AHRS based on MatrixPilot DCM algorithm; ported from Pololu MinIMU-9 example code in turn based on ArduPilot 1.5
DCM Class Reference
DCM AHRS algorithm ported to mbed from Pololu MinIMU-9 in turn ported from ArduPilot 1.5 revised in a more OO style, though no done yet. More...
#include <DCM.h>
Public Member Functions | |
DCM (void) | |
Creates a new DCM AHRS algorithm object. | |
void | Update_step (void) |
A single update cycle for the algorithm; updates the matrix, normalizes it, corrects for gyro drift on 3 axes, (does not yet adjust for acceleration). | |
Data Fields | |
float | roll |
Output: Euler angle: roll. | |
float | pitch |
Output: Euler angle: pitch. | |
float | yaw |
Output: Euler angle: yaw. | |
int | gyro_x |
Input gyro sensor reading X-axis. | |
int | gyro_y |
Input gyro sensor reading Y-axis. | |
int | gyro_z |
Input gyro sensor reading Z-axis. | |
int | accel_x |
Input accelerometer sensor reading X-axis. | |
int | accel_y |
Input accelerometer sensor reading Y-axis. | |
int | accel_z |
Input accelerometer sensor reading Z-axis. | |
float | c_magnetom_x |
Input for the offset corrected & scaled magnetometer reading, X-axis. | |
float | c_magnetom_y |
Input for the offset corrected & scaled magnetometer reading, Y-axis. | |
float | c_magnetom_z |
Input for the offset corrected & scaled magnetometer reading, Z-axis. | |
float | MAG_Heading |
Input for the calculated magnetic heading. | |
float | speed_3d |
Input for the speed (ie, the magnitude of the 3d velocity vector. | |
float | G_Dt |
Input for the integration time (DCM algorithm) |
Detailed Description
DCM AHRS algorithm ported to mbed from Pololu MinIMU-9 in turn ported from ArduPilot 1.5 revised in a more OO style, though no done yet.
Early version. There's more to do here but it's a start, at least.
Warning: Interface will most likely change.
Expects to see a Sensors.h in your project, as follows, with functions that read sensors and set the appropriate "input" member variables below. Eventually I'll change this to a better encapsulated OOP approach.
This approach of an external Sensors.* is an abstraction layer that makes it much, much easier to swap in a different sensor suite versus the original code. You can use Serial, I2C, SPI, Analog, whatever you want, whatever it takes as long as you populate the gyro, accel, and mag vectors before calling Update_step()
#ifndef __SENSORS_H #define __SENSORS_H void Gyro_Init(void); void Read_Gyro(void); void Accel_Init(void); void Read_Accel(void); void Compass_Init(void); void Read_Compass(void); void Calculate_Offsets(void); void Compass_Heading(void); #endif
Definition at line 96 of file DCM.h.
Constructor & Destructor Documentation
Member Function Documentation
void Update_step | ( | void | ) |
Field Documentation
float c_magnetom_x |
float c_magnetom_y |
float c_magnetom_z |
float MAG_Heading |
float speed_3d |
Generated on Sat Jul 16 2022 20:25:06 by
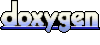