
Rtos API example
Embed:
(wiki syntax)
Show/hide line numbers
FATFileSystem.h
00001 /* mbed Microcontroller Library 00002 * Copyright (c) 2006-2012 ARM Limited 00003 * 00004 * Permission is hereby granted, free of charge, to any person obtaining a copy 00005 * of this software and associated documentation files (the "Software"), to deal 00006 * in the Software without restriction, including without limitation the rights 00007 * to use, copy, modify, merge, publish, distribute, sublicense, and/or sell 00008 * copies of the Software, and to permit persons to whom the Software is 00009 * furnished to do so, subject to the following conditions: 00010 * 00011 * The above copyright notice and this permission notice shall be included in 00012 * all copies or substantial portions of the Software. 00013 * 00014 * THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR 00015 * IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY, 00016 * FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE 00017 * AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER 00018 * LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM, 00019 * OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE 00020 * SOFTWARE. 00021 */ 00022 #ifndef MBED_FATFILESYSTEM_H 00023 #define MBED_FATFILESYSTEM_H 00024 00025 #include "FileSystem.h" 00026 #include "BlockDevice.h" 00027 #include "FileHandle.h" 00028 #include "ff.h" 00029 #include <stdint.h> 00030 #include "PlatformMutex.h" 00031 00032 using namespace mbed; 00033 00034 /** 00035 * FATFileSystem based on ChaN's Fat Filesystem library v0.8 00036 */ 00037 class FATFileSystem : public FileSystem { 00038 public: 00039 /** Lifetime of the FATFileSystem 00040 * 00041 * @param name Name to add filesystem to tree as 00042 * @param bd BlockDevice to mount, may be passed instead to mount call 00043 */ 00044 FATFileSystem(const char *name = NULL, BlockDevice *bd = NULL); 00045 virtual ~FATFileSystem(); 00046 00047 /** Formats a logical drive, FDISK partitioning rule. 00048 * 00049 * The block device to format should be mounted when this function is called. 00050 * 00051 * @param bd 00052 * This is the block device that will be formatted. 00053 * 00054 * @param cluster_size 00055 * This is the number of bytes per cluster. A larger cluster size will decrease 00056 * the overhead of the FAT table, but also increase the minimum file size. The 00057 * cluster_size must be a multiple of the underlying device's allocation unit 00058 * and is currently limited to a max of 32,768 bytes. If zero, a cluster size 00059 * will be determined from the device's allocation unit. Defaults to zero. 00060 * 00061 * @return 0 on success, negative error code on failure 00062 */ 00063 static int format(BlockDevice *bd, bd_size_t cluster_size = 0); 00064 00065 /** Mounts a filesystem to a block device 00066 * 00067 * @param bd BlockDevice to mount to 00068 * @return 0 on success, negative error code on failure 00069 */ 00070 virtual int mount(BlockDevice *bd); 00071 00072 /** Unmounts a filesystem from the underlying block device 00073 * 00074 * @return 0 on success, negative error code on failure 00075 */ 00076 virtual int unmount(); 00077 00078 /** Reformats a filesystem, results in an empty and mounted filesystem 00079 * 00080 * @param bd 00081 * BlockDevice to reformat and mount. If NULL, the mounted 00082 * block device will be used. 00083 * Note: if mount fails, bd must be provided. 00084 * Default: NULL 00085 * 00086 * @param allocation_unit 00087 * This is the number of bytes per cluster size. The valid value is N 00088 * times the sector size. N is a power of 2 from 1 to 128 for FAT 00089 * volume and upto 16MiB for exFAT volume. If zero is given, 00090 * the default allocation unit size is selected by the underlying 00091 * filesystem, which depends on the volume size. 00092 * 00093 * @return 0 on success, negative error code on failure 00094 */ 00095 virtual int reformat(BlockDevice *bd, int allocation_unit); 00096 00097 /** Reformats a filesystem, results in an empty and mounted filesystem 00098 * 00099 * @param bd BlockDevice to reformat and mount. If NULL, the mounted 00100 * block device will be used. 00101 * Note: if mount fails, bd must be provided. 00102 * Default: NULL 00103 * @return 0 on success, negative error code on failure 00104 */ 00105 virtual int reformat(BlockDevice *bd = NULL) 00106 { 00107 // required for virtual inheritance shenanigans 00108 return reformat(bd, 0); 00109 } 00110 00111 /** Remove a file from the filesystem. 00112 * 00113 * @param path The name of the file to remove. 00114 * @return 0 on success, negative error code on failure 00115 */ 00116 virtual int remove(const char *path); 00117 00118 /** Rename a file in the filesystem. 00119 * 00120 * @param path The name of the file to rename. 00121 * @param newpath The name to rename it to 00122 * @return 0 on success, negative error code on failure 00123 */ 00124 virtual int rename(const char *path, const char *newpath); 00125 00126 /** Store information about the file in a stat structure 00127 * 00128 * @param path The name of the file to find information about 00129 * @param st The stat buffer to write to 00130 * @return 0 on success, negative error code on failure 00131 */ 00132 virtual int stat(const char *path, struct stat *st); 00133 00134 /** Create a directory in the filesystem. 00135 * 00136 * @param path The name of the directory to create. 00137 * @param mode The permissions with which to create the directory 00138 * @return 0 on success, negative error code on failure 00139 */ 00140 virtual int mkdir(const char *path, mode_t mode); 00141 00142 protected: 00143 /** Open a file on the filesystem 00144 * 00145 * @param file Destination for the handle to a newly created file 00146 * @param path The name of the file to open 00147 * @param flags The flags to open the file in, one of O_RDONLY, O_WRONLY, O_RDWR, 00148 * bitwise or'd with one of O_CREAT, O_TRUNC, O_APPEND 00149 * @return 0 on success, negative error code on failure 00150 */ 00151 virtual int file_open(fs_file_t *file, const char *path, int flags); 00152 00153 /** Close a file 00154 * 00155 * @param file File handle 00156 * @return 0 on success, negative error code on failure 00157 */ 00158 virtual int file_close(fs_file_t file); 00159 00160 /** Read the contents of a file into a buffer 00161 * 00162 * @param file File handle 00163 * @param buffer The buffer to read in to 00164 * @param len The number of bytes to read 00165 * @return The number of bytes read, 0 at end of file, negative error on failure 00166 */ 00167 virtual ssize_t file_read(fs_file_t file, void *buffer, size_t len); 00168 00169 /** Write the contents of a buffer to a file 00170 * 00171 * @param file File handle 00172 * @param buffer The buffer to write from 00173 * @param len The number of bytes to write 00174 * @return The number of bytes written, negative error on failure 00175 */ 00176 virtual ssize_t file_write(fs_file_t file, const void *buffer, size_t len); 00177 00178 /** Flush any buffers associated with the file 00179 * 00180 * @param file File handle 00181 * @return 0 on success, negative error code on failure 00182 */ 00183 virtual int file_sync(fs_file_t file); 00184 00185 /** Move the file position to a given offset from from a given location 00186 * 00187 * @param file File handle 00188 * @param offset The offset from whence to move to 00189 * @param whence The start of where to seek 00190 * SEEK_SET to start from beginning of file, 00191 * SEEK_CUR to start from current position in file, 00192 * SEEK_END to start from end of file 00193 * @return The new offset of the file 00194 */ 00195 virtual off_t file_seek(fs_file_t file, off_t offset, int whence); 00196 00197 /** Get the file position of the file 00198 * 00199 * @param file File handle 00200 * @return The current offset in the file 00201 */ 00202 virtual off_t file_tell(fs_file_t file); 00203 00204 /** Get the size of the file 00205 * 00206 * @param file File handle 00207 * @return Size of the file in bytes 00208 */ 00209 virtual off_t file_size(fs_file_t file); 00210 00211 /** Open a directory on the filesystem 00212 * 00213 * @param dir Destination for the handle to the directory 00214 * @param path Name of the directory to open 00215 * @return 0 on success, negative error code on failure 00216 */ 00217 virtual int dir_open(fs_dir_t *dir, const char *path); 00218 00219 /** Close a directory 00220 * 00221 * @param dir Dir handle 00222 * @return 0 on success, negative error code on failure 00223 */ 00224 virtual int dir_close(fs_dir_t dir); 00225 00226 /** Read the next directory entry 00227 * 00228 * @param dir Dir handle 00229 * @param ent The directory entry to fill out 00230 * @return 1 on reading a filename, 0 at end of directory, negative error on failure 00231 */ 00232 virtual ssize_t dir_read(fs_dir_t dir, struct dirent *ent); 00233 00234 /** Set the current position of the directory 00235 * 00236 * @param dir Dir handle 00237 * @param offset Offset of the location to seek to, 00238 * must be a value returned from dir_tell 00239 */ 00240 virtual void dir_seek(fs_dir_t dir, off_t offset); 00241 00242 /** Get the current position of the directory 00243 * 00244 * @param dir Dir handle 00245 * @return Position of the directory that can be passed to dir_rewind 00246 */ 00247 virtual off_t dir_tell(fs_dir_t dir); 00248 00249 /** Rewind the current position to the beginning of the directory 00250 * 00251 * @param dir Dir handle 00252 */ 00253 virtual void dir_rewind(fs_dir_t dir); 00254 00255 private: 00256 FATFS _fs; // Work area (file system object) for logical drive 00257 char _fsid[sizeof("0:")]; 00258 int _id; 00259 00260 protected: 00261 virtual void lock(); 00262 virtual void unlock(); 00263 virtual int mount(BlockDevice *bd, bool mount); 00264 }; 00265 00266 #endif
Generated on Sun Jul 17 2022 08:25:22 by
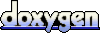