
Rtos API example
FATFileSystem Class Reference
FATFileSystem based on ChaN's Fat Filesystem library v0.8. More...
#include <FATFileSystem.h>
Inherits mbed::FileSystem.
Public Member Functions | |
FATFileSystem (const char *name=NULL, BlockDevice *bd=NULL) | |
Lifetime of the FATFileSystem. | |
virtual int | mount (BlockDevice *bd) |
Mounts a filesystem to a block device. | |
virtual int | unmount () |
Unmounts a filesystem from the underlying block device. | |
virtual int | reformat (BlockDevice *bd, int allocation_unit) |
Reformats a filesystem, results in an empty and mounted filesystem. | |
virtual int | reformat (BlockDevice *bd=NULL) |
Reformats a filesystem, results in an empty and mounted filesystem. | |
virtual int | remove (const char *path) |
Remove a file from the filesystem. | |
virtual int | rename (const char *path, const char *newpath) |
Rename a file in the filesystem. | |
virtual int | stat (const char *path, struct stat *st) |
Store information about the file in a stat structure. | |
virtual int | mkdir (const char *path, mode_t mode) |
Create a directory in the filesystem. | |
MBED_DEPRECATED_SINCE ("mbed-os-5.5","Replaced by `int open(FileHandle **, ...)` for propagating error codes") FileHandle *open(const char *path | |
Open a file on the filesystem. | |
Static Public Member Functions | |
static int | format (BlockDevice *bd, bd_size_t cluster_size=0) |
Formats a logical drive, FDISK partitioning rule. | |
Protected Member Functions | |
virtual int | file_open (fs_file_t *file, const char *path, int flags) |
Open a file on the filesystem. | |
virtual int | file_close (fs_file_t file) |
Close a file. | |
virtual ssize_t | file_read (fs_file_t file, void *buffer, size_t len) |
Read the contents of a file into a buffer. | |
virtual ssize_t | file_write (fs_file_t file, const void *buffer, size_t len) |
Write the contents of a buffer to a file. | |
virtual int | file_sync (fs_file_t file) |
Flush any buffers associated with the file. | |
virtual off_t | file_seek (fs_file_t file, off_t offset, int whence) |
Move the file position to a given offset from from a given location. | |
virtual off_t | file_tell (fs_file_t file) |
Get the file position of the file. | |
virtual off_t | file_size (fs_file_t file) |
Get the size of the file. | |
virtual int | dir_open (fs_dir_t *dir, const char *path) |
Open a directory on the filesystem. | |
virtual int | dir_close (fs_dir_t dir) |
Close a directory. | |
virtual ssize_t | dir_read (fs_dir_t dir, struct dirent *ent) |
Read the next directory entry. | |
virtual void | dir_seek (fs_dir_t dir, off_t offset) |
Set the current position of the directory. | |
virtual off_t | dir_tell (fs_dir_t dir) |
Get the current position of the directory. | |
virtual void | dir_rewind (fs_dir_t dir) |
Rewind the current position to the beginning of the directory. | |
virtual int | file_isatty (fs_file_t file) |
Check if the file in an interactive terminal device If so, line buffered behaviour is used by default. | |
virtual void | file_rewind (fs_file_t file) |
Rewind the file position to the beginning of the file. | |
virtual size_t | dir_size (fs_dir_t dir) |
Get the sizeof the directory. | |
virtual int | open (FileHandle **file, const char *path, int flags) |
Open a file on the filesystem. | |
virtual int | open (DirHandle **dir, const char *path) |
Open a directory on the filesystem. | |
MBED_DEPRECATED ("Invalid copy construction of a NonCopyable resource.") NonCopyable(const NonCopyable &) | |
NonCopyable copy constructor. | |
MBED_DEPRECATED ("Invalid copy assignment of a NonCopyable resource.") NonCopyable &operator | |
NonCopyable copy assignment operator. |
Detailed Description
FATFileSystem based on ChaN's Fat Filesystem library v0.8.
Definition at line 37 of file FATFileSystem.h.
Constructor & Destructor Documentation
FATFileSystem | ( | const char * | name = NULL , |
BlockDevice * | bd = NULL |
||
) |
Lifetime of the FATFileSystem.
- Parameters:
-
name Name to add filesystem to tree as bd BlockDevice to mount, may be passed instead to mount call
Definition at line 265 of file FATFileSystem.cpp.
Member Function Documentation
virtual int dir_close | ( | fs_dir_t | dir ) | [protected, virtual] |
Close a directory.
- Parameters:
-
dir Dir handle
- Returns:
- 0 on success, negative error code on failure
Reimplemented from FileSystem.
virtual int dir_open | ( | fs_dir_t * | dir, |
const char * | path | ||
) | [protected, virtual] |
Open a directory on the filesystem.
- Parameters:
-
dir Destination for the handle to the directory path Name of the directory to open
- Returns:
- 0 on success, negative error code on failure
Reimplemented from FileSystem.
virtual ssize_t dir_read | ( | fs_dir_t | dir, |
struct dirent * | ent | ||
) | [protected, virtual] |
Read the next directory entry.
- Parameters:
-
dir Dir handle ent The directory entry to fill out
- Returns:
- 1 on reading a filename, 0 at end of directory, negative error on failure
Reimplemented from FileSystem.
virtual void dir_rewind | ( | fs_dir_t | dir ) | [protected, virtual] |
Rewind the current position to the beginning of the directory.
- Parameters:
-
dir Dir handle
Reimplemented from FileSystem.
virtual void dir_seek | ( | fs_dir_t | dir, |
off_t | offset | ||
) | [protected, virtual] |
Set the current position of the directory.
- Parameters:
-
dir Dir handle offset Offset of the location to seek to, must be a value returned from dir_tell
Reimplemented from FileSystem.
virtual size_t dir_size | ( | fs_dir_t | dir ) | [protected, virtual, inherited] |
virtual off_t dir_tell | ( | fs_dir_t | dir ) | [protected, virtual] |
Get the current position of the directory.
- Parameters:
-
dir Dir handle
- Returns:
- Position of the directory that can be passed to dir_rewind
Reimplemented from FileSystem.
virtual int file_close | ( | fs_file_t | file ) | [protected, virtual] |
Close a file.
- Parameters:
-
file File handle
- Returns:
- 0 on success, negative error code on failure
Implements FileSystem.
virtual int file_isatty | ( | fs_file_t | file ) | [protected, virtual, inherited] |
Check if the file in an interactive terminal device If so, line buffered behaviour is used by default.
- Parameters:
-
file File handle
- Returns:
- True if the file is a terminal
virtual int file_open | ( | fs_file_t * | file, |
const char * | path, | ||
int | flags | ||
) | [protected, virtual] |
Open a file on the filesystem.
- Parameters:
-
file Destination for the handle to a newly created file path The name of the file to open flags The flags to open the file in, one of O_RDONLY, O_WRONLY, O_RDWR, bitwise or'd with one of O_CREAT, O_TRUNC, O_APPEND
- Returns:
- 0 on success, negative error code on failure
Implements FileSystem.
virtual ssize_t file_read | ( | fs_file_t | file, |
void * | buffer, | ||
size_t | len | ||
) | [protected, virtual] |
Read the contents of a file into a buffer.
- Parameters:
-
file File handle buffer The buffer to read in to len The number of bytes to read
- Returns:
- The number of bytes read, 0 at end of file, negative error on failure
Implements FileSystem.
virtual void file_rewind | ( | fs_file_t | file ) | [protected, virtual, inherited] |
Rewind the file position to the beginning of the file.
- Parameters:
-
file File handle
- Note:
- This is equivalent to file_seek(file, 0, FS_SEEK_SET)
virtual off_t file_seek | ( | fs_file_t | file, |
off_t | offset, | ||
int | whence | ||
) | [protected, virtual] |
Move the file position to a given offset from from a given location.
- Parameters:
-
file File handle offset The offset from whence to move to whence The start of where to seek SEEK_SET to start from beginning of file, SEEK_CUR to start from current position in file, SEEK_END to start from end of file
- Returns:
- The new offset of the file
Implements FileSystem.
virtual off_t file_size | ( | fs_file_t | file ) | [protected, virtual] |
Get the size of the file.
- Parameters:
-
file File handle
- Returns:
- Size of the file in bytes
Reimplemented from FileSystem.
virtual int file_sync | ( | fs_file_t | file ) | [protected, virtual] |
Flush any buffers associated with the file.
- Parameters:
-
file File handle
- Returns:
- 0 on success, negative error code on failure
Reimplemented from FileSystem.
virtual off_t file_tell | ( | fs_file_t | file ) | [protected, virtual] |
Get the file position of the file.
- Parameters:
-
file File handle
- Returns:
- The current offset in the file
Reimplemented from FileSystem.
virtual ssize_t file_write | ( | fs_file_t | file, |
const void * | buffer, | ||
size_t | len | ||
) | [protected, virtual] |
Write the contents of a buffer to a file.
- Parameters:
-
file File handle buffer The buffer to write from len The number of bytes to write
- Returns:
- The number of bytes written, negative error on failure
Implements FileSystem.
int format | ( | BlockDevice * | bd, |
bd_size_t | cluster_size = 0 |
||
) | [static] |
Formats a logical drive, FDISK partitioning rule.
The block device to format should be mounted when this function is called.
- Parameters:
-
bd This is the block device that will be formatted. cluster_size This is the number of bytes per cluster. A larger cluster size will decrease the overhead of the FAT table, but also increase the minimum file size. The cluster_size must be a multiple of the underlying device's allocation unit and is currently limited to a max of 32,768 bytes. If zero, a cluster size will be determined from the device's allocation unit. Defaults to zero.
- Returns:
- 0 on success, negative error code on failure
Definition at line 325 of file FATFileSystem.cpp.
MBED_DEPRECATED | ( | "Invalid copy construction of a NonCopyable< FileBase > resource." | ) | const [protected, inherited] |
NonCopyable copy constructor.
A compile time warning is issued when this function is used and a runtime warning is printed when the copy construction of the non copyable happens.
If you see this warning, your code is probably doing something unspecified. Copy of non copyable resources can lead to resource leak and random error.
Definition at line 171 of file NonCopyable.h.
MBED_DEPRECATED | ( | "Invalid copy assignment of a NonCopyable< FileBase > resource." | ) | [protected, inherited] |
NonCopyable copy assignment operator.
A compile time warning is issued when this function is used and a runtime warning is printed when the copy construction of the non copyable happens.
If you see this warning, your code is probably doing something unspecified. Copy of non copyable resources can lead to resource leak and random error.
MBED_DEPRECATED_SINCE | ( | "mbed-os-5.5" | , |
"Replaced by `int open(FileHandle **, ...)` for propagating error codes" | |||
) | const [inherited] |
Open a file on the filesystem.
- Parameters:
-
path The name of the file to open flags The flags to open the file in, one of O_RDONLY, O_WRONLY, O_RDWR, bitwise or'd with one of O_CREAT, O_TRUNC, O_APPEND
- Returns:
- A file handle on success, NULL on failure
int mkdir | ( | const char * | path, |
mode_t | mode | ||
) | [virtual] |
Create a directory in the filesystem.
- Parameters:
-
path The name of the directory to create. mode The permissions with which to create the directory
- Returns:
- 0 on success, negative error code on failure
Reimplemented from FileSystem.
Definition at line 405 of file FATFileSystem.cpp.
int mount | ( | BlockDevice * | bd ) | [virtual] |
Mounts a filesystem to a block device.
- Parameters:
-
bd BlockDevice to mount to
- Returns:
- 0 on success, negative error code on failure
Implements FileSystem.
Definition at line 278 of file FATFileSystem.cpp.
virtual int open | ( | FileHandle ** | file, |
const char * | filename, | ||
int | flags | ||
) | [protected, virtual, inherited] |
Open a file on the filesystem.
- Parameters:
-
file Destination for the handle to a newly created file filename The name of the file to open flags The flags to open the file in, one of O_RDONLY, O_WRONLY, O_RDWR, bitwise or'd with one of O_CREAT, O_TRUNC, O_APPEND
- Returns:
- 0 on success, negative error code on failure
Implements FileSystemHandle.
virtual int open | ( | DirHandle ** | dir, |
const char * | path | ||
) | [protected, virtual, inherited] |
Open a directory on the filesystem.
- Parameters:
-
dir Destination for the handle to the directory path Name of the directory to open
- Returns:
- 0 on success, negative error code on failure
Reimplemented from FileSystemHandle.
virtual int reformat | ( | BlockDevice * | bd = NULL ) |
[virtual] |
Reformats a filesystem, results in an empty and mounted filesystem.
- Parameters:
-
bd BlockDevice to reformat and mount. If NULL, the mounted block device will be used. Note: if mount fails, bd must be provided. Default: NULL
- Returns:
- 0 on success, negative error code on failure
Reimplemented from FileSystem.
Definition at line 105 of file FATFileSystem.h.
int reformat | ( | BlockDevice * | bd, |
int | allocation_unit | ||
) | [virtual] |
Reformats a filesystem, results in an empty and mounted filesystem.
- Parameters:
-
bd BlockDevice to reformat and mount. If NULL, the mounted block device will be used. Note: if mount fails, bd must be provided. Default: NULL allocation_unit This is the number of bytes per cluster size. The valid value is N times the sector size. N is a power of 2 from 1 to 128 for FAT volume and upto 16MiB for exFAT volume. If zero is given, the default allocation unit size is selected by the underlying filesystem, which depends on the volume size.
- Returns:
- 0 on success, negative error code on failure
Definition at line 348 of file FATFileSystem.cpp.
int remove | ( | const char * | path ) | [virtual] |
Remove a file from the filesystem.
- Parameters:
-
path The name of the file to remove.
- Returns:
- 0 on success, negative error code on failure
Reimplemented from FileSystem.
Definition at line 378 of file FATFileSystem.cpp.
int rename | ( | const char * | path, |
const char * | newpath | ||
) | [virtual] |
Rename a file in the filesystem.
- Parameters:
-
path The name of the file to rename. newpath The name to rename it to
- Returns:
- 0 on success, negative error code on failure
Reimplemented from FileSystem.
Definition at line 391 of file FATFileSystem.cpp.
int stat | ( | const char * | path, |
struct stat * | st | ||
) | [virtual] |
Store information about the file in a stat structure.
- Parameters:
-
path The name of the file to find information about st The stat buffer to write to
- Returns:
- 0 on success, negative error code on failure
Reimplemented from FileSystem.
Definition at line 418 of file FATFileSystem.cpp.
int unmount | ( | ) | [virtual] |
Unmounts a filesystem from the underlying block device.
- Returns:
- 0 on success, negative error code on failure
Implements FileSystem.
Definition at line 308 of file FATFileSystem.cpp.
Generated on Sun Jul 17 2022 08:25:40 by
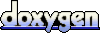