Eigne Matrix Class Library
Dependents: Eigen_test Odometry_test AttitudeEstimation_usingTicker MPU9250_Quaternion_Binary_Serial ... more
ArrayBase.h
00001 // This file is part of Eigen, a lightweight C++ template library 00002 // for linear algebra. 00003 // 00004 // Copyright (C) 2009 Gael Guennebaud <gael.guennebaud@inria.fr> 00005 // 00006 // This Source Code Form is subject to the terms of the Mozilla 00007 // Public License v. 2.0. If a copy of the MPL was not distributed 00008 // with this file, You can obtain one at http://mozilla.org/MPL/2.0/. 00009 00010 #ifndef EIGEN_ARRAYBASE_H 00011 #define EIGEN_ARRAYBASE_H 00012 00013 namespace Eigen { 00014 00015 template<typename ExpressionType> class MatrixWrapper; 00016 00017 /** \class ArrayBase 00018 * \ingroup Core_Module 00019 * 00020 * \brief Base class for all 1D and 2D array, and related expressions 00021 * 00022 * An array is similar to a dense vector or matrix. While matrices are mathematical 00023 * objects with well defined linear algebra operators, an array is just a collection 00024 * of scalar values arranged in a one or two dimensionnal fashion. As the main consequence, 00025 * all operations applied to an array are performed coefficient wise. Furthermore, 00026 * arrays support scalar math functions of the c++ standard library (e.g., std::sin(x)), and convenient 00027 * constructors allowing to easily write generic code working for both scalar values 00028 * and arrays. 00029 * 00030 * This class is the base that is inherited by all array expression types. 00031 * 00032 * \tparam Derived is the derived type, e.g., an array or an expression type. 00033 * 00034 * This class can be extended with the help of the plugin mechanism described on the page 00035 * \ref TopicCustomizingEigen by defining the preprocessor symbol \c EIGEN_ARRAYBASE_PLUGIN. 00036 * 00037 * \sa class MatrixBase, \ref TopicClassHierarchy 00038 */ 00039 template<typename Derived> class ArrayBase 00040 : public DenseBase<Derived> 00041 { 00042 public: 00043 #ifndef EIGEN_PARSED_BY_DOXYGEN 00044 /** The base class for a given storage type. */ 00045 typedef ArrayBase StorageBaseType; 00046 00047 typedef ArrayBase Eigen_BaseClassForSpecializationOfGlobalMathFuncImpl; 00048 00049 typedef typename internal::traits<Derived>::StorageKind StorageKind; 00050 typedef typename internal::traits<Derived>::Index Index; 00051 typedef typename internal::traits<Derived>::Scalar Scalar; 00052 typedef typename internal::packet_traits<Scalar>::type PacketScalar; 00053 typedef typename NumTraits<Scalar>::Real RealScalar; 00054 00055 typedef DenseBase<Derived> Base ; 00056 using Base::operator*; 00057 using Base::RowsAtCompileTime; 00058 using Base::ColsAtCompileTime; 00059 using Base::SizeAtCompileTime; 00060 using Base::MaxRowsAtCompileTime; 00061 using Base::MaxColsAtCompileTime; 00062 using Base::MaxSizeAtCompileTime; 00063 using Base::IsVectorAtCompileTime; 00064 using Base::Flags; 00065 using Base::CoeffReadCost; 00066 00067 using Base::derived; 00068 using Base::const_cast_derived; 00069 using Base::rows; 00070 using Base::cols; 00071 using Base::size; 00072 using Base::coeff; 00073 using Base::coeffRef; 00074 using Base::lazyAssign; 00075 using Base::operator=; 00076 using Base::operator+=; 00077 using Base::operator-=; 00078 using Base::operator*=; 00079 using Base::operator/=; 00080 00081 typedef typename Base::CoeffReturnType CoeffReturnType; 00082 00083 #endif // not EIGEN_PARSED_BY_DOXYGEN 00084 00085 #ifndef EIGEN_PARSED_BY_DOXYGEN 00086 /** \internal the plain matrix type corresponding to this expression. Note that is not necessarily 00087 * exactly the return type of eval(): in the case of plain matrices, the return type of eval() is a const 00088 * reference to a matrix, not a matrix! It is however guaranteed that the return type of eval() is either 00089 * PlainObject or const PlainObject&. 00090 */ 00091 typedef Array<typename internal::traits<Derived>::Scalar, 00092 internal::traits<Derived>::RowsAtCompileTime, 00093 internal::traits<Derived>::ColsAtCompileTime, 00094 AutoAlign | (internal::traits<Derived>::Flags&RowMajorBit ? RowMajor : ColMajor), 00095 internal::traits<Derived>::MaxRowsAtCompileTime, 00096 internal::traits<Derived>::MaxColsAtCompileTime 00097 > PlainObject; 00098 00099 00100 /** \internal Represents a matrix with all coefficients equal to one another*/ 00101 typedef CwiseNullaryOp<internal::scalar_constant_op<Scalar>,Derived> ConstantReturnType; 00102 #endif // not EIGEN_PARSED_BY_DOXYGEN 00103 00104 #define EIGEN_CURRENT_STORAGE_BASE_CLASS Eigen::ArrayBase 00105 # include "../plugins/CommonCwiseUnaryOps.h" 00106 # include "../plugins/MatrixCwiseUnaryOps.h" 00107 # include "../plugins/ArrayCwiseUnaryOps.h" 00108 # include "../plugins/CommonCwiseBinaryOps.h" 00109 # include "../plugins/MatrixCwiseBinaryOps.h" 00110 # include "../plugins/ArrayCwiseBinaryOps.h" 00111 # ifdef EIGEN_ARRAYBASE_PLUGIN 00112 # include EIGEN_ARRAYBASE_PLUGIN 00113 # endif 00114 #undef EIGEN_CURRENT_STORAGE_BASE_CLASS 00115 00116 /** Special case of the template operator=, in order to prevent the compiler 00117 * from generating a default operator= (issue hit with g++ 4.1) 00118 */ 00119 Derived& operator=(const ArrayBase& other) 00120 { 00121 return internal::assign_selector<Derived,Derived>::run(derived(), other.derived()); 00122 } 00123 00124 Derived& operator+=(const Scalar& scalar) 00125 { return *this = derived() + scalar; } 00126 Derived& operator-=(const Scalar& scalar) 00127 { return *this = derived() - scalar; } 00128 00129 template<typename OtherDerived> 00130 Derived& operator+=(const ArrayBase<OtherDerived>& other); 00131 template<typename OtherDerived> 00132 Derived& operator-=(const ArrayBase<OtherDerived>& other); 00133 00134 template<typename OtherDerived> 00135 Derived& operator*=(const ArrayBase<OtherDerived>& other); 00136 00137 template<typename OtherDerived> 00138 Derived& operator/=(const ArrayBase<OtherDerived>& other); 00139 00140 public: 00141 ArrayBase<Derived>& array() { return *this; } 00142 const ArrayBase<Derived>& array() const { return *this; } 00143 00144 /** \returns an \link Eigen::MatrixBase Matrix \endlink expression of this array 00145 * \sa MatrixBase::array() */ 00146 MatrixWrapper<Derived> matrix () { return derived(); } 00147 const MatrixWrapper<const Derived> matrix () const { return derived(); } 00148 00149 // template<typename Dest> 00150 // inline void evalTo(Dest& dst) const { dst = matrix(); } 00151 00152 protected: 00153 ArrayBase() : Base() {} 00154 00155 private: 00156 explicit ArrayBase(Index); 00157 ArrayBase(Index,Index); 00158 template<typename OtherDerived> explicit ArrayBase(const ArrayBase<OtherDerived>&); 00159 protected: 00160 // mixing arrays and matrices is not legal 00161 template<typename OtherDerived> Derived& operator+=(const MatrixBase<OtherDerived>& ) 00162 {EIGEN_STATIC_ASSERT(std::ptrdiff_t(sizeof(typename OtherDerived::Scalar))==-1,YOU_CANNOT_MIX_ARRAYS_AND_MATRICES); return *this;} 00163 // mixing arrays and matrices is not legal 00164 template<typename OtherDerived> Derived& operator-=(const MatrixBase<OtherDerived>& ) 00165 {EIGEN_STATIC_ASSERT(std::ptrdiff_t(sizeof(typename OtherDerived::Scalar))==-1,YOU_CANNOT_MIX_ARRAYS_AND_MATRICES); return *this;} 00166 }; 00167 00168 /** replaces \c *this by \c *this - \a other. 00169 * 00170 * \returns a reference to \c *this 00171 */ 00172 template<typename Derived> 00173 template<typename OtherDerived> 00174 EIGEN_STRONG_INLINE Derived & 00175 ArrayBase<Derived>::operator-=(const ArrayBase<OtherDerived> &other) 00176 { 00177 SelfCwiseBinaryOp<internal::scalar_difference_op<Scalar>, Derived, OtherDerived> tmp(derived()); 00178 tmp = other.derived(); 00179 return derived(); 00180 } 00181 00182 /** replaces \c *this by \c *this + \a other. 00183 * 00184 * \returns a reference to \c *this 00185 */ 00186 template<typename Derived> 00187 template<typename OtherDerived> 00188 EIGEN_STRONG_INLINE Derived & 00189 ArrayBase<Derived>::operator+=(const ArrayBase<OtherDerived>& other) 00190 { 00191 SelfCwiseBinaryOp<internal::scalar_sum_op<Scalar>, Derived, OtherDerived> tmp(derived()); 00192 tmp = other.derived(); 00193 return derived(); 00194 } 00195 00196 /** replaces \c *this by \c *this * \a other coefficient wise. 00197 * 00198 * \returns a reference to \c *this 00199 */ 00200 template<typename Derived> 00201 template<typename OtherDerived> 00202 EIGEN_STRONG_INLINE Derived & 00203 ArrayBase<Derived>::operator*=(const ArrayBase<OtherDerived>& other) 00204 { 00205 SelfCwiseBinaryOp<internal::scalar_product_op<Scalar>, Derived, OtherDerived> tmp(derived()); 00206 tmp = other.derived(); 00207 return derived(); 00208 } 00209 00210 /** replaces \c *this by \c *this / \a other coefficient wise. 00211 * 00212 * \returns a reference to \c *this 00213 */ 00214 template<typename Derived> 00215 template<typename OtherDerived> 00216 EIGEN_STRONG_INLINE Derived & 00217 ArrayBase<Derived>::operator/=(const ArrayBase<OtherDerived>& other) 00218 { 00219 SelfCwiseBinaryOp<internal::scalar_quotient_op<Scalar>, Derived, OtherDerived> tmp(derived()); 00220 tmp = other.derived(); 00221 return derived(); 00222 } 00223 00224 } // end namespace Eigen 00225 00226 #endif // EIGEN_ARRAYBASE_H
Generated on Tue Jul 12 2022 17:46:49 by
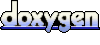