
Code for autonomous ground vehicle, Data Bus, 3rd place winner in 2012 Sparkfun AVC.
Dependencies: Watchdog mbed Schedule SimpleFilter LSM303DLM PinDetect DebounceIn Servo
GeoPosition Class Reference
Geographical position and calculation. More...
#include <GeoPosition.h>
Public Member Functions | |
GeoPosition () | |
Create a new emtpy position object. | |
GeoPosition (double latitude, double longitude) | |
Create a new position with the specified latitude and longitude. | |
double | latitude () |
Get the position's latitude. | |
double | longitude () |
Get the position's longitude. | |
void | set (GeoPosition pos) |
Set the position's location to another position's coordinates. | |
void | set (double latitude, double longitude) |
Set the position's location to the specified coordinates. | |
void | move (float course, float distance) |
Move the location of the position by the specified distance and in the specified direction. | |
float | bearing (GeoPosition from) |
Get the bearing from the specified origin position to this position. | |
float | distance (GeoPosition from) |
Get the distance from the specified origin position to this position. | |
void | setTimestamp (int time) |
Set an arbitrary timestamp on the position. | |
int | getTimestamp (void) |
Return a previously set timestamp on the position. |
Detailed Description
Geographical position and calculation.
Most of this comes from http://www.movable-type.co.uk/scripts/latlong.html
Definition at line 14 of file GeoPosition.h.
Constructor & Destructor Documentation
GeoPosition | ( | ) |
Create a new emtpy position object.
Definition at line 8 of file GeoPosition.cpp.
GeoPosition | ( | double | latitude, |
double | longitude | ||
) |
Create a new position with the specified latitude and longitude.
See set()
- Parameters:
-
latitude is the latitude to set longitude is the longitude to set
Definition at line 12 of file GeoPosition.cpp.
Member Function Documentation
float bearing | ( | GeoPosition | from ) |
Get the bearing from the specified origin position to this position.
To get relative bearing, subtract the result from your heading.
- Parameters:
-
from is the position from which to calculate bearing
- Returns:
- the bearing in degrees
Definition at line 88 of file GeoPosition.cpp.
float distance | ( | GeoPosition | from ) |
Get the distance from the specified origin position to this position.
- Parameters:
-
from is the position from which to calculate distance
- Returns:
- the distance in meters
Definition at line 138 of file GeoPosition.cpp.
int getTimestamp | ( | void | ) |
Return a previously set timestamp on the position.
- Returns:
- the timestamp (e.g., seconds, milliseconds, etc.)
Definition at line 191 of file GeoPosition.cpp.
double latitude | ( | ) |
Get the position's latitude.
- Returns:
- the position's latitude
Definition at line 26 of file GeoPosition.cpp.
double longitude | ( | ) |
Get the position's longitude.
- Returns:
- the position's longitude
Definition at line 31 of file GeoPosition.cpp.
void move | ( | float | course, |
float | distance | ||
) |
Move the location of the position by the specified distance and in the specified direction.
- Parameters:
-
course is the direction of movement in degrees, absolute not relative distance is the distance of movement along the specified course in meters
Definition at line 54 of file GeoPosition.cpp.
void set | ( | double | latitude, |
double | longitude | ||
) |
Set the position's location to the specified coordinates.
- Parameters:
-
latitude is the new latitude to set longitude is the new longitude to set
Definition at line 36 of file GeoPosition.cpp.
void set | ( | GeoPosition | pos ) |
Set the position's location to another position's coordinates.
- Parameters:
-
pos is another position from which coordinates will be copied
Definition at line 42 of file GeoPosition.cpp.
void setTimestamp | ( | int | time ) |
Set an arbitrary timestamp on the position.
- Parameters:
-
timestamp is an integer timestamp, eg., seconds, milliseconds, or whatever
Definition at line 187 of file GeoPosition.cpp.
Generated on Tue Jul 12 2022 14:09:28 by
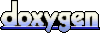