
opencv on mbed
FileStorage Class Reference
[XML/YAML Persistence]
XML/YAML file storage class that encapsulates all the information necessary for writing or reading data to/from a file. More...
#include <persistence.hpp>
Public Types | |
enum | Mode { READ = 0, WRITE = 1, APPEND = 2, MEMORY = 4, FORMAT_MASK = (7<<3), FORMAT_AUTO = 0, FORMAT_XML = (1<<3), FORMAT_YAML = (2<<3) } |
file storage mode More... | |
Public Member Functions | |
CV_WRAP | FileStorage () |
The constructors. | |
CV_WRAP | FileStorage (const String &source, int flags, const String &encoding=String()) |
This is an overloaded member function, provided for convenience. It differs from the above function only in what argument(s) it accepts. | |
FileStorage (CvFileStorage *fs, bool owning=true) | |
virtual | ~FileStorage () |
the destructor. calls release() | |
virtual CV_WRAP bool | open (const String &filename, int flags, const String &encoding=String()) |
Opens a file. | |
virtual CV_WRAP bool | isOpened () const |
Checks whether the file is opened. | |
virtual CV_WRAP void | release () |
Closes the file and releases all the memory buffers. | |
virtual CV_WRAP String | releaseAndGetString () |
Closes the file and releases all the memory buffers. | |
CV_WRAP FileNode | getFirstTopLevelNode () const |
Returns the first element of the top-level mapping. | |
CV_WRAP FileNode | root (int streamidx=0) const |
Returns the top-level mapping. | |
FileNode | operator[] (const String &nodename) const |
Returns the specified element of the top-level mapping. | |
CV_WRAP FileNode | operator[] (const char *nodename) const |
CvFileStorage * | operator* () |
Returns the obsolete C FileStorage structure. | |
const CvFileStorage * | operator* () const |
void | writeRaw (const String &fmt, const uchar *vec, size_t len) |
Writes multiple numbers. | |
void | writeObj (const String &name, const void *obj) |
Writes the registered C structure (CvMat, CvMatND, CvSeq). | |
Static Public Member Functions | |
static String | getDefaultObjectName (const String &filename) |
Returns the normalized object name for the specified name of a file. | |
Data Fields | |
Ptr< CvFileStorage > | fs |
the underlying C FileStorage structure | |
String | elname |
the currently written element | |
std::vector< char > | structs |
the stack of written structures | |
int | state |
the writer state | |
Related Functions | |
(Note that these are not member functions.) | |
CV_EXPORTS FileStorage & | operator<< (FileStorage &fs, const String &str) |
Writes string to a file storage. | |
template<typename _Tp > | |
static FileStorage & | operator<< (FileStorage &fs, const _Tp &value) |
Writes data to a file storage. | |
static FileStorage & | operator<< (FileStorage &fs, const char *str) |
Writes data to a file storage. | |
static FileStorage & | operator<< (FileStorage &fs, char *value) |
Writes data to a file storage. |
Detailed Description
XML/YAML file storage class that encapsulates all the information necessary for writing or reading data to/from a file.
Definition at line 298 of file persistence.hpp.
Member Enumeration Documentation
enum Mode |
file storage mode
- Enumerator:
READ value, open the file for reading
WRITE value, open the file for writing
APPEND value, open the file for appending
MEMORY flag, read data from source or write data to the internal buffer (which is returned by FileStorage::release)
FORMAT_MASK mask for format flags
FORMAT_AUTO flag, auto format
FORMAT_XML flag, XML format
FORMAT_YAML flag, YAML format
Definition at line 302 of file persistence.hpp.
Constructor & Destructor Documentation
CV_WRAP FileStorage | ( | ) |
The constructors.
The full constructor opens the file. Alternatively you can use the default constructor and then call FileStorage::open.
CV_WRAP FileStorage | ( | const String & | source, |
int | flags, | ||
const String & | encoding = String() |
||
) |
This is an overloaded member function, provided for convenience. It differs from the above function only in what argument(s) it accepts.
- Parameters:
-
source Name of the file to open or the text string to read the data from. Extension of the file (.xml or .yml/.yaml) determines its format (XML or YAML respectively). Also you can append .gz to work with compressed files, for example myHugeMatrix.xml.gz. If both FileStorage::WRITE and FileStorage::MEMORY flags are specified, source is used just to specify the output file format (e.g. mydata.xml, .yml etc.). flags Mode of operation. See FileStorage::Mode encoding Encoding of the file. Note that UTF-16 XML encoding is not supported currently and you should use 8-bit encoding instead of it.
FileStorage | ( | CvFileStorage * | fs, |
bool | owning = true |
||
) |
This is an overloaded member function, provided for convenience. It differs from the above function only in what argument(s) it accepts.
virtual ~FileStorage | ( | ) | [virtual] |
the destructor. calls release()
Member Function Documentation
static String getDefaultObjectName | ( | const String & | filename ) | [static] |
Returns the normalized object name for the specified name of a file.
- Parameters:
-
filename Name of a file
- Returns:
- The normalized object name.
CV_WRAP FileNode getFirstTopLevelNode | ( | ) | const |
Returns the first element of the top-level mapping.
- Returns:
- The first element of the top-level mapping.
virtual CV_WRAP bool isOpened | ( | ) | const [virtual] |
Checks whether the file is opened.
- Returns:
- true if the object is associated with the current file and false otherwise. It is a good practice to call this method after you tried to open a file.
virtual CV_WRAP bool open | ( | const String & | filename, |
int | flags, | ||
const String & | encoding = String() |
||
) | [virtual] |
Opens a file.
See description of parameters in FileStorage::FileStorage. The method calls FileStorage::release before opening the file.
- Parameters:
-
filename Name of the file to open or the text string to read the data from. Extension of the file (.xml or .yml/.yaml) determines its format (XML or YAML respectively). Also you can append .gz to work with compressed files, for example myHugeMatrix.xml.gz. If both FileStorage::WRITE and FileStorage::MEMORY flags are specified, source is used just to specify the output file format (e.g. mydata.xml, .yml etc.). flags Mode of operation. One of FileStorage::Mode encoding Encoding of the file. Note that UTF-16 XML encoding is not supported currently and you should use 8-bit encoding instead of it.
CvFileStorage* operator* | ( | ) |
Returns the obsolete C FileStorage structure.
- Returns:
- Pointer to the underlying C FileStorage structure
Definition at line 406 of file persistence.hpp.
const CvFileStorage* operator* | ( | ) | const |
This is an overloaded member function, provided for convenience. It differs from the above function only in what argument(s) it accepts.
Definition at line 409 of file persistence.hpp.
CV_WRAP FileNode operator[] | ( | const char * | nodename ) | const |
This is an overloaded member function, provided for convenience. It differs from the above function only in what argument(s) it accepts.
FileNode operator[] | ( | const String & | nodename ) | const |
Returns the specified element of the top-level mapping.
- Parameters:
-
nodename Name of the file node.
- Returns:
- Node with the given name.
virtual CV_WRAP void release | ( | ) | [virtual] |
Closes the file and releases all the memory buffers.
Call this method after all I/O operations with the storage are finished.
virtual CV_WRAP String releaseAndGetString | ( | ) | [virtual] |
Closes the file and releases all the memory buffers.
Call this method after all I/O operations with the storage are finished. If the storage was opened for writing data and FileStorage::WRITE was specified
CV_WRAP FileNode root | ( | int | streamidx = 0 ) |
const |
Returns the top-level mapping.
- Parameters:
-
streamidx Zero-based index of the stream. In most cases there is only one stream in the file. However, YAML supports multiple streams and so there can be several.
- Returns:
- The top-level mapping.
void writeObj | ( | const String & | name, |
const void * | obj | ||
) |
void writeRaw | ( | const String & | fmt, |
const uchar * | vec, | ||
size_t | len | ||
) |
Writes multiple numbers.
Writes one or more numbers of the specified format to the currently written structure. Usually it is more convenient to use operator `<<` instead of this method.
- Parameters:
-
fmt Specification of each array element, see format specification vec Pointer to the written array. len Number of the uchar elements to write.
Friends And Related Function Documentation
CV_EXPORTS FileStorage & operator<< | ( | FileStorage & | fs, |
const String & | str | ||
) | [related] |
Writes string to a file storage.
static FileStorage & operator<< | ( | FileStorage & | fs, |
const _Tp & | value | ||
) | [related] |
Writes data to a file storage.
Definition at line 1059 of file persistence.hpp.
static FileStorage & operator<< | ( | FileStorage & | fs, |
const char * | str | ||
) | [related] |
Writes data to a file storage.
Definition at line 1074 of file persistence.hpp.
static FileStorage & operator<< | ( | FileStorage & | fs, |
char * | value | ||
) | [related] |
Writes data to a file storage.
Definition at line 1082 of file persistence.hpp.
Field Documentation
String elname |
the currently written element
Definition at line 435 of file persistence.hpp.
the underlying C FileStorage structure
Definition at line 434 of file persistence.hpp.
int state |
the writer state
Definition at line 437 of file persistence.hpp.
std::vector<char> structs |
the stack of written structures
Definition at line 436 of file persistence.hpp.
Generated on Tue Jul 12 2022 16:42:43 by
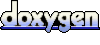