Fork of SSD1289 lib for Landtiger board
Embed:
(wiki syntax)
Show/hide line numbers
ssd1289.cpp
00001 #include "ssd1289.h" 00002 00003 #define LOW 0 00004 #define HIGH 1 00005 #define pulseLow( pin ) pin = LOW; pin = HIGH 00006 #define pulseHigh( pin ) pin = HIGH; pin = LOW 00007 #define swap( type, a, b ) { type tmp = ( a ); ( a ) = ( b ); ( b ) = tmp; } 00008 00009 #ifndef ushort 00010 typedef unsigned short ushort; 00011 #endif 00012 00013 SSD1289::SSD1289( PinName CS_PIN, PinName RESET_PIN, PinName RS_PIN, PinName WR_PIN, BusOut* DATA_PORT, PinName RD_PIN ) 00014 : _lcd_pin_cs( CS_PIN ), _lcd_pin_reset( RESET_PIN ), _lcd_pin_rs( RS_PIN ), _lcd_pin_wr( WR_PIN ) 00015 { 00016 _lcd_port = DATA_PORT; 00017 if ( RD_PIN != NC ) 00018 _lcd_pin_rd = new DigitalOut( RD_PIN ); 00019 else 00020 _lcd_pin_rd = 0; 00021 00022 SetForeground(); 00023 SetBackground(); 00024 _font.font = 0; 00025 } 00026 00027 void SSD1289::Initialize( orientation_t orientation ) 00028 { 00029 _orientation = orientation; 00030 00031 _lcd_pin_reset = HIGH; 00032 wait_ms( 5 ); 00033 _lcd_pin_reset = LOW; 00034 wait_ms( 15 ); 00035 _lcd_pin_reset = HIGH; 00036 _lcd_pin_cs = HIGH; 00037 if ( _lcd_pin_rd != 0 ) 00038 *_lcd_pin_rd = HIGH; 00039 _lcd_pin_wr = HIGH; 00040 wait_ms( 15 ); 00041 00042 00043 writeCmdData( 0x00, 0x0001 ); wait_ms( 1 ); 00044 writeCmdData( 0x03, 0xA8A4 ); wait_ms( 1 ); 00045 writeCmdData( 0x0C, 0x0000 ); wait_ms( 1 ); 00046 writeCmdData( 0x0D, 0x080C ); wait_ms( 1 ); 00047 writeCmdData( 0x0E, 0x2B00 ); wait_ms( 1 ); 00048 writeCmdData( 0x1E, 0x00B7 ); wait_ms( 1 ); 00049 writeCmdData( 0x01, 0x2B3F ); wait_ms( 1 ); 00050 writeCmdData( 0x02, 0x0600 ); wait_ms( 1 ); 00051 writeCmdData( 0x10, 0x0000 ); wait_ms( 1 ); 00052 writeCmdData( 0x11, 0x6070 ); wait_ms( 1 ); 00053 writeCmdData( 0x05, 0x0000 ); wait_ms( 1 ); 00054 writeCmdData( 0x06, 0x0000 ); wait_ms( 1 ); 00055 writeCmdData( 0x16, 0xEF1C ); wait_ms( 1 ); 00056 writeCmdData( 0x17, 0x0003 ); wait_ms( 1 ); 00057 writeCmdData( 0x07, 0x0233 ); wait_ms( 1 ); 00058 writeCmdData( 0x0B, 0x0000 ); wait_ms( 1 ); 00059 writeCmdData( 0x0F, 0x0000 ); wait_ms( 1 ); 00060 writeCmdData( 0x41, 0x0000 ); wait_ms( 1 ); 00061 writeCmdData( 0x42, 0x0000 ); wait_ms( 1 ); 00062 writeCmdData( 0x48, 0x0000 ); wait_ms( 1 ); 00063 writeCmdData( 0x49, 0x013F ); wait_ms( 1 ); 00064 writeCmdData( 0x4A, 0x0000 ); wait_ms( 1 ); 00065 writeCmdData( 0x4B, 0x0000 ); wait_ms( 1 ); 00066 writeCmdData( 0x44, 0xEF00 ); wait_ms( 1 ); 00067 writeCmdData( 0x45, 0x0000 ); wait_ms( 1 ); 00068 writeCmdData( 0x46, 0x013F ); wait_ms( 1 ); 00069 writeCmdData( 0x30, 0x0707 ); wait_ms( 1 ); 00070 writeCmdData( 0x31, 0x0204 ); wait_ms( 1 ); 00071 writeCmdData( 0x32, 0x0204 ); wait_ms( 1 ); 00072 writeCmdData( 0x33, 0x0502 ); wait_ms( 1 ); 00073 writeCmdData( 0x34, 0x0507 ); wait_ms( 1 ); 00074 writeCmdData( 0x35, 0x0204 ); wait_ms( 1 ); 00075 writeCmdData( 0x36, 0x0204 ); wait_ms( 1 ); 00076 writeCmdData( 0x37, 0x0502 ); wait_ms( 1 ); 00077 writeCmdData( 0x3A, 0x0302 ); wait_ms( 1 ); 00078 writeCmdData( 0x3B, 0x0302 ); wait_ms( 1 ); 00079 writeCmdData( 0x23, 0x0000 ); wait_ms( 1 ); 00080 writeCmdData( 0x24, 0x0000 ); wait_ms( 1 ); 00081 writeCmdData( 0x25, 0x8000 ); wait_ms( 1 ); 00082 writeCmdData( 0x4f, 0x0000 ); wait_ms( 1 ); 00083 writeCmdData( 0x4e, 0x0000 ); wait_ms( 1 ); 00084 writeCmd( 0x22 ); 00085 } 00086 00087 void SSD1289::SetForeground( unsigned short color ) 00088 { 00089 _foreground = color; 00090 } 00091 00092 void SSD1289::SetBackground( unsigned short color ) 00093 { 00094 _background = color; 00095 } 00096 00097 void SSD1289::SetFont( const char *font ) 00098 { 00099 _font.font = font; 00100 _font.width = font[ 0 ]; 00101 _font.height = font[ 1 ]; 00102 _font.offset = font[ 2 ]; 00103 _font.numchars = font[ 3 ]; 00104 } 00105 00106 void SSD1289::FillScreen( int color ) 00107 { 00108 unsigned short rgb = color == -1 ? _background : color == -2 ? _foreground : ( unsigned short ) color; 00109 _lcd_pin_cs = LOW; 00110 clearXY(); 00111 _lcd_pin_rs = HIGH; 00112 for ( int i = 0; i < ( ( _disp_width + 1 ) * ( _disp_height + 1 ) ); i++ ) 00113 writeData( rgb ); 00114 _lcd_pin_cs = HIGH; 00115 } 00116 00117 void SSD1289::ClearScreen( void ) 00118 { 00119 FillScreen( -1 ); 00120 } 00121 00122 void SSD1289::DrawPixel( unsigned short x, unsigned short y, int color ) 00123 { 00124 _lcd_pin_cs = LOW; 00125 setXY( x, y, x, y ); 00126 writeData( color == -1 ? _background : 00127 color == -2 ? _foreground : color ); 00128 _lcd_pin_cs = HIGH; 00129 clearXY(); 00130 } 00131 00132 void SSD1289::DrawLine( unsigned short x1, unsigned short y1, unsigned short x2, unsigned short y2, int color ) 00133 { 00134 00135 double delta, tx, ty; 00136 00137 if ( ( ( x2 - x1 ) < 0 ) ) 00138 { 00139 swap( ushort, x1, x2 ) 00140 swap( ushort, y1, y2 ) 00141 } 00142 if ( ( ( y2 - y1 ) < 0 ) ) 00143 { 00144 swap( ushort, x1, x2 ) 00145 swap( ushort, y1, y2 ) 00146 } 00147 00148 if ( y1 == y2 ) 00149 { 00150 if ( x1 > x2 ) 00151 swap( ushort, x1, x2 ) 00152 drawHLine( x1, y1, x2 - x1, color ); 00153 } 00154 else if ( x1 == x2 ) 00155 { 00156 if ( y1 > y2 ) 00157 swap( ushort, y1, y2 ) 00158 drawVLine( x1, y1, y2 - y1, color ); 00159 } 00160 else if ( abs( x2 - x1 ) > abs( y2 - y1 ) ) 00161 { 00162 unsigned short usedColor = color == -1 ? _background : color == -2 ? _foreground : ( unsigned short ) color; 00163 _lcd_pin_cs = LOW; 00164 delta = ( double( y2 - y1 ) / double( x2 - x1 ) ); 00165 ty = double( y1 ); 00166 if ( x1 > x2 ) 00167 { 00168 for ( int i = x1; i >= x2; i-- ) 00169 { 00170 setXY( i, int( ty + 0.5 ), i, int( ty + 0.5 ) ); 00171 writeData( usedColor ); 00172 ty = ty - delta; 00173 } 00174 } 00175 else 00176 { 00177 for ( int i = x1; i <= x2; i++ ) 00178 { 00179 setXY( i, int( ty + 0.5 ), i, int( ty + 0.5 ) ); 00180 writeData( usedColor ); 00181 ty = ty + delta; 00182 } 00183 } 00184 _lcd_pin_cs = HIGH; 00185 } 00186 else 00187 { 00188 unsigned short usedColor = color == -1 ? _background : color == -2 ? _foreground : ( unsigned short ) color; 00189 _lcd_pin_cs = LOW; 00190 delta = ( float( x2 - x1 ) / float( y2 - y1 ) ); 00191 tx = float( x1 ); 00192 if ( y1 > y2 ) 00193 { 00194 for ( int i = y2 + 1; i > y1; i-- ) 00195 { 00196 setXY( int( tx + 0.5 ), i, int( tx + 0.5 ), i ); 00197 writeData( usedColor ); 00198 tx = tx + delta; 00199 } 00200 } 00201 else 00202 { 00203 for ( int i = y1; i < y2 + 1; i++ ) 00204 { 00205 setXY( int( tx + 0.5 ), i, int( tx + 0.5 ), i ); 00206 writeData( usedColor ); 00207 tx = tx + delta; 00208 } 00209 } 00210 _lcd_pin_cs = HIGH; 00211 } 00212 00213 clearXY(); 00214 } 00215 00216 void SSD1289::DrawRect( unsigned short x1, unsigned short y1, unsigned short x2, unsigned short y2, int color ) 00217 { 00218 if ( x1 > x2 ) swap( ushort, x1, x2 ) 00219 if ( y1 > y2 ) swap( ushort, y1, y2 ) 00220 00221 drawHLine( x1, y1, x2 - x1, color ); 00222 drawHLine( x1, y2, x2 - x1, color ); 00223 drawVLine( x1, y1, y2 - y1, color ); 00224 drawVLine( x2, y1, y2 - y1, color ); 00225 } 00226 00227 void SSD1289::DrawRoundRect( unsigned short x1, unsigned short y1, unsigned short x2, unsigned short y2, int color ) 00228 { 00229 if ( x1 > x2 ) swap( ushort, x1, x2 ) 00230 if ( y1 > y2 ) swap( ushort, y1, y2 ) 00231 00232 if ( ( x2 - x1 ) > 4 && ( y2 - y1 ) > 4 ) 00233 { 00234 DrawPixel( x1 + 1, y1 + 1, color ); 00235 DrawPixel( x2 - 1, y1 + 1, color ); 00236 DrawPixel( x1 + 1, y2 - 1, color ); 00237 DrawPixel( x2 - 1, y2 - 1, color ); 00238 drawHLine( x1 + 2, y1, x2 - x1 - 4, color ); 00239 drawHLine( x1 + 2, y2, x2 - x1 - 4, color ); 00240 drawVLine( x1, y1 + 2, y2 - y1 - 4, color ); 00241 drawVLine( x2, y1 + 2, y2 - y1 - 4, color ); 00242 } 00243 } 00244 00245 void SSD1289::FillRect( unsigned short x1, unsigned short y1, unsigned short x2, unsigned short y2, int color ) 00246 { 00247 if ( x1 > x2 ) swap( ushort, x1, x2 ); 00248 if ( y1 > y2 ) swap( ushort, y1, y2 ); 00249 00250 if ( _orientation == PORTRAIT ) 00251 { 00252 for ( int i = 0; i < ( ( y2 - y1 ) / 2 ) + 1; i++ ) 00253 { 00254 drawHLine( x1, y1 + i, x2 - x1, color ); 00255 drawHLine( x1, y2 - i, x2 - x1, color ); 00256 } 00257 } 00258 else 00259 { 00260 for ( int i = 0; i < ( ( x2 - x1 ) / 2 ) + 1; i++ ) 00261 { 00262 drawVLine( x1 + i, y1, y2 - y1, color ); 00263 drawVLine( x2 - i, y1, y2 - y1, color ); 00264 } 00265 } 00266 } 00267 00268 void SSD1289::FillRoundRect( unsigned short x1, unsigned short y1, unsigned short x2, unsigned short y2, int color ) 00269 { 00270 if ( x1 > x2 ) swap( ushort, x1, x2 ) 00271 if ( y1 > y2 ) swap( ushort, y1, y2 ) 00272 00273 if ( ( x2 - x1 ) > 4 && ( y2 - y1 ) > 4 ) 00274 { 00275 for ( int i = 0; i < ( ( y2 - y1 ) / 2 ) + 1; i++ ) 00276 { 00277 switch ( i ) 00278 { 00279 case 0: 00280 drawHLine( x1 + 2, y1 + i, x2 - x1 - 4, color ); 00281 drawHLine( x1 + 2, y2 - i, x2 - x1 - 4, color ); 00282 break; 00283 00284 case 1: 00285 drawHLine( x1 + 1, y1 + i, x2 - x1 - 2, color ); 00286 drawHLine( x1 + 1, y2 - i, x2 - x1 - 2, color ); 00287 break; 00288 00289 default: 00290 drawHLine( x1, y1 + i, x2 - x1, color ); 00291 drawHLine( x1, y2 - i, x2 - x1, color ); 00292 break; 00293 } 00294 } 00295 } 00296 } 00297 00298 void SSD1289::DrawCircle( unsigned short x, unsigned short y, unsigned short radius, int color ) 00299 { 00300 int f = 1 - radius; 00301 int ddF_x = 1; 00302 int ddF_y = -2 * radius; 00303 int x1 = 0; 00304 int y1 = radius; 00305 unsigned short usedColor = color == -1 ? _background : color == -2 ? _foreground : ( unsigned short ) color; 00306 00307 _lcd_pin_cs = LOW; 00308 setXY( x, y + radius, x, y + radius ); 00309 writeData( usedColor ); 00310 setXY( x, y - radius, x, y - radius ); 00311 writeData( usedColor ); 00312 setXY( x + radius, y, x + radius, y ); 00313 writeData( usedColor ); 00314 setXY( x - radius, y, x - radius, y ); 00315 writeData( usedColor ); 00316 00317 while ( x1 < y1 ) 00318 { 00319 if ( f >= 0 ) 00320 { 00321 y1--; 00322 ddF_y += 2; 00323 f += ddF_y; 00324 } 00325 x1++; 00326 ddF_x += 2; 00327 f += ddF_x; 00328 setXY( x + x1, y + y1, x + x1, y + y1 ); 00329 writeData( usedColor ); 00330 setXY( x - x1, y + y1, x - x1, y + y1 ); 00331 writeData( usedColor ); 00332 setXY( x + x1, y - y1, x + x1, y - y1 ); 00333 writeData( usedColor ); 00334 setXY( x - x1, y - y1, x - x1, y - y1 ); 00335 writeData( usedColor ); 00336 setXY( x + y1, y + x1, x + y1, y + x1 ); 00337 writeData( usedColor ); 00338 setXY( x - y1, y + x1, x - y1, y + x1 ); 00339 writeData( usedColor ); 00340 setXY( x + y1, y - x1, x + y1, y - x1 ); 00341 writeData( usedColor ); 00342 setXY( x - y1, y - x1, x - y1, y - x1 ); 00343 writeData( usedColor ); 00344 } 00345 _lcd_pin_cs = HIGH; 00346 clearXY(); 00347 } 00348 00349 void SSD1289::FillCircle( unsigned short x, unsigned short y, unsigned short radius, int color ) 00350 { 00351 unsigned short usedColor = color == -1 ? _background : color == -2 ? _foreground : ( unsigned short ) color; 00352 _lcd_pin_cs = LOW; 00353 for ( int y1 = -radius; y1 <= radius; y1++ ) 00354 for ( int x1 = -radius; x1 <= radius; x1++ ) 00355 if ( x1 * x1 + y1 * y1 <= radius * radius ) 00356 { 00357 setXY( x + x1, y + y1, x + x1, y + y1 ); 00358 writeData( usedColor ); 00359 } 00360 _lcd_pin_cs = HIGH; 00361 clearXY(); 00362 } 00363 00364 void SSD1289::Print( const char *str, unsigned short x, unsigned short y, int fgColor, int bgColor, unsigned short deg ) 00365 { 00366 int stl, i; 00367 00368 stl = strlen( str ); 00369 00370 if ( _orientation == PORTRAIT ) 00371 { 00372 if ( x == RIGHT ) 00373 x = ( _disp_width + 1 ) - ( stl * _font.width ); 00374 if ( x == CENTER ) 00375 x = ( ( _disp_width + 1 ) - ( stl * _font.width ) ) / 2; 00376 } 00377 else 00378 { 00379 if ( x == RIGHT ) 00380 x = ( _disp_height + 1 ) - ( stl * _font.width ); 00381 if ( x == CENTER ) 00382 x = ( ( _disp_height + 1 ) - ( stl * _font.width ) ) / 2; 00383 } 00384 00385 for ( i = 0; i < stl; i++ ) 00386 if ( deg == 0 ) 00387 printChar( *str++, x + ( i * ( _font.width ) ), y, fgColor, bgColor ); 00388 else 00389 rotateChar( *str++, x, y, i, fgColor, bgColor, deg ); 00390 } 00391 00392 void SSD1289::DrawBitmap( unsigned short x, unsigned short y, unsigned short sx, unsigned short sy, bitmap_t data, unsigned char scale ) 00393 { 00394 int tx, ty, tc, tsx, tsy; 00395 00396 if ( scale == 1 ) 00397 { 00398 if ( _orientation == PORTRAIT ) 00399 { 00400 _lcd_pin_cs = LOW; 00401 setXY( x, y, x + sx - 1, y + sy - 1 ); 00402 for ( tc = 0; tc < ( sx * sy ); tc++ ) 00403 writeData( data[ tc ] ); 00404 _lcd_pin_cs = HIGH; 00405 } 00406 else 00407 { 00408 _lcd_pin_cs = LOW; 00409 for ( ty = 0; ty < sy; ty++ ) 00410 { 00411 setXY( x, y + ty, x + sx - 1, y + ty ); 00412 for ( tx = sx; tx >= 0; tx-- ) 00413 writeData( data[ ( ty * sx ) + tx ] ); 00414 } 00415 _lcd_pin_cs = HIGH; 00416 } 00417 } 00418 else 00419 { 00420 if ( _orientation == PORTRAIT ) 00421 { 00422 _lcd_pin_cs = LOW; 00423 for ( ty = 0; ty < sy; ty++ ) 00424 { 00425 setXY( x, y + ( ty * scale ), x + ( ( sx * scale ) - 1 ), y + ( ty * scale ) + scale ); 00426 for ( tsy = 0; tsy < scale; tsy++ ) 00427 for ( tx = 0; tx < sx; tx++ ) 00428 for ( tsx = 0; tsx < scale; tsx++ ) 00429 writeData( data[ ( ty * sx ) + tx ] ); 00430 } 00431 _lcd_pin_cs = HIGH; 00432 } 00433 else 00434 { 00435 _lcd_pin_cs = LOW; 00436 for ( ty = 0; ty < sy; ty++ ) 00437 { 00438 for ( tsy = 0; tsy < scale; tsy++ ) 00439 { 00440 setXY( x, y + ( ty * scale ) + tsy, x + ( ( sx * scale ) - 1 ), y + ( ty * scale ) + tsy ); 00441 for ( tx = sx; tx >= 0; tx-- ) 00442 for ( tsx = 0; tsx < scale; tsx++ ) 00443 writeData( data[ ( ty * sx ) + tx ] ); 00444 } 00445 } 00446 _lcd_pin_cs = HIGH; 00447 } 00448 } 00449 clearXY(); 00450 } 00451 00452 void SSD1289::DrawBitmap( unsigned short x, unsigned short y, unsigned short sx, unsigned short sy, bitmap_t data, unsigned short deg, unsigned short rox, unsigned short roy ) 00453 { 00454 int tx, ty, newx, newy; 00455 double radian; 00456 radian = deg * 0.0175; 00457 00458 if ( deg == 0 ) 00459 DrawBitmap( x, y, sx, sy, data ); 00460 else 00461 { 00462 _lcd_pin_cs = LOW; 00463 for ( ty = 0; ty < sy; ty++ ) 00464 for ( tx = 0; tx < sx; tx++ ) 00465 { 00466 newx = x + rox + ( ( ( tx - rox ) * cos( radian ) ) - ( ( ty - roy ) * sin( radian ) ) ); 00467 newy = y + roy + ( ( ( ty - roy ) * cos( radian ) ) + ( ( tx - rox ) * sin( radian ) ) ); 00468 00469 setXY( newx, newy, newx, newy ); 00470 writeData( data[ ( ty * sx ) + tx ] ); 00471 } 00472 _lcd_pin_cs = HIGH; 00473 } 00474 clearXY(); 00475 } 00476 00477 void SSD1289::writeCmd( unsigned short cmd ) 00478 { 00479 _lcd_pin_rs = LOW; 00480 _lcd_pin_cs = LOW; 00481 _lcd_port->write( cmd ); 00482 pulseLow( _lcd_pin_wr ); 00483 _lcd_pin_cs = HIGH; 00484 } 00485 00486 void SSD1289::writeData( unsigned short data ) 00487 { 00488 _lcd_pin_rs = HIGH; 00489 _lcd_pin_cs = LOW; 00490 _lcd_port->write( data ); 00491 pulseLow( _lcd_pin_wr ); 00492 _lcd_pin_cs = HIGH; 00493 } 00494 00495 void SSD1289::writeCmdData( unsigned short cmd, unsigned short data ) 00496 { 00497 writeCmd( cmd ); 00498 writeData( data ); 00499 } 00500 00501 void SSD1289::setXY( uint16_t x1, uint16_t y1, uint16_t x2, uint16_t y2 ) 00502 { 00503 if ( _orientation == LANDSCAPE ) 00504 { 00505 swap( uint16_t, x1, y1 ) 00506 swap( uint16_t, x2, y2 ) 00507 y1 = _disp_height - y1; 00508 y2 = _disp_height - y2; 00509 swap( uint16_t, y1, y2 ) 00510 } 00511 00512 writeCmdData( 0x44, ( x2 << 8 ) + x1 ); 00513 writeCmdData( 0x45, y1 ); 00514 writeCmdData( 0x46, y2 ); 00515 writeCmdData( 0x4e, x1 ); 00516 writeCmdData( 0x4f, y1 ); 00517 writeCmd( 0x22 ); 00518 } 00519 00520 void SSD1289::clearXY() 00521 { 00522 if ( _orientation == PORTRAIT ) 00523 setXY( 0, 0, _disp_width, _disp_height ); 00524 else 00525 setXY( 0, 0, _disp_height, _disp_width ); 00526 } 00527 00528 void SSD1289::drawHLine( unsigned short x, unsigned short y, unsigned short len, int color ) 00529 { 00530 unsigned short usedColor = color == -1 ? _background : color == -2 ? _foreground : ( unsigned short ) color; 00531 00532 _lcd_pin_cs = LOW; 00533 setXY( x, y, x + len, y ); 00534 for ( int i = 0; i < len + 1; i++ ) 00535 writeData( usedColor ); 00536 _lcd_pin_cs = HIGH; 00537 clearXY(); 00538 } 00539 00540 void SSD1289::drawVLine( unsigned short x, unsigned short y, unsigned short len, int color ) 00541 { 00542 unsigned short usedColor = color == -1 ? _background : color == -2 ? _foreground : ( unsigned short ) color; 00543 00544 _lcd_pin_cs = LOW; 00545 setXY( x, y, x, y + len ); 00546 for ( int i = 0; i < len; i++ ) 00547 writeData( usedColor ); 00548 _lcd_pin_cs = HIGH; 00549 clearXY(); 00550 } 00551 00552 void SSD1289::printChar( char c, unsigned short x, unsigned short y, int fgColor, int bgColor ) 00553 { 00554 uint8_t i, ch; 00555 uint16_t j; 00556 uint16_t temp; 00557 unsigned short usedColorFG = fgColor == -1 ? _background : fgColor == -2 ? _foreground : ( unsigned short ) fgColor; 00558 unsigned short usedColorBG = bgColor == -1 ? _background : bgColor == -2 ? _foreground : ( unsigned short ) bgColor; 00559 00560 _lcd_pin_cs = LOW; 00561 00562 if ( _orientation == PORTRAIT ) 00563 { 00564 setXY( x, y, x + _font.width - 1, y + _font.height - 1 ); 00565 00566 temp = ( ( c - _font.offset ) * ( ( _font.width / 8 ) * _font.height ) ) + 4; 00567 for ( j = 0; j < ( ( _font.width / 8 ) * _font.height ); j++ ) 00568 { 00569 ch = _font.font[ temp ]; 00570 for ( i = 0; i < 8; i++ ) 00571 { 00572 if ( ( ch & ( 1 << ( 7 - i ) ) ) != 0 ) 00573 writeData( usedColorFG ); 00574 else 00575 writeData( usedColorBG ); 00576 } 00577 temp++; 00578 } 00579 } 00580 else 00581 { 00582 temp = ( ( c - _font.offset ) * ( ( _font.width / 8 ) * _font.height ) ) + 4; 00583 00584 for ( j = 0; j < ( ( _font.width / 8 ) * _font.height ); j += ( _font.width / 8 ) ) 00585 { 00586 setXY( x, y + ( j / ( _font.width / 8 ) ), x + _font.width - 1, y + ( j / ( _font.width / 8 ) ) ); 00587 for ( int zz = ( _font.width / 8 ) - 1; zz >= 0; zz-- ) 00588 { 00589 ch = _font.font[ temp + zz ]; 00590 for ( i = 0; i < 8; i++ ) 00591 { 00592 if ( ( ch & ( 1 << i ) ) != 0 ) 00593 writeData( usedColorFG ); 00594 else 00595 writeData( usedColorBG ); 00596 } 00597 } 00598 temp += ( _font.width / 8 ); 00599 } 00600 } 00601 _lcd_pin_cs = HIGH; 00602 clearXY(); 00603 } 00604 00605 void SSD1289::rotateChar( char c, unsigned short x, unsigned short y, int pos, int fgColor, int bgColor, unsigned short deg ) 00606 { 00607 uint8_t i, j, ch; 00608 uint16_t temp; 00609 int newx, newy; 00610 double radian; 00611 radian = deg * 0.0175; 00612 00613 unsigned short usedColorFG = fgColor == -1 ? _background : fgColor == -2 ? _foreground : ( unsigned short ) fgColor; 00614 unsigned short usedColorBG = bgColor == -1 ? _background : bgColor == -2 ? _foreground : ( unsigned short ) bgColor; 00615 00616 _lcd_pin_cs = LOW; 00617 00618 temp = ( ( c - _font.offset ) * ( ( _font.width / 8 ) * _font.height ) ) + 4; 00619 for ( j = 0; j < _font.height; j++ ) 00620 { 00621 for ( int zz = 0; zz < ( _font.width / 8 ); zz++ ) 00622 { 00623 ch = _font.font[ temp + zz ]; 00624 for ( i = 0; i < 8; i++ ) 00625 { 00626 newx = x + ( ( ( i + ( zz * 8 ) + ( pos * _font.width ) ) * cos( radian ) ) - ( ( j ) * sin( radian ) ) ); 00627 newy = y + ( ( ( j ) * cos( radian ) ) + ( ( i + ( zz * 8 ) + ( pos * _font.width ) ) * sin( radian ) ) ); 00628 00629 setXY( newx, newy, newx + 1, newy + 1 ); 00630 00631 if ( ( ch & ( 1 << ( 7 - i ) ) ) != 0 ) 00632 writeData( usedColorFG ); 00633 else 00634 writeData( usedColorBG ); 00635 } 00636 } 00637 temp += ( _font.width / 8 ); 00638 } 00639 _lcd_pin_cs = HIGH; 00640 clearXY(); 00641 }
Generated on Wed Jul 13 2022 06:38:58 by
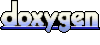