
Rtos API example
Embed:
(wiki syntax)
Show/hide line numbers
SafeBool.h
Go to the documentation of this file.
00001 /* mbed Microcontroller Library 00002 * Copyright (c) 2006-2013 ARM Limited 00003 * 00004 * Licensed under the Apache License, Version 2.0 (the "License"); 00005 * you may not use this file except in compliance with the License. 00006 * You may obtain a copy of the License at 00007 * 00008 * http://www.apache.org/licenses/LICENSE-2.0 00009 * 00010 * Unless required by applicable law or agreed to in writing, software 00011 * distributed under the License is distributed on an "AS IS" BASIS, 00012 * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 00013 * See the License for the specific language governing permissions and 00014 * limitations under the License. 00015 */ 00016 00017 #ifndef BLE_API_SAFE_BOOL_H_ 00018 #define BLE_API_SAFE_BOOL_H_ 00019 00020 /* Safe bool idiom, see: http://www.artima.com/cppsource/safebool.html */ 00021 00022 /** 00023 * @file 00024 * @addtogroup ble 00025 * @{ 00026 * @addtogroup common 00027 * @{ 00028 */ 00029 00030 /** 00031 * Private namespace used to host details of the SafeBool implementation. 00032 */ 00033 namespace SafeBool_ { 00034 /** 00035 * Base class of all SafeBool instances. 00036 * 00037 * This nontemplate base class exists to reduce the number of instantiation of 00038 * the trueTag function. 00039 */ 00040 class base { 00041 template<typename> 00042 friend class SafeBool; 00043 00044 protected: 00045 /** 00046 * The bool type is a pointer to method that can be used in boolean context. 00047 */ 00048 typedef void (base::*BoolType_t)() const; 00049 00050 /** 00051 * Nonimplemented call, use to disallow conversion between unrelated types. 00052 */ 00053 void invalidTag() const; 00054 00055 /** 00056 * Special member function that indicates a true value. 00057 */ 00058 void trueTag() const {} 00059 }; 00060 00061 00062 } 00063 00064 /** 00065 * Safe conversion of objects in boolean context. 00066 * 00067 * Classes wanting evaluation of their instances in boolean context must derive 00068 * publicly from this class rather than implementing the easy to misuse 00069 * operator bool(). 00070 * 00071 * Descendant classes must implement the function bool toBool() const to enable 00072 * the safe conversion in boolean context. 00073 * 00074 * @tparam T Type of the derived class 00075 * 00076 * @code 00077 * 00078 * class A : public SafeBool<A> { 00079 * public: 00080 * 00081 * // boolean conversion 00082 * bool toBool() const { 00083 * 00084 * } 00085 * }; 00086 * 00087 * class B : public SafeBool<B> { 00088 * public: 00089 * 00090 * // boolean conversion 00091 * bool toBool() const { 00092 * 00093 * } 00094 * }; 00095 * 00096 * A a; 00097 * B b; 00098 * 00099 * // will compile 00100 * if(a) { 00101 * 00102 * } 00103 * 00104 * // compilation error 00105 * if(a == b) { 00106 * 00107 * } 00108 * @endcode 00109 */ 00110 template <typename T> 00111 class SafeBool : public SafeBool_::base { 00112 public: 00113 /** 00114 * Bool operator implementation, derived class must provide a bool 00115 * toBool() const function. 00116 */ 00117 operator BoolType_t() const 00118 { 00119 return (static_cast<const T*>(this))->toBool() 00120 ? &SafeBool<T>::trueTag : 0; 00121 } 00122 }; 00123 00124 /** 00125 * Avoid conversion to bool between different classes. 00126 * 00127 * @important Will generate a compile time error if instantiated. 00128 */ 00129 template <typename T, typename U> 00130 void operator==(const SafeBool<T>& lhs,const SafeBool<U>& rhs) 00131 { 00132 lhs.invalidTag(); 00133 // return false; 00134 } 00135 00136 /** 00137 * Avoid conversion to bool between different classes. 00138 * 00139 * @important Will generate a compile time error if instantiated. 00140 */ 00141 template <typename T,typename U> 00142 void operator!=(const SafeBool<T>& lhs,const SafeBool<U>& rhs) 00143 { 00144 lhs.invalidTag(); 00145 // return false; 00146 } 00147 00148 /** 00149 * @} 00150 * @} 00151 */ 00152 00153 00154 #endif /* BLE_API_SAFE_BOOL_H_ */
Generated on Sun Jul 17 2022 08:25:29 by
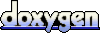