
opencv on mbed
HostMem Class Reference
[Data Structures]
Class with reference counting wrapping special memory type allocation functions from CUDA. More...
#include <cuda.hpp>
Public Member Functions | |
HostMem (InputArray arr, AllocType alloc_type=PAGE_LOCKED) | |
creates from host memory with coping data | |
void | swap (HostMem &b) |
swaps with other smart pointer | |
HostMem | clone () const |
returns deep copy of the matrix, i.e. the data is copied | |
void | create (int rows, int cols, int type) |
allocates new matrix data unless the matrix already has specified size and type. | |
HostMem | reshape (int cn, int rows=0) const |
creates alternative HostMem header for the same data, with different number of channels and/or different number of rows | |
void | release () |
decrements reference counter and released memory if needed. | |
Mat | createMatHeader () const |
returns matrix header with disabled reference counting for HostMem data. | |
GpuMat | createGpuMatHeader () const |
Maps CPU memory to GPU address space and creates the cuda::GpuMat header without reference counting for it. |
Detailed Description
Class with reference counting wrapping special memory type allocation functions from CUDA.
Its interface is also Mat-like but with additional memory type parameters.
- **PAGE_LOCKED** sets a page locked memory type used commonly for fast and asynchronous uploading/downloading data from/to GPU.
- **SHARED** specifies a zero copy memory allocation that enables mapping the host memory to GPU address space, if supported.
- **WRITE_COMBINED** sets the write combined buffer that is not cached by CPU. Such buffers are used to supply GPU with data when GPU only reads it. The advantage is a better CPU cache utilization.
- Note:
- Allocation size of such memory types is usually limited. For more details, see *CUDA 2.2 Pinned Memory APIs* document or *CUDA C Programming Guide*.
Definition at line 353 of file core/cuda.hpp.
Constructor & Destructor Documentation
HostMem | ( | InputArray | arr, |
AllocType | alloc_type = PAGE_LOCKED |
||
) | [explicit] |
creates from host memory with coping data
Member Function Documentation
HostMem clone | ( | ) | const |
returns deep copy of the matrix, i.e. the data is copied
void create | ( | int | rows, |
int | cols, | ||
int | type | ||
) |
allocates new matrix data unless the matrix already has specified size and type.
GpuMat createGpuMatHeader | ( | ) | const |
Maps CPU memory to GPU address space and creates the cuda::GpuMat header without reference counting for it.
This can be done only if memory was allocated with the SHARED flag and if it is supported by the hardware. Laptops often share video and CPU memory, so address spaces can be mapped, which eliminates an extra copy.
Mat createMatHeader | ( | ) | const |
returns matrix header with disabled reference counting for HostMem data.
void release | ( | ) |
decrements reference counter and released memory if needed.
HostMem reshape | ( | int | cn, |
int | rows = 0 |
||
) | const |
creates alternative HostMem header for the same data, with different number of channels and/or different number of rows
void swap | ( | HostMem & | b ) |
swaps with other smart pointer
Generated on Tue Jul 12 2022 16:42:44 by
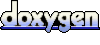