A library which allows the playing of Wav files using the TLV320
Dependents: RSALB_hbridge_helloworld RSALB_lobster WavPlayer_test AudioCODEC_HelloWorld
TLV320 Class Reference
A class to control the I2C part of the TLV320. More...
#include <TLV320.h>
Public Member Functions | |
TLV320 (PinName i2c_sda, PinName i2c_scl) | |
Create an instance of the TLV320 class. | |
TLV320 (PinName i2c_sda, PinName i2c_scl, bool cs_level) | |
Create an instance of the TLV320 class. | |
void | power (bool on_off) |
Control the power of the device. | |
void | input_select (int input) |
Control the input source of the device. | |
void | headphone_volume (float h_volume) |
Set the headphone volume. | |
void | linein_volume (float li_volume) |
Set the line in pre-amp volume. | |
void | microphone_boost (bool mic_boost) |
Turn on/off the microphone pre-amp boost. | |
void | input_mute (bool mute) |
Mute the input. | |
void | output_mute (bool mute) |
Mute the output. | |
void | input_power (bool on_off) |
Turn on/off the input stage. | |
void | output_power (bool on_off) |
Turn on/off the output stage. | |
void | wordsize (int words) |
Select the word size. | |
void | master (bool master) |
Select interface mode: Master or Slave. | |
void | frequency (int freq) |
Select the sample rate. | |
void | input_highpass (bool enabled) |
Enable the input highpass filter. | |
void | output_softmute (bool enabled) |
Enable the output soft mute. | |
void | interface_switch (bool on_off) |
Turn on and off the I2S. | |
void | reset () |
Reset the device and settings. | |
void | sidetone (float sidetone_vol) |
Set the microphone sidetone volume. | |
void | bypass (bool bypass_en) |
Set the analog bypass. | |
void | deemphasis (char code) |
Set the deemphasis frequency. | |
void | adc_highpass (bool enable) |
Enable the input highpass filter. | |
void | start () |
Start the device sending/recieving etc. | |
void | stop () |
Stop the device sending/recieving etc. |
Detailed Description
A class to control the I2C part of the TLV320.
Definition at line 60 of file TLV320.h.
Constructor & Destructor Documentation
TLV320 | ( | PinName | i2c_sda, |
PinName | i2c_scl | ||
) |
Create an instance of the TLV320 class.
- Parameters:
-
i2c_sda The SDA pin of the I2C i2c_scl The SCL pin of the I2C
Definition at line 15 of file TLV320.cpp.
TLV320 | ( | PinName | i2c_sda, |
PinName | i2c_scl, | ||
bool | cs_level | ||
) |
Create an instance of the TLV320 class.
- Parameters:
-
i2c_sda The SDA pin of the I2C i2c_scl The SCL pin of the I2C cs_level The level of the CS pin on the TLV320
Definition at line 21 of file TLV320.cpp.
Member Function Documentation
void adc_highpass | ( | bool | enable ) |
Enable the input highpass filter.
- Parameters:
-
enable Enable the input highpass filter enabled(true)
void bypass | ( | bool | bypass_en ) |
Set the analog bypass.
- Parameters:
-
bypass_en Enable the bypass: enabled(true)
Definition at line 182 of file TLV320.cpp.
void deemphasis | ( | char | code ) |
Set the deemphasis frequency.
- Parameters:
-
code The deemphasis code: TLV320_DE_EMPH_DISABLED, TLV320_DE_EMPH_32KHZ, TLV320_DE_EMPH_44KHZ, TLV320_DE_EMPH_48KHZ
Definition at line 169 of file TLV320.cpp.
void frequency | ( | int | freq ) |
Select the sample rate.
- Parameters:
-
freq Frequency: 96/48/32/8 kHz
Definition at line 143 of file TLV320.cpp.
void headphone_volume | ( | float | h_volume ) |
Set the headphone volume.
- Parameters:
-
h_volume The desired headphone volume: 0->1
Definition at line 70 of file TLV320.cpp.
void input_highpass | ( | bool | enabled ) |
Enable the input highpass filter.
- Parameters:
-
enabled Input highpass filter enabled
Definition at line 149 of file TLV320.cpp.
void input_mute | ( | bool | mute ) |
void input_power | ( | bool | on_off ) |
Turn on/off the input stage.
- Parameters:
-
on_off Input stage on(true)/off(false)
Definition at line 108 of file TLV320.cpp.
void input_select | ( | int | input ) |
Control the input source of the device.
- Parameters:
-
input Select the source of the input of the device: TLV320_LINE, TLV320_MIC, TLV320_NO_IN
Definition at line 32 of file TLV320.cpp.
void interface_switch | ( | bool | on_off ) |
Turn on and off the I2S.
- Parameters:
-
on_off Switch the I2S interface on(true)/off(false)
Definition at line 159 of file TLV320.cpp.
void linein_volume | ( | float | li_volume ) |
Set the line in pre-amp volume.
- Parameters:
-
li_volume The desired line in volume: 0->1
Definition at line 77 of file TLV320.cpp.
void master | ( | bool | master ) |
Select interface mode: Master or Slave.
- Parameters:
-
master Interface mode: master(true)/slave
Definition at line 138 of file TLV320.cpp.
void microphone_boost | ( | bool | mic_boost ) |
Turn on/off the microphone pre-amp boost.
- Parameters:
-
mic_boost Boost on or off
Definition at line 84 of file TLV320.cpp.
void output_mute | ( | bool | mute ) |
void output_power | ( | bool | on_off ) |
Turn on/off the output stage.
- Parameters:
-
on_off Output stage on(true)/off(false)
Definition at line 126 of file TLV320.cpp.
void output_softmute | ( | bool | enabled ) |
Enable the output soft mute.
- Parameters:
-
enabled Output soft mute enabled
Definition at line 154 of file TLV320.cpp.
void power | ( | bool | on_off ) |
Control the power of the device.
- Parameters:
-
on_off The power state
Definition at line 27 of file TLV320.cpp.
void reset | ( | ) |
Reset the device and settings.
Definition at line 174 of file TLV320.cpp.
void sidetone | ( | float | sidetone_vol ) |
Set the microphone sidetone volume.
- Parameters:
-
sidetone_volume The volume of the sidetone: 0->1
Definition at line 164 of file TLV320.cpp.
void start | ( | ) |
Start the device sending/recieving etc.
Definition at line 178 of file TLV320.cpp.
void stop | ( | ) |
Stop the device sending/recieving etc.
Definition at line 187 of file TLV320.cpp.
void wordsize | ( | int | words ) |
Generated on Tue Jul 12 2022 17:42:18 by
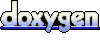