
opencv on mbed
UMat Class Reference
[Basic structures]
#include <mat.hpp>
Public Member Functions | |
UMat (UMatUsageFlags usageFlags=USAGE_DEFAULT) | |
default constructor | |
UMat (int rows, int cols, int type, UMatUsageFlags usageFlags=USAGE_DEFAULT) | |
constructs 2D matrix of the specified size and type | |
UMat (int rows, int cols, int type, const Scalar &s, UMatUsageFlags usageFlags=USAGE_DEFAULT) | |
constucts 2D matrix and fills it with the specified value _s. | |
UMat (int ndims, const int *sizes, int type, UMatUsageFlags usageFlags=USAGE_DEFAULT) | |
constructs n-dimensional matrix | |
UMat (const UMat &m) | |
copy constructor | |
UMat (const UMat &m, const Range &rowRange, const Range &colRange=Range::all()) | |
creates a matrix header for a part of the bigger matrix | |
template<typename _Tp > | |
UMat (const std::vector< _Tp > &vec, bool copyData=false) | |
builds matrix from std::vector with or without copying the data | |
template<typename _Tp , int n> | |
UMat (const Vec< _Tp, n > &vec, bool copyData=true) | |
builds matrix from cv::Vec; the data is copied by default | |
template<typename _Tp , int m, int n> | |
UMat (const Matx< _Tp, m, n > &mtx, bool copyData=true) | |
builds matrix from cv::Matx; the data is copied by default | |
template<typename _Tp > | |
UMat (const Point_< _Tp > &pt, bool copyData=true) | |
builds matrix from a 2D point | |
template<typename _Tp > | |
UMat (const Point3_< _Tp > &pt, bool copyData=true) | |
builds matrix from a 3D point | |
template<typename _Tp > | |
UMat (const MatCommaInitializer_< _Tp > &commaInitializer) | |
builds matrix from comma initializer | |
~UMat () | |
destructor - calls release() | |
UMat & | operator= (const UMat &m) |
assignment operators | |
UMat | row (int y) const |
returns a new matrix header for the specified row | |
UMat | col (int x) const |
returns a new matrix header for the specified column | |
UMat | rowRange (int startrow, int endrow) const |
... for the specified row span | |
UMat | colRange (int startcol, int endcol) const |
... for the specified column span | |
UMat | diag (int d=0) const |
... for the specified diagonal | |
UMat | clone () const |
returns deep copy of the matrix, i.e. the data is copied | |
void | copyTo (OutputArray m) const |
copies the matrix content to "m". | |
void | copyTo (OutputArray m, InputArray mask) const |
copies those matrix elements to "m" that are marked with non-zero mask elements. | |
void | convertTo (OutputArray m, int rtype, double alpha=1, double beta=0) const |
converts matrix to another datatype with optional scalng. See cvConvertScale. | |
UMat & | operator= (const Scalar &s) |
sets every matrix element to s | |
UMat & | setTo (InputArray value, InputArray mask=noArray()) |
sets some of the matrix elements to s, according to the mask | |
UMat | reshape (int cn, int rows=0) const |
creates alternative matrix header for the same data, with different | |
UMat | t () const |
matrix transposition by means of matrix expressions | |
UMat | inv (int method=DECOMP_LU) const |
matrix inversion by means of matrix expressions | |
UMat | mul (InputArray m, double scale=1) const |
per-element matrix multiplication by means of matrix expressions | |
double | dot (InputArray m) const |
computes dot-product | |
void | create (int rows, int cols, int type, UMatUsageFlags usageFlags=USAGE_DEFAULT) |
allocates new matrix data unless the matrix already has specified size and type. | |
void | addref () |
increases the reference counter; use with care to avoid memleaks | |
void | release () |
decreases reference counter; | |
void | deallocate () |
deallocates the matrix data | |
void | copySize (const UMat &m) |
internal use function; properly re-allocates _size, _step arrays | |
void | locateROI (Size &wholeSize, Point &ofs) const |
locates matrix header within a parent matrix. See below | |
UMat & | adjustROI (int dtop, int dbottom, int dleft, int dright) |
moves/resizes the current matrix ROI inside the parent matrix. | |
UMat | operator() (Range rowRange, Range colRange) const |
extracts a rectangular sub-matrix | |
bool | isContinuous () const |
returns true iff the matrix data is continuous | |
bool | isSubmatrix () const |
returns true if the matrix is a submatrix of another matrix | |
size_t | elemSize () const |
returns element size in bytes, | |
size_t | elemSize1 () const |
returns the size of element channel in bytes. | |
int | type () const |
returns element type, similar to CV_MAT_TYPE(cvmat->type) | |
int | depth () const |
returns element type, similar to CV_MAT_DEPTH(cvmat->type) | |
int | channels () const |
returns element type, similar to CV_MAT_CN(cvmat->type) | |
size_t | step1 (int i=0) const |
returns step/elemSize1() | |
bool | empty () const |
returns true if matrix data is NULL | |
size_t | total () const |
returns the total number of matrix elements | |
int | checkVector (int elemChannels, int depth=-1, bool requireContinuous=true) const |
returns N if the matrix is 1-channel (N x ptdim) or ptdim-channel (1 x N) or (N x 1); negative number otherwise | |
Static Public Member Functions | |
static UMat | diag (const UMat &d) |
constructs a square diagonal matrix which main diagonal is vector "d" | |
static UMat | zeros (int rows, int cols, int type) |
Matlab-style matrix initialization. | |
static MatAllocator * | getStdAllocator () |
and the standard allocator | |
Data Fields | |
int | flags |
int | dims |
the matrix dimensionality, >= 2 | |
int | rows |
the number of rows and columns or (-1, -1) when the matrix has more than 2 dimensions | |
MatAllocator * | allocator |
custom allocator |
Detailed Description
Definition at line 2147 of file mat.hpp.
Constructor & Destructor Documentation
UMat | ( | UMatUsageFlags | usageFlags = USAGE_DEFAULT ) |
default constructor
UMat | ( | int | rows, |
int | cols, | ||
int | type, | ||
UMatUsageFlags | usageFlags = USAGE_DEFAULT |
||
) |
constructs 2D matrix of the specified size and type
UMat | ( | int | rows, |
int | cols, | ||
int | type, | ||
const Scalar & | s, | ||
UMatUsageFlags | usageFlags = USAGE_DEFAULT |
||
) |
constucts 2D matrix and fills it with the specified value _s.
UMat | ( | int | ndims, |
const int * | sizes, | ||
int | type, | ||
UMatUsageFlags | usageFlags = USAGE_DEFAULT |
||
) |
constructs n-dimensional matrix
creates a matrix header for a part of the bigger matrix
UMat | ( | const std::vector< _Tp > & | vec, |
bool | copyData = false |
||
) | [explicit] |
builds matrix from std::vector with or without copying the data
builds matrix from cv::Vec; the data is copied by default
builds matrix from cv::Matx; the data is copied by default
UMat | ( | const MatCommaInitializer_< _Tp > & | commaInitializer ) | [explicit] |
builds matrix from comma initializer
Member Function Documentation
void addref | ( | ) |
increases the reference counter; use with care to avoid memleaks
UMat& adjustROI | ( | int | dtop, |
int | dbottom, | ||
int | dleft, | ||
int | dright | ||
) |
moves/resizes the current matrix ROI inside the parent matrix.
int channels | ( | ) | const |
returns element type, similar to CV_MAT_CN(cvmat->type)
int checkVector | ( | int | elemChannels, |
int | depth = -1 , |
||
bool | requireContinuous = true |
||
) | const |
returns N if the matrix is 1-channel (N x ptdim) or ptdim-channel (1 x N) or (N x 1); negative number otherwise
UMat clone | ( | ) | const |
returns deep copy of the matrix, i.e. the data is copied
UMat col | ( | int | x ) | const |
returns a new matrix header for the specified column
UMat colRange | ( | int | startcol, |
int | endcol | ||
) | const |
... for the specified column span
void convertTo | ( | OutputArray | m, |
int | rtype, | ||
double | alpha = 1 , |
||
double | beta = 0 |
||
) | const |
converts matrix to another datatype with optional scalng. See cvConvertScale.
void copySize | ( | const UMat & | m ) |
internal use function; properly re-allocates _size, _step arrays
void copyTo | ( | OutputArray | m ) | const |
copies the matrix content to "m".
void copyTo | ( | OutputArray | m, |
InputArray | mask | ||
) | const |
copies those matrix elements to "m" that are marked with non-zero mask elements.
void create | ( | int | rows, |
int | cols, | ||
int | type, | ||
UMatUsageFlags | usageFlags = USAGE_DEFAULT |
||
) |
allocates new matrix data unless the matrix already has specified size and type.
void deallocate | ( | ) |
deallocates the matrix data
int depth | ( | ) | const |
returns element type, similar to CV_MAT_DEPTH(cvmat->type)
UMat diag | ( | int | d = 0 ) |
const |
... for the specified diagonal
constructs a square diagonal matrix which main diagonal is vector "d"
double dot | ( | InputArray | m ) | const |
computes dot-product
size_t elemSize | ( | ) | const |
returns element size in bytes,
size_t elemSize1 | ( | ) | const |
returns the size of element channel in bytes.
bool empty | ( | ) | const |
returns true if matrix data is NULL
static MatAllocator* getStdAllocator | ( | ) | [static] |
and the standard allocator
UMat inv | ( | int | method = DECOMP_LU ) |
const |
matrix inversion by means of matrix expressions
bool isContinuous | ( | ) | const |
returns true iff the matrix data is continuous
bool isSubmatrix | ( | ) | const |
returns true if the matrix is a submatrix of another matrix
locates matrix header within a parent matrix. See below
UMat mul | ( | InputArray | m, |
double | scale = 1 |
||
) | const |
per-element matrix multiplication by means of matrix expressions
void release | ( | ) |
decreases reference counter;
UMat reshape | ( | int | cn, |
int | rows = 0 |
||
) | const |
creates alternative matrix header for the same data, with different
UMat row | ( | int | y ) | const |
returns a new matrix header for the specified row
UMat rowRange | ( | int | startrow, |
int | endrow | ||
) | const |
... for the specified row span
UMat& setTo | ( | InputArray | value, |
InputArray | mask = noArray() |
||
) |
sets some of the matrix elements to s, according to the mask
size_t step1 | ( | int | i = 0 ) |
const |
returns step/elemSize1()
UMat t | ( | ) | const |
matrix transposition by means of matrix expressions
size_t total | ( | ) | const |
returns the total number of matrix elements
int type | ( | ) | const |
returns element type, similar to CV_MAT_TYPE(cvmat->type)
static UMat zeros | ( | int | rows, |
int | cols, | ||
int | type | ||
) | [static] |
Matlab-style matrix initialization.
Field Documentation
int flags |
Generated on Tue Jul 12 2022 16:42:43 by
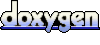