Library for uVGAIII
uVGAIII Class Reference
This is a class for uVGAIII. More...
#include <uVGAIII.h>
Public Member Functions | |
void | cls () |
Clear the entire screen using the current background colour. | |
void | reset () |
Reset screen. | |
void | baudrate (int speed) |
Set serial Baud rate (both sides : screen and mbed) | |
void | set_volume (char value) |
Set internal speaker to specified value. | |
void | screen_mode (char mode) |
Set the graphics orientation. | |
void | background_color (int color) |
Set background color of the screen. | |
void | circle (int x, int y, int radius, int color) |
Draw a circle. | |
void | filled_circle (int x, int y, int radius, int color) |
Draw a filled circle. | |
void | triangle (int x1, int y1, int x2, int y2, int x3, int y3, int color) |
Draw a triangle. | |
void | filled_triangle (int x1, int y1, int x2, int y2, int x3, int y3, int color) |
Draw a filled triangle. | |
void | line (int x1, int y1, int x2, int y2, int color) |
Draw a line. | |
void | rectangle (int x1, int y1, int x2, int y2, int color) |
Draw a rectangle. | |
void | filled_rectangle (int, int, int, int, int) |
Draw a filled rectangle. | |
void | ellipse (int x, int y, int radius_x, int radius_y, int color) |
Draw a ellipse. | |
void | filled_ellipse (int x, int y, int radius_x, int radius_y, int color) |
Draw a filled ellipse. | |
void | button (int state, int x, int y, int buttoncolor, int txtcolor, int font, int txtWidth, int txtHeight, char *text) |
Draw a button. | |
void | panel (int state, int x, int y, int Width, int Height, int color) |
Draw a panel. | |
void | slider (char mode, int x1, int y1, int x2, int y2, int color, int scale, int value) |
Draw a slider. | |
void | put_pixel (int x, int y, int color) |
Draw a pixel. | |
int | read_pixel (int x, int y) |
Read the color value of the pixel. | |
void | screen_copy (int xs, int ys, int xd, int yd, int width, int height) |
Copy an area of a screen. | |
void | clipping (char value) |
Enable or disable the ability for Clipping to be used. | |
void | set_clipping_win (int x1, int y1, int x2, int y2) |
Specifiy the clipping window region on the screen. | |
void | extend_clip_region () |
Force the clip region to the extent of the last text. | |
void | change_color (int oldColor, int newColor) |
Change all old color pixels to new color within the clipping window area. | |
void | move_origin (int xpos, int ypos) |
Move the origin to a new position. | |
void | line_pattern (int pattern) |
Set the line pattern. | |
void | outline_color (int color) |
Set the outline color for rectangles and circles. | |
void | transparency (char mode) |
Set whether to enable the transparency. | |
void | transparent_color (int color) |
Set the color to be transparent. | |
void | graphics_parameters (int function, int value) |
Set graphics parameters. | |
void | set_font (char mode) |
Set font mode. | |
void | move_cursor (int line, int column) |
Move teh text cursor to a screen postion. | |
void | text_opacity (char mode) |
Set whether or not the 'background' pixels are drawn. | |
void | text_width (int multiplier) |
Set the text width multiplier between 1 and 16. | |
void | text_height (int multiplier) |
Set the text height multiplier between 1 and 16. | |
void | text_x_gap (int pixelcount) |
Set the pixel gap between characters(x-axis) | |
void | text_y_gap (int pixelcount) |
Set the pixel gap between characters(y-axis) | |
void | text_bold (char mode) |
Set the bold attribute for the text. | |
void | text_inverse (char mode) |
Inverse the background and foreground color of the text. | |
void | text_italic (char mode) |
Set the text to italic. | |
void | text_underline (char mode) |
Set the text to underlined. | |
void | text_attributes (int value) |
Control several functions grouped, Text Bold, Text Italic, Text Inverse, Text Underlined. | |
void | put_char (char c) |
Print a single character on the display. | |
void | put_string (char *s) |
Print a string on the display. | |
void | text_fgd_color (int color) |
Set the text foreground color. | |
void | text_bgd_color (int color) |
Set the text background color. | |
void | char_width (char c) |
calculate the width in pixel units for a character | |
void | char_height (char c) |
calculate the height in pixel units for a character | |
void | putc (char c) |
place char at current cursor position used by virtual printf function _putc | |
void | puts (char *s) |
place string at current cursor position | |
void | detect_touch_region (int x1, int y1, int x2, int y2) |
Specify a new touch detect region on the screen. | |
void | touch_get_x (int *x) |
Get X coordinates of the touch. | |
void | touch_get_y (int *y) |
Get Y coordinates of the touch. | |
void | touch_set (char mode) |
Set various touch screen related parameters. | |
int | touch_status (void) |
Get the status of the screen. |
Detailed Description
This is a class for uVGAIII.
Definition at line 208 of file uVGAIII.h.
Member Function Documentation
void background_color | ( | int | color ) |
Set background color of the screen.
- Parameters:
-
color 24 bits in total with the most significant byte representing the red part and the middle byte representing the green part and the least significant byte representing the blue part
Definition at line 65 of file uVGAIII_Graphics.cpp.
void baudrate | ( | int | speed ) |
Set serial Baud rate (both sides : screen and mbed)
- Parameters:
-
Speed Correct BAUD value (see uVGAIII.h)
Definition at line 141 of file uVGAIII_main.cpp.
void button | ( | int | state, |
int | x, | ||
int | y, | ||
int | buttoncolor, | ||
int | txtcolor, | ||
int | font, | ||
int | txtWidth, | ||
int | txtHeight, | ||
char * | text | ||
) |
Draw a button.
- Parameters:
-
state appearance of button, 0 = Button depressed; 1 = Button raised x x-coordinate of the top left corner of the button y y-coordinate of the top left corner of the button buttoncolor color of the button txtcolor color of the text font Specifies the Font ID txtWidth Set the width of the text txtHeight Set the height of the text text Specifies the text string
Definition at line 426 of file uVGAIII_Graphics.cpp.
void change_color | ( | int | oldColor, |
int | newColor | ||
) |
Change all old color pixels to new color within the clipping window area.
- Parameters:
-
oldColor Sample color to be changed within the clipping window newColor New color to change all occurrences of old color within the clipping window
Definition at line 37 of file uVGAIII_Graphics.cpp.
void char_height | ( | char | c ) |
calculate the height in pixel units for a character
- Parameters:
-
c Character for the height calculation
Definition at line 83 of file uVGAIII_Text.cpp.
void char_width | ( | char | c ) |
calculate the width in pixel units for a character
- Parameters:
-
c Character for the width calculation
Definition at line 67 of file uVGAIII_Text.cpp.
void circle | ( | int | x, |
int | y, | ||
int | radius, | ||
int | color | ||
) |
Draw a circle.
- Parameters:
-
x x-coordinate of the center y y-coordinate of the center radius radius of the circle color color of the outline of the circle
Definition at line 123 of file uVGAIII_Graphics.cpp.
void clipping | ( | char | value ) |
Enable or disable the ability for Clipping to be used.
- Parameters:
-
value 0 = Clipping Disabled, 1 = Clipping Enabled
Definition at line 663 of file uVGAIII_Graphics.cpp.
void cls | ( | ) |
Clear the entire screen using the current background colour.
Definition at line 25 of file uVGAIII_Graphics.cpp.
void detect_touch_region | ( | int | x1, |
int | y1, | ||
int | x2, | ||
int | y2 | ||
) |
Specify a new touch detect region on the screen.
- Parameters:
-
x1 x-coordinate of the top left corner of the region y1 y-coordinate of the top left corner of the region x2 x-coordinate of the bottom right corner of the region y2 y-coordinate of the bottom rigth corner of the region
Definition at line 88 of file uVGAIII_Touch.cpp.
void ellipse | ( | int | x, |
int | y, | ||
int | radius_x, | ||
int | radius_y, | ||
int | color | ||
) |
Draw a ellipse.
- Parameters:
-
x x-coordinate of the center y y-coordinate of the center radius_x x-radius of the ellipse redius_y y-radius of the ellipse color color of the outline of the rectangle
Definition at line 360 of file uVGAIII_Graphics.cpp.
void extend_clip_region | ( | ) |
Force the clip region to the extent of the last text.
Definition at line 706 of file uVGAIII_Graphics.cpp.
void filled_circle | ( | int | x, |
int | y, | ||
int | radius, | ||
int | color | ||
) |
Draw a filled circle.
- Parameters:
-
x x-coordinate of the center y y-coordinate of the center radius radius of the circle color color of the circle
Definition at line 153 of file uVGAIII_Graphics.cpp.
void filled_ellipse | ( | int | x, |
int | y, | ||
int | radius_x, | ||
int | radius_y, | ||
int | color | ||
) |
Draw a filled ellipse.
- Parameters:
-
x x-coordinate of the center y y-coordinate of the center radius_x x-radius of the ellipse redius_y y-radius of the ellipse color color of the rectangle
Definition at line 393 of file uVGAIII_Graphics.cpp.
void filled_rectangle | ( | int | x1, |
int | y1, | ||
int | x2, | ||
int | y2, | ||
int | color | ||
) |
Draw a filled rectangle.
- Parameters:
-
x1 x-coordinate of the first vertice y1 y-coordinate of the first vertice x2 x-coordinate of the second vertice y2 y-coordinate of the second vertice color color of the rectangle
Definition at line 327 of file uVGAIII_Graphics.cpp.
void filled_triangle | ( | int | x1, |
int | y1, | ||
int | x2, | ||
int | y2, | ||
int | x3, | ||
int | y3, | ||
int | color | ||
) |
Draw a filled triangle.
- Parameters:
-
x1 x-coordinate of the first vertice y1 y-coordinate of the first vertice x2 x-coordinate of the second vertice y2 y-coordinate of the second vertice x3 x-coordinate of the third vertice y3 y-coordinate of the third vertice color color of the triangle
Definition at line 222 of file uVGAIII_Graphics.cpp.
void graphics_parameters | ( | int | function, |
int | value | ||
) |
Set graphics parameters.
- Parameters:
-
function 18 = Object Color, 32 = Screen Resolution, 33 = Page Display, 34 = Page Read, 35 = Page Write value different values to be applied according to different functions
Definition at line 816 of file uVGAIII_Graphics.cpp.
void line | ( | int | x1, |
int | y1, | ||
int | x2, | ||
int | y2, | ||
int | color | ||
) |
Draw a line.
- Parameters:
-
x1 x-coordinate of the first vertice y1 y-coordinate of the first vertice x2 x-coordinate of the second vertice y2 y-coordinate of the second vertice color color of the line
Definition at line 261 of file uVGAIII_Graphics.cpp.
void line_pattern | ( | int | pattern ) |
Set the line pattern.
- Parameters:
-
pattern Correct value 0 to 65535 = number of bits in the line are turned off to form a pattern
Definition at line 740 of file uVGAIII_Graphics.cpp.
void move_cursor | ( | int | line, |
int | column | ||
) |
Move teh text cursor to a screen postion.
- Parameters:
-
line Line position column Column position
Definition at line 268 of file uVGAIII_Text.cpp.
void move_origin | ( | int | xpos, |
int | ypos | ||
) |
Move the origin to a new position.
- Parameters:
-
xpos x-coordinate of the new origin ypos y-coordinate of the new origin
Definition at line 720 of file uVGAIII_Graphics.cpp.
void outline_color | ( | int | color ) |
Set the outline color for rectangles and circles.
- Parameters:
-
color 24 bits in total of the color value
Definition at line 774 of file uVGAIII_Graphics.cpp.
void panel | ( | int | state, |
int | x, | ||
int | y, | ||
int | Width, | ||
int | Height, | ||
int | color | ||
) |
Draw a panel.
- Parameters:
-
state appearance of panel, 0 = recessed; 1 = raised x x-coordinate of the top left corner of the button y y-coordinate of the top left corner of the button Width Set the width of the panel Height Set the height of the panel color Set the color of the panel
Definition at line 478 of file uVGAIII_Graphics.cpp.
void put_char | ( | char | c ) |
Print a single character on the display.
- Parameters:
-
c Character to be print on the screen
Definition at line 290 of file uVGAIII_Text.cpp.
void put_pixel | ( | int | x, |
int | y, | ||
int | color | ||
) |
Draw a pixel.
- Parameters:
-
x x-coordinate of the pixel y y-coordinate of the pixel color Set the color of pixel
Definition at line 556 of file uVGAIII_Graphics.cpp.
void put_string | ( | char * | s ) |
Print a string on the display.
- Parameters:
-
s A Null terminated string
Definition at line 307 of file uVGAIII_Text.cpp.
void putc | ( | char | c ) |
place char at current cursor position used by virtual printf function _putc
- Parameters:
-
c Character to be printed out
Definition at line 372 of file uVGAIII_Text.cpp.
void puts | ( | char * | s ) |
place string at current cursor position
- Parameters:
-
s String to be printed out
Definition at line 407 of file uVGAIII_Text.cpp.
int read_pixel | ( | int | x, |
int | y | ||
) |
Read the color value of the pixel.
- Parameters:
-
x x-coordinate of the pixel y y-coordinate of the pixel
- Returns:
- the color value of the pixel
Definition at line 583 of file uVGAIII_Graphics.cpp.
void rectangle | ( | int | x1, |
int | y1, | ||
int | x2, | ||
int | y2, | ||
int | color | ||
) |
Draw a rectangle.
- Parameters:
-
x1 x-coordinate of the first vertice y1 y-coordinate of the first vertice x2 x-coordinate of the second vertice y2 y-coordinate of the second vertice color color of the outline of the rectangle
Definition at line 294 of file uVGAIII_Graphics.cpp.
void reset | ( | ) |
Reset screen.
Definition at line 102 of file uVGAIII_main.cpp.
void screen_copy | ( | int | xs, |
int | ys, | ||
int | xd, | ||
int | yd, | ||
int | width, | ||
int | height | ||
) |
Copy an area of a screen.
- Parameters:
-
xs x-coordinate of the top left corner of the area to be copied ys y-coordinate of the top left corner of the area to be copied xd x-coordinate of the top left corner of the area to be pasted yd y-coordinate of the top left corner of the area to be pasted width Set the width of the copied area width Set the height of the copied area
Definition at line 630 of file uVGAIII_Graphics.cpp.
void screen_mode | ( | char | mode ) |
Set the graphics orientation.
- Parameters:
-
mode LANDSCAPE, LANDSCAPE_R, PORTRAIT, PORTRAIT_R
Definition at line 90 of file uVGAIII_Graphics.cpp.
void set_clipping_win | ( | int | x1, |
int | y1, | ||
int | x2, | ||
int | y2 | ||
) |
Specifiy the clipping window region on the screen.
- Parameters:
-
x1 x-coordinate of the top left corner of the clipping window y1 y-coordinate of the top left corner of the clipping window x2 x-coordinate of the bottom right corner of the clipping window y2 y-coordinate of the bottom right corner of the clipping window
Definition at line 680 of file uVGAIII_Graphics.cpp.
void set_font | ( | char | mode ) |
Set font mode.
- Parameters:
-
mode 0 for FONT1 = system font; 1 for FONT2; 2 for FONT3 = Default font
Definition at line 23 of file uVGAIII_Text.cpp.
void set_volume | ( | char | value ) |
Set internal speaker to specified value.
- Parameters:
-
value Correct range is 8 - 127
Definition at line 274 of file uVGAIII_main.cpp.
void slider | ( | char | mode, |
int | x1, | ||
int | y1, | ||
int | x2, | ||
int | y2, | ||
int | color, | ||
int | scale, | ||
int | value | ||
) |
Draw a slider.
- Parameters:
-
mode Mode = 0: Slider indented, mode = 1: Slider raised, mode 2: Slider hidden x1 x-coordinate of the top left position of the slider y1 y-coordinate of the top left position of the slider x2 x-coordinate of the bottom right position of the slider y2 y-coordinate of the bottom right position of the slider color Set the color of slider scale Set the full scale range of the slider for the thumb from 0 to n value If value positive, sets the relative position of the thumb on the slider bar, else set thumb to ABS position of the negative number
Definition at line 514 of file uVGAIII_Graphics.cpp.
void text_attributes | ( | int | value ) |
Control several functions grouped, Text Bold, Text Italic, Text Inverse, Text Underlined.
- Parameters:
-
value DEC 16 for BOLD, DEC 32 for ITALIC, DEC 64 for INVERSE, DEC 128 for UNDERLINED
Definition at line 251 of file uVGAIII_Text.cpp.
void text_bgd_color | ( | int | color ) |
Set the text background color.
- Parameters:
-
color Specify the color to be set
Definition at line 351 of file uVGAIII_Text.cpp.
void text_bold | ( | char | mode ) |
Set the bold attribute for the text.
- Parameters:
-
mode 1 for ON, 0 for OFF
Definition at line 183 of file uVGAIII_Text.cpp.
void text_fgd_color | ( | int | color ) |
Set the text foreground color.
- Parameters:
-
color Specify the color to be set
Definition at line 328 of file uVGAIII_Text.cpp.
void text_height | ( | int | multiplier ) |
Set the text height multiplier between 1 and 16.
- Parameters:
-
multiplier Height multiplier 1 to 16
Definition at line 132 of file uVGAIII_Text.cpp.
void text_inverse | ( | char | mode ) |
Inverse the background and foreground color of the text.
- Parameters:
-
mode 1 for ON, 0 for OFF
Definition at line 200 of file uVGAIII_Text.cpp.
void text_italic | ( | char | mode ) |
Set the text to italic.
- Parameters:
-
mode 1 for ON, 0 for OFF
Definition at line 217 of file uVGAIII_Text.cpp.
void text_opacity | ( | char | mode ) |
Set whether or not the 'background' pixels are drawn.
- Parameters:
-
mode 1 for ON(opaque), 0 for OFF(transparent)
Definition at line 99 of file uVGAIII_Text.cpp.
void text_underline | ( | char | mode ) |
Set the text to underlined.
- Parameters:
-
mode 1 for ON, 0 for OFF
Definition at line 234 of file uVGAIII_Text.cpp.
void text_width | ( | int | multiplier ) |
Set the text width multiplier between 1 and 16.
- Parameters:
-
multiplier Width multiplier 1 to 16
Definition at line 115 of file uVGAIII_Text.cpp.
void text_x_gap | ( | int | pixelcount ) |
Set the pixel gap between characters(x-axis)
- Parameters:
-
pixelcount 0 to 32
Definition at line 166 of file uVGAIII_Text.cpp.
void text_y_gap | ( | int | pixelcount ) |
Set the pixel gap between characters(y-axis)
- Parameters:
-
pixelcount 0 to 32
Definition at line 149 of file uVGAIII_Text.cpp.
void touch_get_x | ( | int * | x ) |
Get X coordinates of the touch.
- Parameters:
-
x X coordinate of the touch
Definition at line 36 of file uVGAIII_Touch.cpp.
void touch_get_y | ( | int * | y ) |
Get Y coordinates of the touch.
- Parameters:
-
y Y coordinate of the touch
Definition at line 53 of file uVGAIII_Touch.cpp.
void touch_set | ( | char | mode ) |
Set various touch screen related parameters.
- Parameters:
-
mode Mode = 0: enable and initialise touch screen hardware; mode = 1: disable the touch screen; mode = 2: reset the current active region to default which is the full screen area
Definition at line 70 of file uVGAIII_Touch.cpp.
int touch_status | ( | void | ) |
Get the status of the screen.
- Returns:
- Status of the screen: 0 = INVALID/NOTOUCH, 1 = PRESS, 2 = RELEASE, 3 = MOVING
Definition at line 23 of file uVGAIII_Touch.cpp.
void transparency | ( | char | mode ) |
Set whether to enable the transparency.
- Parameters:
-
mode 0 = Transparency OFF, 1 = Transparency ON
Definition at line 757 of file uVGAIII_Graphics.cpp.
void transparent_color | ( | int | color ) |
Set the color to be transparent.
- Parameters:
-
color Color to be set to be transparent
Definition at line 795 of file uVGAIII_Graphics.cpp.
void triangle | ( | int | x1, |
int | y1, | ||
int | x2, | ||
int | y2, | ||
int | x3, | ||
int | y3, | ||
int | color | ||
) |
Draw a triangle.
- Parameters:
-
x1 x-coordinate of the first vertice y1 y-coordinate of the first vertice x2 x-coordinate of the second vertice y2 y-coordinate of the second vertice x3 x-coordinate of the third vertice y3 y-coordinate of the third vertice color color of the outline of the triangle
Definition at line 183 of file uVGAIII_Graphics.cpp.
Generated on Tue Jul 12 2022 17:04:14 by
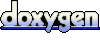