
Rtos API example
BlockDevice Class Reference
A hardware device capable of writing and reading blocks. More...
#include <BlockDevice.h>
Inherited by ChainingBlockDevice, HeapBlockDevice, MBRBlockDevice, ProfilingBlockDevice, SlicingBlockDevice, and USBHostMSD.
Public Member Functions | |
virtual | ~BlockDevice () |
Lifetime of a block device. | |
virtual int | init ()=0 |
Initialize a block device. | |
virtual int | deinit ()=0 |
Deinitialize a block device. | |
virtual int | read (void *buffer, bd_addr_t addr, bd_size_t size)=0 |
Read blocks from a block device. | |
virtual int | program (const void *buffer, bd_addr_t addr, bd_size_t size)=0 |
Program blocks to a block device. | |
virtual int | erase (bd_addr_t addr, bd_size_t size) |
Erase blocks on a block device. | |
virtual int | trim (bd_addr_t addr, bd_size_t size) |
Mark blocks as no longer in use. | |
virtual bd_size_t | get_read_size () const =0 |
Get the size of a readable block. | |
virtual bd_size_t | get_program_size () const =0 |
Get the size of a programable block. | |
virtual bd_size_t | get_erase_size () const |
Get the size of a eraseable block. | |
virtual bd_size_t | size () const =0 |
Get the total size of the underlying device. | |
bool | is_valid_read (bd_addr_t addr, bd_size_t size) const |
Convenience function for checking block read validity. | |
bool | is_valid_program (bd_addr_t addr, bd_size_t size) const |
Convenience function for checking block program validity. | |
bool | is_valid_erase (bd_addr_t addr, bd_size_t size) const |
Convenience function for checking block erase validity. |
Detailed Description
A hardware device capable of writing and reading blocks.
Definition at line 43 of file BlockDevice.h.
Constructor & Destructor Documentation
virtual ~BlockDevice | ( | ) | [virtual] |
Lifetime of a block device.
Definition at line 48 of file BlockDevice.h.
Member Function Documentation
virtual int deinit | ( | ) | [pure virtual] |
Deinitialize a block device.
- Returns:
- 0 on success or a negative error code on failure
Implemented in ChainingBlockDevice, HeapBlockDevice, MBRBlockDevice, ProfilingBlockDevice, SlicingBlockDevice, and USBHostMSD.
virtual int erase | ( | bd_addr_t | addr, |
bd_size_t | size | ||
) | [virtual] |
Erase blocks on a block device.
The state of an erased block is undefined until it has been programmed
- Parameters:
-
addr Address of block to begin erasing size Size to erase in bytes, must be a multiple of erase block size
- Returns:
- 0 on success, negative error code on failure
Reimplemented in ChainingBlockDevice, HeapBlockDevice, MBRBlockDevice, ProfilingBlockDevice, SlicingBlockDevice, and USBHostMSD.
Definition at line 94 of file BlockDevice.h.
virtual bd_size_t get_erase_size | ( | ) | const [virtual] |
Get the size of a eraseable block.
- Returns:
- Size of a eraseable block in bytes
- Note:
- Must be a multiple of the program size
Reimplemented in ChainingBlockDevice, HeapBlockDevice, MBRBlockDevice, ProfilingBlockDevice, SlicingBlockDevice, and USBHostMSD.
Definition at line 133 of file BlockDevice.h.
virtual bd_size_t get_program_size | ( | ) | const [pure virtual] |
Get the size of a programable block.
- Returns:
- Size of a programable block in bytes
- Note:
- Must be a multiple of the read size
Implemented in ChainingBlockDevice, HeapBlockDevice, MBRBlockDevice, ProfilingBlockDevice, SlicingBlockDevice, and USBHostMSD.
virtual bd_size_t get_read_size | ( | ) | const [pure virtual] |
Get the size of a readable block.
- Returns:
- Size of a readable block in bytes
Implemented in ChainingBlockDevice, HeapBlockDevice, MBRBlockDevice, ProfilingBlockDevice, SlicingBlockDevice, and USBHostMSD.
virtual int init | ( | ) | [pure virtual] |
Initialize a block device.
- Returns:
- 0 on success or a negative error code on failure
Implemented in ChainingBlockDevice, HeapBlockDevice, MBRBlockDevice, ProfilingBlockDevice, SlicingBlockDevice, and USBHostMSD.
bool is_valid_erase | ( | bd_addr_t | addr, |
bd_size_t | size | ||
) | const |
Convenience function for checking block erase validity.
- Parameters:
-
addr Address of block to begin erasing size Size to erase in bytes
- Returns:
- True if erase is valid for underlying block device
Definition at line 178 of file BlockDevice.h.
bool is_valid_program | ( | bd_addr_t | addr, |
bd_size_t | size | ||
) | const |
Convenience function for checking block program validity.
- Parameters:
-
addr Address of block to begin writing to size Size to write in bytes
- Returns:
- True if program is valid for underlying block device
Definition at line 164 of file BlockDevice.h.
bool is_valid_read | ( | bd_addr_t | addr, |
bd_size_t | size | ||
) | const |
Convenience function for checking block read validity.
- Parameters:
-
addr Address of block to begin reading from size Size to read in bytes
- Returns:
- True if read is valid for underlying block device
Definition at line 150 of file BlockDevice.h.
virtual int program | ( | const void * | buffer, |
bd_addr_t | addr, | ||
bd_size_t | size | ||
) | [pure virtual] |
Program blocks to a block device.
The blocks must have been erased prior to being programmed
If a failure occurs, it is not possible to determine how many bytes succeeded
- Parameters:
-
buffer Buffer of data to write to blocks addr Address of block to begin writing to size Size to write in bytes, must be a multiple of program block size
- Returns:
- 0 on success, negative error code on failure
Implemented in ChainingBlockDevice, HeapBlockDevice, MBRBlockDevice, ProfilingBlockDevice, SlicingBlockDevice, and USBHostMSD.
virtual int read | ( | void * | buffer, |
bd_addr_t | addr, | ||
bd_size_t | size | ||
) | [pure virtual] |
Read blocks from a block device.
If a failure occurs, it is not possible to determine how many bytes succeeded
- Parameters:
-
buffer Buffer to write blocks to addr Address of block to begin reading from size Size to read in bytes, must be a multiple of read block size
- Returns:
- 0 on success, negative error code on failure
Implemented in ChainingBlockDevice, HeapBlockDevice, MBRBlockDevice, ProfilingBlockDevice, SlicingBlockDevice, and USBHostMSD.
virtual bd_size_t size | ( | ) | const [pure virtual] |
Get the total size of the underlying device.
- Returns:
- Size of the underlying device in bytes
Implemented in ChainingBlockDevice, HeapBlockDevice, MBRBlockDevice, ProfilingBlockDevice, SlicingBlockDevice, and USBHostMSD.
virtual int trim | ( | bd_addr_t | addr, |
bd_size_t | size | ||
) | [virtual] |
Mark blocks as no longer in use.
This function provides a hint to the underlying block device that a region of blocks is no longer in use and may be erased without side effects. Erase must still be called before programming, but trimming allows flash-translation-layers to schedule erases when the device is not busy.
- Parameters:
-
addr Address of block to mark as unused size Size to mark as unused in bytes, must be a multiple of erase block size
- Returns:
- 0 on success, negative error code on failure
Definition at line 110 of file BlockDevice.h.
Generated on Sun Jul 17 2022 08:25:39 by
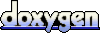