStorageOrders "storage order", and defaults to column-major. The latter controls alignment, which is required 00036 * for vectorization. It defaults to aligning matrices except for fixed sizes that aren't a multiple of the packet size. 00037 * \tparam _MaxRows Maximum number of rows. Defaults to \a _Rows (\ref maxrows "note"). 00038 * \tparam _MaxCols Maximum number of columns. Defaults to \a _Cols (\ref maxrows "note"). 00039 * 00040 * Eigen provides a number of typedefs covering the usual cases. Here are some examples: 00041 * 00042 * \li \c Matrix2d is a 2x2 square matrix of doubles (\c Matrix<double, 2, 2>) 00043 * \li \c Vector4f is a vector of 4 floats (\c Matrix<float, 4, 1>) 00044 * \li \c RowVector3i is a row-vector of 3 ints (\c Matrix<int, 1, 3>) 00045 * 00046 * \li \c MatrixXf is a dynamic-size matrix of floats (\c Matrix<float, Dynamic, Dynamic>) 00047 * \li \c VectorXf is a dynamic-size vector of floats (\c Matrix<float, Dynamic, 1>) 00048 * 00049 * \li \c Matrix2Xf is a partially fixed-size (dynamic-size) matrix of floats (\c Matrix<float, 2, Dynamic>) 00050 * \li \c MatrixX3d is a partially dynamic-size (fixed-size) matrix of double (\c Matrix<double, Dynamic, 3>) 00051 * 00052 * See \link matrixtypedefs this page \endlink for a complete list of predefined \em %Matrix and \em Vector typedefs. 00053 * 00054 * You can access elements of vectors and matrices using normal subscripting: 00055 * 00056 * \code 00057 * Eigen::VectorXd v(10); 00058 * v[0] = 0.1; 00059 * v[1] = 0.2; 00060 * v(0) = 0.3; 00061 * v(1) = 0.4; 00062 * 00063 * Eigen::MatrixXi m(10, 10); 00064 * m(0, 1) = 1; 00065 * m(0, 2) = 2; 00066 * m(0, 3) = 3; 00067 * \endcode 00068 * 00069 * This class can be extended with the help of the plugin mechanism described on the page 00070 * \ref TopicCustomizingEigen by defining the preprocessor symbol \c EIGEN_MATRIX_PLUGIN. 00071 * 00072 * <i><b>Some notes:</b></i> 00073 * 00074 * <dl> 00075 * <dt><b>\anchor dense Dense versus sparse:</b></dt> 00076 * <dd>This %Matrix class handles dense, not sparse matrices and vectors. For sparse matrices and vectors, see the Sparse module. 00077 * 00078 * Dense matrices and vectors are plain usual arrays of coefficients. All the coefficients are stored, in an ordinary contiguous array. 00079 * This is unlike Sparse matrices and vectors where the coefficients are stored as a list of nonzero coefficients.</dd> 00080 * 00081 * <dt><b>\anchor fixedsize Fixed-size versus dynamic-size:</b></dt> 00082 * <dd>Fixed-size means that the numbers of rows and columns are known are compile-time. In this case, Eigen allocates the array 00083 * of coefficients as a fixed-size array, as a class member. This makes sense for very small matrices, typically up to 4x4, sometimes up 00084 * to 16x16. Larger matrices should be declared as dynamic-size even if one happens to know their size at compile-time. 00085 * 00086 * Dynamic-size means that the numbers of rows or columns are not necessarily known at compile-time. In this case they are runtime 00087 * variables, and the array of coefficients is allocated dynamically on the heap. 00088 * 00089 * Note that \em dense matrices, be they Fixed-size or Dynamic-size, <em>do not</em> expand dynamically in the sense of a std::map. 00090 * If you want this behavior, see the Sparse module.</dd> 00091 * 00092 * <dt><b>\anchor maxrows _MaxRows and _MaxCols:</b></dt> 00093 * <dd>In most cases, one just leaves these parameters to the default values. 00094 * These parameters mean the maximum size of rows and columns that the matrix may have. They are useful in cases 00095 * when the exact numbers of rows and columns are not known are compile-time, but it is known at compile-time that they cannot 00096 * exceed a certain value. This happens when taking dynamic-size blocks inside fixed-size matrices: in this case _MaxRows and _MaxCols 00097 * are the dimensions of the original matrix, while _Rows and _Cols are Dynamic.</dd> 00098 * </dl> 00099 * 00100 * \see MatrixBase for the majority of the API methods for matrices, \ref TopicClassHierarchy, 00101 * \ref TopicStorageOrders 00102 */ 00103 00104 namespace internal { 00105 template<typename _Scalar, int _Rows, int _Cols, int _Options, int _MaxRows, int _MaxCols> 00106 struct traits<Matrix<_Scalar, _Rows, _Cols, _Options, _MaxRows, _MaxCols> > 00107 { 00108 typedef _Scalar Scalar; 00109 typedef Dense StorageKind; 00110 typedef DenseIndex Index; 00111 typedef MatrixXpr XprKind; 00112 enum { 00113 RowsAtCompileTime = _Rows, 00114 ColsAtCompileTime = _Cols, 00115 MaxRowsAtCompileTime = _MaxRows, 00116 MaxColsAtCompileTime = _MaxCols, 00117 Flags = compute_matrix_flags<_Scalar, _Rows, _Cols, _Options, _MaxRows, _MaxCols>::ret, 00118 CoeffReadCost = NumTraits<Scalar>::ReadCost, 00119 Options = _Options, 00120 InnerStrideAtCompileTime = 1, 00121 OuterStrideAtCompileTime = (Options&RowMajor) ? ColsAtCompileTime : RowsAtCompileTime 00122 }; 00123 }; 00124 } 00125 00126 template<typename _Scalar, int _Rows, int _Cols, int _Options, int _MaxRows, int _MaxCols> 00127 class Matrix 00128 : public PlainObjectBase<Matrix<_Scalar, _Rows, _Cols, _Options, _MaxRows, _MaxCols> > 00129 { 00130 public: 00131 00132 /** \brief Base class typedef. 00133 * \sa PlainObjectBase 00134 */ 00135 typedef PlainObjectBase<Matrix> Base; 00136 00137 enum { Options = _Options }; 00138 00139 EIGEN_DENSE_PUBLIC_INTERFACE(Matrix) 00140 00141 typedef typename Base::PlainObject PlainObject; 00142 00143 using Base::base; 00144 using Base::coeffRef; 00145 00146 /** 00147 * \brief Assigns matrices to each other. 00148 * 00149 * \note This is a special case of the templated operator=. Its purpose is 00150 * to prevent a default operator= from hiding the templated operator=. 00151 * 00152 * \callgraph 00153 */ 00154 EIGEN_STRONG_INLINE Matrix& operator=(const Matrix& other) 00155 { 00156 return Base::_set(other); 00157 } 00158 00159 /** \internal 00160 * \brief Copies the value of the expression \a other into \c *this with automatic resizing. 00161 * 00162 * *this might be resized to match the dimensions of \a other. If *this was a null matrix (not already initialized), 00163 * it will be initialized. 00164 * 00165 * Note that copying a row-vector into a vector (and conversely) is allowed. 00166 * The resizing, if any, is then done in the appropriate way so that row-vectors 00167 * remain row-vectors and vectors remain vectors. 00168 */ 00169 template<typename OtherDerived> 00170 EIGEN_STRONG_INLINE Matrix& operator=(const MatrixBase<OtherDerived>& other) 00171 { 00172 return Base::_set(other); 00173 } 00174 00175 /* Here, doxygen failed to copy the brief information when using \copydoc */ 00176 00177 /** 00178 * \brief Copies the generic expression \a other into *this. 00179 * \copydetails DenseBase::operator=(const EigenBase<OtherDerived> &other) 00180 */ 00181 template<typename OtherDerived> 00182 EIGEN_STRONG_INLINE Matrix& operator=(const EigenBase<OtherDerived> &other) 00183 { 00184 return Base::operator=(other); 00185 } 00186 00187 template<typename OtherDerived> 00188 EIGEN_STRONG_INLINE Matrix& operator=(const ReturnByValue<OtherDerived>& func) 00189 { 00190 return Base::operator=(func); 00191 } 00192 00193 /** \brief Default constructor. 00194 * 00195 * For fixed-size matrices, does nothing. 00196 * 00197 * For dynamic-size matrices, creates an empty matrix of size 0. Does not allocate any array. Such a matrix 00198 * is called a null matrix. This constructor is the unique way to create null matrices: resizing 00199 * a matrix to 0 is not supported. 00200 * 00201 * \sa resize(Index,Index) 00202 */ 00203 EIGEN_STRONG_INLINE Matrix() : Base() 00204 { 00205 Base::_check_template_params(); 00206 EIGEN_INITIALIZE_COEFFS_IF_THAT_OPTION_IS_ENABLED 00207 } 00208 00209 // FIXME is it still needed 00210 Matrix(internal::constructor_without_unaligned_array_assert) 00211 : Base(internal::constructor_without_unaligned_array_assert()) 00212 { Base::_check_template_params(); EIGEN_INITIALIZE_COEFFS_IF_THAT_OPTION_IS_ENABLED } 00213 00214 #ifdef EIGEN_HAVE_RVALUE_REFERENCES 00215 Matrix(Matrix&& other) 00216 : Base(std::move(other)) 00217 { 00218 Base::_check_template_params(); 00219 if (RowsAtCompileTime!=Dynamic && ColsAtCompileTime!=Dynamic) 00220 Base::_set_noalias(other); 00221 } 00222 Matrix& operator=(Matrix&& other) 00223 { 00224 other.swap(*this); 00225 return *this; 00226 } 00227 #endif 00228 00229 /** \brief Constructs a vector or row-vector with given dimension. \only_for_vectors 00230 * 00231 * Note that this is only useful for dynamic-size vectors. For fixed-size vectors, 00232 * it is redundant to pass the dimension here, so it makes more sense to use the default 00233 * constructor Matrix() instead. 00234 */ 00235 EIGEN_STRONG_INLINE explicit Matrix(Index dim) 00236 : Base(dim, RowsAtCompileTime == 1 ? 1 : dim, ColsAtCompileTime == 1 ? 1 : dim) 00237 { 00238 Base::_check_template_params(); 00239 EIGEN_STATIC_ASSERT_VECTOR_ONLY(Matrix) 00240 eigen_assert(dim >= 0); 00241 eigen_assert(SizeAtCompileTime == Dynamic || SizeAtCompileTime == dim); 00242 EIGEN_INITIALIZE_COEFFS_IF_THAT_OPTION_IS_ENABLED 00243 } 00244 00245 #ifndef EIGEN_PARSED_BY_DOXYGEN 00246 template<typename T0, typename T1> 00247 EIGEN_STRONG_INLINE Matrix(const T0& x, const T1& y) 00248 { 00249 Base::_check_template_params(); 00250 Base::template _init2<T0,T1>(x, y); 00251 } 00252 #else 00253 /** \brief Constructs an uninitialized matrix with \a rows rows and \a cols columns. 00254 * 00255 * This is useful for dynamic-size matrices. For fixed-size matrices, 00256 * it is redundant to pass these parameters, so one should use the default constructor 00257 * Matrix() instead. */ 00258 Matrix(Index rows, Index cols); 00259 /** \brief Constructs an initialized 2D vector with given coefficients */ 00260 Matrix(const Scalar& x, const Scalar& y); 00261 #endif 00262 00263 /** \brief Constructs an initialized 3D vector with given coefficients */ 00264 EIGEN_STRONG_INLINE Matrix(const Scalar& x, const Scalar& y, const Scalar& z) 00265 { 00266 Base::_check_template_params(); 00267 EIGEN_STATIC_ASSERT_VECTOR_SPECIFIC_SIZE(Matrix, 3) 00268 m_storage.data()[0] = x; 00269 m_storage.data()[1] = y; 00270 m_storage.data()[2] = z; 00271 } 00272 /** \brief Constructs an initialized 4D vector with given coefficients */ 00273 EIGEN_STRONG_INLINE Matrix(const Scalar& x, const Scalar& y, const Scalar& z, const Scalar& w) 00274 { 00275 Base::_check_template_params(); 00276 EIGEN_STATIC_ASSERT_VECTOR_SPECIFIC_SIZE(Matrix, 4) 00277 m_storage.data()[0] = x; 00278 m_storage.data()[1] = y; 00279 m_storage.data()[2] = z; 00280 m_storage.data()[3] = w; 00281 } 00282 00283 explicit Matrix(const Scalar *data); 00284 00285 /** \brief Constructor copying the value of the expression \a other */ 00286 template<typename OtherDerived> 00287 EIGEN_STRONG_INLINE Matrix(const MatrixBase<OtherDerived>& other) 00288 : Base(other.rows() * other.cols(), other.rows(), other.cols()) 00289 { 00290 // This test resides here, to bring the error messages closer to the user. Normally, these checks 00291 // are performed deeply within the library, thus causing long and scary error traces. 00292 EIGEN_STATIC_ASSERT((internal::is_same<Scalar, typename OtherDerived::Scalar>::value), 00293 YOU_MIXED_DIFFERENT_NUMERIC_TYPES__YOU_NEED_TO_USE_THE_CAST_METHOD_OF_MATRIXBASE_TO_CAST_NUMERIC_TYPES_EXPLICITLY) 00294 00295 Base::_check_template_params(); 00296 Base::_set_noalias(other); 00297 } 00298 /** \brief Copy constructor */ 00299 EIGEN_STRONG_INLINE Matrix(const Matrix& other) 00300 : Base(other.rows() * other.cols(), other.rows(), other.cols()) 00301 { 00302 Base::_check_template_params(); 00303 Base::_set_noalias(other); 00304 } 00305 /** \brief Copy constructor with in-place evaluation */ 00306 template<typename OtherDerived> 00307 EIGEN_STRONG_INLINE Matrix(const ReturnByValue<OtherDerived>& other) 00308 { 00309 Base::_check_template_params(); 00310 Base::resize(other.rows(), other.cols()); 00311 other.evalTo(*this); 00312 } 00313 00314 /** \brief Copy constructor for generic expressions. 00315 * \sa MatrixBase::operator=(const EigenBase<OtherDerived>&) 00316 */ 00317 template<typename OtherDerived> 00318 EIGEN_STRONG_INLINE Matrix(const EigenBase<OtherDerived> &other) 00319 : Base(other.derived().rows() * other.derived().cols(), other.derived().rows(), other.derived().cols()) 00320 { 00321 Base::_check_template_params(); 00322 Base::_resize_to_match(other); 00323 // FIXME/CHECK: isn't *this = other.derived() more efficient. it allows to 00324 // go for pure _set() implementations, right? 00325 *this = other; 00326 } 00327 00328 /** \internal 00329 * \brief Override MatrixBase::swap() since for dynamic-sized matrices 00330 * of same type it is enough to swap the data pointers. 00331 */ 00332 template<typename OtherDerived> 00333 void swap(MatrixBase<OtherDerived> const & other) 00334 { this->_swap(other.derived()); } 00335 00336 inline Index innerStride() const { return 1; } 00337 inline Index outerStride() const { return this->innerSize(); } 00338 00339 /////////// Geometry module /////////// 00340 00341 template<typename OtherDerived> 00342 explicit Matrix(const RotationBase<OtherDerived,ColsAtCompileTime>& r); 00343 template<typename OtherDerived> 00344 Matrix& operator=(const RotationBase<OtherDerived,ColsAtCompileTime>& r); 00345 00346 #ifdef EIGEN2_SUPPORT 00347 template<typename OtherDerived> 00348 explicit Matrix(const eigen2_RotationBase<OtherDerived,ColsAtCompileTime>& r); 00349 template<typename OtherDerived> 00350 Matrix& operator=(const eigen2_RotationBase<OtherDerived,ColsAtCompileTime>& r); 00351 #endif 00352 00353 // allow to extend Matrix outside Eigen 00354 #ifdef EIGEN_MATRIX_PLUGIN 00355 #include EIGEN_MATRIX_PLUGIN 00356 #endif 00357 00358 protected: 00359 template <typename Derived, typename OtherDerived, bool IsVector> 00360 friend struct internal::conservative_resize_like_impl; 00361 00362 using Base::m_storage; 00363 }; 00364 00365 /** \defgroup matrixtypedefs Global matrix typedefs 00366 * 00367 * \ingroup Core_Module 00368 * 00369 * Eigen defines several typedef shortcuts for most common matrix and vector types. 00370 * 00371 * The general patterns are the following: 00372 * 00373 * \c MatrixSizeType where \c Size can be \c 2,\c 3,\c 4 for fixed size square matrices or \c X for dynamic size, 00374 * and where \c Type can be \c i for integer, \c f for float, \c d for double, \c cf for complex float, \c cd 00375 * for complex double. 00376 * 00377 * For example, \c Matrix3d is a fixed-size 3x3 matrix type of doubles, and \c MatrixXf is a dynamic-size matrix of floats. 00378 * 00379 * There are also \c VectorSizeType and \c RowVectorSizeType which are self-explanatory. For example, \c Vector4cf is 00380 * a fixed-size vector of 4 complex floats. 00381 * 00382 * \sa class Matrix 00383 */ 00384 00385 #define EIGEN_MAKE_TYPEDEFS(Type, TypeSuffix, Size, SizeSuffix) \ 00386 /** \ingroup matrixtypedefs */ \ 00387 typedef Matrix<Type, Size, Size> Matrix##SizeSuffix##TypeSuffix; \ 00388 /** \ingroup matrixtypedefs */ \ 00389 typedef Matrix<Type, Size, 1> Vector##SizeSuffix##TypeSuffix; \ 00390 /** \ingroup matrixtypedefs */ \ 00391 typedef Matrix<Type, 1, Size> RowVector##SizeSuffix##TypeSuffix; 00392 00393 #define EIGEN_MAKE_FIXED_TYPEDEFS(Type, TypeSuffix, Size) \ 00394 /** \ingroup matrixtypedefs */ \ 00395 typedef Matrix<Type, Size, Dynamic> Matrix##Size##X##TypeSuffix; \ 00396 /** \ingroup matrixtypedefs */ \ 00397 typedef Matrix<Type, Dynamic, Size> Matrix##X##Size##TypeSuffix; 00398 00399 #define EIGEN_MAKE_TYPEDEFS_ALL_SIZES(Type, TypeSuffix) \ 00400 EIGEN_MAKE_TYPEDEFS(Type, TypeSuffix, 2, 2) \ 00401 EIGEN_MAKE_TYPEDEFS(Type, TypeSuffix, 3, 3) \ 00402 EIGEN_MAKE_TYPEDEFS(Type, TypeSuffix, 4, 4) \ 00403 EIGEN_MAKE_TYPEDEFS(Type, TypeSuffix, Dynamic, X) \ 00404 EIGEN_MAKE_FIXED_TYPEDEFS(Type, TypeSuffix, 2) \ 00405 EIGEN_MAKE_FIXED_TYPEDEFS(Type, TypeSuffix, 3) \ 00406 EIGEN_MAKE_FIXED_TYPEDEFS(Type, TypeSuffix, 4) 00407 00408 EIGEN_MAKE_TYPEDEFS_ALL_SIZES(int, i) 00409 EIGEN_MAKE_TYPEDEFS_ALL_SIZES(float, f) 00410 EIGEN_MAKE_TYPEDEFS_ALL_SIZES(double, d) 00411 EIGEN_MAKE_TYPEDEFS_ALL_SIZES(std::complex<float>, cf) 00412 EIGEN_MAKE_TYPEDEFS_ALL_SIZES(std::complex<double>, cd) 00413 00414 #undef EIGEN_MAKE_TYPEDEFS_ALL_SIZES 00415 #undef EIGEN_MAKE_TYPEDEFS 00416 #undef EIGEN_MAKE_FIXED_TYPEDEFS 00417 00418 } // end namespace Eigen 00419 00420 #endif // EIGEN_MATRIX_H
Generated on Tue Jul 12 2022 17:46:57 by
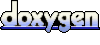