Fork of SSD1289 lib for Landtiger board
Embed:
(wiki syntax)
Show/hide line numbers
ssd1289.h
Go to the documentation of this file.
00001 /** \file ssd1289.h 00002 * \brief mbed TFT LCD library for displays with the SSD1289 LCD controller. 00003 * 00004 * A known display with this type of controller chip is the ITDB02-3.2S from 00005 * http://imall.iteadstudio.com 00006 * 00007 * This library is based on the Arduino/chipKIT UTFT library by Henning 00008 * Karlsen, http://henningkarlsen.com/electronics/library.php?id=52 00009 * 00010 * Copyright (C)2010-2012 Henning Karlsen. All right reserved. 00011 * Copyright (C)2012 Todor Todorov. 00012 * 00013 * This library is free software; you can redistribute it and/or 00014 * modify it under the terms of the GNU Lesser General Public 00015 * License as published by the Free Software Foundation; either 00016 * version 2.1 of the License, or (at your option) any later version. 00017 * 00018 * This library is distributed in the hope that it will be useful, 00019 * but WITHOUT ANY WARRANTY; without even the implied warranty of 00020 * MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the GNU 00021 * Lesser General Public License for more details. 00022 * 00023 * You should have received a copy of the GNU Lesser General Public 00024 * License along with this library; if not, write to: 00025 * 00026 * Free Software Foundation, Inc. 00027 * 51 Franklin St, 5th Floor, Boston, MA 02110-1301, USA 00028 * 00029 *********************************************************************/ 00030 00031 #ifndef SSD1289_H 00032 #define SSD1289_H 00033 00034 #include "mbed.h" 00035 #include "fonts.h" 00036 00037 /** \def RGB(r,g,b) 00038 * \brief Creates a RGB-565 color value. 00039 * 00040 * Displays which use 16 bits to assign colors to a specific pixel, use 00041 * 5 bits for the red component, 6 bits for the green component and 5 00042 * bits for the blue component. This macro converts the 3 full-size 00043 * RGB bytes into one 16-bit RGB-565 value. 00044 */ 00045 #define RGB( r, g, b ) ( ( ( ( r ) & 248 ) | ( ( g ) >> 5 ) ) << 8 ) | ( ( ( ( g ) & 28 ) << 3 ) | ( ( b ) >> 3 ) ) 00046 /** \def COLOR_BLACK 00047 * \brief Shorthand for RGB( 0, 0, 0 ). 00048 */ 00049 #define COLOR_BLACK RGB( 0x00, 0x00, 0x00 ) 00050 /** \def COLOR_WHITE 00051 * \brief Shorthand for RGB( 255, 255, 255 ). 00052 */ 00053 #define COLOR_WHITE RGB( 0xFF, 0xFF, 0xFF ) 00054 /** \def COLOR_RED 00055 * \brief Shorthand for RGB( 255, 0, 0 ). 00056 */ 00057 #define COLOR_RED RGB( 0xFF, 0x00, 0x00 ) 00058 /** \def COLOR_GREEN 00059 * \brief Shorthand for RGB( 255, 255, 255 ). 00060 */ 00061 #define COLOR_GREEN RGB( 0x00, 0xFF, 0x00 ) 00062 /** \def COLOR_BLIUE 00063 * \brief Shorthand for RGB( 0, 255, 0 ). 00064 */ 00065 #define COLOR_BLIUE RGB( 0x00, 0x00, 0xFF ) 00066 00067 /** \typedef orientation_t 00068 * \brief Display orientation. 00069 */ 00070 typedef enum Orientation_enum 00071 { 00072 PORTRAIT = 0, /**< Display height is bigger than its width. */ 00073 LANDSCAPE = 1, /**< Display width is bigger than its height. */ 00074 } orientation_t; 00075 00076 /** \typedef align_t 00077 * \brief Horizontal text alignment on the line. 00078 */ 00079 typedef enum Alignment_enum 00080 { 00081 LEFT = 0, /**< Left-oriented, naturally gravitate closer to the left edge of the screen. */ 00082 CENTER = 9998, /**< Center-oriented, try to fit in the middle of the available space with equal free space to the left and right of the text. */ 00083 RIGHT = 9999, /**< Right-oriented, naturally gravitate closer to the right edge of the screen, leaving any remaining free space to the left of the text. */ 00084 } align_t; 00085 00086 /** \typedef font_metrics_t 00087 * \brief Describes fonts and their properties. 00088 * \sa Comments in fonts.h 00089 */ 00090 typedef struct Font_struct 00091 { 00092 const char* font; /**< A pointer to the first byte in the font. */ 00093 unsigned char width; /**< The width of each character, in pixels. */ 00094 unsigned char height; /**< Height of each character, in pixels. */ 00095 unsigned char offset; /**< Offset of the first character in the font. */ 00096 unsigned char numchars; /**< Count of the available characters in the font. */ 00097 } font_metrics_t; 00098 00099 /** \typedef bitmap_t 00100 * \brief Pointer to the start of a block of pixel data, describing a picture. 00101 */ 00102 typedef unsigned short* bitmap_t; 00103 00104 /** Represents a LCD instance. 00105 * 00106 * This is the utility class, through which the display can be manipulated 00107 * and graphics objects can be shown to the user. A known display, which 00108 * works with this library is the ITDB02-3.2S from iTeadStudio - a RGB TFT 00109 * with 240x320 pixels resolution and 65K colors, using 16-bit interface. 00110 * 00111 * The display needs 20 or 21 pins to work with mbed, so it is possibly not 00112 * the best of choices out there, but other than that it uses +3.3V for 00113 * power and logic, as well as the backlight, thus can be interfaced directly 00114 * to the mbed without the need of shields or level shifters as with Arduino. 00115 * 00116 * How to use: 00117 * \code 00118 * // include the library, this will also pull in the header for the provided fonts 00119 * #include "ssd1289.h" 00120 * 00121 * // prepare the data bus for writing commands and pixel data 00122 * BusOut dataBus( p30, p29, p28, p27, p26, p25, p24, p23, p22, p21, p20, p19, p18, p17, p16, p15 ); // 16 pins 00123 * // create the lcd instance 00124 * SSD1289 lcd( p14, p13, p12, p11, &dataBus ); // control pins and data bus 00125 * 00126 * int main() 00127 * { 00128 * // initialize display - place it in standard portrait mode and set background to black and 00129 * // foreground to white color. 00130 * lcd.Initialize(); 00131 * // set current font to the smallest 8x12 pixels font. 00132 * lcd.SetFont( Font8x12 ); 00133 * // print something on the screen 00134 * lcd.Print( "Hello, World!", CENTER, 25 ); // align text to center horizontally and use starndard colors 00135 * 00136 * while ( 1 ) { } 00137 * } 00138 * 00139 * \endcode 00140 */ 00141 class SSD1289 00142 { 00143 public: 00144 /** Creates a new instance of the class. 00145 * 00146 * \param CS_PIN Pin for the ChipSelect signal. 00147 * \param RESET_PIN Pin for the RESET line. 00148 * \param RS_PIN Pin for the RS signal. 00149 * \param WR_PIN Pin for the WR signal. 00150 * \param DATA_PORT Address of the data bus for transfer of commands and pixel data. 00151 * \param RD_PIN Pin for the RD signal. This line is not needed by the driver, so if you would like to 00152 * use the pin on the mbed for something else, just pull-up the respective pin on the LCD high, 00153 * and do not assign a value to this parameter when creating the controller instance. 00154 */ 00155 SSD1289( PinName CS_PIN, PinName RESET_PIN, PinName RS_PIN, PinName WR_PIN, BusOut* DATA_PORT, PinName RD_PIN = NC ); 00156 00157 /** Initialize display. 00158 * 00159 * Wakes up the display from sleep, initializes power parameters. 00160 * This function must be called first, befor any painting on the 00161 * display is done, otherwise the positioning of graphical elements 00162 * will not work properly and any paynt operation will not be visible 00163 * or produce garbage. 00164 * 00165 * \param oritentation The display orientation, landscape is default. 00166 */ 00167 void Initialize( orientation_t orientation = LANDSCAPE ); 00168 00169 /** Set the foreground color for painting. 00170 * 00171 * This is the default foreground color to be used in painting operations. 00172 * If a specific output function allows for a different color to be specified 00173 * in place, the new setting will be used for that single operation only and 00174 * will not change this value. 00175 * 00176 * \param color The color to be used. The value must be in RGB-565 format. 00177 * \sa #RGB(r,g,b) 00178 */ 00179 void SetForeground( unsigned short color = COLOR_WHITE ); 00180 00181 /** Set the background color for painting. 00182 * 00183 * This is the default color to be used for "empty" pixels while painting. 00184 * If a particular function allows for a different value to be specified 00185 * when the function is called, the new value will be used only for this 00186 * single call and will not change this setting. 00187 * 00188 * \param color The background color as RGB-565 value. 00189 * \sa #RGB(r,g,b) 00190 */ 00191 void SetBackground( unsigned short color = COLOR_BLACK ); 00192 00193 /** Sets the font to be used for painting of text on the screen. 00194 * \param font A pointer to the font data. 00195 * \sa Comments in file fonts.h 00196 */ 00197 void SetFont( const char* font ); 00198 00199 /** Fills the whole screen with a single color. 00200 * \param color The color to be used. The value must be in RGB-565 format. 00201 * \remarks The special values -1 and -2 signify the preset background and foreground colors, respectively. 00202 * The backround color is the default. 00203 */ 00204 void FillScreen( int color = -1 ); 00205 00206 /** Clears the screen. 00207 * 00208 * This is the same as calling #FillScreen() or #FillScreen( -1 ) to use the background color. 00209 */ 00210 void ClearScreen( void ); 00211 00212 /** Draws a pixel at the specified location. 00213 * 00214 * By default the function will use the preset foreground color, but the background 00215 * or a custom color could be used as well. 00216 * 00217 * \param x The horizontal offset of the pixel from the upper left corner of the screen. 00218 * \param y The vertical offset of the pixel from the upper left corner of the screen. 00219 * \param color The color to be used. Must be in RGB-565 format, or -1 for background and -2 for foreground color. 00220 */ 00221 void DrawPixel( unsigned short x, unsigned short y, int color = -2 ); 00222 00223 /** Draws a line. 00224 * 00225 * \param x1 Horizontal offset of the beginning point of the line. 00226 * \param y1 Vertical offset of the beginning point of the line. 00227 * \param x2 Horizontal offset of the end point of the line. 00228 * \param y2 Verical offset of the end point of the line. 00229 * \param color The color to use for painting, in RGB-565 format, or -1 for background, or -2 for foreground. 00230 */ 00231 void DrawLine( unsigned short x1, unsigned short y1, unsigned short x2, unsigned short y2, int color = -2 ); 00232 00233 /** Paints a rectangle. 00234 * 00235 * \param x1 The horizontal offset of the beginning point of one of the rectangle's diagonals. 00236 * \param y1 The vertical offset of the beginning point of one of the rectangle's diagonals. 00237 * \param x2 The horizontal offset of the end point of the same of the rectangle's diagonals. 00238 * \param y2 The vertical offset of the end point of the same of the rectangle's diagonals. 00239 * \param color The color to use for painting. -1 indicated background, -2 foreground, or custom color in RGB-565 format. 00240 */ 00241 void DrawRect( unsigned short x1, unsigned short y1, unsigned short x2, unsigned short y2, int color = -2 ); 00242 00243 /** Paints a rectangle and fills it with the paint color. 00244 * 00245 * \param x1 The horizontal offset of the beginning point of one of the rectangle's diagonals. 00246 * \param y1 The vertical offset of the beginning point of one of the rectangle's diagonals. 00247 * \param x2 The horizontal offset of the end point of the same of the rectangle's diagonals. 00248 * \param y2 The vertical offset of the end point of the same of the rectangle's diagonals. 00249 * \param color The color to use for painting. -1 indicated background, -2 foreground, or custom color in RGB-565 format. 00250 */ 00251 void DrawRoundRect( unsigned short x1, unsigned short y1, unsigned short x2, unsigned short y2, int color = -2 ); 00252 00253 /** Paints a rectangle with rounded corners. 00254 * 00255 * \param x1 The horizontal offset of the beginning point of one of the rectangle's diagonals. 00256 * \param y1 The vertical offset of the beginning point of one of the rectangle's diagonals. 00257 * \param x2 The horizontal offset of the end point of the same of the rectangle's diagonals. 00258 * \param y2 The vertical offset of the end point of the same of the rectangle's diagonals. 00259 * \param color The color to use for painting. -1 indicated background, -2 foreground, or custom color in RGB-565 format. 00260 */ 00261 void FillRect( unsigned short x1, unsigned short y1, unsigned short x2, unsigned short y2, int color = -2 ); 00262 00263 /** Paints a rectangle with rounded corners and fills it with the paint color. 00264 * 00265 * \param x1 The horizontal offset of the beginning point of one of the rectangle's diagonals. 00266 * \param y1 The vertical offset of the beginning point of one of the rectangle's diagonals. 00267 * \param x2 The horizontal offset of the end point of the same of the rectangle's diagonals. 00268 * \param y2 The vertical offset of the end point of the same of the rectangle's diagonals. 00269 * \param color The color to use for painting. -1 indicated background, -2 foreground, or custom color in RGB-565 format. 00270 */ 00271 void FillRoundRect( unsigned short x1, unsigned short y1, unsigned short x2, unsigned short y2, int color = -2 ); 00272 00273 /** Paints a circle. 00274 * 00275 * \param x The offset of the circle's center from the left edge of the screen. 00276 * \param y The offset of the circle's center from the top edge of the screen. 00277 * \param radius The circle's radius. 00278 * \param color The color to use for painting. -1 indicated background, -2 foreground, or custom color in RGB-565 format. 00279 */ 00280 void DrawCircle( unsigned short x, unsigned short y, unsigned short radius, int color = -2 ); 00281 00282 /** Paints a circle and fills it with the paint color. 00283 * 00284 * \param x The offset of the circle's center from the left edge of the screen. 00285 * \param y The offset of the circle's center from the top edge of the screen. 00286 * \param radius The circle's radius. 00287 * \param color The color to use for painting. -1 indicated background, -2 foreground, or custom color in RGB-565 format. 00288 */ 00289 void FillCircle( unsigned short x, unsigned short y, unsigned short radius, int color = -2 ); 00290 00291 /** Print a text on the screen. 00292 * 00293 * \param str The text. 00294 * \param x The horizontal offset form the left edge of the screen. The special values LEFT, CENTER, 00295 * or RIGHT can be used instead of pixel offset to indicate the text's horizontal alignment. 00296 * \param y The vertical offset of the text from the top of the screen. 00297 * \param fgColor The foreground to use for painting the text; -1 indicates background color, -2 the foreground setting, or custom color. 00298 * \param bgColor The color to use for painting the empty pixels; -1 indicates the background color, -2 the foreground setting, or custom color. 00299 * \param deg If different than 0, the text will be rotated at an angle this many degrees around its starting point. Default is not to ratate. 00300 */ 00301 void Print( const char *str, unsigned short x, unsigned short y, int fgColor = -2, int bgColor = -1, unsigned short deg = 0 ); 00302 00303 /** Draw an image on the screen. 00304 * 00305 * The pixels of the picture must be in the RGB-565 format. The data can be provided 00306 * as an array in a source or a header file. To convert an image file to the appropriate 00307 * format, a special utility must be utilized. One such tool is provided by Henning Karlsen, 00308 * the author of the UTFT display liberary and can be downloaded for free from his web site: 00309 * http://henningkarlsen.com/electronics/library.php?id=52 00310 * 00311 * \param x Horizontal offset of the first pixel of the image. 00312 * \param y Vertical offset of the first pixel of the image 00313 * \param sx Width of the image. 00314 * \param sy Height of the image. 00315 * \param data Image pixel array. 00316 * \param scale A value of 1 will produce an image with its original size, while a different value will scale the image. 00317 */ 00318 void DrawBitmap( unsigned short x, unsigned short y, unsigned short sx, unsigned short sy, bitmap_t data, unsigned char scale = 1 ); 00319 00320 /** Draw an image on the screen. 00321 * 00322 * The pixels of the picture must be in the RGB-565 format. The data can be provided 00323 * as an array in a source or a header file. To convert an image file to the appropriate 00324 * format, a special utility must be utilized. One such tool is provided by Henning Karlsen, 00325 * the author of the UTFT display liberary and can be downloaded for free from his web site: 00326 * http://henningkarlsen.com/electronics/library.php?id=52 00327 * 00328 * \param x Horizontal offset of the first pixel of the image. 00329 * \param y Vertical offset of the first pixel of the image 00330 * \param sx Width of the image. 00331 * \param sy Height of the image. 00332 * \param data Image pixel array. 00333 * \param deg Angle to rotate the image before painting on screen, in degrees. 00334 * \param rox 00335 * \param roy 00336 */ 00337 void DrawBitmap( unsigned short x, unsigned short y, unsigned short sx, unsigned short sy, bitmap_t data, unsigned short deg, unsigned short rox, unsigned short roy ); 00338 00339 private: 00340 DigitalOut _lcd_pin_cs, _lcd_pin_reset, _lcd_pin_rs, _lcd_pin_wr; 00341 BusOut* _lcd_port; 00342 DigitalOut* _lcd_pin_rd; 00343 orientation_t _orientation; 00344 static const long _disp_width = 239; 00345 static const long _disp_height = 319; 00346 unsigned short _foreground, _background; 00347 font_metrics_t _font; 00348 00349 private: 00350 virtual void writeCmd( unsigned short cmd ); 00351 virtual void writeData( unsigned short data ); 00352 virtual void writeCmdData( unsigned short cmd, unsigned short data ); 00353 00354 void setXY( uint16_t x1, uint16_t y1, uint16_t x2, uint16_t y2 ); 00355 void clearXY( void ); 00356 void drawHLine( unsigned short x, unsigned short y, unsigned short len, int color = -2 ); 00357 void drawVLine( unsigned short x, unsigned short y, unsigned short len, int color = -2 ); 00358 00359 void printChar( char c, unsigned short x, unsigned short y, int fgColor = -2, int bgColor = -1 ); 00360 void rotateChar( char c, unsigned short x, unsigned short y, int pos, int fgColor = -2, int bgColor = -1, unsigned short deg = 0 ); 00361 }; 00362 00363 #endif /* SSD1289_H */
Generated on Wed Jul 13 2022 06:38:58 by
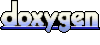