
Rtos API example
Embed:
(wiki syntax)
Show/hide line numbers
Dir.h
00001 /* mbed Microcontroller Library 00002 * Copyright (c) 2015 ARM Limited 00003 * 00004 * Licensed under the Apache License, Version 2.0 (the "License"); 00005 * you may not use this file except in compliance with the License. 00006 * You may obtain a copy of the License at 00007 * 00008 * http://www.apache.org/licenses/LICENSE-2.0 00009 * 00010 * Unless required by applicable law or agreed to in writing, software 00011 * distributed under the License is distributed on an "AS IS" BASIS, 00012 * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 00013 * See the License for the specific language governing permissions and 00014 * limitations under the License. 00015 */ 00016 00017 #ifndef DIR_H 00018 #define DIR_H 00019 00020 #include "filesystem/FileSystem.h" 00021 #include "platform/DirHandle.h" 00022 00023 namespace mbed { 00024 /** \addtogroup filesystem */ 00025 /** @{*/ 00026 00027 00028 /** Dir class 00029 */ 00030 class Dir : public DirHandle { 00031 public: 00032 /** Create an uninitialized directory 00033 * 00034 * Must call open to initialize the directory on a file system 00035 */ 00036 Dir(); 00037 00038 /** Open a directory on a filesystem 00039 * 00040 * @param fs Filesystem as target for a directory 00041 * @param path Name of the directory to open 00042 */ 00043 Dir(FileSystem *fs, const char *path); 00044 00045 /** Destroy a file 00046 * 00047 * Closes file if the file is still open 00048 */ 00049 virtual ~Dir(); 00050 00051 /** Open a directory on the filesystem 00052 * 00053 * @param fs Filesystem as target for a directory 00054 * @param path Name of the directory to open 00055 * @return 0 on success, negative error code on failure 00056 */ 00057 virtual int open(FileSystem *fs, const char *path); 00058 00059 /** Close a directory 00060 * 00061 * @return 0 on success, negative error code on failure 00062 */ 00063 virtual int close(); 00064 00065 /** Read the next directory entry 00066 * 00067 * @param ent The directory entry to fill out 00068 * @return 1 on reading a filename, 0 at end of directory, negative error on failure 00069 */ 00070 virtual ssize_t read(struct dirent *ent); 00071 00072 /** Set the current position of the directory 00073 * 00074 * @param offset Offset of the location to seek to, 00075 * must be a value returned from tell 00076 */ 00077 virtual void seek(off_t offset); 00078 00079 /** Get the current position of the directory 00080 * 00081 * @return Position of the directory that can be passed to rewind 00082 */ 00083 virtual off_t tell(); 00084 00085 /** Rewind the current position to the beginning of the directory 00086 */ 00087 virtual void rewind(); 00088 00089 /** Get the sizeof the directory 00090 * 00091 * @return Number of files in the directory 00092 */ 00093 virtual size_t size(); 00094 00095 private: 00096 FileSystem *_fs; 00097 fs_dir_t _dir; 00098 }; 00099 00100 00101 /** @}*/ 00102 } // namespace mbed 00103 00104 #endif
Generated on Sun Jul 17 2022 08:25:21 by
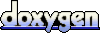