CMSIS DSP Library from CMSIS 2.0. See http://www.onarm.com/cmsis/ for full details
Dependents: K22F_DSP_Matrix_least_square BNO055-ELEC3810 1BNO055 ECE4180Project--Slave2 ... more
Sine Cosine
[Controller Functions]
Computes the trigonometric sine and cosine values using a combination of table lookup and linear interpolation. More...
Functions | |
void | arm_sin_cos_f32 (float32_t theta, float32_t *pSinVal, float32_t *pCosVal) |
Floating-point sin_cos function. | |
void | arm_sin_cos_q31 (q31_t theta, q31_t *pSinVal, q31_t *pCosVal) |
Q31 sin_cos function. | |
Variables | |
static const float32_t | cosTable [360] |
static const float32_t | sinTable [360] |
static const int32_t | sinTableQ31 [360] |
static const int32_t | cosTableQ31 [360] |
Detailed Description
Computes the trigonometric sine and cosine values using a combination of table lookup and linear interpolation.
There are separate functions for Q31 and floating-point data types. The input to the floating-point version is in degrees while the fixed-point Q31 have a scaled input with the range [-1 1) mapping to [-180 180) degrees.
The implementation is based on table lookup using 360 values together with linear interpolation. The steps used are:
- Calculation of the nearest integer table index.
- Compute the fractional portion (fract) of the input.
- Fetch the value corresponding to
index
from sine table toy0
and also value fromindex+1
toy1
. - Sine value is computed as
*psinVal = y0 + (fract * (y1 - y0))
. - Fetch the value corresponding to
index
from cosine table toy0
and also value fromindex+1
toy1
. - Cosine value is computed as
*pcosVal = y0 + (fract * (y1 - y0))
.
Function Documentation
void arm_sin_cos_f32 | ( | float32_t | theta, |
float32_t * | pSinVal, | ||
float32_t * | pCosVal | ||
) |
Floating-point sin_cos function.
- Parameters:
-
[in] theta input value in degrees [out] *pSinVal points to the processed sine output. [out] *pCosVal points to the processed cos output.
- Returns:
- none.
Definition at line 364 of file arm_sin_cos_f32.c.
void arm_sin_cos_q31 | ( | q31_t | theta, |
q31_t * | pSinVal, | ||
q31_t * | pCosVal | ||
) |
Q31 sin_cos function.
- Parameters:
-
[in] theta scaled input value in degrees [out] *pSinVal points to the processed sine output. [out] *pCosVal points to the processed cosine output.
- Returns:
- none.
The Q31 input value is in the range [-1 +1) and is mapped to a degree value in the range [-180 180).
Definition at line 261 of file arm_sin_cos_q31.c.
Variable Documentation
const float32_t cosTable[360] [static] |
- Cosine Table is generated from following loop
for(i = 0; i < 360; i++) { cosTable[i]= cos((i-180) * PI/180.0); }
Definition at line 68 of file arm_sin_cos_f32.c.
const int32_t cosTableQ31[360] [static] |
- Cosine Table is generated from following loop
for(i = 0; i < 360; i++) { cosTable[i]= cos((i-180) * PI/180.0); }
- Convert above coefficients to fixed point 1.31 format.
Definition at line 154 of file arm_sin_cos_q31.c.
const float32_t sinTable[360] [static] |
- Sine Table is generated from following loop
for(i = 0; i < 360; i++) { sinTable[i]= sin((i-180) * PI/180.0); }
Definition at line 216 of file arm_sin_cos_f32.c.
const int32_t sinTableQ31[360] [static] |
- Sine Table is generated from following loop
for(i = 0; i < 360; i++) { sinTable[i]= sin((i-180) * PI/180.0); }
Convert above coefficients to fixed point 1.31 format.
Definition at line 48 of file arm_sin_cos_q31.c.
Generated on Tue Jul 12 2022 14:13:55 by
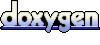