
opencv on mbed
FileNode Class Reference
[XML/YAML Persistence]
File Storage Node class. More...
#include <persistence.hpp>
Public Types | |
enum | Type { NONE = 0, INT = 1, REAL = 2, FLOAT = REAL, STR = 3, STRING = STR, REF = 4, SEQ = 5, MAP = 6 , FLOW = 8, USER = 16, EMPTY = 32, NAMED = 64 } |
type of the file storage node More... | |
Public Member Functions | |
CV_WRAP | FileNode () |
The constructors. | |
FileNode (const CvFileStorage *fs, const CvFileNode *node) | |
This is an overloaded member function, provided for convenience. It differs from the above function only in what argument(s) it accepts. | |
FileNode (const FileNode &node) | |
This is an overloaded member function, provided for convenience. It differs from the above function only in what argument(s) it accepts. | |
FileNode | operator[] (const String &nodename) const |
Returns element of a mapping node or a sequence node. | |
CV_WRAP FileNode | operator[] (const char *nodename) const |
This is an overloaded member function, provided for convenience. It differs from the above function only in what argument(s) it accepts. | |
CV_WRAP FileNode | operator[] (int i) const |
This is an overloaded member function, provided for convenience. It differs from the above function only in what argument(s) it accepts. | |
CV_WRAP int | type () const |
Returns type of the node. | |
CV_WRAP bool | empty () const |
returns true if the node is empty | |
CV_WRAP bool | isNone () const |
returns true if the node is a "none" object | |
CV_WRAP bool | isSeq () const |
returns true if the node is a sequence | |
CV_WRAP bool | isMap () const |
returns true if the node is a mapping | |
CV_WRAP bool | isInt () const |
returns true if the node is an integer | |
CV_WRAP bool | isReal () const |
returns true if the node is a floating-point number | |
CV_WRAP bool | isString () const |
returns true if the node is a text string | |
CV_WRAP bool | isNamed () const |
returns true if the node has a name | |
CV_WRAP String | name () const |
returns the node name or an empty string if the node is nameless | |
CV_WRAP size_t | size () const |
returns the number of elements in the node, if it is a sequence or mapping, or 1 otherwise. | |
operator int () const | |
returns the node content as an integer. If the node stores floating-point number, it is rounded. | |
operator float () const | |
returns the node content as float | |
operator double () const | |
returns the node content as double | |
operator String () const | |
returns the node content as text string | |
CvFileNode * | operator* () |
returns pointer to the underlying file node | |
const CvFileNode * | operator* () const |
returns pointer to the underlying file node | |
FileNodeIterator | begin () const |
returns iterator pointing to the first node element | |
FileNodeIterator | end () const |
returns iterator pointing to the element following the last node element | |
void | readRaw (const String &fmt, uchar *vec, size_t len) const |
Reads node elements to the buffer with the specified format. | |
void * | readObj () const |
reads the registered object and returns pointer to it | |
Related Functions | |
(Note that these are not member functions.) | |
template<typename _Tp > | |
static void | operator>> (const FileNode &n, _Tp &value) |
Reads data from a file storage. | |
template<typename _Tp > | |
static void | operator>> (const FileNode &n, std::vector< _Tp > &vec) |
Reads data from a file storage. |
Detailed Description
File Storage Node class.
The node is used to store each and every element of the file storage opened for reading. When XML/YAML file is read, it is first parsed and stored in the memory as a hierarchical collection of nodes. Each node can be a “leaf” that is contain a single number or a string, or be a collection of other nodes. There can be named collections (mappings) where each element has a name and it is accessed by a name, and ordered collections (sequences) where elements do not have names but rather accessed by index. Type of the file node can be determined using FileNode::type method.
Note that file nodes are only used for navigating file storages opened for reading. When a file storage is opened for writing, no data is stored in memory after it is written.
Definition at line 454 of file persistence.hpp.
Member Enumeration Documentation
enum Type |
type of the file storage node
- Enumerator:
Definition at line 458 of file persistence.hpp.
Constructor & Destructor Documentation
CV_WRAP FileNode | ( | ) |
The constructors.
These constructors are used to create a default file node, construct it from obsolete structures or from the another file node.
FileNode | ( | const CvFileStorage * | fs, |
const CvFileNode * | node | ||
) |
This is an overloaded member function, provided for convenience. It differs from the above function only in what argument(s) it accepts.
- Parameters:
-
fs Pointer to the obsolete file storage structure. node File node to be used as initialization for the created file node.
This is an overloaded member function, provided for convenience. It differs from the above function only in what argument(s) it accepts.
- Parameters:
-
node File node to be used as initialization for the created file node.
Member Function Documentation
FileNodeIterator begin | ( | ) | const |
returns iterator pointing to the first node element
CV_WRAP bool empty | ( | ) | const |
returns true if the node is empty
FileNodeIterator end | ( | ) | const |
returns iterator pointing to the element following the last node element
CV_WRAP bool isInt | ( | ) | const |
returns true if the node is an integer
CV_WRAP bool isMap | ( | ) | const |
returns true if the node is a mapping
CV_WRAP bool isNamed | ( | ) | const |
returns true if the node has a name
CV_WRAP bool isNone | ( | ) | const |
returns true if the node is a "none" object
CV_WRAP bool isReal | ( | ) | const |
returns true if the node is a floating-point number
CV_WRAP bool isSeq | ( | ) | const |
returns true if the node is a sequence
CV_WRAP bool isString | ( | ) | const |
returns true if the node is a text string
CV_WRAP String name | ( | ) | const |
returns the node name or an empty string if the node is nameless
operator double | ( | ) | const |
returns the node content as double
operator float | ( | ) | const |
returns the node content as float
operator int | ( | ) | const |
returns the node content as an integer. If the node stores floating-point number, it is rounded.
operator String | ( | ) | const |
returns the node content as text string
CvFileNode* operator* | ( | ) |
returns pointer to the underlying file node
const CvFileNode* operator* | ( | ) | const |
returns pointer to the underlying file node
CV_WRAP FileNode operator[] | ( | int | i ) | const |
This is an overloaded member function, provided for convenience. It differs from the above function only in what argument(s) it accepts.
- Parameters:
-
i Index of an element in the sequence node.
CV_WRAP FileNode operator[] | ( | const char * | nodename ) | const |
This is an overloaded member function, provided for convenience. It differs from the above function only in what argument(s) it accepts.
- Parameters:
-
nodename Name of an element in the mapping node.
FileNode operator[] | ( | const String & | nodename ) | const |
Returns element of a mapping node or a sequence node.
- Parameters:
-
nodename Name of an element in the mapping node.
- Returns:
- Returns the element with the given identifier.
void* readObj | ( | ) | const |
reads the registered object and returns pointer to it
void readRaw | ( | const String & | fmt, |
uchar * | vec, | ||
size_t | len | ||
) | const |
Reads node elements to the buffer with the specified format.
Usually it is more convenient to use operator `>>` instead of this method.
- Parameters:
-
fmt Specification of each array element. See format specification vec Pointer to the destination array. len Number of elements to read. If it is greater than number of remaining elements then all of them will be read.
CV_WRAP size_t size | ( | ) | const |
returns the number of elements in the node, if it is a sequence or mapping, or 1 otherwise.
CV_WRAP int type | ( | ) | const |
Returns type of the node.
- Returns:
- Type of the node. See FileNode::Type
Friends And Related Function Documentation
static void operator>> | ( | const FileNode & | n, |
_Tp & | value | ||
) | [related] |
Reads data from a file storage.
Definition at line 1119 of file persistence.hpp.
static void operator>> | ( | const FileNode & | n, |
std::vector< _Tp > & | vec | ||
) | [related] |
Reads data from a file storage.
Definition at line 1127 of file persistence.hpp.
Generated on Tue Jul 12 2022 16:42:43 by
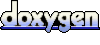