
Rtos API example
Embed:
(wiki syntax)
Show/hide line numbers
DirHandle.h
00001 /* mbed Microcontroller Library 00002 * Copyright (c) 2006-2013 ARM Limited 00003 * 00004 * Licensed under the Apache License, Version 2.0 (the "License"); 00005 * you may not use this file except in compliance with the License. 00006 * You may obtain a copy of the License at 00007 * 00008 * http://www.apache.org/licenses/LICENSE-2.0 00009 * 00010 * Unless required by applicable law or agreed to in writing, software 00011 * distributed under the License is distributed on an "AS IS" BASIS, 00012 * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 00013 * See the License for the specific language governing permissions and 00014 * limitations under the License. 00015 */ 00016 #ifndef MBED_DIRHANDLE_H 00017 #define MBED_DIRHANDLE_H 00018 00019 #include <stdint.h> 00020 #include "platform/platform.h" 00021 #include "platform/FileHandle.h" 00022 #include "platform/NonCopyable.h" 00023 00024 namespace mbed { 00025 /** \addtogroup platform */ 00026 /** @{*/ 00027 /** 00028 * \defgroup platform_DirHandle DirHandle functions 00029 * @{ 00030 */ 00031 00032 00033 /** Represents a directory stream. Objects of this type are returned 00034 * by an opendir function. The core functions are read and seek, 00035 * but only a subset needs to be provided. 00036 * 00037 * If a FileSystemLike class defines the opendir method, then the 00038 * directories of an object of that type can be accessed by 00039 * DIR *d = opendir("/example/directory") (or opendir("/example") 00040 * to open the root of the filesystem), and then using readdir(d) etc. 00041 * 00042 * The root directory is considered to contain all FileHandle and 00043 * FileSystem objects, so the DIR* returned by opendir("/") will 00044 * reflect this. 00045 * 00046 * @note to create a directory, @see Dir 00047 * @note Synchronization level: Set by subclass 00048 */ 00049 class DirHandle : private NonCopyable<DirHandle> { 00050 public: 00051 virtual ~DirHandle() {} 00052 00053 /** Read the next directory entry 00054 * 00055 * @param ent The directory entry to fill out 00056 * @return 1 on reading a filename, 0 at end of directory, negative error on failure 00057 */ 00058 virtual ssize_t read(struct dirent *ent) = 0; 00059 00060 /** Close a directory 00061 * 00062 * @return 0 on success, negative error code on failure 00063 */ 00064 virtual int close() = 0; 00065 00066 /** Set the current position of the directory 00067 * 00068 * @param offset Offset of the location to seek to, 00069 * must be a value returned from tell 00070 */ 00071 virtual void seek(off_t offset) = 0; 00072 00073 /** Get the current position of the directory 00074 * 00075 * @return Position of the directory that can be passed to rewind 00076 */ 00077 virtual off_t tell() = 0; 00078 00079 /** Rewind the current position to the beginning of the directory 00080 */ 00081 virtual void rewind() = 0; 00082 00083 /** Get the sizeof the directory 00084 * 00085 * @return Number of files in the directory 00086 */ 00087 virtual size_t size() 00088 { 00089 off_t off = tell(); 00090 size_t size = 0; 00091 struct dirent *ent = new struct dirent; 00092 00093 rewind(); 00094 while (read(ent) > 0) { 00095 size += 1; 00096 } 00097 seek(off); 00098 00099 delete ent; 00100 return size; 00101 } 00102 00103 /** Closes the directory. 00104 * 00105 * @returns 00106 * 0 on success, 00107 * -1 on error. 00108 */ 00109 MBED_DEPRECATED_SINCE("mbed-os-5.4", "Replaced by DirHandle::close") 00110 virtual int closedir() { return close(); }; 00111 00112 /** Return the directory entry at the current position, and 00113 * advances the position to the next entry. 00114 * 00115 * @returns 00116 * A pointer to a dirent structure representing the 00117 * directory entry at the current position, or NULL on reaching 00118 * end of directory or error. 00119 */ 00120 MBED_DEPRECATED_SINCE("mbed-os-5.4", "Replaced by DirHandle::read") 00121 virtual struct dirent *readdir() 00122 { 00123 static struct dirent ent; 00124 return (read(&ent) > 0) ? &ent : NULL; 00125 } 00126 00127 /** Resets the position to the beginning of the directory. 00128 */ 00129 MBED_DEPRECATED_SINCE("mbed-os-5.4", "Replaced by DirHandle::rewind") 00130 virtual void rewinddir() { rewind(); } 00131 00132 /** Returns the current position of the DirHandle. 00133 * 00134 * @returns 00135 * the current position, 00136 * -1 on error. 00137 */ 00138 MBED_DEPRECATED_SINCE("mbed-os-5.4", "Replaced by DirHandle::tell") 00139 virtual off_t telldir() { return tell(); } 00140 00141 /** Sets the position of the DirHandle. 00142 * 00143 * @param location The location to seek to. Must be a value returned by telldir. 00144 */ 00145 MBED_DEPRECATED_SINCE("mbed-os-5.4", "Replaced by DirHandle::seek") 00146 virtual void seekdir(off_t location) { seek(location); } 00147 }; 00148 00149 /**@}*/ 00150 00151 /**@}*/ 00152 } // namespace mbed 00153 00154 #endif /* MBED_DIRHANDLE_H */
Generated on Sun Jul 17 2022 08:25:21 by
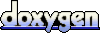