
opencv on mbed
Scalar_< _Tp > Class Template Reference
[Basic structures]
Template class for a 4-element vector derived from Vec. More...
#include <types.hpp>
Inherits Vec< _Tp, 4 >.
Public Member Functions | |
Scalar_ () | |
various constructors | |
template<typename T2 > | |
operator Scalar_< T2 > () const | |
conversion to another data type | |
Scalar_< _Tp > | mul (const Scalar_< _Tp > &a, double scale=1) const |
per-element product | |
Scalar_< _Tp > | conj () const |
conjugation (makes sense for complex numbers and quaternions) | |
Vec | mul (const Vec< _Tp, cn > &v) const |
per-element multiplication | |
Matx< _Tp, m, n > | mul (const Matx< _Tp, m, n > &a) const |
multiply two matrices element-wise | |
Vec | cross (const Vec &v) const |
operator Vec< T2, cn > () const | |
conversion to another data type | |
const _Tp & | operator[] (int i) const |
const _Tp & | operator() (int i) const |
1D element access | |
const _Tp & | operator() (int i, int j) const |
element access | |
diag_type | diag () const |
extract the matrix diagonal | |
_Tp | dot (const Matx< _Tp, m, n > &v) const |
dot product computed with the default precision | |
double | ddot (const Matx< _Tp, m, n > &v) const |
dot product computed in double-precision arithmetics | |
operator Matx< T2, m, n > () const | |
conversion to another data type | |
Matx< _Tp, m1, n1 > | reshape () const |
change the matrix shape | |
Matx< _Tp, m1, n1 > | get_minor (int i, int j) const |
extract part of the matrix | |
Matx< _Tp, 1, n > | row (int i) const |
extract the matrix row | |
Matx< _Tp, m, 1 > | col (int i) const |
extract the matrix column | |
Matx< _Tp, n, m > | t () const |
transpose the matrix | |
Matx< _Tp, n, m > | inv (int method=DECOMP_LU, bool *p_is_ok=NULL) const |
invert the matrix | |
Matx< _Tp, n, l > | solve (const Matx< _Tp, m, l > &rhs, int flags=DECOMP_LU) const |
solve linear system | |
Matx< _Tp, m, n > | div (const Matx< _Tp, m, n > &a) const |
divide two matrices element-wise | |
Static Public Member Functions | |
static Scalar_< _Tp > | all (_Tp v0) |
returns a scalar with all elements set to v0 |
Detailed Description
template<typename _Tp>
class cv::Scalar_< _Tp >
Template class for a 4-element vector derived from Vec.
Being derived from Vec<_Tp, 4> , Scalar_ and Scalar can be used just as typical 4-element vectors. In addition, they can be converted to/from CvScalar . The type Scalar is widely used in OpenCV to pass pixel values.
Definition at line 570 of file types.hpp.
Constructor & Destructor Documentation
Scalar_ | ( | ) |
various constructors
Member Function Documentation
static Scalar_<_Tp> all | ( | _Tp | v0 ) | [static] |
returns a scalar with all elements set to v0
Reimplemented from Vec< _Tp, 4 >.
Matx<_Tp, m, 1> col | ( | int | i ) | const [inherited] |
extract the matrix column
Scalar_<_Tp> conj | ( | ) | const |
conjugation (makes sense for complex numbers and quaternions)
Reimplemented from Vec< _Tp, 4 >.
cross product of the two 3D vectors.
For other dimensionalities the exception is raised
double ddot | ( | const Matx< _Tp, m, n > & | v ) | const [inherited] |
dot product computed in double-precision arithmetics
diag_type diag | ( | ) | const [inherited] |
extract the matrix diagonal
divide two matrices element-wise
_Tp dot | ( | const Matx< _Tp, m, n > & | v ) | const [inherited] |
dot product computed with the default precision
Matx<_Tp, m1, n1> get_minor | ( | int | i, |
int | j | ||
) | const [inherited] |
extract part of the matrix
Matx<_Tp, n, m> inv | ( | int | method = DECOMP_LU , |
bool * | p_is_ok = NULL |
||
) | const [inherited] |
invert the matrix
multiply two matrices element-wise
operator Matx< T2, m, n > | ( | ) | const [inherited] |
conversion to another data type
operator Scalar_< T2 > | ( | ) | const |
conversion to another data type
operator Vec< T2, cn > | ( | ) | const [inherited] |
conversion to another data type
const _Tp& operator() | ( | int | i, |
int | j | ||
) | const [inherited] |
element access
const _Tp& operator() | ( | int | i ) | const [inherited] |
1D element access
Reimplemented from Matx< _Tp, cn, 1 >.
const _Tp& operator[] | ( | int | i ) | const [inherited] |
element access
Matx<_Tp, m1, n1> reshape | ( | ) | const [inherited] |
change the matrix shape
Matx<_Tp, 1, n> row | ( | int | i ) | const [inherited] |
extract the matrix row
solve linear system
Matx<_Tp, n, m> t | ( | ) | const [inherited] |
transpose the matrix
Generated on Tue Jul 12 2022 16:42:43 by
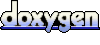