
opencv on mbed
C structures and operations
[Core functionality]
Data Structures | |
struct | CvNArrayIterator |
matrix iterator: used for n-ary operations on dense arrays More... | |
struct | _IplImage |
The IplImage is taken from the Intel Image Processing Library, in which the format is native. More... | |
struct | CvMat |
Matrix elements are stored row by row. More... | |
struct | CvMatND |
struct | CvRect |
struct | CvTermCriteria |
struct | CvBox2D |
struct | CvLineIterator |
Line iterator state: More... | |
struct | CvScalar |
struct | CvAttrList |
List of attributes. More... | |
struct | CvStringHashNode |
All the keys (names) of elements in the readed file storage are stored in the hash to speed up the lookup operations: More... | |
struct | CvFileNode |
Basic element of the file storage - scalar or collection: More... | |
struct | CvTypeInfo |
Type information. More... | |
Modules | |
Connections with C++ | |
Typedefs | |
typedef struct CvNArrayIterator | CvNArrayIterator |
matrix iterator: used for n-ary operations on dense arrays | |
typedef int(CV_CDECL * | CvCmpFunc )(const void *a, const void *b, void *userdata) |
a < b ? -1 : a > b ? 1 : 0 | |
typedef struct CvFileStorage | CvFileStorage |
"black box" representation of the file storage associated with a file on disk. | |
typedef void | CvArr |
This is the "metatype" used *only* as a function parameter. | |
typedef struct ifdef __cplusplus CV_EXPORTS endif _IplImage | IplImage |
The IplImage is taken from the Intel Image Processing Library, in which the format is native. | |
typedef struct CvMat | CvMat |
Matrix elements are stored row by row. | |
typedef struct ifdef __cplusplus CV_EXPORTS endif CvMatND | CvMatND |
typedef struct CvRect | CvRect |
typedef struct CvTermCriteria | CvTermCriteria |
typedef struct CvBox2D | CvBox2D |
typedef struct CvLineIterator | CvLineIterator |
Line iterator state: | |
typedef struct CvScalar | CvScalar |
typedef struct CvFileStorage | CvFileStorage |
"black box" file storage | |
typedef struct CvAttrList | CvAttrList |
List of attributes. | |
typedef struct CvStringHashNode | CvStringHashNode |
All the keys (names) of elements in the readed file storage are stored in the hash to speed up the lookup operations: | |
typedef struct CvFileNode | CvFileNode |
Basic element of the file storage - scalar or collection: | |
typedef struct CvTypeInfo | CvTypeInfo |
Type information. | |
Enumerations | |
enum | { CV_StsOk = 0, CV_StsBackTrace = -1, CV_StsError = -2, CV_StsInternal = -3, CV_StsNoMem = -4, CV_StsBadArg = -5, CV_StsBadFunc = -6, CV_StsNoConv = -7, CV_StsAutoTrace = -8, CV_HeaderIsNull = -9, CV_BadImageSize = -10, CV_BadOffset = -11 , CV_StsNullPtr = -27, CV_StsVecLengthErr = -28, CV_StsFilterStructContentErr = -29, CV_StsKernelStructContentErr = -30, CV_StsFilterOffsetErr = -31, CV_StsBadSize = -201, CV_StsDivByZero = -202, CV_StsInplaceNotSupported = -203, CV_StsObjectNotFound = -204, CV_StsUnmatchedFormats = -205, CV_StsBadFlag = -206, CV_StsBadPoint = -207, CV_StsBadMask = -208, CV_StsUnmatchedSizes = -209, CV_StsUnsupportedFormat = -210, CV_StsOutOfRange = -211, CV_StsParseError = -212, CV_StsNotImplemented = -213, CV_StsBadMemBlock = -214, CV_StsAssert = -215 } |
Functions | |
CV_INLINE void | cvDecRefData (CvArr *arr) |
Decrements an array data reference counter. | |
CV_INLINE int | cvIncRefData (CvArr *arr) |
Increments array data reference counter. | |
CV_INLINE CvMat * | cvGetRow (const CvArr *arr, CvMat *submat, int row) |
This is an overloaded member function, provided for convenience. It differs from the above function only in what argument(s) it accepts. | |
CV_INLINE CvMat * | cvGetCol (const CvArr *arr, CvMat *submat, int col) |
This is an overloaded member function, provided for convenience. It differs from the above function only in what argument(s) it accepts. | |
CV_INLINE void | cvReleaseMatND (CvMatND **mat) |
Deallocates a multi-dimensional array. | |
CVAPI (CvSparseNode *) cvInitSparseMatIterator(const CvSparseMat *mat | |
Initializes sparse array elements iterator. | |
CV_INLINE CvSparseNode * | cvGetNextSparseNode (CvSparseMatIterator *mat_iterator) |
Returns the next sparse matrix element. | |
CVAPI (uchar *) cvPtr1D(const CvArr *arr | |
Return pointer to a particular array element. | |
CVAPI (CvScalar) cvGet1D(const CvArr *arr | |
Return a specific array element. | |
CVAPI (double) cvGetReal1D(const CvArr *arr | |
Return a specific element of single-channel 1D, 2D, 3D or nD array. | |
CVAPI (CvArr *) cvReshapeMatND(const CvArr *arr | |
Changes the shape of a multi-dimensional array without copying the data. | |
CVAPI (CvSize) cvGetSize(const CvArr *arr) | |
Returns size of matrix or image ROI. | |
CVAPI (CvTermCriteria) cvCheckTermCriteria(CvTermCriteria criteria | |
checks termination criteria validity and sets eps to default_eps (if it is not set), max_iter to default_max_iters (if it is not set) | |
CV_INLINE void | cvSubS (const CvArr *src, CvScalar value, CvArr *dst, const CvArr *mask CV_DEFAULT(NULL)) |
dst(mask) = src(mask) - value = src(mask) + (-value) | |
CVAPI (float) cvFastArctan(float y | |
Fast arctangent calculation. | |
CVAPI (CvMemStorage *) cvCreateMemStorage(int block_size CV_DEFAULT(0)) | |
Creates new memory storage. | |
CVAPI (CvString) cvMemStorageAllocString(CvMemStorage *storage | |
Allocates string in memory storage. | |
CVAPI (CvSeq *) cvCreateSeq(int seq_flags | |
Creates new empty sequence that will reside in the specified storage. | |
CVAPI (schar *) cvSeqPush(CvSeq *seq | |
Adds new element to the end of sequence. | |
CVAPI (CvSet *) cvCreateSet(int set_flags | |
Creates a new set. | |
CV_INLINE CvSetElem * | cvSetNew (CvSet *set_header) |
Fast variant of cvSetAdd. | |
CV_INLINE void | cvSetRemoveByPtr (CvSet *set_header, void *elem) |
Removes set element given its pointer. | |
CV_INLINE CvSetElem * | cvGetSetElem (const CvSet *set_header, int idx) |
Returns a set element by index. | |
CVAPI (CvGraph *) cvCreateGraph(int graph_flags | |
Creates new graph. | |
CVAPI (CvGraphEdge *) cvFindGraphEdge(const CvGraph *graph | |
Find edge connecting two vertices. | |
CVAPI (CvGraphScanner *) cvCreateGraphScanner(CvGraph *graph | |
Creates new graph scanner. | |
CVAPI (CvFileStorage *) cvOpenFileStorage(const char *filename | |
Opens file storage for reading or writing data. | |
CVAPI (const char *) cvAttrValue(const CvAttrList *attr | |
returns attribute value or 0 (NULL) if there is no such attribute | |
CVAPI (CvStringHashNode *) cvGetHashedKey(CvFileStorage *fs | |
Returns a unique pointer for a given name. | |
CVAPI (CvFileNode *) cvGetRootFileNode(const CvFileStorage *fs | |
Retrieves one of the top-level nodes of the file storage. | |
CV_INLINE int | cvReadInt (const CvFileNode *node, int default_value CV_DEFAULT(0)) |
Retrieves an integer value from a file node. | |
CV_INLINE int | cvReadIntByName (const CvFileStorage *fs, const CvFileNode *map, const char *name, int default_value CV_DEFAULT(0)) |
Finds a file node and returns its value. | |
CV_INLINE double | cvReadReal (const CvFileNode *node, double default_value CV_DEFAULT(0.)) |
Retrieves a floating-point value from a file node. | |
CV_INLINE double | cvReadRealByName (const CvFileStorage *fs, const CvFileNode *map, const char *name, double default_value CV_DEFAULT(0.)) |
Finds a file node and returns its value. | |
CV_INLINE const char * | cvReadString (const CvFileNode *node, const char *default_value CV_DEFAULT(NULL)) |
Retrieves a text string from a file node. | |
CV_INLINE const char * | cvReadStringByName (const CvFileStorage *fs, const CvFileNode *map, const char *name, const char *default_value CV_DEFAULT(NULL)) |
Finds a file node by its name and returns its value. | |
CV_INLINE void * | cvReadByName (CvFileStorage *fs, const CvFileNode *map, const char *name, CvAttrList *attributes CV_DEFAULT(NULL)) |
Finds an object by name and decodes it. | |
CVAPI (CvTypeInfo *) cvFirstType(void) | |
Returns the beginning of a type list. | |
CVAPI (int64) cvGetTickCount(void) | |
helper functions for RNG initialization and accurate time measurement: uses internal clock counter on x86 | |
CVAPI (CvErrorCallback) cvRedirectError(CvErrorCallback error_handler | |
Assigns a new error-handling function. | |
CV_INLINE CvRNG | cvRNG (int64 seed CV_DEFAULT(-1)) |
Initializes a random number generator state. | |
CV_INLINE unsigned | cvRandInt (CvRNG *rng) |
Returns a 32-bit unsigned integer and updates RNG. | |
CV_INLINE double | cvRandReal (CvRNG *rng) |
Returns a floating-point random number and updates RNG. | |
CV_INLINE CvMat | cvMat (int rows, int cols, int type, void *data CV_DEFAULT(NULL)) |
Inline constructor. | |
CV_INLINE double | cvmGet (const CvMat *mat, int row, int col) |
Returns the particular element of single-channel floating-point matrix. | |
CV_INLINE void | cvmSet (CvMat *mat, int row, int col, double value) |
Sets a specific element of a single-channel floating-point matrix. | |
CV_INLINE CvRect | cvRect (int x, int y, int width, int height) |
constructs CvRect structure. | |
CV_INLINE CvPoint | cvPoint (int x, int y) |
constructs CvPoint structure. | |
CV_INLINE CvPoint2D32f | cvPoint2D32f (double x, double y) |
constructs CvPoint2D32f structure. | |
CV_INLINE CvPoint2D32f | cvPointTo32f (CvPoint point) |
converts CvPoint to CvPoint2D32f. | |
CV_INLINE CvPoint | cvPointFrom32f (CvPoint2D32f point) |
converts CvPoint2D32f to CvPoint. | |
CV_INLINE CvPoint3D32f | cvPoint3D32f (double x, double y, double z) |
constructs CvPoint3D32f structure. | |
CV_INLINE CvPoint2D64f | cvPoint2D64f (double x, double y) |
constructs CvPoint2D64f structure. | |
CV_INLINE CvPoint3D64f | cvPoint3D64f (double x, double y, double z) |
constructs CvPoint3D64f structure. | |
CV_INLINE CvSize | cvSize (int width, int height) |
constructs CvSize structure. | |
CV_INLINE CvSize2D32f | cvSize2D32f (double width, double height) |
constructs CvSize2D32f structure. | |
CV_INLINE CvAttrList | cvAttrList (const char **attr CV_DEFAULT(NULL), CvAttrList *next CV_DEFAULT(NULL)) |
initializes CvAttrList structure | |
CVAPI (int) cvRANSACUpdateNumIters(double p | |
initializes iterator that traverses through several arrays simulteneously (the function together with cvNextArraySlice is used for N-ari element-wise operations) | |
Graph | |
We represent a graph as a set of vertices. Vertices contain their adjacency lists (more exactly, pointers to first incoming or outcoming edge (or 0 if isolated vertex)). Edges are stored in another set. There is a singly-linked list of incoming/outcoming edges for each vertex. Each edge consists of:
A graph may be oriented or not. In the latter case, edges between vertex i to vertex j are not distinguished during search operations.
| |
typedef struct CvGraphEdge | CvGraphEdge |
typedef struct CvGraphVtx | CvGraphVtx |
typedef struct CvGraphVtx2D | CvGraphVtx2D |
typedef struct CvGraph | CvGraph |
Typedef Documentation
typedef void CvArr |
This is the "metatype" used *only* as a function parameter.
It denotes that the function accepts arrays of multiple types, such as IplImage*, CvMat* or even CvSeq* sometimes. The particular array type is determined at runtime by analyzing the first 4 bytes of the header. In C++ interface the role of CvArr is played by InputArray and OutputArray.
Definition at line 114 of file core/types_c.h.
typedef struct CvAttrList CvAttrList |
List of attributes.
:
In the current implementation, attributes are used to pass extra parameters when writing user objects (see cvWrite). XML attributes inside tags are not supported, aside from the object type specification (type_id attribute).
- See also:
- cvAttrList, cvAttrValue
typedef int(CV_CDECL* CvCmpFunc)(const void *a, const void *b, void *userdata) |
typedef struct CvFileNode CvFileNode |
Basic element of the file storage - scalar or collection:
typedef struct CvFileStorage CvFileStorage |
"black box" representation of the file storage associated with a file on disk.
Several functions that are described below take CvFileStorage\* as inputs and allow the user to save or to load hierarchical collections that consist of scalar values, standard CXCore objects (such as matrices, sequences, graphs), and user-defined objects.
OpenCV can read and write data in XML (<http://www.w3c.org/XML>) or YAML (<http://www.yaml.org>) formats. Below is an example of 3x3 floating-point identity matrix A, stored in XML and YAML files using CXCore functions: XML:
{.xml} <?xml version="1.0"> <opencv_storage> <A type_id="opencv-matrix"> <rows>3</rows> <cols>3</cols> <dt>f</dt> <data>1. 0. 0. 0. 1. 0. 0. 0. 1.</data> </A> </opencv_storage>
YAML:
{.yaml} %YAML:1.0 A: !!opencv-matrix rows: 3 cols: 3 dt: f data: [ 1., 0., 0., 0., 1., 0., 0., 0., 1.]
As it can be seen from the examples, XML uses nested tags to represent hierarchy, while YAML uses indentation for that purpose (similar to the Python programming language).
The same functions can read and write data in both formats; the particular format is determined by the extension of the opened file, ".xml" for XML files and ".yml" or ".yaml" for YAML.
Definition at line 90 of file persistence.hpp.
typedef struct CvFileStorage CvFileStorage |
"black box" file storage
Definition at line 1659 of file core/types_c.h.
typedef struct CvLineIterator CvLineIterator |
Line iterator state:
Matrix elements are stored row by row.
Element (i, j) (i - 0-based row index, j - 0-based column index) of a matrix can be retrieved or modified using CV_MAT_ELEM macro:
uchar pixval = CV_MAT_ELEM(grayimg, uchar, i, j) CV_MAT_ELEM(cameraMatrix, float, 0, 2) = image.width*0.5f;
To access multiple-channel matrices, you can use CV_MAT_ELEM(matrix, type, i, j\*nchannels + channel_idx).
typedef struct CvNArrayIterator CvNArrayIterator |
matrix iterator: used for n-ary operations on dense arrays
typedef struct CvStringHashNode CvStringHashNode |
All the keys (names) of elements in the readed file storage are stored in the hash to speed up the lookup operations:
typedef struct CvTermCriteria CvTermCriteria |
- See also:
- TermCriteria
typedef struct CvTypeInfo CvTypeInfo |
Type information.
The structure contains information about one of the standard or user-defined types. Instances of the type may or may not contain a pointer to the corresponding CvTypeInfo structure. In any case, there is a way to find the type info structure for a given object using the cvTypeOf function. Alternatively, type info can be found by type name using cvFindType, which is used when an object is read from file storage. The user can register a new type with cvRegisterType that adds the type information structure into the beginning of the type list. Thus, it is possible to create specialized types from generic standard types and override the basic methods.
The IplImage is taken from the Intel Image Processing Library, in which the format is native.
OpenCV only supports a subset of possible IplImage formats, as outlined in the parameter list above.
In addition to the above restrictions, OpenCV handles ROIs differently. OpenCV functions require that the image size or ROI size of all source and destination images match exactly. On the other hand, the Intel Image Processing Library processes the area of intersection between the source and destination images (or ROIs), allowing them to vary independently.
Enumeration Type Documentation
anonymous enum |
- See also:
- cv::Error::Code
- Enumerator:
Definition at line 119 of file core/types_c.h.
Function Documentation
CVAPI | ( | int | ) |
initializes iterator that traverses through several arrays simulteneously (the function together with cvNextArraySlice is used for N-ari element-wise operations)
Returns the polygon points which make up the given ellipse.
Initializes line iterator.
Clips the line segment connecting *pt1 and *pt2 by the rectangular window.
Checks whether the contour is convex or not (returns 1 if convex, 0 if not)
Finds minimum enclosing circle for a set of points.
Retrieves outer and optionally inner boundaries of white (non-zero) connected components in the black (zero) background.
Output to MessageBox(WIN32)
Output to console(fprintf(stderr,...))
Output nothing.
Maps IPP error codes to the counterparts from OpenCV.
Retrieves detailed information about the last error occured.
Sets error processing mode, returns previously used mode.
Retrives current error processing mode.
Get current OpenCV error status.
get index of the thread being executed
retrieve/set the number of threads used in OpenMP implementations
Loads optimized functions from IPP, MKL etc.
Get next graph element.
Count number of edges incident to the vertex.
Link two vertices specifed by indices or pointers if they are not connected or return pointer to already existing edge connecting the vertices.
Removes vertex from the graph together with all incident edges.
Adds new vertex to the graph.
Adds new element to the set and returns pointer to it.
Splits sequence into one or more equivalence classes using the specified criteria.
Returns current sequence reader position (currently observed sequence element)
Calculates index of the specified sequence element.
Calculates length of sequence slice (with support of negative indices).
Finds optimal DFT vector size >= size0.
Calculates number of non-zero pixels.
Solves linear system (src1)*(dst) = (src2) (returns 0 if src1 is a singular and CV_LU method is used)
Finds real roots of a cubic equation.
Checks array values for NaNs, Infs or simply for too large numbers (if CV_CHECK_RANGE is set).
Returns array size along the specified dimension.
Return number of array dimensions.
Returns type of array elements.
returns zero value if iteration is finished, non-zero (slice length) otherwise
The function returns type of the array elements. In the case of IplImage the type is converted to CvMat-like representation. For example, if the image has been created as:
The code cvGetElemType(img) will return CV_8UC3.
- Parameters:
-
arr Input array
The function returns the array dimensionality and the array of dimension sizes. In the case of IplImage or CvMat it always returns 2 regardless of number of image/matrix rows. For example, the following code calculates total number of array elements:
int sizes[CV_MAX_DIM]; int i, total = 1; int dims = cvGetDims(arr, size); for(i = 0; i < dims; i++ ) total *= sizes[i];
- Parameters:
-
arr Input array sizes Optional output vector of the array dimension sizes. For 2d arrays the number of rows (height) goes first, number of columns (width) next. arr Input array index Zero-based dimension index (for matrices 0 means number of rows, 1 means number of columns; for images 0 means height, 1 means width)
If CV_CHECK_QUIET is set, no runtime errors is raised (function returns zero value in case of "bad" values). Otherwise cvError is called
Returns -1 if element does not belong to the sequence
Functions return 1 if a new edge was created, 0 otherwise
or switches back to pure C code
- See also:
- cv::findContours, cvStartFindContours, cvFindNextContour, cvSubstituteContour, cvEndFindContours
- cv::minEnclosingCircle
- cv::isContourConvex
(0<=x<img_size.width, 0<=y<img_size.height).
- See also:
- cv::clipLine
Initially, line_iterator->ptr will point to pt1 (or pt2, see left_to_right description) location in the image. Returns the number of pixels on the line between the ending points.
- See also:
- cv::LineIterator
The ellipse is define by the box of size 'axes' rotated 'angle' around the 'center'. A partial sweep of the ellipse arc can be done by spcifying arc_start and arc_end to be something other than 0 and 360, respectively. The input array 'pts' must be large enough to hold the result. The total number of points stored into 'pts' is returned by this function.
- See also:
- cv::ellipse2Poly
CVAPI | ( | CvSeq * | ) |
Creates new empty sequence that will reside in the specified storage.
Finds circles in the image.
Finds lines on binary image using one of several methods.
Initializes sequence header for a matrix (column or row vector) of points.
Finds convexity defects for the contour.
Calculates exact convex hull of 2d point set.
Approximates a single polygonal curve (contour) or a tree of polygonal curves (contours)
Approximates Freeman chain(s) with a polygonal curve.
Releases contour scanner and returns pointer to the first outer contour.
Gathers pointers to all the sequences, accessible from the `first`, to the single sequence.
Extracts sequence slice (with or without copying sequence elements)
Creates sequence header for array.
Closes sequence writer, updates sequence header and returns pointer to the resultant sequence (which may be useful if the sequence was created using cvStartWriteSeq))
After that all the operations on sequences that do not alter the content can be applied to the resultant sequence
- See also:
- cvFindContours
This is a standalone contour approximation routine, not represented in the new interface. When cvFindContours retrieves contours as Freeman chains, it calls the function to get approximated contours, represented as polygons.
- Parameters:
-
src_seq Pointer to the approximated Freeman chain that can refer to other chains. storage Storage location for the resulting polylines. method Approximation method (see the description of the function :ocvFindContours ). parameter Method parameter (not used now). minimal_perimeter Approximates only those contours whose perimeters are not less than minimal_perimeter . Other chains are removed from the resulting structure. recursive Recursion flag. If it is non-zero, the function approximates all chains that can be obtained from chain by using the h_next or v_next links. Otherwise, the single input chain is approximated.
- See also:
- cvStartReadChainPoints, cvReadChainPoint
- cv::approxPolyDP
- cv::convexHull
- cv::convexityDefects
a wrapper for cvMakeSeqHeaderForArray (it does not initialize bounding rectangle!!!)
line_storage is either memory storage or 1 x _max number of lines_ CvMat, its number of columns is changed by the function. method is one of CV_HOUGH_*; rho, theta and threshold are used for each of those methods; param1 ~ line length, param2 ~ line gap - for probabilistic, param1 ~ srn, param2 ~ stn - for multi-scale
- See also:
- cv::HoughLines
- cv::HoughCircles
CVAPI | ( | schar * | ) |
Adds new element to the end of sequence.
Finds element in a [sorted] sequence.
Retrieves pointer to specified sequence element.
Inserts a new element in the middle of sequence.
Adds new element to the beginning of sequence.
Returns pointer to the element
Returns pointer to it
cvSeqInsert(seq,0,elem) == cvSeqPushFront(seq,elem)
Negative indices are supported and mean counting from the end (e.g -1 means the last sequence element)
CVAPI | ( | CvArr * | ) | const |
Changes the shape of a multi-dimensional array without copying the data.
Fills matrix with given range of numbers.
The function is an advanced version of cvReshape that can work with multi-dimensional arrays as well (though it can work with ordinary images and matrices) and change the number of dimensions.
Below are the two samples from the cvReshape description rewritten using cvReshapeMatND:
IplImage* color_img = cvCreateImage(cvSize(320,240), IPL_DEPTH_8U, 3); IplImage gray_img_hdr, *gray_img; gray_img = (IplImage*)cvReshapeMatND(color_img, sizeof(gray_img_hdr), &gray_img_hdr, 1, 0, 0); ... int size[] = { 2, 2, 2 }; CvMatND * mat = cvCreateMatND(3, size, CV_32F); CvMat row_header, *row; row = (CvMat*)cvReshapeMatND(mat, sizeof(row_header), &row_header, 0, 1, 0);
In C, the header file for this function includes a convenient macro cvReshapeND that does away with the sizeof_header parameter. So, the lines containing the call to cvReshapeMatND in the examples may be replaced as follow:
gray_img = (IplImage*)cvReshapeND(color_img, &gray_img_hdr, 1, 0, 0); ... row = (CvMat*)cvReshapeND(mat, &row_header, 0, 1, 0);
- Parameters:
-
arr Input array sizeof_header Size of output header to distinguish between IplImage, CvMat and CvMatND output headers header Output header to be filled new_cn New number of channels. new_cn = 0 means that the number of channels remains unchanged. new_dims New number of dimensions. new_dims = 0 means that the number of dimensions remains the same. new_sizes Array of new dimension sizes. Only new_dims-1 values are used, because the total number of elements must remain the same. Thus, if new_dims = 1, new_sizes array is not used.
CVAPI | ( | CvSize | ) | const |
Returns size of matrix or image ROI.
The function returns number of rows (CvSize::height) and number of columns (CvSize::width) of the input matrix or image. In the case of image the size of ROI is returned.
- Parameters:
-
arr array header
CVAPI | ( | CvTermCriteria | ) |
checks termination criteria validity and sets eps to default_eps (if it is not set), max_iter to default_max_iters (if it is not set)
CVAPI | ( | CvSet * | ) |
Creates a new set.
CVAPI | ( | CvGraph * | ) |
Creates new graph.
Creates a copy of graph.
CVAPI | ( | CvGraphEdge * | ) | const |
Find edge connecting two vertices.
CVAPI | ( | CvSparseNode * | ) | const |
Initializes sparse array elements iterator.
The function initializes iterator of sparse array elements and returns pointer to the first element, or NULL if the array is empty.
- Parameters:
-
mat Input array mat_iterator Initialized iterator
CVAPI | ( | CvGraphScanner * | ) |
Creates new graph scanner.
CVAPI | ( | float | ) |
Fast arctangent calculation.
Fast cubic root calculation.
CVAPI | ( | CvFileStorage * | ) | const |
Opens file storage for reading or writing data.
The function opens file storage for reading or writing data. In the latter case, a new file is created or an existing file is rewritten. The type of the read or written file is determined by the filename extension: .xml for XML and .yml or .yaml for YAML. The function returns a pointer to the CvFileStorage structure. If the file cannot be opened then the function returns NULL.
- Parameters:
-
filename Name of the file associated with the storage memstorage Memory storage used for temporary data and for : storing dynamic structures, such as CvSeq or CvGraph . If it is NULL, a temporary memory storage is created and used. flags Can be one of the following: > - **CV_STORAGE_READ** the storage is open for reading > - **CV_STORAGE_WRITE** the storage is open for writing encoding
CVAPI | ( | const char * | ) | const |
returns attribute value or 0 (NULL) if there is no such attribute
Retrieves textual description of the error given its code.
Returns the name of a file node.
The function returns the name of a file node or NULL, if the file node does not have a name or if node is NULL.
- Parameters:
-
node File node
CVAPI | ( | CvStringHashNode * | ) |
Returns a unique pointer for a given name.
The function returns a unique pointer for each particular file node name. This pointer can be then passed to the cvGetFileNode function that is faster than cvGetFileNodeByName because it compares text strings by comparing pointers rather than the strings' content.
Consider the following example where an array of points is encoded as a sequence of 2-entry maps:
points:
- { x: 10, y: 10 }
- { x: 20, y: 20 }
- { x: 30, y: 30 }
# ...
Then, it is possible to get hashed "x" and "y" pointers to speed up decoding of the points. :
#include "cxcore.h" int main( int argc, char** argv ) { CvFileStorage* fs = cvOpenFileStorage( "points.yml", 0, CV_STORAGE_READ ); CvStringHashNode* x_key = cvGetHashedNode( fs, "x", -1, 1 ); CvStringHashNode* y_key = cvGetHashedNode( fs, "y", -1, 1 ); CvFileNode* points = cvGetFileNodeByName( fs, 0, "points" ); if( CV_NODE_IS_SEQ(points->tag) ) { CvSeq* seq = points->data.seq; int i, total = seq->total; CvSeqReader reader; cvStartReadSeq( seq, &reader, 0 ); for( i = 0; i < total; i++ ) { CvFileNode* pt = (CvFileNode*)reader.ptr; #if 1 // faster variant CvFileNode* xnode = cvGetFileNode( fs, pt, x_key, 0 ); CvFileNode* ynode = cvGetFileNode( fs, pt, y_key, 0 ); assert( xnode && CV_NODE_IS_INT(xnode->tag) && ynode && CV_NODE_IS_INT(ynode->tag)); int x = xnode->data.i; // or x = cvReadInt( xnode, 0 ); int y = ynode->data.i; // or y = cvReadInt( ynode, 0 ); #elif 1 // slower variant; does not use x_key & y_key CvFileNode* xnode = cvGetFileNodeByName( fs, pt, "x" ); CvFileNode* ynode = cvGetFileNodeByName( fs, pt, "y" ); assert( xnode && CV_NODE_IS_INT(xnode->tag) && ynode && CV_NODE_IS_INT(ynode->tag)); int x = xnode->data.i; // or x = cvReadInt( xnode, 0 ); int y = ynode->data.i; // or y = cvReadInt( ynode, 0 ); #else // the slowest yet the easiest to use variant int x = cvReadIntByName( fs, pt, "x", 0 ); int y = cvReadIntByName( fs, pt, "y", 0 ); #endif CV_NEXT_SEQ_ELEM( seq->elem_size, reader ); printf(" } } cvReleaseFileStorage( &fs ); return 0; }
Please note that whatever method of accessing a map you are using, it is still much slower than using plain sequences; for example, in the above example, it is more efficient to encode the points as pairs of integers in a single numeric sequence.
- Parameters:
-
fs File storage name Literal node name len Length of the name (if it is known apriori), or -1 if it needs to be calculated create_missing Flag that specifies, whether an absent key should be added into the hash table
CVAPI | ( | CvFileNode * | ) | const |
Retrieves one of the top-level nodes of the file storage.
Finds a node in a map or file storage.
The function returns one of the top-level file nodes. The top-level nodes do not have a name, they correspond to the streams that are stored one after another in the file storage. If the index is out of range, the function returns a NULL pointer, so all the top-level nodes can be iterated by subsequent calls to the function with stream_index=0,1,..., until the NULL pointer is returned. This function can be used as a base for recursive traversal of the file storage.
- Parameters:
-
fs File storage stream_index Zero-based index of the stream. See cvStartNextStream . In most cases, there is only one stream in the file; however, there can be several.
The function finds a file node. It is a faster version of cvGetFileNodeByName (see cvGetHashedKey discussion). Also, the function can insert a new node, if it is not in the map yet.
- Parameters:
-
fs File storage map The parent map. If it is NULL, the function searches a top-level node. If both map and key are NULLs, the function returns the root file node - a map that contains top-level nodes. key Unique pointer to the node name, retrieved with cvGetHashedKey create_missing Flag that specifies whether an absent node should be added to the map
The function finds a file node by name. The node is searched either in map or, if the pointer is NULL, among the top-level file storage nodes. Using this function for maps and cvGetSeqElem (or sequence reader) for sequences, it is possible to navigate through the file storage. To speed up multiple queries for a certain key (e.g., in the case of an array of structures) one may use a combination of cvGetHashedKey and cvGetFileNode.
- Parameters:
-
fs File storage map The parent map. If it is NULL, the function searches in all the top-level nodes (streams), starting with the first one. name The file node name
CVAPI | ( | uchar * | ) | const |
Return pointer to a particular array element.
The functions return a pointer to a specific array element. Number of array dimension should match to the number of indices passed to the function except for cvPtr1D function that can be used for sequential access to 1D, 2D or nD dense arrays.
The functions can be used for sparse arrays as well - if the requested node does not exist they create it and set it to zero.
All these as well as other functions accessing array elements ( cvGetND , cvGetRealND , cvSet , cvSetND , cvSetRealND ) raise an error in case if the element index is out of range.
- Parameters:
-
arr Input array idx0 The first zero-based component of the element index type Optional output parameter: type of matrix elements
This is an overloaded member function, provided for convenience. It differs from the above function only in what argument(s) it accepts.
- Parameters:
-
arr Input array idx Array of the element indices type Optional output parameter: type of matrix elements create_node Optional input parameter for sparse matrices. Non-zero value of the parameter means that the requested element is created if it does not exist already. precalc_hashval Optional input parameter for sparse matrices. If the pointer is not NULL, the function does not recalculate the node hash value, but takes it from the specified location. It is useful for speeding up pair-wise operations (TODO: provide an example)
CVAPI | ( | CvScalar | ) | const |
Return a specific array element.
Calculates mean value of array elements.
Finds sum of array elements.
Calculates trace of the matrix (sum of elements on the main diagonal)
The functions return a specific array element. In the case of a sparse array the functions return 0 if the requested node does not exist (no new node is created by the functions).
- Parameters:
-
arr Input array idx0 The first zero-based component of the element index
This is an overloaded member function, provided for convenience. It differs from the above function only in what argument(s) it accepts.
- Parameters:
-
arr Input array idx Array of the element indices
CVAPI | ( | CvTypeInfo * | ) |
Returns the beginning of a type list.
Returns the type of an object.
Finds a type by its name.
The function returns the first type in the list of registered types. Navigation through the list can be done via the prev and next fields of the CvTypeInfo structure.
The function finds a registered type by its name. It returns NULL if there is no type with the specified name.
- Parameters:
-
type_name Type name
The function finds the type of a given object. It iterates through the list of registered types and calls the is_instance function/method for every type info structure with that object until one of them returns non-zero or until the whole list has been traversed. In the latter case, the function returns NULL.
- Parameters:
-
struct_ptr The object pointer
CVAPI | ( | int64 | ) |
helper functions for RNG initialization and accurate time measurement: uses internal clock counter on x86
CVAPI | ( | CvErrorCallback | ) |
Assigns a new error-handling function.
CVAPI | ( | double | ) | const |
Return a specific element of single-channel 1D, 2D, 3D or nD array.
Finds norm, difference norm or relative difference norm for an array (or two arrays)
Calculates Mahalanobis(weighted) distance.
Calculates determinant of input matrix.
Inverts matrix.
Calculates the dot product of two arrays in Euclidean metrics.
Returns a specific element of a single-channel array. If the array has multiple channels, a runtime error is raised. Note that Get?D functions can be used safely for both single-channel and multiple-channel arrays though they are a bit slower.
In the case of a sparse array the functions return 0 if the requested node does not exist (no new node is created by the functions).
- Parameters:
-
arr Input array. Must have a single channel. idx0 The first zero-based component of the element index
This is an overloaded member function, provided for convenience. It differs from the above function only in what argument(s) it accepts.
- Parameters:
-
arr Input array. Must have a single channel. idx Array of the element indices
The function calculates and returns the Euclidean dot product of two arrays.
In the case of multiple channel arrays, the results for all channels are accumulated. In particular, cvDotProduct(a,a) where a is a complex vector, will return . The function can process multi-dimensional arrays, row by row, layer by layer, and so on.
- Parameters:
-
src1 The first source array src2 The second source array
- See also:
- ref core_c_NormFlags "flags"
CVAPI | ( | CvMemStorage * | ) |
Creates new memory storage.
Creates a memory storage that will borrow memory blocks from parent storage.
block_size == 0 means that default, somewhat optimal size, is used (currently, it is 64K)
CVAPI | ( | CvString | ) |
Allocates string in memory storage.
CV_INLINE CvAttrList cvAttrList | ( | const char **attr | CV_DEFAULTNULL, |
CvAttrList *next | CV_DEFAULTNULL | ||
) |
initializes CvAttrList structure
Definition at line 1688 of file core/types_c.h.
CV_INLINE void cvDecRefData | ( | CvArr * | arr ) |
Decrements an array data reference counter.
The function decrements the data reference counter in a CvMat or CvMatND if the reference counter
pointer is not NULL. If the counter reaches zero, the data is deallocated. In the current implementation the reference counter is not NULL only if the data was allocated using the cvCreateData function. The counter will be NULL in other cases such as: external data was assigned to the header using cvSetData, header is part of a larger matrix or image, or the header was converted from an image or n-dimensional matrix header.
- Parameters:
-
arr Pointer to an array header
CV_INLINE CvSparseNode* cvGetNextSparseNode | ( | CvSparseMatIterator * | mat_iterator ) |
Returns the next sparse matrix element.
The function moves iterator to the next sparse matrix element and returns pointer to it. In the current version there is no any particular order of the elements, because they are stored in the hash table. The sample below demonstrates how to iterate through the sparse matrix:
// print all the non-zero sparse matrix elements and compute their sum double sum = 0; int i, dims = cvGetDims(sparsemat); CvSparseMatIterator it; CvSparseNode* node = cvInitSparseMatIterator(sparsemat, &it); for(; node != 0; node = cvGetNextSparseNode(&it)) { int* idx = CV_NODE_IDX(array, node); float val = *(float*)CV_NODE_VAL(array, node); printf("M"); for(i = 0; i < dims; i++ ) printf("[%d]", idx[i]); printf("=%g\n", val); sum += val; } printf("nTotal sum = %g\n", sum);
- Parameters:
-
mat_iterator Sparse array iterator
CV_INLINE CvSetElem* cvGetSetElem | ( | const CvSet * | set_header, |
int | idx | ||
) |
CV_INLINE int cvIncRefData | ( | CvArr * | arr ) |
CV_INLINE CvMat cvMat | ( | int | rows, |
int | cols, | ||
int | type, | ||
void *data | CV_DEFAULTNULL | ||
) |
Inline constructor.
No data is allocated internally!!! (Use together with cvCreateData, or use cvCreateMat instead to get a matrix with allocated data):
Definition at line 509 of file core/types_c.h.
CV_INLINE double cvmGet | ( | const CvMat * | mat, |
int | row, | ||
int | col | ||
) |
Returns the particular element of single-channel floating-point matrix.
The function is a fast replacement for cvGetReal2D in the case of single-channel floating-point matrices. It is faster because it is inline, it does fewer checks for array type and array element type, and it checks for the row and column ranges only in debug mode.
- Parameters:
-
mat Input matrix row The zero-based index of row col The zero-based index of column
Definition at line 557 of file core/types_c.h.
CV_INLINE void cvmSet | ( | CvMat * | mat, |
int | row, | ||
int | col, | ||
double | value | ||
) |
Sets a specific element of a single-channel floating-point matrix.
The function is a fast replacement for cvSetReal2D in the case of single-channel floating-point matrices. It is faster because it is inline, it does fewer checks for array type and array element type, and it checks for the row and column ranges only in debug mode.
- Parameters:
-
mat The matrix row The zero-based index of row col The zero-based index of column value The new value of the matrix element
Definition at line 584 of file core/types_c.h.
CV_INLINE CvPoint cvPoint | ( | int | x, |
int | y | ||
) |
constructs CvPoint structure.
Definition at line 882 of file core/types_c.h.
CV_INLINE CvPoint2D32f cvPoint2D32f | ( | double | x, |
double | y | ||
) |
constructs CvPoint2D32f structure.
Definition at line 909 of file core/types_c.h.
CV_INLINE CvPoint2D64f cvPoint2D64f | ( | double | x, |
double | y | ||
) |
constructs CvPoint2D64f structure.
Definition at line 973 of file core/types_c.h.
CV_INLINE CvPoint3D32f cvPoint3D32f | ( | double | x, |
double | y, | ||
double | z | ||
) |
constructs CvPoint3D32f structure.
Definition at line 953 of file core/types_c.h.
CV_INLINE CvPoint3D64f cvPoint3D64f | ( | double | x, |
double | y, | ||
double | z | ||
) |
constructs CvPoint3D64f structure.
Definition at line 993 of file core/types_c.h.
CV_INLINE CvPoint cvPointFrom32f | ( | CvPoint2D32f | point ) |
converts CvPoint2D32f to CvPoint.
Definition at line 926 of file core/types_c.h.
CV_INLINE CvPoint2D32f cvPointTo32f | ( | CvPoint | point ) |
converts CvPoint to CvPoint2D32f.
Definition at line 920 of file core/types_c.h.
CV_INLINE unsigned cvRandInt | ( | CvRNG * | rng ) |
Returns a 32-bit unsigned integer and updates RNG.
The function returns a uniformly-distributed random 32-bit unsigned integer and updates the RNG state. It is similar to the rand() function from the C runtime library, except that OpenCV functions always generates a 32-bit random number, regardless of the platform.
- Parameters:
-
rng CvRNG state initialized by cvRNG.
Definition at line 228 of file core/types_c.h.
CV_INLINE double cvRandReal | ( | CvRNG * | rng ) |
Returns a floating-point random number and updates RNG.
The function returns a uniformly-distributed random floating-point number between 0 and 1 (1 is not included).
- Parameters:
-
rng RNG state initialized by cvRNG
Definition at line 242 of file core/types_c.h.
CV_INLINE void* cvReadByName | ( | CvFileStorage * | fs, |
const CvFileNode * | map, | ||
const char * | name, | ||
CvAttrList *attributes | CV_DEFAULTNULL | ||
) |
Finds an object by name and decodes it.
The function is a simple superposition of cvGetFileNodeByName and cvRead.
- Parameters:
-
fs File storage map The parent map. If it is NULL, the function searches a top-level node. name The node name attributes Unused parameter
CV_INLINE int cvReadInt | ( | const CvFileNode * | node, |
int default_value | CV_DEFAULT0 | ||
) |
Retrieves an integer value from a file node.
The function returns an integer that is represented by the file node. If the file node is NULL, the default_value is returned (thus, it is convenient to call the function right after cvGetFileNode without checking for a NULL pointer). If the file node has type CV_NODE_INT, then node->data.i is returned. If the file node has type CV_NODE_REAL, then node->data.f is converted to an integer and returned. Otherwise the error is reported.
- Parameters:
-
node File node default_value The value that is returned if node is NULL
CV_INLINE int cvReadIntByName | ( | const CvFileStorage * | fs, |
const CvFileNode * | map, | ||
const char * | name, | ||
int default_value | CV_DEFAULT0 | ||
) |
Finds a file node and returns its value.
The function is a simple superposition of cvGetFileNodeByName and cvReadInt.
- Parameters:
-
fs File storage map The parent map. If it is NULL, the function searches a top-level node. name The node name default_value The value that is returned if the file node is not found
CV_INLINE double cvReadReal | ( | const CvFileNode * | node, |
double default_value | CV_DEFAULT0. | ||
) |
Retrieves a floating-point value from a file node.
The function returns a floating-point value that is represented by the file node. If the file node is NULL, the default_value is returned (thus, it is convenient to call the function right after cvGetFileNode without checking for a NULL pointer). If the file node has type CV_NODE_REAL , then node->data.f is returned. If the file node has type CV_NODE_INT , then node-:math:>data.f is converted to floating-point and returned. Otherwise the result is not determined.
- Parameters:
-
node File node default_value The value that is returned if node is NULL
CV_INLINE double cvReadRealByName | ( | const CvFileStorage * | fs, |
const CvFileNode * | map, | ||
const char * | name, | ||
double default_value | CV_DEFAULT0. | ||
) |
Finds a file node and returns its value.
The function is a simple superposition of cvGetFileNodeByName and cvReadReal .
- Parameters:
-
fs File storage map The parent map. If it is NULL, the function searches a top-level node. name The node name default_value The value that is returned if the file node is not found
CV_INLINE const char* cvReadString | ( | const CvFileNode * | node, |
const char *default_value | CV_DEFAULTNULL | ||
) |
Retrieves a text string from a file node.
The function returns a text string that is represented by the file node. If the file node is NULL, the default_value is returned (thus, it is convenient to call the function right after cvGetFileNode without checking for a NULL pointer). If the file node has type CV_NODE_STR , then node-:math:>data.str.ptr is returned. Otherwise the result is not determined.
- Parameters:
-
node File node default_value The value that is returned if node is NULL
CV_INLINE const char* cvReadStringByName | ( | const CvFileStorage * | fs, |
const CvFileNode * | map, | ||
const char * | name, | ||
const char *default_value | CV_DEFAULTNULL | ||
) |
Finds a file node by its name and returns its value.
The function is a simple superposition of cvGetFileNodeByName and cvReadString .
- Parameters:
-
fs File storage map The parent map. If it is NULL, the function searches a top-level node. name The node name default_value The value that is returned if the file node is not found
CV_INLINE CvRect cvRect | ( | int | x, |
int | y, | ||
int | width, | ||
int | height | ||
) |
constructs CvRect structure.
Definition at line 796 of file core/types_c.h.
CV_INLINE void cvReleaseMatND | ( | CvMatND ** | mat ) |
Deallocates a multi-dimensional array.
The function decrements the array data reference counter and releases the array header. If the reference counter reaches 0, it also deallocates the data. :
if(*mat ) cvDecRefData(*mat); cvFree((void**)mat);
- Parameters:
-
mat Double pointer to the array
CV_INLINE CvRNG cvRNG | ( | int64 seed | CV_DEFAULT-1 ) |
Initializes a random number generator state.
The function initializes a random number generator and returns the state. The pointer to the state can be then passed to the cvRandInt, cvRandReal and cvRandArr functions. In the current implementation a multiply-with-carry generator is used.
- Parameters:
-
seed 64-bit value used to initiate a random sequence
- See also:
- the C++ class RNG replaced CvRNG.
Definition at line 215 of file core/types_c.h.
CV_INLINE CvSetElem* cvSetNew | ( | CvSet * | set_header ) |
CV_INLINE void cvSetRemoveByPtr | ( | CvSet * | set_header, |
void * | elem | ||
) |
CV_INLINE CvSize cvSize | ( | int | width, |
int | height | ||
) |
constructs CvSize structure.
Definition at line 1023 of file core/types_c.h.
CV_INLINE CvSize2D32f cvSize2D32f | ( | double | width, |
double | height | ||
) |
constructs CvSize2D32f structure.
Definition at line 1049 of file core/types_c.h.
Generated on Tue Jul 12 2022 16:42:41 by
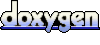