Fork of SSD1289 lib for Landtiger board
SSD1289 Class Reference
Represents a LCD instance. More...
#include <ssd1289.h>
Public Member Functions | |
SSD1289 (PinName CS_PIN, PinName RESET_PIN, PinName RS_PIN, PinName WR_PIN, BusOut *DATA_PORT, PinName RD_PIN=NC) | |
Creates a new instance of the class. | |
void | Initialize (orientation_t orientation=LANDSCAPE) |
Initialize display. | |
void | SetForeground (unsigned short color=COLOR_WHITE) |
Set the foreground color for painting. | |
void | SetBackground (unsigned short color=COLOR_BLACK) |
Set the background color for painting. | |
void | SetFont (const char *font) |
Sets the font to be used for painting of text on the screen. | |
void | FillScreen (int color=-1) |
Fills the whole screen with a single color. | |
void | ClearScreen (void) |
Clears the screen. | |
void | DrawPixel (unsigned short x, unsigned short y, int color=-2) |
Draws a pixel at the specified location. | |
void | DrawLine (unsigned short x1, unsigned short y1, unsigned short x2, unsigned short y2, int color=-2) |
Draws a line. | |
void | DrawRect (unsigned short x1, unsigned short y1, unsigned short x2, unsigned short y2, int color=-2) |
Paints a rectangle. | |
void | DrawRoundRect (unsigned short x1, unsigned short y1, unsigned short x2, unsigned short y2, int color=-2) |
Paints a rectangle and fills it with the paint color. | |
void | FillRect (unsigned short x1, unsigned short y1, unsigned short x2, unsigned short y2, int color=-2) |
Paints a rectangle with rounded corners. | |
void | FillRoundRect (unsigned short x1, unsigned short y1, unsigned short x2, unsigned short y2, int color=-2) |
Paints a rectangle with rounded corners and fills it with the paint color. | |
void | DrawCircle (unsigned short x, unsigned short y, unsigned short radius, int color=-2) |
Paints a circle. | |
void | FillCircle (unsigned short x, unsigned short y, unsigned short radius, int color=-2) |
Paints a circle and fills it with the paint color. | |
void | Print (const char *str, unsigned short x, unsigned short y, int fgColor=-2, int bgColor=-1, unsigned short deg=0) |
Print a text on the screen. | |
void | DrawBitmap (unsigned short x, unsigned short y, unsigned short sx, unsigned short sy, bitmap_t data, unsigned char scale=1) |
Draw an image on the screen. | |
void | DrawBitmap (unsigned short x, unsigned short y, unsigned short sx, unsigned short sy, bitmap_t data, unsigned short deg, unsigned short rox, unsigned short roy) |
Draw an image on the screen. |
Detailed Description
Represents a LCD instance.
This is the utility class, through which the display can be manipulated and graphics objects can be shown to the user. A known display, which works with this library is the ITDB02-3.2S from iTeadStudio - a RGB TFT with 240x320 pixels resolution and 65K colors, using 16-bit interface.
The display needs 20 or 21 pins to work with mbed, so it is possibly not the best of choices out there, but other than that it uses +3.3V for power and logic, as well as the backlight, thus can be interfaced directly to the mbed without the need of shields or level shifters as with Arduino.
How to use:
// include the library, this will also pull in the header for the provided fonts #include "ssd1289.h" // prepare the data bus for writing commands and pixel data BusOut dataBus( p30, p29, p28, p27, p26, p25, p24, p23, p22, p21, p20, p19, p18, p17, p16, p15 ); // 16 pins // create the lcd instance SSD1289 lcd( p14, p13, p12, p11, &dataBus ); // control pins and data bus int main() { // initialize display - place it in standard portrait mode and set background to black and // foreground to white color. lcd.Initialize(); // set current font to the smallest 8x12 pixels font. lcd.SetFont( Font8x12 ); // print something on the screen lcd.Print( "Hello, World!", CENTER, 25 ); // align text to center horizontally and use starndard colors while ( 1 ) { } }
Definition at line 141 of file ssd1289.h.
Constructor & Destructor Documentation
SSD1289 | ( | PinName | CS_PIN, |
PinName | RESET_PIN, | ||
PinName | RS_PIN, | ||
PinName | WR_PIN, | ||
BusOut * | DATA_PORT, | ||
PinName | RD_PIN = NC |
||
) |
Creates a new instance of the class.
- Parameters:
-
CS_PIN Pin for the ChipSelect signal. RESET_PIN Pin for the RESET line. RS_PIN Pin for the RS signal. WR_PIN Pin for the WR signal. DATA_PORT Address of the data bus for transfer of commands and pixel data. RD_PIN Pin for the RD signal. This line is not needed by the driver, so if you would like to use the pin on the mbed for something else, just pull-up the respective pin on the LCD high, and do not assign a value to this parameter when creating the controller instance.
Definition at line 13 of file ssd1289.cpp.
Member Function Documentation
void ClearScreen | ( | void | ) |
Clears the screen.
This is the same as calling FillScreen() or FillScreen( -1 ) to use the background color.
Definition at line 117 of file ssd1289.cpp.
void DrawBitmap | ( | unsigned short | x, |
unsigned short | y, | ||
unsigned short | sx, | ||
unsigned short | sy, | ||
bitmap_t | data, | ||
unsigned char | scale = 1 |
||
) |
Draw an image on the screen.
The pixels of the picture must be in the RGB-565 format. The data can be provided as an array in a source or a header file. To convert an image file to the appropriate format, a special utility must be utilized. One such tool is provided by Henning Karlsen, the author of the UTFT display liberary and can be downloaded for free from his web site: http://henningkarlsen.com/electronics/library.php?id=52
- Parameters:
-
x Horizontal offset of the first pixel of the image. y Vertical offset of the first pixel of the image sx Width of the image. sy Height of the image. data Image pixel array. scale A value of 1 will produce an image with its original size, while a different value will scale the image.
Definition at line 392 of file ssd1289.cpp.
void DrawBitmap | ( | unsigned short | x, |
unsigned short | y, | ||
unsigned short | sx, | ||
unsigned short | sy, | ||
bitmap_t | data, | ||
unsigned short | deg, | ||
unsigned short | rox, | ||
unsigned short | roy | ||
) |
Draw an image on the screen.
The pixels of the picture must be in the RGB-565 format. The data can be provided as an array in a source or a header file. To convert an image file to the appropriate format, a special utility must be utilized. One such tool is provided by Henning Karlsen, the author of the UTFT display liberary and can be downloaded for free from his web site: http://henningkarlsen.com/electronics/library.php?id=52
- Parameters:
-
x Horizontal offset of the first pixel of the image. y Vertical offset of the first pixel of the image sx Width of the image. sy Height of the image. data Image pixel array. deg Angle to rotate the image before painting on screen, in degrees. rox roy
Definition at line 452 of file ssd1289.cpp.
void DrawCircle | ( | unsigned short | x, |
unsigned short | y, | ||
unsigned short | radius, | ||
int | color = -2 |
||
) |
Paints a circle.
- Parameters:
-
x The offset of the circle's center from the left edge of the screen. y The offset of the circle's center from the top edge of the screen. radius The circle's radius. color The color to use for painting. -1 indicated background, -2 foreground, or custom color in RGB-565 format.
Definition at line 298 of file ssd1289.cpp.
void DrawLine | ( | unsigned short | x1, |
unsigned short | y1, | ||
unsigned short | x2, | ||
unsigned short | y2, | ||
int | color = -2 |
||
) |
Draws a line.
- Parameters:
-
x1 Horizontal offset of the beginning point of the line. y1 Vertical offset of the beginning point of the line. x2 Horizontal offset of the end point of the line. y2 Verical offset of the end point of the line. color The color to use for painting, in RGB-565 format, or -1 for background, or -2 for foreground.
Definition at line 132 of file ssd1289.cpp.
void DrawPixel | ( | unsigned short | x, |
unsigned short | y, | ||
int | color = -2 |
||
) |
Draws a pixel at the specified location.
By default the function will use the preset foreground color, but the background or a custom color could be used as well.
- Parameters:
-
x The horizontal offset of the pixel from the upper left corner of the screen. y The vertical offset of the pixel from the upper left corner of the screen. color The color to be used. Must be in RGB-565 format, or -1 for background and -2 for foreground color.
Definition at line 122 of file ssd1289.cpp.
void DrawRect | ( | unsigned short | x1, |
unsigned short | y1, | ||
unsigned short | x2, | ||
unsigned short | y2, | ||
int | color = -2 |
||
) |
Paints a rectangle.
- Parameters:
-
x1 The horizontal offset of the beginning point of one of the rectangle's diagonals. y1 The vertical offset of the beginning point of one of the rectangle's diagonals. x2 The horizontal offset of the end point of the same of the rectangle's diagonals. y2 The vertical offset of the end point of the same of the rectangle's diagonals. color The color to use for painting. -1 indicated background, -2 foreground, or custom color in RGB-565 format.
Definition at line 216 of file ssd1289.cpp.
void DrawRoundRect | ( | unsigned short | x1, |
unsigned short | y1, | ||
unsigned short | x2, | ||
unsigned short | y2, | ||
int | color = -2 |
||
) |
Paints a rectangle and fills it with the paint color.
- Parameters:
-
x1 The horizontal offset of the beginning point of one of the rectangle's diagonals. y1 The vertical offset of the beginning point of one of the rectangle's diagonals. x2 The horizontal offset of the end point of the same of the rectangle's diagonals. y2 The vertical offset of the end point of the same of the rectangle's diagonals. color The color to use for painting. -1 indicated background, -2 foreground, or custom color in RGB-565 format.
Definition at line 227 of file ssd1289.cpp.
void FillCircle | ( | unsigned short | x, |
unsigned short | y, | ||
unsigned short | radius, | ||
int | color = -2 |
||
) |
Paints a circle and fills it with the paint color.
- Parameters:
-
x The offset of the circle's center from the left edge of the screen. y The offset of the circle's center from the top edge of the screen. radius The circle's radius. color The color to use for painting. -1 indicated background, -2 foreground, or custom color in RGB-565 format.
Definition at line 349 of file ssd1289.cpp.
void FillRect | ( | unsigned short | x1, |
unsigned short | y1, | ||
unsigned short | x2, | ||
unsigned short | y2, | ||
int | color = -2 |
||
) |
Paints a rectangle with rounded corners.
- Parameters:
-
x1 The horizontal offset of the beginning point of one of the rectangle's diagonals. y1 The vertical offset of the beginning point of one of the rectangle's diagonals. x2 The horizontal offset of the end point of the same of the rectangle's diagonals. y2 The vertical offset of the end point of the same of the rectangle's diagonals. color The color to use for painting. -1 indicated background, -2 foreground, or custom color in RGB-565 format.
Definition at line 245 of file ssd1289.cpp.
void FillRoundRect | ( | unsigned short | x1, |
unsigned short | y1, | ||
unsigned short | x2, | ||
unsigned short | y2, | ||
int | color = -2 |
||
) |
Paints a rectangle with rounded corners and fills it with the paint color.
- Parameters:
-
x1 The horizontal offset of the beginning point of one of the rectangle's diagonals. y1 The vertical offset of the beginning point of one of the rectangle's diagonals. x2 The horizontal offset of the end point of the same of the rectangle's diagonals. y2 The vertical offset of the end point of the same of the rectangle's diagonals. color The color to use for painting. -1 indicated background, -2 foreground, or custom color in RGB-565 format.
Definition at line 268 of file ssd1289.cpp.
void FillScreen | ( | int | color = -1 ) |
Fills the whole screen with a single color.
- Parameters:
-
color The color to be used. The value must be in RGB-565 format.
- Remarks:
- The special values -1 and -2 signify the preset background and foreground colors, respectively. The backround color is the default.
Definition at line 106 of file ssd1289.cpp.
void Initialize | ( | orientation_t | orientation = LANDSCAPE ) |
Initialize display.
Wakes up the display from sleep, initializes power parameters. This function must be called first, befor any painting on the display is done, otherwise the positioning of graphical elements will not work properly and any paynt operation will not be visible or produce garbage.
- Parameters:
-
oritentation The display orientation, landscape is default.
Definition at line 27 of file ssd1289.cpp.
void Print | ( | const char * | str, |
unsigned short | x, | ||
unsigned short | y, | ||
int | fgColor = -2 , |
||
int | bgColor = -1 , |
||
unsigned short | deg = 0 |
||
) |
Print a text on the screen.
- Parameters:
-
str The text. x The horizontal offset form the left edge of the screen. The special values LEFT, CENTER, or RIGHT can be used instead of pixel offset to indicate the text's horizontal alignment. y The vertical offset of the text from the top of the screen. fgColor The foreground to use for painting the text; -1 indicates background color, -2 the foreground setting, or custom color. bgColor The color to use for painting the empty pixels; -1 indicates the background color, -2 the foreground setting, or custom color. deg If different than 0, the text will be rotated at an angle this many degrees around its starting point. Default is not to ratate.
Definition at line 364 of file ssd1289.cpp.
void SetBackground | ( | unsigned short | color = COLOR_BLACK ) |
Set the background color for painting.
This is the default color to be used for "empty" pixels while painting. If a particular function allows for a different value to be specified when the function is called, the new value will be used only for this single call and will not change this setting.
- Parameters:
-
color The background color as RGB-565 value.
- See also:
- RGB(r,g,b)
Definition at line 92 of file ssd1289.cpp.
void SetFont | ( | const char * | font ) |
Sets the font to be used for painting of text on the screen.
- Parameters:
-
font A pointer to the font data.
- See also:
- Comments in file fonts.h
Definition at line 97 of file ssd1289.cpp.
void SetForeground | ( | unsigned short | color = COLOR_WHITE ) |
Set the foreground color for painting.
This is the default foreground color to be used in painting operations. If a specific output function allows for a different color to be specified in place, the new setting will be used for that single operation only and will not change this value.
- Parameters:
-
color The color to be used. The value must be in RGB-565 format.
- See also:
- RGB(r,g,b)
Definition at line 87 of file ssd1289.cpp.
Generated on Wed Jul 13 2022 06:38:58 by
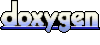