Fork of the official mbed C/C++ SDK provides the software platform and libraries to build your applications. The fork has the documentation converted to Doxygen format
Dependents: NervousPuppySprintOne NervousPuppySprint2602 Robot WarehouseBot1 ... more
Fork of mbed by
Ethernet Class Reference
An ethernet interface, to use with the ethernet pins. More...
#include <Ethernet.h>
Inherits mbed::Base.
Public Member Functions | |
Ethernet () | |
Initialise the ethernet interface. | |
virtual | ~Ethernet () |
Powers the hardware down. | |
int | write (const char *data, int size) |
Writes into an outgoing ethernet packet. | |
int | send () |
Send an outgoing ethernet packet. | |
int | receive () |
Recevies an arrived ethernet packet. | |
int | read (char *data, int size) |
Read from an recevied ethernet packet. | |
void | address (char *mac) |
Gives the ethernet address of the mbed. | |
int | link () |
Returns if an ethernet link is pressent or not. | |
void | set_link (Mode mode) |
Sets the speed and duplex parameters of an ethernet link. | |
void | register_object (const char *name) |
Registers this object with the given name, so that it can be looked up with lookup. | |
const char * | name () |
Returns the name of the object. | |
virtual bool | rpc (const char *method, const char *arguments, char *result) |
Call the given method with the given arguments, and write the result into the string pointed to by result. | |
virtual struct rpc_method * | get_rpc_methods () |
Returns a pointer to an array describing the rpc methods supported by this object, terminated by either RPC_METHOD_END or RPC_METHOD_SUPER(Superclass). | |
Static Public Member Functions | |
static bool | rpc (const char *name, const char *method, const char *arguments, char *result) |
Use the lookup function to lookup an object and, if successful, call its rpc method. | |
static Base * | lookup (const char *name, unsigned int len) |
Lookup and return the object that has the given name. | |
template<class C > | |
static void | add_rpc_class () |
Add the class to the list of classes which can have static methods called via rpc (the static methods which can be called are defined by that class' get_rpc_class() static method). |
Detailed Description
An ethernet interface, to use with the ethernet pins.
Example:
// Read destination and source from every ethernet packet #include "mbed.h" Ethernet eth; int main() { char buf[0x600]; while(1) { int size = eth.receive(); if(size > 0) { eth.read(buf, size); printf("Destination: %02X:%02X:%02X:%02X:%02X:%02X\n", buf[0], buf[1], buf[2], buf[3], buf[4], buf[5]); printf("Source: %02X:%02X:%02X:%02X:%02X:%02X\n", buf[6], buf[7], buf[8], buf[9], buf[10], buf[11]); } wait(1); } }
Definition at line 44 of file Ethernet.h.
Constructor & Destructor Documentation
Ethernet | ( | ) |
Initialise the ethernet interface.
virtual ~Ethernet | ( | ) | [virtual] |
Powers the hardware down.
Member Function Documentation
static void add_rpc_class | ( | ) | [static, inherited] |
void address | ( | char * | mac ) |
Gives the ethernet address of the mbed.
- Parameters:
-
mac Must be a pointer to a 6 byte char array to copy the ethernet address in.
virtual struct rpc_method* get_rpc_methods | ( | ) | [read, virtual, inherited] |
Returns a pointer to an array describing the rpc methods supported by this object, terminated by either RPC_METHOD_END or RPC_METHOD_SUPER(Superclass).
Example
class Example : public Base { int foo(int a, int b) { return a + b; } virtual const struct rpc_method *get_rpc_methods() { static const rpc_method rpc_methods[] = { { "foo", generic_caller<int, Example, int, int, &Example::foo> }, RPC_METHOD_SUPER(Base) }; return rpc_methods; } };
Reimplemented in AnalogIn, AnalogOut, BusIn, BusInOut, BusOut, DigitalIn, DigitalInOut, DigitalOut, PwmOut, Serial, SPI, and Timer.
int link | ( | ) |
Returns if an ethernet link is pressent or not.
It takes a wile after Ethernet initializion to show up.
- Returns:
- 0 if no ethernet link is pressent, 1 if an ethernet link is pressent.
Example:
// Using the Ethernet link function #include "mbed.h" Ethernet eth; int main() { wait(1); // Needed after startup. if (eth.link()) { printf("online\n"); } else { printf("offline\n"); } }
static Base* lookup | ( | const char * | name, |
unsigned int | len | ||
) | [static, inherited] |
Lookup and return the object that has the given name.
- Parameters:
-
name the name to lookup. len the length of name.
const char* name | ( | ) | [inherited] |
Returns the name of the object.
- Returns:
- The name of the object, or NULL if it has no name.
int read | ( | char * | data, |
int | size | ||
) |
Read from an recevied ethernet packet.
After receive returnd a number bigger than 0it is possible to read bytes from this packet. Read will write up to size bytes into data.
It is possible to use read multible times. Each time read will start reading after the last read byte before.
- Returns:
- The number of byte read.
int receive | ( | ) |
Recevies an arrived ethernet packet.
Receiving an ethernet packet will drop the last received ethernet packet and make a new ethernet packet ready to read. If no ethernet packet is arrived it will return 0.
- Returns:
- 0 if no ethernet packet is arrived, or the size of the arrived packet.
void register_object | ( | const char * | name ) | [inherited] |
Registers this object with the given name, so that it can be looked up with lookup.
If this object has already been registered, then this just changes the name.
- Parameters:
-
name The name to give the object. If NULL we do nothing.
virtual bool rpc | ( | const char * | method, |
const char * | arguments, | ||
char * | result | ||
) | [virtual, inherited] |
Call the given method with the given arguments, and write the result into the string pointed to by result.
The default implementation calls rpc_methods to determine the supported methods.
- Parameters:
-
method The name of the method to call. arguments A list of arguments separated by spaces. result A pointer to a string to write the result into. May be NULL, in which case nothing is written.
- Returns:
- true if method corresponds to a valid rpc method, or false otherwise.
static bool rpc | ( | const char * | name, |
const char * | method, | ||
const char * | arguments, | ||
char * | result | ||
) | [static, inherited] |
Use the lookup function to lookup an object and, if successful, call its rpc method.
- Returns:
- false if name does not correspond to an object, otherwise the return value of the call to the object's rpc method.
int send | ( | ) |
Send an outgoing ethernet packet.
After filling in the data in an ethernet packet it must be send. Send will provide a new packet to write to.
- Returns:
- 0 if the sending was failed, 1 if the package is successfully sent.
void set_link | ( | Mode | mode ) |
Sets the speed and duplex parameters of an ethernet link.
- AutoNegotiate Auto negotiate speed and duplex
- HalfDuplex10 10 Mbit, half duplex
- FullDuplex10 10 Mbit, full duplex
- HalfDuplex100 100 Mbit, half duplex
- FullDuplex100 100 Mbit, full duplex
- Parameters:
-
mode the speed and duplex mode to set the link to:
int write | ( | const char * | data, |
int | size | ||
) |
Writes into an outgoing ethernet packet.
It will append size bytes of data to the previously written bytes.
- Parameters:
-
data An array to write. size The size of data.
- Returns:
- The number of written bytes.
Generated on Tue Jul 12 2022 11:27:31 by
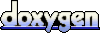