
opencv on mbed
NAryMatIterator Class Reference
[Basic structures]
n-ary multi-dimensional array iterator. More...
#include <mat.hpp>
Public Member Functions | |
NAryMatIterator () | |
the default constructor | |
NAryMatIterator (const Mat **arrays, uchar **ptrs, int narrays=-1) | |
the full constructor taking arbitrary number of n-dim matrices | |
NAryMatIterator (const Mat **arrays, Mat *planes, int narrays=-1) | |
the full constructor taking arbitrary number of n-dim matrices | |
void | init (const Mat **arrays, Mat *planes, uchar **ptrs, int narrays=-1) |
the separate iterator initialization method | |
NAryMatIterator & | operator++ () |
proceeds to the next plane of every iterated matrix | |
NAryMatIterator | operator++ (int) |
proceeds to the next plane of every iterated matrix (postfix increment operator) | |
Data Fields | |
const Mat ** | arrays |
the iterated arrays | |
Mat * | planes |
the current planes | |
uchar ** | ptrs |
data pointers | |
int | narrays |
the number of arrays | |
size_t | nplanes |
the number of hyper-planes that the iterator steps through | |
size_t | size |
the size of each segment (in elements) |
Detailed Description
n-ary multi-dimensional array iterator.
Use the class to implement unary, binary, and, generally, n-ary element-wise operations on multi-dimensional arrays. Some of the arguments of an n-ary function may be continuous arrays, some may be not. It is possible to use conventional MatIterator 's for each array but incrementing all of the iterators after each small operations may be a big overhead. In this case consider using NAryMatIterator to iterate through several matrices simultaneously as long as they have the same geometry (dimensionality and all the dimension sizes are the same). On each iteration `it.planes[0]`, `it.planes[1]`,... will be the slices of the corresponding matrices.
The example below illustrates how you can compute a normalized and threshold 3D color histogram:
void computeNormalizedColorHist(const Mat& image, Mat& hist, int N, double minProb) { const int histSize[] = {N, N, N}; // make sure that the histogram has a proper size and type hist.create(3, histSize, CV_32F); // and clear it hist = Scalar(0); // the loop below assumes that the image // is a 8-bit 3-channel. check it. CV_Assert(image.type() == CV_8UC3); MatConstIterator_<Vec3b> it = image.begin<Vec3b>(), it_end = image.end<Vec3b>(); for( ; it != it_end; ++it ) { const Vec3b& pix = *it; hist.at<float>(pix[0]*N/256, pix[1]*N/256, pix[2]*N/256) += 1.f; } minProb *= image.rows*image.cols; Mat plane; NAryMatIterator it(&hist, &plane, 1); double s = 0; // iterate through the matrix. on each iteration // it.planes[*] (of type Mat) will be set to the current plane. for(int p = 0; p < it.nplanes; p++, ++it) { threshold(it.planes[0], it.planes[0], minProb, 0, THRESH_TOZERO ); s += sum(it.planes[0])[0]; } s = 1./s; it = NAryMatIterator(&hist, &plane, 1); for(int p = 0; p < it.nplanes; p++, ++it) it.planes[0] *= s; }
Definition at line 3156 of file mat.hpp.
Constructor & Destructor Documentation
NAryMatIterator | ( | ) |
the default constructor
NAryMatIterator | ( | const Mat ** | arrays, |
uchar ** | ptrs, | ||
int | narrays = -1 |
||
) |
the full constructor taking arbitrary number of n-dim matrices
NAryMatIterator | ( | const Mat ** | arrays, |
Mat * | planes, | ||
int | narrays = -1 |
||
) |
the full constructor taking arbitrary number of n-dim matrices
Member Function Documentation
the separate iterator initialization method
NAryMatIterator operator++ | ( | int | ) |
proceeds to the next plane of every iterated matrix (postfix increment operator)
NAryMatIterator& operator++ | ( | ) |
proceeds to the next plane of every iterated matrix
Field Documentation
size_t nplanes |
Generated on Tue Jul 12 2022 16:42:43 by
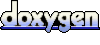