
Code for autonomous ground vehicle, Data Bus, 3rd place winner in 2012 Sparkfun AVC.
Dependencies: Watchdog mbed Schedule SimpleFilter LSM303DLM PinDetect DebounceIn Servo
Config.h
00001 #ifndef __CONFIG_H 00002 #define __CONFIG_H 00003 00004 #include "GeoPosition.h" 00005 #include "CartPosition.h" 00006 00007 /** Text-based configuration; reads config file and stores in fields 00008 */ 00009 class Config { 00010 public: 00011 Config(); 00012 00013 bool load(); 00014 00015 float interceptDist; // used for course correction steering calculation 00016 float waypointDist; // distance threshold to waypoint 00017 float brakeDist; // braking distance 00018 float declination; 00019 float compassGain; // probably don't need this anymore 00020 float yawGain; // probably don't need this anymore 00021 GeoPosition wpt[10]; // Waypoints, lat/lon coords 00022 CartPosition cwpt[10]; // Waypoints, cartesian coords 00023 unsigned int wptCount; // number of active waypoints 00024 int escMin; // minimum ESC value; brake 00025 int escZero; // zero throttle 00026 int escMax; // max throttle 00027 float topSpeed; // top speed to achieve on the straights 00028 float turnSpeed; // speed for turns 00029 float startSpeed; // speed for start 00030 float minRadius; // minimum turning radius (calculated) 00031 float speedKp; // Speed PID proportional gain 00032 float speedKi; // Speed PID integral gain 00033 float speedKd; // Speed PID derivative gain 00034 float steerZero; // zero steering aka center point 00035 float steerGain; // gain factor for steering algorithm 00036 float steerGainAngle; // angle below which steering gain takes effect 00037 float cteKi; // cross track error integral gain 00038 bool usePP; // use pure pursuit algorithm? 00039 float curbThreshold; // distance at which curb avoid takes place 00040 float curbGain; // gain of curb avoid steering 00041 // acceleration profile? 00042 // braking profile? 00043 float gyroBias; // this needs to be 3d 00044 // float gyroScale[3]; 00045 float magOffset[3]; 00046 float magScale[3]; 00047 // float accelOffset[3]; 00048 // float accelScale[3]; 00049 int gpsType; 00050 int gpsBaud; 00051 00052 }; 00053 00054 #endif
Generated on Tue Jul 12 2022 14:09:25 by
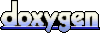