
Rtos API example
Dir Class Reference
[Filesystem]
#include <Dir.h>
Inherits mbed::DirHandle.
Public Member Functions | |
Dir () | |
Create an uninitialized directory. | |
Dir (FileSystem *fs, const char *path) | |
Open a directory on a filesystem. | |
virtual | ~Dir () |
Destroy a file. | |
virtual int | open (FileSystem *fs, const char *path) |
Open a directory on the filesystem. | |
virtual int | close () |
Close a directory. | |
virtual ssize_t | read (struct dirent *ent) |
Read the next directory entry. | |
virtual void | seek (off_t offset) |
Set the current position of the directory. | |
virtual off_t | tell () |
Get the current position of the directory. | |
virtual void | rewind () |
Rewind the current position to the beginning of the directory. | |
virtual size_t | size () |
Get the sizeof the directory. | |
MBED_DEPRECATED_SINCE ("mbed-os-5.4","Replaced by DirHandle::close") virtual int closedir() | |
Closes the directory. | |
MBED_DEPRECATED_SINCE ("mbed-os-5.4","Replaced by DirHandle::read") virtual struct dirent *readdir() | |
Return the directory entry at the current position, and advances the position to the next entry. | |
MBED_DEPRECATED_SINCE ("mbed-os-5.4","Replaced by DirHandle::rewind") virtual void rewinddir() | |
Resets the position to the beginning of the directory. | |
MBED_DEPRECATED_SINCE ("mbed-os-5.4","Replaced by DirHandle::tell") virtual off_t telldir() | |
Returns the current position of the DirHandle. | |
MBED_DEPRECATED_SINCE ("mbed-os-5.4","Replaced by DirHandle::seek") virtual void seekdir(off_t location) | |
Sets the position of the DirHandle. |
Detailed Description
Dir class.
Definition at line 30 of file Dir.h.
Constructor & Destructor Documentation
Dir | ( | ) |
Create an uninitialized directory.
Must call open to initialize the directory on a file system
Dir | ( | FileSystem * | fs, |
const char * | path | ||
) |
Open a directory on a filesystem.
- Parameters:
-
fs Filesystem as target for a directory path Name of the directory to open
virtual ~Dir | ( | ) | [virtual] |
Destroy a file.
Closes file if the file is still open
Member Function Documentation
virtual int close | ( | ) | [virtual] |
MBED_DEPRECATED_SINCE | ( | "mbed-os-5.4" | , |
"Replaced by DirHandle::close" | |||
) | [inherited] |
Closes the directory.
- Returns:
- 0 on success, -1 on error.
Definition at line 109 of file DirHandle.h.
MBED_DEPRECATED_SINCE | ( | "mbed-os-5.4" | , |
"Replaced by DirHandle::tell" | |||
) | [inherited] |
Returns the current position of the DirHandle.
- Returns:
- the current position, -1 on error.
Definition at line 138 of file DirHandle.h.
MBED_DEPRECATED_SINCE | ( | "mbed-os-5.4" | , |
"Replaced by DirHandle::read" | |||
) | [inherited] |
Return the directory entry at the current position, and advances the position to the next entry.
- Returns:
- A pointer to a dirent structure representing the directory entry at the current position, or NULL on reaching end of directory or error.
Definition at line 120 of file DirHandle.h.
MBED_DEPRECATED_SINCE | ( | "mbed-os-5.4" | , |
"Replaced by DirHandle::rewind" | |||
) | [inherited] |
Resets the position to the beginning of the directory.
Definition at line 129 of file DirHandle.h.
MBED_DEPRECATED_SINCE | ( | "mbed-os-5.4" | , |
"Replaced by DirHandle::seek" | |||
) | [inherited] |
Sets the position of the DirHandle.
- Parameters:
-
location The location to seek to. Must be a value returned by telldir.
Definition at line 145 of file DirHandle.h.
virtual int open | ( | FileSystem * | fs, |
const char * | path | ||
) | [virtual] |
Open a directory on the filesystem.
- Parameters:
-
fs Filesystem as target for a directory path Name of the directory to open
- Returns:
- 0 on success, negative error code on failure
virtual ssize_t read | ( | struct dirent * | ent ) | [virtual] |
Read the next directory entry.
- Parameters:
-
ent The directory entry to fill out
- Returns:
- 1 on reading a filename, 0 at end of directory, negative error on failure
Implements DirHandle.
virtual void rewind | ( | ) | [virtual] |
Rewind the current position to the beginning of the directory.
Implements DirHandle.
virtual void seek | ( | off_t | offset ) | [virtual] |
Set the current position of the directory.
- Parameters:
-
offset Offset of the location to seek to, must be a value returned from tell
Implements DirHandle.
virtual size_t size | ( | ) | [virtual] |
Get the sizeof the directory.
- Returns:
- Number of files in the directory
Reimplemented from DirHandle.
virtual off_t tell | ( | ) | [virtual] |
Get the current position of the directory.
- Returns:
- Position of the directory that can be passed to rewind
Implements DirHandle.
Generated on Sun Jul 17 2022 08:25:43 by
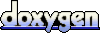