A library which allows the playing of Wav files using the TLV320
Dependents: RSALB_hbridge_helloworld RSALB_lobster WavPlayer_test AudioCODEC_HelloWorld
TLV320.cpp
00001 #include "TLV320.h" 00002 00003 #define TLV320_HP_VOL_DF_MASK 0x80 00004 00005 00006 #define TLV320_DF_hp_vol_left 0.5 00007 #define TLV320_DF_hp_vol_right 0.5 00008 #define TLV320_DF_li_vol_left 0.5 00009 #define TLV320_DF_li_vol_right 0.5 00010 #define TLV320_DF_sdt_vol 0 00011 00012 const uint8_t base_address = 0x1A; 00013 00014 00015 TLV320::TLV320(PinName i2c_sda, PinName i2c_scl): i2c(i2c_sda,i2c_scl) { 00016 address = base_address; 00017 defaulter(); 00018 form_cmd(all); 00019 } 00020 00021 TLV320::TLV320(PinName i2c_sda, PinName i2c_scl, bool cs_level): i2c(i2c_sda,i2c_scl) { 00022 address = base_address + (1*cs_level); 00023 defaulter(); 00024 form_cmd(all); 00025 } 00026 00027 void TLV320::power(bool on_off) { 00028 device_all_pwr = on_off; 00029 form_cmd(power_control); 00030 } 00031 00032 void TLV320::input_select(int input) { 00033 00034 switch(input) 00035 { 00036 case TLV320_NO_IN: 00037 device_adc_pwr = false; 00038 device_mic_pwr = false; 00039 device_lni_pwr = false; 00040 form_cmd(power_control); 00041 break; 00042 case TLV320_LINE: 00043 device_adc_pwr = true; 00044 device_lni_pwr = true; 00045 device_mic_pwr = false; 00046 ADC_source = TLV320_LINE; 00047 form_cmd(power_control); 00048 form_cmd(path_analog); 00049 break; 00050 case TLV320_MIC: 00051 device_adc_pwr = true; 00052 device_lni_pwr = false; 00053 device_mic_pwr = true; 00054 ADC_source = TLV320_MIC; 00055 form_cmd(power_control); 00056 form_cmd(path_analog); 00057 break; 00058 default: 00059 device_adc_pwr = df_device_adc_pwr; 00060 device_mic_pwr = df_device_mic_pwr; 00061 device_lni_pwr = df_device_lni_pwr; 00062 ADC_source = df_ADC_source; 00063 form_cmd(power_control); 00064 form_cmd(path_analog); 00065 break; 00066 } 00067 ADC_source_old = ADC_source; 00068 } 00069 00070 void TLV320::headphone_volume(float h_volume) { 00071 hp_vol_left = h_volume; 00072 hp_vol_right = h_volume; 00073 form_cmd(headphone_vol_left); 00074 form_cmd(headphone_vol_right); 00075 } 00076 00077 void TLV320::linein_volume(float li_volume) { 00078 li_vol_left = li_volume; 00079 li_vol_right = li_volume; 00080 form_cmd(line_in_vol_left); 00081 form_cmd(line_in_vol_right); 00082 } 00083 00084 void TLV320::microphone_boost(bool mic_boost) { 00085 mic_boost_ = mic_boost; 00086 } 00087 00088 void TLV320::input_mute(bool mute) { 00089 if(ADC_source == TLV320_MIC) 00090 { 00091 mic_mute = mute; 00092 form_cmd(path_analog); 00093 } 00094 else 00095 { 00096 li_mute_left = mute; 00097 li_mute_right = mute; 00098 form_cmd(line_in_vol_left); 00099 form_cmd(line_in_vol_right); 00100 } 00101 } 00102 00103 void TLV320::output_mute(bool mute) { 00104 out_mute = mute; 00105 form_cmd(path_digital); 00106 } 00107 00108 void TLV320::input_power(bool on_off) { 00109 00110 device_adc_pwr = on_off; 00111 00112 if(ADC_source == TLV320_MIC) 00113 { 00114 device_mic_pwr = on_off; 00115 device_lni_pwr = false; 00116 } 00117 else 00118 { 00119 device_mic_pwr = false; 00120 device_lni_pwr = on_off; 00121 } 00122 00123 form_cmd(power_control); 00124 } 00125 00126 void TLV320::output_power(bool on_off) { 00127 device_dac_pwr = on_off; 00128 device_out_pwr = on_off; 00129 00130 form_cmd(power_control); 00131 } 00132 00133 void TLV320::wordsize(int words) { 00134 device_bitlength = words; 00135 form_cmd(interface_format); 00136 } 00137 00138 void TLV320::master(bool master) { 00139 device_master = master; 00140 form_cmd(interface_format); 00141 } 00142 00143 void TLV320::frequency(int freq) { 00144 ADC_rate = freq; 00145 DAC_rate = freq; 00146 form_cmd(sample_rate); 00147 } 00148 00149 void TLV320::input_highpass(bool enabled) { 00150 ADC_highpass_enable = enabled; 00151 form_cmd(path_digital); 00152 } 00153 00154 void TLV320::output_softmute(bool enabled) { 00155 out_mute = enabled; 00156 form_cmd(path_digital); 00157 } 00158 00159 void TLV320::interface_switch(bool on_off) { 00160 device_interface_active = on_off; 00161 form_cmd(interface_activation); 00162 } 00163 00164 void TLV320::sidetone(float sidetone_vol) { 00165 sdt_vol = sidetone_vol; 00166 form_cmd(path_analog); 00167 } 00168 00169 void TLV320::deemphasis(char code) { 00170 de_emph_code = code & 0x03; 00171 form_cmd(path_digital); 00172 } 00173 00174 void TLV320::reset() { 00175 form_cmd(reset_reg); 00176 } 00177 00178 void TLV320::start() { 00179 interface_switch(true); 00180 } 00181 00182 void TLV320::bypass(bool enable) { 00183 bypass_ = enable; 00184 form_cmd(path_analog); 00185 } 00186 00187 void TLV320::stop() { 00188 interface_switch(false); 00189 } 00190 00191 void TLV320::command(reg_address add, uint16_t cmd) { 00192 char temp[2]; 00193 temp[0] = (char(add)<<1) | ((cmd >> 6) & 0x01); 00194 temp[1] = (cmd & 0xFF); 00195 i2c.write((address<<1), temp, 2); 00196 } 00197 00198 void TLV320::form_cmd(reg_address add) { 00199 uint16_t cmd = 0; 00200 int temp = 0; 00201 bool mute; 00202 switch(add) 00203 { 00204 case line_in_vol_left: 00205 temp = int(li_vol_left * 32) - 1; 00206 mute = li_mute_left; 00207 00208 if(temp < 0) 00209 { 00210 temp = 0; 00211 mute = true; 00212 } 00213 cmd = temp & 0x1F; 00214 cmd |= mute << 7; 00215 break; 00216 case line_in_vol_right: 00217 temp = int(li_vol_right * 32) - 1; 00218 mute = li_mute_right; 00219 if(temp < 0) 00220 { 00221 temp = 0; 00222 mute = true; 00223 } 00224 cmd = temp & 0x1F; 00225 cmd |= mute << 7; 00226 break; 00227 00228 case headphone_vol_left: 00229 temp = int(hp_vol_left * 80) + 47; 00230 cmd = TLV320_HP_VOL_DF_MASK; 00231 cmd |= temp & 0x7F; 00232 break; 00233 case headphone_vol_right: 00234 temp = int(hp_vol_right * 80) + 47; 00235 cmd = TLV320_HP_VOL_DF_MASK; 00236 cmd |= temp & 0x7F; 00237 break; 00238 00239 case path_analog: 00240 temp = int(sdt_vol * 5); 00241 char vol_code = 0; 00242 switch(temp) 00243 { 00244 case 5: 00245 vol_code = 0x0C; 00246 break; 00247 case 0: 00248 vol_code = 0x00; 00249 break; 00250 default: 00251 vol_code = ((0x04 - temp)&0x07) | 0x08; 00252 break; 00253 } 00254 cmd = vol_code << 5; 00255 cmd |= 1 << 4; 00256 cmd |= bypass_ << 3; 00257 cmd |= ADC_source << 2; 00258 cmd |= mic_mute << 1; 00259 cmd |= mic_boost_; 00260 break; 00261 00262 case path_digital: 00263 cmd |= out_mute << 3; 00264 cmd |= ((de_emph_code & 0x3) << 1); 00265 cmd |= ADC_highpass_enable; 00266 break; 00267 00268 case power_control: 00269 cmd |= !device_all_pwr << 7; 00270 cmd |= !device_clk_pwr << 6; 00271 cmd |= !device_osc_pwr << 5; 00272 cmd |= !device_out_pwr << 4; 00273 cmd |= !device_dac_pwr << 3; 00274 cmd |= !device_adc_pwr << 2; 00275 cmd |= !device_mic_pwr << 1; 00276 cmd |= !device_lni_pwr << 0; 00277 break; 00278 00279 case interface_format: 00280 cmd |= device_master << 6; 00281 cmd |= device_lrswap << 5; 00282 cmd |= device_lrws << 4; 00283 temp = 0; 00284 switch(device_bitlength) 00285 { 00286 case 16: 00287 temp = 0; 00288 break; 00289 case 20: 00290 temp = 1; 00291 break; 00292 case 24: 00293 temp = 2; 00294 break; 00295 case 32: 00296 temp = 3; 00297 break; 00298 } 00299 cmd |= (temp & 0x03) << 2; 00300 cmd |= (device_data_form & 0x03); 00301 break; 00302 00303 case sample_rate: 00304 temp = gen_samplerate(); 00305 cmd = device_usb_mode; 00306 cmd |= (temp & 0x03) << 1; 00307 cmd |= device_clk_in_div << 6; 00308 cmd |= device_clk_out_div << 7; 00309 break; 00310 00311 case interface_activation: 00312 cmd = device_interface_active; 00313 break; 00314 00315 case reset_reg: 00316 cmd = 0; 00317 break; 00318 00319 case all: 00320 for( int i = line_in_vol_left; i <= reset_reg; i++) 00321 { 00322 form_cmd((reg_address)i); 00323 } 00324 break; 00325 } 00326 if(add != all) command(add , cmd); 00327 } 00328 00329 void TLV320::defaulter() { 00330 hp_vol_left = TLV320_DF_hp_vol_left; 00331 hp_vol_right = TLV320_DF_hp_vol_right; 00332 li_vol_left = TLV320_DF_li_vol_left; 00333 li_vol_right = TLV320_DF_li_vol_right; 00334 sdt_vol = TLV320_DF_sdt_vol; 00335 bypass_ = df_bypass_; 00336 00337 ADC_source = df_ADC_source; 00338 ADC_source_old = df_ADC_source; 00339 00340 mic_mute = df_mic_mute; 00341 li_mute_left = df_li_mute_left; 00342 li_mute_right = df_li_mute_right; 00343 00344 00345 mic_boost_ = df_mic_boost_; 00346 out_mute = df_out_mute; 00347 de_emph_code = df_de_emph_code; 00348 ADC_highpass_enable = df_ADC_highpass_enable; 00349 00350 device_all_pwr = df_device_all_pwr; 00351 device_clk_pwr = df_device_clk_pwr; 00352 device_osc_pwr = df_device_osc_pwr; 00353 device_out_pwr = df_device_out_pwr; 00354 device_dac_pwr = df_device_dac_pwr; 00355 device_adc_pwr = df_device_dac_pwr; 00356 device_mic_pwr = df_device_mic_pwr; 00357 device_lni_pwr = df_device_lni_pwr; 00358 00359 device_master = df_device_master; 00360 device_lrswap = df_device_lrswap; 00361 device_lrws = df_device_lrws; 00362 device_bitlength = df_device_bitlength; 00363 00364 00365 ADC_rate = df_ADC_rate; 00366 DAC_rate = df_DAC_rate; 00367 00368 device_interface_active = df_device_interface_active; 00369 } 00370 00371 char TLV320::gen_samplerate() { 00372 char temp = 0; 00373 switch(ADC_rate) 00374 { 00375 case 96000: 00376 temp = 0x0E; 00377 break; 00378 case 48000: 00379 temp = 0x00; 00380 if(DAC_rate == 8000) temp = 0x02; 00381 break; 00382 case 32000: 00383 temp = 0x0C; 00384 break; 00385 case 8000: 00386 temp = 0x03; 00387 if(DAC_rate == 48000) temp = 0x04; 00388 break; 00389 default: 00390 temp = 0x00; 00391 break; 00392 } 00393 return temp; 00394 } 00395 00396
Generated on Tue Jul 12 2022 17:42:18 by
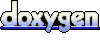