
opencv on mbed
Embed:
(wiki syntax)
Show/hide line numbers
mat.hpp
00001 /*M/////////////////////////////////////////////////////////////////////////////////////// 00002 // 00003 // IMPORTANT: READ BEFORE DOWNLOADING, COPYING, INSTALLING OR USING. 00004 // 00005 // By downloading, copying, installing or using the software you agree to this license. 00006 // If you do not agree to this license, do not download, install, 00007 // copy or use the software. 00008 // 00009 // 00010 // License Agreement 00011 // For Open Source Computer Vision Library 00012 // 00013 // Copyright (C) 2000-2008, Intel Corporation, all rights reserved. 00014 // Copyright (C) 2009, Willow Garage Inc., all rights reserved. 00015 // Copyright (C) 2013, OpenCV Foundation, all rights reserved. 00016 // Third party copyrights are property of their respective owners. 00017 // 00018 // Redistribution and use in source and binary forms, with or without modification, 00019 // are permitted provided that the following conditions are met: 00020 // 00021 // * Redistribution's of source code must retain the above copyright notice, 00022 // this list of conditions and the following disclaimer. 00023 // 00024 // * Redistribution's in binary form must reproduce the above copyright notice, 00025 // this list of conditions and the following disclaimer in the documentation 00026 // and/or other materials provided with the distribution. 00027 // 00028 // * The name of the copyright holders may not be used to endorse or promote products 00029 // derived from this software without specific prior written permission. 00030 // 00031 // This software is provided by the copyright holders and contributors "as is" and 00032 // any express or implied warranties, including, but not limited to, the implied 00033 // warranties of merchantability and fitness for a particular purpose are disclaimed. 00034 // In no event shall the Intel Corporation or contributors be liable for any direct, 00035 // indirect, incidental, special, exemplary, or consequential damages 00036 // (including, but not limited to, procurement of substitute goods or services; 00037 // loss of use, data, or profits; or business interruption) however caused 00038 // and on any theory of liability, whether in contract, strict liability, 00039 // or tort (including negligence or otherwise) arising in any way out of 00040 // the use of this software, even if advised of the possibility of such damage. 00041 // 00042 //M*/ 00043 00044 #ifndef __OPENCV_CORE_MAT_HPP__ 00045 #define __OPENCV_CORE_MAT_HPP__ 00046 00047 #ifndef __cplusplus 00048 # error mat.hpp header must be compiled as C++ 00049 #endif 00050 00051 #include "opencv2/core/matx.hpp" 00052 #include "opencv2/core/types.hpp" 00053 00054 #include "opencv2/core/bufferpool.hpp" 00055 00056 namespace cv 00057 { 00058 00059 //! @addtogroup core_basic 00060 //! @{ 00061 00062 enum { ACCESS_READ=1<<24, ACCESS_WRITE=1<<25, 00063 ACCESS_RW=3<<24, ACCESS_MASK=ACCESS_RW, ACCESS_FAST=1<<26 }; 00064 00065 class CV_EXPORTS _OutputArray; 00066 00067 //////////////////////// Input/Output Array Arguments ///////////////////////////////// 00068 00069 /** @brief This is the proxy class for passing read-only input arrays into OpenCV functions. 00070 00071 It is defined as: 00072 @code 00073 typedef const _InputArray& InputArray; 00074 @endcode 00075 where _InputArray is a class that can be constructed from `Mat`, `Mat_<T>`, `Matx<T, m, n>`, 00076 `std::vector<T>`, `std::vector<std::vector<T> >` or `std::vector<Mat>`. It can also be constructed 00077 from a matrix expression. 00078 00079 Since this is mostly implementation-level class, and its interface may change in future versions, we 00080 do not describe it in details. There are a few key things, though, that should be kept in mind: 00081 00082 - When you see in the reference manual or in OpenCV source code a function that takes 00083 InputArray, it means that you can actually pass `Mat`, `Matx`, `vector<T>` etc. (see above the 00084 complete list). 00085 - Optional input arguments: If some of the input arrays may be empty, pass cv::noArray() (or 00086 simply cv::Mat() as you probably did before). 00087 - The class is designed solely for passing parameters. That is, normally you *should not* 00088 declare class members, local and global variables of this type. 00089 - If you want to design your own function or a class method that can operate of arrays of 00090 multiple types, you can use InputArray (or OutputArray) for the respective parameters. Inside 00091 a function you should use _InputArray::getMat() method to construct a matrix header for the 00092 array (without copying data). _InputArray::kind() can be used to distinguish Mat from 00093 `vector<>` etc., but normally it is not needed. 00094 00095 Here is how you can use a function that takes InputArray : 00096 @code 00097 std::vector<Point2f> vec; 00098 // points or a circle 00099 for( int i = 0; i < 30; i++ ) 00100 vec.push_back(Point2f((float)(100 + 30*cos(i*CV_PI*2/5)), 00101 (float)(100 - 30*sin(i*CV_PI*2/5)))); 00102 cv::transform(vec, vec, cv::Matx23f(0.707, -0.707, 10, 0.707, 0.707, 20)); 00103 @endcode 00104 That is, we form an STL vector containing points, and apply in-place affine transformation to the 00105 vector using the 2x3 matrix created inline as `Matx<float, 2, 3>` instance. 00106 00107 Here is how such a function can be implemented (for simplicity, we implement a very specific case of 00108 it, according to the assertion statement inside) : 00109 @code 00110 void myAffineTransform(InputArray _src, OutputArray _dst, InputArray _m) 00111 { 00112 // get Mat headers for input arrays. This is O(1) operation, 00113 // unless _src and/or _m are matrix expressions. 00114 Mat src = _src.getMat(), m = _m.getMat(); 00115 CV_Assert( src.type() == CV_32FC2 && m.type() == CV_32F && m.size() == Size(3, 2) ); 00116 00117 // [re]create the output array so that it has the proper size and type. 00118 // In case of Mat it calls Mat::create, in case of STL vector it calls vector::resize. 00119 _dst.create(src.size(), src.type()); 00120 Mat dst = _dst.getMat(); 00121 00122 for( int i = 0; i < src.rows; i++ ) 00123 for( int j = 0; j < src.cols; j++ ) 00124 { 00125 Point2f pt = src.at<Point2f>(i, j); 00126 dst.at<Point2f>(i, j) = Point2f(m.at<float>(0, 0)*pt.x + 00127 m.at<float>(0, 1)*pt.y + 00128 m.at<float>(0, 2), 00129 m.at<float>(1, 0)*pt.x + 00130 m.at<float>(1, 1)*pt.y + 00131 m.at<float>(1, 2)); 00132 } 00133 } 00134 @endcode 00135 There is another related type, InputArrayOfArrays, which is currently defined as a synonym for 00136 InputArray: 00137 @code 00138 typedef InputArray InputArrayOfArrays; 00139 @endcode 00140 It denotes function arguments that are either vectors of vectors or vectors of matrices. A separate 00141 synonym is needed to generate Python/Java etc. wrappers properly. At the function implementation 00142 level their use is similar, but _InputArray::getMat(idx) should be used to get header for the 00143 idx-th component of the outer vector and _InputArray::size().area() should be used to find the 00144 number of components (vectors/matrices) of the outer vector. 00145 */ 00146 class CV_EXPORTS _InputArray 00147 { 00148 public: 00149 enum { 00150 KIND_SHIFT = 16, 00151 FIXED_TYPE = 0x8000 << KIND_SHIFT, 00152 FIXED_SIZE = 0x4000 << KIND_SHIFT, 00153 KIND_MASK = 31 << KIND_SHIFT, 00154 00155 NONE = 0 << KIND_SHIFT, 00156 MAT = 1 << KIND_SHIFT, 00157 MATX = 2 << KIND_SHIFT, 00158 STD_VECTOR = 3 << KIND_SHIFT, 00159 STD_VECTOR_VECTOR = 4 << KIND_SHIFT, 00160 STD_VECTOR_MAT = 5 << KIND_SHIFT, 00161 EXPR = 6 << KIND_SHIFT, 00162 OPENGL_BUFFER = 7 << KIND_SHIFT, 00163 CUDA_HOST_MEM = 8 << KIND_SHIFT, 00164 CUDA_GPU_MAT = 9 << KIND_SHIFT, 00165 UMAT =10 << KIND_SHIFT, 00166 STD_VECTOR_UMAT =11 << KIND_SHIFT, 00167 STD_BOOL_VECTOR =12 << KIND_SHIFT, 00168 STD_VECTOR_CUDA_GPU_MAT = 13 << KIND_SHIFT 00169 }; 00170 00171 _InputArray(); 00172 _InputArray(int _flags, void* _obj); 00173 _InputArray(const Mat& m); 00174 _InputArray(const MatExpr& expr); 00175 _InputArray(const std::vector<Mat>& vec); 00176 template<typename _Tp> _InputArray(const Mat_<_Tp>& m); 00177 template<typename _Tp> _InputArray(const std::vector<_Tp>& vec); 00178 _InputArray(const std::vector<bool>& vec); 00179 template<typename _Tp> _InputArray(const std::vector<std::vector<_Tp> >& vec); 00180 template<typename _Tp> _InputArray(const std::vector<Mat_<_Tp> >& vec); 00181 template<typename _Tp> _InputArray(const _Tp* vec, int n); 00182 template<typename _Tp, int m, int n> _InputArray(const Matx<_Tp, m, n>& matx); 00183 _InputArray(const double& val); 00184 _InputArray(const cuda::GpuMat& d_mat); 00185 _InputArray(const std::vector<cuda::GpuMat>& d_mat_array); 00186 _InputArray(const ogl::Buffer& buf); 00187 _InputArray(const cuda::HostMem& cuda_mem); 00188 template<typename _Tp> _InputArray(const cudev::GpuMat_<_Tp>& m); 00189 _InputArray(const UMat & um); 00190 _InputArray(const std::vector<UMat>& umv); 00191 00192 Mat getMat(int idx=-1) const; 00193 Mat getMat_(int idx=-1) const; 00194 UMat getUMat(int idx=-1) const; 00195 void getMatVector(std::vector<Mat>& mv) const; 00196 void getUMatVector(std::vector<UMat>& umv) const; 00197 void getGpuMatVector(std::vector<cuda::GpuMat>& gpumv) const; 00198 cuda::GpuMat getGpuMat() const; 00199 ogl::Buffer getOGlBuffer() const; 00200 00201 int getFlags() const; 00202 void* getObj() const; 00203 Size getSz() const; 00204 00205 int kind() const; 00206 int dims(int i=-1) const; 00207 int cols(int i=-1) const; 00208 int rows(int i=-1) const; 00209 Size size(int i=-1) const; 00210 int sizend(int* sz, int i=-1) const; 00211 bool sameSize(const _InputArray& arr) const; 00212 size_t total(int i=-1) const; 00213 int type(int i=-1) const; 00214 int depth(int i=-1) const; 00215 int channels(int i=-1) const; 00216 bool isContinuous(int i=-1) const; 00217 bool isSubmatrix(int i=-1) const; 00218 bool empty() const; 00219 void copyTo(const _OutputArray& arr) const; 00220 void copyTo(const _OutputArray& arr, const _InputArray & mask) const; 00221 size_t offset(int i=-1) const; 00222 size_t step(int i=-1) const; 00223 bool isMat() const; 00224 bool isUMat() const; 00225 bool isMatVector() const; 00226 bool isUMatVector() const; 00227 bool isMatx() const; 00228 bool isVector() const; 00229 bool isGpuMatVector() const; 00230 ~_InputArray(); 00231 00232 protected: 00233 int flags; 00234 void* obj; 00235 Size sz; 00236 00237 void init(int _flags, const void* _obj); 00238 void init(int _flags, const void* _obj, Size _sz); 00239 }; 00240 00241 00242 /** @brief This type is very similar to InputArray except that it is used for input/output and output function 00243 parameters. 00244 00245 Just like with InputArray, OpenCV users should not care about OutputArray, they just pass `Mat`, 00246 `vector<T>` etc. to the functions. The same limitation as for `InputArray`: *Do not explicitly 00247 create OutputArray instances* applies here too. 00248 00249 If you want to make your function polymorphic (i.e. accept different arrays as output parameters), 00250 it is also not very difficult. Take the sample above as the reference. Note that 00251 _OutputArray::create() needs to be called before _OutputArray::getMat(). This way you guarantee 00252 that the output array is properly allocated. 00253 00254 Optional output parameters. If you do not need certain output array to be computed and returned to 00255 you, pass cv::noArray(), just like you would in the case of optional input array. At the 00256 implementation level, use _OutputArray::needed() to check if certain output array needs to be 00257 computed or not. 00258 00259 There are several synonyms for OutputArray that are used to assist automatic Python/Java/... wrapper 00260 generators: 00261 @code 00262 typedef OutputArray OutputArrayOfArrays; 00263 typedef OutputArray InputOutputArray; 00264 typedef OutputArray InputOutputArrayOfArrays; 00265 @endcode 00266 */ 00267 class CV_EXPORTS _OutputArray : public _InputArray 00268 { 00269 public: 00270 enum 00271 { 00272 DEPTH_MASK_8U = 1 << CV_8U, 00273 DEPTH_MASK_8S = 1 << CV_8S, 00274 DEPTH_MASK_16U = 1 << CV_16U, 00275 DEPTH_MASK_16S = 1 << CV_16S, 00276 DEPTH_MASK_32S = 1 << CV_32S, 00277 DEPTH_MASK_32F = 1 << CV_32F, 00278 DEPTH_MASK_64F = 1 << CV_64F, 00279 DEPTH_MASK_ALL = (DEPTH_MASK_64F<<1)-1, 00280 DEPTH_MASK_ALL_BUT_8S = DEPTH_MASK_ALL & ~DEPTH_MASK_8S, 00281 DEPTH_MASK_FLT = DEPTH_MASK_32F + DEPTH_MASK_64F 00282 }; 00283 00284 _OutputArray(); 00285 _OutputArray(int _flags, void* _obj); 00286 _OutputArray(Mat& m); 00287 _OutputArray(std::vector<Mat>& vec); 00288 _OutputArray(cuda::GpuMat& d_mat); 00289 _OutputArray(std::vector<cuda::GpuMat>& d_mat); 00290 _OutputArray(ogl::Buffer& buf); 00291 _OutputArray(cuda::HostMem& cuda_mem); 00292 template<typename _Tp> _OutputArray(cudev::GpuMat_<_Tp>& m); 00293 template<typename _Tp> _OutputArray(std::vector<_Tp>& vec); 00294 _OutputArray(std::vector<bool>& vec); 00295 template<typename _Tp> _OutputArray(std::vector<std::vector<_Tp> >& vec); 00296 template<typename _Tp> _OutputArray(std::vector<Mat_<_Tp> >& vec); 00297 template<typename _Tp> _OutputArray(Mat_<_Tp>& m); 00298 template<typename _Tp> _OutputArray(_Tp* vec, int n); 00299 template<typename _Tp, int m, int n> _OutputArray(Matx<_Tp, m, n>& matx); 00300 _OutputArray(UMat & m); 00301 _OutputArray(std::vector<UMat>& vec); 00302 00303 _OutputArray(const Mat& m); 00304 _OutputArray(const std::vector<Mat>& vec); 00305 _OutputArray(const cuda::GpuMat& d_mat); 00306 _OutputArray(const std::vector<cuda::GpuMat>& d_mat); 00307 _OutputArray(const ogl::Buffer& buf); 00308 _OutputArray(const cuda::HostMem& cuda_mem); 00309 template<typename _Tp> _OutputArray(const cudev::GpuMat_<_Tp>& m); 00310 template<typename _Tp> _OutputArray(const std::vector<_Tp>& vec); 00311 template<typename _Tp> _OutputArray(const std::vector<std::vector<_Tp> >& vec); 00312 template<typename _Tp> _OutputArray(const std::vector<Mat_<_Tp> >& vec); 00313 template<typename _Tp> _OutputArray(const Mat_<_Tp>& m); 00314 template<typename _Tp> _OutputArray(const _Tp* vec, int n); 00315 template<typename _Tp, int m, int n> _OutputArray(const Matx<_Tp, m, n>& matx); 00316 _OutputArray(const UMat & m); 00317 _OutputArray(const std::vector<UMat>& vec); 00318 00319 bool fixedSize() const; 00320 bool fixedType() const; 00321 bool needed() const; 00322 Mat& getMatRef(int i=-1) const; 00323 UMat & getUMatRef(int i=-1) const; 00324 cuda::GpuMat& getGpuMatRef() const; 00325 std::vector<cuda::GpuMat>& getGpuMatVecRef() const; 00326 ogl::Buffer& getOGlBufferRef() const; 00327 cuda::HostMem& getHostMemRef() const; 00328 void create(Size sz, int type, int i=-1, bool allowTransposed=false, int fixedDepthMask=0) const; 00329 void create(int rows, int cols, int type, int i=-1, bool allowTransposed=false, int fixedDepthMask=0) const; 00330 void create(int dims, const int* size, int type, int i=-1, bool allowTransposed=false, int fixedDepthMask=0) const; 00331 void createSameSize(const _InputArray& arr, int mtype) const; 00332 void release() const; 00333 void clear() const; 00334 void setTo(const _InputArray& value, const _InputArray & mask = _InputArray()) const; 00335 00336 void assign(const UMat & u) const; 00337 void assign(const Mat& m) const; 00338 }; 00339 00340 00341 class CV_EXPORTS _InputOutputArray : public _OutputArray 00342 { 00343 public: 00344 _InputOutputArray(); 00345 _InputOutputArray(int _flags, void* _obj); 00346 _InputOutputArray(Mat& m); 00347 _InputOutputArray(std::vector<Mat>& vec); 00348 _InputOutputArray(cuda::GpuMat& d_mat); 00349 _InputOutputArray(ogl::Buffer& buf); 00350 _InputOutputArray(cuda::HostMem& cuda_mem); 00351 template<typename _Tp> _InputOutputArray(cudev::GpuMat_<_Tp>& m); 00352 template<typename _Tp> _InputOutputArray(std::vector<_Tp>& vec); 00353 _InputOutputArray(std::vector<bool>& vec); 00354 template<typename _Tp> _InputOutputArray(std::vector<std::vector<_Tp> >& vec); 00355 template<typename _Tp> _InputOutputArray(std::vector<Mat_<_Tp> >& vec); 00356 template<typename _Tp> _InputOutputArray(Mat_<_Tp>& m); 00357 template<typename _Tp> _InputOutputArray(_Tp* vec, int n); 00358 template<typename _Tp, int m, int n> _InputOutputArray(Matx<_Tp, m, n>& matx); 00359 _InputOutputArray(UMat & m); 00360 _InputOutputArray(std::vector<UMat>& vec); 00361 00362 _InputOutputArray(const Mat& m); 00363 _InputOutputArray(const std::vector<Mat>& vec); 00364 _InputOutputArray(const cuda::GpuMat& d_mat); 00365 _InputOutputArray(const std::vector<cuda::GpuMat>& d_mat); 00366 _InputOutputArray(const ogl::Buffer& buf); 00367 _InputOutputArray(const cuda::HostMem& cuda_mem); 00368 template<typename _Tp> _InputOutputArray(const cudev::GpuMat_<_Tp>& m); 00369 template<typename _Tp> _InputOutputArray(const std::vector<_Tp>& vec); 00370 template<typename _Tp> _InputOutputArray(const std::vector<std::vector<_Tp> >& vec); 00371 template<typename _Tp> _InputOutputArray(const std::vector<Mat_<_Tp> >& vec); 00372 template<typename _Tp> _InputOutputArray(const Mat_<_Tp>& m); 00373 template<typename _Tp> _InputOutputArray(const _Tp* vec, int n); 00374 template<typename _Tp, int m, int n> _InputOutputArray(const Matx<_Tp, m, n>& matx); 00375 _InputOutputArray(const UMat & m); 00376 _InputOutputArray(const std::vector<UMat>& vec); 00377 }; 00378 00379 typedef const _InputArray& InputArray; 00380 typedef InputArray InputArrayOfArrays; 00381 typedef const _OutputArray& OutputArray; 00382 typedef OutputArray OutputArrayOfArrays; 00383 typedef const _InputOutputArray& InputOutputArray; 00384 typedef InputOutputArray InputOutputArrayOfArrays; 00385 00386 CV_EXPORTS InputOutputArray noArray(); 00387 00388 /////////////////////////////////// MatAllocator ////////////////////////////////////// 00389 00390 //! Usage flags for allocator 00391 enum UMatUsageFlags 00392 { 00393 USAGE_DEFAULT = 0, 00394 00395 // buffer allocation policy is platform and usage specific 00396 USAGE_ALLOCATE_HOST_MEMORY = 1 << 0, 00397 USAGE_ALLOCATE_DEVICE_MEMORY = 1 << 1, 00398 USAGE_ALLOCATE_SHARED_MEMORY = 1 << 2, // It is not equal to: USAGE_ALLOCATE_HOST_MEMORY | USAGE_ALLOCATE_DEVICE_MEMORY 00399 00400 __UMAT_USAGE_FLAGS_32BIT = 0x7fffffff // Binary compatibility hint 00401 }; 00402 00403 struct CV_EXPORTS UMatData; 00404 00405 /** @brief Custom array allocator 00406 */ 00407 class CV_EXPORTS MatAllocator 00408 { 00409 public: 00410 MatAllocator() {} 00411 virtual ~MatAllocator() {} 00412 00413 // let's comment it off for now to detect and fix all the uses of allocator 00414 //virtual void allocate(int dims, const int* sizes, int type, int*& refcount, 00415 // uchar*& datastart, uchar*& data, size_t* step) = 0; 00416 //virtual void deallocate(int* refcount, uchar* datastart, uchar* data) = 0; 00417 virtual UMatData* allocate(int dims, const int* sizes, int type, 00418 void* data, size_t* step, int flags, UMatUsageFlags usageFlags) const = 0; 00419 virtual bool allocate(UMatData* data, int accessflags, UMatUsageFlags usageFlags) const = 0; 00420 virtual void deallocate(UMatData* data) const = 0; 00421 virtual void map(UMatData* data, int accessflags) const; 00422 virtual void unmap(UMatData* data) const; 00423 virtual void download(UMatData* data, void* dst, int dims, const size_t sz[], 00424 const size_t srcofs[], const size_t srcstep[], 00425 const size_t dststep[]) const; 00426 virtual void upload(UMatData* data, const void* src, int dims, const size_t sz[], 00427 const size_t dstofs[], const size_t dststep[], 00428 const size_t srcstep[]) const; 00429 virtual void copy(UMatData* srcdata, UMatData* dstdata, int dims, const size_t sz[], 00430 const size_t srcofs[], const size_t srcstep[], 00431 const size_t dstofs[], const size_t dststep[], bool sync) const; 00432 00433 // default implementation returns DummyBufferPoolController 00434 virtual BufferPoolController* getBufferPoolController(const char* id = NULL) const; 00435 }; 00436 00437 00438 //////////////////////////////// MatCommaInitializer ////////////////////////////////// 00439 00440 /** @brief Comma-separated Matrix Initializer 00441 00442 The class instances are usually not created explicitly. 00443 Instead, they are created on "matrix << firstValue" operator. 00444 00445 The sample below initializes 2x2 rotation matrix: 00446 00447 \code 00448 double angle = 30, a = cos(angle*CV_PI/180), b = sin(angle*CV_PI/180); 00449 Mat R = (Mat_<double>(2,2) << a, -b, b, a); 00450 \endcode 00451 */ 00452 template<typename _Tp> class MatCommaInitializer_ 00453 { 00454 public: 00455 //! the constructor, created by "matrix << firstValue" operator, where matrix is cv::Mat 00456 MatCommaInitializer_(Mat_<_Tp>* _m); 00457 //! the operator that takes the next value and put it to the matrix 00458 template<typename T2> MatCommaInitializer_<_Tp>& operator , (T2 v); 00459 //! another form of conversion operator 00460 operator Mat_<_Tp>() const; 00461 protected: 00462 MatIterator_<_Tp> it; 00463 }; 00464 00465 00466 /////////////////////////////////////// Mat /////////////////////////////////////////// 00467 00468 // note that umatdata might be allocated together 00469 // with the matrix data, not as a separate object. 00470 // therefore, it does not have constructor or destructor; 00471 // it should be explicitly initialized using init(). 00472 struct CV_EXPORTS UMatData 00473 { 00474 enum { COPY_ON_MAP=1, HOST_COPY_OBSOLETE=2, 00475 DEVICE_COPY_OBSOLETE=4, TEMP_UMAT=8, TEMP_COPIED_UMAT=24, 00476 USER_ALLOCATED=32, DEVICE_MEM_MAPPED=64}; 00477 UMatData(const MatAllocator* allocator); 00478 ~UMatData(); 00479 00480 // provide atomic access to the structure 00481 void lock(); 00482 void unlock(); 00483 00484 bool hostCopyObsolete() const; 00485 bool deviceCopyObsolete() const; 00486 bool deviceMemMapped() const; 00487 bool copyOnMap() const; 00488 bool tempUMat() const; 00489 bool tempCopiedUMat() const; 00490 void markHostCopyObsolete(bool flag); 00491 void markDeviceCopyObsolete(bool flag); 00492 void markDeviceMemMapped(bool flag); 00493 00494 const MatAllocator* prevAllocator; 00495 const MatAllocator* currAllocator; 00496 int urefcount; 00497 int refcount; 00498 uchar* data; 00499 uchar* origdata; 00500 size_t size; 00501 00502 int flags; 00503 void* handle; 00504 void* userdata; 00505 int allocatorFlags_; 00506 int mapcount; 00507 UMatData* originalUMatData; 00508 }; 00509 00510 00511 struct CV_EXPORTS UMatDataAutoLock 00512 { 00513 explicit UMatDataAutoLock(UMatData* u); 00514 ~UMatDataAutoLock(); 00515 UMatData* u; 00516 }; 00517 00518 00519 struct CV_EXPORTS MatSize 00520 { 00521 explicit MatSize(int* _p); 00522 Size operator()() const; 00523 const int& operator[](int i) const; 00524 int& operator[](int i); 00525 operator const int*() const; 00526 bool operator == (const MatSize& sz) const; 00527 bool operator != (const MatSize& sz) const; 00528 00529 int* p; 00530 }; 00531 00532 struct CV_EXPORTS MatStep 00533 { 00534 MatStep(); 00535 explicit MatStep(size_t s); 00536 const size_t& operator[](int i) const; 00537 size_t& operator[](int i); 00538 operator size_t() const; 00539 MatStep& operator = (size_t s); 00540 00541 size_t* p; 00542 size_t buf[2]; 00543 protected: 00544 MatStep& operator = (const MatStep&); 00545 }; 00546 00547 /** @example cout_mat.cpp 00548 An example demonstrating the serial out capabilities of cv::Mat 00549 */ 00550 00551 /** @brief n-dimensional dense array class 00552 00553 The class Mat represents an n-dimensional dense numerical single-channel or multi-channel array. It 00554 can be used to store real or complex-valued vectors and matrices, grayscale or color images, voxel 00555 volumes, vector fields, point clouds, tensors, histograms (though, very high-dimensional histograms 00556 may be better stored in a SparseMat ). The data layout of the array `M` is defined by the array 00557 `M.step[]`, so that the address of element \f$(i_0,...,i_{M.dims-1})\f$, where \f$0\leq i_k<M.size[k]\f$, is 00558 computed as: 00559 \f[addr(M_{i_0,...,i_{M.dims-1}}) = M.data + M.step[0]*i_0 + M.step[1]*i_1 + ... + M.step[M.dims-1]*i_{M.dims-1}\f] 00560 In case of a 2-dimensional array, the above formula is reduced to: 00561 \f[addr(M_{i,j}) = M.data + M.step[0]*i + M.step[1]*j\f] 00562 Note that `M.step[i] >= M.step[i+1]` (in fact, `M.step[i] >= M.step[i+1]*M.size[i+1]` ). This means 00563 that 2-dimensional matrices are stored row-by-row, 3-dimensional matrices are stored plane-by-plane, 00564 and so on. M.step[M.dims-1] is minimal and always equal to the element size M.elemSize() . 00565 00566 So, the data layout in Mat is fully compatible with CvMat, IplImage, and CvMatND types from OpenCV 00567 1.x. It is also compatible with the majority of dense array types from the standard toolkits and 00568 SDKs, such as Numpy (ndarray), Win32 (independent device bitmaps), and others, that is, with any 00569 array that uses *steps* (or *strides*) to compute the position of a pixel. Due to this 00570 compatibility, it is possible to make a Mat header for user-allocated data and process it in-place 00571 using OpenCV functions. 00572 00573 There are many different ways to create a Mat object. The most popular options are listed below: 00574 00575 - Use the create(nrows, ncols, type) method or the similar Mat(nrows, ncols, type[, fillValue]) 00576 constructor. A new array of the specified size and type is allocated. type has the same meaning as 00577 in the cvCreateMat method. For example, CV_8UC1 means a 8-bit single-channel array, CV_32FC2 00578 means a 2-channel (complex) floating-point array, and so on. 00579 @code 00580 // make a 7x7 complex matrix filled with 1+3j. 00581 Mat M(7,7,CV_32FC2,Scalar(1,3)); 00582 // and now turn M to a 100x60 15-channel 8-bit matrix. 00583 // The old content will be deallocated 00584 M.create(100,60,CV_8UC(15)); 00585 @endcode 00586 As noted in the introduction to this chapter, create() allocates only a new array when the shape 00587 or type of the current array are different from the specified ones. 00588 00589 - Create a multi-dimensional array: 00590 @code 00591 // create a 100x100x100 8-bit array 00592 int sz[] = {100, 100, 100}; 00593 Mat bigCube(3, sz, CV_8U, Scalar::all(0)); 00594 @endcode 00595 It passes the number of dimensions =1 to the Mat constructor but the created array will be 00596 2-dimensional with the number of columns set to 1. So, Mat::dims is always >= 2 (can also be 0 00597 when the array is empty). 00598 00599 - Use a copy constructor or assignment operator where there can be an array or expression on the 00600 right side (see below). As noted in the introduction, the array assignment is an O(1) operation 00601 because it only copies the header and increases the reference counter. The Mat::clone() method can 00602 be used to get a full (deep) copy of the array when you need it. 00603 00604 - Construct a header for a part of another array. It can be a single row, single column, several 00605 rows, several columns, rectangular region in the array (called a *minor* in algebra) or a 00606 diagonal. Such operations are also O(1) because the new header references the same data. You can 00607 actually modify a part of the array using this feature, for example: 00608 @code 00609 // add the 5-th row, multiplied by 3 to the 3rd row 00610 M.row(3) = M.row(3) + M.row(5)*3; 00611 // now copy the 7-th column to the 1-st column 00612 // M.col(1) = M.col(7); // this will not work 00613 Mat M1 = M.col(1); 00614 M.col(7).copyTo(M1); 00615 // create a new 320x240 image 00616 Mat img(Size(320,240),CV_8UC3); 00617 // select a ROI 00618 Mat roi(img, Rect(10,10,100,100)); 00619 // fill the ROI with (0,255,0) (which is green in RGB space); 00620 // the original 320x240 image will be modified 00621 roi = Scalar(0,255,0); 00622 @endcode 00623 Due to the additional datastart and dataend members, it is possible to compute a relative 00624 sub-array position in the main *container* array using locateROI(): 00625 @code 00626 Mat A = Mat::eye(10, 10, CV_32S); 00627 // extracts A columns, 1 (inclusive) to 3 (exclusive). 00628 Mat B = A(Range::all(), Range(1, 3)); 00629 // extracts B rows, 5 (inclusive) to 9 (exclusive). 00630 // that is, C \~ A(Range(5, 9), Range(1, 3)) 00631 Mat C = B(Range(5, 9), Range::all()); 00632 Size size; Point ofs; 00633 C.locateROI(size, ofs); 00634 // size will be (width=10,height=10) and the ofs will be (x=1, y=5) 00635 @endcode 00636 As in case of whole matrices, if you need a deep copy, use the `clone()` method of the extracted 00637 sub-matrices. 00638 00639 - Make a header for user-allocated data. It can be useful to do the following: 00640 -# Process "foreign" data using OpenCV (for example, when you implement a DirectShow\* filter or 00641 a processing module for gstreamer, and so on). For example: 00642 @code 00643 void process_video_frame(const unsigned char* pixels, 00644 int width, int height, int step) 00645 { 00646 Mat img(height, width, CV_8UC3, pixels, step); 00647 GaussianBlur(img, img, Size(7,7), 1.5, 1.5); 00648 } 00649 @endcode 00650 -# Quickly initialize small matrices and/or get a super-fast element access. 00651 @code 00652 double m[3][3] = {{a, b, c}, {d, e, f}, {g, h, i}}; 00653 Mat M = Mat(3, 3, CV_64F, m).inv(); 00654 @endcode 00655 . 00656 Partial yet very common cases of this *user-allocated data* case are conversions from CvMat and 00657 IplImage to Mat. For this purpose, there is function cv::cvarrToMat taking pointers to CvMat or 00658 IplImage and the optional flag indicating whether to copy the data or not. 00659 @snippet samples/cpp/image.cpp iplimage 00660 00661 - Use MATLAB-style array initializers, zeros(), ones(), eye(), for example: 00662 @code 00663 // create a double-precision identity martix and add it to M. 00664 M += Mat::eye(M.rows, M.cols, CV_64F); 00665 @endcode 00666 00667 - Use a comma-separated initializer: 00668 @code 00669 // create a 3x3 double-precision identity matrix 00670 Mat M = (Mat_<double>(3,3) << 1, 0, 0, 0, 1, 0, 0, 0, 1); 00671 @endcode 00672 With this approach, you first call a constructor of the Mat class with the proper parameters, and 00673 then you just put `<< operator` followed by comma-separated values that can be constants, 00674 variables, expressions, and so on. Also, note the extra parentheses required to avoid compilation 00675 errors. 00676 00677 Once the array is created, it is automatically managed via a reference-counting mechanism. If the 00678 array header is built on top of user-allocated data, you should handle the data by yourself. The 00679 array data is deallocated when no one points to it. If you want to release the data pointed by a 00680 array header before the array destructor is called, use Mat::release(). 00681 00682 The next important thing to learn about the array class is element access. This manual already 00683 described how to compute an address of each array element. Normally, you are not required to use the 00684 formula directly in the code. If you know the array element type (which can be retrieved using the 00685 method Mat::type() ), you can access the element \f$M_{ij}\f$ of a 2-dimensional array as: 00686 @code 00687 M.at<double>(i,j) += 1.f; 00688 @endcode 00689 assuming that `M` is a double-precision floating-point array. There are several variants of the method 00690 at for a different number of dimensions. 00691 00692 If you need to process a whole row of a 2D array, the most efficient way is to get the pointer to 00693 the row first, and then just use the plain C operator [] : 00694 @code 00695 // compute sum of positive matrix elements 00696 // (assuming that M isa double-precision matrix) 00697 double sum=0; 00698 for(int i = 0; i < M.rows; i++) 00699 { 00700 const double* Mi = M.ptr<double>(i); 00701 for(int j = 0; j < M.cols; j++) 00702 sum += std::max(Mi[j], 0.); 00703 } 00704 @endcode 00705 Some operations, like the one above, do not actually depend on the array shape. They just process 00706 elements of an array one by one (or elements from multiple arrays that have the same coordinates, 00707 for example, array addition). Such operations are called *element-wise*. It makes sense to check 00708 whether all the input/output arrays are continuous, namely, have no gaps at the end of each row. If 00709 yes, process them as a long single row: 00710 @code 00711 // compute the sum of positive matrix elements, optimized variant 00712 double sum=0; 00713 int cols = M.cols, rows = M.rows; 00714 if(M.isContinuous()) 00715 { 00716 cols *= rows; 00717 rows = 1; 00718 } 00719 for(int i = 0; i < rows; i++) 00720 { 00721 const double* Mi = M.ptr<double>(i); 00722 for(int j = 0; j < cols; j++) 00723 sum += std::max(Mi[j], 0.); 00724 } 00725 @endcode 00726 In case of the continuous matrix, the outer loop body is executed just once. So, the overhead is 00727 smaller, which is especially noticeable in case of small matrices. 00728 00729 Finally, there are STL-style iterators that are smart enough to skip gaps between successive rows: 00730 @code 00731 // compute sum of positive matrix elements, iterator-based variant 00732 double sum=0; 00733 MatConstIterator_<double> it = M.begin<double>(), it_end = M.end<double>(); 00734 for(; it != it_end; ++it) 00735 sum += std::max(*it, 0.); 00736 @endcode 00737 The matrix iterators are random-access iterators, so they can be passed to any STL algorithm, 00738 including std::sort(). 00739 */ 00740 class CV_EXPORTS Mat 00741 { 00742 public: 00743 /** 00744 These are various constructors that form a matrix. As noted in the AutomaticAllocation, often 00745 the default constructor is enough, and the proper matrix will be allocated by an OpenCV function. 00746 The constructed matrix can further be assigned to another matrix or matrix expression or can be 00747 allocated with Mat::create . In the former case, the old content is de-referenced. 00748 */ 00749 Mat(); 00750 00751 /** @overload 00752 @param rows Number of rows in a 2D array. 00753 @param cols Number of columns in a 2D array. 00754 @param type Array type. Use CV_8UC1, ..., CV_64FC4 to create 1-4 channel matrices, or 00755 CV_8UC(n), ..., CV_64FC(n) to create multi-channel (up to CV_CN_MAX channels) matrices. 00756 */ 00757 Mat(int rows, int cols, int type); 00758 00759 /** @overload 00760 @param size 2D array size: Size(cols, rows) . In the Size() constructor, the number of rows and the 00761 number of columns go in the reverse order. 00762 @param type Array type. Use CV_8UC1, ..., CV_64FC4 to create 1-4 channel matrices, or 00763 CV_8UC(n), ..., CV_64FC(n) to create multi-channel (up to CV_CN_MAX channels) matrices. 00764 */ 00765 Mat(Size size, int type); 00766 00767 /** @overload 00768 @param rows Number of rows in a 2D array. 00769 @param cols Number of columns in a 2D array. 00770 @param type Array type. Use CV_8UC1, ..., CV_64FC4 to create 1-4 channel matrices, or 00771 CV_8UC(n), ..., CV_64FC(n) to create multi-channel (up to CV_CN_MAX channels) matrices. 00772 @param s An optional value to initialize each matrix element with. To set all the matrix elements to 00773 the particular value after the construction, use the assignment operator 00774 Mat::operator=(const Scalar& value) . 00775 */ 00776 Mat(int rows, int cols, int type, const Scalar & s); 00777 00778 /** @overload 00779 @param size 2D array size: Size(cols, rows) . In the Size() constructor, the number of rows and the 00780 number of columns go in the reverse order. 00781 @param type Array type. Use CV_8UC1, ..., CV_64FC4 to create 1-4 channel matrices, or 00782 CV_8UC(n), ..., CV_64FC(n) to create multi-channel (up to CV_CN_MAX channels) matrices. 00783 @param s An optional value to initialize each matrix element with. To set all the matrix elements to 00784 the particular value after the construction, use the assignment operator 00785 Mat::operator=(const Scalar& value) . 00786 */ 00787 Mat(Size size, int type, const Scalar & s); 00788 00789 /** @overload 00790 @param ndims Array dimensionality. 00791 @param sizes Array of integers specifying an n-dimensional array shape. 00792 @param type Array type. Use CV_8UC1, ..., CV_64FC4 to create 1-4 channel matrices, or 00793 CV_8UC(n), ..., CV_64FC(n) to create multi-channel (up to CV_CN_MAX channels) matrices. 00794 */ 00795 Mat(int ndims, const int* sizes, int type); 00796 00797 /** @overload 00798 @param ndims Array dimensionality. 00799 @param sizes Array of integers specifying an n-dimensional array shape. 00800 @param type Array type. Use CV_8UC1, ..., CV_64FC4 to create 1-4 channel matrices, or 00801 CV_8UC(n), ..., CV_64FC(n) to create multi-channel (up to CV_CN_MAX channels) matrices. 00802 @param s An optional value to initialize each matrix element with. To set all the matrix elements to 00803 the particular value after the construction, use the assignment operator 00804 Mat::operator=(const Scalar& value) . 00805 */ 00806 Mat(int ndims, const int* sizes, int type, const Scalar & s); 00807 00808 /** @overload 00809 @param m Array that (as a whole or partly) is assigned to the constructed matrix. No data is copied 00810 by these constructors. Instead, the header pointing to m data or its sub-array is constructed and 00811 associated with it. The reference counter, if any, is incremented. So, when you modify the matrix 00812 formed using such a constructor, you also modify the corresponding elements of m . If you want to 00813 have an independent copy of the sub-array, use Mat::clone() . 00814 */ 00815 Mat(const Mat& m); 00816 00817 /** @overload 00818 @param rows Number of rows in a 2D array. 00819 @param cols Number of columns in a 2D array. 00820 @param type Array type. Use CV_8UC1, ..., CV_64FC4 to create 1-4 channel matrices, or 00821 CV_8UC(n), ..., CV_64FC(n) to create multi-channel (up to CV_CN_MAX channels) matrices. 00822 @param data Pointer to the user data. Matrix constructors that take data and step parameters do not 00823 allocate matrix data. Instead, they just initialize the matrix header that points to the specified 00824 data, which means that no data is copied. This operation is very efficient and can be used to 00825 process external data using OpenCV functions. The external data is not automatically deallocated, so 00826 you should take care of it. 00827 @param step Number of bytes each matrix row occupies. The value should include the padding bytes at 00828 the end of each row, if any. If the parameter is missing (set to AUTO_STEP ), no padding is assumed 00829 and the actual step is calculated as cols*elemSize(). See Mat::elemSize. 00830 */ 00831 Mat(int rows, int cols, int type, void* data, size_t step=AUTO_STEP); 00832 00833 /** @overload 00834 @param size 2D array size: Size(cols, rows) . In the Size() constructor, the number of rows and the 00835 number of columns go in the reverse order. 00836 @param type Array type. Use CV_8UC1, ..., CV_64FC4 to create 1-4 channel matrices, or 00837 CV_8UC(n), ..., CV_64FC(n) to create multi-channel (up to CV_CN_MAX channels) matrices. 00838 @param data Pointer to the user data. Matrix constructors that take data and step parameters do not 00839 allocate matrix data. Instead, they just initialize the matrix header that points to the specified 00840 data, which means that no data is copied. This operation is very efficient and can be used to 00841 process external data using OpenCV functions. The external data is not automatically deallocated, so 00842 you should take care of it. 00843 @param step Number of bytes each matrix row occupies. The value should include the padding bytes at 00844 the end of each row, if any. If the parameter is missing (set to AUTO_STEP ), no padding is assumed 00845 and the actual step is calculated as cols*elemSize(). See Mat::elemSize. 00846 */ 00847 Mat(Size size, int type, void* data, size_t step=AUTO_STEP); 00848 00849 /** @overload 00850 @param ndims Array dimensionality. 00851 @param sizes Array of integers specifying an n-dimensional array shape. 00852 @param type Array type. Use CV_8UC1, ..., CV_64FC4 to create 1-4 channel matrices, or 00853 CV_8UC(n), ..., CV_64FC(n) to create multi-channel (up to CV_CN_MAX channels) matrices. 00854 @param data Pointer to the user data. Matrix constructors that take data and step parameters do not 00855 allocate matrix data. Instead, they just initialize the matrix header that points to the specified 00856 data, which means that no data is copied. This operation is very efficient and can be used to 00857 process external data using OpenCV functions. The external data is not automatically deallocated, so 00858 you should take care of it. 00859 @param steps Array of ndims-1 steps in case of a multi-dimensional array (the last step is always 00860 set to the element size). If not specified, the matrix is assumed to be continuous. 00861 */ 00862 Mat(int ndims, const int* sizes, int type, void* data, const size_t* steps=0); 00863 00864 /** @overload 00865 @param m Array that (as a whole or partly) is assigned to the constructed matrix. No data is copied 00866 by these constructors. Instead, the header pointing to m data or its sub-array is constructed and 00867 associated with it. The reference counter, if any, is incremented. So, when you modify the matrix 00868 formed using such a constructor, you also modify the corresponding elements of m . If you want to 00869 have an independent copy of the sub-array, use Mat::clone() . 00870 @param rowRange Range of the m rows to take. As usual, the range start is inclusive and the range 00871 end is exclusive. Use Range::all() to take all the rows. 00872 @param colRange Range of the m columns to take. Use Range::all() to take all the columns. 00873 */ 00874 Mat(const Mat& m, const Range& rowRange, const Range& colRange=Range::all()); 00875 00876 /** @overload 00877 @param m Array that (as a whole or partly) is assigned to the constructed matrix. No data is copied 00878 by these constructors. Instead, the header pointing to m data or its sub-array is constructed and 00879 associated with it. The reference counter, if any, is incremented. So, when you modify the matrix 00880 formed using such a constructor, you also modify the corresponding elements of m . If you want to 00881 have an independent copy of the sub-array, use Mat::clone() . 00882 @param roi Region of interest. 00883 */ 00884 Mat(const Mat& m, const Rect& roi); 00885 00886 /** @overload 00887 @param m Array that (as a whole or partly) is assigned to the constructed matrix. No data is copied 00888 by these constructors. Instead, the header pointing to m data or its sub-array is constructed and 00889 associated with it. The reference counter, if any, is incremented. So, when you modify the matrix 00890 formed using such a constructor, you also modify the corresponding elements of m . If you want to 00891 have an independent copy of the sub-array, use Mat::clone() . 00892 @param ranges Array of selected ranges of m along each dimensionality. 00893 */ 00894 Mat(const Mat& m, const Range* ranges); 00895 00896 /** @overload 00897 @param vec STL vector whose elements form the matrix. The matrix has a single column and the number 00898 of rows equal to the number of vector elements. Type of the matrix matches the type of vector 00899 elements. The constructor can handle arbitrary types, for which there is a properly declared 00900 DataType . This means that the vector elements must be primitive numbers or uni-type numerical 00901 tuples of numbers. Mixed-type structures are not supported. The corresponding constructor is 00902 explicit. Since STL vectors are not automatically converted to Mat instances, you should write 00903 Mat(vec) explicitly. Unless you copy the data into the matrix ( copyData=true ), no new elements 00904 will be added to the vector because it can potentially yield vector data reallocation, and, thus, 00905 the matrix data pointer will be invalid. 00906 @param copyData Flag to specify whether the underlying data of the STL vector should be copied 00907 to (true) or shared with (false) the newly constructed matrix. When the data is copied, the 00908 allocated buffer is managed using Mat reference counting mechanism. While the data is shared, 00909 the reference counter is NULL, and you should not deallocate the data until the matrix is not 00910 destructed. 00911 */ 00912 template<typename _Tp> explicit Mat(const std::vector<_Tp>& vec, bool copyData=false); 00913 00914 /** @overload 00915 */ 00916 template<typename _Tp, int n> explicit Mat(const Vec<_Tp, n>& vec, bool copyData=true); 00917 00918 /** @overload 00919 */ 00920 template<typename _Tp, int m, int n> explicit Mat(const Matx<_Tp, m, n>& mtx, bool copyData=true); 00921 00922 /** @overload 00923 */ 00924 template<typename _Tp> explicit Mat(const Point_<_Tp>& pt, bool copyData=true); 00925 00926 /** @overload 00927 */ 00928 template<typename _Tp> explicit Mat(const Point3_<_Tp>& pt, bool copyData=true); 00929 00930 /** @overload 00931 */ 00932 template<typename _Tp> explicit Mat(const MatCommaInitializer_<_Tp>& commaInitializer); 00933 00934 //! download data from GpuMat 00935 explicit Mat(const cuda::GpuMat& m); 00936 00937 //! destructor - calls release() 00938 ~Mat(); 00939 00940 /** @brief assignment operators 00941 00942 These are available assignment operators. Since they all are very different, make sure to read the 00943 operator parameters description. 00944 @param m Assigned, right-hand-side matrix. Matrix assignment is an O(1) operation. This means that 00945 no data is copied but the data is shared and the reference counter, if any, is incremented. Before 00946 assigning new data, the old data is de-referenced via Mat::release . 00947 */ 00948 Mat& operator = (const Mat& m); 00949 00950 /** @overload 00951 @param expr Assigned matrix expression object. As opposite to the first form of the assignment 00952 operation, the second form can reuse already allocated matrix if it has the right size and type to 00953 fit the matrix expression result. It is automatically handled by the real function that the matrix 00954 expressions is expanded to. For example, C=A+B is expanded to add(A, B, C), and add takes care of 00955 automatic C reallocation. 00956 */ 00957 Mat& operator = (const MatExpr& expr); 00958 00959 //! retrieve UMat from Mat 00960 UMat getUMat(int accessFlags, UMatUsageFlags usageFlags = USAGE_DEFAULT) const; 00961 00962 /** @brief Creates a matrix header for the specified matrix row. 00963 00964 The method makes a new header for the specified matrix row and returns it. This is an O(1) 00965 operation, regardless of the matrix size. The underlying data of the new matrix is shared with the 00966 original matrix. Here is the example of one of the classical basic matrix processing operations, 00967 axpy, used by LU and many other algorithms: 00968 @code 00969 inline void matrix_axpy(Mat& A, int i, int j, double alpha) 00970 { 00971 A.row(i) += A.row(j)*alpha; 00972 } 00973 @endcode 00974 @note In the current implementation, the following code does not work as expected: 00975 @code 00976 Mat A; 00977 ... 00978 A.row(i) = A.row(j); // will not work 00979 @endcode 00980 This happens because A.row(i) forms a temporary header that is further assigned to another header. 00981 Remember that each of these operations is O(1), that is, no data is copied. Thus, the above 00982 assignment is not true if you may have expected the j-th row to be copied to the i-th row. To 00983 achieve that, you should either turn this simple assignment into an expression or use the 00984 Mat::copyTo method: 00985 @code 00986 Mat A; 00987 ... 00988 // works, but looks a bit obscure. 00989 A.row(i) = A.row(j) + 0; 00990 // this is a bit longer, but the recommended method. 00991 A.row(j).copyTo(A.row(i)); 00992 @endcode 00993 @param y A 0-based row index. 00994 */ 00995 Mat row(int y) const; 00996 00997 /** @brief Creates a matrix header for the specified matrix column. 00998 00999 The method makes a new header for the specified matrix column and returns it. This is an O(1) 01000 operation, regardless of the matrix size. The underlying data of the new matrix is shared with the 01001 original matrix. See also the Mat::row description. 01002 @param x A 0-based column index. 01003 */ 01004 Mat col(int x) const; 01005 01006 /** @brief Creates a matrix header for the specified row span. 01007 01008 The method makes a new header for the specified row span of the matrix. Similarly to Mat::row and 01009 Mat::col , this is an O(1) operation. 01010 @param startrow An inclusive 0-based start index of the row span. 01011 @param endrow An exclusive 0-based ending index of the row span. 01012 */ 01013 Mat rowRange(int startrow, int endrow) const; 01014 01015 /** @overload 01016 @param r Range structure containing both the start and the end indices. 01017 */ 01018 Mat rowRange(const Range& r) const; 01019 01020 /** @brief Creates a matrix header for the specified column span. 01021 01022 The method makes a new header for the specified column span of the matrix. Similarly to Mat::row and 01023 Mat::col , this is an O(1) operation. 01024 @param startcol An inclusive 0-based start index of the column span. 01025 @param endcol An exclusive 0-based ending index of the column span. 01026 */ 01027 Mat colRange(int startcol, int endcol) const; 01028 01029 /** @overload 01030 @param r Range structure containing both the start and the end indices. 01031 */ 01032 Mat colRange(const Range& r) const; 01033 01034 /** @brief Extracts a diagonal from a matrix 01035 01036 The method makes a new header for the specified matrix diagonal. The new matrix is represented as a 01037 single-column matrix. Similarly to Mat::row and Mat::col, this is an O(1) operation. 01038 @param d index of the diagonal, with the following values: 01039 - `d=0` is the main diagonal. 01040 - `d>0` is a diagonal from the lower half. For example, d=1 means the diagonal is set 01041 immediately below the main one. 01042 - `d<0` is a diagonal from the upper half. For example, d=-1 means the diagonal is set 01043 immediately above the main one. 01044 */ 01045 Mat diag(int d=0) const; 01046 01047 /** @brief creates a diagonal matrix 01048 01049 The method makes a new header for the specified matrix diagonal. The new matrix is represented as a 01050 single-column matrix. Similarly to Mat::row and Mat::col, this is an O(1) operation. 01051 @param d Single-column matrix that forms a diagonal matrix 01052 */ 01053 static Mat diag(const Mat& d); 01054 01055 /** @brief Creates a full copy of the array and the underlying data. 01056 01057 The method creates a full copy of the array. The original step[] is not taken into account. So, the 01058 array copy is a continuous array occupying total()*elemSize() bytes. 01059 */ 01060 Mat clone() const; 01061 01062 /** @brief Copies the matrix to another one. 01063 01064 The method copies the matrix data to another matrix. Before copying the data, the method invokes : 01065 @code 01066 m.create(this->size(), this->type()); 01067 @endcode 01068 so that the destination matrix is reallocated if needed. While m.copyTo(m); works flawlessly, the 01069 function does not handle the case of a partial overlap between the source and the destination 01070 matrices. 01071 01072 When the operation mask is specified, if the Mat::create call shown above reallocates the matrix, 01073 the newly allocated matrix is initialized with all zeros before copying the data. 01074 @param m Destination matrix. If it does not have a proper size or type before the operation, it is 01075 reallocated. 01076 */ 01077 void copyTo( OutputArray m ) const; 01078 01079 /** @overload 01080 @param m Destination matrix. If it does not have a proper size or type before the operation, it is 01081 reallocated. 01082 @param mask Operation mask. Its non-zero elements indicate which matrix elements need to be copied. 01083 The mask has to be of type CV_8U and can have 1 or multiple channels. 01084 */ 01085 void copyTo( OutputArray m, InputArray mask ) const; 01086 01087 /** @brief Converts an array to another data type with optional scaling. 01088 01089 The method converts source pixel values to the target data type. saturate_cast<> is applied at 01090 the end to avoid possible overflows: 01091 01092 \f[m(x,y) = saturate \_ cast<rType>( \alpha (*this)(x,y) + \beta )\f] 01093 @param m output matrix; if it does not have a proper size or type before the operation, it is 01094 reallocated. 01095 @param rtype desired output matrix type or, rather, the depth since the number of channels are the 01096 same as the input has; if rtype is negative, the output matrix will have the same type as the input. 01097 @param alpha optional scale factor. 01098 @param beta optional delta added to the scaled values. 01099 */ 01100 void convertTo( OutputArray m, int rtype, double alpha=1, double beta=0 ) const; 01101 01102 /** @brief Provides a functional form of convertTo. 01103 01104 This is an internally used method called by the @ref MatrixExpressions engine. 01105 @param m Destination array. 01106 @param type Desired destination array depth (or -1 if it should be the same as the source type). 01107 */ 01108 void assignTo( Mat& m, int type=-1 ) const; 01109 01110 /** @brief Sets all or some of the array elements to the specified value. 01111 @param s Assigned scalar converted to the actual array type. 01112 */ 01113 Mat& operator = (const Scalar & s); 01114 01115 /** @brief Sets all or some of the array elements to the specified value. 01116 01117 This is an advanced variant of the Mat::operator=(const Scalar& s) operator. 01118 @param value Assigned scalar converted to the actual array type. 01119 @param mask Operation mask of the same size as \*this. 01120 */ 01121 Mat& setTo(InputArray value, InputArray mask=noArray()); 01122 01123 /** @brief Changes the shape and/or the number of channels of a 2D matrix without copying the data. 01124 01125 The method makes a new matrix header for \*this elements. The new matrix may have a different size 01126 and/or different number of channels. Any combination is possible if: 01127 - No extra elements are included into the new matrix and no elements are excluded. Consequently, 01128 the product rows\*cols\*channels() must stay the same after the transformation. 01129 - No data is copied. That is, this is an O(1) operation. Consequently, if you change the number of 01130 rows, or the operation changes the indices of elements row in some other way, the matrix must be 01131 continuous. See Mat::isContinuous . 01132 01133 For example, if there is a set of 3D points stored as an STL vector, and you want to represent the 01134 points as a 3xN matrix, do the following: 01135 @code 01136 std::vector<Point3f> vec; 01137 ... 01138 Mat pointMat = Mat(vec). // convert vector to Mat, O(1) operation 01139 reshape(1). // make Nx3 1-channel matrix out of Nx1 3-channel. 01140 // Also, an O(1) operation 01141 t(); // finally, transpose the Nx3 matrix. 01142 // This involves copying all the elements 01143 @endcode 01144 @param cn New number of channels. If the parameter is 0, the number of channels remains the same. 01145 @param rows New number of rows. If the parameter is 0, the number of rows remains the same. 01146 */ 01147 Mat reshape(int cn, int rows=0) const; 01148 01149 /** @overload */ 01150 Mat reshape(int cn, int newndims, const int* newsz) const; 01151 01152 /** @brief Transposes a matrix. 01153 01154 The method performs matrix transposition by means of matrix expressions. It does not perform the 01155 actual transposition but returns a temporary matrix transposition object that can be further used as 01156 a part of more complex matrix expressions or can be assigned to a matrix: 01157 @code 01158 Mat A1 = A + Mat::eye(A.size(), A.type())*lambda; 01159 Mat C = A1.t()*A1; // compute (A + lambda*I)^t * (A + lamda*I) 01160 @endcode 01161 */ 01162 MatExpr t() const; 01163 01164 /** @brief Inverses a matrix. 01165 01166 The method performs a matrix inversion by means of matrix expressions. This means that a temporary 01167 matrix inversion object is returned by the method and can be used further as a part of more complex 01168 matrix expressions or can be assigned to a matrix. 01169 @param method Matrix inversion method. One of cv::DecompTypes 01170 */ 01171 MatExpr inv(int method=DECOMP_LU) const; 01172 01173 /** @brief Performs an element-wise multiplication or division of the two matrices. 01174 01175 The method returns a temporary object encoding per-element array multiplication, with optional 01176 scale. Note that this is not a matrix multiplication that corresponds to a simpler "\*" operator. 01177 01178 Example: 01179 @code 01180 Mat C = A.mul(5/B); // equivalent to divide(A, B, C, 5) 01181 @endcode 01182 @param m Another array of the same type and the same size as \*this, or a matrix expression. 01183 @param scale Optional scale factor. 01184 */ 01185 MatExpr mul(InputArray m, double scale=1) const; 01186 01187 /** @brief Computes a cross-product of two 3-element vectors. 01188 01189 The method computes a cross-product of two 3-element vectors. The vectors must be 3-element 01190 floating-point vectors of the same shape and size. The result is another 3-element vector of the 01191 same shape and type as operands. 01192 @param m Another cross-product operand. 01193 */ 01194 Mat cross(InputArray m) const; 01195 01196 /** @brief Computes a dot-product of two vectors. 01197 01198 The method computes a dot-product of two matrices. If the matrices are not single-column or 01199 single-row vectors, the top-to-bottom left-to-right scan ordering is used to treat them as 1D 01200 vectors. The vectors must have the same size and type. If the matrices have more than one channel, 01201 the dot products from all the channels are summed together. 01202 @param m another dot-product operand. 01203 */ 01204 double dot(InputArray m) const; 01205 01206 /** @brief Returns a zero array of the specified size and type. 01207 01208 The method returns a Matlab-style zero array initializer. It can be used to quickly form a constant 01209 array as a function parameter, part of a matrix expression, or as a matrix initializer. : 01210 @code 01211 Mat A; 01212 A = Mat::zeros(3, 3, CV_32F); 01213 @endcode 01214 In the example above, a new matrix is allocated only if A is not a 3x3 floating-point matrix. 01215 Otherwise, the existing matrix A is filled with zeros. 01216 @param rows Number of rows. 01217 @param cols Number of columns. 01218 @param type Created matrix type. 01219 */ 01220 static MatExpr zeros(int rows, int cols, int type); 01221 01222 /** @overload 01223 @param size Alternative to the matrix size specification Size(cols, rows) . 01224 @param type Created matrix type. 01225 */ 01226 static MatExpr zeros(Size size, int type); 01227 01228 /** @overload 01229 @param ndims Array dimensionality. 01230 @param sz Array of integers specifying the array shape. 01231 @param type Created matrix type. 01232 */ 01233 static MatExpr zeros(int ndims, const int* sz, int type); 01234 01235 /** @brief Returns an array of all 1's of the specified size and type. 01236 01237 The method returns a Matlab-style 1's array initializer, similarly to Mat::zeros. Note that using 01238 this method you can initialize an array with an arbitrary value, using the following Matlab idiom: 01239 @code 01240 Mat A = Mat::ones(100, 100, CV_8U)*3; // make 100x100 matrix filled with 3. 01241 @endcode 01242 The above operation does not form a 100x100 matrix of 1's and then multiply it by 3. Instead, it 01243 just remembers the scale factor (3 in this case) and use it when actually invoking the matrix 01244 initializer. 01245 @param rows Number of rows. 01246 @param cols Number of columns. 01247 @param type Created matrix type. 01248 */ 01249 static MatExpr ones(int rows, int cols, int type); 01250 01251 /** @overload 01252 @param size Alternative to the matrix size specification Size(cols, rows) . 01253 @param type Created matrix type. 01254 */ 01255 static MatExpr ones(Size size, int type); 01256 01257 /** @overload 01258 @param ndims Array dimensionality. 01259 @param sz Array of integers specifying the array shape. 01260 @param type Created matrix type. 01261 */ 01262 static MatExpr ones(int ndims, const int* sz, int type); 01263 01264 /** @brief Returns an identity matrix of the specified size and type. 01265 01266 The method returns a Matlab-style identity matrix initializer, similarly to Mat::zeros. Similarly to 01267 Mat::ones, you can use a scale operation to create a scaled identity matrix efficiently: 01268 @code 01269 // make a 4x4 diagonal matrix with 0.1's on the diagonal. 01270 Mat A = Mat::eye(4, 4, CV_32F)*0.1; 01271 @endcode 01272 @param rows Number of rows. 01273 @param cols Number of columns. 01274 @param type Created matrix type. 01275 */ 01276 static MatExpr eye(int rows, int cols, int type); 01277 01278 /** @overload 01279 @param size Alternative matrix size specification as Size(cols, rows) . 01280 @param type Created matrix type. 01281 */ 01282 static MatExpr eye(Size size, int type); 01283 01284 /** @brief Allocates new array data if needed. 01285 01286 This is one of the key Mat methods. Most new-style OpenCV functions and methods that produce arrays 01287 call this method for each output array. The method uses the following algorithm: 01288 01289 -# If the current array shape and the type match the new ones, return immediately. Otherwise, 01290 de-reference the previous data by calling Mat::release. 01291 -# Initialize the new header. 01292 -# Allocate the new data of total()\*elemSize() bytes. 01293 -# Allocate the new, associated with the data, reference counter and set it to 1. 01294 01295 Such a scheme makes the memory management robust and efficient at the same time and helps avoid 01296 extra typing for you. This means that usually there is no need to explicitly allocate output arrays. 01297 That is, instead of writing: 01298 @code 01299 Mat color; 01300 ... 01301 Mat gray(color.rows, color.cols, color.depth()); 01302 cvtColor(color, gray, COLOR_BGR2GRAY); 01303 @endcode 01304 you can simply write: 01305 @code 01306 Mat color; 01307 ... 01308 Mat gray; 01309 cvtColor(color, gray, COLOR_BGR2GRAY); 01310 @endcode 01311 because cvtColor, as well as the most of OpenCV functions, calls Mat::create() for the output array 01312 internally. 01313 @param rows New number of rows. 01314 @param cols New number of columns. 01315 @param type New matrix type. 01316 */ 01317 void create(int rows, int cols, int type); 01318 01319 /** @overload 01320 @param size Alternative new matrix size specification: Size(cols, rows) 01321 @param type New matrix type. 01322 */ 01323 void create(Size size, int type); 01324 01325 /** @overload 01326 @param ndims New array dimensionality. 01327 @param sizes Array of integers specifying a new array shape. 01328 @param type New matrix type. 01329 */ 01330 void create(int ndims, const int* sizes, int type); 01331 01332 /** @brief Increments the reference counter. 01333 01334 The method increments the reference counter associated with the matrix data. If the matrix header 01335 points to an external data set (see Mat::Mat ), the reference counter is NULL, and the method has no 01336 effect in this case. Normally, to avoid memory leaks, the method should not be called explicitly. It 01337 is called implicitly by the matrix assignment operator. The reference counter increment is an atomic 01338 operation on the platforms that support it. Thus, it is safe to operate on the same matrices 01339 asynchronously in different threads. 01340 */ 01341 void addref(); 01342 01343 /** @brief Decrements the reference counter and deallocates the matrix if needed. 01344 01345 The method decrements the reference counter associated with the matrix data. When the reference 01346 counter reaches 0, the matrix data is deallocated and the data and the reference counter pointers 01347 are set to NULL's. If the matrix header points to an external data set (see Mat::Mat ), the 01348 reference counter is NULL, and the method has no effect in this case. 01349 01350 This method can be called manually to force the matrix data deallocation. But since this method is 01351 automatically called in the destructor, or by any other method that changes the data pointer, it is 01352 usually not needed. The reference counter decrement and check for 0 is an atomic operation on the 01353 platforms that support it. Thus, it is safe to operate on the same matrices asynchronously in 01354 different threads. 01355 */ 01356 void release(); 01357 01358 //! deallocates the matrix data 01359 void deallocate(); 01360 //! internal use function; properly re-allocates _size, _step arrays 01361 void copySize(const Mat& m); 01362 01363 /** @brief Reserves space for the certain number of rows. 01364 01365 The method reserves space for sz rows. If the matrix already has enough space to store sz rows, 01366 nothing happens. If the matrix is reallocated, the first Mat::rows rows are preserved. The method 01367 emulates the corresponding method of the STL vector class. 01368 @param sz Number of rows. 01369 */ 01370 void reserve(size_t sz); 01371 01372 /** @brief Changes the number of matrix rows. 01373 01374 The methods change the number of matrix rows. If the matrix is reallocated, the first 01375 min(Mat::rows, sz) rows are preserved. The methods emulate the corresponding methods of the STL 01376 vector class. 01377 @param sz New number of rows. 01378 */ 01379 void resize(size_t sz); 01380 01381 /** @overload 01382 @param sz New number of rows. 01383 @param s Value assigned to the newly added elements. 01384 */ 01385 void resize(size_t sz, const Scalar & s); 01386 01387 //! internal function 01388 void push_back_(const void* elem); 01389 01390 /** @brief Adds elements to the bottom of the matrix. 01391 01392 The methods add one or more elements to the bottom of the matrix. They emulate the corresponding 01393 method of the STL vector class. When elem is Mat , its type and the number of columns must be the 01394 same as in the container matrix. 01395 @param elem Added element(s). 01396 */ 01397 template<typename _Tp> void push_back(const _Tp& elem); 01398 01399 /** @overload 01400 @param elem Added element(s). 01401 */ 01402 template<typename _Tp> void push_back(const Mat_<_Tp>& elem); 01403 01404 /** @overload 01405 @param m Added line(s). 01406 */ 01407 void push_back(const Mat& m); 01408 01409 /** @brief Removes elements from the bottom of the matrix. 01410 01411 The method removes one or more rows from the bottom of the matrix. 01412 @param nelems Number of removed rows. If it is greater than the total number of rows, an exception 01413 is thrown. 01414 */ 01415 void pop_back(size_t nelems=1); 01416 01417 /** @brief Locates the matrix header within a parent matrix. 01418 01419 After you extracted a submatrix from a matrix using Mat::row, Mat::col, Mat::rowRange, 01420 Mat::colRange, and others, the resultant submatrix points just to the part of the original big 01421 matrix. However, each submatrix contains information (represented by datastart and dataend 01422 fields) that helps reconstruct the original matrix size and the position of the extracted 01423 submatrix within the original matrix. The method locateROI does exactly that. 01424 @param wholeSize Output parameter that contains the size of the whole matrix containing *this* 01425 as a part. 01426 @param ofs Output parameter that contains an offset of *this* inside the whole matrix. 01427 */ 01428 void locateROI( Size& wholeSize, Point& ofs ) const; 01429 01430 /** @brief Adjusts a submatrix size and position within the parent matrix. 01431 01432 The method is complimentary to Mat::locateROI . The typical use of these functions is to determine 01433 the submatrix position within the parent matrix and then shift the position somehow. Typically, it 01434 can be required for filtering operations when pixels outside of the ROI should be taken into 01435 account. When all the method parameters are positive, the ROI needs to grow in all directions by the 01436 specified amount, for example: 01437 @code 01438 A.adjustROI(2, 2, 2, 2); 01439 @endcode 01440 In this example, the matrix size is increased by 4 elements in each direction. The matrix is shifted 01441 by 2 elements to the left and 2 elements up, which brings in all the necessary pixels for the 01442 filtering with the 5x5 kernel. 01443 01444 adjustROI forces the adjusted ROI to be inside of the parent matrix that is boundaries of the 01445 adjusted ROI are constrained by boundaries of the parent matrix. For example, if the submatrix A is 01446 located in the first row of a parent matrix and you called A.adjustROI(2, 2, 2, 2) then A will not 01447 be increased in the upward direction. 01448 01449 The function is used internally by the OpenCV filtering functions, like filter2D , morphological 01450 operations, and so on. 01451 @param dtop Shift of the top submatrix boundary upwards. 01452 @param dbottom Shift of the bottom submatrix boundary downwards. 01453 @param dleft Shift of the left submatrix boundary to the left. 01454 @param dright Shift of the right submatrix boundary to the right. 01455 @sa copyMakeBorder 01456 */ 01457 Mat& adjustROI( int dtop, int dbottom, int dleft, int dright ); 01458 01459 /** @brief Extracts a rectangular submatrix. 01460 01461 The operators make a new header for the specified sub-array of \*this . They are the most 01462 generalized forms of Mat::row, Mat::col, Mat::rowRange, and Mat::colRange . For example, 01463 `A(Range(0, 10), Range::all())` is equivalent to `A.rowRange(0, 10)`. Similarly to all of the above, 01464 the operators are O(1) operations, that is, no matrix data is copied. 01465 @param rowRange Start and end row of the extracted submatrix. The upper boundary is not included. To 01466 select all the rows, use Range::all(). 01467 @param colRange Start and end column of the extracted submatrix. The upper boundary is not included. 01468 To select all the columns, use Range::all(). 01469 */ 01470 Mat operator()( Range rowRange, Range colRange ) const; 01471 01472 /** @overload 01473 @param roi Extracted submatrix specified as a rectangle. 01474 */ 01475 Mat operator()( const Rect& roi ) const; 01476 01477 /** @overload 01478 @param ranges Array of selected ranges along each array dimension. 01479 */ 01480 Mat operator()( const Range* ranges ) const; 01481 01482 // //! converts header to CvMat; no data is copied 01483 // operator CvMat() const; 01484 // //! converts header to CvMatND; no data is copied 01485 // operator CvMatND() const; 01486 // //! converts header to IplImage; no data is copied 01487 // operator IplImage() const; 01488 01489 template<typename _Tp> operator std::vector<_Tp>() const; 01490 template<typename _Tp, int n> operator Vec<_Tp, n>() const; 01491 template<typename _Tp, int m, int n> operator Matx<_Tp, m, n>() const; 01492 01493 /** @brief Reports whether the matrix is continuous or not. 01494 01495 The method returns true if the matrix elements are stored continuously without gaps at the end of 01496 each row. Otherwise, it returns false. Obviously, 1x1 or 1xN matrices are always continuous. 01497 Matrices created with Mat::create are always continuous. But if you extract a part of the matrix 01498 using Mat::col, Mat::diag, and so on, or constructed a matrix header for externally allocated data, 01499 such matrices may no longer have this property. 01500 01501 The continuity flag is stored as a bit in the Mat::flags field and is computed automatically when 01502 you construct a matrix header. Thus, the continuity check is a very fast operation, though 01503 theoretically it could be done as follows: 01504 @code 01505 // alternative implementation of Mat::isContinuous() 01506 bool myCheckMatContinuity(const Mat& m) 01507 { 01508 //return (m.flags & Mat::CONTINUOUS_FLAG) != 0; 01509 return m.rows == 1 || m.step == m.cols*m.elemSize(); 01510 } 01511 @endcode 01512 The method is used in quite a few of OpenCV functions. The point is that element-wise operations 01513 (such as arithmetic and logical operations, math functions, alpha blending, color space 01514 transformations, and others) do not depend on the image geometry. Thus, if all the input and output 01515 arrays are continuous, the functions can process them as very long single-row vectors. The example 01516 below illustrates how an alpha-blending function can be implemented: 01517 @code 01518 template<typename T> 01519 void alphaBlendRGBA(const Mat& src1, const Mat& src2, Mat& dst) 01520 { 01521 const float alpha_scale = (float)std::numeric_limits<T>::max(), 01522 inv_scale = 1.f/alpha_scale; 01523 01524 CV_Assert( src1.type() == src2.type() && 01525 src1.type() == CV_MAKETYPE(DataType<T>::depth, 4) && 01526 src1.size() == src2.size()); 01527 Size size = src1.size(); 01528 dst.create(size, src1.type()); 01529 01530 // here is the idiom: check the arrays for continuity and, 01531 // if this is the case, 01532 // treat the arrays as 1D vectors 01533 if( src1.isContinuous() && src2.isContinuous() && dst.isContinuous() ) 01534 { 01535 size.width *= size.height; 01536 size.height = 1; 01537 } 01538 size.width *= 4; 01539 01540 for( int i = 0; i < size.height; i++ ) 01541 { 01542 // when the arrays are continuous, 01543 // the outer loop is executed only once 01544 const T* ptr1 = src1.ptr<T>(i); 01545 const T* ptr2 = src2.ptr<T>(i); 01546 T* dptr = dst.ptr<T>(i); 01547 01548 for( int j = 0; j < size.width; j += 4 ) 01549 { 01550 float alpha = ptr1[j+3]*inv_scale, beta = ptr2[j+3]*inv_scale; 01551 dptr[j] = saturate_cast<T>(ptr1[j]*alpha + ptr2[j]*beta); 01552 dptr[j+1] = saturate_cast<T>(ptr1[j+1]*alpha + ptr2[j+1]*beta); 01553 dptr[j+2] = saturate_cast<T>(ptr1[j+2]*alpha + ptr2[j+2]*beta); 01554 dptr[j+3] = saturate_cast<T>((1 - (1-alpha)*(1-beta))*alpha_scale); 01555 } 01556 } 01557 } 01558 @endcode 01559 This approach, while being very simple, can boost the performance of a simple element-operation by 01560 10-20 percents, especially if the image is rather small and the operation is quite simple. 01561 01562 Another OpenCV idiom in this function, a call of Mat::create for the destination array, that 01563 allocates the destination array unless it already has the proper size and type. And while the newly 01564 allocated arrays are always continuous, you still need to check the destination array because 01565 Mat::create does not always allocate a new matrix. 01566 */ 01567 bool isContinuous() const; 01568 01569 //! returns true if the matrix is a submatrix of another matrix 01570 bool isSubmatrix() const; 01571 01572 /** @brief Returns the matrix element size in bytes. 01573 01574 The method returns the matrix element size in bytes. For example, if the matrix type is CV_16SC3 , 01575 the method returns 3\*sizeof(short) or 6. 01576 */ 01577 size_t elemSize() const; 01578 01579 /** @brief Returns the size of each matrix element channel in bytes. 01580 01581 The method returns the matrix element channel size in bytes, that is, it ignores the number of 01582 channels. For example, if the matrix type is CV_16SC3 , the method returns sizeof(short) or 2. 01583 */ 01584 size_t elemSize1() const; 01585 01586 /** @brief Returns the type of a matrix element. 01587 01588 The method returns a matrix element type. This is an identifier compatible with the CvMat type 01589 system, like CV_16SC3 or 16-bit signed 3-channel array, and so on. 01590 */ 01591 int type() const; 01592 01593 /** @brief Returns the depth of a matrix element. 01594 01595 The method returns the identifier of the matrix element depth (the type of each individual channel). 01596 For example, for a 16-bit signed element array, the method returns CV_16S . A complete list of 01597 matrix types contains the following values: 01598 - CV_8U - 8-bit unsigned integers ( 0..255 ) 01599 - CV_8S - 8-bit signed integers ( -128..127 ) 01600 - CV_16U - 16-bit unsigned integers ( 0..65535 ) 01601 - CV_16S - 16-bit signed integers ( -32768..32767 ) 01602 - CV_32S - 32-bit signed integers ( -2147483648..2147483647 ) 01603 - CV_32F - 32-bit floating-point numbers ( -FLT_MAX..FLT_MAX, INF, NAN ) 01604 - CV_64F - 64-bit floating-point numbers ( -DBL_MAX..DBL_MAX, INF, NAN ) 01605 */ 01606 int depth() const; 01607 01608 /** @brief Returns the number of matrix channels. 01609 01610 The method returns the number of matrix channels. 01611 */ 01612 int channels() const; 01613 01614 /** @brief Returns a normalized step. 01615 01616 The method returns a matrix step divided by Mat::elemSize1() . It can be useful to quickly access an 01617 arbitrary matrix element. 01618 */ 01619 size_t step1(int i=0) const; 01620 01621 /** @brief Returns true if the array has no elements. 01622 01623 The method returns true if Mat::total() is 0 or if Mat::data is NULL. Because of pop_back() and 01624 resize() methods `M.total() == 0` does not imply that `M.data == NULL`. 01625 */ 01626 bool empty() const; 01627 01628 /** @brief Returns the total number of array elements. 01629 01630 The method returns the number of array elements (a number of pixels if the array represents an 01631 image). 01632 */ 01633 size_t total() const; 01634 01635 //! returns N if the matrix is 1-channel (N x ptdim) or ptdim-channel (1 x N) or (N x 1); negative number otherwise 01636 int checkVector(int elemChannels, int depth=-1, bool requireContinuous=true) const; 01637 01638 /** @brief Returns a pointer to the specified matrix row. 01639 01640 The methods return `uchar*` or typed pointer to the specified matrix row. See the sample in 01641 Mat::isContinuous to know how to use these methods. 01642 @param i0 A 0-based row index. 01643 */ 01644 uchar* ptr(int i0=0); 01645 /** @overload */ 01646 const uchar* ptr(int i0=0) const; 01647 01648 /** @overload */ 01649 uchar* ptr(int i0, int i1); 01650 /** @overload */ 01651 const uchar* ptr(int i0, int i1) const; 01652 01653 /** @overload */ 01654 uchar* ptr(int i0, int i1, int i2); 01655 /** @overload */ 01656 const uchar* ptr(int i0, int i1, int i2) const; 01657 01658 /** @overload */ 01659 uchar* ptr(const int* idx); 01660 /** @overload */ 01661 const uchar* ptr(const int* idx) const; 01662 /** @overload */ 01663 template<int n> uchar* ptr(const Vec<int, n>& idx); 01664 /** @overload */ 01665 template<int n> const uchar* ptr(const Vec<int, n>& idx) const; 01666 01667 /** @overload */ 01668 template<typename _Tp> _Tp* ptr(int i0=0); 01669 /** @overload */ 01670 template<typename _Tp> const _Tp* ptr(int i0=0) const; 01671 /** @overload */ 01672 template<typename _Tp> _Tp* ptr(int i0, int i1); 01673 /** @overload */ 01674 template<typename _Tp> const _Tp* ptr(int i0, int i1) const; 01675 /** @overload */ 01676 template<typename _Tp> _Tp* ptr(int i0, int i1, int i2); 01677 /** @overload */ 01678 template<typename _Tp> const _Tp* ptr(int i0, int i1, int i2) const; 01679 /** @overload */ 01680 template<typename _Tp> _Tp* ptr(const int* idx); 01681 /** @overload */ 01682 template<typename _Tp> const _Tp* ptr(const int* idx) const; 01683 /** @overload */ 01684 template<typename _Tp, int n> _Tp* ptr(const Vec<int, n>& idx); 01685 /** @overload */ 01686 template<typename _Tp, int n> const _Tp* ptr(const Vec<int, n>& idx) const; 01687 01688 /** @brief Returns a reference to the specified array element. 01689 01690 The template methods return a reference to the specified array element. For the sake of higher 01691 performance, the index range checks are only performed in the Debug configuration. 01692 01693 Note that the variants with a single index (i) can be used to access elements of single-row or 01694 single-column 2-dimensional arrays. That is, if, for example, A is a 1 x N floating-point matrix and 01695 B is an M x 1 integer matrix, you can simply write `A.at<float>(k+4)` and `B.at<int>(2*i+1)` 01696 instead of `A.at<float>(0,k+4)` and `B.at<int>(2*i+1,0)`, respectively. 01697 01698 The example below initializes a Hilbert matrix: 01699 @code 01700 Mat H(100, 100, CV_64F); 01701 for(int i = 0; i < H.rows; i++) 01702 for(int j = 0; j < H.cols; j++) 01703 H.at<double>(i,j)=1./(i+j+1); 01704 @endcode 01705 @param i0 Index along the dimension 0 01706 */ 01707 template<typename _Tp> _Tp& at(int i0=0); 01708 /** @overload 01709 @param i0 Index along the dimension 0 01710 */ 01711 template<typename _Tp> const _Tp& at(int i0=0) const; 01712 /** @overload 01713 @param i0 Index along the dimension 0 01714 @param i1 Index along the dimension 1 01715 */ 01716 template<typename _Tp> _Tp& at(int i0, int i1); 01717 /** @overload 01718 @param i0 Index along the dimension 0 01719 @param i1 Index along the dimension 1 01720 */ 01721 template<typename _Tp> const _Tp& at(int i0, int i1) const; 01722 01723 /** @overload 01724 @param i0 Index along the dimension 0 01725 @param i1 Index along the dimension 1 01726 @param i2 Index along the dimension 2 01727 */ 01728 template<typename _Tp> _Tp& at(int i0, int i1, int i2); 01729 /** @overload 01730 @param i0 Index along the dimension 0 01731 @param i1 Index along the dimension 1 01732 @param i2 Index along the dimension 2 01733 */ 01734 template<typename _Tp> const _Tp& at(int i0, int i1, int i2) const; 01735 01736 /** @overload 01737 @param idx Array of Mat::dims indices. 01738 */ 01739 template<typename _Tp> _Tp& at(const int* idx); 01740 /** @overload 01741 @param idx Array of Mat::dims indices. 01742 */ 01743 template<typename _Tp> const _Tp& at(const int* idx) const; 01744 01745 /** @overload */ 01746 template<typename _Tp, int n> _Tp& at(const Vec<int, n>& idx); 01747 /** @overload */ 01748 template<typename _Tp, int n> const _Tp& at(const Vec<int, n>& idx) const; 01749 01750 /** @overload 01751 special versions for 2D arrays (especially convenient for referencing image pixels) 01752 @param pt Element position specified as Point(j,i) . 01753 */ 01754 template<typename _Tp> _Tp& at(Point pt); 01755 /** @overload 01756 special versions for 2D arrays (especially convenient for referencing image pixels) 01757 @param pt Element position specified as Point(j,i) . 01758 */ 01759 template<typename _Tp> const _Tp& at(Point pt) const; 01760 01761 /** @brief Returns the matrix iterator and sets it to the first matrix element. 01762 01763 The methods return the matrix read-only or read-write iterators. The use of matrix iterators is very 01764 similar to the use of bi-directional STL iterators. In the example below, the alpha blending 01765 function is rewritten using the matrix iterators: 01766 @code 01767 template<typename T> 01768 void alphaBlendRGBA(const Mat& src1, const Mat& src2, Mat& dst) 01769 { 01770 typedef Vec<T, 4> VT; 01771 01772 const float alpha_scale = (float)std::numeric_limits<T>::max(), 01773 inv_scale = 1.f/alpha_scale; 01774 01775 CV_Assert( src1.type() == src2.type() && 01776 src1.type() == DataType<VT>::type && 01777 src1.size() == src2.size()); 01778 Size size = src1.size(); 01779 dst.create(size, src1.type()); 01780 01781 MatConstIterator_<VT> it1 = src1.begin<VT>(), it1_end = src1.end<VT>(); 01782 MatConstIterator_<VT> it2 = src2.begin<VT>(); 01783 MatIterator_<VT> dst_it = dst.begin<VT>(); 01784 01785 for( ; it1 != it1_end; ++it1, ++it2, ++dst_it ) 01786 { 01787 VT pix1 = *it1, pix2 = *it2; 01788 float alpha = pix1[3]*inv_scale, beta = pix2[3]*inv_scale; 01789 *dst_it = VT(saturate_cast<T>(pix1[0]*alpha + pix2[0]*beta), 01790 saturate_cast<T>(pix1[1]*alpha + pix2[1]*beta), 01791 saturate_cast<T>(pix1[2]*alpha + pix2[2]*beta), 01792 saturate_cast<T>((1 - (1-alpha)*(1-beta))*alpha_scale)); 01793 } 01794 } 01795 @endcode 01796 */ 01797 template<typename _Tp> MatIterator_<_Tp> begin(); 01798 template<typename _Tp> MatConstIterator_<_Tp> begin() const; 01799 01800 /** @brief Returns the matrix iterator and sets it to the after-last matrix element. 01801 01802 The methods return the matrix read-only or read-write iterators, set to the point following the last 01803 matrix element. 01804 */ 01805 template<typename _Tp> MatIterator_<_Tp> end(); 01806 template<typename _Tp> MatConstIterator_<_Tp> end() const; 01807 01808 /** @brief Invoke with arguments functor, and runs the functor over all matrix element. 01809 01810 The methods runs operation in parallel. Operation is passed by arguments. Operation have to be a 01811 function pointer, a function object or a lambda(C++11). 01812 01813 All of below operation is equal. Put 0xFF to first channel of all matrix elements: 01814 @code 01815 Mat image(1920, 1080, CV_8UC3); 01816 typedef cv::Point3_<uint8_t> Pixel; 01817 01818 // first. raw pointer access. 01819 for (int r = 0; r < image.rows; ++r) { 01820 Pixel* ptr = image.ptr<Pixel>(0, r); 01821 const Pixel* ptr_end = ptr + image.cols; 01822 for (; ptr != ptr_end; ++ptr) { 01823 ptr->x = 255; 01824 } 01825 } 01826 01827 // Using MatIterator. (Simple but there are a Iterator's overhead) 01828 for (Pixel &p : cv::Mat_<Pixel>(image)) { 01829 p.x = 255; 01830 } 01831 01832 // Parallel execution with function object. 01833 struct Operator { 01834 void operator ()(Pixel &pixel, const int * position) { 01835 pixel.x = 255; 01836 } 01837 }; 01838 image.forEach<Pixel>(Operator()); 01839 01840 // Parallel execution using C++11 lambda. 01841 image.forEach<Pixel>([](Pixel &p, const int * position) -> void { 01842 p.x = 255; 01843 }); 01844 @endcode 01845 position parameter is index of current pixel: 01846 @code 01847 // Creating 3D matrix (255 x 255 x 255) typed uint8_t, 01848 // and initialize all elements by the value which equals elements position. 01849 // i.e. pixels (x,y,z) = (1,2,3) is (b,g,r) = (1,2,3). 01850 01851 int sizes[] = { 255, 255, 255 }; 01852 typedef cv::Point3_<uint8_t> Pixel; 01853 01854 Mat_<Pixel> image = Mat::zeros(3, sizes, CV_8UC3); 01855 01856 image.forEachWithPosition([&](Pixel& pixel, const int position[]) -> void{ 01857 pixel.x = position[0]; 01858 pixel.y = position[1]; 01859 pixel.z = position[2]; 01860 }); 01861 @endcode 01862 */ 01863 template<typename _Tp, typename Functor> void forEach(const Functor& operation); 01864 /** @overload */ 01865 template<typename _Tp, typename Functor> void forEach(const Functor& operation) const; 01866 01867 #ifdef CV_CXX_MOVE_SEMANTICS 01868 Mat(Mat&& m); 01869 Mat& operator = (Mat&& m); 01870 #endif 01871 01872 enum { MAGIC_VAL = 0x42FF0000, AUTO_STEP = 0, CONTINUOUS_FLAG = CV_MAT_CONT_FLAG, SUBMATRIX_FLAG = CV_SUBMAT_FLAG }; 01873 enum { MAGIC_MASK = 0xFFFF0000, TYPE_MASK = 0x00000FFF, DEPTH_MASK = 7 }; 01874 01875 /*! includes several bit-fields: 01876 - the magic signature 01877 - continuity flag 01878 - depth 01879 - number of channels 01880 */ 01881 int flags ; 01882 //! the matrix dimensionality, >= 2 01883 int dims; 01884 //! the number of rows and columns or (-1, -1) when the matrix has more than 2 dimensions 01885 int rows, cols; 01886 //! pointer to the data 01887 uchar* data; 01888 01889 //! helper fields used in locateROI and adjustROI 01890 const uchar* datastart; 01891 const uchar* dataend; 01892 const uchar* datalimit; 01893 01894 //! custom allocator 01895 MatAllocator* allocator; 01896 //! and the standard allocator 01897 static MatAllocator* getStdAllocator(); 01898 static MatAllocator* getDefaultAllocator(); 01899 static void setDefaultAllocator(MatAllocator* allocator); 01900 01901 //! interaction with UMat 01902 UMatData* u; 01903 01904 MatSize size; 01905 MatStep step; 01906 01907 protected: 01908 template<typename _Tp, typename Functor> void forEach_impl(const Functor& operation); 01909 }; 01910 01911 01912 ///////////////////////////////// Mat_<_Tp> //////////////////////////////////// 01913 01914 /** @brief Template matrix class derived from Mat 01915 01916 @code 01917 template<typename _Tp> class Mat_ : public Mat 01918 { 01919 public: 01920 // ... some specific methods 01921 // and 01922 // no new extra fields 01923 }; 01924 @endcode 01925 The class `Mat_<_Tp>` is a *thin* template wrapper on top of the Mat class. It does not have any 01926 extra data fields. Nor this class nor Mat has any virtual methods. Thus, references or pointers to 01927 these two classes can be freely but carefully converted one to another. For example: 01928 @code 01929 // create a 100x100 8-bit matrix 01930 Mat M(100,100,CV_8U); 01931 // this will be compiled fine. no any data conversion will be done. 01932 Mat_<float>& M1 = (Mat_<float>&)M; 01933 // the program is likely to crash at the statement below 01934 M1(99,99) = 1.f; 01935 @endcode 01936 While Mat is sufficient in most cases, Mat_ can be more convenient if you use a lot of element 01937 access operations and if you know matrix type at the compilation time. Note that 01938 `Mat::at(int y,int x)` and `Mat_::operator()(int y,int x)` do absolutely the same 01939 and run at the same speed, but the latter is certainly shorter: 01940 @code 01941 Mat_<double> M(20,20); 01942 for(int i = 0; i < M.rows; i++) 01943 for(int j = 0; j < M.cols; j++) 01944 M(i,j) = 1./(i+j+1); 01945 Mat E, V; 01946 eigen(M,E,V); 01947 cout << E.at<double>(0,0)/E.at<double>(M.rows-1,0); 01948 @endcode 01949 To use Mat_ for multi-channel images/matrices, pass Vec as a Mat_ parameter: 01950 @code 01951 // allocate a 320x240 color image and fill it with green (in RGB space) 01952 Mat_<Vec3b> img(240, 320, Vec3b(0,255,0)); 01953 // now draw a diagonal white line 01954 for(int i = 0; i < 100; i++) 01955 img(i,i)=Vec3b(255,255,255); 01956 // and now scramble the 2nd (red) channel of each pixel 01957 for(int i = 0; i < img.rows; i++) 01958 for(int j = 0; j < img.cols; j++) 01959 img(i,j)[2] ^= (uchar)(i ^ j); 01960 @endcode 01961 */ 01962 template<typename _Tp> class Mat_ : public Mat 01963 { 01964 public: 01965 typedef _Tp value_type; 01966 typedef typename DataType<_Tp>::channel_type channel_type; 01967 typedef MatIterator_<_Tp> iterator; 01968 typedef MatConstIterator_<_Tp> const_iterator; 01969 01970 //! default constructor 01971 Mat_(); 01972 //! equivalent to Mat(_rows, _cols, DataType<_Tp>::type) 01973 Mat_(int _rows, int _cols); 01974 //! constructor that sets each matrix element to specified value 01975 Mat_(int _rows, int _cols, const _Tp& value); 01976 //! equivalent to Mat(_size, DataType<_Tp>::type) 01977 explicit Mat_(Size _size); 01978 //! constructor that sets each matrix element to specified value 01979 Mat_(Size _size, const _Tp& value); 01980 //! n-dim array constructor 01981 Mat_(int _ndims, const int* _sizes); 01982 //! n-dim array constructor that sets each matrix element to specified value 01983 Mat_(int _ndims, const int* _sizes, const _Tp& value); 01984 //! copy/conversion contructor. If m is of different type, it's converted 01985 Mat_(const Mat& m); 01986 //! copy constructor 01987 Mat_(const Mat_& m); 01988 //! constructs a matrix on top of user-allocated data. step is in bytes(!!!), regardless of the type 01989 Mat_(int _rows, int _cols, _Tp* _data, size_t _step=AUTO_STEP); 01990 //! constructs n-dim matrix on top of user-allocated data. steps are in bytes(!!!), regardless of the type 01991 Mat_(int _ndims, const int* _sizes, _Tp* _data, const size_t* _steps=0); 01992 //! selects a submatrix 01993 Mat_(const Mat_& m, const Range& rowRange, const Range& colRange=Range::all()); 01994 //! selects a submatrix 01995 Mat_(const Mat_& m, const Rect& roi); 01996 //! selects a submatrix, n-dim version 01997 Mat_(const Mat_& m, const Range* ranges); 01998 //! from a matrix expression 01999 explicit Mat_(const MatExpr& e); 02000 //! makes a matrix out of Vec, std::vector, Point_ or Point3_. The matrix will have a single column 02001 explicit Mat_(const std::vector<_Tp>& vec, bool copyData=false); 02002 template<int n> explicit Mat_(const Vec<typename DataType<_Tp>::channel_type, n>& vec, bool copyData=true); 02003 template<int m, int n> explicit Mat_(const Matx<typename DataType<_Tp>::channel_type, m, n>& mtx, bool copyData=true); 02004 explicit Mat_(const Point_<typename DataType<_Tp>::channel_type>& pt, bool copyData=true); 02005 explicit Mat_(const Point3_<typename DataType<_Tp>::channel_type>& pt, bool copyData=true); 02006 explicit Mat_(const MatCommaInitializer_<_Tp>& commaInitializer); 02007 02008 Mat_& operator = (const Mat& m); 02009 Mat_& operator = (const Mat_& m); 02010 //! set all the elements to s. 02011 Mat_& operator = (const _Tp& s); 02012 //! assign a matrix expression 02013 Mat_& operator = (const MatExpr& e); 02014 02015 //! iterators; they are smart enough to skip gaps in the end of rows 02016 iterator begin(); 02017 iterator end(); 02018 const_iterator begin() const; 02019 const_iterator end() const; 02020 02021 //! template methods for for operation over all matrix elements. 02022 // the operations take care of skipping gaps in the end of rows (if any) 02023 template<typename Functor> void forEach(const Functor& operation); 02024 template<typename Functor> void forEach(const Functor& operation) const; 02025 02026 //! equivalent to Mat::create(_rows, _cols, DataType<_Tp>::type) 02027 void create(int _rows, int _cols); 02028 //! equivalent to Mat::create(_size, DataType<_Tp>::type) 02029 void create(Size _size); 02030 //! equivalent to Mat::create(_ndims, _sizes, DatType<_Tp>::type) 02031 void create(int _ndims, const int* _sizes); 02032 //! cross-product 02033 Mat_ cross(const Mat_& m) const; 02034 //! data type conversion 02035 template<typename T2> operator Mat_<T2>() const; 02036 //! overridden forms of Mat::row() etc. 02037 Mat_ row(int y) const; 02038 Mat_ col(int x) const; 02039 Mat_ diag(int d=0) const; 02040 Mat_ clone() const; 02041 02042 //! overridden forms of Mat::elemSize() etc. 02043 size_t elemSize() const; 02044 size_t elemSize1() const; 02045 int type() const; 02046 int depth() const; 02047 int channels() const; 02048 size_t step1(int i=0) const; 02049 //! returns step()/sizeof(_Tp) 02050 size_t stepT(int i=0) const; 02051 02052 //! overridden forms of Mat::zeros() etc. Data type is omitted, of course 02053 static MatExpr zeros(int rows, int cols); 02054 static MatExpr zeros(Size size); 02055 static MatExpr zeros(int _ndims, const int* _sizes); 02056 static MatExpr ones(int rows, int cols); 02057 static MatExpr ones(Size size); 02058 static MatExpr ones(int _ndims, const int* _sizes); 02059 static MatExpr eye(int rows, int cols); 02060 static MatExpr eye(Size size); 02061 02062 //! some more overriden methods 02063 Mat_& adjustROI( int dtop, int dbottom, int dleft, int dright ); 02064 Mat_ operator()( const Range& rowRange, const Range& colRange ) const; 02065 Mat_ operator()( const Rect& roi ) const; 02066 Mat_ operator()( const Range* ranges ) const; 02067 02068 //! more convenient forms of row and element access operators 02069 _Tp* operator [](int y); 02070 const _Tp* operator [](int y) const; 02071 02072 //! returns reference to the specified element 02073 _Tp& operator ()(const int* idx); 02074 //! returns read-only reference to the specified element 02075 const _Tp& operator ()(const int* idx) const; 02076 02077 //! returns reference to the specified element 02078 template<int n> _Tp& operator ()(const Vec<int, n>& idx); 02079 //! returns read-only reference to the specified element 02080 template<int n> const _Tp& operator ()(const Vec<int, n>& idx) const; 02081 02082 //! returns reference to the specified element (1D case) 02083 _Tp& operator ()(int idx0); 02084 //! returns read-only reference to the specified element (1D case) 02085 const _Tp& operator ()(int idx0) const; 02086 //! returns reference to the specified element (2D case) 02087 _Tp& operator ()(int idx0, int idx1); 02088 //! returns read-only reference to the specified element (2D case) 02089 const _Tp& operator ()(int idx0, int idx1) const; 02090 //! returns reference to the specified element (3D case) 02091 _Tp& operator ()(int idx0, int idx1, int idx2); 02092 //! returns read-only reference to the specified element (3D case) 02093 const _Tp& operator ()(int idx0, int idx1, int idx2) const; 02094 02095 _Tp& operator ()(Point pt); 02096 const _Tp& operator ()(Point pt) const; 02097 02098 //! conversion to vector. 02099 operator std::vector<_Tp>() const; 02100 //! conversion to Vec 02101 template<int n> operator Vec<typename DataType<_Tp>::channel_type, n>() const; 02102 //! conversion to Matx 02103 template<int m, int n> operator Matx<typename DataType<_Tp>::channel_type, m, n>() const; 02104 02105 #ifdef CV_CXX_MOVE_SEMANTICS 02106 Mat_(Mat_&& m); 02107 Mat_& operator = (Mat_&& m); 02108 02109 Mat_(Mat&& m); 02110 Mat_& operator = (Mat&& m); 02111 02112 Mat_(MatExpr&& e); 02113 #endif 02114 }; 02115 02116 typedef Mat_<uchar> Mat1b ; 02117 typedef Mat_<Vec2b> Mat2b; 02118 typedef Mat_<Vec3b> Mat3b; 02119 typedef Mat_<Vec4b> Mat4b; 02120 02121 typedef Mat_<short> Mat1s; 02122 typedef Mat_<Vec2s> Mat2s; 02123 typedef Mat_<Vec3s> Mat3s; 02124 typedef Mat_<Vec4s> Mat4s; 02125 02126 typedef Mat_<ushort> Mat1w; 02127 typedef Mat_<Vec2w> Mat2w; 02128 typedef Mat_<Vec3w> Mat3w; 02129 typedef Mat_<Vec4w> Mat4w; 02130 02131 typedef Mat_<int> Mat1i ; 02132 typedef Mat_<Vec2i> Mat2i; 02133 typedef Mat_<Vec3i> Mat3i; 02134 typedef Mat_<Vec4i> Mat4i; 02135 02136 typedef Mat_<float> Mat1f ; 02137 typedef Mat_<Vec2f> Mat2f; 02138 typedef Mat_<Vec3f> Mat3f; 02139 typedef Mat_<Vec4f> Mat4f; 02140 02141 typedef Mat_<double> Mat1d ; 02142 typedef Mat_<Vec2d> Mat2d; 02143 typedef Mat_<Vec3d> Mat3d; 02144 typedef Mat_<Vec4d> Mat4d; 02145 02146 /** @todo document */ 02147 class CV_EXPORTS UMat 02148 { 02149 public: 02150 //! default constructor 02151 UMat (UMatUsageFlags usageFlags = USAGE_DEFAULT); 02152 //! constructs 2D matrix of the specified size and type 02153 // (_type is CV_8UC1, CV_64FC3, CV_32SC(12) etc.) 02154 UMat (int rows, int cols, int type, UMatUsageFlags usageFlags = USAGE_DEFAULT); 02155 UMat (Size size, int type, UMatUsageFlags usageFlags = USAGE_DEFAULT); 02156 //! constucts 2D matrix and fills it with the specified value _s. 02157 UMat (int rows, int cols, int type, const Scalar & s, UMatUsageFlags usageFlags = USAGE_DEFAULT); 02158 UMat (Size size, int type, const Scalar & s, UMatUsageFlags usageFlags = USAGE_DEFAULT); 02159 02160 //! constructs n-dimensional matrix 02161 UMat (int ndims, const int* sizes, int type, UMatUsageFlags usageFlags = USAGE_DEFAULT); 02162 UMat (int ndims, const int* sizes, int type, const Scalar & s, UMatUsageFlags usageFlags = USAGE_DEFAULT); 02163 02164 //! copy constructor 02165 UMat (const UMat & m); 02166 02167 //! creates a matrix header for a part of the bigger matrix 02168 UMat (const UMat & m, const Range& rowRange, const Range& colRange=Range::all()); 02169 UMat (const UMat & m, const Rect& roi); 02170 UMat (const UMat & m, const Range* ranges); 02171 //! builds matrix from std::vector with or without copying the data 02172 template<typename _Tp> explicit UMat (const std::vector<_Tp>& vec, bool copyData=false); 02173 //! builds matrix from cv::Vec; the data is copied by default 02174 template<typename _Tp, int n> explicit UMat (const Vec<_Tp, n>& vec, bool copyData=true); 02175 //! builds matrix from cv::Matx; the data is copied by default 02176 template<typename _Tp, int m, int n> explicit UMat(const Matx<_Tp, m, n>& mtx, bool copyData=true); 02177 //! builds matrix from a 2D point 02178 template<typename _Tp> explicit UMat(const Point_<_Tp>& pt, bool copyData=true); 02179 //! builds matrix from a 3D point 02180 template<typename _Tp> explicit UMat(const Point3_<_Tp>& pt, bool copyData=true); 02181 //! builds matrix from comma initializer 02182 template<typename _Tp> explicit UMat(const MatCommaInitializer_<_Tp>& commaInitializer); 02183 02184 //! destructor - calls release() 02185 ~UMat(); 02186 //! assignment operators 02187 UMat& operator = (const UMat& m); 02188 02189 Mat getMat(int flags) const; 02190 02191 //! returns a new matrix header for the specified row 02192 UMat row(int y) const; 02193 //! returns a new matrix header for the specified column 02194 UMat col(int x) const; 02195 //! ... for the specified row span 02196 UMat rowRange(int startrow, int endrow) const; 02197 UMat rowRange(const Range& r) const; 02198 //! ... for the specified column span 02199 UMat colRange(int startcol, int endcol) const; 02200 UMat colRange(const Range& r) const; 02201 //! ... for the specified diagonal 02202 // (d=0 - the main diagonal, 02203 // >0 - a diagonal from the lower half, 02204 // <0 - a diagonal from the upper half) 02205 UMat diag(int d=0) const; 02206 //! constructs a square diagonal matrix which main diagonal is vector "d" 02207 static UMat diag(const UMat& d); 02208 02209 //! returns deep copy of the matrix, i.e. the data is copied 02210 UMat clone() const; 02211 //! copies the matrix content to "m". 02212 // It calls m.create(this->size(), this->type()). 02213 void copyTo( OutputArray m ) const; 02214 //! copies those matrix elements to "m" that are marked with non-zero mask elements. 02215 void copyTo( OutputArray m, InputArray mask ) const; 02216 //! converts matrix to another datatype with optional scalng. See cvConvertScale. 02217 void convertTo( OutputArray m, int rtype, double alpha=1, double beta=0 ) const; 02218 02219 void assignTo( UMat& m, int type=-1 ) const; 02220 02221 //! sets every matrix element to s 02222 UMat& operator = (const Scalar & s); 02223 //! sets some of the matrix elements to s, according to the mask 02224 UMat& setTo(InputArray value, InputArray mask=noArray()); 02225 //! creates alternative matrix header for the same data, with different 02226 // number of channels and/or different number of rows. see cvReshape. 02227 UMat reshape(int cn, int rows=0) const; 02228 UMat reshape(int cn, int newndims, const int* newsz) const; 02229 02230 //! matrix transposition by means of matrix expressions 02231 UMat t() const; 02232 //! matrix inversion by means of matrix expressions 02233 UMat inv(int method=DECOMP_LU) const; 02234 //! per-element matrix multiplication by means of matrix expressions 02235 UMat mul(InputArray m, double scale=1) const; 02236 02237 //! computes dot-product 02238 double dot(InputArray m) const; 02239 02240 //! Matlab-style matrix initialization 02241 static UMat zeros(int rows, int cols, int type); 02242 static UMat zeros(Size size, int type); 02243 static UMat zeros(int ndims, const int* sz, int type); 02244 static UMat ones(int rows, int cols, int type); 02245 static UMat ones(Size size, int type); 02246 static UMat ones(int ndims, const int* sz, int type); 02247 static UMat eye(int rows, int cols, int type); 02248 static UMat eye(Size size, int type); 02249 02250 //! allocates new matrix data unless the matrix already has specified size and type. 02251 // previous data is unreferenced if needed. 02252 void create(int rows, int cols, int type, UMatUsageFlags usageFlags = USAGE_DEFAULT); 02253 void create(Size size, int type, UMatUsageFlags usageFlags = USAGE_DEFAULT); 02254 void create(int ndims, const int* sizes, int type, UMatUsageFlags usageFlags = USAGE_DEFAULT); 02255 02256 //! increases the reference counter; use with care to avoid memleaks 02257 void addref(); 02258 //! decreases reference counter; 02259 // deallocates the data when reference counter reaches 0. 02260 void release(); 02261 02262 //! deallocates the matrix data 02263 void deallocate(); 02264 //! internal use function; properly re-allocates _size, _step arrays 02265 void copySize(const UMat& m); 02266 02267 //! locates matrix header within a parent matrix. See below 02268 void locateROI( Size& wholeSize, Point& ofs ) const; 02269 //! moves/resizes the current matrix ROI inside the parent matrix. 02270 UMat& adjustROI( int dtop, int dbottom, int dleft, int dright ); 02271 //! extracts a rectangular sub-matrix 02272 // (this is a generalized form of row, rowRange etc.) 02273 UMat operator()( Range rowRange, Range colRange ) const; 02274 UMat operator()( const Rect& roi ) const; 02275 UMat operator()( const Range* ranges ) const; 02276 02277 //! returns true iff the matrix data is continuous 02278 // (i.e. when there are no gaps between successive rows). 02279 // similar to CV_IS_MAT_CONT(cvmat->type) 02280 bool isContinuous() const; 02281 02282 //! returns true if the matrix is a submatrix of another matrix 02283 bool isSubmatrix() const; 02284 02285 //! returns element size in bytes, 02286 // similar to CV_ELEM_SIZE(cvmat->type) 02287 size_t elemSize() const; 02288 //! returns the size of element channel in bytes. 02289 size_t elemSize1() const; 02290 //! returns element type, similar to CV_MAT_TYPE(cvmat->type) 02291 int type() const; 02292 //! returns element type, similar to CV_MAT_DEPTH(cvmat->type) 02293 int depth() const; 02294 //! returns element type, similar to CV_MAT_CN(cvmat->type) 02295 int channels() const; 02296 //! returns step/elemSize1() 02297 size_t step1(int i=0) const; 02298 //! returns true if matrix data is NULL 02299 bool empty() const; 02300 //! returns the total number of matrix elements 02301 size_t total() const; 02302 02303 //! returns N if the matrix is 1-channel (N x ptdim) or ptdim-channel (1 x N) or (N x 1); negative number otherwise 02304 int checkVector(int elemChannels, int depth=-1, bool requireContinuous=true) const; 02305 02306 #ifdef CV_CXX_MOVE_SEMANTICS 02307 UMat(UMat&& m); 02308 UMat& operator = (UMat&& m); 02309 #endif 02310 02311 void* handle(int accessFlags) const; 02312 void ndoffset(size_t* ofs) const; 02313 02314 enum { MAGIC_VAL = 0x42FF0000, AUTO_STEP = 0, CONTINUOUS_FLAG = CV_MAT_CONT_FLAG, SUBMATRIX_FLAG = CV_SUBMAT_FLAG }; 02315 enum { MAGIC_MASK = 0xFFFF0000, TYPE_MASK = 0x00000FFF, DEPTH_MASK = 7 }; 02316 02317 /*! includes several bit-fields: 02318 - the magic signature 02319 - continuity flag 02320 - depth 02321 - number of channels 02322 */ 02323 int flags ; 02324 //! the matrix dimensionality, >= 2 02325 int dims; 02326 //! the number of rows and columns or (-1, -1) when the matrix has more than 2 dimensions 02327 int rows, cols; 02328 02329 //! custom allocator 02330 MatAllocator* allocator; 02331 UMatUsageFlags usageFlags; // usage flags for allocator 02332 //! and the standard allocator 02333 static MatAllocator* getStdAllocator(); 02334 02335 // black-box container of UMat data 02336 UMatData* u; 02337 02338 // offset of the submatrix (or 0) 02339 size_t offset; 02340 02341 MatSize size; 02342 MatStep step; 02343 02344 protected: 02345 }; 02346 02347 02348 /////////////////////////// multi-dimensional sparse matrix ////////////////////////// 02349 02350 /** @brief The class SparseMat represents multi-dimensional sparse numerical arrays. 02351 02352 Such a sparse array can store elements of any type that Mat can store. *Sparse* means that only 02353 non-zero elements are stored (though, as a result of operations on a sparse matrix, some of its 02354 stored elements can actually become 0. It is up to you to detect such elements and delete them 02355 using SparseMat::erase ). The non-zero elements are stored in a hash table that grows when it is 02356 filled so that the search time is O(1) in average (regardless of whether element is there or not). 02357 Elements can be accessed using the following methods: 02358 - Query operations (SparseMat::ptr and the higher-level SparseMat::ref, SparseMat::value and 02359 SparseMat::find), for example: 02360 @code 02361 const int dims = 5; 02362 int size[] = {10, 10, 10, 10, 10}; 02363 SparseMat sparse_mat(dims, size, CV_32F); 02364 for(int i = 0; i < 1000; i++) 02365 { 02366 int idx[dims]; 02367 for(int k = 0; k < dims; k++) 02368 idx[k] = rand() 02369 sparse_mat.ref<float>(idx) += 1.f; 02370 } 02371 @endcode 02372 - Sparse matrix iterators. They are similar to MatIterator but different from NAryMatIterator. 02373 That is, the iteration loop is familiar to STL users: 02374 @code 02375 // prints elements of a sparse floating-point matrix 02376 // and the sum of elements. 02377 SparseMatConstIterator_<float> 02378 it = sparse_mat.begin<float>(), 02379 it_end = sparse_mat.end<float>(); 02380 double s = 0; 02381 int dims = sparse_mat.dims(); 02382 for(; it != it_end; ++it) 02383 { 02384 // print element indices and the element value 02385 const SparseMat::Node* n = it.node(); 02386 printf("("); 02387 for(int i = 0; i < dims; i++) 02388 printf("%d%s", n->idx[i], i < dims-1 ? ", " : ")"); 02389 printf(": %g\n", it.value<float>()); 02390 s += *it; 02391 } 02392 printf("Element sum is %g\n", s); 02393 @endcode 02394 If you run this loop, you will notice that elements are not enumerated in a logical order 02395 (lexicographical, and so on). They come in the same order as they are stored in the hash table 02396 (semi-randomly). You may collect pointers to the nodes and sort them to get the proper ordering. 02397 Note, however, that pointers to the nodes may become invalid when you add more elements to the 02398 matrix. This may happen due to possible buffer reallocation. 02399 - Combination of the above 2 methods when you need to process 2 or more sparse matrices 02400 simultaneously. For example, this is how you can compute unnormalized cross-correlation of the 2 02401 floating-point sparse matrices: 02402 @code 02403 double cross_corr(const SparseMat& a, const SparseMat& b) 02404 { 02405 const SparseMat *_a = &a, *_b = &b; 02406 // if b contains less elements than a, 02407 // it is faster to iterate through b 02408 if(_a->nzcount() > _b->nzcount()) 02409 std::swap(_a, _b); 02410 SparseMatConstIterator_<float> it = _a->begin<float>(), 02411 it_end = _a->end<float>(); 02412 double ccorr = 0; 02413 for(; it != it_end; ++it) 02414 { 02415 // take the next element from the first matrix 02416 float avalue = *it; 02417 const Node* anode = it.node(); 02418 // and try to find an element with the same index in the second matrix. 02419 // since the hash value depends only on the element index, 02420 // reuse the hash value stored in the node 02421 float bvalue = _b->value<float>(anode->idx,&anode->hashval); 02422 ccorr += avalue*bvalue; 02423 } 02424 return ccorr; 02425 } 02426 @endcode 02427 */ 02428 class CV_EXPORTS SparseMat 02429 { 02430 public: 02431 typedef SparseMatIterator iterator; 02432 typedef SparseMatConstIterator const_iterator; 02433 02434 enum { MAGIC_VAL=0x42FD0000, MAX_DIM=32, HASH_SCALE=0x5bd1e995, HASH_BIT=0x80000000 }; 02435 02436 //! the sparse matrix header 02437 struct CV_EXPORTS Hdr 02438 { 02439 Hdr(int _dims, const int* _sizes, int _type); 02440 void clear(); 02441 int refcount; 02442 int dims; 02443 int valueOffset; 02444 size_t nodeSize; 02445 size_t nodeCount; 02446 size_t freeList; 02447 std::vector<uchar> pool; 02448 std::vector<size_t> hashtab; 02449 int size[MAX_DIM]; 02450 }; 02451 02452 //! sparse matrix node - element of a hash table 02453 struct CV_EXPORTS Node 02454 { 02455 //! hash value 02456 size_t hashval; 02457 //! index of the next node in the same hash table entry 02458 size_t next; 02459 //! index of the matrix element 02460 int idx[MAX_DIM]; 02461 }; 02462 02463 /** @brief Various SparseMat constructors. 02464 */ 02465 SparseMat(); 02466 02467 /** @overload 02468 @param dims Array dimensionality. 02469 @param _sizes Sparce matrix size on all dementions. 02470 @param _type Sparse matrix data type. 02471 */ 02472 SparseMat(int dims, const int* _sizes, int _type); 02473 02474 /** @overload 02475 @param m Source matrix for copy constructor. If m is dense matrix (ocvMat) then it will be converted 02476 to sparse representation. 02477 */ 02478 SparseMat(const SparseMat& m); 02479 02480 /** @overload 02481 @param m Source matrix for copy constructor. If m is dense matrix (ocvMat) then it will be converted 02482 to sparse representation. 02483 */ 02484 explicit SparseMat(const Mat& m); 02485 02486 //! the destructor 02487 ~SparseMat(); 02488 02489 //! assignment operator. This is O(1) operation, i.e. no data is copied 02490 SparseMat& operator = (const SparseMat& m); 02491 //! equivalent to the corresponding constructor 02492 SparseMat& operator = (const Mat& m); 02493 02494 //! creates full copy of the matrix 02495 SparseMat clone() const; 02496 02497 //! copies all the data to the destination matrix. All the previous content of m is erased 02498 void copyTo( SparseMat& m ) const; 02499 //! converts sparse matrix to dense matrix. 02500 void copyTo( Mat& m ) const; 02501 //! multiplies all the matrix elements by the specified scale factor alpha and converts the results to the specified data type 02502 void convertTo( SparseMat& m, int rtype, double alpha=1 ) const; 02503 //! converts sparse matrix to dense n-dim matrix with optional type conversion and scaling. 02504 /*! 02505 @param [out] m - output matrix; if it does not have a proper size or type before the operation, 02506 it is reallocated 02507 @param [in] rtype – desired output matrix type or, rather, the depth since the number of channels 02508 are the same as the input has; if rtype is negative, the output matrix will have the 02509 same type as the input. 02510 @param [in] alpha – optional scale factor 02511 @param [in] beta – optional delta added to the scaled values 02512 */ 02513 void convertTo( Mat& m, int rtype, double alpha=1, double beta=0 ) const; 02514 02515 // not used now 02516 void assignTo( SparseMat& m, int type=-1 ) const; 02517 02518 //! reallocates sparse matrix. 02519 /*! 02520 If the matrix already had the proper size and type, 02521 it is simply cleared with clear(), otherwise, 02522 the old matrix is released (using release()) and the new one is allocated. 02523 */ 02524 void create(int dims, const int* _sizes, int _type); 02525 //! sets all the sparse matrix elements to 0, which means clearing the hash table. 02526 void clear(); 02527 //! manually increments the reference counter to the header. 02528 void addref(); 02529 // decrements the header reference counter. When the counter reaches 0, the header and all the underlying data are deallocated. 02530 void release(); 02531 02532 //! converts sparse matrix to the old-style representation; all the elements are copied. 02533 //operator CvSparseMat*() const; 02534 //! returns the size of each element in bytes (not including the overhead - the space occupied by SparseMat::Node elements) 02535 size_t elemSize() const; 02536 //! returns elemSize()/channels() 02537 size_t elemSize1() const; 02538 02539 //! returns type of sparse matrix elements 02540 int type() const; 02541 //! returns the depth of sparse matrix elements 02542 int depth() const; 02543 //! returns the number of channels 02544 int channels() const; 02545 02546 //! returns the array of sizes, or NULL if the matrix is not allocated 02547 const int* size() const; 02548 //! returns the size of i-th matrix dimension (or 0) 02549 int size(int i) const; 02550 //! returns the matrix dimensionality 02551 int dims() const; 02552 //! returns the number of non-zero elements (=the number of hash table nodes) 02553 size_t nzcount() const; 02554 02555 //! computes the element hash value (1D case) 02556 size_t hash(int i0) const; 02557 //! computes the element hash value (2D case) 02558 size_t hash(int i0, int i1) const; 02559 //! computes the element hash value (3D case) 02560 size_t hash(int i0, int i1, int i2) const; 02561 //! computes the element hash value (nD case) 02562 size_t hash(const int* idx) const; 02563 02564 //!@{ 02565 /*! 02566 specialized variants for 1D, 2D, 3D cases and the generic_type one for n-D case. 02567 return pointer to the matrix element. 02568 - if the element is there (it's non-zero), the pointer to it is returned 02569 - if it's not there and createMissing=false, NULL pointer is returned 02570 - if it's not there and createMissing=true, then the new element 02571 is created and initialized with 0. Pointer to it is returned 02572 - if the optional hashval pointer is not NULL, the element hash value is 02573 not computed, but *hashval is taken instead. 02574 */ 02575 //! returns pointer to the specified element (1D case) 02576 uchar* ptr(int i0, bool createMissing, size_t* hashval=0); 02577 //! returns pointer to the specified element (2D case) 02578 uchar* ptr(int i0, int i1, bool createMissing, size_t* hashval=0); 02579 //! returns pointer to the specified element (3D case) 02580 uchar* ptr(int i0, int i1, int i2, bool createMissing, size_t* hashval=0); 02581 //! returns pointer to the specified element (nD case) 02582 uchar* ptr(const int* idx, bool createMissing, size_t* hashval=0); 02583 //!@} 02584 02585 //!@{ 02586 /*! 02587 return read-write reference to the specified sparse matrix element. 02588 02589 `ref<_Tp>(i0,...[,hashval])` is equivalent to `*(_Tp*)ptr(i0,...,true[,hashval])`. 02590 The methods always return a valid reference. 02591 If the element did not exist, it is created and initialiazed with 0. 02592 */ 02593 //! returns reference to the specified element (1D case) 02594 template<typename _Tp> _Tp& ref(int i0, size_t* hashval=0); 02595 //! returns reference to the specified element (2D case) 02596 template<typename _Tp> _Tp& ref(int i0, int i1, size_t* hashval=0); 02597 //! returns reference to the specified element (3D case) 02598 template<typename _Tp> _Tp& ref(int i0, int i1, int i2, size_t* hashval=0); 02599 //! returns reference to the specified element (nD case) 02600 template<typename _Tp> _Tp& ref(const int* idx, size_t* hashval=0); 02601 //!@} 02602 02603 //!@{ 02604 /*! 02605 return value of the specified sparse matrix element. 02606 02607 `value<_Tp>(i0,...[,hashval])` is equivalent to 02608 @code 02609 { const _Tp* p = find<_Tp>(i0,...[,hashval]); return p ? *p : _Tp(); } 02610 @endcode 02611 02612 That is, if the element did not exist, the methods return 0. 02613 */ 02614 //! returns value of the specified element (1D case) 02615 template<typename _Tp> _Tp value(int i0, size_t* hashval=0) const; 02616 //! returns value of the specified element (2D case) 02617 template<typename _Tp> _Tp value(int i0, int i1, size_t* hashval=0) const; 02618 //! returns value of the specified element (3D case) 02619 template<typename _Tp> _Tp value(int i0, int i1, int i2, size_t* hashval=0) const; 02620 //! returns value of the specified element (nD case) 02621 template<typename _Tp> _Tp value(const int* idx, size_t* hashval=0) const; 02622 //!@} 02623 02624 //!@{ 02625 /*! 02626 Return pointer to the specified sparse matrix element if it exists 02627 02628 `find<_Tp>(i0,...[,hashval])` is equivalent to `(_const Tp*)ptr(i0,...false[,hashval])`. 02629 02630 If the specified element does not exist, the methods return NULL. 02631 */ 02632 //! returns pointer to the specified element (1D case) 02633 template<typename _Tp> const _Tp* find(int i0, size_t* hashval=0) const; 02634 //! returns pointer to the specified element (2D case) 02635 template<typename _Tp> const _Tp* find(int i0, int i1, size_t* hashval=0) const; 02636 //! returns pointer to the specified element (3D case) 02637 template<typename _Tp> const _Tp* find(int i0, int i1, int i2, size_t* hashval=0) const; 02638 //! returns pointer to the specified element (nD case) 02639 template<typename _Tp> const _Tp* find(const int* idx, size_t* hashval=0) const; 02640 //!@} 02641 02642 //! erases the specified element (2D case) 02643 void erase(int i0, int i1, size_t* hashval=0); 02644 //! erases the specified element (3D case) 02645 void erase(int i0, int i1, int i2, size_t* hashval=0); 02646 //! erases the specified element (nD case) 02647 void erase(const int* idx, size_t* hashval=0); 02648 02649 //!@{ 02650 /*! 02651 return the sparse matrix iterator pointing to the first sparse matrix element 02652 */ 02653 //! returns the sparse matrix iterator at the matrix beginning 02654 SparseMatIterator begin(); 02655 //! returns the sparse matrix iterator at the matrix beginning 02656 template<typename _Tp> SparseMatIterator_<_Tp> begin(); 02657 //! returns the read-only sparse matrix iterator at the matrix beginning 02658 SparseMatConstIterator begin() const; 02659 //! returns the read-only sparse matrix iterator at the matrix beginning 02660 template<typename _Tp> SparseMatConstIterator_<_Tp> begin() const; 02661 //!@} 02662 /*! 02663 return the sparse matrix iterator pointing to the element following the last sparse matrix element 02664 */ 02665 //! returns the sparse matrix iterator at the matrix end 02666 SparseMatIterator end(); 02667 //! returns the read-only sparse matrix iterator at the matrix end 02668 SparseMatConstIterator end() const; 02669 //! returns the typed sparse matrix iterator at the matrix end 02670 template<typename _Tp> SparseMatIterator_<_Tp> end(); 02671 //! returns the typed read-only sparse matrix iterator at the matrix end 02672 template<typename _Tp> SparseMatConstIterator_<_Tp> end() const; 02673 02674 //! returns the value stored in the sparse martix node 02675 template<typename _Tp> _Tp& value(Node* n); 02676 //! returns the value stored in the sparse martix node 02677 template<typename _Tp> const _Tp& value(const Node* n) const; 02678 02679 ////////////// some internal-use methods /////////////// 02680 Node* node(size_t nidx); 02681 const Node* node(size_t nidx) const; 02682 02683 uchar* newNode(const int* idx, size_t hashval); 02684 void removeNode(size_t hidx, size_t nidx, size_t previdx); 02685 void resizeHashTab(size_t newsize); 02686 02687 int flags; 02688 Hdr* hdr; 02689 }; 02690 02691 02692 02693 ///////////////////////////////// SparseMat_<_Tp> //////////////////////////////////// 02694 02695 /** @brief Template sparse n-dimensional array class derived from SparseMat 02696 02697 SparseMat_ is a thin wrapper on top of SparseMat created in the same way as Mat_ . It simplifies 02698 notation of some operations: 02699 @code 02700 int sz[] = {10, 20, 30}; 02701 SparseMat_<double> M(3, sz); 02702 ... 02703 M.ref(1, 2, 3) = M(4, 5, 6) + M(7, 8, 9); 02704 @endcode 02705 */ 02706 template<typename _Tp> class SparseMat_ : public SparseMat 02707 { 02708 public: 02709 typedef SparseMatIterator_<_Tp> iterator; 02710 typedef SparseMatConstIterator_<_Tp> const_iterator; 02711 02712 //! the default constructor 02713 SparseMat_(); 02714 //! the full constructor equivelent to SparseMat(dims, _sizes, DataType<_Tp>::type) 02715 SparseMat_(int dims, const int* _sizes); 02716 //! the copy constructor. If DataType<_Tp>.type != m.type(), the m elements are converted 02717 SparseMat_(const SparseMat& m); 02718 //! the copy constructor. This is O(1) operation - no data is copied 02719 SparseMat_(const SparseMat_& m); 02720 //! converts dense matrix to the sparse form 02721 SparseMat_(const Mat& m); 02722 //! converts the old-style sparse matrix to the C++ class. All the elements are copied 02723 //SparseMat_(const CvSparseMat* m); 02724 //! the assignment operator. If DataType<_Tp>.type != m.type(), the m elements are converted 02725 SparseMat_& operator = (const SparseMat& m); 02726 //! the assignment operator. This is O(1) operation - no data is copied 02727 SparseMat_& operator = (const SparseMat_& m); 02728 //! converts dense matrix to the sparse form 02729 SparseMat_& operator = (const Mat& m); 02730 02731 //! makes full copy of the matrix. All the elements are duplicated 02732 SparseMat_ clone() const; 02733 //! equivalent to cv::SparseMat::create(dims, _sizes, DataType<_Tp>::type) 02734 void create(int dims, const int* _sizes); 02735 //! converts sparse matrix to the old-style CvSparseMat. All the elements are copied 02736 //operator CvSparseMat*() const; 02737 02738 //! returns type of the matrix elements 02739 int type() const; 02740 //! returns depth of the matrix elements 02741 int depth() const; 02742 //! returns the number of channels in each matrix element 02743 int channels() const; 02744 02745 //! equivalent to SparseMat::ref<_Tp>(i0, hashval) 02746 _Tp& ref(int i0, size_t* hashval=0); 02747 //! equivalent to SparseMat::ref<_Tp>(i0, i1, hashval) 02748 _Tp& ref(int i0, int i1, size_t* hashval=0); 02749 //! equivalent to SparseMat::ref<_Tp>(i0, i1, i2, hashval) 02750 _Tp& ref(int i0, int i1, int i2, size_t* hashval=0); 02751 //! equivalent to SparseMat::ref<_Tp>(idx, hashval) 02752 _Tp& ref(const int* idx, size_t* hashval=0); 02753 02754 //! equivalent to SparseMat::value<_Tp>(i0, hashval) 02755 _Tp operator()(int i0, size_t* hashval=0) const; 02756 //! equivalent to SparseMat::value<_Tp>(i0, i1, hashval) 02757 _Tp operator()(int i0, int i1, size_t* hashval=0) const; 02758 //! equivalent to SparseMat::value<_Tp>(i0, i1, i2, hashval) 02759 _Tp operator()(int i0, int i1, int i2, size_t* hashval=0) const; 02760 //! equivalent to SparseMat::value<_Tp>(idx, hashval) 02761 _Tp operator()(const int* idx, size_t* hashval=0) const; 02762 02763 //! returns sparse matrix iterator pointing to the first sparse matrix element 02764 SparseMatIterator_<_Tp> begin(); 02765 //! returns read-only sparse matrix iterator pointing to the first sparse matrix element 02766 SparseMatConstIterator_<_Tp> begin() const; 02767 //! returns sparse matrix iterator pointing to the element following the last sparse matrix element 02768 SparseMatIterator_<_Tp> end(); 02769 //! returns read-only sparse matrix iterator pointing to the element following the last sparse matrix element 02770 SparseMatConstIterator_<_Tp> end() const; 02771 }; 02772 02773 02774 02775 ////////////////////////////////// MatConstIterator ////////////////////////////////// 02776 02777 class CV_EXPORTS MatConstIterator 02778 { 02779 public: 02780 typedef uchar* value_type; 02781 typedef ptrdiff_t difference_type; 02782 typedef const uchar** pointer; 02783 typedef uchar* reference; 02784 02785 #ifndef OPENCV_NOSTL 02786 typedef std::random_access_iterator_tag iterator_category; 02787 #endif 02788 02789 //! default constructor 02790 MatConstIterator(); 02791 //! constructor that sets the iterator to the beginning of the matrix 02792 MatConstIterator(const Mat* _m); 02793 //! constructor that sets the iterator to the specified element of the matrix 02794 MatConstIterator(const Mat* _m, int _row, int _col=0); 02795 //! constructor that sets the iterator to the specified element of the matrix 02796 MatConstIterator(const Mat* _m, Point _pt); 02797 //! constructor that sets the iterator to the specified element of the matrix 02798 MatConstIterator(const Mat* _m, const int* _idx); 02799 //! copy constructor 02800 MatConstIterator(const MatConstIterator& it); 02801 02802 //! copy operator 02803 MatConstIterator& operator = (const MatConstIterator& it); 02804 //! returns the current matrix element 02805 const uchar* operator *() const; 02806 //! returns the i-th matrix element, relative to the current 02807 const uchar* operator [](ptrdiff_t i) const; 02808 02809 //! shifts the iterator forward by the specified number of elements 02810 MatConstIterator& operator += (ptrdiff_t ofs); 02811 //! shifts the iterator backward by the specified number of elements 02812 MatConstIterator& operator -= (ptrdiff_t ofs); 02813 //! decrements the iterator 02814 MatConstIterator& operator --(); 02815 //! decrements the iterator 02816 MatConstIterator operator --(int); 02817 //! increments the iterator 02818 MatConstIterator& operator ++(); 02819 //! increments the iterator 02820 MatConstIterator operator ++(int); 02821 //! returns the current iterator position 02822 Point pos() const; 02823 //! returns the current iterator position 02824 void pos(int* _idx) const; 02825 02826 ptrdiff_t lpos() const; 02827 void seek(ptrdiff_t ofs, bool relative = false); 02828 void seek(const int* _idx, bool relative = false); 02829 02830 const Mat* m; 02831 size_t elemSize; 02832 const uchar* ptr; 02833 const uchar* sliceStart; 02834 const uchar* sliceEnd; 02835 }; 02836 02837 02838 02839 ////////////////////////////////// MatConstIterator_ ///////////////////////////////// 02840 02841 /** @brief Matrix read-only iterator 02842 */ 02843 template<typename _Tp> 02844 class MatConstIterator_ : public MatConstIterator 02845 { 02846 public: 02847 typedef _Tp value_type; 02848 typedef ptrdiff_t difference_type; 02849 typedef const _Tp* pointer; 02850 typedef const _Tp& reference; 02851 02852 #ifndef OPENCV_NOSTL 02853 typedef std::random_access_iterator_tag iterator_category; 02854 #endif 02855 02856 //! default constructor 02857 MatConstIterator_(); 02858 //! constructor that sets the iterator to the beginning of the matrix 02859 MatConstIterator_(const Mat_<_Tp>* _m); 02860 //! constructor that sets the iterator to the specified element of the matrix 02861 MatConstIterator_(const Mat_<_Tp>* _m, int _row, int _col=0); 02862 //! constructor that sets the iterator to the specified element of the matrix 02863 MatConstIterator_(const Mat_<_Tp>* _m, Point _pt); 02864 //! constructor that sets the iterator to the specified element of the matrix 02865 MatConstIterator_(const Mat_<_Tp>* _m, const int* _idx); 02866 //! copy constructor 02867 MatConstIterator_(const MatConstIterator_& it); 02868 02869 //! copy operator 02870 MatConstIterator_& operator = (const MatConstIterator_& it); 02871 //! returns the current matrix element 02872 _Tp operator *() const; 02873 //! returns the i-th matrix element, relative to the current 02874 _Tp operator [](ptrdiff_t i) const; 02875 02876 //! shifts the iterator forward by the specified number of elements 02877 MatConstIterator_& operator += (ptrdiff_t ofs); 02878 //! shifts the iterator backward by the specified number of elements 02879 MatConstIterator_& operator -= (ptrdiff_t ofs); 02880 //! decrements the iterator 02881 MatConstIterator_& operator --(); 02882 //! decrements the iterator 02883 MatConstIterator_ operator --(int); 02884 //! increments the iterator 02885 MatConstIterator_& operator ++(); 02886 //! increments the iterator 02887 MatConstIterator_ operator ++(int); 02888 //! returns the current iterator position 02889 Point pos() const; 02890 }; 02891 02892 02893 02894 //////////////////////////////////// MatIterator_ //////////////////////////////////// 02895 02896 /** @brief Matrix read-write iterator 02897 */ 02898 template<typename _Tp> 02899 class MatIterator_ : public MatConstIterator_<_Tp> 02900 { 02901 public: 02902 typedef _Tp* pointer; 02903 typedef _Tp& reference; 02904 02905 #ifndef OPENCV_NOSTL 02906 typedef std::random_access_iterator_tag iterator_category; 02907 #endif 02908 02909 //! the default constructor 02910 MatIterator_(); 02911 //! constructor that sets the iterator to the beginning of the matrix 02912 MatIterator_(Mat_<_Tp>* _m); 02913 //! constructor that sets the iterator to the specified element of the matrix 02914 MatIterator_(Mat_<_Tp>* _m, int _row, int _col=0); 02915 //! constructor that sets the iterator to the specified element of the matrix 02916 MatIterator_(Mat_<_Tp>* _m, Point _pt); 02917 //! constructor that sets the iterator to the specified element of the matrix 02918 MatIterator_(Mat_<_Tp>* _m, const int* _idx); 02919 //! copy constructor 02920 MatIterator_(const MatIterator_& it); 02921 //! copy operator 02922 MatIterator_& operator = (const MatIterator_<_Tp>& it ); 02923 02924 //! returns the current matrix element 02925 _Tp& operator *() const; 02926 //! returns the i-th matrix element, relative to the current 02927 _Tp& operator [](ptrdiff_t i) const; 02928 02929 //! shifts the iterator forward by the specified number of elements 02930 MatIterator_& operator += (ptrdiff_t ofs); 02931 //! shifts the iterator backward by the specified number of elements 02932 MatIterator_& operator -= (ptrdiff_t ofs); 02933 //! decrements the iterator 02934 MatIterator_& operator --(); 02935 //! decrements the iterator 02936 MatIterator_ operator --(int); 02937 //! increments the iterator 02938 MatIterator_& operator ++(); 02939 //! increments the iterator 02940 MatIterator_ operator ++(int); 02941 }; 02942 02943 02944 02945 /////////////////////////////// SparseMatConstIterator /////////////////////////////// 02946 02947 /** @brief Read-Only Sparse Matrix Iterator. 02948 02949 Here is how to use the iterator to compute the sum of floating-point sparse matrix elements: 02950 02951 \code 02952 SparseMatConstIterator it = m.begin(), it_end = m.end(); 02953 double s = 0; 02954 CV_Assert( m.type() == CV_32F ); 02955 for( ; it != it_end; ++it ) 02956 s += it.value<float>(); 02957 \endcode 02958 */ 02959 class CV_EXPORTS SparseMatConstIterator 02960 { 02961 public: 02962 //! the default constructor 02963 SparseMatConstIterator(); 02964 //! the full constructor setting the iterator to the first sparse matrix element 02965 SparseMatConstIterator(const SparseMat* _m); 02966 //! the copy constructor 02967 SparseMatConstIterator(const SparseMatConstIterator& it); 02968 02969 //! the assignment operator 02970 SparseMatConstIterator& operator = (const SparseMatConstIterator& it); 02971 02972 //! template method returning the current matrix element 02973 template<typename _Tp> const _Tp& value() const; 02974 //! returns the current node of the sparse matrix. it.node->idx is the current element index 02975 const SparseMat::Node* node() const; 02976 02977 //! moves iterator to the previous element 02978 SparseMatConstIterator& operator --(); 02979 //! moves iterator to the previous element 02980 SparseMatConstIterator operator --(int); 02981 //! moves iterator to the next element 02982 SparseMatConstIterator& operator ++(); 02983 //! moves iterator to the next element 02984 SparseMatConstIterator operator ++(int); 02985 02986 //! moves iterator to the element after the last element 02987 void seekEnd(); 02988 02989 const SparseMat* m; 02990 size_t hashidx; 02991 uchar* ptr; 02992 }; 02993 02994 02995 02996 ////////////////////////////////// SparseMatIterator ///////////////////////////////// 02997 02998 /** @brief Read-write Sparse Matrix Iterator 02999 03000 The class is similar to cv::SparseMatConstIterator, 03001 but can be used for in-place modification of the matrix elements. 03002 */ 03003 class CV_EXPORTS SparseMatIterator : public SparseMatConstIterator 03004 { 03005 public: 03006 //! the default constructor 03007 SparseMatIterator(); 03008 //! the full constructor setting the iterator to the first sparse matrix element 03009 SparseMatIterator(SparseMat* _m); 03010 //! the full constructor setting the iterator to the specified sparse matrix element 03011 SparseMatIterator(SparseMat* _m, const int* idx); 03012 //! the copy constructor 03013 SparseMatIterator(const SparseMatIterator& it); 03014 03015 //! the assignment operator 03016 SparseMatIterator& operator = (const SparseMatIterator& it); 03017 //! returns read-write reference to the current sparse matrix element 03018 template<typename _Tp> _Tp& value() const; 03019 //! returns pointer to the current sparse matrix node. it.node->idx is the index of the current element (do not modify it!) 03020 SparseMat::Node* node() const; 03021 03022 //! moves iterator to the next element 03023 SparseMatIterator& operator ++(); 03024 //! moves iterator to the next element 03025 SparseMatIterator operator ++(int); 03026 }; 03027 03028 03029 03030 /////////////////////////////// SparseMatConstIterator_ ////////////////////////////// 03031 03032 /** @brief Template Read-Only Sparse Matrix Iterator Class. 03033 03034 This is the derived from SparseMatConstIterator class that 03035 introduces more convenient operator *() for accessing the current element. 03036 */ 03037 template<typename _Tp> class SparseMatConstIterator_ : public SparseMatConstIterator 03038 { 03039 public: 03040 03041 #ifndef OPENCV_NOSTL 03042 typedef std::forward_iterator_tag iterator_category; 03043 #endif 03044 03045 //! the default constructor 03046 SparseMatConstIterator_(); 03047 //! the full constructor setting the iterator to the first sparse matrix element 03048 SparseMatConstIterator_(const SparseMat_<_Tp>* _m); 03049 SparseMatConstIterator_(const SparseMat* _m); 03050 //! the copy constructor 03051 SparseMatConstIterator_(const SparseMatConstIterator_& it); 03052 03053 //! the assignment operator 03054 SparseMatConstIterator_& operator = (const SparseMatConstIterator_& it); 03055 //! the element access operator 03056 const _Tp& operator *() const; 03057 03058 //! moves iterator to the next element 03059 SparseMatConstIterator_& operator ++(); 03060 //! moves iterator to the next element 03061 SparseMatConstIterator_ operator ++(int); 03062 }; 03063 03064 03065 03066 ///////////////////////////////// SparseMatIterator_ ///////////////////////////////// 03067 03068 /** @brief Template Read-Write Sparse Matrix Iterator Class. 03069 03070 This is the derived from cv::SparseMatConstIterator_ class that 03071 introduces more convenient operator *() for accessing the current element. 03072 */ 03073 template<typename _Tp> class SparseMatIterator_ : public SparseMatConstIterator_<_Tp> 03074 { 03075 public: 03076 03077 #ifndef OPENCV_NOSTL 03078 typedef std::forward_iterator_tag iterator_category; 03079 #endif 03080 03081 //! the default constructor 03082 SparseMatIterator_(); 03083 //! the full constructor setting the iterator to the first sparse matrix element 03084 SparseMatIterator_(SparseMat_<_Tp>* _m); 03085 SparseMatIterator_(SparseMat* _m); 03086 //! the copy constructor 03087 SparseMatIterator_(const SparseMatIterator_& it); 03088 03089 //! the assignment operator 03090 SparseMatIterator_& operator = (const SparseMatIterator_& it); 03091 //! returns the reference to the current element 03092 _Tp& operator *() const; 03093 03094 //! moves the iterator to the next element 03095 SparseMatIterator_& operator ++(); 03096 //! moves the iterator to the next element 03097 SparseMatIterator_ operator ++(int); 03098 }; 03099 03100 03101 03102 /////////////////////////////////// NAryMatIterator ////////////////////////////////// 03103 03104 /** @brief n-ary multi-dimensional array iterator. 03105 03106 Use the class to implement unary, binary, and, generally, n-ary element-wise operations on 03107 multi-dimensional arrays. Some of the arguments of an n-ary function may be continuous arrays, some 03108 may be not. It is possible to use conventional MatIterator 's for each array but incrementing all of 03109 the iterators after each small operations may be a big overhead. In this case consider using 03110 NAryMatIterator to iterate through several matrices simultaneously as long as they have the same 03111 geometry (dimensionality and all the dimension sizes are the same). On each iteration `it.planes[0]`, 03112 `it.planes[1]`,... will be the slices of the corresponding matrices. 03113 03114 The example below illustrates how you can compute a normalized and threshold 3D color histogram: 03115 @code 03116 void computeNormalizedColorHist(const Mat& image, Mat& hist, int N, double minProb) 03117 { 03118 const int histSize[] = {N, N, N}; 03119 03120 // make sure that the histogram has a proper size and type 03121 hist.create(3, histSize, CV_32F); 03122 03123 // and clear it 03124 hist = Scalar(0); 03125 03126 // the loop below assumes that the image 03127 // is a 8-bit 3-channel. check it. 03128 CV_Assert(image.type() == CV_8UC3); 03129 MatConstIterator_<Vec3b> it = image.begin<Vec3b>(), 03130 it_end = image.end<Vec3b>(); 03131 for( ; it != it_end; ++it ) 03132 { 03133 const Vec3b& pix = *it; 03134 hist.at<float>(pix[0]*N/256, pix[1]*N/256, pix[2]*N/256) += 1.f; 03135 } 03136 03137 minProb *= image.rows*image.cols; 03138 Mat plane; 03139 NAryMatIterator it(&hist, &plane, 1); 03140 double s = 0; 03141 // iterate through the matrix. on each iteration 03142 // it.planes[*] (of type Mat) will be set to the current plane. 03143 for(int p = 0; p < it.nplanes; p++, ++it) 03144 { 03145 threshold(it.planes[0], it.planes[0], minProb, 0, THRESH_TOZERO); 03146 s += sum(it.planes[0])[0]; 03147 } 03148 03149 s = 1./s; 03150 it = NAryMatIterator(&hist, &plane, 1); 03151 for(int p = 0; p < it.nplanes; p++, ++it) 03152 it.planes[0] *= s; 03153 } 03154 @endcode 03155 */ 03156 class CV_EXPORTS NAryMatIterator 03157 { 03158 public: 03159 //! the default constructor 03160 NAryMatIterator(); 03161 //! the full constructor taking arbitrary number of n-dim matrices 03162 NAryMatIterator(const Mat** arrays, uchar** ptrs, int narrays=-1); 03163 //! the full constructor taking arbitrary number of n-dim matrices 03164 NAryMatIterator(const Mat** arrays, Mat* planes, int narrays=-1); 03165 //! the separate iterator initialization method 03166 void init(const Mat** arrays, Mat* planes, uchar** ptrs, int narrays=-1); 03167 03168 //! proceeds to the next plane of every iterated matrix 03169 NAryMatIterator& operator ++(); 03170 //! proceeds to the next plane of every iterated matrix (postfix increment operator) 03171 NAryMatIterator operator ++(int); 03172 03173 //! the iterated arrays 03174 const Mat** arrays; 03175 //! the current planes 03176 Mat* planes; 03177 //! data pointers 03178 uchar** ptrs; 03179 //! the number of arrays 03180 int narrays; 03181 //! the number of hyper-planes that the iterator steps through 03182 size_t nplanes; 03183 //! the size of each segment (in elements) 03184 size_t size; 03185 protected: 03186 int iterdepth; 03187 size_t idx; 03188 }; 03189 03190 03191 03192 ///////////////////////////////// Matrix Expressions ///////////////////////////////// 03193 03194 class CV_EXPORTS MatOp 03195 { 03196 public: 03197 MatOp(); 03198 virtual ~MatOp(); 03199 03200 virtual bool elementWise(const MatExpr& expr) const; 03201 virtual void assign(const MatExpr& expr, Mat& m, int type=-1) const = 0; 03202 virtual void roi(const MatExpr& expr, const Range& rowRange, 03203 const Range& colRange, MatExpr& res) const; 03204 virtual void diag(const MatExpr& expr, int d, MatExpr& res) const; 03205 virtual void augAssignAdd(const MatExpr& expr, Mat& m) const; 03206 virtual void augAssignSubtract(const MatExpr& expr, Mat& m) const; 03207 virtual void augAssignMultiply(const MatExpr& expr, Mat& m) const; 03208 virtual void augAssignDivide(const MatExpr& expr, Mat& m) const; 03209 virtual void augAssignAnd(const MatExpr& expr, Mat& m) const; 03210 virtual void augAssignOr(const MatExpr& expr, Mat& m) const; 03211 virtual void augAssignXor(const MatExpr& expr, Mat& m) const; 03212 03213 virtual void add(const MatExpr& expr1, const MatExpr& expr2, MatExpr& res) const; 03214 virtual void add(const MatExpr& expr1, const Scalar & s, MatExpr& res) const; 03215 03216 virtual void subtract(const MatExpr& expr1, const MatExpr& expr2, MatExpr& res) const; 03217 virtual void subtract(const Scalar & s, const MatExpr& expr, MatExpr& res) const; 03218 03219 virtual void multiply(const MatExpr& expr1, const MatExpr& expr2, MatExpr& res, double scale=1) const; 03220 virtual void multiply(const MatExpr& expr1, double s, MatExpr& res) const; 03221 03222 virtual void divide(const MatExpr& expr1, const MatExpr& expr2, MatExpr& res, double scale=1) const; 03223 virtual void divide(double s, const MatExpr& expr, MatExpr& res) const; 03224 03225 virtual void abs(const MatExpr& expr, MatExpr& res) const; 03226 03227 virtual void transpose(const MatExpr& expr, MatExpr& res) const; 03228 virtual void matmul(const MatExpr& expr1, const MatExpr& expr2, MatExpr& res) const; 03229 virtual void invert(const MatExpr& expr, int method, MatExpr& res) const; 03230 03231 virtual Size size(const MatExpr& expr) const; 03232 virtual int type(const MatExpr& expr) const; 03233 }; 03234 03235 /** @brief Matrix expression representation 03236 @anchor MatrixExpressions 03237 This is a list of implemented matrix operations that can be combined in arbitrary complex 03238 expressions (here A, B stand for matrices ( Mat ), s for a scalar ( Scalar ), alpha for a 03239 real-valued scalar ( double )): 03240 - Addition, subtraction, negation: `A+B`, `A-B`, `A+s`, `A-s`, `s+A`, `s-A`, `-A` 03241 - Scaling: `A*alpha` 03242 - Per-element multiplication and division: `A.mul(B)`, `A/B`, `alpha/A` 03243 - Matrix multiplication: `A*B` 03244 - Transposition: `A.t()` (means A<sup>T</sup>) 03245 - Matrix inversion and pseudo-inversion, solving linear systems and least-squares problems: 03246 `A.inv([method]) (~ A<sup>-1</sup>)`, `A.inv([method])*B (~ X: AX=B)` 03247 - Comparison: `A cmpop B`, `A cmpop alpha`, `alpha cmpop A`, where *cmpop* is one of 03248 `>`, `>=`, `==`, `!=`, `<=`, `<`. The result of comparison is an 8-bit single channel mask whose 03249 elements are set to 255 (if the particular element or pair of elements satisfy the condition) or 03250 0. 03251 - Bitwise logical operations: `A logicop B`, `A logicop s`, `s logicop A`, `~A`, where *logicop* is one of 03252 `&`, `|`, `^`. 03253 - Element-wise minimum and maximum: `min(A, B)`, `min(A, alpha)`, `max(A, B)`, `max(A, alpha)` 03254 - Element-wise absolute value: `abs(A)` 03255 - Cross-product, dot-product: `A.cross(B)`, `A.dot(B)` 03256 - Any function of matrix or matrices and scalars that returns a matrix or a scalar, such as norm, 03257 mean, sum, countNonZero, trace, determinant, repeat, and others. 03258 - Matrix initializers ( Mat::eye(), Mat::zeros(), Mat::ones() ), matrix comma-separated 03259 initializers, matrix constructors and operators that extract sub-matrices (see Mat description). 03260 - Mat_<destination_type>() constructors to cast the result to the proper type. 03261 @note Comma-separated initializers and probably some other operations may require additional 03262 explicit Mat() or Mat_<T>() constructor calls to resolve a possible ambiguity. 03263 03264 Here are examples of matrix expressions: 03265 @code 03266 // compute pseudo-inverse of A, equivalent to A.inv(DECOMP_SVD) 03267 SVD svd(A); 03268 Mat pinvA = svd.vt.t()*Mat::diag(1./svd.w)*svd.u.t(); 03269 03270 // compute the new vector of parameters in the Levenberg-Marquardt algorithm 03271 x -= (A.t()*A + lambda*Mat::eye(A.cols,A.cols,A.type())).inv(DECOMP_CHOLESKY)*(A.t()*err); 03272 03273 // sharpen image using "unsharp mask" algorithm 03274 Mat blurred; double sigma = 1, threshold = 5, amount = 1; 03275 GaussianBlur(img, blurred, Size(), sigma, sigma); 03276 Mat lowContrastMask = abs(img - blurred) < threshold; 03277 Mat sharpened = img*(1+amount) + blurred*(-amount); 03278 img.copyTo(sharpened, lowContrastMask); 03279 @endcode 03280 */ 03281 class CV_EXPORTS MatExpr 03282 { 03283 public: 03284 MatExpr(); 03285 explicit MatExpr(const Mat& m); 03286 03287 MatExpr(const MatOp* _op, int _flags, const Mat& _a = Mat(), const Mat& _b = Mat(), 03288 const Mat& _c = Mat(), double _alpha = 1, double _beta = 1, const Scalar & _s = Scalar ()); 03289 03290 operator Mat() const; 03291 template<typename _Tp> operator Mat_<_Tp>() const; 03292 03293 Size size() const; 03294 int type() const; 03295 03296 MatExpr row(int y) const; 03297 MatExpr col(int x) const; 03298 MatExpr diag(int d = 0) const; 03299 MatExpr operator()( const Range& rowRange, const Range& colRange ) const; 03300 MatExpr operator()( const Rect& roi ) const; 03301 03302 MatExpr t() const; 03303 MatExpr inv(int method = DECOMP_LU) const; 03304 MatExpr mul(const MatExpr& e, double scale=1) const; 03305 MatExpr mul(const Mat& m, double scale=1) const; 03306 03307 Mat cross(const Mat& m) const; 03308 double dot(const Mat& m) const; 03309 03310 const MatOp* op; 03311 int flags; 03312 03313 Mat a, b, c; 03314 double alpha, beta; 03315 Scalar s; 03316 }; 03317 03318 //! @} core_basic 03319 03320 //! @relates cv::MatExpr 03321 //! @{ 03322 CV_EXPORTS MatExpr operator + (const Mat& a, const Mat& b); 03323 CV_EXPORTS MatExpr operator + (const Mat& a, const Scalar & s); 03324 CV_EXPORTS MatExpr operator + (const Scalar & s, const Mat& a); 03325 CV_EXPORTS MatExpr operator + (const MatExpr& e, const Mat& m); 03326 CV_EXPORTS MatExpr operator + (const Mat& m, const MatExpr& e); 03327 CV_EXPORTS MatExpr operator + (const MatExpr& e, const Scalar & s); 03328 CV_EXPORTS MatExpr operator + (const Scalar & s, const MatExpr& e); 03329 CV_EXPORTS MatExpr operator + (const MatExpr& e1, const MatExpr& e2); 03330 03331 CV_EXPORTS MatExpr operator - (const Mat& a, const Mat& b); 03332 CV_EXPORTS MatExpr operator - (const Mat& a, const Scalar & s); 03333 CV_EXPORTS MatExpr operator - (const Scalar & s, const Mat& a); 03334 CV_EXPORTS MatExpr operator - (const MatExpr& e, const Mat& m); 03335 CV_EXPORTS MatExpr operator - (const Mat& m, const MatExpr& e); 03336 CV_EXPORTS MatExpr operator - (const MatExpr& e, const Scalar & s); 03337 CV_EXPORTS MatExpr operator - (const Scalar & s, const MatExpr& e); 03338 CV_EXPORTS MatExpr operator - (const MatExpr& e1, const MatExpr& e2); 03339 03340 CV_EXPORTS MatExpr operator - (const Mat& m); 03341 CV_EXPORTS MatExpr operator - (const MatExpr& e); 03342 03343 CV_EXPORTS MatExpr operator * (const Mat& a, const Mat& b); 03344 CV_EXPORTS MatExpr operator * (const Mat& a, double s); 03345 CV_EXPORTS MatExpr operator * (double s, const Mat& a); 03346 CV_EXPORTS MatExpr operator * (const MatExpr& e, const Mat& m); 03347 CV_EXPORTS MatExpr operator * (const Mat& m, const MatExpr& e); 03348 CV_EXPORTS MatExpr operator * (const MatExpr& e, double s); 03349 CV_EXPORTS MatExpr operator * (double s, const MatExpr& e); 03350 CV_EXPORTS MatExpr operator * (const MatExpr& e1, const MatExpr& e2); 03351 03352 CV_EXPORTS MatExpr operator / (const Mat& a, const Mat& b); 03353 CV_EXPORTS MatExpr operator / (const Mat& a, double s); 03354 CV_EXPORTS MatExpr operator / (double s, const Mat& a); 03355 CV_EXPORTS MatExpr operator / (const MatExpr& e, const Mat& m); 03356 CV_EXPORTS MatExpr operator / (const Mat& m, const MatExpr& e); 03357 CV_EXPORTS MatExpr operator / (const MatExpr& e, double s); 03358 CV_EXPORTS MatExpr operator / (double s, const MatExpr& e); 03359 CV_EXPORTS MatExpr operator / (const MatExpr& e1, const MatExpr& e2); 03360 03361 CV_EXPORTS MatExpr operator < (const Mat& a, const Mat& b); 03362 CV_EXPORTS MatExpr operator < (const Mat& a, double s); 03363 CV_EXPORTS MatExpr operator < (double s, const Mat& a); 03364 03365 CV_EXPORTS MatExpr operator <= (const Mat& a, const Mat& b); 03366 CV_EXPORTS MatExpr operator <= (const Mat& a, double s); 03367 CV_EXPORTS MatExpr operator <= (double s, const Mat& a); 03368 03369 CV_EXPORTS MatExpr operator == (const Mat& a, const Mat& b); 03370 CV_EXPORTS MatExpr operator == (const Mat& a, double s); 03371 CV_EXPORTS MatExpr operator == (double s, const Mat& a); 03372 03373 CV_EXPORTS MatExpr operator != (const Mat& a, const Mat& b); 03374 CV_EXPORTS MatExpr operator != (const Mat& a, double s); 03375 CV_EXPORTS MatExpr operator != (double s, const Mat& a); 03376 03377 CV_EXPORTS MatExpr operator >= (const Mat& a, const Mat& b); 03378 CV_EXPORTS MatExpr operator >= (const Mat& a, double s); 03379 CV_EXPORTS MatExpr operator >= (double s, const Mat& a); 03380 03381 CV_EXPORTS MatExpr operator > (const Mat& a, const Mat& b); 03382 CV_EXPORTS MatExpr operator > (const Mat& a, double s); 03383 CV_EXPORTS MatExpr operator > (double s, const Mat& a); 03384 03385 CV_EXPORTS MatExpr operator & (const Mat& a, const Mat& b); 03386 CV_EXPORTS MatExpr operator & (const Mat& a, const Scalar & s); 03387 CV_EXPORTS MatExpr operator & (const Scalar & s, const Mat& a); 03388 03389 CV_EXPORTS MatExpr operator | (const Mat& a, const Mat& b); 03390 CV_EXPORTS MatExpr operator | (const Mat& a, const Scalar & s); 03391 CV_EXPORTS MatExpr operator | (const Scalar & s, const Mat& a); 03392 03393 CV_EXPORTS MatExpr operator ^ (const Mat& a, const Mat& b); 03394 CV_EXPORTS MatExpr operator ^ (const Mat& a, const Scalar & s); 03395 CV_EXPORTS MatExpr operator ^ (const Scalar & s, const Mat& a); 03396 03397 CV_EXPORTS MatExpr operator ~(const Mat& m); 03398 03399 CV_EXPORTS MatExpr min(const Mat& a, const Mat& b); 03400 CV_EXPORTS MatExpr min(const Mat& a, double s); 03401 CV_EXPORTS MatExpr min(double s, const Mat& a); 03402 03403 CV_EXPORTS MatExpr max(const Mat& a, const Mat& b); 03404 CV_EXPORTS MatExpr max(const Mat& a, double s); 03405 CV_EXPORTS MatExpr max(double s, const Mat& a); 03406 03407 /** @brief Calculates an absolute value of each matrix element. 03408 03409 abs is a meta-function that is expanded to one of absdiff or convertScaleAbs forms: 03410 - C = abs(A-B) is equivalent to `absdiff(A, B, C)` 03411 - C = abs(A) is equivalent to `absdiff(A, Scalar::all(0), C)` 03412 - C = `Mat_<Vec<uchar,n> >(abs(A*alpha + beta))` is equivalent to `convertScaleAbs(A, C, alpha, 03413 beta)` 03414 03415 The output matrix has the same size and the same type as the input one except for the last case, 03416 where C is depth=CV_8U . 03417 @param m matrix. 03418 @sa @ref MatrixExpressions, absdiff, convertScaleAbs 03419 */ 03420 CV_EXPORTS MatExpr abs(const Mat& m); 03421 /** @overload 03422 @param e matrix expression. 03423 */ 03424 CV_EXPORTS MatExpr abs(const MatExpr& e); 03425 //! @} relates cv::MatExpr 03426 03427 } // cv 03428 03429 #include "opencv2/core/mat.inl.hpp" 03430 03431 #endif // __OPENCV_CORE_MAT_HPP__ 03432
Generated on Tue Jul 12 2022 16:42:38 by
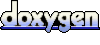