
opencv on mbed
Embed:
(wiki syntax)
Show/hide line numbers
imgproc_c.h
00001 /*M/////////////////////////////////////////////////////////////////////////////////////// 00002 // 00003 // IMPORTANT: READ BEFORE DOWNLOADING, COPYING, INSTALLING OR USING. 00004 // 00005 // By downloading, copying, installing or using the software you agree to this license. 00006 // If you do not agree to this license, do not download, install, 00007 // copy or use the software. 00008 // 00009 // 00010 // License Agreement 00011 // For Open Source Computer Vision Library 00012 // 00013 // Copyright (C) 2000-2008, Intel Corporation, all rights reserved. 00014 // Copyright (C) 2009, Willow Garage Inc., all rights reserved. 00015 // Third party copyrights are property of their respective owners. 00016 // 00017 // Redistribution and use in source and binary forms, with or without modification, 00018 // are permitted provided that the following conditions are met: 00019 // 00020 // * Redistribution's of source code must retain the above copyright notice, 00021 // this list of conditions and the following disclaimer. 00022 // 00023 // * Redistribution's in binary form must reproduce the above copyright notice, 00024 // this list of conditions and the following disclaimer in the documentation 00025 // and/or other materials provided with the distribution. 00026 // 00027 // * The name of the copyright holders may not be used to endorse or promote products 00028 // derived from this software without specific prior written permission. 00029 // 00030 // This software is provided by the copyright holders and contributors "as is" and 00031 // any express or implied warranties, including, but not limited to, the implied 00032 // warranties of merchantability and fitness for a particular purpose are disclaimed. 00033 // In no event shall the Intel Corporation or contributors be liable for any direct, 00034 // indirect, incidental, special, exemplary, or consequential damages 00035 // (including, but not limited to, procurement of substitute goods or services; 00036 // loss of use, data, or profits; or business interruption) however caused 00037 // and on any theory of liability, whether in contract, strict liability, 00038 // or tort (including negligence or otherwise) arising in any way out of 00039 // the use of this software, even if advised of the possibility of such damage. 00040 // 00041 //M*/ 00042 00043 #ifndef __OPENCV_IMGPROC_IMGPROC_C_H__ 00044 #define __OPENCV_IMGPROC_IMGPROC_C_H__ 00045 00046 #include "opencv2/imgproc/types_c.h" 00047 00048 #ifdef __cplusplus 00049 extern "C" { 00050 #endif 00051 00052 /** @addtogroup imgproc_c 00053 @{ 00054 */ 00055 00056 /*********************** Background statistics accumulation *****************************/ 00057 00058 /** @brief Adds image to accumulator 00059 @see cv::accumulate 00060 */ 00061 CVAPI(void) cvAcc( const CvArr* image, CvArr* sum, 00062 const CvArr* mask CV_DEFAULT(NULL) ); 00063 00064 /** @brief Adds squared image to accumulator 00065 @see cv::accumulateSquare 00066 */ 00067 CVAPI(void) cvSquareAcc( const CvArr* image, CvArr* sqsum, 00068 const CvArr* mask CV_DEFAULT(NULL) ); 00069 00070 /** @brief Adds a product of two images to accumulator 00071 @see cv::accumulateProduct 00072 */ 00073 CVAPI(void) cvMultiplyAcc( const CvArr* image1, const CvArr* image2, CvArr* acc, 00074 const CvArr* mask CV_DEFAULT(NULL) ); 00075 00076 /** @brief Adds image to accumulator with weights: acc = acc*(1-alpha) + image*alpha 00077 @see cv::accumulateWeighted 00078 */ 00079 CVAPI(void) cvRunningAvg( const CvArr* image, CvArr* acc, double alpha, 00080 const CvArr* mask CV_DEFAULT(NULL) ); 00081 00082 /****************************************************************************************\ 00083 * Image Processing * 00084 \****************************************************************************************/ 00085 00086 /** Copies source 2D array inside of the larger destination array and 00087 makes a border of the specified type (IPL_BORDER_*) around the copied area. */ 00088 CVAPI(void) cvCopyMakeBorder( const CvArr* src, CvArr* dst, CvPoint offset, 00089 int bordertype, CvScalar value CV_DEFAULT(cvScalarAll(0))); 00090 00091 /** @brief Smooths the image in one of several ways. 00092 00093 @param src The source image 00094 @param dst The destination image 00095 @param smoothtype Type of the smoothing, see SmoothMethod_c 00096 @param size1 The first parameter of the smoothing operation, the aperture width. Must be a 00097 positive odd number (1, 3, 5, ...) 00098 @param size2 The second parameter of the smoothing operation, the aperture height. Ignored by 00099 CV_MEDIAN and CV_BILATERAL methods. In the case of simple scaled/non-scaled and Gaussian blur if 00100 size2 is zero, it is set to size1. Otherwise it must be a positive odd number. 00101 @param sigma1 In the case of a Gaussian parameter this parameter may specify Gaussian \f$\sigma\f$ 00102 (standard deviation). If it is zero, it is calculated from the kernel size: 00103 \f[\sigma = 0.3 (n/2 - 1) + 0.8 \quad \text{where} \quad n= \begin{array}{l l} \mbox{\texttt{size1} for horizontal kernel} \\ \mbox{\texttt{size2} for vertical kernel} \end{array}\f] 00104 Using standard sigma for small kernels ( \f$3\times 3\f$ to \f$7\times 7\f$ ) gives better speed. If 00105 sigma1 is not zero, while size1 and size2 are zeros, the kernel size is calculated from the 00106 sigma (to provide accurate enough operation). 00107 @param sigma2 additional parameter for bilateral filtering 00108 00109 @see cv::GaussianBlur, cv::blur, cv::medianBlur, cv::bilateralFilter. 00110 */ 00111 CVAPI(void) cvSmooth( const CvArr* src, CvArr* dst, 00112 int smoothtype CV_DEFAULT(CV_GAUSSIAN), 00113 int size1 CV_DEFAULT(3), 00114 int size2 CV_DEFAULT(0), 00115 double sigma1 CV_DEFAULT(0), 00116 double sigma2 CV_DEFAULT(0)); 00117 00118 /** @brief Convolves an image with the kernel. 00119 00120 @param src input image. 00121 @param dst output image of the same size and the same number of channels as src. 00122 @param kernel convolution kernel (or rather a correlation kernel), a single-channel floating point 00123 matrix; if you want to apply different kernels to different channels, split the image into 00124 separate color planes using split and process them individually. 00125 @param anchor anchor of the kernel that indicates the relative position of a filtered point within 00126 the kernel; the anchor should lie within the kernel; default value (-1,-1) means that the anchor 00127 is at the kernel center. 00128 00129 @see cv::filter2D 00130 */ 00131 CVAPI(void) cvFilter2D( const CvArr* src, CvArr* dst, const CvMat* kernel, 00132 CvPoint anchor CV_DEFAULT(cvPoint(-1,-1))); 00133 00134 /** @brief Finds integral image: SUM(X,Y) = sum(x<X,y<Y)I(x,y) 00135 @see cv::integral 00136 */ 00137 CVAPI(void) cvIntegral( const CvArr* image, CvArr* sum, 00138 CvArr* sqsum CV_DEFAULT(NULL), 00139 CvArr* tilted_sum CV_DEFAULT(NULL)); 00140 00141 /** @brief Smoothes the input image with gaussian kernel and then down-samples it. 00142 00143 dst_width = floor(src_width/2)[+1], 00144 dst_height = floor(src_height/2)[+1] 00145 @see cv::pyrDown 00146 */ 00147 CVAPI(void) cvPyrDown( const CvArr* src, CvArr* dst, 00148 int filter CV_DEFAULT(CV_GAUSSIAN_5x5) ); 00149 00150 /** @brief Up-samples image and smoothes the result with gaussian kernel. 00151 00152 dst_width = src_width*2, 00153 dst_height = src_height*2 00154 @see cv::pyrUp 00155 */ 00156 CVAPI(void) cvPyrUp( const CvArr* src, CvArr* dst, 00157 int filter CV_DEFAULT(CV_GAUSSIAN_5x5) ); 00158 00159 /** @brief Builds pyramid for an image 00160 @see buildPyramid 00161 */ 00162 CVAPI(CvMat**) cvCreatePyramid( const CvArr* img, int extra_layers, double rate, 00163 const CvSize* layer_sizes CV_DEFAULT(0), 00164 CvArr* bufarr CV_DEFAULT(0), 00165 int calc CV_DEFAULT(1), 00166 int filter CV_DEFAULT(CV_GAUSSIAN_5x5) ); 00167 00168 /** @brief Releases pyramid */ 00169 CVAPI(void) cvReleasePyramid( CvMat*** pyramid, int extra_layers ); 00170 00171 00172 /** @brief Filters image using meanshift algorithm 00173 @see cv::pyrMeanShiftFiltering 00174 */ 00175 CVAPI(void) cvPyrMeanShiftFiltering( const CvArr* src, CvArr* dst, 00176 double sp, double sr, int max_level CV_DEFAULT(1), 00177 CvTermCriteria termcrit CV_DEFAULT(cvTermCriteria(CV_TERMCRIT_ITER+CV_TERMCRIT_EPS,5,1))); 00178 00179 /** @brief Segments image using seed "markers" 00180 @see cv::watershed 00181 */ 00182 CVAPI(void) cvWatershed( const CvArr* image, CvArr* markers ); 00183 00184 /** @brief Calculates an image derivative using generalized Sobel 00185 00186 (aperture_size = 1,3,5,7) or Scharr (aperture_size = -1) operator. 00187 Scharr can be used only for the first dx or dy derivative 00188 @see cv::Sobel 00189 */ 00190 CVAPI(void) cvSobel( const CvArr* src, CvArr* dst, 00191 int xorder, int yorder, 00192 int aperture_size CV_DEFAULT(3)); 00193 00194 /** @brief Calculates the image Laplacian: (d2/dx + d2/dy)I 00195 @see cv::Laplacian 00196 */ 00197 CVAPI(void) cvLaplace( const CvArr* src, CvArr* dst, 00198 int aperture_size CV_DEFAULT(3) ); 00199 00200 /** @brief Converts input array pixels from one color space to another 00201 @see cv::cvtColor 00202 */ 00203 CVAPI(void) cvCvtColor( const CvArr* src, CvArr* dst, int code ); 00204 00205 00206 /** @brief Resizes image (input array is resized to fit the destination array) 00207 @see cv::resize 00208 */ 00209 CVAPI(void) cvResize( const CvArr* src, CvArr* dst, 00210 int interpolation CV_DEFAULT( CV_INTER_LINEAR )); 00211 00212 /** @brief Warps image with affine transform 00213 @note ::cvGetQuadrangleSubPix is similar to ::cvWarpAffine, but the outliers are extrapolated using 00214 replication border mode. 00215 @see cv::warpAffine 00216 */ 00217 CVAPI(void) cvWarpAffine( const CvArr* src, CvArr* dst, const CvMat* map_matrix, 00218 int flags CV_DEFAULT(CV_INTER_LINEAR+CV_WARP_FILL_OUTLIERS), 00219 CvScalar fillval CV_DEFAULT(cvScalarAll(0)) ); 00220 00221 /** @brief Computes affine transform matrix for mapping src[i] to dst[i] (i=0,1,2) 00222 @see cv::getAffineTransform 00223 */ 00224 CVAPI(CvMat*) cvGetAffineTransform( const CvPoint2D32f * src, 00225 const CvPoint2D32f * dst, 00226 CvMat * map_matrix ); 00227 00228 /** @brief Computes rotation_matrix matrix 00229 @see cv::getRotationMatrix2D 00230 */ 00231 CVAPI(CvMat*) cv2DRotationMatrix( CvPoint2D32f center, double angle, 00232 double scale, CvMat* map_matrix ); 00233 00234 /** @brief Warps image with perspective (projective) transform 00235 @see cv::warpPerspective 00236 */ 00237 CVAPI(void) cvWarpPerspective( const CvArr* src, CvArr* dst, const CvMat* map_matrix, 00238 int flags CV_DEFAULT(CV_INTER_LINEAR+CV_WARP_FILL_OUTLIERS), 00239 CvScalar fillval CV_DEFAULT(cvScalarAll(0)) ); 00240 00241 /** @brief Computes perspective transform matrix for mapping src[i] to dst[i] (i=0,1,2,3) 00242 @see cv::getPerspectiveTransform 00243 */ 00244 CVAPI(CvMat*) cvGetPerspectiveTransform( const CvPoint2D32f* src, 00245 const CvPoint2D32f* dst, 00246 CvMat* map_matrix ); 00247 00248 /** @brief Performs generic geometric transformation using the specified coordinate maps 00249 @see cv::remap 00250 */ 00251 CVAPI(void) cvRemap( const CvArr* src, CvArr* dst, 00252 const CvArr* mapx, const CvArr* mapy, 00253 int flags CV_DEFAULT(CV_INTER_LINEAR+CV_WARP_FILL_OUTLIERS), 00254 CvScalar fillval CV_DEFAULT(cvScalarAll(0)) ); 00255 00256 /** @brief Converts mapx & mapy from floating-point to integer formats for cvRemap 00257 @see cv::convertMaps 00258 */ 00259 CVAPI(void) cvConvertMaps( const CvArr* mapx, const CvArr* mapy, 00260 CvArr* mapxy, CvArr* mapalpha ); 00261 00262 /** @brief Performs forward or inverse log-polar image transform 00263 @see cv::logPolar 00264 */ 00265 CVAPI(void) cvLogPolar( const CvArr* src, CvArr* dst, 00266 CvPoint2D32f center, double M, 00267 int flags CV_DEFAULT(CV_INTER_LINEAR+CV_WARP_FILL_OUTLIERS)); 00268 00269 /** Performs forward or inverse linear-polar image transform 00270 @see cv::linearPolar 00271 */ 00272 CVAPI(void) cvLinearPolar( const CvArr* src, CvArr* dst, 00273 CvPoint2D32f center, double maxRadius, 00274 int flags CV_DEFAULT(CV_INTER_LINEAR+CV_WARP_FILL_OUTLIERS)); 00275 00276 /** @brief Transforms the input image to compensate lens distortion 00277 @see cv::undistort 00278 */ 00279 CVAPI(void) cvUndistort2( const CvArr* src, CvArr* dst, 00280 const CvMat* camera_matrix, 00281 const CvMat* distortion_coeffs, 00282 const CvMat* new_camera_matrix CV_DEFAULT(0) ); 00283 00284 /** @brief Computes transformation map from intrinsic camera parameters 00285 that can used by cvRemap 00286 */ 00287 CVAPI(void) cvInitUndistortMap( const CvMat* camera_matrix, 00288 const CvMat* distortion_coeffs, 00289 CvArr* mapx, CvArr* mapy ); 00290 00291 /** @brief Computes undistortion+rectification map for a head of stereo camera 00292 @see cv::initUndistortRectifyMap 00293 */ 00294 CVAPI(void) cvInitUndistortRectifyMap( const CvMat* camera_matrix, 00295 const CvMat* dist_coeffs, 00296 const CvMat *R, const CvMat* new_camera_matrix, 00297 CvArr* mapx, CvArr* mapy ); 00298 00299 /** @brief Computes the original (undistorted) feature coordinates 00300 from the observed (distorted) coordinates 00301 @see cv::undistortPoints 00302 */ 00303 CVAPI(void) cvUndistortPoints( const CvMat* src, CvMat* dst, 00304 const CvMat* camera_matrix, 00305 const CvMat* dist_coeffs, 00306 const CvMat* R CV_DEFAULT(0), 00307 const CvMat* P CV_DEFAULT(0)); 00308 00309 /** @brief Returns a structuring element of the specified size and shape for morphological operations. 00310 00311 @note the created structuring element IplConvKernel\* element must be released in the end using 00312 `cvReleaseStructuringElement(&element)`. 00313 00314 @param cols Width of the structuring element 00315 @param rows Height of the structuring element 00316 @param anchor_x x-coordinate of the anchor 00317 @param anchor_y y-coordinate of the anchor 00318 @param shape element shape that could be one of the cv::MorphShapes_c 00319 @param values integer array of cols*rows elements that specifies the custom shape of the 00320 structuring element, when shape=CV_SHAPE_CUSTOM. 00321 00322 @see cv::getStructuringElement 00323 */ 00324 CVAPI(IplConvKernel*) cvCreateStructuringElementEx( 00325 int cols, int rows, int anchor_x, int anchor_y, 00326 int shape, int* values CV_DEFAULT(NULL) ); 00327 00328 /** @brief releases structuring element 00329 @see cvCreateStructuringElementEx 00330 */ 00331 CVAPI(void) cvReleaseStructuringElement( IplConvKernel** element ); 00332 00333 /** @brief erodes input image (applies minimum filter) one or more times. 00334 If element pointer is NULL, 3x3 rectangular element is used 00335 @see cv::erode 00336 */ 00337 CVAPI(void) cvErode( const CvArr* src, CvArr* dst, 00338 IplConvKernel* element CV_DEFAULT(NULL), 00339 int iterations CV_DEFAULT(1) ); 00340 00341 /** @brief dilates input image (applies maximum filter) one or more times. 00342 00343 If element pointer is NULL, 3x3 rectangular element is used 00344 @see cv::dilate 00345 */ 00346 CVAPI(void) cvDilate( const CvArr* src, CvArr* dst, 00347 IplConvKernel* element CV_DEFAULT(NULL), 00348 int iterations CV_DEFAULT(1) ); 00349 00350 /** @brief Performs complex morphological transformation 00351 @see cv::morphologyEx 00352 */ 00353 CVAPI(void) cvMorphologyEx( const CvArr* src, CvArr* dst, 00354 CvArr* temp, IplConvKernel* element, 00355 int operation, int iterations CV_DEFAULT(1) ); 00356 00357 /** @brief Calculates all spatial and central moments up to the 3rd order 00358 @see cv::moments 00359 */ 00360 CVAPI(void) cvMoments( const CvArr* arr, CvMoments* moments, int binary CV_DEFAULT(0)); 00361 00362 /** @brief Retrieve spatial moments */ 00363 CVAPI(double) cvGetSpatialMoment( CvMoments* moments, int x_order, int y_order ); 00364 /** @brief Retrieve central moments */ 00365 CVAPI(double) cvGetCentralMoment( CvMoments* moments, int x_order, int y_order ); 00366 /** @brief Retrieve normalized central moments */ 00367 CVAPI(double) cvGetNormalizedCentralMoment( CvMoments* moments, 00368 int x_order, int y_order ); 00369 00370 /** @brief Calculates 7 Hu's invariants from precalculated spatial and central moments 00371 @see cv::HuMoments 00372 */ 00373 CVAPI(void) cvGetHuMoments( CvMoments* moments, CvHuMoments* hu_moments ); 00374 00375 /*********************************** data sampling **************************************/ 00376 00377 /** @brief Fetches pixels that belong to the specified line segment and stores them to the buffer. 00378 00379 Returns the number of retrieved points. 00380 @see cv::LineSegmentDetector 00381 */ 00382 CVAPI(int) cvSampleLine( const CvArr* image, CvPoint pt1, CvPoint pt2, void* buffer, 00383 int connectivity CV_DEFAULT(8)); 00384 00385 /** @brief Retrieves the rectangular image region with specified center from the input array. 00386 00387 dst(x,y) <- src(x + center.x - dst_width/2, y + center.y - dst_height/2). 00388 Values of pixels with fractional coordinates are retrieved using bilinear interpolation 00389 @see cv::getRectSubPix 00390 */ 00391 CVAPI(void) cvGetRectSubPix( const CvArr* src, CvArr* dst, CvPoint2D32f center ); 00392 00393 00394 /** @brief Retrieves quadrangle from the input array. 00395 00396 matrixarr = ( a11 a12 | b1 ) dst(x,y) <- src(A[x y]' + b) 00397 ( a21 a22 | b2 ) (bilinear interpolation is used to retrieve pixels 00398 with fractional coordinates) 00399 @see cvWarpAffine 00400 */ 00401 CVAPI(void) cvGetQuadrangleSubPix( const CvArr* src, CvArr* dst, 00402 const CvMat* map_matrix ); 00403 00404 /** @brief Measures similarity between template and overlapped windows in the source image 00405 and fills the resultant image with the measurements 00406 @see cv::matchTemplate 00407 */ 00408 CVAPI(void) cvMatchTemplate( const CvArr* image, const CvArr* templ, 00409 CvArr* result, int method ); 00410 00411 /** @brief Computes earth mover distance between 00412 two weighted point sets (called signatures) 00413 @see cv::EMD 00414 */ 00415 CVAPI(float) cvCalcEMD2( const CvArr* signature1, 00416 const CvArr* signature2, 00417 int distance_type, 00418 CvDistanceFunction distance_func CV_DEFAULT(NULL), 00419 const CvArr* cost_matrix CV_DEFAULT(NULL), 00420 CvArr* flow CV_DEFAULT(NULL), 00421 float* lower_bound CV_DEFAULT(NULL), 00422 void* userdata CV_DEFAULT(NULL)); 00423 00424 /****************************************************************************************\ 00425 * Contours retrieving * 00426 \****************************************************************************************/ 00427 00428 /** @brief Retrieves outer and optionally inner boundaries of white (non-zero) connected 00429 components in the black (zero) background 00430 @see cv::findContours, cvStartFindContours, cvFindNextContour, cvSubstituteContour, cvEndFindContours 00431 */ 00432 CVAPI(int) cvFindContours( CvArr* image, CvMemStorage* storage, CvSeq** first_contour, 00433 int header_size CV_DEFAULT(sizeof(CvContour)), 00434 int mode CV_DEFAULT(CV_RETR_LIST), 00435 int method CV_DEFAULT(CV_CHAIN_APPROX_SIMPLE), 00436 CvPoint offset CV_DEFAULT(cvPoint(0,0))); 00437 00438 /** @brief Initializes contour retrieving process. 00439 00440 Calls cvStartFindContours. 00441 Calls cvFindNextContour until null pointer is returned 00442 or some other condition becomes true. 00443 Calls cvEndFindContours at the end. 00444 @see cvFindContours 00445 */ 00446 CVAPI(CvContourScanner) cvStartFindContours( CvArr* image, CvMemStorage* storage, 00447 int header_size CV_DEFAULT(sizeof(CvContour)), 00448 int mode CV_DEFAULT(CV_RETR_LIST), 00449 int method CV_DEFAULT(CV_CHAIN_APPROX_SIMPLE), 00450 CvPoint offset CV_DEFAULT(cvPoint(0,0))); 00451 00452 /** @brief Retrieves next contour 00453 @see cvFindContours 00454 */ 00455 CVAPI(CvSeq*) cvFindNextContour( CvContourScanner scanner ); 00456 00457 00458 /** @brief Substitutes the last retrieved contour with the new one 00459 00460 (if the substitutor is null, the last retrieved contour is removed from the tree) 00461 @see cvFindContours 00462 */ 00463 CVAPI(void) cvSubstituteContour( CvContourScanner scanner, CvSeq* new_contour ); 00464 00465 00466 /** @brief Releases contour scanner and returns pointer to the first outer contour 00467 @see cvFindContours 00468 */ 00469 CVAPI(CvSeq*) cvEndFindContours( CvContourScanner* scanner ); 00470 00471 /** @brief Approximates Freeman chain(s) with a polygonal curve. 00472 00473 This is a standalone contour approximation routine, not represented in the new interface. When 00474 cvFindContours retrieves contours as Freeman chains, it calls the function to get approximated 00475 contours, represented as polygons. 00476 00477 @param src_seq Pointer to the approximated Freeman chain that can refer to other chains. 00478 @param storage Storage location for the resulting polylines. 00479 @param method Approximation method (see the description of the function :ocvFindContours ). 00480 @param parameter Method parameter (not used now). 00481 @param minimal_perimeter Approximates only those contours whose perimeters are not less than 00482 minimal_perimeter . Other chains are removed from the resulting structure. 00483 @param recursive Recursion flag. If it is non-zero, the function approximates all chains that can 00484 be obtained from chain by using the h_next or v_next links. Otherwise, the single input chain is 00485 approximated. 00486 @see cvStartReadChainPoints, cvReadChainPoint 00487 */ 00488 CVAPI(CvSeq*) cvApproxChains( CvSeq* src_seq, CvMemStorage* storage, 00489 int method CV_DEFAULT(CV_CHAIN_APPROX_SIMPLE), 00490 double parameter CV_DEFAULT(0), 00491 int minimal_perimeter CV_DEFAULT(0), 00492 int recursive CV_DEFAULT(0)); 00493 00494 /** @brief Initializes Freeman chain reader. 00495 00496 The reader is used to iteratively get coordinates of all the chain points. 00497 If the Freeman codes should be read as is, a simple sequence reader should be used 00498 @see cvApproxChains 00499 */ 00500 CVAPI(void) cvStartReadChainPoints( CvChain* chain, CvChainPtReader* reader ); 00501 00502 /** @brief Retrieves the next chain point 00503 @see cvApproxChains 00504 */ 00505 CVAPI(CvPoint) cvReadChainPoint( CvChainPtReader* reader ); 00506 00507 00508 /****************************************************************************************\ 00509 * Contour Processing and Shape Analysis * 00510 \****************************************************************************************/ 00511 00512 /** @brief Approximates a single polygonal curve (contour) or 00513 a tree of polygonal curves (contours) 00514 @see cv::approxPolyDP 00515 */ 00516 CVAPI(CvSeq*) cvApproxPoly( const void* src_seq, 00517 int header_size, CvMemStorage* storage, 00518 int method, double eps, 00519 int recursive CV_DEFAULT(0)); 00520 00521 /** @brief Calculates perimeter of a contour or length of a part of contour 00522 @see cv::arcLength 00523 */ 00524 CVAPI(double) cvArcLength( const void* curve, 00525 CvSlice slice CV_DEFAULT(CV_WHOLE_SEQ), 00526 int is_closed CV_DEFAULT(-1)); 00527 00528 /** same as cvArcLength for closed contour 00529 */ 00530 CV_INLINE double cvContourPerimeter( const void* contour ) 00531 { 00532 return cvArcLength( contour, CV_WHOLE_SEQ, 1 ); 00533 } 00534 00535 00536 /** @brief Calculates contour bounding rectangle (update=1) or 00537 just retrieves pre-calculated rectangle (update=0) 00538 @see cv::boundingRect 00539 */ 00540 CVAPI(CvRect ) cvBoundingRect( CvArr* points, int update CV_DEFAULT(0) ); 00541 00542 /** @brief Calculates area of a contour or contour segment 00543 @see cv::contourArea 00544 */ 00545 CVAPI(double) cvContourArea( const CvArr* contour, 00546 CvSlice slice CV_DEFAULT(CV_WHOLE_SEQ), 00547 int oriented CV_DEFAULT(0)); 00548 00549 /** @brief Finds minimum area rotated rectangle bounding a set of points 00550 @see cv::minAreaRect 00551 */ 00552 CVAPI(CvBox2D ) cvMinAreaRect2( const CvArr* points, 00553 CvMemStorage* storage CV_DEFAULT(NULL)); 00554 00555 /** @brief Finds minimum enclosing circle for a set of points 00556 @see cv::minEnclosingCircle 00557 */ 00558 CVAPI(int) cvMinEnclosingCircle( const CvArr* points, 00559 CvPoint2D32f* center, float* radius ); 00560 00561 /** @brief Compares two contours by matching their moments 00562 @see cv::matchShapes 00563 */ 00564 CVAPI(double) cvMatchShapes( const void* object1, const void* object2, 00565 int method, double parameter CV_DEFAULT(0)); 00566 00567 /** @brief Calculates exact convex hull of 2d point set 00568 @see cv::convexHull 00569 */ 00570 CVAPI(CvSeq*) cvConvexHull2( const CvArr* input, 00571 void* hull_storage CV_DEFAULT(NULL), 00572 int orientation CV_DEFAULT(CV_CLOCKWISE), 00573 int return_points CV_DEFAULT(0)); 00574 00575 /** @brief Checks whether the contour is convex or not (returns 1 if convex, 0 if not) 00576 @see cv::isContourConvex 00577 */ 00578 CVAPI(int) cvCheckContourConvexity( const CvArr* contour ); 00579 00580 00581 /** @brief Finds convexity defects for the contour 00582 @see cv::convexityDefects 00583 */ 00584 CVAPI(CvSeq*) cvConvexityDefects( const CvArr* contour, const CvArr* convexhull, 00585 CvMemStorage* storage CV_DEFAULT(NULL)); 00586 00587 /** @brief Fits ellipse into a set of 2d points 00588 @see cv::fitEllipse 00589 */ 00590 CVAPI(CvBox2D ) cvFitEllipse2( const CvArr* points ); 00591 00592 /** @brief Finds minimum rectangle containing two given rectangles */ 00593 CVAPI(CvRect ) cvMaxRect( const CvRect * rect1, const CvRect * rect2 ); 00594 00595 /** @brief Finds coordinates of the box vertices */ 00596 CVAPI(void) cvBoxPoints( CvBox2D box, CvPoint2D32f pt[4] ); 00597 00598 /** @brief Initializes sequence header for a matrix (column or row vector) of points 00599 00600 a wrapper for cvMakeSeqHeaderForArray (it does not initialize bounding rectangle!!!) */ 00601 CVAPI(CvSeq*) cvPointSeqFromMat( int seq_kind, const CvArr* mat, 00602 CvContour* contour_header, 00603 CvSeqBlock* block ); 00604 00605 /** @brief Checks whether the point is inside polygon, outside, on an edge (at a vertex). 00606 00607 Returns positive, negative or zero value, correspondingly. 00608 Optionally, measures a signed distance between 00609 the point and the nearest polygon edge (measure_dist=1) 00610 @see cv::pointPolygonTest 00611 */ 00612 CVAPI(double) cvPointPolygonTest( const CvArr* contour, 00613 CvPoint2D32f pt, int measure_dist ); 00614 00615 /****************************************************************************************\ 00616 * Histogram functions * 00617 \****************************************************************************************/ 00618 00619 /** @brief Creates a histogram. 00620 00621 The function creates a histogram of the specified size and returns a pointer to the created 00622 histogram. If the array ranges is 0, the histogram bin ranges must be specified later via the 00623 function cvSetHistBinRanges. Though cvCalcHist and cvCalcBackProject may process 8-bit images 00624 without setting bin ranges, they assume they are equally spaced in 0 to 255 bins. 00625 00626 @param dims Number of histogram dimensions. 00627 @param sizes Array of the histogram dimension sizes. 00628 @param type Histogram representation format. CV_HIST_ARRAY means that the histogram data is 00629 represented as a multi-dimensional dense array CvMatND. CV_HIST_SPARSE means that histogram data 00630 is represented as a multi-dimensional sparse array CvSparseMat. 00631 @param ranges Array of ranges for the histogram bins. Its meaning depends on the uniform parameter 00632 value. The ranges are used when the histogram is calculated or backprojected to determine which 00633 histogram bin corresponds to which value/tuple of values from the input image(s). 00634 @param uniform Uniformity flag. If not zero, the histogram has evenly spaced bins and for every 00635 \f$0<=i<cDims\f$ ranges[i] is an array of two numbers: lower and upper boundaries for the i-th 00636 histogram dimension. The whole range [lower,upper] is then split into dims[i] equal parts to 00637 determine the i-th input tuple value ranges for every histogram bin. And if uniform=0 , then the 00638 i-th element of the ranges array contains dims[i]+1 elements: \f$\texttt{lower}_0, 00639 \texttt{upper}_0, \texttt{lower}_1, \texttt{upper}_1 = \texttt{lower}_2, 00640 ... 00641 \texttt{upper}_{dims[i]-1}\f$ where \f$\texttt{lower}_j\f$ and \f$\texttt{upper}_j\f$ are lower 00642 and upper boundaries of the i-th input tuple value for the j-th bin, respectively. In either 00643 case, the input values that are beyond the specified range for a histogram bin are not counted 00644 by cvCalcHist and filled with 0 by cvCalcBackProject. 00645 */ 00646 CVAPI(CvHistogram*) cvCreateHist( int dims, int* sizes, int type, 00647 float** ranges CV_DEFAULT(NULL), 00648 int uniform CV_DEFAULT(1)); 00649 00650 /** @brief Sets the bounds of the histogram bins. 00651 00652 This is a standalone function for setting bin ranges in the histogram. For a more detailed 00653 description of the parameters ranges and uniform, see the :ocvCalcHist function that can initialize 00654 the ranges as well. Ranges for the histogram bins must be set before the histogram is calculated or 00655 the backproject of the histogram is calculated. 00656 00657 @param hist Histogram. 00658 @param ranges Array of bin ranges arrays. See :ocvCreateHist for details. 00659 @param uniform Uniformity flag. See :ocvCreateHist for details. 00660 */ 00661 CVAPI(void) cvSetHistBinRanges( CvHistogram* hist, float** ranges, 00662 int uniform CV_DEFAULT(1)); 00663 00664 /** @brief Makes a histogram out of an array. 00665 00666 The function initializes the histogram, whose header and bins are allocated by the user. 00667 cvReleaseHist does not need to be called afterwards. Only dense histograms can be initialized this 00668 way. The function returns hist. 00669 00670 @param dims Number of the histogram dimensions. 00671 @param sizes Array of the histogram dimension sizes. 00672 @param hist Histogram header initialized by the function. 00673 @param data Array used to store histogram bins. 00674 @param ranges Histogram bin ranges. See cvCreateHist for details. 00675 @param uniform Uniformity flag. See cvCreateHist for details. 00676 */ 00677 CVAPI(CvHistogram*) cvMakeHistHeaderForArray( 00678 int dims, int* sizes, CvHistogram* hist, 00679 float* data, float** ranges CV_DEFAULT(NULL), 00680 int uniform CV_DEFAULT(1)); 00681 00682 /** @brief Releases the histogram. 00683 00684 The function releases the histogram (header and the data). The pointer to the histogram is cleared 00685 by the function. If \*hist pointer is already NULL, the function does nothing. 00686 00687 @param hist Double pointer to the released histogram. 00688 */ 00689 CVAPI(void) cvReleaseHist( CvHistogram** hist ); 00690 00691 /** @brief Clears the histogram. 00692 00693 The function sets all of the histogram bins to 0 in case of a dense histogram and removes all 00694 histogram bins in case of a sparse array. 00695 00696 @param hist Histogram. 00697 */ 00698 CVAPI(void) cvClearHist( CvHistogram* hist ); 00699 00700 /** @brief Finds the minimum and maximum histogram bins. 00701 00702 The function finds the minimum and maximum histogram bins and their positions. All of output 00703 arguments are optional. Among several extremas with the same value the ones with the minimum index 00704 (in the lexicographical order) are returned. In case of several maximums or minimums, the earliest 00705 in the lexicographical order (extrema locations) is returned. 00706 00707 @param hist Histogram. 00708 @param min_value Pointer to the minimum value of the histogram. 00709 @param max_value Pointer to the maximum value of the histogram. 00710 @param min_idx Pointer to the array of coordinates for the minimum. 00711 @param max_idx Pointer to the array of coordinates for the maximum. 00712 */ 00713 CVAPI(void) cvGetMinMaxHistValue( const CvHistogram* hist, 00714 float* min_value, float* max_value, 00715 int* min_idx CV_DEFAULT(NULL), 00716 int* max_idx CV_DEFAULT(NULL)); 00717 00718 00719 /** @brief Normalizes the histogram. 00720 00721 The function normalizes the histogram bins by scaling them so that the sum of the bins becomes equal 00722 to factor. 00723 00724 @param hist Pointer to the histogram. 00725 @param factor Normalization factor. 00726 */ 00727 CVAPI(void) cvNormalizeHist( CvHistogram* hist, double factor ); 00728 00729 00730 /** @brief Thresholds the histogram. 00731 00732 The function clears histogram bins that are below the specified threshold. 00733 00734 @param hist Pointer to the histogram. 00735 @param threshold Threshold level. 00736 */ 00737 CVAPI(void) cvThreshHist( CvHistogram* hist, double threshold ); 00738 00739 00740 /** Compares two histogram */ 00741 CVAPI(double) cvCompareHist( const CvHistogram* hist1, 00742 const CvHistogram* hist2, 00743 int method); 00744 00745 /** @brief Copies a histogram. 00746 00747 The function makes a copy of the histogram. If the second histogram pointer \*dst is NULL, a new 00748 histogram of the same size as src is created. Otherwise, both histograms must have equal types and 00749 sizes. Then the function copies the bin values of the source histogram to the destination histogram 00750 and sets the same bin value ranges as in src. 00751 00752 @param src Source histogram. 00753 @param dst Pointer to the destination histogram. 00754 */ 00755 CVAPI(void) cvCopyHist( const CvHistogram* src, CvHistogram** dst ); 00756 00757 00758 /** @brief Calculates bayesian probabilistic histograms 00759 (each or src and dst is an array of _number_ histograms */ 00760 CVAPI(void) cvCalcBayesianProb( CvHistogram** src, int number, 00761 CvHistogram** dst); 00762 00763 /** @brief Calculates array histogram 00764 @see cv::calcHist 00765 */ 00766 CVAPI(void) cvCalcArrHist( CvArr** arr, CvHistogram* hist, 00767 int accumulate CV_DEFAULT(0), 00768 const CvArr* mask CV_DEFAULT(NULL) ); 00769 00770 /** @overload */ 00771 CV_INLINE void cvCalcHist ( IplImage** image, CvHistogram* hist, 00772 int accumulate CV_DEFAULT(0), 00773 const CvArr* mask CV_DEFAULT(NULL) ) 00774 { 00775 cvCalcArrHist( (CvArr**)image, hist, accumulate, mask ); 00776 } 00777 00778 /** @brief Calculates back project 00779 @see cvCalcBackProject, cv::calcBackProject 00780 */ 00781 CVAPI(void) cvCalcArrBackProject( CvArr** image, CvArr* dst, 00782 const CvHistogram* hist ); 00783 00784 #define cvCalcBackProject(image, dst, hist) cvCalcArrBackProject((CvArr**)image, dst, hist) 00785 00786 00787 /** @brief Locates a template within an image by using a histogram comparison. 00788 00789 The function calculates the back projection by comparing histograms of the source image patches with 00790 the given histogram. The function is similar to matchTemplate, but instead of comparing the raster 00791 patch with all its possible positions within the search window, the function CalcBackProjectPatch 00792 compares histograms. See the algorithm diagram below: 00793 00794  00795 00796 @param image Source images (though, you may pass CvMat\*\* as well). 00797 @param dst Destination image. 00798 @param range 00799 @param hist Histogram. 00800 @param method Comparison method passed to cvCompareHist (see the function description). 00801 @param factor Normalization factor for histograms that affects the normalization scale of the 00802 destination image. Pass 1 if not sure. 00803 00804 @see cvCalcBackProjectPatch 00805 */ 00806 CVAPI(void) cvCalcArrBackProjectPatch( CvArr** image, CvArr* dst, CvSize range, 00807 CvHistogram* hist, int method, 00808 double factor ); 00809 00810 #define cvCalcBackProjectPatch( image, dst, range, hist, method, factor ) \ 00811 cvCalcArrBackProjectPatch( (CvArr**)image, dst, range, hist, method, factor ) 00812 00813 00814 /** @brief Divides one histogram by another. 00815 00816 The function calculates the object probability density from two histograms as: 00817 00818 \f[\texttt{disthist} (I)= \forkthree{0}{if \(\texttt{hist1}(I)=0\)}{\texttt{scale}}{if \(\texttt{hist1}(I) \ne 0\) and \(\texttt{hist2}(I) > \texttt{hist1}(I)\)}{\frac{\texttt{hist2}(I) \cdot \texttt{scale}}{\texttt{hist1}(I)}}{if \(\texttt{hist1}(I) \ne 0\) and \(\texttt{hist2}(I) \le \texttt{hist1}(I)\)}\f] 00819 00820 @param hist1 First histogram (the divisor). 00821 @param hist2 Second histogram. 00822 @param dst_hist Destination histogram. 00823 @param scale Scale factor for the destination histogram. 00824 */ 00825 CVAPI(void) cvCalcProbDensity( const CvHistogram* hist1, const CvHistogram* hist2, 00826 CvHistogram* dst_hist, double scale CV_DEFAULT(255) ); 00827 00828 /** @brief equalizes histogram of 8-bit single-channel image 00829 @see cv::equalizeHist 00830 */ 00831 CVAPI(void) cvEqualizeHist( const CvArr* src, CvArr* dst ); 00832 00833 00834 /** @brief Applies distance transform to binary image 00835 @see cv::distanceTransform 00836 */ 00837 CVAPI(void) cvDistTransform( const CvArr* src, CvArr* dst, 00838 int distance_type CV_DEFAULT(CV_DIST_L2), 00839 int mask_size CV_DEFAULT(3), 00840 const float* mask CV_DEFAULT(NULL), 00841 CvArr* labels CV_DEFAULT(NULL), 00842 int labelType CV_DEFAULT(CV_DIST_LABEL_CCOMP)); 00843 00844 00845 /** @brief Applies fixed-level threshold to grayscale image. 00846 00847 This is a basic operation applied before retrieving contours 00848 @see cv::threshold 00849 */ 00850 CVAPI(double) cvThreshold( const CvArr* src, CvArr* dst, 00851 double threshold, double max_value, 00852 int threshold_type ); 00853 00854 /** @brief Applies adaptive threshold to grayscale image. 00855 00856 The two parameters for methods CV_ADAPTIVE_THRESH_MEAN_C and 00857 CV_ADAPTIVE_THRESH_GAUSSIAN_C are: 00858 neighborhood size (3, 5, 7 etc.), 00859 and a constant subtracted from mean (...,-3,-2,-1,0,1,2,3,...) 00860 @see cv::adaptiveThreshold 00861 */ 00862 CVAPI(void) cvAdaptiveThreshold( const CvArr* src, CvArr* dst, double max_value, 00863 int adaptive_method CV_DEFAULT(CV_ADAPTIVE_THRESH_MEAN_C), 00864 int threshold_type CV_DEFAULT(CV_THRESH_BINARY), 00865 int block_size CV_DEFAULT(3), 00866 double param1 CV_DEFAULT(5)); 00867 00868 /** @brief Fills the connected component until the color difference gets large enough 00869 @see cv::floodFill 00870 */ 00871 CVAPI(void) cvFloodFill( CvArr* image, CvPoint seed_point, 00872 CvScalar new_val, CvScalar lo_diff CV_DEFAULT(cvScalarAll(0)), 00873 CvScalar up_diff CV_DEFAULT(cvScalarAll(0)), 00874 CvConnectedComp* comp CV_DEFAULT(NULL), 00875 int flags CV_DEFAULT(4), 00876 CvArr* mask CV_DEFAULT(NULL)); 00877 00878 /****************************************************************************************\ 00879 * Feature detection * 00880 \****************************************************************************************/ 00881 00882 /** @brief Runs canny edge detector 00883 @see cv::Canny 00884 */ 00885 CVAPI(void) cvCanny( const CvArr* image, CvArr* edges, double threshold1, 00886 double threshold2, int aperture_size CV_DEFAULT(3) ); 00887 00888 /** @brief Calculates constraint image for corner detection 00889 00890 Dx^2 * Dyy + Dxx * Dy^2 - 2 * Dx * Dy * Dxy. 00891 Applying threshold to the result gives coordinates of corners 00892 @see cv::preCornerDetect 00893 */ 00894 CVAPI(void) cvPreCornerDetect( const CvArr* image, CvArr* corners, 00895 int aperture_size CV_DEFAULT(3) ); 00896 00897 /** @brief Calculates eigen values and vectors of 2x2 00898 gradient covariation matrix at every image pixel 00899 @see cv::cornerEigenValsAndVecs 00900 */ 00901 CVAPI(void) cvCornerEigenValsAndVecs( const CvArr* image, CvArr* eigenvv, 00902 int block_size, int aperture_size CV_DEFAULT(3) ); 00903 00904 /** @brief Calculates minimal eigenvalue for 2x2 gradient covariation matrix at 00905 every image pixel 00906 @see cv::cornerMinEigenVal 00907 */ 00908 CVAPI(void) cvCornerMinEigenVal( const CvArr* image, CvArr* eigenval, 00909 int block_size, int aperture_size CV_DEFAULT(3) ); 00910 00911 /** @brief Harris corner detector: 00912 00913 Calculates det(M) - k*(trace(M)^2), where M is 2x2 gradient covariation matrix for each pixel 00914 @see cv::cornerHarris 00915 */ 00916 CVAPI(void) cvCornerHarris( const CvArr* image, CvArr* harris_response, 00917 int block_size, int aperture_size CV_DEFAULT(3), 00918 double k CV_DEFAULT(0.04) ); 00919 00920 /** @brief Adjust corner position using some sort of gradient search 00921 @see cv::cornerSubPix 00922 */ 00923 CVAPI(void) cvFindCornerSubPix( const CvArr* image, CvPoint2D32f* corners, 00924 int count, CvSize win, CvSize zero_zone, 00925 CvTermCriteria criteria ); 00926 00927 /** @brief Finds a sparse set of points within the selected region 00928 that seem to be easy to track 00929 @see cv::goodFeaturesToTrack 00930 */ 00931 CVAPI(void) cvGoodFeaturesToTrack( const CvArr* image, CvArr* eig_image, 00932 CvArr* temp_image, CvPoint2D32f* corners, 00933 int* corner_count, double quality_level, 00934 double min_distance, 00935 const CvArr* mask CV_DEFAULT(NULL), 00936 int block_size CV_DEFAULT(3), 00937 int use_harris CV_DEFAULT(0), 00938 double k CV_DEFAULT(0.04) ); 00939 00940 /** @brief Finds lines on binary image using one of several methods. 00941 00942 line_storage is either memory storage or 1 x _max number of lines_ CvMat, its 00943 number of columns is changed by the function. 00944 method is one of CV_HOUGH_*; 00945 rho, theta and threshold are used for each of those methods; 00946 param1 ~ line length, param2 ~ line gap - for probabilistic, 00947 param1 ~ srn, param2 ~ stn - for multi-scale 00948 @see cv::HoughLines 00949 */ 00950 CVAPI(CvSeq*) cvHoughLines2( CvArr* image, void* line_storage, int method, 00951 double rho, double theta, int threshold, 00952 double param1 CV_DEFAULT(0), double param2 CV_DEFAULT(0), 00953 double min_theta CV_DEFAULT(0), double max_theta CV_DEFAULT(CV_PI)); 00954 00955 /** @brief Finds circles in the image 00956 @see cv::HoughCircles 00957 */ 00958 CVAPI(CvSeq*) cvHoughCircles( CvArr* image, void* circle_storage, 00959 int method, double dp, double min_dist, 00960 double param1 CV_DEFAULT(100), 00961 double param2 CV_DEFAULT(100), 00962 int min_radius CV_DEFAULT(0), 00963 int max_radius CV_DEFAULT(0)); 00964 00965 /** @brief Fits a line into set of 2d or 3d points in a robust way (M-estimator technique) 00966 @see cv::fitLine 00967 */ 00968 CVAPI(void) cvFitLine( const CvArr* points, int dist_type, double param, 00969 double reps, double aeps, float* line ); 00970 00971 /****************************************************************************************\ 00972 * Drawing * 00973 \****************************************************************************************/ 00974 00975 /****************************************************************************************\ 00976 * Drawing functions work with images/matrices of arbitrary type. * 00977 * For color images the channel order is BGR[A] * 00978 * Antialiasing is supported only for 8-bit image now. * 00979 * All the functions include parameter color that means rgb value (that may be * 00980 * constructed with CV_RGB macro) for color images and brightness * 00981 * for grayscale images. * 00982 * If a drawn figure is partially or completely outside of the image, it is clipped.* 00983 \****************************************************************************************/ 00984 00985 #define CV_RGB( r, g, b ) cvScalar( (b), (g), (r), 0 ) 00986 #define CV_FILLED -1 00987 00988 #define CV_AA 16 00989 00990 /** @brief Draws 4-connected, 8-connected or antialiased line segment connecting two points 00991 @see cv::line 00992 */ 00993 CVAPI(void) cvLine( CvArr* img, CvPoint pt1, CvPoint pt2, 00994 CvScalar color, int thickness CV_DEFAULT(1), 00995 int line_type CV_DEFAULT(8), int shift CV_DEFAULT(0) ); 00996 00997 /** @brief Draws a rectangle given two opposite corners of the rectangle (pt1 & pt2) 00998 00999 if thickness<0 (e.g. thickness == CV_FILLED), the filled box is drawn 01000 @see cv::rectangle 01001 */ 01002 CVAPI(void) cvRectangle( CvArr* img, CvPoint pt1, CvPoint pt2, 01003 CvScalar color, int thickness CV_DEFAULT(1), 01004 int line_type CV_DEFAULT(8), 01005 int shift CV_DEFAULT(0)); 01006 01007 /** @brief Draws a rectangle specified by a CvRect structure 01008 @see cv::rectangle 01009 */ 01010 CVAPI(void) cvRectangleR( CvArr* img, CvRect r, 01011 CvScalar color, int thickness CV_DEFAULT(1), 01012 int line_type CV_DEFAULT(8), 01013 int shift CV_DEFAULT(0)); 01014 01015 01016 /** @brief Draws a circle with specified center and radius. 01017 01018 Thickness works in the same way as with cvRectangle 01019 @see cv::circle 01020 */ 01021 CVAPI(void) cvCircle( CvArr* img, CvPoint center, int radius, 01022 CvScalar color, int thickness CV_DEFAULT(1), 01023 int line_type CV_DEFAULT(8), int shift CV_DEFAULT(0)); 01024 01025 /** @brief Draws ellipse outline, filled ellipse, elliptic arc or filled elliptic sector 01026 01027 depending on _thickness_, _start_angle_ and _end_angle_ parameters. The resultant figure 01028 is rotated by _angle_. All the angles are in degrees 01029 @see cv::ellipse 01030 */ 01031 CVAPI(void) cvEllipse( CvArr* img, CvPoint center, CvSize axes, 01032 double angle, double start_angle, double end_angle, 01033 CvScalar color, int thickness CV_DEFAULT(1), 01034 int line_type CV_DEFAULT(8), int shift CV_DEFAULT(0)); 01035 01036 CV_INLINE void cvEllipseBox( CvArr* img, CvBox2D box, CvScalar color, 01037 int thickness CV_DEFAULT(1), 01038 int line_type CV_DEFAULT(8), int shift CV_DEFAULT(0) ) 01039 { 01040 CvSize axes; 01041 axes.width = cvRound(box.size.width*0.5); 01042 axes.height = cvRound(box.size.height*0.5); 01043 01044 cvEllipse( img, cvPointFrom32f( box.center ), axes, box.angle, 01045 0, 360, color, thickness, line_type, shift ); 01046 } 01047 01048 /** @brief Fills convex or monotonous polygon. 01049 @see cv::fillConvexPoly 01050 */ 01051 CVAPI(void) cvFillConvexPoly( CvArr* img, const CvPoint* pts, int npts, CvScalar color, 01052 int line_type CV_DEFAULT(8), int shift CV_DEFAULT(0)); 01053 01054 /** @brief Fills an area bounded by one or more arbitrary polygons 01055 @see cv::fillPoly 01056 */ 01057 CVAPI(void) cvFillPoly( CvArr* img, CvPoint** pts, const int* npts, 01058 int contours, CvScalar color, 01059 int line_type CV_DEFAULT(8), int shift CV_DEFAULT(0) ); 01060 01061 /** @brief Draws one or more polygonal curves 01062 @see cv::polylines 01063 */ 01064 CVAPI(void) cvPolyLine( CvArr* img, CvPoint** pts, const int* npts, int contours, 01065 int is_closed, CvScalar color, int thickness CV_DEFAULT(1), 01066 int line_type CV_DEFAULT(8), int shift CV_DEFAULT(0) ); 01067 01068 #define cvDrawRect cvRectangle 01069 #define cvDrawLine cvLine 01070 #define cvDrawCircle cvCircle 01071 #define cvDrawEllipse cvEllipse 01072 #define cvDrawPolyLine cvPolyLine 01073 01074 /** @brief Clips the line segment connecting *pt1 and *pt2 01075 by the rectangular window 01076 01077 (0<=x<img_size.width, 0<=y<img_size.height). 01078 @see cv::clipLine 01079 */ 01080 CVAPI(int) cvClipLine( CvSize img_size, CvPoint* pt1, CvPoint* pt2 ); 01081 01082 /** @brief Initializes line iterator. 01083 01084 Initially, line_iterator->ptr will point to pt1 (or pt2, see left_to_right description) location in 01085 the image. Returns the number of pixels on the line between the ending points. 01086 @see cv::LineIterator 01087 */ 01088 CVAPI(int) cvInitLineIterator( const CvArr* image, CvPoint pt1, CvPoint pt2, 01089 CvLineIterator* line_iterator, 01090 int connectivity CV_DEFAULT(8), 01091 int left_to_right CV_DEFAULT(0)); 01092 01093 #define CV_NEXT_LINE_POINT( line_iterator ) \ 01094 { \ 01095 int _line_iterator_mask = (line_iterator).err < 0 ? -1 : 0; \ 01096 (line_iterator).err += (line_iterator).minus_delta + \ 01097 ((line_iterator).plus_delta & _line_iterator_mask); \ 01098 (line_iterator).ptr += (line_iterator).minus_step + \ 01099 ((line_iterator).plus_step & _line_iterator_mask); \ 01100 } 01101 01102 01103 #define CV_FONT_HERSHEY_SIMPLEX 0 01104 #define CV_FONT_HERSHEY_PLAIN 1 01105 #define CV_FONT_HERSHEY_DUPLEX 2 01106 #define CV_FONT_HERSHEY_COMPLEX 3 01107 #define CV_FONT_HERSHEY_TRIPLEX 4 01108 #define CV_FONT_HERSHEY_COMPLEX_SMALL 5 01109 #define CV_FONT_HERSHEY_SCRIPT_SIMPLEX 6 01110 #define CV_FONT_HERSHEY_SCRIPT_COMPLEX 7 01111 01112 #define CV_FONT_ITALIC 16 01113 01114 #define CV_FONT_VECTOR0 CV_FONT_HERSHEY_SIMPLEX 01115 01116 01117 /** Font structure */ 01118 typedef struct CvFont 01119 { 01120 const char* nameFont; //Qt:nameFont 01121 CvScalar color; //Qt:ColorFont -> cvScalar(blue_component, green_component, red_component[, alpha_component]) 01122 int font_face; //Qt: bool italic /** =CV_FONT_* */ 01123 const int* ascii; //!< font data and metrics 01124 const int* greek; 01125 const int* cyrillic; 01126 float hscale, vscale; 01127 float shear; //!< slope coefficient: 0 - normal, >0 - italic 01128 int thickness; //!< Qt: weight /** letters thickness */ 01129 float dx; //!< horizontal interval between letters 01130 int line_type; //!< Qt: PointSize 01131 } 01132 CvFont; 01133 01134 /** @brief Initializes font structure (OpenCV 1.x API). 01135 01136 The function initializes the font structure that can be passed to text rendering functions. 01137 01138 @param font Pointer to the font structure initialized by the function 01139 @param font_face Font name identifier. See cv::HersheyFonts and corresponding old CV_* identifiers. 01140 @param hscale Horizontal scale. If equal to 1.0f , the characters have the original width 01141 depending on the font type. If equal to 0.5f , the characters are of half the original width. 01142 @param vscale Vertical scale. If equal to 1.0f , the characters have the original height depending 01143 on the font type. If equal to 0.5f , the characters are of half the original height. 01144 @param shear Approximate tangent of the character slope relative to the vertical line. A zero 01145 value means a non-italic font, 1.0f means about a 45 degree slope, etc. 01146 @param thickness Thickness of the text strokes 01147 @param line_type Type of the strokes, see line description 01148 01149 @sa cvPutText 01150 */ 01151 CVAPI(void) cvInitFont( CvFont* font, int font_face, 01152 double hscale, double vscale, 01153 double shear CV_DEFAULT(0), 01154 int thickness CV_DEFAULT(1), 01155 int line_type CV_DEFAULT(8)); 01156 01157 CV_INLINE CvFont cvFont( double scale, int thickness CV_DEFAULT(1) ) 01158 { 01159 CvFont font; 01160 cvInitFont( &font, CV_FONT_HERSHEY_PLAIN, scale, scale, 0, thickness, CV_AA ); 01161 return font; 01162 } 01163 01164 /** @brief Renders text stroke with specified font and color at specified location. 01165 CvFont should be initialized with cvInitFont 01166 @see cvInitFont, cvGetTextSize, cvFont, cv::putText 01167 */ 01168 CVAPI(void) cvPutText( CvArr* img, const char* text, CvPoint org, 01169 const CvFont* font, CvScalar color ); 01170 01171 /** @brief Calculates bounding box of text stroke (useful for alignment) 01172 @see cv::getTextSize 01173 */ 01174 CVAPI(void) cvGetTextSize( const char* text_string, const CvFont* font, 01175 CvSize* text_size, int* baseline ); 01176 01177 /** @brief Unpacks color value 01178 01179 if arrtype is CV_8UC?, _color_ is treated as packed color value, otherwise the first channels 01180 (depending on arrtype) of destination scalar are set to the same value = _color_ 01181 */ 01182 CVAPI(CvScalar ) cvColorToScalar( double packed_color, int arrtype ); 01183 01184 /** @brief Returns the polygon points which make up the given ellipse. 01185 01186 The ellipse is define by the box of size 'axes' rotated 'angle' around the 'center'. A partial 01187 sweep of the ellipse arc can be done by spcifying arc_start and arc_end to be something other than 01188 0 and 360, respectively. The input array 'pts' must be large enough to hold the result. The total 01189 number of points stored into 'pts' is returned by this function. 01190 @see cv::ellipse2Poly 01191 */ 01192 CVAPI(int) cvEllipse2Poly( CvPoint center, CvSize axes, 01193 int angle, int arc_start, int arc_end, CvPoint * pts, int delta ); 01194 01195 /** @brief Draws contour outlines or filled interiors on the image 01196 @see cv::drawContours 01197 */ 01198 CVAPI(void) cvDrawContours( CvArr *img, CvSeq* contour, 01199 CvScalar external_color, CvScalar hole_color, 01200 int max_level, int thickness CV_DEFAULT(1), 01201 int line_type CV_DEFAULT(8), 01202 CvPoint offset CV_DEFAULT(cvPoint(0,0))); 01203 01204 /** @} */ 01205 01206 #ifdef __cplusplus 01207 } 01208 #endif 01209 01210 #endif 01211
Generated on Tue Jul 12 2022 16:42:38 by
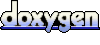