Eigne Matrix Class Library
Dependents: Eigen_test Odometry_test AttitudeEstimation_usingTicker MPU9250_Quaternion_Binary_Serial ... more
DenseBase.h
00001 // This file is part of Eigen, a lightweight C++ template library 00002 // for linear algebra. 00003 // 00004 // Copyright (C) 2007-2010 Benoit Jacob <jacob.benoit.1@gmail.com> 00005 // Copyright (C) 2008-2010 Gael Guennebaud <gael.guennebaud@inria.fr> 00006 // 00007 // This Source Code Form is subject to the terms of the Mozilla 00008 // Public License v. 2.0. If a copy of the MPL was not distributed 00009 // with this file, You can obtain one at http://mozilla.org/MPL/2.0/. 00010 00011 #ifndef EIGEN_DENSEBASE_H 00012 #define EIGEN_DENSEBASE_H 00013 00014 namespace Eigen { 00015 00016 namespace internal { 00017 00018 // The index type defined by EIGEN_DEFAULT_DENSE_INDEX_TYPE must be a signed type. 00019 // This dummy function simply aims at checking that at compile time. 00020 static inline void check_DenseIndex_is_signed() { 00021 EIGEN_STATIC_ASSERT(NumTraits<DenseIndex>::IsSigned,THE_INDEX_TYPE_MUST_BE_A_SIGNED_TYPE); 00022 } 00023 00024 } // end namespace internal 00025 00026 /** \class DenseBase 00027 * \ingroup Core_Module 00028 * 00029 * \brief Base class for all dense matrices, vectors, and arrays 00030 * 00031 * This class is the base that is inherited by all dense objects (matrix, vector, arrays, 00032 * and related expression types). The common Eigen API for dense objects is contained in this class. 00033 * 00034 * \tparam Derived is the derived type, e.g., a matrix type or an expression. 00035 * 00036 * This class can be extended with the help of the plugin mechanism described on the page 00037 * \ref TopicCustomizingEigen by defining the preprocessor symbol \c EIGEN_DENSEBASE_PLUGIN. 00038 * 00039 * \sa \ref TopicClassHierarchy 00040 */ 00041 template<typename Derived> class DenseBase 00042 #ifndef EIGEN_PARSED_BY_DOXYGEN 00043 : public internal::special_scalar_op_base<Derived, typename internal::traits<Derived>::Scalar, 00044 typename NumTraits<typename internal::traits<Derived>::Scalar>::Real, 00045 DenseCoeffsBase<Derived> > 00046 #else 00047 : public DenseCoeffsBase<Derived> 00048 #endif // not EIGEN_PARSED_BY_DOXYGEN 00049 { 00050 public: 00051 00052 class InnerIterator; 00053 00054 typedef typename internal::traits<Derived>::StorageKind StorageKind; 00055 00056 /** \brief The type of indices 00057 * \details To change this, \c \#define the preprocessor symbol \c EIGEN_DEFAULT_DENSE_INDEX_TYPE. 00058 * \sa \ref TopicPreprocessorDirectives. 00059 */ 00060 typedef typename internal::traits<Derived>::Index Index; 00061 00062 typedef typename internal::traits<Derived>::Scalar Scalar; 00063 typedef typename internal::packet_traits<Scalar>::type PacketScalar; 00064 typedef typename NumTraits<Scalar>::Real RealScalar; 00065 typedef internal::special_scalar_op_base<Derived,Scalar,RealScalar, DenseCoeffsBase<Derived> > Base; 00066 00067 using Base::operator*; 00068 using Base::derived; 00069 using Base::const_cast_derived; 00070 using Base::rows; 00071 using Base::cols; 00072 using Base::size; 00073 using Base::rowIndexByOuterInner; 00074 using Base::colIndexByOuterInner; 00075 using Base::coeff; 00076 using Base::coeffByOuterInner; 00077 using Base::packet; 00078 using Base::packetByOuterInner; 00079 using Base::writePacket; 00080 using Base::writePacketByOuterInner; 00081 using Base::coeffRef; 00082 using Base::coeffRefByOuterInner; 00083 using Base::copyCoeff; 00084 using Base::copyCoeffByOuterInner; 00085 using Base::copyPacket; 00086 using Base::copyPacketByOuterInner; 00087 using Base::operator(); 00088 using Base::operator[]; 00089 using Base::x; 00090 using Base::y; 00091 using Base::z; 00092 using Base::w; 00093 using Base::stride; 00094 using Base::innerStride; 00095 using Base::outerStride; 00096 using Base::rowStride; 00097 using Base::colStride; 00098 typedef typename Base::CoeffReturnType CoeffReturnType; 00099 00100 enum { 00101 00102 RowsAtCompileTime = internal::traits<Derived>::RowsAtCompileTime, 00103 /**< The number of rows at compile-time. This is just a copy of the value provided 00104 * by the \a Derived type. If a value is not known at compile-time, 00105 * it is set to the \a Dynamic constant. 00106 * \sa MatrixBase::rows(), MatrixBase::cols(), ColsAtCompileTime, SizeAtCompileTime */ 00107 00108 ColsAtCompileTime = internal::traits<Derived>::ColsAtCompileTime, 00109 /**< The number of columns at compile-time. This is just a copy of the value provided 00110 * by the \a Derived type. If a value is not known at compile-time, 00111 * it is set to the \a Dynamic constant. 00112 * \sa MatrixBase::rows(), MatrixBase::cols(), RowsAtCompileTime, SizeAtCompileTime */ 00113 00114 00115 SizeAtCompileTime = (internal::size_at_compile_time<internal::traits<Derived>::RowsAtCompileTime, 00116 internal::traits<Derived>::ColsAtCompileTime>::ret), 00117 /**< This is equal to the number of coefficients, i.e. the number of 00118 * rows times the number of columns, or to \a Dynamic if this is not 00119 * known at compile-time. \sa RowsAtCompileTime, ColsAtCompileTime */ 00120 00121 MaxRowsAtCompileTime = internal::traits<Derived>::MaxRowsAtCompileTime, 00122 /**< This value is equal to the maximum possible number of rows that this expression 00123 * might have. If this expression might have an arbitrarily high number of rows, 00124 * this value is set to \a Dynamic. 00125 * 00126 * This value is useful to know when evaluating an expression, in order to determine 00127 * whether it is possible to avoid doing a dynamic memory allocation. 00128 * 00129 * \sa RowsAtCompileTime, MaxColsAtCompileTime, MaxSizeAtCompileTime 00130 */ 00131 00132 MaxColsAtCompileTime = internal::traits<Derived>::MaxColsAtCompileTime, 00133 /**< This value is equal to the maximum possible number of columns that this expression 00134 * might have. If this expression might have an arbitrarily high number of columns, 00135 * this value is set to \a Dynamic. 00136 * 00137 * This value is useful to know when evaluating an expression, in order to determine 00138 * whether it is possible to avoid doing a dynamic memory allocation. 00139 * 00140 * \sa ColsAtCompileTime, MaxRowsAtCompileTime, MaxSizeAtCompileTime 00141 */ 00142 00143 MaxSizeAtCompileTime = (internal::size_at_compile_time<internal::traits<Derived>::MaxRowsAtCompileTime, 00144 internal::traits<Derived>::MaxColsAtCompileTime>::ret), 00145 /**< This value is equal to the maximum possible number of coefficients that this expression 00146 * might have. If this expression might have an arbitrarily high number of coefficients, 00147 * this value is set to \a Dynamic. 00148 * 00149 * This value is useful to know when evaluating an expression, in order to determine 00150 * whether it is possible to avoid doing a dynamic memory allocation. 00151 * 00152 * \sa SizeAtCompileTime, MaxRowsAtCompileTime, MaxColsAtCompileTime 00153 */ 00154 00155 IsVectorAtCompileTime = internal::traits<Derived>::MaxRowsAtCompileTime == 1 00156 || internal::traits<Derived>::MaxColsAtCompileTime == 1, 00157 /**< This is set to true if either the number of rows or the number of 00158 * columns is known at compile-time to be equal to 1. Indeed, in that case, 00159 * we are dealing with a column-vector (if there is only one column) or with 00160 * a row-vector (if there is only one row). */ 00161 00162 Flags = internal::traits<Derived>::Flags, 00163 /**< This stores expression \ref flags flags which may or may not be inherited by new expressions 00164 * constructed from this one. See the \ref flags "list of flags". 00165 */ 00166 00167 IsRowMajor = int(Flags) & RowMajorBit, /**< True if this expression has row-major storage order. */ 00168 00169 InnerSizeAtCompileTime = int(IsVectorAtCompileTime) ? int(SizeAtCompileTime) 00170 : int(IsRowMajor) ? int(ColsAtCompileTime) : int(RowsAtCompileTime), 00171 00172 CoeffReadCost = internal::traits<Derived>::CoeffReadCost, 00173 /**< This is a rough measure of how expensive it is to read one coefficient from 00174 * this expression. 00175 */ 00176 00177 InnerStrideAtCompileTime = internal::inner_stride_at_compile_time<Derived>::ret, 00178 OuterStrideAtCompileTime = internal::outer_stride_at_compile_time<Derived>::ret 00179 }; 00180 00181 enum { ThisConstantIsPrivateInPlainObjectBase }; 00182 00183 /** \returns the number of nonzero coefficients which is in practice the number 00184 * of stored coefficients. */ 00185 inline Index nonZeros() const { return size(); } 00186 00187 /** \returns the outer size. 00188 * 00189 * \note For a vector, this returns just 1. For a matrix (non-vector), this is the major dimension 00190 * with respect to the \ref TopicStorageOrders "storage order", i.e., the number of columns for a 00191 * column-major matrix, and the number of rows for a row-major matrix. */ 00192 Index outerSize() const 00193 { 00194 return IsVectorAtCompileTime ? 1 00195 : int(IsRowMajor) ? this->rows() : this->cols(); 00196 } 00197 00198 /** \returns the inner size. 00199 * 00200 * \note For a vector, this is just the size. For a matrix (non-vector), this is the minor dimension 00201 * with respect to the \ref TopicStorageOrders "storage order", i.e., the number of rows for a 00202 * column-major matrix, and the number of columns for a row-major matrix. */ 00203 Index innerSize() const 00204 { 00205 return IsVectorAtCompileTime ? this->size() 00206 : int(IsRowMajor) ? this->cols() : this->rows(); 00207 } 00208 00209 /** Only plain matrices/arrays, not expressions, may be resized; therefore the only useful resize methods are 00210 * Matrix::resize() and Array::resize(). The present method only asserts that the new size equals the old size, and does 00211 * nothing else. 00212 */ 00213 void resize(Index newSize) 00214 { 00215 EIGEN_ONLY_USED_FOR_DEBUG(newSize); 00216 eigen_assert(newSize == this->size() 00217 && "DenseBase::resize() does not actually allow to resize."); 00218 } 00219 /** Only plain matrices/arrays, not expressions, may be resized; therefore the only useful resize methods are 00220 * Matrix::resize() and Array::resize(). The present method only asserts that the new size equals the old size, and does 00221 * nothing else. 00222 */ 00223 void resize(Index nbRows, Index nbCols) 00224 { 00225 EIGEN_ONLY_USED_FOR_DEBUG(nbRows); 00226 EIGEN_ONLY_USED_FOR_DEBUG(nbCols); 00227 eigen_assert(nbRows == this->rows() && nbCols == this->cols() 00228 && "DenseBase::resize() does not actually allow to resize."); 00229 } 00230 00231 #ifndef EIGEN_PARSED_BY_DOXYGEN 00232 00233 /** \internal Represents a matrix with all coefficients equal to one another*/ 00234 typedef CwiseNullaryOp<internal::scalar_constant_op<Scalar>,Derived> ConstantReturnType; 00235 /** \internal Represents a vector with linearly spaced coefficients that allows sequential access only. */ 00236 typedef CwiseNullaryOp<internal::linspaced_op<Scalar,false>,Derived> SequentialLinSpacedReturnType; 00237 /** \internal Represents a vector with linearly spaced coefficients that allows random access. */ 00238 typedef CwiseNullaryOp<internal::linspaced_op<Scalar,true>,Derived> RandomAccessLinSpacedReturnType; 00239 /** \internal the return type of MatrixBase::eigenvalues() */ 00240 typedef Matrix<typename NumTraits<typename internal::traits<Derived>::Scalar>::Real, internal::traits<Derived>::ColsAtCompileTime, 1> EigenvaluesReturnType; 00241 00242 #endif // not EIGEN_PARSED_BY_DOXYGEN 00243 00244 /** Copies \a other into *this. \returns a reference to *this. */ 00245 template<typename OtherDerived> 00246 Derived& operator=(const DenseBase<OtherDerived>& other); 00247 00248 /** Special case of the template operator=, in order to prevent the compiler 00249 * from generating a default operator= (issue hit with g++ 4.1) 00250 */ 00251 Derived& operator=(const DenseBase& other); 00252 00253 template<typename OtherDerived> 00254 Derived& operator=(const EigenBase<OtherDerived> &other); 00255 00256 template<typename OtherDerived> 00257 Derived& operator+=(const EigenBase<OtherDerived> &other); 00258 00259 template<typename OtherDerived> 00260 Derived& operator-=(const EigenBase<OtherDerived> &other); 00261 00262 template<typename OtherDerived> 00263 Derived& operator=(const ReturnByValue<OtherDerived>& func); 00264 00265 /** \internal Copies \a other into *this without evaluating other. \returns a reference to *this. */ 00266 template<typename OtherDerived> 00267 Derived& lazyAssign(const DenseBase<OtherDerived>& other); 00268 00269 /** \internal Evaluates \a other into *this. \returns a reference to *this. */ 00270 template<typename OtherDerived> 00271 Derived& lazyAssign(const ReturnByValue<OtherDerived>& other); 00272 00273 CommaInitializer<Derived> operator<< (const Scalar& s); 00274 00275 template<unsigned int Added,unsigned int Removed> 00276 const Flagged<Derived, Added, Removed> flagged() const; 00277 00278 template<typename OtherDerived> 00279 CommaInitializer<Derived> operator<< (const DenseBase<OtherDerived>& other); 00280 00281 Eigen::Transpose<Derived> transpose(); 00282 typedef typename internal::add_const<Transpose<const Derived> >::type ConstTransposeReturnType; 00283 ConstTransposeReturnType transpose() const; 00284 void transposeInPlace(); 00285 #ifndef EIGEN_NO_DEBUG 00286 protected: 00287 template<typename OtherDerived> 00288 void checkTransposeAliasing(const OtherDerived& other) const; 00289 public: 00290 #endif 00291 00292 00293 static const ConstantReturnType 00294 Constant(Index rows, Index cols, const Scalar& value); 00295 static const ConstantReturnType 00296 Constant(Index size, const Scalar& value); 00297 static const ConstantReturnType 00298 Constant(const Scalar& value); 00299 00300 static const SequentialLinSpacedReturnType 00301 LinSpaced(Sequential_t, Index size, const Scalar& low, const Scalar& high); 00302 static const RandomAccessLinSpacedReturnType 00303 LinSpaced(Index size, const Scalar& low, const Scalar& high); 00304 static const SequentialLinSpacedReturnType 00305 LinSpaced(Sequential_t, const Scalar& low, const Scalar& high); 00306 static const RandomAccessLinSpacedReturnType 00307 LinSpaced(const Scalar& low, const Scalar& high); 00308 00309 template<typename CustomNullaryOp> 00310 static const CwiseNullaryOp<CustomNullaryOp, Derived> 00311 NullaryExpr(Index rows, Index cols, const CustomNullaryOp& func); 00312 template<typename CustomNullaryOp> 00313 static const CwiseNullaryOp<CustomNullaryOp, Derived> 00314 NullaryExpr(Index size, const CustomNullaryOp& func); 00315 template<typename CustomNullaryOp> 00316 static const CwiseNullaryOp<CustomNullaryOp, Derived> 00317 NullaryExpr(const CustomNullaryOp& func); 00318 00319 static const ConstantReturnType Zero(Index rows, Index cols); 00320 static const ConstantReturnType Zero(Index size); 00321 static const ConstantReturnType Zero(); 00322 static const ConstantReturnType Ones(Index rows, Index cols); 00323 static const ConstantReturnType Ones(Index size); 00324 static const ConstantReturnType Ones(); 00325 00326 void fill(const Scalar& value); 00327 Derived& setConstant(const Scalar& value); 00328 Derived& setLinSpaced(Index size, const Scalar& low, const Scalar& high); 00329 Derived& setLinSpaced(const Scalar& low, const Scalar& high); 00330 Derived& setZero(); 00331 Derived& setOnes(); 00332 Derived& setRandom(); 00333 00334 template<typename OtherDerived> 00335 bool isApprox(const DenseBase<OtherDerived>& other, 00336 const RealScalar& prec = NumTraits<Scalar>::dummy_precision()) const; 00337 bool isMuchSmallerThan(const RealScalar& other, 00338 const RealScalar& prec = NumTraits<Scalar>::dummy_precision()) const; 00339 template<typename OtherDerived> 00340 bool isMuchSmallerThan(const DenseBase<OtherDerived>& other, 00341 const RealScalar& prec = NumTraits<Scalar>::dummy_precision()) const; 00342 00343 bool isApproxToConstant(const Scalar& value, const RealScalar& prec = NumTraits<Scalar>::dummy_precision()) const; 00344 bool isConstant(const Scalar& value, const RealScalar& prec = NumTraits<Scalar>::dummy_precision()) const; 00345 bool isZero(const RealScalar& prec = NumTraits<Scalar>::dummy_precision()) const; 00346 bool isOnes(const RealScalar& prec = NumTraits<Scalar>::dummy_precision()) const; 00347 00348 inline bool hasNaN() const; 00349 inline bool allFinite() const; 00350 00351 inline Derived& operator*=(const Scalar& other); 00352 inline Derived& operator/=(const Scalar& other); 00353 00354 typedef typename internal::add_const_on_value_type<typename internal::eval<Derived>::type>::type EvalReturnType; 00355 /** \returns the matrix or vector obtained by evaluating this expression. 00356 * 00357 * Notice that in the case of a plain matrix or vector (not an expression) this function just returns 00358 * a const reference, in order to avoid a useless copy. 00359 */ 00360 EIGEN_STRONG_INLINE EvalReturnType eval() const 00361 { 00362 // Even though MSVC does not honor strong inlining when the return type 00363 // is a dynamic matrix, we desperately need strong inlining for fixed 00364 // size types on MSVC. 00365 return typename internal::eval<Derived>::type(derived()); 00366 } 00367 00368 /** swaps *this with the expression \a other. 00369 * 00370 */ 00371 template<typename OtherDerived> 00372 void swap(const DenseBase<OtherDerived>& other, 00373 int = OtherDerived::ThisConstantIsPrivateInPlainObjectBase) 00374 { 00375 SwapWrapper<Derived>(derived()).lazyAssign(other.derived()); 00376 } 00377 00378 /** swaps *this with the matrix or array \a other. 00379 * 00380 */ 00381 template<typename OtherDerived> 00382 void swap(PlainObjectBase<OtherDerived>& other) 00383 { 00384 SwapWrapper<Derived>(derived()).lazyAssign(other.derived()); 00385 } 00386 00387 00388 inline const NestByValue<Derived> nestByValue() const; 00389 inline const ForceAlignedAccess<Derived> forceAlignedAccess() const; 00390 inline ForceAlignedAccess<Derived> forceAlignedAccess(); 00391 template<bool Enable> inline const typename internal::conditional<Enable,ForceAlignedAccess<Derived>,Derived&>::type forceAlignedAccessIf() const; 00392 template<bool Enable> inline typename internal::conditional<Enable,ForceAlignedAccess<Derived>,Derived&>::type forceAlignedAccessIf(); 00393 00394 Scalar sum() const; 00395 Scalar mean() const; 00396 Scalar trace() const; 00397 00398 Scalar prod() const; 00399 00400 typename internal::traits<Derived>::Scalar minCoeff() const; 00401 typename internal::traits<Derived>::Scalar maxCoeff() const; 00402 00403 template<typename IndexType> 00404 typename internal::traits<Derived>::Scalar minCoeff(IndexType* row, IndexType* col) const; 00405 template<typename IndexType> 00406 typename internal::traits<Derived>::Scalar maxCoeff(IndexType* row, IndexType* col) const; 00407 template<typename IndexType> 00408 typename internal::traits<Derived>::Scalar minCoeff(IndexType* index) const; 00409 template<typename IndexType> 00410 typename internal::traits<Derived>::Scalar maxCoeff(IndexType* index) const; 00411 00412 template<typename BinaryOp> 00413 typename internal::result_of<BinaryOp(typename internal::traits<Derived>::Scalar)>::type 00414 redux(const BinaryOp& func) const; 00415 00416 template<typename Visitor> 00417 void visit(Visitor& func) const; 00418 00419 inline const WithFormat<Derived> format(const IOFormat& fmt) const; 00420 00421 /** \returns the unique coefficient of a 1x1 expression */ 00422 CoeffReturnType value() const 00423 { 00424 EIGEN_STATIC_ASSERT_SIZE_1x1(Derived) 00425 eigen_assert(this->rows() == 1 && this->cols() == 1); 00426 return derived().coeff(0,0); 00427 } 00428 00429 bool all(void) const; 00430 bool any(void) const; 00431 Index count() const; 00432 00433 typedef VectorwiseOp<Derived, Horizontal> RowwiseReturnType; 00434 typedef const VectorwiseOp<const Derived, Horizontal> ConstRowwiseReturnType; 00435 typedef VectorwiseOp<Derived, Vertical> ColwiseReturnType; 00436 typedef const VectorwiseOp<const Derived, Vertical> ConstColwiseReturnType; 00437 00438 ConstRowwiseReturnType rowwise() const; 00439 RowwiseReturnType rowwise(); 00440 ConstColwiseReturnType colwise() const; 00441 ColwiseReturnType colwise(); 00442 00443 static const CwiseNullaryOp<internal::scalar_random_op<Scalar>,Derived> Random(Index rows, Index cols); 00444 static const CwiseNullaryOp<internal::scalar_random_op<Scalar>,Derived> Random(Index size); 00445 static const CwiseNullaryOp<internal::scalar_random_op<Scalar>,Derived> Random(); 00446 00447 template<typename ThenDerived,typename ElseDerived> 00448 const Select<Derived,ThenDerived,ElseDerived> 00449 select(const DenseBase<ThenDerived>& thenMatrix, 00450 const DenseBase<ElseDerived>& elseMatrix) const; 00451 00452 template<typename ThenDerived> 00453 inline const Select<Derived,ThenDerived, typename ThenDerived::ConstantReturnType> 00454 select(const DenseBase<ThenDerived>& thenMatrix, const typename ThenDerived::Scalar& elseScalar) const; 00455 00456 template<typename ElseDerived> 00457 inline const Select<Derived, typename ElseDerived::ConstantReturnType, ElseDerived > 00458 select(const typename ElseDerived::Scalar& thenScalar, const DenseBase<ElseDerived>& elseMatrix) const; 00459 00460 template<int p> RealScalar lpNorm() const; 00461 00462 template<int RowFactor, int ColFactor> 00463 inline const Replicate<Derived,RowFactor,ColFactor> replicate() const; 00464 00465 typedef Replicate<Derived,Dynamic,Dynamic> ReplicateReturnType; 00466 inline const ReplicateReturnType replicate(Index rowFacor,Index colFactor) const; 00467 00468 typedef Reverse<Derived, BothDirections> ReverseReturnType; 00469 typedef const Reverse<const Derived, BothDirections> ConstReverseReturnType; 00470 ReverseReturnType reverse(); 00471 ConstReverseReturnType reverse() const; 00472 void reverseInPlace(); 00473 00474 #define EIGEN_CURRENT_STORAGE_BASE_CLASS Eigen::DenseBase 00475 # include "../plugins/BlockMethods.h" 00476 # ifdef EIGEN_DENSEBASE_PLUGIN 00477 # include EIGEN_DENSEBASE_PLUGIN 00478 # endif 00479 #undef EIGEN_CURRENT_STORAGE_BASE_CLASS 00480 00481 #ifdef EIGEN2_SUPPORT 00482 00483 Block<Derived> corner(CornerType type, Index cRows, Index cCols); 00484 const Block<Derived> corner(CornerType type, Index cRows, Index cCols) const; 00485 template<int CRows, int CCols> 00486 Block<Derived, CRows, CCols> corner(CornerType type); 00487 template<int CRows, int CCols> 00488 const Block<Derived, CRows, CCols> corner(CornerType type) const; 00489 00490 #endif // EIGEN2_SUPPORT 00491 00492 00493 // disable the use of evalTo for dense objects with a nice compilation error 00494 template<typename Dest> inline void evalTo(Dest& ) const 00495 { 00496 EIGEN_STATIC_ASSERT((internal::is_same<Dest,void>::value),THE_EVAL_EVALTO_FUNCTION_SHOULD_NEVER_BE_CALLED_FOR_DENSE_OBJECTS); 00497 } 00498 00499 protected: 00500 /** Default constructor. Do nothing. */ 00501 DenseBase() 00502 { 00503 /* Just checks for self-consistency of the flags. 00504 * Only do it when debugging Eigen, as this borders on paranoiac and could slow compilation down 00505 */ 00506 #ifdef EIGEN_INTERNAL_DEBUGGING 00507 EIGEN_STATIC_ASSERT((EIGEN_IMPLIES(MaxRowsAtCompileTime==1 && MaxColsAtCompileTime!=1, int(IsRowMajor)) 00508 && EIGEN_IMPLIES(MaxColsAtCompileTime==1 && MaxRowsAtCompileTime!=1, int(!IsRowMajor))), 00509 INVALID_STORAGE_ORDER_FOR_THIS_VECTOR_EXPRESSION) 00510 #endif 00511 } 00512 00513 private: 00514 explicit DenseBase(int); 00515 DenseBase(int,int); 00516 template<typename OtherDerived> explicit DenseBase(const DenseBase<OtherDerived>&); 00517 }; 00518 00519 } // end namespace Eigen 00520 00521 #endif // EIGEN_DENSEBASE_H
Generated on Tue Jul 12 2022 17:46:52 by
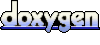