CMSIS DSP Library from CMSIS 2.0. See http://www.onarm.com/cmsis/ for full details
Dependents: K22F_DSP_Matrix_least_square BNO055-ELEC3810 1BNO055 ECE4180Project--Slave2 ... more
arm_sin_cos_f32.c
00001 /* ---------------------------------------------------------------------- 00002 * Copyright (C) 2010 ARM Limited. All rights reserved. 00003 * 00004 * $Date: 29. November 2010 00005 * $Revision: V1.0.3 00006 * 00007 * Project: CMSIS DSP Library 00008 * Title: arm_sin_cos_f32.c 00009 * 00010 * Description: Sine and Cosine calculation for floating-point values. 00011 * 00012 * Target Processor: Cortex-M4/Cortex-M3 00013 * 00014 * Version 1.0.3 2010/11/29 00015 * Re-organized the CMSIS folders and updated documentation. 00016 * 00017 * Version 1.0.2 2010/11/11 00018 * Documentation updated. 00019 * 00020 * Version 1.0.1 2010/10/05 00021 * Production release and review comments incorporated. 00022 * 00023 * Version 1.0.0 2010/09/20 00024 * Production release and review comments incorporated. 00025 * -------------------------------------------------------------------- */ 00026 00027 #include "arm_math.h" 00028 00029 /** 00030 * @ingroup groupController 00031 */ 00032 00033 /** 00034 * @defgroup SinCos Sine Cosine 00035 * 00036 * Computes the trigonometric sine and cosine values using a combination of table lookup 00037 * and linear interpolation. 00038 * There are separate functions for Q31 and floating-point data types. 00039 * The input to the floating-point version is in degrees while the 00040 * fixed-point Q31 have a scaled input with the range 00041 * [-1 1) mapping to [-180 180) degrees. 00042 * 00043 * The implementation is based on table lookup using 360 values together with linear interpolation. 00044 * The steps used are: 00045 * -# Calculation of the nearest integer table index. 00046 * -# Compute the fractional portion (fract) of the input. 00047 * -# Fetch the value corresponding to \c index from sine table to \c y0 and also value from \c index+1 to \c y1. 00048 * -# Sine value is computed as <code> *psinVal = y0 + (fract * (y1 - y0))</code>. 00049 * -# Fetch the value corresponding to \c index from cosine table to \c y0 and also value from \c index+1 to \c y1. 00050 * -# Cosine value is computed as <code> *pcosVal = y0 + (fract * (y1 - y0))</code>. 00051 */ 00052 00053 /** 00054 * @addtogroup SinCos 00055 * @{ 00056 */ 00057 00058 00059 /** 00060 * \par 00061 * Cosine Table is generated from following loop 00062 * <pre>for(i = 0; i < 360; i++) 00063 * { 00064 * cosTable[i]= cos((i-180) * PI/180.0); 00065 * } </pre> 00066 */ 00067 00068 static const float32_t cosTable [360] = { 00069 -0.999847695156391270f, -0.999390827019095760f, -0.998629534754573830f, 00070 -0.997564050259824200f, -0.996194698091745550f, -0.994521895368273290f, 00071 -0.992546151641321980f, -0.990268068741570250f, 00072 -0.987688340595137660f, -0.984807753012208020f, -0.981627183447663980f, 00073 -0.978147600733805690f, -0.974370064785235250f, -0.970295726275996470f, 00074 -0.965925826289068200f, -0.961261695938318670f, 00075 -0.956304755963035440f, -0.951056516295153530f, -0.945518575599316740f, 00076 -0.939692620785908320f, -0.933580426497201740f, -0.927183854566787310f, 00077 -0.920504853452440150f, -0.913545457642600760f, 00078 -0.906307787036649940f, -0.898794046299167040f, -0.891006524188367790f, 00079 -0.882947592858926770f, -0.874619707139395740f, -0.866025403784438710f, 00080 -0.857167300702112220f, -0.848048096156425960f, 00081 -0.838670567945424160f, -0.829037572555041620f, -0.819152044288991580f, 00082 -0.809016994374947340f, -0.798635510047292940f, -0.788010753606721900f, 00083 -0.777145961456970680f, -0.766044443118977900f, 00084 -0.754709580222772010f, -0.743144825477394130f, -0.731353701619170460f, 00085 -0.719339800338651300f, -0.707106781186547460f, -0.694658370458997030f, 00086 -0.681998360062498370f, -0.669130606358858240f, 00087 -0.656059028990507500f, -0.642787609686539360f, -0.629320391049837280f, 00088 -0.615661475325658290f, -0.601815023152048380f, -0.587785252292473030f, 00089 -0.573576436351045830f, -0.559192903470746680f, 00090 -0.544639035015027080f, -0.529919264233204790f, -0.515038074910054270f, 00091 -0.499999999999999780f, -0.484809620246337000f, -0.469471562785890530f, 00092 -0.453990499739546750f, -0.438371146789077510f, 00093 -0.422618261740699330f, -0.406736643075800100f, -0.390731128489273600f, 00094 -0.374606593415912070f, -0.358367949545300270f, -0.342020143325668710f, 00095 -0.325568154457156420f, -0.309016994374947340f, 00096 -0.292371704722736660f, -0.275637355816999050f, -0.258819045102520850f, 00097 -0.241921895599667790f, -0.224951054343864810f, -0.207911690817759120f, 00098 -0.190808995376544800f, -0.173648177666930300f, 00099 -0.156434465040231040f, -0.139173100960065350f, -0.121869343405147370f, 00100 -0.104528463267653330f, -0.087155742747658235f, -0.069756473744125330f, 00101 -0.052335956242943620f, -0.034899496702500733f, 00102 -0.017452406437283477f, 0.000000000000000061f, 0.017452406437283376f, 00103 0.034899496702501080f, 0.052335956242943966f, 0.069756473744125455f, 00104 0.087155742747658138f, 0.104528463267653460f, 00105 0.121869343405147490f, 0.139173100960065690f, 0.156434465040230920f, 00106 0.173648177666930410f, 0.190808995376544920f, 0.207911690817759450f, 00107 0.224951054343864920f, 0.241921895599667900f, 00108 0.258819045102520740f, 0.275637355816999160f, 0.292371704722736770f, 00109 0.309016994374947450f, 0.325568154457156760f, 0.342020143325668820f, 00110 0.358367949545300380f, 0.374606593415911960f, 00111 0.390731128489273940f, 0.406736643075800210f, 0.422618261740699440f, 00112 0.438371146789077460f, 0.453990499739546860f, 0.469471562785890860f, 00113 0.484809620246337110f, 0.500000000000000110f, 00114 0.515038074910054380f, 0.529919264233204900f, 0.544639035015027200f, 00115 0.559192903470746790f, 0.573576436351046050f, 0.587785252292473140f, 00116 0.601815023152048270f, 0.615661475325658290f, 00117 0.629320391049837500f, 0.642787609686539360f, 0.656059028990507280f, 00118 0.669130606358858240f, 0.681998360062498480f, 0.694658370458997370f, 00119 0.707106781186547570f, 0.719339800338651190f, 00120 0.731353701619170570f, 0.743144825477394240f, 0.754709580222772010f, 00121 0.766044443118978010f, 0.777145961456970900f, 0.788010753606722010f, 00122 0.798635510047292830f, 0.809016994374947450f, 00123 0.819152044288991800f, 0.829037572555041620f, 0.838670567945424050f, 00124 0.848048096156425960f, 0.857167300702112330f, 0.866025403784438710f, 00125 0.874619707139395740f, 0.882947592858926990f, 00126 0.891006524188367900f, 0.898794046299167040f, 0.906307787036649940f, 00127 0.913545457642600870f, 0.920504853452440370f, 0.927183854566787420f, 00128 0.933580426497201740f, 0.939692620785908430f, 00129 0.945518575599316850f, 0.951056516295153530f, 0.956304755963035440f, 00130 0.961261695938318890f, 0.965925826289068310f, 0.970295726275996470f, 00131 0.974370064785235250f, 0.978147600733805690f, 00132 0.981627183447663980f, 0.984807753012208020f, 0.987688340595137770f, 00133 0.990268068741570360f, 0.992546151641321980f, 0.994521895368273290f, 00134 0.996194698091745550f, 0.997564050259824200f, 00135 0.998629534754573830f, 0.999390827019095760f, 0.999847695156391270f, 00136 1.000000000000000000f, 0.999847695156391270f, 0.999390827019095760f, 00137 0.998629534754573830f, 0.997564050259824200f, 00138 0.996194698091745550f, 0.994521895368273290f, 0.992546151641321980f, 00139 0.990268068741570360f, 0.987688340595137770f, 0.984807753012208020f, 00140 0.981627183447663980f, 0.978147600733805690f, 00141 0.974370064785235250f, 0.970295726275996470f, 0.965925826289068310f, 00142 0.961261695938318890f, 0.956304755963035440f, 0.951056516295153530f, 00143 0.945518575599316850f, 0.939692620785908430f, 00144 0.933580426497201740f, 0.927183854566787420f, 0.920504853452440370f, 00145 0.913545457642600870f, 0.906307787036649940f, 0.898794046299167040f, 00146 0.891006524188367900f, 0.882947592858926990f, 00147 0.874619707139395740f, 0.866025403784438710f, 0.857167300702112330f, 00148 0.848048096156425960f, 0.838670567945424050f, 0.829037572555041620f, 00149 0.819152044288991800f, 0.809016994374947450f, 00150 0.798635510047292830f, 0.788010753606722010f, 0.777145961456970900f, 00151 0.766044443118978010f, 0.754709580222772010f, 0.743144825477394240f, 00152 0.731353701619170570f, 0.719339800338651190f, 00153 0.707106781186547570f, 0.694658370458997370f, 0.681998360062498480f, 00154 0.669130606358858240f, 0.656059028990507280f, 0.642787609686539360f, 00155 0.629320391049837500f, 0.615661475325658290f, 00156 0.601815023152048270f, 0.587785252292473140f, 0.573576436351046050f, 00157 0.559192903470746790f, 0.544639035015027200f, 0.529919264233204900f, 00158 0.515038074910054380f, 0.500000000000000110f, 00159 0.484809620246337110f, 0.469471562785890860f, 0.453990499739546860f, 00160 0.438371146789077460f, 0.422618261740699440f, 0.406736643075800210f, 00161 0.390731128489273940f, 0.374606593415911960f, 00162 0.358367949545300380f, 0.342020143325668820f, 0.325568154457156760f, 00163 0.309016994374947450f, 0.292371704722736770f, 0.275637355816999160f, 00164 0.258819045102520740f, 0.241921895599667900f, 00165 0.224951054343864920f, 0.207911690817759450f, 0.190808995376544920f, 00166 0.173648177666930410f, 0.156434465040230920f, 0.139173100960065690f, 00167 0.121869343405147490f, 0.104528463267653460f, 00168 0.087155742747658138f, 0.069756473744125455f, 0.052335956242943966f, 00169 0.034899496702501080f, 0.017452406437283376f, 0.000000000000000061f, 00170 -0.017452406437283477f, -0.034899496702500733f, 00171 -0.052335956242943620f, -0.069756473744125330f, -0.087155742747658235f, 00172 -0.104528463267653330f, -0.121869343405147370f, -0.139173100960065350f, 00173 -0.156434465040231040f, -0.173648177666930300f, 00174 -0.190808995376544800f, -0.207911690817759120f, -0.224951054343864810f, 00175 -0.241921895599667790f, -0.258819045102520850f, -0.275637355816999050f, 00176 -0.292371704722736660f, -0.309016994374947340f, 00177 -0.325568154457156420f, -0.342020143325668710f, -0.358367949545300270f, 00178 -0.374606593415912070f, -0.390731128489273600f, -0.406736643075800100f, 00179 -0.422618261740699330f, -0.438371146789077510f, 00180 -0.453990499739546750f, -0.469471562785890530f, -0.484809620246337000f, 00181 -0.499999999999999780f, -0.515038074910054270f, -0.529919264233204790f, 00182 -0.544639035015027080f, -0.559192903470746680f, 00183 -0.573576436351045830f, -0.587785252292473030f, -0.601815023152048380f, 00184 -0.615661475325658290f, -0.629320391049837280f, -0.642787609686539360f, 00185 -0.656059028990507500f, -0.669130606358858240f, 00186 -0.681998360062498370f, -0.694658370458997030f, -0.707106781186547460f, 00187 -0.719339800338651300f, -0.731353701619170460f, -0.743144825477394130f, 00188 -0.754709580222772010f, -0.766044443118977900f, 00189 -0.777145961456970680f, -0.788010753606721900f, -0.798635510047292940f, 00190 -0.809016994374947340f, -0.819152044288991580f, -0.829037572555041620f, 00191 -0.838670567945424160f, -0.848048096156425960f, 00192 -0.857167300702112220f, -0.866025403784438710f, -0.874619707139395740f, 00193 -0.882947592858926770f, -0.891006524188367790f, -0.898794046299167040f, 00194 -0.906307787036649940f, -0.913545457642600760f, 00195 -0.920504853452440150f, -0.927183854566787310f, -0.933580426497201740f, 00196 -0.939692620785908320f, -0.945518575599316740f, -0.951056516295153530f, 00197 -0.956304755963035440f, -0.961261695938318670f, 00198 -0.965925826289068200f, -0.970295726275996470f, -0.974370064785235250f, 00199 -0.978147600733805690f, -0.981627183447663980f, -0.984807753012208020f, 00200 -0.987688340595137660f, -0.990268068741570250f, 00201 -0.992546151641321980f, -0.994521895368273290f, -0.996194698091745550f, 00202 -0.997564050259824200f, -0.998629534754573830f, -0.999390827019095760f, 00203 -0.999847695156391270f, -1.000000000000000000f 00204 }; 00205 00206 /** 00207 * \par 00208 * Sine Table is generated from following loop 00209 * <pre>for(i = 0; i < 360; i++) 00210 * { 00211 * sinTable[i]= sin((i-180) * PI/180.0); 00212 * } </pre> 00213 */ 00214 00215 00216 static const float32_t sinTable [360] = { 00217 -0.017452406437283439f, -0.034899496702500699f, -0.052335956242943807f, 00218 -0.069756473744125524f, -0.087155742747658638f, -0.104528463267653730f, 00219 -0.121869343405147550f, -0.139173100960065740f, 00220 -0.156434465040230980f, -0.173648177666930280f, -0.190808995376544970f, 00221 -0.207911690817759310f, -0.224951054343864780f, -0.241921895599667730f, 00222 -0.258819045102521020f, -0.275637355816999660f, 00223 -0.292371704722737050f, -0.309016994374947510f, -0.325568154457156980f, 00224 -0.342020143325668880f, -0.358367949545300210f, -0.374606593415912240f, 00225 -0.390731128489274160f, -0.406736643075800430f, 00226 -0.422618261740699500f, -0.438371146789077290f, -0.453990499739546860f, 00227 -0.469471562785891080f, -0.484809620246337170f, -0.499999999999999940f, 00228 -0.515038074910054380f, -0.529919264233204900f, 00229 -0.544639035015026860f, -0.559192903470746900f, -0.573576436351046380f, 00230 -0.587785252292473250f, -0.601815023152048160f, -0.615661475325658400f, 00231 -0.629320391049837720f, -0.642787609686539470f, 00232 -0.656059028990507280f, -0.669130606358858350f, -0.681998360062498590f, 00233 -0.694658370458997140f, -0.707106781186547570f, -0.719339800338651410f, 00234 -0.731353701619170570f, -0.743144825477394240f, 00235 -0.754709580222771790f, -0.766044443118978010f, -0.777145961456971010f, 00236 -0.788010753606722010f, -0.798635510047292720f, -0.809016994374947450f, 00237 -0.819152044288992020f, -0.829037572555041740f, 00238 -0.838670567945424050f, -0.848048096156426070f, -0.857167300702112330f, 00239 -0.866025403784438710f, -0.874619707139395850f, -0.882947592858927100f, 00240 -0.891006524188367900f, -0.898794046299166930f, 00241 -0.906307787036650050f, -0.913545457642600980f, -0.920504853452440370f, 00242 -0.927183854566787420f, -0.933580426497201740f, -0.939692620785908430f, 00243 -0.945518575599316850f, -0.951056516295153640f, 00244 -0.956304755963035550f, -0.961261695938318890f, -0.965925826289068310f, 00245 -0.970295726275996470f, -0.974370064785235250f, -0.978147600733805690f, 00246 -0.981627183447663980f, -0.984807753012208020f, 00247 -0.987688340595137660f, -0.990268068741570360f, -0.992546151641322090f, 00248 -0.994521895368273400f, -0.996194698091745550f, -0.997564050259824200f, 00249 -0.998629534754573830f, -0.999390827019095760f, 00250 -0.999847695156391270f, -1.000000000000000000f, -0.999847695156391270f, 00251 -0.999390827019095760f, -0.998629534754573830f, -0.997564050259824200f, 00252 -0.996194698091745550f, -0.994521895368273290f, 00253 -0.992546151641321980f, -0.990268068741570250f, -0.987688340595137770f, 00254 -0.984807753012208020f, -0.981627183447663980f, -0.978147600733805580f, 00255 -0.974370064785235250f, -0.970295726275996470f, 00256 -0.965925826289068310f, -0.961261695938318890f, -0.956304755963035440f, 00257 -0.951056516295153530f, -0.945518575599316740f, -0.939692620785908320f, 00258 -0.933580426497201740f, -0.927183854566787420f, 00259 -0.920504853452440260f, -0.913545457642600870f, -0.906307787036649940f, 00260 -0.898794046299167040f, -0.891006524188367790f, -0.882947592858926880f, 00261 -0.874619707139395740f, -0.866025403784438600f, 00262 -0.857167300702112220f, -0.848048096156426070f, -0.838670567945423940f, 00263 -0.829037572555041740f, -0.819152044288991800f, -0.809016994374947450f, 00264 -0.798635510047292830f, -0.788010753606722010f, 00265 -0.777145961456970790f, -0.766044443118978010f, -0.754709580222772010f, 00266 -0.743144825477394240f, -0.731353701619170460f, -0.719339800338651080f, 00267 -0.707106781186547460f, -0.694658370458997250f, 00268 -0.681998360062498480f, -0.669130606358858240f, -0.656059028990507160f, 00269 -0.642787609686539250f, -0.629320391049837390f, -0.615661475325658180f, 00270 -0.601815023152048270f, -0.587785252292473140f, 00271 -0.573576436351046050f, -0.559192903470746900f, -0.544639035015027080f, 00272 -0.529919264233204900f, -0.515038074910054160f, -0.499999999999999940f, 00273 -0.484809620246337060f, -0.469471562785890810f, 00274 -0.453990499739546750f, -0.438371146789077400f, -0.422618261740699440f, 00275 -0.406736643075800150f, -0.390731128489273720f, -0.374606593415912010f, 00276 -0.358367949545300270f, -0.342020143325668710f, 00277 -0.325568154457156640f, -0.309016994374947400f, -0.292371704722736770f, 00278 -0.275637355816999160f, -0.258819045102520740f, -0.241921895599667730f, 00279 -0.224951054343865000f, -0.207911690817759310f, 00280 -0.190808995376544800f, -0.173648177666930330f, -0.156434465040230870f, 00281 -0.139173100960065440f, -0.121869343405147480f, -0.104528463267653460f, 00282 -0.087155742747658166f, -0.069756473744125302f, 00283 -0.052335956242943828f, -0.034899496702500969f, -0.017452406437283512f, 00284 0.000000000000000000f, 0.017452406437283512f, 0.034899496702500969f, 00285 0.052335956242943828f, 0.069756473744125302f, 00286 0.087155742747658166f, 0.104528463267653460f, 0.121869343405147480f, 00287 0.139173100960065440f, 0.156434465040230870f, 0.173648177666930330f, 00288 0.190808995376544800f, 0.207911690817759310f, 00289 0.224951054343865000f, 0.241921895599667730f, 0.258819045102520740f, 00290 0.275637355816999160f, 0.292371704722736770f, 0.309016994374947400f, 00291 0.325568154457156640f, 0.342020143325668710f, 00292 0.358367949545300270f, 0.374606593415912010f, 0.390731128489273720f, 00293 0.406736643075800150f, 0.422618261740699440f, 0.438371146789077400f, 00294 0.453990499739546750f, 0.469471562785890810f, 00295 0.484809620246337060f, 0.499999999999999940f, 0.515038074910054160f, 00296 0.529919264233204900f, 0.544639035015027080f, 0.559192903470746900f, 00297 0.573576436351046050f, 0.587785252292473140f, 00298 0.601815023152048270f, 0.615661475325658180f, 0.629320391049837390f, 00299 0.642787609686539250f, 0.656059028990507160f, 0.669130606358858240f, 00300 0.681998360062498480f, 0.694658370458997250f, 00301 0.707106781186547460f, 0.719339800338651080f, 0.731353701619170460f, 00302 0.743144825477394240f, 0.754709580222772010f, 0.766044443118978010f, 00303 0.777145961456970790f, 0.788010753606722010f, 00304 0.798635510047292830f, 0.809016994374947450f, 0.819152044288991800f, 00305 0.829037572555041740f, 0.838670567945423940f, 0.848048096156426070f, 00306 0.857167300702112220f, 0.866025403784438600f, 00307 0.874619707139395740f, 0.882947592858926880f, 0.891006524188367790f, 00308 0.898794046299167040f, 0.906307787036649940f, 0.913545457642600870f, 00309 0.920504853452440260f, 0.927183854566787420f, 00310 0.933580426497201740f, 0.939692620785908320f, 0.945518575599316740f, 00311 0.951056516295153530f, 0.956304755963035440f, 0.961261695938318890f, 00312 0.965925826289068310f, 0.970295726275996470f, 00313 0.974370064785235250f, 0.978147600733805580f, 0.981627183447663980f, 00314 0.984807753012208020f, 0.987688340595137770f, 0.990268068741570250f, 00315 0.992546151641321980f, 0.994521895368273290f, 00316 0.996194698091745550f, 0.997564050259824200f, 0.998629534754573830f, 00317 0.999390827019095760f, 0.999847695156391270f, 1.000000000000000000f, 00318 0.999847695156391270f, 0.999390827019095760f, 00319 0.998629534754573830f, 0.997564050259824200f, 0.996194698091745550f, 00320 0.994521895368273400f, 0.992546151641322090f, 0.990268068741570360f, 00321 0.987688340595137660f, 0.984807753012208020f, 00322 0.981627183447663980f, 0.978147600733805690f, 0.974370064785235250f, 00323 0.970295726275996470f, 0.965925826289068310f, 0.961261695938318890f, 00324 0.956304755963035550f, 0.951056516295153640f, 00325 0.945518575599316850f, 0.939692620785908430f, 0.933580426497201740f, 00326 0.927183854566787420f, 0.920504853452440370f, 0.913545457642600980f, 00327 0.906307787036650050f, 0.898794046299166930f, 00328 0.891006524188367900f, 0.882947592858927100f, 0.874619707139395850f, 00329 0.866025403784438710f, 0.857167300702112330f, 0.848048096156426070f, 00330 0.838670567945424050f, 0.829037572555041740f, 00331 0.819152044288992020f, 0.809016994374947450f, 0.798635510047292720f, 00332 0.788010753606722010f, 0.777145961456971010f, 0.766044443118978010f, 00333 0.754709580222771790f, 0.743144825477394240f, 00334 0.731353701619170570f, 0.719339800338651410f, 0.707106781186547570f, 00335 0.694658370458997140f, 0.681998360062498590f, 0.669130606358858350f, 00336 0.656059028990507280f, 0.642787609686539470f, 00337 0.629320391049837720f, 0.615661475325658400f, 0.601815023152048160f, 00338 0.587785252292473250f, 0.573576436351046380f, 0.559192903470746900f, 00339 0.544639035015026860f, 0.529919264233204900f, 00340 0.515038074910054380f, 0.499999999999999940f, 0.484809620246337170f, 00341 0.469471562785891080f, 0.453990499739546860f, 0.438371146789077290f, 00342 0.422618261740699500f, 0.406736643075800430f, 00343 0.390731128489274160f, 0.374606593415912240f, 0.358367949545300210f, 00344 0.342020143325668880f, 0.325568154457156980f, 0.309016994374947510f, 00345 0.292371704722737050f, 0.275637355816999660f, 00346 0.258819045102521020f, 0.241921895599667730f, 0.224951054343864780f, 00347 0.207911690817759310f, 0.190808995376544970f, 0.173648177666930280f, 00348 0.156434465040230980f, 0.139173100960065740f, 00349 0.121869343405147550f, 0.104528463267653730f, 0.087155742747658638f, 00350 0.069756473744125524f, 0.052335956242943807f, 0.034899496702500699f, 00351 0.017452406437283439f, 0.000000000000000122f 00352 }; 00353 00354 00355 /** 00356 * @brief Floating-point sin_cos function. 00357 * @param[in] theta input value in degrees 00358 * @param[out] *pSinVal points to the processed sine output. 00359 * @param[out] *pCosVal points to the processed cos output. 00360 * @return none. 00361 */ 00362 00363 00364 void arm_sin_cos_f32( 00365 float32_t theta, 00366 float32_t * pSinVal, 00367 float32_t * pCosVal) 00368 { 00369 uint32_t i; /* Index for reading nearwst output values */ 00370 float32_t x1 = -179.0f; /* Initial input value */ 00371 float32_t y0, y1; /* nearest output values */ 00372 float32_t fract; /* fractional part of input */ 00373 00374 /* Calculation of fractional part */ 00375 if(theta > 0.0f) 00376 { 00377 fract = theta - (float32_t) ((int32_t) theta); 00378 } 00379 else 00380 { 00381 fract = (theta - (float32_t) ((int32_t) theta)) + 1.0f; 00382 } 00383 00384 /* index calculation for reading nearest output values */ 00385 i = (uint32_t) (theta - x1); 00386 00387 /* reading nearest sine output values */ 00388 y0 = sinTable [i]; 00389 y1 = sinTable [i + 1u]; 00390 00391 /* Calculation of sine value */ 00392 *pSinVal = y0 + (fract * (y1 - y0)); 00393 00394 /* reading nearest cosine output values */ 00395 y0 = cosTable [i]; 00396 y1 = cosTable [i + 1u]; 00397 00398 /* Calculation of cosine value */ 00399 *pCosVal = y0 + (fract * (y1 - y0)); 00400 00401 } 00402 00403 /** 00404 * @} end of SinCos group 00405 */
Generated on Tue Jul 12 2022 14:13:54 by
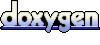