
Programming of the DDS-60 (AD9851) frequency synthesizer from AmQRP http://midnightdesignsolutions.com/dds60/index.html I had to use long, floating math in order to get accurate frequency output.
Dependencies: TextLCD mbed ChaNFS
AD9851 Class Reference
A AD9851 driver interface to control the DDS-60 from AmQRP. More...
#include <AD9851.h>
Public Member Functions | |
AD9851 (PinName SDO, PinName CLK, PinName LEN) | |
Create a frequency selector object. |
Detailed Description
A AD9851 driver interface to control the DDS-60 from AmQRP.
#include "mbed.h" #include "AD9851.h" AD9851 freqSyn(p13, p14, p15); //sdo, clk, len int main() { freqSyn.AD9851Enable(); //turn on AD9851 freqSyn.AD9851Disable(); //turn off AD9851 float Fdata; Fdata = freqSyn.GetBaseValue(); //get Base frequency Fdata = freqSyn.GetIfValue(); //get IF offset frequency freqSyn.SetBaseValue(7320000.0); //set new Base frequency (0.0 - 179,999,999.999MHz) freqSyn.SetIfValue(1620000.0); //set new IF frequency (0.0 - 179,999,999.999MHz) freqSyn.SetM6Value('A'); //set multiplier value 0 = 30MHz max, 6 = 180MHz max, A = auto //functions below are results from CalcNewValue() bool ErFlag; ErFlag = freqSyn.CalcNewValue(); //calculate new AD9851 value, based on Base, IF and M6 values //if ok, new value output to AD9851 //note: reverts to old values if Base + IF * M6 is over limit, sets error flag //ERROR occured with new values used by CalcNewValue() ErFlag = freqSyn.GetErrFlagValue(); //get error value from last time CalsNewValue() was executed unsigned int UIdata; UIdata = freqSyn.GetFD32Value(); //get 32 bit hex value of AD9851 data char Cdata; Cdata = freqSyn.GetFortyValue(); //get 8 bit hex value of AD9851 control register (enable & M6). Phase offset always 0 }
Definition at line 44 of file AD9851.h.
Constructor & Destructor Documentation
AD9851 | ( | PinName | SDO, |
PinName | CLK, | ||
PinName | LEN | ||
) |
Create a frequency selector object.
- Parameters:
-
SDO serial data out CLK serial clock - data clocked on + edge LEN data latch enable strobe - high pulse after all 40 bits of data clocked in
Definition at line 29 of file AD9851.cpp.
Generated on Wed Jul 13 2022 20:00:24 by
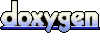